Conditionally swap elements position in a row
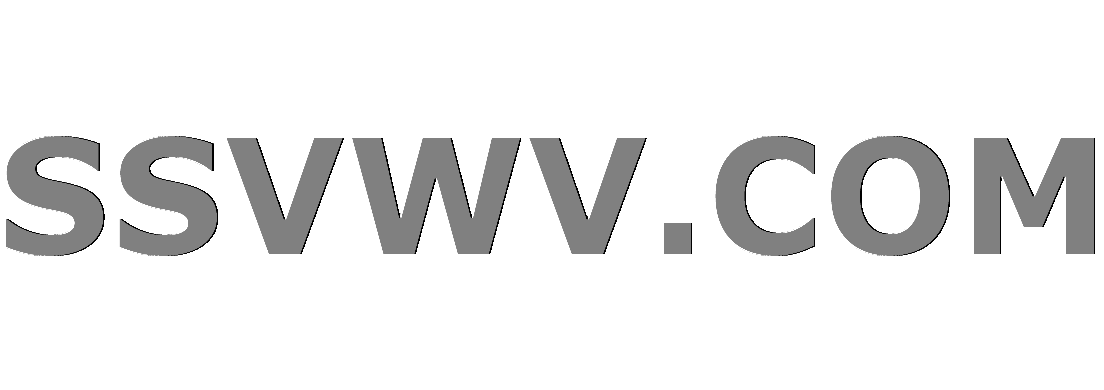
Multi tool use
I have a component that displays a row in a grid-system simil-bootstrap. It is as simple as an image on the left and some body text on the right, both pulled dynamically from a CMS.
I am trying to add a condition that would display the text first and then the image, or vice versa.
ng-container
and ng-template
seems like the way to go, but I can't figure out how.
I am looking for something like:
<ng-container *ngIf="content.switched; then Image and Text; else Text and Image"></ng-container>
<ng-template #Image> ... </ng-template>
<ng-template #Text> ... </ng-template>
Thanks!

add a comment |
I have a component that displays a row in a grid-system simil-bootstrap. It is as simple as an image on the left and some body text on the right, both pulled dynamically from a CMS.
I am trying to add a condition that would display the text first and then the image, or vice versa.
ng-container
and ng-template
seems like the way to go, but I can't figure out how.
I am looking for something like:
<ng-container *ngIf="content.switched; then Image and Text; else Text and Image"></ng-container>
<ng-template #Image> ... </ng-template>
<ng-template #Text> ... </ng-template>
Thanks!

add a comment |
I have a component that displays a row in a grid-system simil-bootstrap. It is as simple as an image on the left and some body text on the right, both pulled dynamically from a CMS.
I am trying to add a condition that would display the text first and then the image, or vice versa.
ng-container
and ng-template
seems like the way to go, but I can't figure out how.
I am looking for something like:
<ng-container *ngIf="content.switched; then Image and Text; else Text and Image"></ng-container>
<ng-template #Image> ... </ng-template>
<ng-template #Text> ... </ng-template>
Thanks!

I have a component that displays a row in a grid-system simil-bootstrap. It is as simple as an image on the left and some body text on the right, both pulled dynamically from a CMS.
I am trying to add a condition that would display the text first and then the image, or vice versa.
ng-container
and ng-template
seems like the way to go, but I can't figure out how.
I am looking for something like:
<ng-container *ngIf="content.switched; then Image and Text; else Text and Image"></ng-container>
<ng-template #Image> ... </ng-template>
<ng-template #Text> ... </ng-template>
Thanks!


asked Nov 25 '18 at 13:43
TommazoTommazo
82
82
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
I guess the best way to do this is by using CSS Flexboxes (row vs row-reverse). If you cannot use Flexbox, or just want to to this using Angular, a suitable approach could be using the directive's or component's ViewContainerRef to add the templates in the desired order:
@Component({
...
})
public class AnyComponent implements OnInit {
@ViewChild('Image')
public imageTemplate: TemplateRef<any;>
@ViewChild('Text') // grabs a reference to the <ng-template #Text>
public textTemplate: TemplateRef<any;>
constructor(private viewContainer: ViewContainerRef) {}
public ngOnInit(): void {
if(condition) {
this.viewContainer.createEmbeddedView(this.textTemplate);
this.viewContainer.createEmbeddedView(this.imageTemplate);
} else {
this.viewContainer.createEmbeddedView(this.imageTemplate);
this.viewContainer.createEmbeddedView(this.textTemplate);
}
}
}
Thanks! I ended up using the css solution but the Angular one was also very helpful!
– Tommazo
Nov 26 '18 at 8:00
add a comment |
It can be done with two ng-container
elements, each one displaying a template with the ngTemplateOutlet directive and selecting the template with a conditional expression:
<ng-container *ngTemplateOutlet="content.switched ? Image : Text"></ng-container>
<ng-container *ngTemplateOutlet="content.switched ? Text : Image"></ng-container>
<ng-template #Image>...</ng-template>
<ng-template #Text>...</ng-template>
See this stackblitz for a demo.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53468083%2fconditionally-swap-elements-position-in-a-row%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I guess the best way to do this is by using CSS Flexboxes (row vs row-reverse). If you cannot use Flexbox, or just want to to this using Angular, a suitable approach could be using the directive's or component's ViewContainerRef to add the templates in the desired order:
@Component({
...
})
public class AnyComponent implements OnInit {
@ViewChild('Image')
public imageTemplate: TemplateRef<any;>
@ViewChild('Text') // grabs a reference to the <ng-template #Text>
public textTemplate: TemplateRef<any;>
constructor(private viewContainer: ViewContainerRef) {}
public ngOnInit(): void {
if(condition) {
this.viewContainer.createEmbeddedView(this.textTemplate);
this.viewContainer.createEmbeddedView(this.imageTemplate);
} else {
this.viewContainer.createEmbeddedView(this.imageTemplate);
this.viewContainer.createEmbeddedView(this.textTemplate);
}
}
}
Thanks! I ended up using the css solution but the Angular one was also very helpful!
– Tommazo
Nov 26 '18 at 8:00
add a comment |
I guess the best way to do this is by using CSS Flexboxes (row vs row-reverse). If you cannot use Flexbox, or just want to to this using Angular, a suitable approach could be using the directive's or component's ViewContainerRef to add the templates in the desired order:
@Component({
...
})
public class AnyComponent implements OnInit {
@ViewChild('Image')
public imageTemplate: TemplateRef<any;>
@ViewChild('Text') // grabs a reference to the <ng-template #Text>
public textTemplate: TemplateRef<any;>
constructor(private viewContainer: ViewContainerRef) {}
public ngOnInit(): void {
if(condition) {
this.viewContainer.createEmbeddedView(this.textTemplate);
this.viewContainer.createEmbeddedView(this.imageTemplate);
} else {
this.viewContainer.createEmbeddedView(this.imageTemplate);
this.viewContainer.createEmbeddedView(this.textTemplate);
}
}
}
Thanks! I ended up using the css solution but the Angular one was also very helpful!
– Tommazo
Nov 26 '18 at 8:00
add a comment |
I guess the best way to do this is by using CSS Flexboxes (row vs row-reverse). If you cannot use Flexbox, or just want to to this using Angular, a suitable approach could be using the directive's or component's ViewContainerRef to add the templates in the desired order:
@Component({
...
})
public class AnyComponent implements OnInit {
@ViewChild('Image')
public imageTemplate: TemplateRef<any;>
@ViewChild('Text') // grabs a reference to the <ng-template #Text>
public textTemplate: TemplateRef<any;>
constructor(private viewContainer: ViewContainerRef) {}
public ngOnInit(): void {
if(condition) {
this.viewContainer.createEmbeddedView(this.textTemplate);
this.viewContainer.createEmbeddedView(this.imageTemplate);
} else {
this.viewContainer.createEmbeddedView(this.imageTemplate);
this.viewContainer.createEmbeddedView(this.textTemplate);
}
}
}
I guess the best way to do this is by using CSS Flexboxes (row vs row-reverse). If you cannot use Flexbox, or just want to to this using Angular, a suitable approach could be using the directive's or component's ViewContainerRef to add the templates in the desired order:
@Component({
...
})
public class AnyComponent implements OnInit {
@ViewChild('Image')
public imageTemplate: TemplateRef<any;>
@ViewChild('Text') // grabs a reference to the <ng-template #Text>
public textTemplate: TemplateRef<any;>
constructor(private viewContainer: ViewContainerRef) {}
public ngOnInit(): void {
if(condition) {
this.viewContainer.createEmbeddedView(this.textTemplate);
this.viewContainer.createEmbeddedView(this.imageTemplate);
} else {
this.viewContainer.createEmbeddedView(this.imageTemplate);
this.viewContainer.createEmbeddedView(this.textTemplate);
}
}
}
answered Nov 25 '18 at 15:51
A.WinnenA.Winnen
9021110
9021110
Thanks! I ended up using the css solution but the Angular one was also very helpful!
– Tommazo
Nov 26 '18 at 8:00
add a comment |
Thanks! I ended up using the css solution but the Angular one was also very helpful!
– Tommazo
Nov 26 '18 at 8:00
Thanks! I ended up using the css solution but the Angular one was also very helpful!
– Tommazo
Nov 26 '18 at 8:00
Thanks! I ended up using the css solution but the Angular one was also very helpful!
– Tommazo
Nov 26 '18 at 8:00
add a comment |
It can be done with two ng-container
elements, each one displaying a template with the ngTemplateOutlet directive and selecting the template with a conditional expression:
<ng-container *ngTemplateOutlet="content.switched ? Image : Text"></ng-container>
<ng-container *ngTemplateOutlet="content.switched ? Text : Image"></ng-container>
<ng-template #Image>...</ng-template>
<ng-template #Text>...</ng-template>
See this stackblitz for a demo.
add a comment |
It can be done with two ng-container
elements, each one displaying a template with the ngTemplateOutlet directive and selecting the template with a conditional expression:
<ng-container *ngTemplateOutlet="content.switched ? Image : Text"></ng-container>
<ng-container *ngTemplateOutlet="content.switched ? Text : Image"></ng-container>
<ng-template #Image>...</ng-template>
<ng-template #Text>...</ng-template>
See this stackblitz for a demo.
add a comment |
It can be done with two ng-container
elements, each one displaying a template with the ngTemplateOutlet directive and selecting the template with a conditional expression:
<ng-container *ngTemplateOutlet="content.switched ? Image : Text"></ng-container>
<ng-container *ngTemplateOutlet="content.switched ? Text : Image"></ng-container>
<ng-template #Image>...</ng-template>
<ng-template #Text>...</ng-template>
See this stackblitz for a demo.
It can be done with two ng-container
elements, each one displaying a template with the ngTemplateOutlet directive and selecting the template with a conditional expression:
<ng-container *ngTemplateOutlet="content.switched ? Image : Text"></ng-container>
<ng-container *ngTemplateOutlet="content.switched ? Text : Image"></ng-container>
<ng-template #Image>...</ng-template>
<ng-template #Text>...</ng-template>
See this stackblitz for a demo.
answered Nov 25 '18 at 18:32


ConnorsFanConnorsFan
31.7k43263
31.7k43263
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53468083%2fconditionally-swap-elements-position-in-a-row%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MuciycmNGaBNB loV,YactRj,e f hKraztk,f5soKtqC1G BWMhD7ouleA0quQXfchUyEAkKHp