Validating json payload against swagger file - json-schema-validator
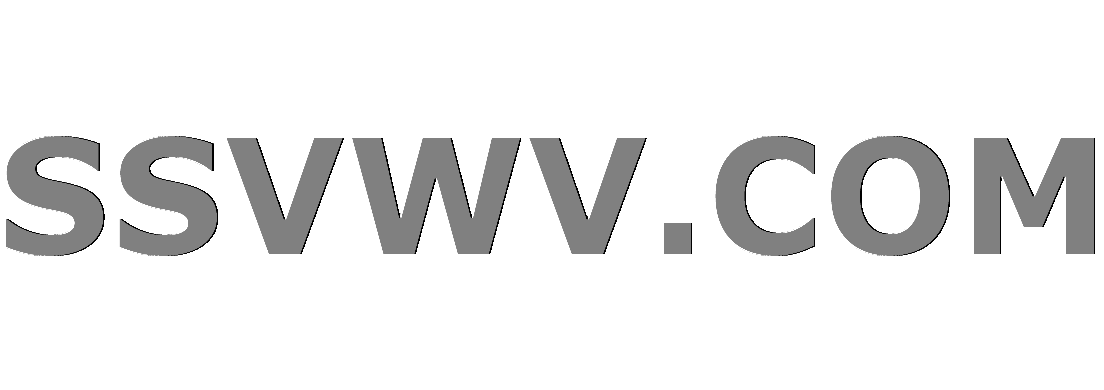
Multi tool use
up vote
0
down vote
favorite
I am trying to validate a json payload against a swagger file that contains the service agreement. I am using the json-schema-validator(2.1.7) library to achieve this, but at the moment it's not validating against the specified patterns or min/max length.
Java Code:
public void validateJsonData(final String jsonData) throws IOException, ProcessingException {
ClassLoader classLoader = getClass().getClassLoader();
File jsonSchemaFile = new File (classLoader.getResource("coachingStatusUpdate.json").getFile());
String jsonSchema = new String(Files.readAllBytes(jsonSchemaFile.toPath()));
final JsonNode dataNode = JsonLoader.fromString(jsonData);
final JsonNode schemaNode = JsonLoader.fromString(jsonSchema);
final JsonSchemaFactory factory = JsonSchemaFactory.byDefault();
JsonValidator jsonValidator = factory.getValidator();
ProcessingReport report = jsonValidator.validate(schemaNode, dataNode);
System.out.println(report);
if (!report.toString().contains("success")) {
throw new ProcessingException (
report.toString());
}
}
Message I am sending through
{
"coachingStatus": "LEAD",
"coachingStatusReason": "ABUSIVE",
"correlationID": -1,
"effectiveDate": "2018-10-30",
"externalEntityIdentifier": "string" }
The swagger definition:
{
"swagger": "2.0",
"info": {
"version": "1.0.0",
"title": "Test",
"termsOfService": "http://www.test.co.za",
"license": {
"name": "Test"
}
},
"host": "localhost:9001",
"basePath": "/test/services",
"tags": [
{
"name": "status-update-controller",
"description": "Submission of member status updates"
}
],
"paths": {
"/statusUpdate": {
"put": {
"tags": [
"status-update-controller"
],
"summary": "statusUpdate",
"operationId": "statusUpdateUsingPUT",
"consumes": [
"application/json;charset=UTF-8"
],
"produces": [
"application/json;charset=UTF-8"
],
"parameters": [
{
"in": "body",
"name": "statusUpdateRequest",
"description": "statusUpdateRequest",
"required": true,
"schema": {
"$ref": "#/definitions/statusUpdateRequest"
}
}
],
"responses": {
"200": {
"description": "Received member status update",
"schema": {
"$ref": "#/definitions/ResponseEntity"
}
},
"400": {
"description": "Bad Request"
},
"401": {
"description": "Unauthorized"
},
"408": {
"description": "Request Timeout"
},
"500": {
"description": "Generic Error"
},
"502": {
"description": "Bad Gateway"
},
"503": {
"description": "Service Unavailable"
}
}
}
}
},
"definitions": {
"statusUpdateRequest": {
"type": "object",
"required": [
"coachingStatus",
"correlationID",
"effectiveDate",
"externalEntityIdentifier"
],
"properties": {
"coachingStatus": {
"type": "string",
"description": "Status of patient enrollment returned by Guidepost",
"enum": [
"LEAD",
"PROSPECT",
"ACTIVE_NEW",
"ACTIVE",
"MAINTENANCE",
"SUSPENDED",
"DECEASED",
"TERMINATED"
]
},
"coachingStatusReason": {
"type": "string",
"description": "Status reason of patient enrollment returned by Guidepost",
"enum": [
"ABUSIVE",
"BAD_LEAD",
"CHANGED_DOCTOR",
"DOCTOR_COMPLAINT",
"DOCTOR_WITHDREW",
"GRADUATED",
"NON_CONTACTABLE",
"NON_PARTICIPATION",
"NON_PAYMENT",
"OFF_LABEL_MEDICATION_USAGE",
"PATIENT_WITHDREW",
"MEDICAL_DISQUALIFICATION",
"PREGNANT",
"EXISTING"
]
},
"correlationID": {
"type": "integer",
"format": "int32",
"description": "Unique reference generated to uniquely identify a transaction",
"minimum": 1,
"maximum": 9999999
},
"effectiveDate": {
"type": "string",
"format": "date",
"description": "Date of when the member status update was performed"
},
"externalEntityIdentifier": {
"type": "string",
"description": "Unique reference to represent the entity",
"minLength": 1,
"maxLength": 100
}
}
},
"ResponseEntity": {
"type": "object",
"properties": {
"body": {
"type": "object"
},
"statusCode": {
"type": "string",
"enum": [
"100",
"101",
"102",
"103",
"200",
"201",
"202",
"203",
"204",
"205",
"206",
"207",
"208",
"226",
"300",
"301",
"302",
"303",
"304",
"305",
"307",
"308",
"400",
"401",
"402",
"403",
"404",
"405",
"406",
"407",
"408",
"409",
"410",
"411",
"412",
"413",
"414",
"415",
"416",
"417",
"418",
"419",
"420",
"421",
"422",
"423",
"424",
"426",
"428",
"429",
"431",
"451",
"500",
"501",
"502",
"503",
"504",
"505",
"506",
"507",
"508",
"509",
"510",
"511"
]
},
"statusCodeValue": {
"type": "integer",
"format": "int32"
}
}
}
}
}
As you can see I am sending through a correlationID of -1, which should fail validation, but at the moment is's returning as successful:
com.github.fge.jsonschema.report.ListProcessingReport: success
java json swagger jsonschema json-schema-validator
add a comment |
up vote
0
down vote
favorite
I am trying to validate a json payload against a swagger file that contains the service agreement. I am using the json-schema-validator(2.1.7) library to achieve this, but at the moment it's not validating against the specified patterns or min/max length.
Java Code:
public void validateJsonData(final String jsonData) throws IOException, ProcessingException {
ClassLoader classLoader = getClass().getClassLoader();
File jsonSchemaFile = new File (classLoader.getResource("coachingStatusUpdate.json").getFile());
String jsonSchema = new String(Files.readAllBytes(jsonSchemaFile.toPath()));
final JsonNode dataNode = JsonLoader.fromString(jsonData);
final JsonNode schemaNode = JsonLoader.fromString(jsonSchema);
final JsonSchemaFactory factory = JsonSchemaFactory.byDefault();
JsonValidator jsonValidator = factory.getValidator();
ProcessingReport report = jsonValidator.validate(schemaNode, dataNode);
System.out.println(report);
if (!report.toString().contains("success")) {
throw new ProcessingException (
report.toString());
}
}
Message I am sending through
{
"coachingStatus": "LEAD",
"coachingStatusReason": "ABUSIVE",
"correlationID": -1,
"effectiveDate": "2018-10-30",
"externalEntityIdentifier": "string" }
The swagger definition:
{
"swagger": "2.0",
"info": {
"version": "1.0.0",
"title": "Test",
"termsOfService": "http://www.test.co.za",
"license": {
"name": "Test"
}
},
"host": "localhost:9001",
"basePath": "/test/services",
"tags": [
{
"name": "status-update-controller",
"description": "Submission of member status updates"
}
],
"paths": {
"/statusUpdate": {
"put": {
"tags": [
"status-update-controller"
],
"summary": "statusUpdate",
"operationId": "statusUpdateUsingPUT",
"consumes": [
"application/json;charset=UTF-8"
],
"produces": [
"application/json;charset=UTF-8"
],
"parameters": [
{
"in": "body",
"name": "statusUpdateRequest",
"description": "statusUpdateRequest",
"required": true,
"schema": {
"$ref": "#/definitions/statusUpdateRequest"
}
}
],
"responses": {
"200": {
"description": "Received member status update",
"schema": {
"$ref": "#/definitions/ResponseEntity"
}
},
"400": {
"description": "Bad Request"
},
"401": {
"description": "Unauthorized"
},
"408": {
"description": "Request Timeout"
},
"500": {
"description": "Generic Error"
},
"502": {
"description": "Bad Gateway"
},
"503": {
"description": "Service Unavailable"
}
}
}
}
},
"definitions": {
"statusUpdateRequest": {
"type": "object",
"required": [
"coachingStatus",
"correlationID",
"effectiveDate",
"externalEntityIdentifier"
],
"properties": {
"coachingStatus": {
"type": "string",
"description": "Status of patient enrollment returned by Guidepost",
"enum": [
"LEAD",
"PROSPECT",
"ACTIVE_NEW",
"ACTIVE",
"MAINTENANCE",
"SUSPENDED",
"DECEASED",
"TERMINATED"
]
},
"coachingStatusReason": {
"type": "string",
"description": "Status reason of patient enrollment returned by Guidepost",
"enum": [
"ABUSIVE",
"BAD_LEAD",
"CHANGED_DOCTOR",
"DOCTOR_COMPLAINT",
"DOCTOR_WITHDREW",
"GRADUATED",
"NON_CONTACTABLE",
"NON_PARTICIPATION",
"NON_PAYMENT",
"OFF_LABEL_MEDICATION_USAGE",
"PATIENT_WITHDREW",
"MEDICAL_DISQUALIFICATION",
"PREGNANT",
"EXISTING"
]
},
"correlationID": {
"type": "integer",
"format": "int32",
"description": "Unique reference generated to uniquely identify a transaction",
"minimum": 1,
"maximum": 9999999
},
"effectiveDate": {
"type": "string",
"format": "date",
"description": "Date of when the member status update was performed"
},
"externalEntityIdentifier": {
"type": "string",
"description": "Unique reference to represent the entity",
"minLength": 1,
"maxLength": 100
}
}
},
"ResponseEntity": {
"type": "object",
"properties": {
"body": {
"type": "object"
},
"statusCode": {
"type": "string",
"enum": [
"100",
"101",
"102",
"103",
"200",
"201",
"202",
"203",
"204",
"205",
"206",
"207",
"208",
"226",
"300",
"301",
"302",
"303",
"304",
"305",
"307",
"308",
"400",
"401",
"402",
"403",
"404",
"405",
"406",
"407",
"408",
"409",
"410",
"411",
"412",
"413",
"414",
"415",
"416",
"417",
"418",
"419",
"420",
"421",
"422",
"423",
"424",
"426",
"428",
"429",
"431",
"451",
"500",
"501",
"502",
"503",
"504",
"505",
"506",
"507",
"508",
"509",
"510",
"511"
]
},
"statusCodeValue": {
"type": "integer",
"format": "int32"
}
}
}
}
}
As you can see I am sending through a correlationID of -1, which should fail validation, but at the moment is's returning as successful:
com.github.fge.jsonschema.report.ListProcessingReport: success
java json swagger jsonschema json-schema-validator
can you post the whole schema?
– slimane
Nov 19 at 12:53
@slimane I added the whole schema
– Hendrien
Nov 19 at 13:00
Are you sure this json-schema-validator supports OpenAPI/Swagger schemas? OpenAPI format differs from vanilla JSON Schema.
– Helen
Nov 19 at 13:49
@Helen I am unsure, I was unable to find any other components that can validate against schema files
– Hendrien
Nov 19 at 14:44
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am trying to validate a json payload against a swagger file that contains the service agreement. I am using the json-schema-validator(2.1.7) library to achieve this, but at the moment it's not validating against the specified patterns or min/max length.
Java Code:
public void validateJsonData(final String jsonData) throws IOException, ProcessingException {
ClassLoader classLoader = getClass().getClassLoader();
File jsonSchemaFile = new File (classLoader.getResource("coachingStatusUpdate.json").getFile());
String jsonSchema = new String(Files.readAllBytes(jsonSchemaFile.toPath()));
final JsonNode dataNode = JsonLoader.fromString(jsonData);
final JsonNode schemaNode = JsonLoader.fromString(jsonSchema);
final JsonSchemaFactory factory = JsonSchemaFactory.byDefault();
JsonValidator jsonValidator = factory.getValidator();
ProcessingReport report = jsonValidator.validate(schemaNode, dataNode);
System.out.println(report);
if (!report.toString().contains("success")) {
throw new ProcessingException (
report.toString());
}
}
Message I am sending through
{
"coachingStatus": "LEAD",
"coachingStatusReason": "ABUSIVE",
"correlationID": -1,
"effectiveDate": "2018-10-30",
"externalEntityIdentifier": "string" }
The swagger definition:
{
"swagger": "2.0",
"info": {
"version": "1.0.0",
"title": "Test",
"termsOfService": "http://www.test.co.za",
"license": {
"name": "Test"
}
},
"host": "localhost:9001",
"basePath": "/test/services",
"tags": [
{
"name": "status-update-controller",
"description": "Submission of member status updates"
}
],
"paths": {
"/statusUpdate": {
"put": {
"tags": [
"status-update-controller"
],
"summary": "statusUpdate",
"operationId": "statusUpdateUsingPUT",
"consumes": [
"application/json;charset=UTF-8"
],
"produces": [
"application/json;charset=UTF-8"
],
"parameters": [
{
"in": "body",
"name": "statusUpdateRequest",
"description": "statusUpdateRequest",
"required": true,
"schema": {
"$ref": "#/definitions/statusUpdateRequest"
}
}
],
"responses": {
"200": {
"description": "Received member status update",
"schema": {
"$ref": "#/definitions/ResponseEntity"
}
},
"400": {
"description": "Bad Request"
},
"401": {
"description": "Unauthorized"
},
"408": {
"description": "Request Timeout"
},
"500": {
"description": "Generic Error"
},
"502": {
"description": "Bad Gateway"
},
"503": {
"description": "Service Unavailable"
}
}
}
}
},
"definitions": {
"statusUpdateRequest": {
"type": "object",
"required": [
"coachingStatus",
"correlationID",
"effectiveDate",
"externalEntityIdentifier"
],
"properties": {
"coachingStatus": {
"type": "string",
"description": "Status of patient enrollment returned by Guidepost",
"enum": [
"LEAD",
"PROSPECT",
"ACTIVE_NEW",
"ACTIVE",
"MAINTENANCE",
"SUSPENDED",
"DECEASED",
"TERMINATED"
]
},
"coachingStatusReason": {
"type": "string",
"description": "Status reason of patient enrollment returned by Guidepost",
"enum": [
"ABUSIVE",
"BAD_LEAD",
"CHANGED_DOCTOR",
"DOCTOR_COMPLAINT",
"DOCTOR_WITHDREW",
"GRADUATED",
"NON_CONTACTABLE",
"NON_PARTICIPATION",
"NON_PAYMENT",
"OFF_LABEL_MEDICATION_USAGE",
"PATIENT_WITHDREW",
"MEDICAL_DISQUALIFICATION",
"PREGNANT",
"EXISTING"
]
},
"correlationID": {
"type": "integer",
"format": "int32",
"description": "Unique reference generated to uniquely identify a transaction",
"minimum": 1,
"maximum": 9999999
},
"effectiveDate": {
"type": "string",
"format": "date",
"description": "Date of when the member status update was performed"
},
"externalEntityIdentifier": {
"type": "string",
"description": "Unique reference to represent the entity",
"minLength": 1,
"maxLength": 100
}
}
},
"ResponseEntity": {
"type": "object",
"properties": {
"body": {
"type": "object"
},
"statusCode": {
"type": "string",
"enum": [
"100",
"101",
"102",
"103",
"200",
"201",
"202",
"203",
"204",
"205",
"206",
"207",
"208",
"226",
"300",
"301",
"302",
"303",
"304",
"305",
"307",
"308",
"400",
"401",
"402",
"403",
"404",
"405",
"406",
"407",
"408",
"409",
"410",
"411",
"412",
"413",
"414",
"415",
"416",
"417",
"418",
"419",
"420",
"421",
"422",
"423",
"424",
"426",
"428",
"429",
"431",
"451",
"500",
"501",
"502",
"503",
"504",
"505",
"506",
"507",
"508",
"509",
"510",
"511"
]
},
"statusCodeValue": {
"type": "integer",
"format": "int32"
}
}
}
}
}
As you can see I am sending through a correlationID of -1, which should fail validation, but at the moment is's returning as successful:
com.github.fge.jsonschema.report.ListProcessingReport: success
java json swagger jsonschema json-schema-validator
I am trying to validate a json payload against a swagger file that contains the service agreement. I am using the json-schema-validator(2.1.7) library to achieve this, but at the moment it's not validating against the specified patterns or min/max length.
Java Code:
public void validateJsonData(final String jsonData) throws IOException, ProcessingException {
ClassLoader classLoader = getClass().getClassLoader();
File jsonSchemaFile = new File (classLoader.getResource("coachingStatusUpdate.json").getFile());
String jsonSchema = new String(Files.readAllBytes(jsonSchemaFile.toPath()));
final JsonNode dataNode = JsonLoader.fromString(jsonData);
final JsonNode schemaNode = JsonLoader.fromString(jsonSchema);
final JsonSchemaFactory factory = JsonSchemaFactory.byDefault();
JsonValidator jsonValidator = factory.getValidator();
ProcessingReport report = jsonValidator.validate(schemaNode, dataNode);
System.out.println(report);
if (!report.toString().contains("success")) {
throw new ProcessingException (
report.toString());
}
}
Message I am sending through
{
"coachingStatus": "LEAD",
"coachingStatusReason": "ABUSIVE",
"correlationID": -1,
"effectiveDate": "2018-10-30",
"externalEntityIdentifier": "string" }
The swagger definition:
{
"swagger": "2.0",
"info": {
"version": "1.0.0",
"title": "Test",
"termsOfService": "http://www.test.co.za",
"license": {
"name": "Test"
}
},
"host": "localhost:9001",
"basePath": "/test/services",
"tags": [
{
"name": "status-update-controller",
"description": "Submission of member status updates"
}
],
"paths": {
"/statusUpdate": {
"put": {
"tags": [
"status-update-controller"
],
"summary": "statusUpdate",
"operationId": "statusUpdateUsingPUT",
"consumes": [
"application/json;charset=UTF-8"
],
"produces": [
"application/json;charset=UTF-8"
],
"parameters": [
{
"in": "body",
"name": "statusUpdateRequest",
"description": "statusUpdateRequest",
"required": true,
"schema": {
"$ref": "#/definitions/statusUpdateRequest"
}
}
],
"responses": {
"200": {
"description": "Received member status update",
"schema": {
"$ref": "#/definitions/ResponseEntity"
}
},
"400": {
"description": "Bad Request"
},
"401": {
"description": "Unauthorized"
},
"408": {
"description": "Request Timeout"
},
"500": {
"description": "Generic Error"
},
"502": {
"description": "Bad Gateway"
},
"503": {
"description": "Service Unavailable"
}
}
}
}
},
"definitions": {
"statusUpdateRequest": {
"type": "object",
"required": [
"coachingStatus",
"correlationID",
"effectiveDate",
"externalEntityIdentifier"
],
"properties": {
"coachingStatus": {
"type": "string",
"description": "Status of patient enrollment returned by Guidepost",
"enum": [
"LEAD",
"PROSPECT",
"ACTIVE_NEW",
"ACTIVE",
"MAINTENANCE",
"SUSPENDED",
"DECEASED",
"TERMINATED"
]
},
"coachingStatusReason": {
"type": "string",
"description": "Status reason of patient enrollment returned by Guidepost",
"enum": [
"ABUSIVE",
"BAD_LEAD",
"CHANGED_DOCTOR",
"DOCTOR_COMPLAINT",
"DOCTOR_WITHDREW",
"GRADUATED",
"NON_CONTACTABLE",
"NON_PARTICIPATION",
"NON_PAYMENT",
"OFF_LABEL_MEDICATION_USAGE",
"PATIENT_WITHDREW",
"MEDICAL_DISQUALIFICATION",
"PREGNANT",
"EXISTING"
]
},
"correlationID": {
"type": "integer",
"format": "int32",
"description": "Unique reference generated to uniquely identify a transaction",
"minimum": 1,
"maximum": 9999999
},
"effectiveDate": {
"type": "string",
"format": "date",
"description": "Date of when the member status update was performed"
},
"externalEntityIdentifier": {
"type": "string",
"description": "Unique reference to represent the entity",
"minLength": 1,
"maxLength": 100
}
}
},
"ResponseEntity": {
"type": "object",
"properties": {
"body": {
"type": "object"
},
"statusCode": {
"type": "string",
"enum": [
"100",
"101",
"102",
"103",
"200",
"201",
"202",
"203",
"204",
"205",
"206",
"207",
"208",
"226",
"300",
"301",
"302",
"303",
"304",
"305",
"307",
"308",
"400",
"401",
"402",
"403",
"404",
"405",
"406",
"407",
"408",
"409",
"410",
"411",
"412",
"413",
"414",
"415",
"416",
"417",
"418",
"419",
"420",
"421",
"422",
"423",
"424",
"426",
"428",
"429",
"431",
"451",
"500",
"501",
"502",
"503",
"504",
"505",
"506",
"507",
"508",
"509",
"510",
"511"
]
},
"statusCodeValue": {
"type": "integer",
"format": "int32"
}
}
}
}
}
As you can see I am sending through a correlationID of -1, which should fail validation, but at the moment is's returning as successful:
com.github.fge.jsonschema.report.ListProcessingReport: success
java json swagger jsonschema json-schema-validator
java json swagger jsonschema json-schema-validator
edited Nov 19 at 13:00
asked Nov 19 at 12:36
Hendrien
31110
31110
can you post the whole schema?
– slimane
Nov 19 at 12:53
@slimane I added the whole schema
– Hendrien
Nov 19 at 13:00
Are you sure this json-schema-validator supports OpenAPI/Swagger schemas? OpenAPI format differs from vanilla JSON Schema.
– Helen
Nov 19 at 13:49
@Helen I am unsure, I was unable to find any other components that can validate against schema files
– Hendrien
Nov 19 at 14:44
add a comment |
can you post the whole schema?
– slimane
Nov 19 at 12:53
@slimane I added the whole schema
– Hendrien
Nov 19 at 13:00
Are you sure this json-schema-validator supports OpenAPI/Swagger schemas? OpenAPI format differs from vanilla JSON Schema.
– Helen
Nov 19 at 13:49
@Helen I am unsure, I was unable to find any other components that can validate against schema files
– Hendrien
Nov 19 at 14:44
can you post the whole schema?
– slimane
Nov 19 at 12:53
can you post the whole schema?
– slimane
Nov 19 at 12:53
@slimane I added the whole schema
– Hendrien
Nov 19 at 13:00
@slimane I added the whole schema
– Hendrien
Nov 19 at 13:00
Are you sure this json-schema-validator supports OpenAPI/Swagger schemas? OpenAPI format differs from vanilla JSON Schema.
– Helen
Nov 19 at 13:49
Are you sure this json-schema-validator supports OpenAPI/Swagger schemas? OpenAPI format differs from vanilla JSON Schema.
– Helen
Nov 19 at 13:49
@Helen I am unsure, I was unable to find any other components that can validate against schema files
– Hendrien
Nov 19 at 14:44
@Helen I am unsure, I was unable to find any other components that can validate against schema files
– Hendrien
Nov 19 at 14:44
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
json-schema-validator seems to work with pure JSON Schema only. OpenAPI Specification uses an extended subset of JSON Schema, so the schema format is different. You need a library that can validate specifically against OpenAPI/Swagger definitions. Since you use Java, try one of the following:
SchemaValidator class from the Swagger Inflector library- Atlassian's swagger-request-validator
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
json-schema-validator seems to work with pure JSON Schema only. OpenAPI Specification uses an extended subset of JSON Schema, so the schema format is different. You need a library that can validate specifically against OpenAPI/Swagger definitions. Since you use Java, try one of the following:
SchemaValidator class from the Swagger Inflector library- Atlassian's swagger-request-validator
add a comment |
up vote
1
down vote
json-schema-validator seems to work with pure JSON Schema only. OpenAPI Specification uses an extended subset of JSON Schema, so the schema format is different. You need a library that can validate specifically against OpenAPI/Swagger definitions. Since you use Java, try one of the following:
SchemaValidator class from the Swagger Inflector library- Atlassian's swagger-request-validator
add a comment |
up vote
1
down vote
up vote
1
down vote
json-schema-validator seems to work with pure JSON Schema only. OpenAPI Specification uses an extended subset of JSON Schema, so the schema format is different. You need a library that can validate specifically against OpenAPI/Swagger definitions. Since you use Java, try one of the following:
SchemaValidator class from the Swagger Inflector library- Atlassian's swagger-request-validator
json-schema-validator seems to work with pure JSON Schema only. OpenAPI Specification uses an extended subset of JSON Schema, so the schema format is different. You need a library that can validate specifically against OpenAPI/Swagger definitions. Since you use Java, try one of the following:
SchemaValidator class from the Swagger Inflector library- Atlassian's swagger-request-validator
answered Nov 19 at 15:22


Helen
31.7k471126
31.7k471126
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53374810%2fvalidating-json-payload-against-swagger-file-json-schema-validator%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
K9fjr gg 1QP8hpmPE hZ59gY3d,v,iDCw PCq TZZ9FdGc6bEhI3eA
can you post the whole schema?
– slimane
Nov 19 at 12:53
@slimane I added the whole schema
– Hendrien
Nov 19 at 13:00
Are you sure this json-schema-validator supports OpenAPI/Swagger schemas? OpenAPI format differs from vanilla JSON Schema.
– Helen
Nov 19 at 13:49
@Helen I am unsure, I was unable to find any other components that can validate against schema files
– Hendrien
Nov 19 at 14:44