Simple factory vs Factory method
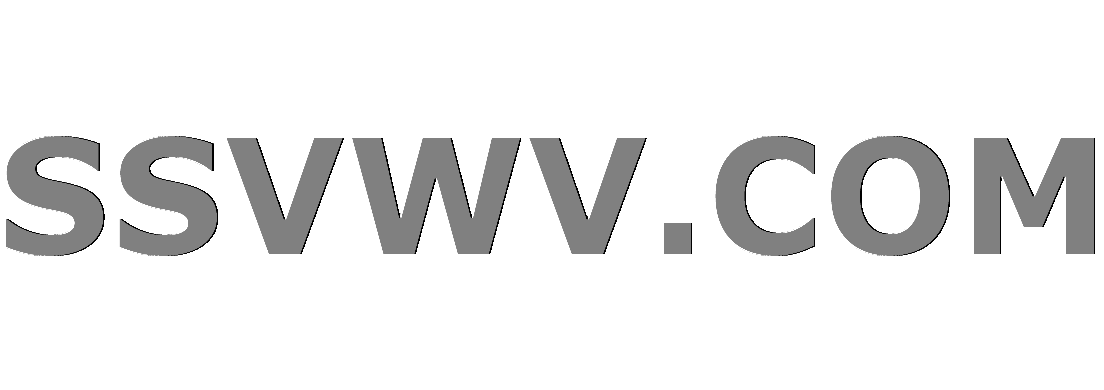
Multi tool use
up vote
1
down vote
favorite
Simple factory:
Factory method:
hey everyone. I am looking for the difference between simple factory and factory method.. I know the structural difference(images above), but I cant understand difference in use cases. for example, this is the explanation for factory method:
In Factory Method pattern we will introduce a new interface called
‘IMobileFactory’ and two concrete implementation’s ‘NokiaFactory’ and
‘IphoneFactory’. These concrete classes control the object creation.
In my example the client want a Nokia object. So the steps are given
below.
1.The client will load a reference to ‘NokiaFactory’. But Client won’t refer the ‘NokiaFactory’ class directly like the Simple Factory
pattern. The client refers the concrete implementation through an
interface ‘IMobileFactory’.
2.Then the Client call the ‘CreateMobile()’ method that will return an object of type ‘IMobile’.
3.Here we have to inform the client the concrete implementation to be used through some configuration or parameters.
I can't understand the first step:
But Client won’t refer the ‘NokiaFactory’ class directly like the
Simple Factory pattern. The client refers the concrete implementation
through an interface ‘IMobileFactory’.
so client writes something like this:
IMobileFactory factory = LoadFactory("NokiaFactory");
so why is that useful and better? what's the benefit? why shouldn't I just write this:
NokiaFactory factory = new NokiaFactory();
or what about that:
IMobileFactory factory = new NokiaFactory();
java oop design-patterns factory-pattern factory-method
add a comment |
up vote
1
down vote
favorite
Simple factory:
Factory method:
hey everyone. I am looking for the difference between simple factory and factory method.. I know the structural difference(images above), but I cant understand difference in use cases. for example, this is the explanation for factory method:
In Factory Method pattern we will introduce a new interface called
‘IMobileFactory’ and two concrete implementation’s ‘NokiaFactory’ and
‘IphoneFactory’. These concrete classes control the object creation.
In my example the client want a Nokia object. So the steps are given
below.
1.The client will load a reference to ‘NokiaFactory’. But Client won’t refer the ‘NokiaFactory’ class directly like the Simple Factory
pattern. The client refers the concrete implementation through an
interface ‘IMobileFactory’.
2.Then the Client call the ‘CreateMobile()’ method that will return an object of type ‘IMobile’.
3.Here we have to inform the client the concrete implementation to be used through some configuration or parameters.
I can't understand the first step:
But Client won’t refer the ‘NokiaFactory’ class directly like the
Simple Factory pattern. The client refers the concrete implementation
through an interface ‘IMobileFactory’.
so client writes something like this:
IMobileFactory factory = LoadFactory("NokiaFactory");
so why is that useful and better? what's the benefit? why shouldn't I just write this:
NokiaFactory factory = new NokiaFactory();
or what about that:
IMobileFactory factory = new NokiaFactory();
java oop design-patterns factory-pattern factory-method
Not really a fan of either; I would rather the author inject the reference to anIMobileFactory
instance during the constructor call. Either way, the goal is to avoid directly referencing concrete classes and use interfaces. Using an interface will make your code more flexible for testing / new use cases because it is not coupled to a single implementation.
– flakes
yesterday
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
Simple factory:
Factory method:
hey everyone. I am looking for the difference between simple factory and factory method.. I know the structural difference(images above), but I cant understand difference in use cases. for example, this is the explanation for factory method:
In Factory Method pattern we will introduce a new interface called
‘IMobileFactory’ and two concrete implementation’s ‘NokiaFactory’ and
‘IphoneFactory’. These concrete classes control the object creation.
In my example the client want a Nokia object. So the steps are given
below.
1.The client will load a reference to ‘NokiaFactory’. But Client won’t refer the ‘NokiaFactory’ class directly like the Simple Factory
pattern. The client refers the concrete implementation through an
interface ‘IMobileFactory’.
2.Then the Client call the ‘CreateMobile()’ method that will return an object of type ‘IMobile’.
3.Here we have to inform the client the concrete implementation to be used through some configuration or parameters.
I can't understand the first step:
But Client won’t refer the ‘NokiaFactory’ class directly like the
Simple Factory pattern. The client refers the concrete implementation
through an interface ‘IMobileFactory’.
so client writes something like this:
IMobileFactory factory = LoadFactory("NokiaFactory");
so why is that useful and better? what's the benefit? why shouldn't I just write this:
NokiaFactory factory = new NokiaFactory();
or what about that:
IMobileFactory factory = new NokiaFactory();
java oop design-patterns factory-pattern factory-method
Simple factory:
Factory method:
hey everyone. I am looking for the difference between simple factory and factory method.. I know the structural difference(images above), but I cant understand difference in use cases. for example, this is the explanation for factory method:
In Factory Method pattern we will introduce a new interface called
‘IMobileFactory’ and two concrete implementation’s ‘NokiaFactory’ and
‘IphoneFactory’. These concrete classes control the object creation.
In my example the client want a Nokia object. So the steps are given
below.
1.The client will load a reference to ‘NokiaFactory’. But Client won’t refer the ‘NokiaFactory’ class directly like the Simple Factory
pattern. The client refers the concrete implementation through an
interface ‘IMobileFactory’.
2.Then the Client call the ‘CreateMobile()’ method that will return an object of type ‘IMobile’.
3.Here we have to inform the client the concrete implementation to be used through some configuration or parameters.
I can't understand the first step:
But Client won’t refer the ‘NokiaFactory’ class directly like the
Simple Factory pattern. The client refers the concrete implementation
through an interface ‘IMobileFactory’.
so client writes something like this:
IMobileFactory factory = LoadFactory("NokiaFactory");
so why is that useful and better? what's the benefit? why shouldn't I just write this:
NokiaFactory factory = new NokiaFactory();
or what about that:
IMobileFactory factory = new NokiaFactory();
java oop design-patterns factory-pattern factory-method
java oop design-patterns factory-pattern factory-method
edited yesterday
asked yesterday


O. Shekriladze
406
406
Not really a fan of either; I would rather the author inject the reference to anIMobileFactory
instance during the constructor call. Either way, the goal is to avoid directly referencing concrete classes and use interfaces. Using an interface will make your code more flexible for testing / new use cases because it is not coupled to a single implementation.
– flakes
yesterday
add a comment |
Not really a fan of either; I would rather the author inject the reference to anIMobileFactory
instance during the constructor call. Either way, the goal is to avoid directly referencing concrete classes and use interfaces. Using an interface will make your code more flexible for testing / new use cases because it is not coupled to a single implementation.
– flakes
yesterday
Not really a fan of either; I would rather the author inject the reference to an
IMobileFactory
instance during the constructor call. Either way, the goal is to avoid directly referencing concrete classes and use interfaces. Using an interface will make your code more flexible for testing / new use cases because it is not coupled to a single implementation.– flakes
yesterday
Not really a fan of either; I would rather the author inject the reference to an
IMobileFactory
instance during the constructor call. Either way, the goal is to avoid directly referencing concrete classes and use interfaces. Using an interface will make your code more flexible for testing / new use cases because it is not coupled to a single implementation.– flakes
yesterday
add a comment |
3 Answers
3
active
oldest
votes
up vote
2
down vote
accepted
So your question is about comparing this design #1:
IMobileFactory factory = LoadFactory("NokiaFactory");
to this design #2:
NokiaFactory factory = new NokiaFactory(); // or:
IMobileFactory factory = new NokiaFactory();
As you see the biggest difference is that, while the client in the design #1 does not know about any concrete type like NokiaFactory
or IPhoneFactory
, the client in the design #2 does.
The downside of knowing about concrete things like NokiaFactory
or IPhoneFactory
should be well-known. If you want to make changes to these types, for example, you want to add a new method to NokiaFactory
and this method is not a part of the IMobileFactory
interface, then the client code will be affected unnecessarily. The client does not care about the new method, but its code must be recompiled/redeployed.
UPDATE
To explain more.
For example, a new method named Foo
is added to the NokiaFactory
class:
class NokiaFactory {
// old code
public void Foo() { ... }
}
Foo
is not a method of the IMobileFactory
interface but it is added to NokiaFactory
because there is another client who requires the method, and that client is happy to depend on NokiaFactory
class. In other words, that client would welcome changes from NokiaFactory
class, but the first client would not.
I cant understand this part: "you want to add a new method to NokiaFactory and this method is not a part of the IMobileFactory interface, then client code will be affected unnecessarily". could you explain me this clearly?
– O. Shekriladze
yesterday
Yes, edited my answer.
– Nghia Bui
yesterday
add a comment |
up vote
2
down vote
You need the interface to avoid creating different flow per type, for 10 types you will have 1 lines of code instead of 10
IMobileFactory factory = loadFactory(myFactoryName);
instead of per type:
NokiaFactory factory = new NokiaFactory();
...
IphoneFactory factory = new IphoneFactory();
...
The difference between using loadFactory
method to new NokiaFactory()
is that you don't need to explicitly create a new object, you delegate object creation to loadFactory
which returns relevant object
add a comment |
up vote
0
down vote
From the design perspective:
Use SimpleFactory when the types of Objects are not fixed. For example: Phones my manufacturer - Nokia, iPhone. May be you want to add a new manufacturer tomorrow.
Use Factory method when the types of Objects are fixed. For example: Phones by type - Landline and Mobile.
Finally, it comes down to how you want to design your system. What you want to be extendable.
binpress.com/factory-design-pattern/… according to this article it's vice-versa
– O. Shekriladze
yesterday
As I understand the post, it says the same thing I mentioned here. Use Factory method for Toys by location and use SimpleFactory for Toys by type.
– Dakshinamurthy Karra
yesterday
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
So your question is about comparing this design #1:
IMobileFactory factory = LoadFactory("NokiaFactory");
to this design #2:
NokiaFactory factory = new NokiaFactory(); // or:
IMobileFactory factory = new NokiaFactory();
As you see the biggest difference is that, while the client in the design #1 does not know about any concrete type like NokiaFactory
or IPhoneFactory
, the client in the design #2 does.
The downside of knowing about concrete things like NokiaFactory
or IPhoneFactory
should be well-known. If you want to make changes to these types, for example, you want to add a new method to NokiaFactory
and this method is not a part of the IMobileFactory
interface, then the client code will be affected unnecessarily. The client does not care about the new method, but its code must be recompiled/redeployed.
UPDATE
To explain more.
For example, a new method named Foo
is added to the NokiaFactory
class:
class NokiaFactory {
// old code
public void Foo() { ... }
}
Foo
is not a method of the IMobileFactory
interface but it is added to NokiaFactory
because there is another client who requires the method, and that client is happy to depend on NokiaFactory
class. In other words, that client would welcome changes from NokiaFactory
class, but the first client would not.
I cant understand this part: "you want to add a new method to NokiaFactory and this method is not a part of the IMobileFactory interface, then client code will be affected unnecessarily". could you explain me this clearly?
– O. Shekriladze
yesterday
Yes, edited my answer.
– Nghia Bui
yesterday
add a comment |
up vote
2
down vote
accepted
So your question is about comparing this design #1:
IMobileFactory factory = LoadFactory("NokiaFactory");
to this design #2:
NokiaFactory factory = new NokiaFactory(); // or:
IMobileFactory factory = new NokiaFactory();
As you see the biggest difference is that, while the client in the design #1 does not know about any concrete type like NokiaFactory
or IPhoneFactory
, the client in the design #2 does.
The downside of knowing about concrete things like NokiaFactory
or IPhoneFactory
should be well-known. If you want to make changes to these types, for example, you want to add a new method to NokiaFactory
and this method is not a part of the IMobileFactory
interface, then the client code will be affected unnecessarily. The client does not care about the new method, but its code must be recompiled/redeployed.
UPDATE
To explain more.
For example, a new method named Foo
is added to the NokiaFactory
class:
class NokiaFactory {
// old code
public void Foo() { ... }
}
Foo
is not a method of the IMobileFactory
interface but it is added to NokiaFactory
because there is another client who requires the method, and that client is happy to depend on NokiaFactory
class. In other words, that client would welcome changes from NokiaFactory
class, but the first client would not.
I cant understand this part: "you want to add a new method to NokiaFactory and this method is not a part of the IMobileFactory interface, then client code will be affected unnecessarily". could you explain me this clearly?
– O. Shekriladze
yesterday
Yes, edited my answer.
– Nghia Bui
yesterday
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
So your question is about comparing this design #1:
IMobileFactory factory = LoadFactory("NokiaFactory");
to this design #2:
NokiaFactory factory = new NokiaFactory(); // or:
IMobileFactory factory = new NokiaFactory();
As you see the biggest difference is that, while the client in the design #1 does not know about any concrete type like NokiaFactory
or IPhoneFactory
, the client in the design #2 does.
The downside of knowing about concrete things like NokiaFactory
or IPhoneFactory
should be well-known. If you want to make changes to these types, for example, you want to add a new method to NokiaFactory
and this method is not a part of the IMobileFactory
interface, then the client code will be affected unnecessarily. The client does not care about the new method, but its code must be recompiled/redeployed.
UPDATE
To explain more.
For example, a new method named Foo
is added to the NokiaFactory
class:
class NokiaFactory {
// old code
public void Foo() { ... }
}
Foo
is not a method of the IMobileFactory
interface but it is added to NokiaFactory
because there is another client who requires the method, and that client is happy to depend on NokiaFactory
class. In other words, that client would welcome changes from NokiaFactory
class, but the first client would not.
So your question is about comparing this design #1:
IMobileFactory factory = LoadFactory("NokiaFactory");
to this design #2:
NokiaFactory factory = new NokiaFactory(); // or:
IMobileFactory factory = new NokiaFactory();
As you see the biggest difference is that, while the client in the design #1 does not know about any concrete type like NokiaFactory
or IPhoneFactory
, the client in the design #2 does.
The downside of knowing about concrete things like NokiaFactory
or IPhoneFactory
should be well-known. If you want to make changes to these types, for example, you want to add a new method to NokiaFactory
and this method is not a part of the IMobileFactory
interface, then the client code will be affected unnecessarily. The client does not care about the new method, but its code must be recompiled/redeployed.
UPDATE
To explain more.
For example, a new method named Foo
is added to the NokiaFactory
class:
class NokiaFactory {
// old code
public void Foo() { ... }
}
Foo
is not a method of the IMobileFactory
interface but it is added to NokiaFactory
because there is another client who requires the method, and that client is happy to depend on NokiaFactory
class. In other words, that client would welcome changes from NokiaFactory
class, but the first client would not.
edited yesterday
answered yesterday
Nghia Bui
1,398811
1,398811
I cant understand this part: "you want to add a new method to NokiaFactory and this method is not a part of the IMobileFactory interface, then client code will be affected unnecessarily". could you explain me this clearly?
– O. Shekriladze
yesterday
Yes, edited my answer.
– Nghia Bui
yesterday
add a comment |
I cant understand this part: "you want to add a new method to NokiaFactory and this method is not a part of the IMobileFactory interface, then client code will be affected unnecessarily". could you explain me this clearly?
– O. Shekriladze
yesterday
Yes, edited my answer.
– Nghia Bui
yesterday
I cant understand this part: "you want to add a new method to NokiaFactory and this method is not a part of the IMobileFactory interface, then client code will be affected unnecessarily". could you explain me this clearly?
– O. Shekriladze
yesterday
I cant understand this part: "you want to add a new method to NokiaFactory and this method is not a part of the IMobileFactory interface, then client code will be affected unnecessarily". could you explain me this clearly?
– O. Shekriladze
yesterday
Yes, edited my answer.
– Nghia Bui
yesterday
Yes, edited my answer.
– Nghia Bui
yesterday
add a comment |
up vote
2
down vote
You need the interface to avoid creating different flow per type, for 10 types you will have 1 lines of code instead of 10
IMobileFactory factory = loadFactory(myFactoryName);
instead of per type:
NokiaFactory factory = new NokiaFactory();
...
IphoneFactory factory = new IphoneFactory();
...
The difference between using loadFactory
method to new NokiaFactory()
is that you don't need to explicitly create a new object, you delegate object creation to loadFactory
which returns relevant object
add a comment |
up vote
2
down vote
You need the interface to avoid creating different flow per type, for 10 types you will have 1 lines of code instead of 10
IMobileFactory factory = loadFactory(myFactoryName);
instead of per type:
NokiaFactory factory = new NokiaFactory();
...
IphoneFactory factory = new IphoneFactory();
...
The difference between using loadFactory
method to new NokiaFactory()
is that you don't need to explicitly create a new object, you delegate object creation to loadFactory
which returns relevant object
add a comment |
up vote
2
down vote
up vote
2
down vote
You need the interface to avoid creating different flow per type, for 10 types you will have 1 lines of code instead of 10
IMobileFactory factory = loadFactory(myFactoryName);
instead of per type:
NokiaFactory factory = new NokiaFactory();
...
IphoneFactory factory = new IphoneFactory();
...
The difference between using loadFactory
method to new NokiaFactory()
is that you don't need to explicitly create a new object, you delegate object creation to loadFactory
which returns relevant object
You need the interface to avoid creating different flow per type, for 10 types you will have 1 lines of code instead of 10
IMobileFactory factory = loadFactory(myFactoryName);
instead of per type:
NokiaFactory factory = new NokiaFactory();
...
IphoneFactory factory = new IphoneFactory();
...
The difference between using loadFactory
method to new NokiaFactory()
is that you don't need to explicitly create a new object, you delegate object creation to loadFactory
which returns relevant object
edited yesterday
answered yesterday


user7294900
18.2k93056
18.2k93056
add a comment |
add a comment |
up vote
0
down vote
From the design perspective:
Use SimpleFactory when the types of Objects are not fixed. For example: Phones my manufacturer - Nokia, iPhone. May be you want to add a new manufacturer tomorrow.
Use Factory method when the types of Objects are fixed. For example: Phones by type - Landline and Mobile.
Finally, it comes down to how you want to design your system. What you want to be extendable.
binpress.com/factory-design-pattern/… according to this article it's vice-versa
– O. Shekriladze
yesterday
As I understand the post, it says the same thing I mentioned here. Use Factory method for Toys by location and use SimpleFactory for Toys by type.
– Dakshinamurthy Karra
yesterday
add a comment |
up vote
0
down vote
From the design perspective:
Use SimpleFactory when the types of Objects are not fixed. For example: Phones my manufacturer - Nokia, iPhone. May be you want to add a new manufacturer tomorrow.
Use Factory method when the types of Objects are fixed. For example: Phones by type - Landline and Mobile.
Finally, it comes down to how you want to design your system. What you want to be extendable.
binpress.com/factory-design-pattern/… according to this article it's vice-versa
– O. Shekriladze
yesterday
As I understand the post, it says the same thing I mentioned here. Use Factory method for Toys by location and use SimpleFactory for Toys by type.
– Dakshinamurthy Karra
yesterday
add a comment |
up vote
0
down vote
up vote
0
down vote
From the design perspective:
Use SimpleFactory when the types of Objects are not fixed. For example: Phones my manufacturer - Nokia, iPhone. May be you want to add a new manufacturer tomorrow.
Use Factory method when the types of Objects are fixed. For example: Phones by type - Landline and Mobile.
Finally, it comes down to how you want to design your system. What you want to be extendable.
From the design perspective:
Use SimpleFactory when the types of Objects are not fixed. For example: Phones my manufacturer - Nokia, iPhone. May be you want to add a new manufacturer tomorrow.
Use Factory method when the types of Objects are fixed. For example: Phones by type - Landline and Mobile.
Finally, it comes down to how you want to design your system. What you want to be extendable.
answered yesterday
Dakshinamurthy Karra
4,0331921
4,0331921
binpress.com/factory-design-pattern/… according to this article it's vice-versa
– O. Shekriladze
yesterday
As I understand the post, it says the same thing I mentioned here. Use Factory method for Toys by location and use SimpleFactory for Toys by type.
– Dakshinamurthy Karra
yesterday
add a comment |
binpress.com/factory-design-pattern/… according to this article it's vice-versa
– O. Shekriladze
yesterday
As I understand the post, it says the same thing I mentioned here. Use Factory method for Toys by location and use SimpleFactory for Toys by type.
– Dakshinamurthy Karra
yesterday
binpress.com/factory-design-pattern/… according to this article it's vice-versa
– O. Shekriladze
yesterday
binpress.com/factory-design-pattern/… according to this article it's vice-versa
– O. Shekriladze
yesterday
As I understand the post, it says the same thing I mentioned here. Use Factory method for Toys by location and use SimpleFactory for Toys by type.
– Dakshinamurthy Karra
yesterday
As I understand the post, it says the same thing I mentioned here. Use Factory method for Toys by location and use SimpleFactory for Toys by type.
– Dakshinamurthy Karra
yesterday
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53372254%2fsimple-factory-vs-factory-method%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZC5 UvQRXC,8S5,EJQmq8GtWfa5u,ybIUu
Not really a fan of either; I would rather the author inject the reference to an
IMobileFactory
instance during the constructor call. Either way, the goal is to avoid directly referencing concrete classes and use interfaces. Using an interface will make your code more flexible for testing / new use cases because it is not coupled to a single implementation.– flakes
yesterday