Stop Playing a Video from QMediaPlayer at Position X
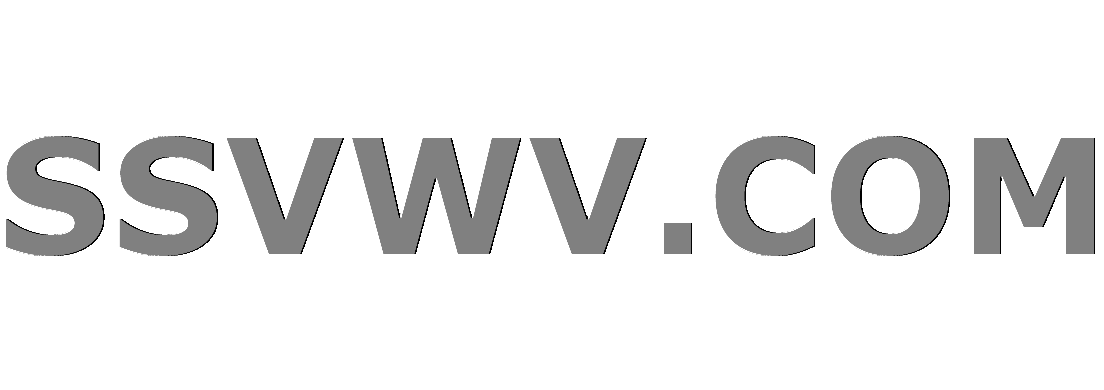
Multi tool use
I am new to Qt and I am using QMediaPlayer in one of my GUI projects and I want to stop the loaded video at a certain position X (input from user on a Line Edit) how would I be able to do this? I know I am able to set a starting position just by doing player->setPosition(Y) where Y is an integer but what about an ending position?
c++ qt
add a comment |
I am new to Qt and I am using QMediaPlayer in one of my GUI projects and I want to stop the loaded video at a certain position X (input from user on a Line Edit) how would I be able to do this? I know I am able to set a starting position just by doing player->setPosition(Y) where Y is an integer but what about an ending position?
c++ qt
add a comment |
I am new to Qt and I am using QMediaPlayer in one of my GUI projects and I want to stop the loaded video at a certain position X (input from user on a Line Edit) how would I be able to do this? I know I am able to set a starting position just by doing player->setPosition(Y) where Y is an integer but what about an ending position?
c++ qt
I am new to Qt and I am using QMediaPlayer in one of my GUI projects and I want to stop the loaded video at a certain position X (input from user on a Line Edit) how would I be able to do this? I know I am able to set a starting position just by doing player->setPosition(Y) where Y is an integer but what about an ending position?
c++ qt
c++ qt
asked Nov 26 '18 at 3:19
patelvrajnpatelvrajn
416
416
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
One lesser option would be to use position()
which returns the current position as a qint64
- if you call the play()
method for your QMediaPlayer
then use something like
while (player.position() < input) {}
player.stop(); // Or player.pause();
it will wait until the input
position is reached. But the drawback to that approach is the blocking while
loop and without knowing the intended application I don't know if that would be appropriate. It is probably better to use the QMediaPlayer::positionChanged
signal (which is emitted based on the QMediaPlayer
's notifyInterval
), something like
connect(player, SIGNAL(positionChanged(qint64)), this, SLOT(checkPosition());
where it is assumed this
is the receiver and both player
and input
are scoped such that they are available to the slot checkPosition()
. checkPosition()
then looks something like
checkPosition() {
if (player.position() > input()) {
player.stop(); // Or player.pause();
}
}
Of course you can also pass the player
and the input
to the checkPosition()
slot but I neglected that for simplicity. Hope this helps.
I was just about to suggest this. using position() was the only thing I could think of also.
– cecil merrel aka bringrainfire
Nov 26 '18 at 4:38
This helped alot, thank you! However, in case it leads to a segment fault which I have to fix now.
– patelvrajn
Nov 26 '18 at 8:23
The segment fault was caused by not disconnecting the positionChanged(qint64) signal from the checkPosition() slot after pausing/stopping the video in the checkPosition() slot function.
– patelvrajn
Nov 27 '18 at 0:11
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474376%2fstop-playing-a-video-from-qmediaplayer-at-position-x%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
One lesser option would be to use position()
which returns the current position as a qint64
- if you call the play()
method for your QMediaPlayer
then use something like
while (player.position() < input) {}
player.stop(); // Or player.pause();
it will wait until the input
position is reached. But the drawback to that approach is the blocking while
loop and without knowing the intended application I don't know if that would be appropriate. It is probably better to use the QMediaPlayer::positionChanged
signal (which is emitted based on the QMediaPlayer
's notifyInterval
), something like
connect(player, SIGNAL(positionChanged(qint64)), this, SLOT(checkPosition());
where it is assumed this
is the receiver and both player
and input
are scoped such that they are available to the slot checkPosition()
. checkPosition()
then looks something like
checkPosition() {
if (player.position() > input()) {
player.stop(); // Or player.pause();
}
}
Of course you can also pass the player
and the input
to the checkPosition()
slot but I neglected that for simplicity. Hope this helps.
I was just about to suggest this. using position() was the only thing I could think of also.
– cecil merrel aka bringrainfire
Nov 26 '18 at 4:38
This helped alot, thank you! However, in case it leads to a segment fault which I have to fix now.
– patelvrajn
Nov 26 '18 at 8:23
The segment fault was caused by not disconnecting the positionChanged(qint64) signal from the checkPosition() slot after pausing/stopping the video in the checkPosition() slot function.
– patelvrajn
Nov 27 '18 at 0:11
add a comment |
One lesser option would be to use position()
which returns the current position as a qint64
- if you call the play()
method for your QMediaPlayer
then use something like
while (player.position() < input) {}
player.stop(); // Or player.pause();
it will wait until the input
position is reached. But the drawback to that approach is the blocking while
loop and without knowing the intended application I don't know if that would be appropriate. It is probably better to use the QMediaPlayer::positionChanged
signal (which is emitted based on the QMediaPlayer
's notifyInterval
), something like
connect(player, SIGNAL(positionChanged(qint64)), this, SLOT(checkPosition());
where it is assumed this
is the receiver and both player
and input
are scoped such that they are available to the slot checkPosition()
. checkPosition()
then looks something like
checkPosition() {
if (player.position() > input()) {
player.stop(); // Or player.pause();
}
}
Of course you can also pass the player
and the input
to the checkPosition()
slot but I neglected that for simplicity. Hope this helps.
I was just about to suggest this. using position() was the only thing I could think of also.
– cecil merrel aka bringrainfire
Nov 26 '18 at 4:38
This helped alot, thank you! However, in case it leads to a segment fault which I have to fix now.
– patelvrajn
Nov 26 '18 at 8:23
The segment fault was caused by not disconnecting the positionChanged(qint64) signal from the checkPosition() slot after pausing/stopping the video in the checkPosition() slot function.
– patelvrajn
Nov 27 '18 at 0:11
add a comment |
One lesser option would be to use position()
which returns the current position as a qint64
- if you call the play()
method for your QMediaPlayer
then use something like
while (player.position() < input) {}
player.stop(); // Or player.pause();
it will wait until the input
position is reached. But the drawback to that approach is the blocking while
loop and without knowing the intended application I don't know if that would be appropriate. It is probably better to use the QMediaPlayer::positionChanged
signal (which is emitted based on the QMediaPlayer
's notifyInterval
), something like
connect(player, SIGNAL(positionChanged(qint64)), this, SLOT(checkPosition());
where it is assumed this
is the receiver and both player
and input
are scoped such that they are available to the slot checkPosition()
. checkPosition()
then looks something like
checkPosition() {
if (player.position() > input()) {
player.stop(); // Or player.pause();
}
}
Of course you can also pass the player
and the input
to the checkPosition()
slot but I neglected that for simplicity. Hope this helps.
One lesser option would be to use position()
which returns the current position as a qint64
- if you call the play()
method for your QMediaPlayer
then use something like
while (player.position() < input) {}
player.stop(); // Or player.pause();
it will wait until the input
position is reached. But the drawback to that approach is the blocking while
loop and without knowing the intended application I don't know if that would be appropriate. It is probably better to use the QMediaPlayer::positionChanged
signal (which is emitted based on the QMediaPlayer
's notifyInterval
), something like
connect(player, SIGNAL(positionChanged(qint64)), this, SLOT(checkPosition());
where it is assumed this
is the receiver and both player
and input
are scoped such that they are available to the slot checkPosition()
. checkPosition()
then looks something like
checkPosition() {
if (player.position() > input()) {
player.stop(); // Or player.pause();
}
}
Of course you can also pass the player
and the input
to the checkPosition()
slot but I neglected that for simplicity. Hope this helps.
answered Nov 26 '18 at 3:59


William MillerWilliam Miller
1,572317
1,572317
I was just about to suggest this. using position() was the only thing I could think of also.
– cecil merrel aka bringrainfire
Nov 26 '18 at 4:38
This helped alot, thank you! However, in case it leads to a segment fault which I have to fix now.
– patelvrajn
Nov 26 '18 at 8:23
The segment fault was caused by not disconnecting the positionChanged(qint64) signal from the checkPosition() slot after pausing/stopping the video in the checkPosition() slot function.
– patelvrajn
Nov 27 '18 at 0:11
add a comment |
I was just about to suggest this. using position() was the only thing I could think of also.
– cecil merrel aka bringrainfire
Nov 26 '18 at 4:38
This helped alot, thank you! However, in case it leads to a segment fault which I have to fix now.
– patelvrajn
Nov 26 '18 at 8:23
The segment fault was caused by not disconnecting the positionChanged(qint64) signal from the checkPosition() slot after pausing/stopping the video in the checkPosition() slot function.
– patelvrajn
Nov 27 '18 at 0:11
I was just about to suggest this. using position() was the only thing I could think of also.
– cecil merrel aka bringrainfire
Nov 26 '18 at 4:38
I was just about to suggest this. using position() was the only thing I could think of also.
– cecil merrel aka bringrainfire
Nov 26 '18 at 4:38
This helped alot, thank you! However, in case it leads to a segment fault which I have to fix now.
– patelvrajn
Nov 26 '18 at 8:23
This helped alot, thank you! However, in case it leads to a segment fault which I have to fix now.
– patelvrajn
Nov 26 '18 at 8:23
The segment fault was caused by not disconnecting the positionChanged(qint64) signal from the checkPosition() slot after pausing/stopping the video in the checkPosition() slot function.
– patelvrajn
Nov 27 '18 at 0:11
The segment fault was caused by not disconnecting the positionChanged(qint64) signal from the checkPosition() slot after pausing/stopping the video in the checkPosition() slot function.
– patelvrajn
Nov 27 '18 at 0:11
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474376%2fstop-playing-a-video-from-qmediaplayer-at-position-x%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6shCLRQytCkq2TJ e4Dz0SbjOK N b,8WBQvBmA6CLT9J0A9vsyc,5UPb7f xN 0VagJaFktn