Best practice for casting an inherited object to the correct child class
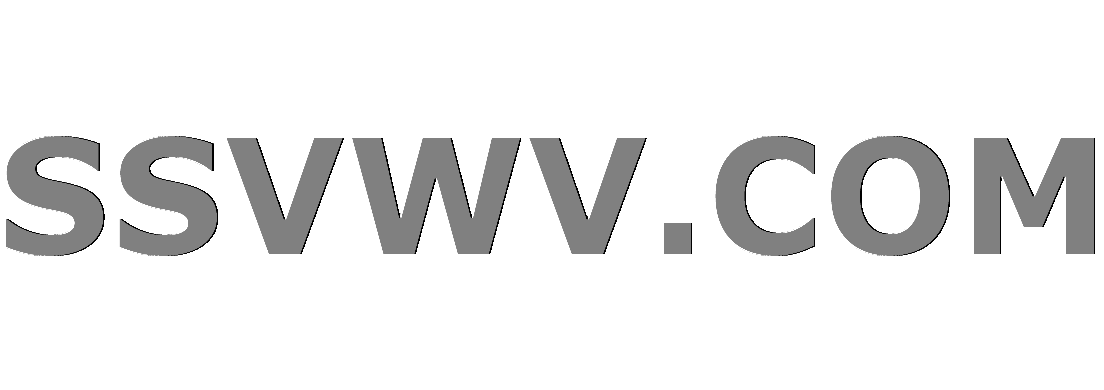
Multi tool use
up vote
-4
down vote
favorite
I have two class, each of them has several children:
class ContainerGeneral {...};
class ContainerTypeA : ContainerGeneral {
public:
void doSomethingA();
};
class ContainerTypeB : ContainerGeneral {
void doSomethingB();
};
class InterpreterGeneral {
protected:
ContainerGeneral* container;
};
class InterpreterTypeA : InterpreterGeneral {
public:
void saveContainer(ContainerTypeA* cont) {
container = cont;
}
};
class InterpreterTypeB : InterpreterGeneral {
public:
void saveContainer(ContainerTypeB* cont) {
container = cont;
}
};
The Interpreter
classes use to a container of a corresponding type (A
to A
, B
to B
, General
to General
). To do this I added a to InterpreterGeneral
a member pointer to a ContainerGeneral
object. I want InterpreterGeneral
to address this object as a ContainerGeneral
, but I want that the inherited classes would be able to address the same container as a container of the appropriate type. I can do it by casting the pointer to the inherited class when addressing it (examples only for A
to save on space):
(ContainerTypeA*)container->doSomethingA();
Or by adding a new member pointer of the inherited type that would point to the same place as container:
class InterpreterTypeA : InterpreterGeneral {
public:
void saveContainer(ContainerTypeA* cont) {
containerA = cont;
container = cont;
}
void doSomething() {
containerA->doSomethingA();
}
private:
ContainerTypeA* containerA;
};
What is the best practice in this case, and is there a way to do this as clean as possible, without casting every time, and without adding new members that don't hold any "new" information?
c++ inheritance
add a comment |
up vote
-4
down vote
favorite
I have two class, each of them has several children:
class ContainerGeneral {...};
class ContainerTypeA : ContainerGeneral {
public:
void doSomethingA();
};
class ContainerTypeB : ContainerGeneral {
void doSomethingB();
};
class InterpreterGeneral {
protected:
ContainerGeneral* container;
};
class InterpreterTypeA : InterpreterGeneral {
public:
void saveContainer(ContainerTypeA* cont) {
container = cont;
}
};
class InterpreterTypeB : InterpreterGeneral {
public:
void saveContainer(ContainerTypeB* cont) {
container = cont;
}
};
The Interpreter
classes use to a container of a corresponding type (A
to A
, B
to B
, General
to General
). To do this I added a to InterpreterGeneral
a member pointer to a ContainerGeneral
object. I want InterpreterGeneral
to address this object as a ContainerGeneral
, but I want that the inherited classes would be able to address the same container as a container of the appropriate type. I can do it by casting the pointer to the inherited class when addressing it (examples only for A
to save on space):
(ContainerTypeA*)container->doSomethingA();
Or by adding a new member pointer of the inherited type that would point to the same place as container:
class InterpreterTypeA : InterpreterGeneral {
public:
void saveContainer(ContainerTypeA* cont) {
containerA = cont;
container = cont;
}
void doSomething() {
containerA->doSomethingA();
}
private:
ContainerTypeA* containerA;
};
What is the best practice in this case, and is there a way to do this as clean as possible, without casting every time, and without adding new members that don't hold any "new" information?
c++ inheritance
3
Yes, make a virtualdoSomething
inContainerGeneral
and don't bother with inheriting fromInterpreterGeneral
.
– freakish
yesterday
add a comment |
up vote
-4
down vote
favorite
up vote
-4
down vote
favorite
I have two class, each of them has several children:
class ContainerGeneral {...};
class ContainerTypeA : ContainerGeneral {
public:
void doSomethingA();
};
class ContainerTypeB : ContainerGeneral {
void doSomethingB();
};
class InterpreterGeneral {
protected:
ContainerGeneral* container;
};
class InterpreterTypeA : InterpreterGeneral {
public:
void saveContainer(ContainerTypeA* cont) {
container = cont;
}
};
class InterpreterTypeB : InterpreterGeneral {
public:
void saveContainer(ContainerTypeB* cont) {
container = cont;
}
};
The Interpreter
classes use to a container of a corresponding type (A
to A
, B
to B
, General
to General
). To do this I added a to InterpreterGeneral
a member pointer to a ContainerGeneral
object. I want InterpreterGeneral
to address this object as a ContainerGeneral
, but I want that the inherited classes would be able to address the same container as a container of the appropriate type. I can do it by casting the pointer to the inherited class when addressing it (examples only for A
to save on space):
(ContainerTypeA*)container->doSomethingA();
Or by adding a new member pointer of the inherited type that would point to the same place as container:
class InterpreterTypeA : InterpreterGeneral {
public:
void saveContainer(ContainerTypeA* cont) {
containerA = cont;
container = cont;
}
void doSomething() {
containerA->doSomethingA();
}
private:
ContainerTypeA* containerA;
};
What is the best practice in this case, and is there a way to do this as clean as possible, without casting every time, and without adding new members that don't hold any "new" information?
c++ inheritance
I have two class, each of them has several children:
class ContainerGeneral {...};
class ContainerTypeA : ContainerGeneral {
public:
void doSomethingA();
};
class ContainerTypeB : ContainerGeneral {
void doSomethingB();
};
class InterpreterGeneral {
protected:
ContainerGeneral* container;
};
class InterpreterTypeA : InterpreterGeneral {
public:
void saveContainer(ContainerTypeA* cont) {
container = cont;
}
};
class InterpreterTypeB : InterpreterGeneral {
public:
void saveContainer(ContainerTypeB* cont) {
container = cont;
}
};
The Interpreter
classes use to a container of a corresponding type (A
to A
, B
to B
, General
to General
). To do this I added a to InterpreterGeneral
a member pointer to a ContainerGeneral
object. I want InterpreterGeneral
to address this object as a ContainerGeneral
, but I want that the inherited classes would be able to address the same container as a container of the appropriate type. I can do it by casting the pointer to the inherited class when addressing it (examples only for A
to save on space):
(ContainerTypeA*)container->doSomethingA();
Or by adding a new member pointer of the inherited type that would point to the same place as container:
class InterpreterTypeA : InterpreterGeneral {
public:
void saveContainer(ContainerTypeA* cont) {
containerA = cont;
container = cont;
}
void doSomething() {
containerA->doSomethingA();
}
private:
ContainerTypeA* containerA;
};
What is the best practice in this case, and is there a way to do this as clean as possible, without casting every time, and without adding new members that don't hold any "new" information?
c++ inheritance
c++ inheritance
edited yesterday
asked yesterday
SIMEL
4,1331967103
4,1331967103
3
Yes, make a virtualdoSomething
inContainerGeneral
and don't bother with inheriting fromInterpreterGeneral
.
– freakish
yesterday
add a comment |
3
Yes, make a virtualdoSomething
inContainerGeneral
and don't bother with inheriting fromInterpreterGeneral
.
– freakish
yesterday
3
3
Yes, make a virtual
doSomething
in ContainerGeneral
and don't bother with inheriting from InterpreterGeneral
.– freakish
yesterday
Yes, make a virtual
doSomething
in ContainerGeneral
and don't bother with inheriting from InterpreterGeneral
.– freakish
yesterday
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
accepted
If you need concrete type information about the container instance within InterpreterTypeA
and InterpreterTypeB
, express this in your code by not storing a GeneralContainer*
data member in GeneralInterpreter
(protected
data member are quite debatable in any case), but instead concrete ContainerTypeA*
and ContainerTypeB*
data members in the subclasses InterpreterTypeA
and InterpreterTypeB
. Storing a base class pointer and then circumventing its limitation by casting hides the fact that concrete type information is required.
Besides, there is not much wrong with either providing empty default implementations of doSomethingA()
and doSomethingB()
in the container base class, or turn them into virtual
pure member functions and shift the empty implementation into ContainerTypeA
and ContainerTypeB
. Then, it's safe to just call them - when it's the undesired concrete type, they won't do anything.
A last, pedantic side note: I don't see any reason to call derived classes in a hierarchy "son" classes. The common term is "child" class.
I accepted this answer because of the second part, creating empty implementations ofdoSomethingA
forB
and the other way around. I need a pointer tocontainer
in the parent class because it uses the common function of that class, so the first part doesn't apply here.
– SIMEL
yesterday
add a comment |
up vote
0
down vote
The problem you are dealing with has a solution in the form of virtual functions. Try this:
class ContainerGeneral {
public:
virtual void doSomething() = 0;
};
class ContainerTypeA : public ContainerGeneral {
public:
void doSomething() {
std::cout << "Hello A!" << std::endl;
};
};
class ContainerTypeB : public ContainerGeneral {
public:
void doSomething() {
std::cout << "Hello B!" << std::endl;
};
};
With that you don't need to bother with inheriting from InterpreterGeneral
at all:
class InterpreterGeneral {
public:
void doSomething()
{
container->doSomething();
}
private:
ContainerGeneral* container;
};
Side note: This of course generates some runtime overhead. You can avoid it if you don't need polymorphism at runtime. Have a look at the static polymorphism. Only if you're a ninja.
The problem with this answer is that it assumes thatdoSomethingA
anddoSomethingB
have something in common, when in fact, they don't have anything in common at all. If I was able to have a function that has a name that makes sense for all children I would use it, but here there is just none. You can assume that the containers are animals and one of them is a bird and the other is a dog,doSomethingA
islayAnEgg
anddoSomethingB
isgoForAWalk
. Creating a common function name would mean that the name is meaningless and creates an unreadable code.
– SIMEL
yesterday
@SIMEL You have to rething your design. You say that they are unrelated and yet it seems that you want to treat them the same in theInterpreterGeneral
class. That doesn't add up.
– freakish
yesterday
they are related, they are all of the same type and have a lot of shared functionality. It's just that the parts that are different are very different, those are binary files that represent the same initial data in different formats. So the "end user" has the same interface and some of the binary data is the same. But some of the data is entirely different, not just the same data written in a different way, but also each format has data that the other don't.
– SIMEL
yesterday
@SIMEL So basically each class is a parser, yes? Sounds like a perfect case for virtual functions that accepts a stream and returns an object of common structure.
– freakish
yesterday
Also have a look at the single responsibility principle. I encourage to use it.
– freakish
yesterday
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
If you need concrete type information about the container instance within InterpreterTypeA
and InterpreterTypeB
, express this in your code by not storing a GeneralContainer*
data member in GeneralInterpreter
(protected
data member are quite debatable in any case), but instead concrete ContainerTypeA*
and ContainerTypeB*
data members in the subclasses InterpreterTypeA
and InterpreterTypeB
. Storing a base class pointer and then circumventing its limitation by casting hides the fact that concrete type information is required.
Besides, there is not much wrong with either providing empty default implementations of doSomethingA()
and doSomethingB()
in the container base class, or turn them into virtual
pure member functions and shift the empty implementation into ContainerTypeA
and ContainerTypeB
. Then, it's safe to just call them - when it's the undesired concrete type, they won't do anything.
A last, pedantic side note: I don't see any reason to call derived classes in a hierarchy "son" classes. The common term is "child" class.
I accepted this answer because of the second part, creating empty implementations ofdoSomethingA
forB
and the other way around. I need a pointer tocontainer
in the parent class because it uses the common function of that class, so the first part doesn't apply here.
– SIMEL
yesterday
add a comment |
up vote
0
down vote
accepted
If you need concrete type information about the container instance within InterpreterTypeA
and InterpreterTypeB
, express this in your code by not storing a GeneralContainer*
data member in GeneralInterpreter
(protected
data member are quite debatable in any case), but instead concrete ContainerTypeA*
and ContainerTypeB*
data members in the subclasses InterpreterTypeA
and InterpreterTypeB
. Storing a base class pointer and then circumventing its limitation by casting hides the fact that concrete type information is required.
Besides, there is not much wrong with either providing empty default implementations of doSomethingA()
and doSomethingB()
in the container base class, or turn them into virtual
pure member functions and shift the empty implementation into ContainerTypeA
and ContainerTypeB
. Then, it's safe to just call them - when it's the undesired concrete type, they won't do anything.
A last, pedantic side note: I don't see any reason to call derived classes in a hierarchy "son" classes. The common term is "child" class.
I accepted this answer because of the second part, creating empty implementations ofdoSomethingA
forB
and the other way around. I need a pointer tocontainer
in the parent class because it uses the common function of that class, so the first part doesn't apply here.
– SIMEL
yesterday
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
If you need concrete type information about the container instance within InterpreterTypeA
and InterpreterTypeB
, express this in your code by not storing a GeneralContainer*
data member in GeneralInterpreter
(protected
data member are quite debatable in any case), but instead concrete ContainerTypeA*
and ContainerTypeB*
data members in the subclasses InterpreterTypeA
and InterpreterTypeB
. Storing a base class pointer and then circumventing its limitation by casting hides the fact that concrete type information is required.
Besides, there is not much wrong with either providing empty default implementations of doSomethingA()
and doSomethingB()
in the container base class, or turn them into virtual
pure member functions and shift the empty implementation into ContainerTypeA
and ContainerTypeB
. Then, it's safe to just call them - when it's the undesired concrete type, they won't do anything.
A last, pedantic side note: I don't see any reason to call derived classes in a hierarchy "son" classes. The common term is "child" class.
If you need concrete type information about the container instance within InterpreterTypeA
and InterpreterTypeB
, express this in your code by not storing a GeneralContainer*
data member in GeneralInterpreter
(protected
data member are quite debatable in any case), but instead concrete ContainerTypeA*
and ContainerTypeB*
data members in the subclasses InterpreterTypeA
and InterpreterTypeB
. Storing a base class pointer and then circumventing its limitation by casting hides the fact that concrete type information is required.
Besides, there is not much wrong with either providing empty default implementations of doSomethingA()
and doSomethingB()
in the container base class, or turn them into virtual
pure member functions and shift the empty implementation into ContainerTypeA
and ContainerTypeB
. Then, it's safe to just call them - when it's the undesired concrete type, they won't do anything.
A last, pedantic side note: I don't see any reason to call derived classes in a hierarchy "son" classes. The common term is "child" class.
answered yesterday


lubgr
9,09321543
9,09321543
I accepted this answer because of the second part, creating empty implementations ofdoSomethingA
forB
and the other way around. I need a pointer tocontainer
in the parent class because it uses the common function of that class, so the first part doesn't apply here.
– SIMEL
yesterday
add a comment |
I accepted this answer because of the second part, creating empty implementations ofdoSomethingA
forB
and the other way around. I need a pointer tocontainer
in the parent class because it uses the common function of that class, so the first part doesn't apply here.
– SIMEL
yesterday
I accepted this answer because of the second part, creating empty implementations of
doSomethingA
for B
and the other way around. I need a pointer to container
in the parent class because it uses the common function of that class, so the first part doesn't apply here.– SIMEL
yesterday
I accepted this answer because of the second part, creating empty implementations of
doSomethingA
for B
and the other way around. I need a pointer to container
in the parent class because it uses the common function of that class, so the first part doesn't apply here.– SIMEL
yesterday
add a comment |
up vote
0
down vote
The problem you are dealing with has a solution in the form of virtual functions. Try this:
class ContainerGeneral {
public:
virtual void doSomething() = 0;
};
class ContainerTypeA : public ContainerGeneral {
public:
void doSomething() {
std::cout << "Hello A!" << std::endl;
};
};
class ContainerTypeB : public ContainerGeneral {
public:
void doSomething() {
std::cout << "Hello B!" << std::endl;
};
};
With that you don't need to bother with inheriting from InterpreterGeneral
at all:
class InterpreterGeneral {
public:
void doSomething()
{
container->doSomething();
}
private:
ContainerGeneral* container;
};
Side note: This of course generates some runtime overhead. You can avoid it if you don't need polymorphism at runtime. Have a look at the static polymorphism. Only if you're a ninja.
The problem with this answer is that it assumes thatdoSomethingA
anddoSomethingB
have something in common, when in fact, they don't have anything in common at all. If I was able to have a function that has a name that makes sense for all children I would use it, but here there is just none. You can assume that the containers are animals and one of them is a bird and the other is a dog,doSomethingA
islayAnEgg
anddoSomethingB
isgoForAWalk
. Creating a common function name would mean that the name is meaningless and creates an unreadable code.
– SIMEL
yesterday
@SIMEL You have to rething your design. You say that they are unrelated and yet it seems that you want to treat them the same in theInterpreterGeneral
class. That doesn't add up.
– freakish
yesterday
they are related, they are all of the same type and have a lot of shared functionality. It's just that the parts that are different are very different, those are binary files that represent the same initial data in different formats. So the "end user" has the same interface and some of the binary data is the same. But some of the data is entirely different, not just the same data written in a different way, but also each format has data that the other don't.
– SIMEL
yesterday
@SIMEL So basically each class is a parser, yes? Sounds like a perfect case for virtual functions that accepts a stream and returns an object of common structure.
– freakish
yesterday
Also have a look at the single responsibility principle. I encourage to use it.
– freakish
yesterday
add a comment |
up vote
0
down vote
The problem you are dealing with has a solution in the form of virtual functions. Try this:
class ContainerGeneral {
public:
virtual void doSomething() = 0;
};
class ContainerTypeA : public ContainerGeneral {
public:
void doSomething() {
std::cout << "Hello A!" << std::endl;
};
};
class ContainerTypeB : public ContainerGeneral {
public:
void doSomething() {
std::cout << "Hello B!" << std::endl;
};
};
With that you don't need to bother with inheriting from InterpreterGeneral
at all:
class InterpreterGeneral {
public:
void doSomething()
{
container->doSomething();
}
private:
ContainerGeneral* container;
};
Side note: This of course generates some runtime overhead. You can avoid it if you don't need polymorphism at runtime. Have a look at the static polymorphism. Only if you're a ninja.
The problem with this answer is that it assumes thatdoSomethingA
anddoSomethingB
have something in common, when in fact, they don't have anything in common at all. If I was able to have a function that has a name that makes sense for all children I would use it, but here there is just none. You can assume that the containers are animals and one of them is a bird and the other is a dog,doSomethingA
islayAnEgg
anddoSomethingB
isgoForAWalk
. Creating a common function name would mean that the name is meaningless and creates an unreadable code.
– SIMEL
yesterday
@SIMEL You have to rething your design. You say that they are unrelated and yet it seems that you want to treat them the same in theInterpreterGeneral
class. That doesn't add up.
– freakish
yesterday
they are related, they are all of the same type and have a lot of shared functionality. It's just that the parts that are different are very different, those are binary files that represent the same initial data in different formats. So the "end user" has the same interface and some of the binary data is the same. But some of the data is entirely different, not just the same data written in a different way, but also each format has data that the other don't.
– SIMEL
yesterday
@SIMEL So basically each class is a parser, yes? Sounds like a perfect case for virtual functions that accepts a stream and returns an object of common structure.
– freakish
yesterday
Also have a look at the single responsibility principle. I encourage to use it.
– freakish
yesterday
add a comment |
up vote
0
down vote
up vote
0
down vote
The problem you are dealing with has a solution in the form of virtual functions. Try this:
class ContainerGeneral {
public:
virtual void doSomething() = 0;
};
class ContainerTypeA : public ContainerGeneral {
public:
void doSomething() {
std::cout << "Hello A!" << std::endl;
};
};
class ContainerTypeB : public ContainerGeneral {
public:
void doSomething() {
std::cout << "Hello B!" << std::endl;
};
};
With that you don't need to bother with inheriting from InterpreterGeneral
at all:
class InterpreterGeneral {
public:
void doSomething()
{
container->doSomething();
}
private:
ContainerGeneral* container;
};
Side note: This of course generates some runtime overhead. You can avoid it if you don't need polymorphism at runtime. Have a look at the static polymorphism. Only if you're a ninja.
The problem you are dealing with has a solution in the form of virtual functions. Try this:
class ContainerGeneral {
public:
virtual void doSomething() = 0;
};
class ContainerTypeA : public ContainerGeneral {
public:
void doSomething() {
std::cout << "Hello A!" << std::endl;
};
};
class ContainerTypeB : public ContainerGeneral {
public:
void doSomething() {
std::cout << "Hello B!" << std::endl;
};
};
With that you don't need to bother with inheriting from InterpreterGeneral
at all:
class InterpreterGeneral {
public:
void doSomething()
{
container->doSomething();
}
private:
ContainerGeneral* container;
};
Side note: This of course generates some runtime overhead. You can avoid it if you don't need polymorphism at runtime. Have a look at the static polymorphism. Only if you're a ninja.
answered yesterday
freakish
38.4k592131
38.4k592131
The problem with this answer is that it assumes thatdoSomethingA
anddoSomethingB
have something in common, when in fact, they don't have anything in common at all. If I was able to have a function that has a name that makes sense for all children I would use it, but here there is just none. You can assume that the containers are animals and one of them is a bird and the other is a dog,doSomethingA
islayAnEgg
anddoSomethingB
isgoForAWalk
. Creating a common function name would mean that the name is meaningless and creates an unreadable code.
– SIMEL
yesterday
@SIMEL You have to rething your design. You say that they are unrelated and yet it seems that you want to treat them the same in theInterpreterGeneral
class. That doesn't add up.
– freakish
yesterday
they are related, they are all of the same type and have a lot of shared functionality. It's just that the parts that are different are very different, those are binary files that represent the same initial data in different formats. So the "end user" has the same interface and some of the binary data is the same. But some of the data is entirely different, not just the same data written in a different way, but also each format has data that the other don't.
– SIMEL
yesterday
@SIMEL So basically each class is a parser, yes? Sounds like a perfect case for virtual functions that accepts a stream and returns an object of common structure.
– freakish
yesterday
Also have a look at the single responsibility principle. I encourage to use it.
– freakish
yesterday
add a comment |
The problem with this answer is that it assumes thatdoSomethingA
anddoSomethingB
have something in common, when in fact, they don't have anything in common at all. If I was able to have a function that has a name that makes sense for all children I would use it, but here there is just none. You can assume that the containers are animals and one of them is a bird and the other is a dog,doSomethingA
islayAnEgg
anddoSomethingB
isgoForAWalk
. Creating a common function name would mean that the name is meaningless and creates an unreadable code.
– SIMEL
yesterday
@SIMEL You have to rething your design. You say that they are unrelated and yet it seems that you want to treat them the same in theInterpreterGeneral
class. That doesn't add up.
– freakish
yesterday
they are related, they are all of the same type and have a lot of shared functionality. It's just that the parts that are different are very different, those are binary files that represent the same initial data in different formats. So the "end user" has the same interface and some of the binary data is the same. But some of the data is entirely different, not just the same data written in a different way, but also each format has data that the other don't.
– SIMEL
yesterday
@SIMEL So basically each class is a parser, yes? Sounds like a perfect case for virtual functions that accepts a stream and returns an object of common structure.
– freakish
yesterday
Also have a look at the single responsibility principle. I encourage to use it.
– freakish
yesterday
The problem with this answer is that it assumes that
doSomethingA
and doSomethingB
have something in common, when in fact, they don't have anything in common at all. If I was able to have a function that has a name that makes sense for all children I would use it, but here there is just none. You can assume that the containers are animals and one of them is a bird and the other is a dog, doSomethingA
is layAnEgg
and doSomethingB
is goForAWalk
. Creating a common function name would mean that the name is meaningless and creates an unreadable code.– SIMEL
yesterday
The problem with this answer is that it assumes that
doSomethingA
and doSomethingB
have something in common, when in fact, they don't have anything in common at all. If I was able to have a function that has a name that makes sense for all children I would use it, but here there is just none. You can assume that the containers are animals and one of them is a bird and the other is a dog, doSomethingA
is layAnEgg
and doSomethingB
is goForAWalk
. Creating a common function name would mean that the name is meaningless and creates an unreadable code.– SIMEL
yesterday
@SIMEL You have to rething your design. You say that they are unrelated and yet it seems that you want to treat them the same in the
InterpreterGeneral
class. That doesn't add up.– freakish
yesterday
@SIMEL You have to rething your design. You say that they are unrelated and yet it seems that you want to treat them the same in the
InterpreterGeneral
class. That doesn't add up.– freakish
yesterday
they are related, they are all of the same type and have a lot of shared functionality. It's just that the parts that are different are very different, those are binary files that represent the same initial data in different formats. So the "end user" has the same interface and some of the binary data is the same. But some of the data is entirely different, not just the same data written in a different way, but also each format has data that the other don't.
– SIMEL
yesterday
they are related, they are all of the same type and have a lot of shared functionality. It's just that the parts that are different are very different, those are binary files that represent the same initial data in different formats. So the "end user" has the same interface and some of the binary data is the same. But some of the data is entirely different, not just the same data written in a different way, but also each format has data that the other don't.
– SIMEL
yesterday
@SIMEL So basically each class is a parser, yes? Sounds like a perfect case for virtual functions that accepts a stream and returns an object of common structure.
– freakish
yesterday
@SIMEL So basically each class is a parser, yes? Sounds like a perfect case for virtual functions that accepts a stream and returns an object of common structure.
– freakish
yesterday
Also have a look at the single responsibility principle. I encourage to use it.
– freakish
yesterday
Also have a look at the single responsibility principle. I encourage to use it.
– freakish
yesterday
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53372234%2fbest-practice-for-casting-an-inherited-object-to-the-correct-child-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Rcf,KXUf7 FURbESWo47Exb,4,MP L,iVajymd7Cb,kI,bJV0h1nIuPueU6NUg8ujtwNF43V13U5WZ 6jLr
3
Yes, make a virtual
doSomething
inContainerGeneral
and don't bother with inheriting fromInterpreterGeneral
.– freakish
yesterday