Invalid X-CSRF-Token request header using fetchAPI and Drupal as decoupled back-end
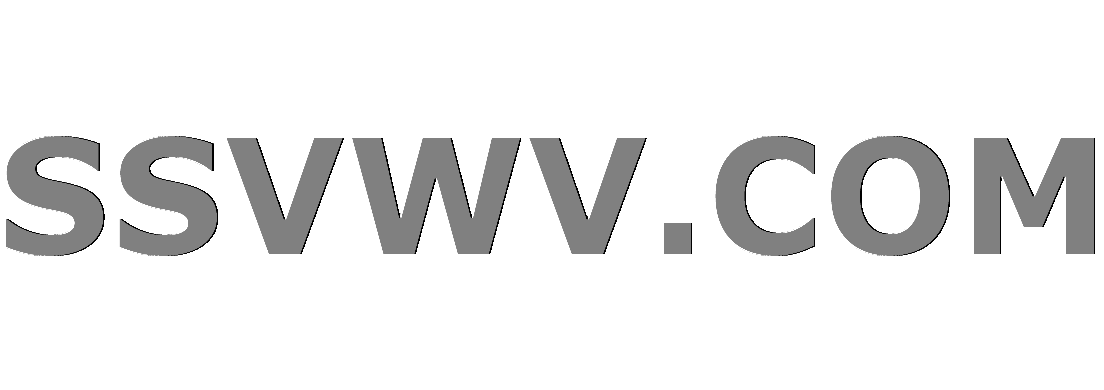
Multi tool use
up vote
0
down vote
favorite
First of all, I am a starting developer. I apologize for making possible misconceptions.
I am trying to make a Reactjs application which communicates with a decoupled Drupal 8 back-end by using fetchAPI.
I want to make an authentication system by using session cookies. Getting the cookie from the Drupal site, and setting it in the browser works fine. I can include the cookie in HTTP requests. However, in addition to the cookie, Drupal also wants a 'x-csrf-token' to be included in the HTTP request header. This token can be acquired with a HTTP GET request to the Drupal site. So when a user logs in, I request both the cookie and the x-csrf-token, and I store the token in React's application state using Redux.
Now on the POST request I am trying to make, I get the token from the Redux store and include it in the HTTP request using the 'X-CSRF-Token' header. This gives me a 403 error with the following response: 'X-CSRF-Token request header is invalid'. The exact same request in combination with getting the cookie and token works fine in postman, so I do not know why I am getting this error in the browser.
I tried multiple browsers and different formats for the token, but I still can not get this to work.
(Note: I am using RESTful webservices from Drupal core with cookie authentication enabled.)
Getting the X-CSRF-Token:
export function getCsrfToken() {
return function(dispatch) {
fetch("http://drupalsite.local/rest/session/token", {
method: "GET"
})
.then(res => res.text())
.catch(err => {
console.log(err)
})
.then(token => {
console.log(token);
dispatch({
type: FETCH_CSRF_TOKEN,
payload: token
});
})
.catch(err => {
console.log(err);
});
};
}
The POST request:
export function post(name, csrfToken) {
const data = JSON.stringify({
title: [
{
value: name
}
],
type: [
{
target_id: "test"
}
]
});
return function(dispatch) {
fetch("http://drupalsite.local/node", {
method: "POST",
credentials: "include",
headers: new Headers({
"Content-Type": "application/json",
Accept: "application/json",
"X-CSRF-TOKEN": csrfToken
}),
body: data
})
.then(res => {
dispatch({
type: POST_DATA_CORE_REST,
payload: res
});
})
.catch(err => {
console.log(err);
});
};
}
HTTP headers
reactjs drupal httprequest fetch-api csrf-protection
New contributor
L. Smans is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
First of all, I am a starting developer. I apologize for making possible misconceptions.
I am trying to make a Reactjs application which communicates with a decoupled Drupal 8 back-end by using fetchAPI.
I want to make an authentication system by using session cookies. Getting the cookie from the Drupal site, and setting it in the browser works fine. I can include the cookie in HTTP requests. However, in addition to the cookie, Drupal also wants a 'x-csrf-token' to be included in the HTTP request header. This token can be acquired with a HTTP GET request to the Drupal site. So when a user logs in, I request both the cookie and the x-csrf-token, and I store the token in React's application state using Redux.
Now on the POST request I am trying to make, I get the token from the Redux store and include it in the HTTP request using the 'X-CSRF-Token' header. This gives me a 403 error with the following response: 'X-CSRF-Token request header is invalid'. The exact same request in combination with getting the cookie and token works fine in postman, so I do not know why I am getting this error in the browser.
I tried multiple browsers and different formats for the token, but I still can not get this to work.
(Note: I am using RESTful webservices from Drupal core with cookie authentication enabled.)
Getting the X-CSRF-Token:
export function getCsrfToken() {
return function(dispatch) {
fetch("http://drupalsite.local/rest/session/token", {
method: "GET"
})
.then(res => res.text())
.catch(err => {
console.log(err)
})
.then(token => {
console.log(token);
dispatch({
type: FETCH_CSRF_TOKEN,
payload: token
});
})
.catch(err => {
console.log(err);
});
};
}
The POST request:
export function post(name, csrfToken) {
const data = JSON.stringify({
title: [
{
value: name
}
],
type: [
{
target_id: "test"
}
]
});
return function(dispatch) {
fetch("http://drupalsite.local/node", {
method: "POST",
credentials: "include",
headers: new Headers({
"Content-Type": "application/json",
Accept: "application/json",
"X-CSRF-TOKEN": csrfToken
}),
body: data
})
.then(res => {
dispatch({
type: POST_DATA_CORE_REST,
payload: res
});
})
.catch(err => {
console.log(err);
});
};
}
HTTP headers
reactjs drupal httprequest fetch-api csrf-protection
New contributor
L. Smans is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
drupal.org/project/drupal/issues/2976542 seems possibly relevant
– sideshowbarker
Nov 19 at 23:13
I do not think it is relevant for my question, because I am sure I have the right token. Thanks for helping though.
– L. Smans
Nov 21 at 8:24
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
First of all, I am a starting developer. I apologize for making possible misconceptions.
I am trying to make a Reactjs application which communicates with a decoupled Drupal 8 back-end by using fetchAPI.
I want to make an authentication system by using session cookies. Getting the cookie from the Drupal site, and setting it in the browser works fine. I can include the cookie in HTTP requests. However, in addition to the cookie, Drupal also wants a 'x-csrf-token' to be included in the HTTP request header. This token can be acquired with a HTTP GET request to the Drupal site. So when a user logs in, I request both the cookie and the x-csrf-token, and I store the token in React's application state using Redux.
Now on the POST request I am trying to make, I get the token from the Redux store and include it in the HTTP request using the 'X-CSRF-Token' header. This gives me a 403 error with the following response: 'X-CSRF-Token request header is invalid'. The exact same request in combination with getting the cookie and token works fine in postman, so I do not know why I am getting this error in the browser.
I tried multiple browsers and different formats for the token, but I still can not get this to work.
(Note: I am using RESTful webservices from Drupal core with cookie authentication enabled.)
Getting the X-CSRF-Token:
export function getCsrfToken() {
return function(dispatch) {
fetch("http://drupalsite.local/rest/session/token", {
method: "GET"
})
.then(res => res.text())
.catch(err => {
console.log(err)
})
.then(token => {
console.log(token);
dispatch({
type: FETCH_CSRF_TOKEN,
payload: token
});
})
.catch(err => {
console.log(err);
});
};
}
The POST request:
export function post(name, csrfToken) {
const data = JSON.stringify({
title: [
{
value: name
}
],
type: [
{
target_id: "test"
}
]
});
return function(dispatch) {
fetch("http://drupalsite.local/node", {
method: "POST",
credentials: "include",
headers: new Headers({
"Content-Type": "application/json",
Accept: "application/json",
"X-CSRF-TOKEN": csrfToken
}),
body: data
})
.then(res => {
dispatch({
type: POST_DATA_CORE_REST,
payload: res
});
})
.catch(err => {
console.log(err);
});
};
}
HTTP headers
reactjs drupal httprequest fetch-api csrf-protection
New contributor
L. Smans is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
First of all, I am a starting developer. I apologize for making possible misconceptions.
I am trying to make a Reactjs application which communicates with a decoupled Drupal 8 back-end by using fetchAPI.
I want to make an authentication system by using session cookies. Getting the cookie from the Drupal site, and setting it in the browser works fine. I can include the cookie in HTTP requests. However, in addition to the cookie, Drupal also wants a 'x-csrf-token' to be included in the HTTP request header. This token can be acquired with a HTTP GET request to the Drupal site. So when a user logs in, I request both the cookie and the x-csrf-token, and I store the token in React's application state using Redux.
Now on the POST request I am trying to make, I get the token from the Redux store and include it in the HTTP request using the 'X-CSRF-Token' header. This gives me a 403 error with the following response: 'X-CSRF-Token request header is invalid'. The exact same request in combination with getting the cookie and token works fine in postman, so I do not know why I am getting this error in the browser.
I tried multiple browsers and different formats for the token, but I still can not get this to work.
(Note: I am using RESTful webservices from Drupal core with cookie authentication enabled.)
Getting the X-CSRF-Token:
export function getCsrfToken() {
return function(dispatch) {
fetch("http://drupalsite.local/rest/session/token", {
method: "GET"
})
.then(res => res.text())
.catch(err => {
console.log(err)
})
.then(token => {
console.log(token);
dispatch({
type: FETCH_CSRF_TOKEN,
payload: token
});
})
.catch(err => {
console.log(err);
});
};
}
The POST request:
export function post(name, csrfToken) {
const data = JSON.stringify({
title: [
{
value: name
}
],
type: [
{
target_id: "test"
}
]
});
return function(dispatch) {
fetch("http://drupalsite.local/node", {
method: "POST",
credentials: "include",
headers: new Headers({
"Content-Type": "application/json",
Accept: "application/json",
"X-CSRF-TOKEN": csrfToken
}),
body: data
})
.then(res => {
dispatch({
type: POST_DATA_CORE_REST,
payload: res
});
})
.catch(err => {
console.log(err);
});
};
}
HTTP headers
reactjs drupal httprequest fetch-api csrf-protection
reactjs drupal httprequest fetch-api csrf-protection
New contributor
L. Smans is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
L. Smans is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
L. Smans is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Nov 19 at 13:08
L. Smans
11
11
New contributor
L. Smans is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
L. Smans is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
L. Smans is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
drupal.org/project/drupal/issues/2976542 seems possibly relevant
– sideshowbarker
Nov 19 at 23:13
I do not think it is relevant for my question, because I am sure I have the right token. Thanks for helping though.
– L. Smans
Nov 21 at 8:24
add a comment |
drupal.org/project/drupal/issues/2976542 seems possibly relevant
– sideshowbarker
Nov 19 at 23:13
I do not think it is relevant for my question, because I am sure I have the right token. Thanks for helping though.
– L. Smans
Nov 21 at 8:24
drupal.org/project/drupal/issues/2976542 seems possibly relevant
– sideshowbarker
Nov 19 at 23:13
drupal.org/project/drupal/issues/2976542 seems possibly relevant
– sideshowbarker
Nov 19 at 23:13
I do not think it is relevant for my question, because I am sure I have the right token. Thanks for helping though.
– L. Smans
Nov 21 at 8:24
I do not think it is relevant for my question, because I am sure I have the right token. Thanks for helping though.
– L. Smans
Nov 21 at 8:24
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
L. Smans is a new contributor. Be nice, and check out our Code of Conduct.
L. Smans is a new contributor. Be nice, and check out our Code of Conduct.
L. Smans is a new contributor. Be nice, and check out our Code of Conduct.
L. Smans is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53375351%2finvalid-x-csrf-token-request-header-using-fetchapi-and-drupal-as-decoupled-back%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3p5EYGPKVndvp9fdlAxLntr HjPXNR2 bMOD4zcBQtZHp390JULvAL1x2 DEV4Ux,VCBubY 1nR9iGf P4aWYWXpyVqqqljT,S
drupal.org/project/drupal/issues/2976542 seems possibly relevant
– sideshowbarker
Nov 19 at 23:13
I do not think it is relevant for my question, because I am sure I have the right token. Thanks for helping though.
– L. Smans
Nov 21 at 8:24