how to store objects into hashmap as key and its insert into asending order without using comparator and...
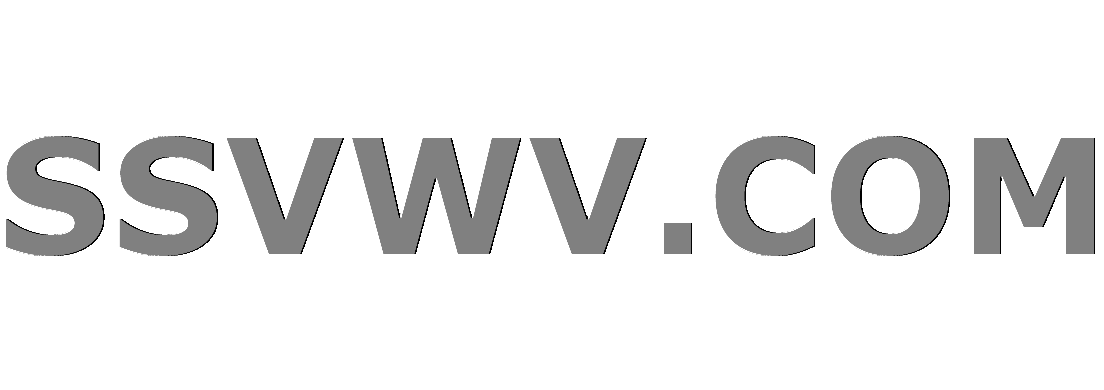
Multi tool use
I want to store Objects in the Hash map as key and while Retrieving time i want a ascending order based on Employee id.and i dont want to use comparator or comaprable interfaces just sorting based on hashcode and equals method is it possible.
public class Employee {
private String name;
private int id;
public String getName() {
return name;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + id;
// result = prime * result + ((name == null) ? 0 : name.hashCode());
System.out.println("hashcode value :::"+result + "id :::"+id);
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
Employee other = (Employee) obj;
if (id != other.id) {
return false;
}
if (name == null) {
if (other.name != null) {
return false;
}
} else if (!name.equals(other.name)) {
return false;
}
return true;
}
public void setName(String name) {
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
package info.test;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class MainClass {
public static void main(String args) {
Employee e1 = new Employee();
e1.setId(2);
e1.setName("name");
Employee e2 = new Employee();
e2.setId(35);
e2.setName("name");
Employee e3 = new Employee();
e3.setId(4);
e3.setName("name");
Employee e4 = new Employee();
e4.setId(3);
e4.setName("name");
Map<Employee, String> linkedHashMap = new HashMap<Employee,String>();
linkedHashMap.put(e1, "e");
linkedHashMap.put(e2, "e");
linkedHashMap.put(e3, "e");
linkedHashMap.put(e4, "e");
Set<Employee> keySet = linkedHashMap.keySet();
for(Employee e:keySet){
//System.out.println(e.getId());
}
}}
its work some samples like
if give ids like 2 55 4 3 and output is 2 3 4 55
and for some samples its not working
2 35 4 3 and output 2 35 3 4
java hashmap logic hashcode
add a comment |
I want to store Objects in the Hash map as key and while Retrieving time i want a ascending order based on Employee id.and i dont want to use comparator or comaprable interfaces just sorting based on hashcode and equals method is it possible.
public class Employee {
private String name;
private int id;
public String getName() {
return name;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + id;
// result = prime * result + ((name == null) ? 0 : name.hashCode());
System.out.println("hashcode value :::"+result + "id :::"+id);
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
Employee other = (Employee) obj;
if (id != other.id) {
return false;
}
if (name == null) {
if (other.name != null) {
return false;
}
} else if (!name.equals(other.name)) {
return false;
}
return true;
}
public void setName(String name) {
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
package info.test;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class MainClass {
public static void main(String args) {
Employee e1 = new Employee();
e1.setId(2);
e1.setName("name");
Employee e2 = new Employee();
e2.setId(35);
e2.setName("name");
Employee e3 = new Employee();
e3.setId(4);
e3.setName("name");
Employee e4 = new Employee();
e4.setId(3);
e4.setName("name");
Map<Employee, String> linkedHashMap = new HashMap<Employee,String>();
linkedHashMap.put(e1, "e");
linkedHashMap.put(e2, "e");
linkedHashMap.put(e3, "e");
linkedHashMap.put(e4, "e");
Set<Employee> keySet = linkedHashMap.keySet();
for(Employee e:keySet){
//System.out.println(e.getId());
}
}}
its work some samples like
if give ids like 2 55 4 3 and output is 2 3 4 55
and for some samples its not working
2 35 4 3 and output 2 35 3 4
java hashmap logic hashcode
2
No, it's not. A HashMap is not a sorted map, and if you used a sorted map, you would need a comparator, because you can't tell whether somthing is bigger, lower or equal to something else without that.
– JB Nizet
Nov 24 '18 at 8:24
just sorting based on hashcode and equals method
. How would that be?hashCode
returns a value that is used to scatter the entries along the internal array that comprises aHashMap
, whileequals
compares two objects for equality and returns aboolean
, saying whether the objects are equal or not. How would you sort based on these two methods, if you are never saying which object should come before the other? I mean, you need to compare if you want some sorting to occur. This is basic maths and basic common sense. You can't sort based on equality and a value used to separate stuff.
– Federico Peralta Schaffner
Nov 25 '18 at 3:05
add a comment |
I want to store Objects in the Hash map as key and while Retrieving time i want a ascending order based on Employee id.and i dont want to use comparator or comaprable interfaces just sorting based on hashcode and equals method is it possible.
public class Employee {
private String name;
private int id;
public String getName() {
return name;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + id;
// result = prime * result + ((name == null) ? 0 : name.hashCode());
System.out.println("hashcode value :::"+result + "id :::"+id);
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
Employee other = (Employee) obj;
if (id != other.id) {
return false;
}
if (name == null) {
if (other.name != null) {
return false;
}
} else if (!name.equals(other.name)) {
return false;
}
return true;
}
public void setName(String name) {
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
package info.test;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class MainClass {
public static void main(String args) {
Employee e1 = new Employee();
e1.setId(2);
e1.setName("name");
Employee e2 = new Employee();
e2.setId(35);
e2.setName("name");
Employee e3 = new Employee();
e3.setId(4);
e3.setName("name");
Employee e4 = new Employee();
e4.setId(3);
e4.setName("name");
Map<Employee, String> linkedHashMap = new HashMap<Employee,String>();
linkedHashMap.put(e1, "e");
linkedHashMap.put(e2, "e");
linkedHashMap.put(e3, "e");
linkedHashMap.put(e4, "e");
Set<Employee> keySet = linkedHashMap.keySet();
for(Employee e:keySet){
//System.out.println(e.getId());
}
}}
its work some samples like
if give ids like 2 55 4 3 and output is 2 3 4 55
and for some samples its not working
2 35 4 3 and output 2 35 3 4
java hashmap logic hashcode
I want to store Objects in the Hash map as key and while Retrieving time i want a ascending order based on Employee id.and i dont want to use comparator or comaprable interfaces just sorting based on hashcode and equals method is it possible.
public class Employee {
private String name;
private int id;
public String getName() {
return name;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + id;
// result = prime * result + ((name == null) ? 0 : name.hashCode());
System.out.println("hashcode value :::"+result + "id :::"+id);
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
Employee other = (Employee) obj;
if (id != other.id) {
return false;
}
if (name == null) {
if (other.name != null) {
return false;
}
} else if (!name.equals(other.name)) {
return false;
}
return true;
}
public void setName(String name) {
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
package info.test;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class MainClass {
public static void main(String args) {
Employee e1 = new Employee();
e1.setId(2);
e1.setName("name");
Employee e2 = new Employee();
e2.setId(35);
e2.setName("name");
Employee e3 = new Employee();
e3.setId(4);
e3.setName("name");
Employee e4 = new Employee();
e4.setId(3);
e4.setName("name");
Map<Employee, String> linkedHashMap = new HashMap<Employee,String>();
linkedHashMap.put(e1, "e");
linkedHashMap.put(e2, "e");
linkedHashMap.put(e3, "e");
linkedHashMap.put(e4, "e");
Set<Employee> keySet = linkedHashMap.keySet();
for(Employee e:keySet){
//System.out.println(e.getId());
}
}}
its work some samples like
if give ids like 2 55 4 3 and output is 2 3 4 55
and for some samples its not working
2 35 4 3 and output 2 35 3 4
java hashmap logic hashcode
java hashmap logic hashcode
asked Nov 24 '18 at 8:20
RajuRaju
374418
374418
2
No, it's not. A HashMap is not a sorted map, and if you used a sorted map, you would need a comparator, because you can't tell whether somthing is bigger, lower or equal to something else without that.
– JB Nizet
Nov 24 '18 at 8:24
just sorting based on hashcode and equals method
. How would that be?hashCode
returns a value that is used to scatter the entries along the internal array that comprises aHashMap
, whileequals
compares two objects for equality and returns aboolean
, saying whether the objects are equal or not. How would you sort based on these two methods, if you are never saying which object should come before the other? I mean, you need to compare if you want some sorting to occur. This is basic maths and basic common sense. You can't sort based on equality and a value used to separate stuff.
– Federico Peralta Schaffner
Nov 25 '18 at 3:05
add a comment |
2
No, it's not. A HashMap is not a sorted map, and if you used a sorted map, you would need a comparator, because you can't tell whether somthing is bigger, lower or equal to something else without that.
– JB Nizet
Nov 24 '18 at 8:24
just sorting based on hashcode and equals method
. How would that be?hashCode
returns a value that is used to scatter the entries along the internal array that comprises aHashMap
, whileequals
compares two objects for equality and returns aboolean
, saying whether the objects are equal or not. How would you sort based on these two methods, if you are never saying which object should come before the other? I mean, you need to compare if you want some sorting to occur. This is basic maths and basic common sense. You can't sort based on equality and a value used to separate stuff.
– Federico Peralta Schaffner
Nov 25 '18 at 3:05
2
2
No, it's not. A HashMap is not a sorted map, and if you used a sorted map, you would need a comparator, because you can't tell whether somthing is bigger, lower or equal to something else without that.
– JB Nizet
Nov 24 '18 at 8:24
No, it's not. A HashMap is not a sorted map, and if you used a sorted map, you would need a comparator, because you can't tell whether somthing is bigger, lower or equal to something else without that.
– JB Nizet
Nov 24 '18 at 8:24
just sorting based on hashcode and equals method
. How would that be? hashCode
returns a value that is used to scatter the entries along the internal array that comprises a HashMap
, while equals
compares two objects for equality and returns a boolean
, saying whether the objects are equal or not. How would you sort based on these two methods, if you are never saying which object should come before the other? I mean, you need to compare if you want some sorting to occur. This is basic maths and basic common sense. You can't sort based on equality and a value used to separate stuff.– Federico Peralta Schaffner
Nov 25 '18 at 3:05
just sorting based on hashcode and equals method
. How would that be? hashCode
returns a value that is used to scatter the entries along the internal array that comprises a HashMap
, while equals
compares two objects for equality and returns a boolean
, saying whether the objects are equal or not. How would you sort based on these two methods, if you are never saying which object should come before the other? I mean, you need to compare if you want some sorting to occur. This is basic maths and basic common sense. You can't sort based on equality and a value used to separate stuff.– Federico Peralta Schaffner
Nov 25 '18 at 3:05
add a comment |
1 Answer
1
active
oldest
votes
You were so close. This
Map<Employee, String> linkedHashMap = new HashMap<Employee,String>();
should be LinkedHashMap
which preserved insertion order like
Map<Employee, String> linkedHashMap = new LinkedHashMap<>();
If it should be sorted in natural order use a TreeMap
like
Map<Employee, String> linkedHashMap = new TreeMap<>();
Thanks for ur answer if a use TreeMap then i need to implement comparable interface to employee class but i dont want like that ,its any possible to do sorting with hashcode and equals method
– Raju
Nov 24 '18 at 8:39
1
@Raju its any possible to do sorting with hashcode and equals method No. For hashcode to work properly the hashes must be well distributed. That means they are fundamentally not usable for sorting.
– Elliott Frisch
Nov 24 '18 at 8:57
1
@Raju how would you do sorting based on hashCode and equals? If two objects the have the same hashCode, but are equal - which one goes first? You could just create a comparator and point it to the hashCode, but are the values sorted by this any better than being random?
– Jakg
Nov 24 '18 at 10:13
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53456424%2fhow-to-store-objects-into-hashmap-as-key-and-its-insert-into-asending-order-with%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You were so close. This
Map<Employee, String> linkedHashMap = new HashMap<Employee,String>();
should be LinkedHashMap
which preserved insertion order like
Map<Employee, String> linkedHashMap = new LinkedHashMap<>();
If it should be sorted in natural order use a TreeMap
like
Map<Employee, String> linkedHashMap = new TreeMap<>();
Thanks for ur answer if a use TreeMap then i need to implement comparable interface to employee class but i dont want like that ,its any possible to do sorting with hashcode and equals method
– Raju
Nov 24 '18 at 8:39
1
@Raju its any possible to do sorting with hashcode and equals method No. For hashcode to work properly the hashes must be well distributed. That means they are fundamentally not usable for sorting.
– Elliott Frisch
Nov 24 '18 at 8:57
1
@Raju how would you do sorting based on hashCode and equals? If two objects the have the same hashCode, but are equal - which one goes first? You could just create a comparator and point it to the hashCode, but are the values sorted by this any better than being random?
– Jakg
Nov 24 '18 at 10:13
add a comment |
You were so close. This
Map<Employee, String> linkedHashMap = new HashMap<Employee,String>();
should be LinkedHashMap
which preserved insertion order like
Map<Employee, String> linkedHashMap = new LinkedHashMap<>();
If it should be sorted in natural order use a TreeMap
like
Map<Employee, String> linkedHashMap = new TreeMap<>();
Thanks for ur answer if a use TreeMap then i need to implement comparable interface to employee class but i dont want like that ,its any possible to do sorting with hashcode and equals method
– Raju
Nov 24 '18 at 8:39
1
@Raju its any possible to do sorting with hashcode and equals method No. For hashcode to work properly the hashes must be well distributed. That means they are fundamentally not usable for sorting.
– Elliott Frisch
Nov 24 '18 at 8:57
1
@Raju how would you do sorting based on hashCode and equals? If two objects the have the same hashCode, but are equal - which one goes first? You could just create a comparator and point it to the hashCode, but are the values sorted by this any better than being random?
– Jakg
Nov 24 '18 at 10:13
add a comment |
You were so close. This
Map<Employee, String> linkedHashMap = new HashMap<Employee,String>();
should be LinkedHashMap
which preserved insertion order like
Map<Employee, String> linkedHashMap = new LinkedHashMap<>();
If it should be sorted in natural order use a TreeMap
like
Map<Employee, String> linkedHashMap = new TreeMap<>();
You were so close. This
Map<Employee, String> linkedHashMap = new HashMap<Employee,String>();
should be LinkedHashMap
which preserved insertion order like
Map<Employee, String> linkedHashMap = new LinkedHashMap<>();
If it should be sorted in natural order use a TreeMap
like
Map<Employee, String> linkedHashMap = new TreeMap<>();
answered Nov 24 '18 at 8:29


Elliott FrischElliott Frisch
154k1393184
154k1393184
Thanks for ur answer if a use TreeMap then i need to implement comparable interface to employee class but i dont want like that ,its any possible to do sorting with hashcode and equals method
– Raju
Nov 24 '18 at 8:39
1
@Raju its any possible to do sorting with hashcode and equals method No. For hashcode to work properly the hashes must be well distributed. That means they are fundamentally not usable for sorting.
– Elliott Frisch
Nov 24 '18 at 8:57
1
@Raju how would you do sorting based on hashCode and equals? If two objects the have the same hashCode, but are equal - which one goes first? You could just create a comparator and point it to the hashCode, but are the values sorted by this any better than being random?
– Jakg
Nov 24 '18 at 10:13
add a comment |
Thanks for ur answer if a use TreeMap then i need to implement comparable interface to employee class but i dont want like that ,its any possible to do sorting with hashcode and equals method
– Raju
Nov 24 '18 at 8:39
1
@Raju its any possible to do sorting with hashcode and equals method No. For hashcode to work properly the hashes must be well distributed. That means they are fundamentally not usable for sorting.
– Elliott Frisch
Nov 24 '18 at 8:57
1
@Raju how would you do sorting based on hashCode and equals? If two objects the have the same hashCode, but are equal - which one goes first? You could just create a comparator and point it to the hashCode, but are the values sorted by this any better than being random?
– Jakg
Nov 24 '18 at 10:13
Thanks for ur answer if a use TreeMap then i need to implement comparable interface to employee class but i dont want like that ,its any possible to do sorting with hashcode and equals method
– Raju
Nov 24 '18 at 8:39
Thanks for ur answer if a use TreeMap then i need to implement comparable interface to employee class but i dont want like that ,its any possible to do sorting with hashcode and equals method
– Raju
Nov 24 '18 at 8:39
1
1
@Raju its any possible to do sorting with hashcode and equals method No. For hashcode to work properly the hashes must be well distributed. That means they are fundamentally not usable for sorting.
– Elliott Frisch
Nov 24 '18 at 8:57
@Raju its any possible to do sorting with hashcode and equals method No. For hashcode to work properly the hashes must be well distributed. That means they are fundamentally not usable for sorting.
– Elliott Frisch
Nov 24 '18 at 8:57
1
1
@Raju how would you do sorting based on hashCode and equals? If two objects the have the same hashCode, but are equal - which one goes first? You could just create a comparator and point it to the hashCode, but are the values sorted by this any better than being random?
– Jakg
Nov 24 '18 at 10:13
@Raju how would you do sorting based on hashCode and equals? If two objects the have the same hashCode, but are equal - which one goes first? You could just create a comparator and point it to the hashCode, but are the values sorted by this any better than being random?
– Jakg
Nov 24 '18 at 10:13
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53456424%2fhow-to-store-objects-into-hashmap-as-key-and-its-insert-into-asending-order-with%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
YEFi34
2
No, it's not. A HashMap is not a sorted map, and if you used a sorted map, you would need a comparator, because you can't tell whether somthing is bigger, lower or equal to something else without that.
– JB Nizet
Nov 24 '18 at 8:24
just sorting based on hashcode and equals method
. How would that be?hashCode
returns a value that is used to scatter the entries along the internal array that comprises aHashMap
, whileequals
compares two objects for equality and returns aboolean
, saying whether the objects are equal or not. How would you sort based on these two methods, if you are never saying which object should come before the other? I mean, you need to compare if you want some sorting to occur. This is basic maths and basic common sense. You can't sort based on equality and a value used to separate stuff.– Federico Peralta Schaffner
Nov 25 '18 at 3:05