how to order a dictionary based on key by daywise in python
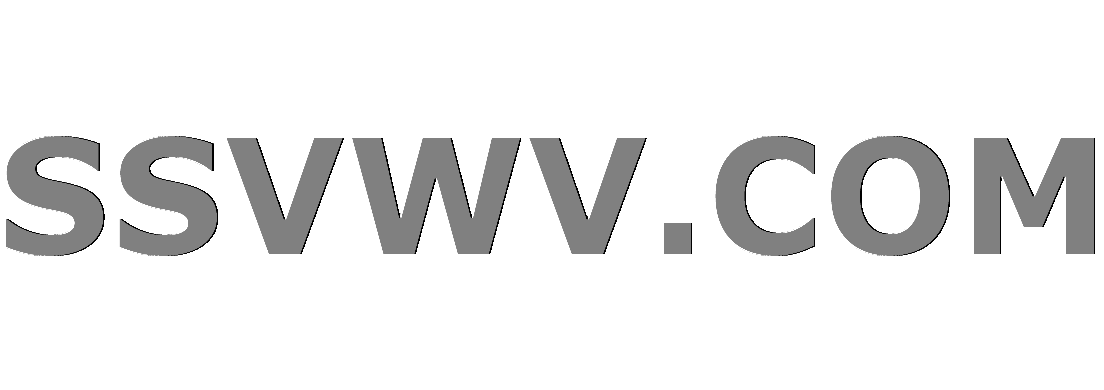
Multi tool use
up vote
1
down vote
favorite
I had 2 list
daydate=['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']
percentday=['0%', '17%', '27%', '11%', '7%', '19%', '19%']
i have converted into dictionary by doing
daydic=dict(zip(daydate, percentday))
the output is coming like
{'Monday': '17%', 'Tuesday': '27%', 'Friday': '19%', 'Wednesday': '11%', 'Thursday': '7%', 'Sunday': '0%', 'Saturday': '19%'}
i want to sort this dictionary like the order of elements in daywise like below
{'Sunday': '0%','Monday': '17%', 'Tuesday': '27%', 'Wednesday': '11%', 'Thursday': '7%', , 'Friday': '19%','Saturday': '19%'}
Help me
python list dictionary calendar ordereddict
add a comment |
up vote
1
down vote
favorite
I had 2 list
daydate=['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']
percentday=['0%', '17%', '27%', '11%', '7%', '19%', '19%']
i have converted into dictionary by doing
daydic=dict(zip(daydate, percentday))
the output is coming like
{'Monday': '17%', 'Tuesday': '27%', 'Friday': '19%', 'Wednesday': '11%', 'Thursday': '7%', 'Sunday': '0%', 'Saturday': '19%'}
i want to sort this dictionary like the order of elements in daywise like below
{'Sunday': '0%','Monday': '17%', 'Tuesday': '27%', 'Wednesday': '11%', 'Thursday': '7%', , 'Friday': '19%','Saturday': '19%'}
Help me
python list dictionary calendar ordereddict
3
Dictionaries don't have a sorting. You can sort it when you print, by sorting the keys
– planetmaker
Nov 19 at 12:39
1
@TomdeGeus: i want to sort by daywise,i.e., week day wise. Anyway thanks
– vtustudent
Nov 19 at 12:41
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I had 2 list
daydate=['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']
percentday=['0%', '17%', '27%', '11%', '7%', '19%', '19%']
i have converted into dictionary by doing
daydic=dict(zip(daydate, percentday))
the output is coming like
{'Monday': '17%', 'Tuesday': '27%', 'Friday': '19%', 'Wednesday': '11%', 'Thursday': '7%', 'Sunday': '0%', 'Saturday': '19%'}
i want to sort this dictionary like the order of elements in daywise like below
{'Sunday': '0%','Monday': '17%', 'Tuesday': '27%', 'Wednesday': '11%', 'Thursday': '7%', , 'Friday': '19%','Saturday': '19%'}
Help me
python list dictionary calendar ordereddict
I had 2 list
daydate=['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']
percentday=['0%', '17%', '27%', '11%', '7%', '19%', '19%']
i have converted into dictionary by doing
daydic=dict(zip(daydate, percentday))
the output is coming like
{'Monday': '17%', 'Tuesday': '27%', 'Friday': '19%', 'Wednesday': '11%', 'Thursday': '7%', 'Sunday': '0%', 'Saturday': '19%'}
i want to sort this dictionary like the order of elements in daywise like below
{'Sunday': '0%','Monday': '17%', 'Tuesday': '27%', 'Wednesday': '11%', 'Thursday': '7%', , 'Friday': '19%','Saturday': '19%'}
Help me
python list dictionary calendar ordereddict
python list dictionary calendar ordereddict
edited Nov 19 at 12:53
asked Nov 19 at 12:36
vtustudent
276
276
3
Dictionaries don't have a sorting. You can sort it when you print, by sorting the keys
– planetmaker
Nov 19 at 12:39
1
@TomdeGeus: i want to sort by daywise,i.e., week day wise. Anyway thanks
– vtustudent
Nov 19 at 12:41
add a comment |
3
Dictionaries don't have a sorting. You can sort it when you print, by sorting the keys
– planetmaker
Nov 19 at 12:39
1
@TomdeGeus: i want to sort by daywise,i.e., week day wise. Anyway thanks
– vtustudent
Nov 19 at 12:41
3
3
Dictionaries don't have a sorting. You can sort it when you print, by sorting the keys
– planetmaker
Nov 19 at 12:39
Dictionaries don't have a sorting. You can sort it when you print, by sorting the keys
– planetmaker
Nov 19 at 12:39
1
1
@TomdeGeus: i want to sort by daywise,i.e., week day wise. Anyway thanks
– vtustudent
Nov 19 at 12:41
@TomdeGeus: i want to sort by daywise,i.e., week day wise. Anyway thanks
– vtustudent
Nov 19 at 12:41
add a comment |
3 Answers
3
active
oldest
votes
up vote
6
down vote
Dictionaries should not be considered ordered
They are, in fact, insertion ordered in Python 3.6+ (officially 3.7+), but even so you should prefer to use OrderedDict
for a robust ordered mapping.
In addition, you should never have to type days of the week manually, you can import from calendar.day_name
and rotate via deque
:
from calendar import day_name
from collections import deque, OrderedDict
daydate = deque(day_name)
daydate.rotate(1)
percentday = ['0%', '17%', '27%', '11%', '7%', '19%', '19%']
res = OrderedDict(zip(daydate, percentday))
OrderedDict([('Sunday', '0%'),
('Monday', '17%'),
('Tuesday', '27%'),
('Wednesday', '11%'),
('Thursday', '7%'),
('Friday', '19%'),
('Saturday', '19%')])
add a comment |
up vote
0
down vote
As told, a dict is not ordered, but you can order before to convert to dict.
daydate=['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']
percentday=['0%', '17%', '27%', '11%', '7%', '19%', '19%']
Sort by day, just rotate the zip:
dayzip = list(zip(daydate, percentday))
dayzip = dayzip[1-len(dayzip):] + dayzip[:1-len(dayzip)]
daydict = dict(dayzip)
#=> {'Sunday': '0%', 'Monday': '17%', 'Tuesday': '27%', 'Wednesday': '11%', 'Thursday': '7%', 'Friday': '19%', 'Saturday': '19%'}
To sort by percentage:
dayzip = list(zip(daydate, percentday))
dayzip.sort(key=lambda x: float(x[1][:-1]) )
daydict = dict(dayzip)
#=> [('Sunday', '0%'), ('Monday', '17%'), ('Tuesday', '27%'), ('Wednesday', '11%'), ('Thursday', '7%'), ('Friday', '19%'), ('Saturday', '19%')]
#=> {'Sunday': '0%', 'Thursday': '7%', 'Wednesday': '11%', 'Monday': '17%', 'Friday': '19%', 'Saturday': '19%', 'Tuesday': '27%'}
But OP wants to sort by day, not by value.
– jpp
Nov 19 at 12:46
Oops! In case, rotate stackoverflow.com/questions/9457832/python-list-rotation. I''l edit.
– iGian
Nov 19 at 12:48
add a comment |
up vote
0
down vote
You can try this:
from collections import OrderedDict
daydic={'Friday': '19%', 'Wednesday': '11%', 'Monday': '17%', 'Thursday': '7%', 'Saturday': '19%', 'Sunday': '0%', 'Tuesday': '27%'}
weeks=['Sunday','Monday','Tuesday','Wednesday','Thursday','Friday','Saturday']
print(OrderedDict(sorted(daydic.items(),key =lambda x:weeks.index(x[0]))))
This will output as:
OrderedDict([('Sunday', '0%'), ('Monday', '17%'), ('Tuesday', '27%'), ('Wednesday', '11%'),
('Thursday', '7%'), ('Friday', '19%'), ('Saturday', '19%')])
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
6
down vote
Dictionaries should not be considered ordered
They are, in fact, insertion ordered in Python 3.6+ (officially 3.7+), but even so you should prefer to use OrderedDict
for a robust ordered mapping.
In addition, you should never have to type days of the week manually, you can import from calendar.day_name
and rotate via deque
:
from calendar import day_name
from collections import deque, OrderedDict
daydate = deque(day_name)
daydate.rotate(1)
percentday = ['0%', '17%', '27%', '11%', '7%', '19%', '19%']
res = OrderedDict(zip(daydate, percentday))
OrderedDict([('Sunday', '0%'),
('Monday', '17%'),
('Tuesday', '27%'),
('Wednesday', '11%'),
('Thursday', '7%'),
('Friday', '19%'),
('Saturday', '19%')])
add a comment |
up vote
6
down vote
Dictionaries should not be considered ordered
They are, in fact, insertion ordered in Python 3.6+ (officially 3.7+), but even so you should prefer to use OrderedDict
for a robust ordered mapping.
In addition, you should never have to type days of the week manually, you can import from calendar.day_name
and rotate via deque
:
from calendar import day_name
from collections import deque, OrderedDict
daydate = deque(day_name)
daydate.rotate(1)
percentday = ['0%', '17%', '27%', '11%', '7%', '19%', '19%']
res = OrderedDict(zip(daydate, percentday))
OrderedDict([('Sunday', '0%'),
('Monday', '17%'),
('Tuesday', '27%'),
('Wednesday', '11%'),
('Thursday', '7%'),
('Friday', '19%'),
('Saturday', '19%')])
add a comment |
up vote
6
down vote
up vote
6
down vote
Dictionaries should not be considered ordered
They are, in fact, insertion ordered in Python 3.6+ (officially 3.7+), but even so you should prefer to use OrderedDict
for a robust ordered mapping.
In addition, you should never have to type days of the week manually, you can import from calendar.day_name
and rotate via deque
:
from calendar import day_name
from collections import deque, OrderedDict
daydate = deque(day_name)
daydate.rotate(1)
percentday = ['0%', '17%', '27%', '11%', '7%', '19%', '19%']
res = OrderedDict(zip(daydate, percentday))
OrderedDict([('Sunday', '0%'),
('Monday', '17%'),
('Tuesday', '27%'),
('Wednesday', '11%'),
('Thursday', '7%'),
('Friday', '19%'),
('Saturday', '19%')])
Dictionaries should not be considered ordered
They are, in fact, insertion ordered in Python 3.6+ (officially 3.7+), but even so you should prefer to use OrderedDict
for a robust ordered mapping.
In addition, you should never have to type days of the week manually, you can import from calendar.day_name
and rotate via deque
:
from calendar import day_name
from collections import deque, OrderedDict
daydate = deque(day_name)
daydate.rotate(1)
percentday = ['0%', '17%', '27%', '11%', '7%', '19%', '19%']
res = OrderedDict(zip(daydate, percentday))
OrderedDict([('Sunday', '0%'),
('Monday', '17%'),
('Tuesday', '27%'),
('Wednesday', '11%'),
('Thursday', '7%'),
('Friday', '19%'),
('Saturday', '19%')])
answered Nov 19 at 12:42


jpp
83.5k194896
83.5k194896
add a comment |
add a comment |
up vote
0
down vote
As told, a dict is not ordered, but you can order before to convert to dict.
daydate=['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']
percentday=['0%', '17%', '27%', '11%', '7%', '19%', '19%']
Sort by day, just rotate the zip:
dayzip = list(zip(daydate, percentday))
dayzip = dayzip[1-len(dayzip):] + dayzip[:1-len(dayzip)]
daydict = dict(dayzip)
#=> {'Sunday': '0%', 'Monday': '17%', 'Tuesday': '27%', 'Wednesday': '11%', 'Thursday': '7%', 'Friday': '19%', 'Saturday': '19%'}
To sort by percentage:
dayzip = list(zip(daydate, percentday))
dayzip.sort(key=lambda x: float(x[1][:-1]) )
daydict = dict(dayzip)
#=> [('Sunday', '0%'), ('Monday', '17%'), ('Tuesday', '27%'), ('Wednesday', '11%'), ('Thursday', '7%'), ('Friday', '19%'), ('Saturday', '19%')]
#=> {'Sunday': '0%', 'Thursday': '7%', 'Wednesday': '11%', 'Monday': '17%', 'Friday': '19%', 'Saturday': '19%', 'Tuesday': '27%'}
But OP wants to sort by day, not by value.
– jpp
Nov 19 at 12:46
Oops! In case, rotate stackoverflow.com/questions/9457832/python-list-rotation. I''l edit.
– iGian
Nov 19 at 12:48
add a comment |
up vote
0
down vote
As told, a dict is not ordered, but you can order before to convert to dict.
daydate=['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']
percentday=['0%', '17%', '27%', '11%', '7%', '19%', '19%']
Sort by day, just rotate the zip:
dayzip = list(zip(daydate, percentday))
dayzip = dayzip[1-len(dayzip):] + dayzip[:1-len(dayzip)]
daydict = dict(dayzip)
#=> {'Sunday': '0%', 'Monday': '17%', 'Tuesday': '27%', 'Wednesday': '11%', 'Thursday': '7%', 'Friday': '19%', 'Saturday': '19%'}
To sort by percentage:
dayzip = list(zip(daydate, percentday))
dayzip.sort(key=lambda x: float(x[1][:-1]) )
daydict = dict(dayzip)
#=> [('Sunday', '0%'), ('Monday', '17%'), ('Tuesday', '27%'), ('Wednesday', '11%'), ('Thursday', '7%'), ('Friday', '19%'), ('Saturday', '19%')]
#=> {'Sunday': '0%', 'Thursday': '7%', 'Wednesday': '11%', 'Monday': '17%', 'Friday': '19%', 'Saturday': '19%', 'Tuesday': '27%'}
But OP wants to sort by day, not by value.
– jpp
Nov 19 at 12:46
Oops! In case, rotate stackoverflow.com/questions/9457832/python-list-rotation. I''l edit.
– iGian
Nov 19 at 12:48
add a comment |
up vote
0
down vote
up vote
0
down vote
As told, a dict is not ordered, but you can order before to convert to dict.
daydate=['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']
percentday=['0%', '17%', '27%', '11%', '7%', '19%', '19%']
Sort by day, just rotate the zip:
dayzip = list(zip(daydate, percentday))
dayzip = dayzip[1-len(dayzip):] + dayzip[:1-len(dayzip)]
daydict = dict(dayzip)
#=> {'Sunday': '0%', 'Monday': '17%', 'Tuesday': '27%', 'Wednesday': '11%', 'Thursday': '7%', 'Friday': '19%', 'Saturday': '19%'}
To sort by percentage:
dayzip = list(zip(daydate, percentday))
dayzip.sort(key=lambda x: float(x[1][:-1]) )
daydict = dict(dayzip)
#=> [('Sunday', '0%'), ('Monday', '17%'), ('Tuesday', '27%'), ('Wednesday', '11%'), ('Thursday', '7%'), ('Friday', '19%'), ('Saturday', '19%')]
#=> {'Sunday': '0%', 'Thursday': '7%', 'Wednesday': '11%', 'Monday': '17%', 'Friday': '19%', 'Saturday': '19%', 'Tuesday': '27%'}
As told, a dict is not ordered, but you can order before to convert to dict.
daydate=['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']
percentday=['0%', '17%', '27%', '11%', '7%', '19%', '19%']
Sort by day, just rotate the zip:
dayzip = list(zip(daydate, percentday))
dayzip = dayzip[1-len(dayzip):] + dayzip[:1-len(dayzip)]
daydict = dict(dayzip)
#=> {'Sunday': '0%', 'Monday': '17%', 'Tuesday': '27%', 'Wednesday': '11%', 'Thursday': '7%', 'Friday': '19%', 'Saturday': '19%'}
To sort by percentage:
dayzip = list(zip(daydate, percentday))
dayzip.sort(key=lambda x: float(x[1][:-1]) )
daydict = dict(dayzip)
#=> [('Sunday', '0%'), ('Monday', '17%'), ('Tuesday', '27%'), ('Wednesday', '11%'), ('Thursday', '7%'), ('Friday', '19%'), ('Saturday', '19%')]
#=> {'Sunday': '0%', 'Thursday': '7%', 'Wednesday': '11%', 'Monday': '17%', 'Friday': '19%', 'Saturday': '19%', 'Tuesday': '27%'}
edited Nov 19 at 12:52
answered Nov 19 at 12:45


iGian
2,5142620
2,5142620
But OP wants to sort by day, not by value.
– jpp
Nov 19 at 12:46
Oops! In case, rotate stackoverflow.com/questions/9457832/python-list-rotation. I''l edit.
– iGian
Nov 19 at 12:48
add a comment |
But OP wants to sort by day, not by value.
– jpp
Nov 19 at 12:46
Oops! In case, rotate stackoverflow.com/questions/9457832/python-list-rotation. I''l edit.
– iGian
Nov 19 at 12:48
But OP wants to sort by day, not by value.
– jpp
Nov 19 at 12:46
But OP wants to sort by day, not by value.
– jpp
Nov 19 at 12:46
Oops! In case, rotate stackoverflow.com/questions/9457832/python-list-rotation. I''l edit.
– iGian
Nov 19 at 12:48
Oops! In case, rotate stackoverflow.com/questions/9457832/python-list-rotation. I''l edit.
– iGian
Nov 19 at 12:48
add a comment |
up vote
0
down vote
You can try this:
from collections import OrderedDict
daydic={'Friday': '19%', 'Wednesday': '11%', 'Monday': '17%', 'Thursday': '7%', 'Saturday': '19%', 'Sunday': '0%', 'Tuesday': '27%'}
weeks=['Sunday','Monday','Tuesday','Wednesday','Thursday','Friday','Saturday']
print(OrderedDict(sorted(daydic.items(),key =lambda x:weeks.index(x[0]))))
This will output as:
OrderedDict([('Sunday', '0%'), ('Monday', '17%'), ('Tuesday', '27%'), ('Wednesday', '11%'),
('Thursday', '7%'), ('Friday', '19%'), ('Saturday', '19%')])
add a comment |
up vote
0
down vote
You can try this:
from collections import OrderedDict
daydic={'Friday': '19%', 'Wednesday': '11%', 'Monday': '17%', 'Thursday': '7%', 'Saturday': '19%', 'Sunday': '0%', 'Tuesday': '27%'}
weeks=['Sunday','Monday','Tuesday','Wednesday','Thursday','Friday','Saturday']
print(OrderedDict(sorted(daydic.items(),key =lambda x:weeks.index(x[0]))))
This will output as:
OrderedDict([('Sunday', '0%'), ('Monday', '17%'), ('Tuesday', '27%'), ('Wednesday', '11%'),
('Thursday', '7%'), ('Friday', '19%'), ('Saturday', '19%')])
add a comment |
up vote
0
down vote
up vote
0
down vote
You can try this:
from collections import OrderedDict
daydic={'Friday': '19%', 'Wednesday': '11%', 'Monday': '17%', 'Thursday': '7%', 'Saturday': '19%', 'Sunday': '0%', 'Tuesday': '27%'}
weeks=['Sunday','Monday','Tuesday','Wednesday','Thursday','Friday','Saturday']
print(OrderedDict(sorted(daydic.items(),key =lambda x:weeks.index(x[0]))))
This will output as:
OrderedDict([('Sunday', '0%'), ('Monday', '17%'), ('Tuesday', '27%'), ('Wednesday', '11%'),
('Thursday', '7%'), ('Friday', '19%'), ('Saturday', '19%')])
You can try this:
from collections import OrderedDict
daydic={'Friday': '19%', 'Wednesday': '11%', 'Monday': '17%', 'Thursday': '7%', 'Saturday': '19%', 'Sunday': '0%', 'Tuesday': '27%'}
weeks=['Sunday','Monday','Tuesday','Wednesday','Thursday','Friday','Saturday']
print(OrderedDict(sorted(daydic.items(),key =lambda x:weeks.index(x[0]))))
This will output as:
OrderedDict([('Sunday', '0%'), ('Monday', '17%'), ('Tuesday', '27%'), ('Wednesday', '11%'),
('Thursday', '7%'), ('Friday', '19%'), ('Saturday', '19%')])
answered Nov 19 at 12:53
Somya Avasthi
164
164
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53374820%2fhow-to-order-a-dictionary-based-on-key-by-daywise-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MSlZU39,FMS78H8zbLcQDCXMINyzUHASOg4uUzA,eJiYmrusF7hn3
3
Dictionaries don't have a sorting. You can sort it when you print, by sorting the keys
– planetmaker
Nov 19 at 12:39
1
@TomdeGeus: i want to sort by daywise,i.e., week day wise. Anyway thanks
– vtustudent
Nov 19 at 12:41