Javascript : Store JSON data in array
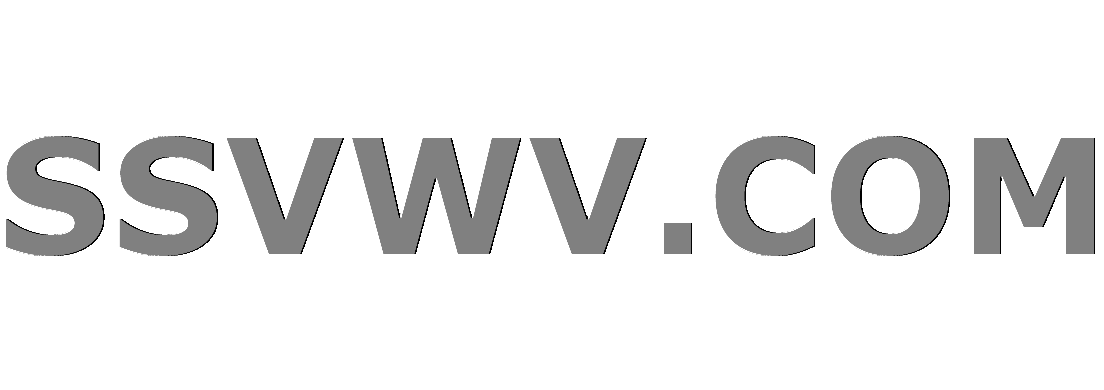
Multi tool use
up vote
1
down vote
favorite
I have JSON like this.
[{
"address": "A-D-1",
"batch": [{
"batch_number": "B-123",
"cost": [{
"cost": "C1"
}]
}]
},
{
"address": "A-85-1",
"batch": [{
"batch_number": "B-6562",
"cost": [{
"cost": "C16464"
}]
}]
},
{
"address": "A-522-1",
"batch": [{
"batch_number": "B-4511",
"cost": [{
"cost": "C8745"
}]
}]
}]
I would like to store my JSON data to array.
let data = JSON.parse('[{"address":"A-D-1","batch":[{"batch_number":"B-123","cost":[{"cost":"C1"}]}]},{"address":"A-85-1","batch":[{"batch_number":"B-6562","cost":[{"cost":"C16464"}]}]},{"address":"A-522-1","batch":[{"batch_number":"B-4511","cost":[{"cost":"C8745"}]}]}]');
for (let i = 0; i < data.length; i++) {
if (data[i].batch !== undefined && data[i].batch !== null && data[i].batch.length !== undefined && data[i].batch.length > 0) {
let batchLength = data[i].batch.length
let newObject = {}
let newArray =
for (let j = 0; j < batchLength; j++) {
if (data[i].batch !== undefined && data[i].batch[j].cost !== null && data[i].batch[j].cost.length !== undefined && data[i].batch[j].cost.length > 0) {
let costLength = data[i].batch[j].cost.length
for (let k = 0; k < costLength; k++) {
newObject.location = data[i].address
newObject.batch.number = data[i].batch[j].batch_number ? data[i].batch[j].batch_number : ''
newObject.cogs = data[i].batch[j].cost[k].cost ? data[i].batch[j].cost[k].cost : ''
newArray.push(newObject)
}
}
}
}
}
I have stored JSON in data
variable.
I have tried below code. but I always get last index as repeatative.
Expected Output :
[
{
"address":"A-D-1",
"batch":{
"batch_number":"B-123"
},
"cost":"C1"
},
{
"address":"A-85-1",
"batch":{
"batch_number":"B-6562"
},
"cost":"C16464"
},
{
"address":"A-522-1",
"batch":{
"batch_number":"B-4511"
},
"cost":"C8745"
}
]
Any help would be great.
Thank You.
javascript json
add a comment |
up vote
1
down vote
favorite
I have JSON like this.
[{
"address": "A-D-1",
"batch": [{
"batch_number": "B-123",
"cost": [{
"cost": "C1"
}]
}]
},
{
"address": "A-85-1",
"batch": [{
"batch_number": "B-6562",
"cost": [{
"cost": "C16464"
}]
}]
},
{
"address": "A-522-1",
"batch": [{
"batch_number": "B-4511",
"cost": [{
"cost": "C8745"
}]
}]
}]
I would like to store my JSON data to array.
let data = JSON.parse('[{"address":"A-D-1","batch":[{"batch_number":"B-123","cost":[{"cost":"C1"}]}]},{"address":"A-85-1","batch":[{"batch_number":"B-6562","cost":[{"cost":"C16464"}]}]},{"address":"A-522-1","batch":[{"batch_number":"B-4511","cost":[{"cost":"C8745"}]}]}]');
for (let i = 0; i < data.length; i++) {
if (data[i].batch !== undefined && data[i].batch !== null && data[i].batch.length !== undefined && data[i].batch.length > 0) {
let batchLength = data[i].batch.length
let newObject = {}
let newArray =
for (let j = 0; j < batchLength; j++) {
if (data[i].batch !== undefined && data[i].batch[j].cost !== null && data[i].batch[j].cost.length !== undefined && data[i].batch[j].cost.length > 0) {
let costLength = data[i].batch[j].cost.length
for (let k = 0; k < costLength; k++) {
newObject.location = data[i].address
newObject.batch.number = data[i].batch[j].batch_number ? data[i].batch[j].batch_number : ''
newObject.cogs = data[i].batch[j].cost[k].cost ? data[i].batch[j].cost[k].cost : ''
newArray.push(newObject)
}
}
}
}
}
I have stored JSON in data
variable.
I have tried below code. but I always get last index as repeatative.
Expected Output :
[
{
"address":"A-D-1",
"batch":{
"batch_number":"B-123"
},
"cost":"C1"
},
{
"address":"A-85-1",
"batch":{
"batch_number":"B-6562"
},
"cost":"C16464"
},
{
"address":"A-522-1",
"batch":{
"batch_number":"B-4511"
},
"cost":"C8745"
}
]
Any help would be great.
Thank You.
javascript json
Do you receive the JSON data as String?
– Mis94
Nov 19 at 17:30
I get as an array of objects.
– Brijesh Patel
Nov 19 at 17:31
How should the result look like?
– tevemadar
Nov 19 at 17:32
@tevemadar. I have edited my question. Can you please check ?
– Brijesh Patel
Nov 19 at 17:38
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have JSON like this.
[{
"address": "A-D-1",
"batch": [{
"batch_number": "B-123",
"cost": [{
"cost": "C1"
}]
}]
},
{
"address": "A-85-1",
"batch": [{
"batch_number": "B-6562",
"cost": [{
"cost": "C16464"
}]
}]
},
{
"address": "A-522-1",
"batch": [{
"batch_number": "B-4511",
"cost": [{
"cost": "C8745"
}]
}]
}]
I would like to store my JSON data to array.
let data = JSON.parse('[{"address":"A-D-1","batch":[{"batch_number":"B-123","cost":[{"cost":"C1"}]}]},{"address":"A-85-1","batch":[{"batch_number":"B-6562","cost":[{"cost":"C16464"}]}]},{"address":"A-522-1","batch":[{"batch_number":"B-4511","cost":[{"cost":"C8745"}]}]}]');
for (let i = 0; i < data.length; i++) {
if (data[i].batch !== undefined && data[i].batch !== null && data[i].batch.length !== undefined && data[i].batch.length > 0) {
let batchLength = data[i].batch.length
let newObject = {}
let newArray =
for (let j = 0; j < batchLength; j++) {
if (data[i].batch !== undefined && data[i].batch[j].cost !== null && data[i].batch[j].cost.length !== undefined && data[i].batch[j].cost.length > 0) {
let costLength = data[i].batch[j].cost.length
for (let k = 0; k < costLength; k++) {
newObject.location = data[i].address
newObject.batch.number = data[i].batch[j].batch_number ? data[i].batch[j].batch_number : ''
newObject.cogs = data[i].batch[j].cost[k].cost ? data[i].batch[j].cost[k].cost : ''
newArray.push(newObject)
}
}
}
}
}
I have stored JSON in data
variable.
I have tried below code. but I always get last index as repeatative.
Expected Output :
[
{
"address":"A-D-1",
"batch":{
"batch_number":"B-123"
},
"cost":"C1"
},
{
"address":"A-85-1",
"batch":{
"batch_number":"B-6562"
},
"cost":"C16464"
},
{
"address":"A-522-1",
"batch":{
"batch_number":"B-4511"
},
"cost":"C8745"
}
]
Any help would be great.
Thank You.
javascript json
I have JSON like this.
[{
"address": "A-D-1",
"batch": [{
"batch_number": "B-123",
"cost": [{
"cost": "C1"
}]
}]
},
{
"address": "A-85-1",
"batch": [{
"batch_number": "B-6562",
"cost": [{
"cost": "C16464"
}]
}]
},
{
"address": "A-522-1",
"batch": [{
"batch_number": "B-4511",
"cost": [{
"cost": "C8745"
}]
}]
}]
I would like to store my JSON data to array.
let data = JSON.parse('[{"address":"A-D-1","batch":[{"batch_number":"B-123","cost":[{"cost":"C1"}]}]},{"address":"A-85-1","batch":[{"batch_number":"B-6562","cost":[{"cost":"C16464"}]}]},{"address":"A-522-1","batch":[{"batch_number":"B-4511","cost":[{"cost":"C8745"}]}]}]');
for (let i = 0; i < data.length; i++) {
if (data[i].batch !== undefined && data[i].batch !== null && data[i].batch.length !== undefined && data[i].batch.length > 0) {
let batchLength = data[i].batch.length
let newObject = {}
let newArray =
for (let j = 0; j < batchLength; j++) {
if (data[i].batch !== undefined && data[i].batch[j].cost !== null && data[i].batch[j].cost.length !== undefined && data[i].batch[j].cost.length > 0) {
let costLength = data[i].batch[j].cost.length
for (let k = 0; k < costLength; k++) {
newObject.location = data[i].address
newObject.batch.number = data[i].batch[j].batch_number ? data[i].batch[j].batch_number : ''
newObject.cogs = data[i].batch[j].cost[k].cost ? data[i].batch[j].cost[k].cost : ''
newArray.push(newObject)
}
}
}
}
}
I have stored JSON in data
variable.
I have tried below code. but I always get last index as repeatative.
Expected Output :
[
{
"address":"A-D-1",
"batch":{
"batch_number":"B-123"
},
"cost":"C1"
},
{
"address":"A-85-1",
"batch":{
"batch_number":"B-6562"
},
"cost":"C16464"
},
{
"address":"A-522-1",
"batch":{
"batch_number":"B-4511"
},
"cost":"C8745"
}
]
Any help would be great.
Thank You.
let data = JSON.parse('[{"address":"A-D-1","batch":[{"batch_number":"B-123","cost":[{"cost":"C1"}]}]},{"address":"A-85-1","batch":[{"batch_number":"B-6562","cost":[{"cost":"C16464"}]}]},{"address":"A-522-1","batch":[{"batch_number":"B-4511","cost":[{"cost":"C8745"}]}]}]');
for (let i = 0; i < data.length; i++) {
if (data[i].batch !== undefined && data[i].batch !== null && data[i].batch.length !== undefined && data[i].batch.length > 0) {
let batchLength = data[i].batch.length
let newObject = {}
let newArray =
for (let j = 0; j < batchLength; j++) {
if (data[i].batch !== undefined && data[i].batch[j].cost !== null && data[i].batch[j].cost.length !== undefined && data[i].batch[j].cost.length > 0) {
let costLength = data[i].batch[j].cost.length
for (let k = 0; k < costLength; k++) {
newObject.location = data[i].address
newObject.batch.number = data[i].batch[j].batch_number ? data[i].batch[j].batch_number : ''
newObject.cogs = data[i].batch[j].cost[k].cost ? data[i].batch[j].cost[k].cost : ''
newArray.push(newObject)
}
}
}
}
}
let data = JSON.parse('[{"address":"A-D-1","batch":[{"batch_number":"B-123","cost":[{"cost":"C1"}]}]},{"address":"A-85-1","batch":[{"batch_number":"B-6562","cost":[{"cost":"C16464"}]}]},{"address":"A-522-1","batch":[{"batch_number":"B-4511","cost":[{"cost":"C8745"}]}]}]');
for (let i = 0; i < data.length; i++) {
if (data[i].batch !== undefined && data[i].batch !== null && data[i].batch.length !== undefined && data[i].batch.length > 0) {
let batchLength = data[i].batch.length
let newObject = {}
let newArray =
for (let j = 0; j < batchLength; j++) {
if (data[i].batch !== undefined && data[i].batch[j].cost !== null && data[i].batch[j].cost.length !== undefined && data[i].batch[j].cost.length > 0) {
let costLength = data[i].batch[j].cost.length
for (let k = 0; k < costLength; k++) {
newObject.location = data[i].address
newObject.batch.number = data[i].batch[j].batch_number ? data[i].batch[j].batch_number : ''
newObject.cogs = data[i].batch[j].cost[k].cost ? data[i].batch[j].cost[k].cost : ''
newArray.push(newObject)
}
}
}
}
}
javascript json
javascript json
edited Nov 19 at 17:58
tevemadar
4,1952723
4,1952723
asked Nov 19 at 17:11


Brijesh Patel
376
376
Do you receive the JSON data as String?
– Mis94
Nov 19 at 17:30
I get as an array of objects.
– Brijesh Patel
Nov 19 at 17:31
How should the result look like?
– tevemadar
Nov 19 at 17:32
@tevemadar. I have edited my question. Can you please check ?
– Brijesh Patel
Nov 19 at 17:38
add a comment |
Do you receive the JSON data as String?
– Mis94
Nov 19 at 17:30
I get as an array of objects.
– Brijesh Patel
Nov 19 at 17:31
How should the result look like?
– tevemadar
Nov 19 at 17:32
@tevemadar. I have edited my question. Can you please check ?
– Brijesh Patel
Nov 19 at 17:38
Do you receive the JSON data as String?
– Mis94
Nov 19 at 17:30
Do you receive the JSON data as String?
– Mis94
Nov 19 at 17:30
I get as an array of objects.
– Brijesh Patel
Nov 19 at 17:31
I get as an array of objects.
– Brijesh Patel
Nov 19 at 17:31
How should the result look like?
– tevemadar
Nov 19 at 17:32
How should the result look like?
– tevemadar
Nov 19 at 17:32
@tevemadar. I have edited my question. Can you please check ?
– Brijesh Patel
Nov 19 at 17:38
@tevemadar. I have edited my question. Can you please check ?
– Brijesh Patel
Nov 19 at 17:38
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
You mean something like this?
const someArray = [{
"address": "A-D-1",
"batch": [{
"batch_number": "B-123",
"cost": [{
"cost": "C1"
}]
}]
}, {
"address": "A-85-1",
"batch": [{
"batch_number": "B-6562",
"cost": [{
"cost": "C16464"
}]
}]
}, {
"address": "A-522-1",
"batch": [{
"batch_number": "B-4511",
"cost": [{
"cost": "C8745"
}]
}]
}]
console.log(someArray.map(item => {
return {
address:item.address,
batch_number: item.batch && item.batch[0].batch_number,
cost: item.batch && item.batch[0].cost[0].cost
}
}))
yes but I can have multiple arrays inside each array. so how can I solve that issue ?
– Brijesh Patel
Nov 19 at 17:29
add a comment |
up vote
0
down vote
You can use below method to retrieve the same in array format.
const data = JSON.parse(someArray);
console.log (data[0].address);
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You mean something like this?
const someArray = [{
"address": "A-D-1",
"batch": [{
"batch_number": "B-123",
"cost": [{
"cost": "C1"
}]
}]
}, {
"address": "A-85-1",
"batch": [{
"batch_number": "B-6562",
"cost": [{
"cost": "C16464"
}]
}]
}, {
"address": "A-522-1",
"batch": [{
"batch_number": "B-4511",
"cost": [{
"cost": "C8745"
}]
}]
}]
console.log(someArray.map(item => {
return {
address:item.address,
batch_number: item.batch && item.batch[0].batch_number,
cost: item.batch && item.batch[0].cost[0].cost
}
}))
yes but I can have multiple arrays inside each array. so how can I solve that issue ?
– Brijesh Patel
Nov 19 at 17:29
add a comment |
up vote
0
down vote
You mean something like this?
const someArray = [{
"address": "A-D-1",
"batch": [{
"batch_number": "B-123",
"cost": [{
"cost": "C1"
}]
}]
}, {
"address": "A-85-1",
"batch": [{
"batch_number": "B-6562",
"cost": [{
"cost": "C16464"
}]
}]
}, {
"address": "A-522-1",
"batch": [{
"batch_number": "B-4511",
"cost": [{
"cost": "C8745"
}]
}]
}]
console.log(someArray.map(item => {
return {
address:item.address,
batch_number: item.batch && item.batch[0].batch_number,
cost: item.batch && item.batch[0].cost[0].cost
}
}))
yes but I can have multiple arrays inside each array. so how can I solve that issue ?
– Brijesh Patel
Nov 19 at 17:29
add a comment |
up vote
0
down vote
up vote
0
down vote
You mean something like this?
const someArray = [{
"address": "A-D-1",
"batch": [{
"batch_number": "B-123",
"cost": [{
"cost": "C1"
}]
}]
}, {
"address": "A-85-1",
"batch": [{
"batch_number": "B-6562",
"cost": [{
"cost": "C16464"
}]
}]
}, {
"address": "A-522-1",
"batch": [{
"batch_number": "B-4511",
"cost": [{
"cost": "C8745"
}]
}]
}]
console.log(someArray.map(item => {
return {
address:item.address,
batch_number: item.batch && item.batch[0].batch_number,
cost: item.batch && item.batch[0].cost[0].cost
}
}))
You mean something like this?
const someArray = [{
"address": "A-D-1",
"batch": [{
"batch_number": "B-123",
"cost": [{
"cost": "C1"
}]
}]
}, {
"address": "A-85-1",
"batch": [{
"batch_number": "B-6562",
"cost": [{
"cost": "C16464"
}]
}]
}, {
"address": "A-522-1",
"batch": [{
"batch_number": "B-4511",
"cost": [{
"cost": "C8745"
}]
}]
}]
console.log(someArray.map(item => {
return {
address:item.address,
batch_number: item.batch && item.batch[0].batch_number,
cost: item.batch && item.batch[0].cost[0].cost
}
}))
const someArray = [{
"address": "A-D-1",
"batch": [{
"batch_number": "B-123",
"cost": [{
"cost": "C1"
}]
}]
}, {
"address": "A-85-1",
"batch": [{
"batch_number": "B-6562",
"cost": [{
"cost": "C16464"
}]
}]
}, {
"address": "A-522-1",
"batch": [{
"batch_number": "B-4511",
"cost": [{
"cost": "C8745"
}]
}]
}]
console.log(someArray.map(item => {
return {
address:item.address,
batch_number: item.batch && item.batch[0].batch_number,
cost: item.batch && item.batch[0].cost[0].cost
}
}))
const someArray = [{
"address": "A-D-1",
"batch": [{
"batch_number": "B-123",
"cost": [{
"cost": "C1"
}]
}]
}, {
"address": "A-85-1",
"batch": [{
"batch_number": "B-6562",
"cost": [{
"cost": "C16464"
}]
}]
}, {
"address": "A-522-1",
"batch": [{
"batch_number": "B-4511",
"cost": [{
"cost": "C8745"
}]
}]
}]
console.log(someArray.map(item => {
return {
address:item.address,
batch_number: item.batch && item.batch[0].batch_number,
cost: item.batch && item.batch[0].cost[0].cost
}
}))
answered Nov 19 at 17:28
Arulmozhi Manikandan
1665
1665
yes but I can have multiple arrays inside each array. so how can I solve that issue ?
– Brijesh Patel
Nov 19 at 17:29
add a comment |
yes but I can have multiple arrays inside each array. so how can I solve that issue ?
– Brijesh Patel
Nov 19 at 17:29
yes but I can have multiple arrays inside each array. so how can I solve that issue ?
– Brijesh Patel
Nov 19 at 17:29
yes but I can have multiple arrays inside each array. so how can I solve that issue ?
– Brijesh Patel
Nov 19 at 17:29
add a comment |
up vote
0
down vote
You can use below method to retrieve the same in array format.
const data = JSON.parse(someArray);
console.log (data[0].address);
add a comment |
up vote
0
down vote
You can use below method to retrieve the same in array format.
const data = JSON.parse(someArray);
console.log (data[0].address);
add a comment |
up vote
0
down vote
up vote
0
down vote
You can use below method to retrieve the same in array format.
const data = JSON.parse(someArray);
console.log (data[0].address);
You can use below method to retrieve the same in array format.
const data = JSON.parse(someArray);
console.log (data[0].address);
answered Nov 19 at 17:40
Murali
34
34
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53379594%2fjavascript-store-json-data-in-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Edjyh,O RIcsiaz2,FHbQXqAEttYjcWllF4rZxzjxJ R9s96i6AG,q,gBFtU EEnIKK,xpEmp,03NEN4l4,ycKQ,dXevlcU
Do you receive the JSON data as String?
– Mis94
Nov 19 at 17:30
I get as an array of objects.
– Brijesh Patel
Nov 19 at 17:31
How should the result look like?
– tevemadar
Nov 19 at 17:32
@tevemadar. I have edited my question. Can you please check ?
– Brijesh Patel
Nov 19 at 17:38