Using ClaimsIdentity to retrieve user profile via AAD graph
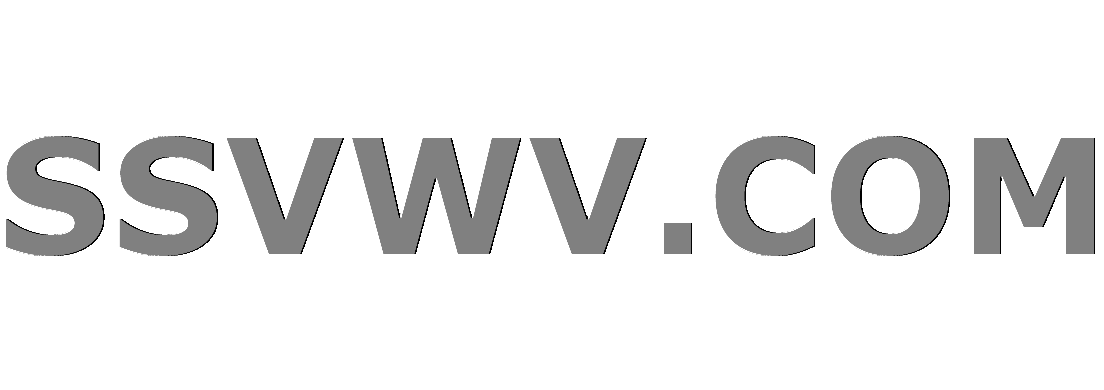
Multi tool use
up vote
0
down vote
favorite
I am building user authentication based on Azure Active Directory using OpenId Connect.
I can successfully log into my website by using my account in AAD.
After I'm logged in, I would like to retrieve my account information via the AAD graph API.
I can get the user ID via the ClaimsIdentity:
ClaimsIdentity identity = this.User.Identity as ClaimsIdentity;
string userID = identity.GetUserId();
And I can get user information via the graph API:
public IUser GetProfileById(string userID)
{
ActiveDirectoryClient client = GetAadClient();
var user = client.Users.Where(x => x.ObjectId == userID).ExecuteSingleAsync().Result;
return user;
}
However, the user ID that is stored on the ClaimsPrincipal does not seem to be the Object ID in AAD, so I'll need to find some other way to retrieve the user information. Another option might be to find the user based on the email address, but I can't find out how to do that either.
Any help or suggestions will be appreciated.
c#

add a comment |
up vote
0
down vote
favorite
I am building user authentication based on Azure Active Directory using OpenId Connect.
I can successfully log into my website by using my account in AAD.
After I'm logged in, I would like to retrieve my account information via the AAD graph API.
I can get the user ID via the ClaimsIdentity:
ClaimsIdentity identity = this.User.Identity as ClaimsIdentity;
string userID = identity.GetUserId();
And I can get user information via the graph API:
public IUser GetProfileById(string userID)
{
ActiveDirectoryClient client = GetAadClient();
var user = client.Users.Where(x => x.ObjectId == userID).ExecuteSingleAsync().Result;
return user;
}
However, the user ID that is stored on the ClaimsPrincipal does not seem to be the Object ID in AAD, so I'll need to find some other way to retrieve the user information. Another option might be to find the user based on the email address, but I can't find out how to do that either.
Any help or suggestions will be appreciated.
c#

Have you checked the other claims available on the identity via a debugger? The object id should be there.
– juunas
May 25 at 13:01
Thanks @juunas you are totally right, I found the object ID in one of the claims
– Bart van der Drift
May 25 at 13:56
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am building user authentication based on Azure Active Directory using OpenId Connect.
I can successfully log into my website by using my account in AAD.
After I'm logged in, I would like to retrieve my account information via the AAD graph API.
I can get the user ID via the ClaimsIdentity:
ClaimsIdentity identity = this.User.Identity as ClaimsIdentity;
string userID = identity.GetUserId();
And I can get user information via the graph API:
public IUser GetProfileById(string userID)
{
ActiveDirectoryClient client = GetAadClient();
var user = client.Users.Where(x => x.ObjectId == userID).ExecuteSingleAsync().Result;
return user;
}
However, the user ID that is stored on the ClaimsPrincipal does not seem to be the Object ID in AAD, so I'll need to find some other way to retrieve the user information. Another option might be to find the user based on the email address, but I can't find out how to do that either.
Any help or suggestions will be appreciated.
c#

I am building user authentication based on Azure Active Directory using OpenId Connect.
I can successfully log into my website by using my account in AAD.
After I'm logged in, I would like to retrieve my account information via the AAD graph API.
I can get the user ID via the ClaimsIdentity:
ClaimsIdentity identity = this.User.Identity as ClaimsIdentity;
string userID = identity.GetUserId();
And I can get user information via the graph API:
public IUser GetProfileById(string userID)
{
ActiveDirectoryClient client = GetAadClient();
var user = client.Users.Where(x => x.ObjectId == userID).ExecuteSingleAsync().Result;
return user;
}
However, the user ID that is stored on the ClaimsPrincipal does not seem to be the Object ID in AAD, so I'll need to find some other way to retrieve the user information. Another option might be to find the user based on the email address, but I can't find out how to do that either.
Any help or suggestions will be appreciated.
c#

c#

edited May 25 at 12:29
asked May 25 at 12:22


Bart van der Drift
704620
704620
Have you checked the other claims available on the identity via a debugger? The object id should be there.
– juunas
May 25 at 13:01
Thanks @juunas you are totally right, I found the object ID in one of the claims
– Bart van der Drift
May 25 at 13:56
add a comment |
Have you checked the other claims available on the identity via a debugger? The object id should be there.
– juunas
May 25 at 13:01
Thanks @juunas you are totally right, I found the object ID in one of the claims
– Bart van der Drift
May 25 at 13:56
Have you checked the other claims available on the identity via a debugger? The object id should be there.
– juunas
May 25 at 13:01
Have you checked the other claims available on the identity via a debugger? The object id should be there.
– juunas
May 25 at 13:01
Thanks @juunas you are totally right, I found the object ID in one of the claims
– Bart van der Drift
May 25 at 13:56
Thanks @juunas you are totally right, I found the object ID in one of the claims
– Bart van der Drift
May 25 at 13:56
add a comment |
2 Answers
2
active
oldest
votes
up vote
2
down vote
accepted
Thanks to @juunas I found out the type:
ClaimsIdentity identity = this.User.Identity as ClaimsIdentity;
string objectID = identity.Claims.FirstOrDefault(x => x.Type == "http://schemas.microsoft.com/identity/claims/objectidentifier")?.Value;
Then the object ID can be used to obtain the user information via the AAD graph API.
1
first of all thank you, but you can call FirstOrDefault() directly from 'identity.Claims' this will help to lighten the memory
– maliness
May 29 at 12:55
1
Thank you @maliness, I have updated my answer with your suggestion.
– Bart van der Drift
May 31 at 7:45
add a comment |
up vote
0
down vote
Here's a quick answer for anyone else still looking.
public void GetUserFromAD(GraphServiceClient gsc)
{
try
{
ClaimsIdentity identity = ClaimsPrincipal.Current.Identity as ClaimsIdentity;
string objectID = identity.Claims.FirstOrDefault(x => x.Type == "http://schemas.microsoft.com/identity/claims/objectidentifier")?.Value;
var user = gsc.Users[objectID].Request().GetAsync().Result;
}
catch(Exception ex)
{
// do something.
}
}
Note you can use direct lookup using Users[objectid] rather than enumerating through the result list.
New contributor
harlowkk is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Thanks to @juunas I found out the type:
ClaimsIdentity identity = this.User.Identity as ClaimsIdentity;
string objectID = identity.Claims.FirstOrDefault(x => x.Type == "http://schemas.microsoft.com/identity/claims/objectidentifier")?.Value;
Then the object ID can be used to obtain the user information via the AAD graph API.
1
first of all thank you, but you can call FirstOrDefault() directly from 'identity.Claims' this will help to lighten the memory
– maliness
May 29 at 12:55
1
Thank you @maliness, I have updated my answer with your suggestion.
– Bart van der Drift
May 31 at 7:45
add a comment |
up vote
2
down vote
accepted
Thanks to @juunas I found out the type:
ClaimsIdentity identity = this.User.Identity as ClaimsIdentity;
string objectID = identity.Claims.FirstOrDefault(x => x.Type == "http://schemas.microsoft.com/identity/claims/objectidentifier")?.Value;
Then the object ID can be used to obtain the user information via the AAD graph API.
1
first of all thank you, but you can call FirstOrDefault() directly from 'identity.Claims' this will help to lighten the memory
– maliness
May 29 at 12:55
1
Thank you @maliness, I have updated my answer with your suggestion.
– Bart van der Drift
May 31 at 7:45
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Thanks to @juunas I found out the type:
ClaimsIdentity identity = this.User.Identity as ClaimsIdentity;
string objectID = identity.Claims.FirstOrDefault(x => x.Type == "http://schemas.microsoft.com/identity/claims/objectidentifier")?.Value;
Then the object ID can be used to obtain the user information via the AAD graph API.
Thanks to @juunas I found out the type:
ClaimsIdentity identity = this.User.Identity as ClaimsIdentity;
string objectID = identity.Claims.FirstOrDefault(x => x.Type == "http://schemas.microsoft.com/identity/claims/objectidentifier")?.Value;
Then the object ID can be used to obtain the user information via the AAD graph API.
edited May 31 at 7:44
answered May 25 at 13:55


Bart van der Drift
704620
704620
1
first of all thank you, but you can call FirstOrDefault() directly from 'identity.Claims' this will help to lighten the memory
– maliness
May 29 at 12:55
1
Thank you @maliness, I have updated my answer with your suggestion.
– Bart van der Drift
May 31 at 7:45
add a comment |
1
first of all thank you, but you can call FirstOrDefault() directly from 'identity.Claims' this will help to lighten the memory
– maliness
May 29 at 12:55
1
Thank you @maliness, I have updated my answer with your suggestion.
– Bart van der Drift
May 31 at 7:45
1
1
first of all thank you, but you can call FirstOrDefault() directly from 'identity.Claims' this will help to lighten the memory
– maliness
May 29 at 12:55
first of all thank you, but you can call FirstOrDefault() directly from 'identity.Claims' this will help to lighten the memory
– maliness
May 29 at 12:55
1
1
Thank you @maliness, I have updated my answer with your suggestion.
– Bart van der Drift
May 31 at 7:45
Thank you @maliness, I have updated my answer with your suggestion.
– Bart van der Drift
May 31 at 7:45
add a comment |
up vote
0
down vote
Here's a quick answer for anyone else still looking.
public void GetUserFromAD(GraphServiceClient gsc)
{
try
{
ClaimsIdentity identity = ClaimsPrincipal.Current.Identity as ClaimsIdentity;
string objectID = identity.Claims.FirstOrDefault(x => x.Type == "http://schemas.microsoft.com/identity/claims/objectidentifier")?.Value;
var user = gsc.Users[objectID].Request().GetAsync().Result;
}
catch(Exception ex)
{
// do something.
}
}
Note you can use direct lookup using Users[objectid] rather than enumerating through the result list.
New contributor
harlowkk is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
Here's a quick answer for anyone else still looking.
public void GetUserFromAD(GraphServiceClient gsc)
{
try
{
ClaimsIdentity identity = ClaimsPrincipal.Current.Identity as ClaimsIdentity;
string objectID = identity.Claims.FirstOrDefault(x => x.Type == "http://schemas.microsoft.com/identity/claims/objectidentifier")?.Value;
var user = gsc.Users[objectID].Request().GetAsync().Result;
}
catch(Exception ex)
{
// do something.
}
}
Note you can use direct lookup using Users[objectid] rather than enumerating through the result list.
New contributor
harlowkk is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
up vote
0
down vote
Here's a quick answer for anyone else still looking.
public void GetUserFromAD(GraphServiceClient gsc)
{
try
{
ClaimsIdentity identity = ClaimsPrincipal.Current.Identity as ClaimsIdentity;
string objectID = identity.Claims.FirstOrDefault(x => x.Type == "http://schemas.microsoft.com/identity/claims/objectidentifier")?.Value;
var user = gsc.Users[objectID].Request().GetAsync().Result;
}
catch(Exception ex)
{
// do something.
}
}
Note you can use direct lookup using Users[objectid] rather than enumerating through the result list.
New contributor
harlowkk is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Here's a quick answer for anyone else still looking.
public void GetUserFromAD(GraphServiceClient gsc)
{
try
{
ClaimsIdentity identity = ClaimsPrincipal.Current.Identity as ClaimsIdentity;
string objectID = identity.Claims.FirstOrDefault(x => x.Type == "http://schemas.microsoft.com/identity/claims/objectidentifier")?.Value;
var user = gsc.Users[objectID].Request().GetAsync().Result;
}
catch(Exception ex)
{
// do something.
}
}
Note you can use direct lookup using Users[objectid] rather than enumerating through the result list.
New contributor
harlowkk is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 45 mins ago
New contributor
harlowkk is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 2 hours ago
harlowkk
11
11
New contributor
harlowkk is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
harlowkk is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
harlowkk is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f50529142%2fusing-claimsidentity-to-retrieve-user-profile-via-aad-graph%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
h7lwF7KNwI,81EQD h9QOIF,q5ogRyVrwNqNpR OnisjqB36 O2q,W4YH
Have you checked the other claims available on the identity via a debugger? The object id should be there.
– juunas
May 25 at 13:01
Thanks @juunas you are totally right, I found the object ID in one of the claims
– Bart van der Drift
May 25 at 13:56