Why does defaulted-move-ctor inhibit implicit-copy-ctor but not defaulted-copy-ctor?
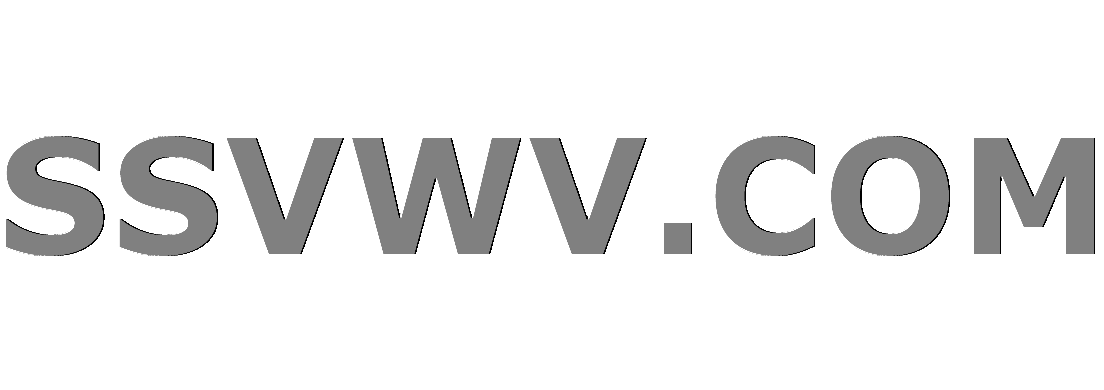
Multi tool use
I seem to have encountered an example in which a defaulted move constructor seems to count as both user-declared and not-user declared depending on the situation:
struct Foo {
int a;
Foo():a(42){};
//Foo(const Foo & other) = default;
Foo(Foo && other) = default;
};
int main() {
Foo f;
Foo g = f;
}
results in this:
test.cpp:11:9: error: call to implicitly-deleted copy constructor of 'Foo'
Foo g = f;
^ ~
test.cpp:5:5: note: copy constructor is implicitly deleted because 'Foo' has a user-declared move constructor
Foo(Foo && other) = default;
^
The compiler error is expected, since cppreference tell us this:
The implicitly-declared or defaulted copy constructor for class T is
defined as deleted if any of the following conditions are true:
[...]
T has a user-defined move constructor or move assignment operator;
Note, that the defaulted constructor apparently counts as "user-declared" here.
However, if we now remove the comments from line 4, thereby explicitly defaulting the copy constructor, the program will compile without error, even though the statement I quoted above specifies:
The implicitly-declared or defaulted copy constructor
This seems like a contradiction. Is this an error in cppreference or am I simply confused?
c++ standards copy-constructor default-copy-constructor
add a comment |
I seem to have encountered an example in which a defaulted move constructor seems to count as both user-declared and not-user declared depending on the situation:
struct Foo {
int a;
Foo():a(42){};
//Foo(const Foo & other) = default;
Foo(Foo && other) = default;
};
int main() {
Foo f;
Foo g = f;
}
results in this:
test.cpp:11:9: error: call to implicitly-deleted copy constructor of 'Foo'
Foo g = f;
^ ~
test.cpp:5:5: note: copy constructor is implicitly deleted because 'Foo' has a user-declared move constructor
Foo(Foo && other) = default;
^
The compiler error is expected, since cppreference tell us this:
The implicitly-declared or defaulted copy constructor for class T is
defined as deleted if any of the following conditions are true:
[...]
T has a user-defined move constructor or move assignment operator;
Note, that the defaulted constructor apparently counts as "user-declared" here.
However, if we now remove the comments from line 4, thereby explicitly defaulting the copy constructor, the program will compile without error, even though the statement I quoted above specifies:
The implicitly-declared or defaulted copy constructor
This seems like a contradiction. Is this an error in cppreference or am I simply confused?
c++ standards copy-constructor default-copy-constructor
add a comment |
I seem to have encountered an example in which a defaulted move constructor seems to count as both user-declared and not-user declared depending on the situation:
struct Foo {
int a;
Foo():a(42){};
//Foo(const Foo & other) = default;
Foo(Foo && other) = default;
};
int main() {
Foo f;
Foo g = f;
}
results in this:
test.cpp:11:9: error: call to implicitly-deleted copy constructor of 'Foo'
Foo g = f;
^ ~
test.cpp:5:5: note: copy constructor is implicitly deleted because 'Foo' has a user-declared move constructor
Foo(Foo && other) = default;
^
The compiler error is expected, since cppreference tell us this:
The implicitly-declared or defaulted copy constructor for class T is
defined as deleted if any of the following conditions are true:
[...]
T has a user-defined move constructor or move assignment operator;
Note, that the defaulted constructor apparently counts as "user-declared" here.
However, if we now remove the comments from line 4, thereby explicitly defaulting the copy constructor, the program will compile without error, even though the statement I quoted above specifies:
The implicitly-declared or defaulted copy constructor
This seems like a contradiction. Is this an error in cppreference or am I simply confused?
c++ standards copy-constructor default-copy-constructor
I seem to have encountered an example in which a defaulted move constructor seems to count as both user-declared and not-user declared depending on the situation:
struct Foo {
int a;
Foo():a(42){};
//Foo(const Foo & other) = default;
Foo(Foo && other) = default;
};
int main() {
Foo f;
Foo g = f;
}
results in this:
test.cpp:11:9: error: call to implicitly-deleted copy constructor of 'Foo'
Foo g = f;
^ ~
test.cpp:5:5: note: copy constructor is implicitly deleted because 'Foo' has a user-declared move constructor
Foo(Foo && other) = default;
^
The compiler error is expected, since cppreference tell us this:
The implicitly-declared or defaulted copy constructor for class T is
defined as deleted if any of the following conditions are true:
[...]
T has a user-defined move constructor or move assignment operator;
Note, that the defaulted constructor apparently counts as "user-declared" here.
However, if we now remove the comments from line 4, thereby explicitly defaulting the copy constructor, the program will compile without error, even though the statement I quoted above specifies:
The implicitly-declared or defaulted copy constructor
This seems like a contradiction. Is this an error in cppreference or am I simply confused?
c++ standards copy-constructor default-copy-constructor
c++ standards copy-constructor default-copy-constructor
edited Nov 22 '18 at 2:19
JMC
asked Nov 22 '18 at 2:10
JMCJMC
18819
18819
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
This looks like an error on cppreference's part. Declaring a move constructor/assignment operator only deletes an implicitly declared copy constructor. Indeed declaring a copy constructor as default is user-declaring it and thus that doesn't apply to this case.
add a comment |
Why does defaulted-move-ctor inhibit implicit-copy-ctor but not defaulted-copy-ctor?
Because when you define the constructor as defaulted you’re reestablishing the delete effect caused by the move constructor, defaulting the copy constructor has the same effect as explicitly defining it doing what the compiler would do.
1
It can be deleted, if that is what would happen to the implicitly-declared one. stackoverflow.com/a/48987654/5001448 This is an example of an expliclitly defaulted constructor being effectively deleted, and removed from the overload pool.
– JMC
Nov 22 '18 at 2:56
that part of the post is related to the effect the members of the class has over the constructor of the enclosing class something different to your case.
– Jans
Nov 22 '18 at 3:08
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53422961%2fwhy-does-defaulted-move-ctor-inhibit-implicit-copy-ctor-but-not-defaulted-copy-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
This looks like an error on cppreference's part. Declaring a move constructor/assignment operator only deletes an implicitly declared copy constructor. Indeed declaring a copy constructor as default is user-declaring it and thus that doesn't apply to this case.
add a comment |
This looks like an error on cppreference's part. Declaring a move constructor/assignment operator only deletes an implicitly declared copy constructor. Indeed declaring a copy constructor as default is user-declaring it and thus that doesn't apply to this case.
add a comment |
This looks like an error on cppreference's part. Declaring a move constructor/assignment operator only deletes an implicitly declared copy constructor. Indeed declaring a copy constructor as default is user-declaring it and thus that doesn't apply to this case.
This looks like an error on cppreference's part. Declaring a move constructor/assignment operator only deletes an implicitly declared copy constructor. Indeed declaring a copy constructor as default is user-declaring it and thus that doesn't apply to this case.
answered Nov 22 '18 at 2:39


0x499602D20x499602D2
67.4k26118204
67.4k26118204
add a comment |
add a comment |
Why does defaulted-move-ctor inhibit implicit-copy-ctor but not defaulted-copy-ctor?
Because when you define the constructor as defaulted you’re reestablishing the delete effect caused by the move constructor, defaulting the copy constructor has the same effect as explicitly defining it doing what the compiler would do.
1
It can be deleted, if that is what would happen to the implicitly-declared one. stackoverflow.com/a/48987654/5001448 This is an example of an expliclitly defaulted constructor being effectively deleted, and removed from the overload pool.
– JMC
Nov 22 '18 at 2:56
that part of the post is related to the effect the members of the class has over the constructor of the enclosing class something different to your case.
– Jans
Nov 22 '18 at 3:08
add a comment |
Why does defaulted-move-ctor inhibit implicit-copy-ctor but not defaulted-copy-ctor?
Because when you define the constructor as defaulted you’re reestablishing the delete effect caused by the move constructor, defaulting the copy constructor has the same effect as explicitly defining it doing what the compiler would do.
1
It can be deleted, if that is what would happen to the implicitly-declared one. stackoverflow.com/a/48987654/5001448 This is an example of an expliclitly defaulted constructor being effectively deleted, and removed from the overload pool.
– JMC
Nov 22 '18 at 2:56
that part of the post is related to the effect the members of the class has over the constructor of the enclosing class something different to your case.
– Jans
Nov 22 '18 at 3:08
add a comment |
Why does defaulted-move-ctor inhibit implicit-copy-ctor but not defaulted-copy-ctor?
Because when you define the constructor as defaulted you’re reestablishing the delete effect caused by the move constructor, defaulting the copy constructor has the same effect as explicitly defining it doing what the compiler would do.
Why does defaulted-move-ctor inhibit implicit-copy-ctor but not defaulted-copy-ctor?
Because when you define the constructor as defaulted you’re reestablishing the delete effect caused by the move constructor, defaulting the copy constructor has the same effect as explicitly defining it doing what the compiler would do.
edited Nov 22 '18 at 3:05
answered Nov 22 '18 at 2:41
JansJans
8,73122635
8,73122635
1
It can be deleted, if that is what would happen to the implicitly-declared one. stackoverflow.com/a/48987654/5001448 This is an example of an expliclitly defaulted constructor being effectively deleted, and removed from the overload pool.
– JMC
Nov 22 '18 at 2:56
that part of the post is related to the effect the members of the class has over the constructor of the enclosing class something different to your case.
– Jans
Nov 22 '18 at 3:08
add a comment |
1
It can be deleted, if that is what would happen to the implicitly-declared one. stackoverflow.com/a/48987654/5001448 This is an example of an expliclitly defaulted constructor being effectively deleted, and removed from the overload pool.
– JMC
Nov 22 '18 at 2:56
that part of the post is related to the effect the members of the class has over the constructor of the enclosing class something different to your case.
– Jans
Nov 22 '18 at 3:08
1
1
It can be deleted, if that is what would happen to the implicitly-declared one. stackoverflow.com/a/48987654/5001448 This is an example of an expliclitly defaulted constructor being effectively deleted, and removed from the overload pool.
– JMC
Nov 22 '18 at 2:56
It can be deleted, if that is what would happen to the implicitly-declared one. stackoverflow.com/a/48987654/5001448 This is an example of an expliclitly defaulted constructor being effectively deleted, and removed from the overload pool.
– JMC
Nov 22 '18 at 2:56
that part of the post is related to the effect the members of the class has over the constructor of the enclosing class something different to your case.
– Jans
Nov 22 '18 at 3:08
that part of the post is related to the effect the members of the class has over the constructor of the enclosing class something different to your case.
– Jans
Nov 22 '18 at 3:08
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53422961%2fwhy-does-defaulted-move-ctor-inhibit-implicit-copy-ctor-but-not-defaulted-copy-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
RK,5v4J LnpzdTV9zKkA9oHKC,k,UcmD0t B2I3L86XWxpzNcea2,E1lt,XZMf,XStjbIaET7