How to assign list as observation in data table?
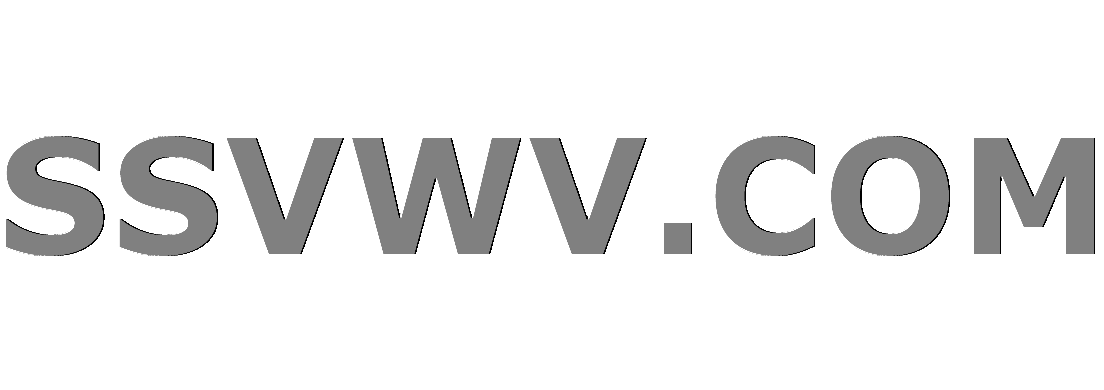
Multi tool use
up vote
3
down vote
favorite
I have a data table that contains character observations:
library(data.table)
library(stringr)
DT = data.table(strings = c('AAABD', 'BBDA', 'AACBDAA', 'ABACD'))
I would like to create a variable that contains counts of 'A', 'AA', and 'AAA' in each observation as a list. To do this I have created a function foo:
foo <- function(str) {
n <- str_count(str, 'A')
n2 <- str_count(str, 'AA')
n3 <- str_count(str, 'AAA')
df <- list('n' = n, 'n2' = n2, 'n3' = n3)
return(df)
}
I apply this function to DT to create a new variable for count observation as a list:
DT[, count := foo(strings)]
When I do this, I receive this error:
Warning message:
In `[.data.table`(DT, , `:=`(counts, foo(strings))) :
Supplied 3 items to be assigned to 4 items of column 'counts' (recycled leaving remainder of 1 items).
The data table returned has count variable lists of size 4 instead of size 3 and does not represent the amount of 'A', 'AA', and 'AAA' accurately for each string observation in variable strings
. How can I assign a list as an observation in a data table?
r dataframe data.table
add a comment |
up vote
3
down vote
favorite
I have a data table that contains character observations:
library(data.table)
library(stringr)
DT = data.table(strings = c('AAABD', 'BBDA', 'AACBDAA', 'ABACD'))
I would like to create a variable that contains counts of 'A', 'AA', and 'AAA' in each observation as a list. To do this I have created a function foo:
foo <- function(str) {
n <- str_count(str, 'A')
n2 <- str_count(str, 'AA')
n3 <- str_count(str, 'AAA')
df <- list('n' = n, 'n2' = n2, 'n3' = n3)
return(df)
}
I apply this function to DT to create a new variable for count observation as a list:
DT[, count := foo(strings)]
When I do this, I receive this error:
Warning message:
In `[.data.table`(DT, , `:=`(counts, foo(strings))) :
Supplied 3 items to be assigned to 4 items of column 'counts' (recycled leaving remainder of 1 items).
The data table returned has count variable lists of size 4 instead of size 3 and does not represent the amount of 'A', 'AA', and 'AAA' accurately for each string observation in variable strings
. How can I assign a list as an observation in a data table?
r dataframe data.table
I understand what you're trying to do. However, it is unclear to me how you want you output? Can you provide or describe your wanted output?
– Anders Ellern Bilgrau
Nov 19 at 22:12
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
I have a data table that contains character observations:
library(data.table)
library(stringr)
DT = data.table(strings = c('AAABD', 'BBDA', 'AACBDAA', 'ABACD'))
I would like to create a variable that contains counts of 'A', 'AA', and 'AAA' in each observation as a list. To do this I have created a function foo:
foo <- function(str) {
n <- str_count(str, 'A')
n2 <- str_count(str, 'AA')
n3 <- str_count(str, 'AAA')
df <- list('n' = n, 'n2' = n2, 'n3' = n3)
return(df)
}
I apply this function to DT to create a new variable for count observation as a list:
DT[, count := foo(strings)]
When I do this, I receive this error:
Warning message:
In `[.data.table`(DT, , `:=`(counts, foo(strings))) :
Supplied 3 items to be assigned to 4 items of column 'counts' (recycled leaving remainder of 1 items).
The data table returned has count variable lists of size 4 instead of size 3 and does not represent the amount of 'A', 'AA', and 'AAA' accurately for each string observation in variable strings
. How can I assign a list as an observation in a data table?
r dataframe data.table
I have a data table that contains character observations:
library(data.table)
library(stringr)
DT = data.table(strings = c('AAABD', 'BBDA', 'AACBDAA', 'ABACD'))
I would like to create a variable that contains counts of 'A', 'AA', and 'AAA' in each observation as a list. To do this I have created a function foo:
foo <- function(str) {
n <- str_count(str, 'A')
n2 <- str_count(str, 'AA')
n3 <- str_count(str, 'AAA')
df <- list('n' = n, 'n2' = n2, 'n3' = n3)
return(df)
}
I apply this function to DT to create a new variable for count observation as a list:
DT[, count := foo(strings)]
When I do this, I receive this error:
Warning message:
In `[.data.table`(DT, , `:=`(counts, foo(strings))) :
Supplied 3 items to be assigned to 4 items of column 'counts' (recycled leaving remainder of 1 items).
The data table returned has count variable lists of size 4 instead of size 3 and does not represent the amount of 'A', 'AA', and 'AAA' accurately for each string observation in variable strings
. How can I assign a list as an observation in a data table?
r dataframe data.table
r dataframe data.table
asked Nov 19 at 22:08
Avlayort
183
183
I understand what you're trying to do. However, it is unclear to me how you want you output? Can you provide or describe your wanted output?
– Anders Ellern Bilgrau
Nov 19 at 22:12
add a comment |
I understand what you're trying to do. However, it is unclear to me how you want you output? Can you provide or describe your wanted output?
– Anders Ellern Bilgrau
Nov 19 at 22:12
I understand what you're trying to do. However, it is unclear to me how you want you output? Can you provide or describe your wanted output?
– Anders Ellern Bilgrau
Nov 19 at 22:12
I understand what you're trying to do. However, it is unclear to me how you want you output? Can you provide or describe your wanted output?
– Anders Ellern Bilgrau
Nov 19 at 22:12
add a comment |
2 Answers
2
active
oldest
votes
up vote
3
down vote
accepted
You need to transpose
the list
:
foo <- function(str) {
n <- str_count(str, 'A')
n2 <- str_count(str, 'AA')
n3 <- str_count(str, 'AAA')
df <- transpose(list('n' = n, 'n2' = n2, 'n3' = n3)) # <- add transpose
return(df)
}
DT[, count := foo(strings)]
DT
# strings count
# 1: AAABD 3,1,1
# 2: BBDA 1,0,0
# 3: AACBDAA 4,2,0
# 4: ABACD 2,0,0
str(DT)
# Classes ‘data.table’ and 'data.frame': 4 obs. of 2 variables:
# $ strings: chr "AAABD" "BBDA" "AACBDAA" "ABACD"
# $ count :List of 4
# ..$ : int 3 1 1
# ..$ : int 1 0 0
# ..$ : int 4 2 0
# ..$ : int 2 0 0
Thank you, this is exactly the solution I was looking for! As a follow up question, is it possible to subset an item of the list from the data table to use in ggplot? Thank you.
– Avlayort
Nov 20 at 18:17
I would like to be able to plot the first item (or second or third) of the list incount
against another variable in my data set. In the data set I am working with, it is useful to havecount
as a list for simplicity of statistical analysis but also because it makes the data set less wieldy and easier to peruse for data exploration (I have over 100 variables in my data set). Having it as a list, however, has posed a new challenge in subsetting specific items in the list to use as a variable to plot against.
– Avlayort
Nov 20 at 19:03
2
For plotting (especially withggplot
) I think you should avoid list columns and rather keep your data tidy (tidier), with one observation per row. That said, to extract the values of a certain row, e.g. 3, as a vector:DT[ , count[[3]]]
orunlist(DT[3]$count)
.
– Henrik
Nov 20 at 19:14
Thank you for your help, your answer was able to help me determine the solution I was looking for:ggplot(data = DT, aes(x = unlist(lapply(count, '[', 1)), y = unlist(lapply(count, '[', 2)))) + geom_point()
– Avlayort
Nov 20 at 20:01
add a comment |
up vote
3
down vote
Assigning a list of columns to a single name can result in the warning message. Instead it can be
DT[, c('n', 'n2', 'n3') := .(str_count(strings, 'A'),
str_count(strings, 'AA'), str_count(strings, 'AAA'))]
1
Surely there is an existing Q&A for this? (I searched but couldn't find one)
– smci
Nov 19 at 22:46
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
You need to transpose
the list
:
foo <- function(str) {
n <- str_count(str, 'A')
n2 <- str_count(str, 'AA')
n3 <- str_count(str, 'AAA')
df <- transpose(list('n' = n, 'n2' = n2, 'n3' = n3)) # <- add transpose
return(df)
}
DT[, count := foo(strings)]
DT
# strings count
# 1: AAABD 3,1,1
# 2: BBDA 1,0,0
# 3: AACBDAA 4,2,0
# 4: ABACD 2,0,0
str(DT)
# Classes ‘data.table’ and 'data.frame': 4 obs. of 2 variables:
# $ strings: chr "AAABD" "BBDA" "AACBDAA" "ABACD"
# $ count :List of 4
# ..$ : int 3 1 1
# ..$ : int 1 0 0
# ..$ : int 4 2 0
# ..$ : int 2 0 0
Thank you, this is exactly the solution I was looking for! As a follow up question, is it possible to subset an item of the list from the data table to use in ggplot? Thank you.
– Avlayort
Nov 20 at 18:17
I would like to be able to plot the first item (or second or third) of the list incount
against another variable in my data set. In the data set I am working with, it is useful to havecount
as a list for simplicity of statistical analysis but also because it makes the data set less wieldy and easier to peruse for data exploration (I have over 100 variables in my data set). Having it as a list, however, has posed a new challenge in subsetting specific items in the list to use as a variable to plot against.
– Avlayort
Nov 20 at 19:03
2
For plotting (especially withggplot
) I think you should avoid list columns and rather keep your data tidy (tidier), with one observation per row. That said, to extract the values of a certain row, e.g. 3, as a vector:DT[ , count[[3]]]
orunlist(DT[3]$count)
.
– Henrik
Nov 20 at 19:14
Thank you for your help, your answer was able to help me determine the solution I was looking for:ggplot(data = DT, aes(x = unlist(lapply(count, '[', 1)), y = unlist(lapply(count, '[', 2)))) + geom_point()
– Avlayort
Nov 20 at 20:01
add a comment |
up vote
3
down vote
accepted
You need to transpose
the list
:
foo <- function(str) {
n <- str_count(str, 'A')
n2 <- str_count(str, 'AA')
n3 <- str_count(str, 'AAA')
df <- transpose(list('n' = n, 'n2' = n2, 'n3' = n3)) # <- add transpose
return(df)
}
DT[, count := foo(strings)]
DT
# strings count
# 1: AAABD 3,1,1
# 2: BBDA 1,0,0
# 3: AACBDAA 4,2,0
# 4: ABACD 2,0,0
str(DT)
# Classes ‘data.table’ and 'data.frame': 4 obs. of 2 variables:
# $ strings: chr "AAABD" "BBDA" "AACBDAA" "ABACD"
# $ count :List of 4
# ..$ : int 3 1 1
# ..$ : int 1 0 0
# ..$ : int 4 2 0
# ..$ : int 2 0 0
Thank you, this is exactly the solution I was looking for! As a follow up question, is it possible to subset an item of the list from the data table to use in ggplot? Thank you.
– Avlayort
Nov 20 at 18:17
I would like to be able to plot the first item (or second or third) of the list incount
against another variable in my data set. In the data set I am working with, it is useful to havecount
as a list for simplicity of statistical analysis but also because it makes the data set less wieldy and easier to peruse for data exploration (I have over 100 variables in my data set). Having it as a list, however, has posed a new challenge in subsetting specific items in the list to use as a variable to plot against.
– Avlayort
Nov 20 at 19:03
2
For plotting (especially withggplot
) I think you should avoid list columns and rather keep your data tidy (tidier), with one observation per row. That said, to extract the values of a certain row, e.g. 3, as a vector:DT[ , count[[3]]]
orunlist(DT[3]$count)
.
– Henrik
Nov 20 at 19:14
Thank you for your help, your answer was able to help me determine the solution I was looking for:ggplot(data = DT, aes(x = unlist(lapply(count, '[', 1)), y = unlist(lapply(count, '[', 2)))) + geom_point()
– Avlayort
Nov 20 at 20:01
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
You need to transpose
the list
:
foo <- function(str) {
n <- str_count(str, 'A')
n2 <- str_count(str, 'AA')
n3 <- str_count(str, 'AAA')
df <- transpose(list('n' = n, 'n2' = n2, 'n3' = n3)) # <- add transpose
return(df)
}
DT[, count := foo(strings)]
DT
# strings count
# 1: AAABD 3,1,1
# 2: BBDA 1,0,0
# 3: AACBDAA 4,2,0
# 4: ABACD 2,0,0
str(DT)
# Classes ‘data.table’ and 'data.frame': 4 obs. of 2 variables:
# $ strings: chr "AAABD" "BBDA" "AACBDAA" "ABACD"
# $ count :List of 4
# ..$ : int 3 1 1
# ..$ : int 1 0 0
# ..$ : int 4 2 0
# ..$ : int 2 0 0
You need to transpose
the list
:
foo <- function(str) {
n <- str_count(str, 'A')
n2 <- str_count(str, 'AA')
n3 <- str_count(str, 'AAA')
df <- transpose(list('n' = n, 'n2' = n2, 'n3' = n3)) # <- add transpose
return(df)
}
DT[, count := foo(strings)]
DT
# strings count
# 1: AAABD 3,1,1
# 2: BBDA 1,0,0
# 3: AACBDAA 4,2,0
# 4: ABACD 2,0,0
str(DT)
# Classes ‘data.table’ and 'data.frame': 4 obs. of 2 variables:
# $ strings: chr "AAABD" "BBDA" "AACBDAA" "ABACD"
# $ count :List of 4
# ..$ : int 3 1 1
# ..$ : int 1 0 0
# ..$ : int 4 2 0
# ..$ : int 2 0 0
edited Nov 19 at 22:52
answered Nov 19 at 22:26
Henrik
40.4k991107
40.4k991107
Thank you, this is exactly the solution I was looking for! As a follow up question, is it possible to subset an item of the list from the data table to use in ggplot? Thank you.
– Avlayort
Nov 20 at 18:17
I would like to be able to plot the first item (or second or third) of the list incount
against another variable in my data set. In the data set I am working with, it is useful to havecount
as a list for simplicity of statistical analysis but also because it makes the data set less wieldy and easier to peruse for data exploration (I have over 100 variables in my data set). Having it as a list, however, has posed a new challenge in subsetting specific items in the list to use as a variable to plot against.
– Avlayort
Nov 20 at 19:03
2
For plotting (especially withggplot
) I think you should avoid list columns and rather keep your data tidy (tidier), with one observation per row. That said, to extract the values of a certain row, e.g. 3, as a vector:DT[ , count[[3]]]
orunlist(DT[3]$count)
.
– Henrik
Nov 20 at 19:14
Thank you for your help, your answer was able to help me determine the solution I was looking for:ggplot(data = DT, aes(x = unlist(lapply(count, '[', 1)), y = unlist(lapply(count, '[', 2)))) + geom_point()
– Avlayort
Nov 20 at 20:01
add a comment |
Thank you, this is exactly the solution I was looking for! As a follow up question, is it possible to subset an item of the list from the data table to use in ggplot? Thank you.
– Avlayort
Nov 20 at 18:17
I would like to be able to plot the first item (or second or third) of the list incount
against another variable in my data set. In the data set I am working with, it is useful to havecount
as a list for simplicity of statistical analysis but also because it makes the data set less wieldy and easier to peruse for data exploration (I have over 100 variables in my data set). Having it as a list, however, has posed a new challenge in subsetting specific items in the list to use as a variable to plot against.
– Avlayort
Nov 20 at 19:03
2
For plotting (especially withggplot
) I think you should avoid list columns and rather keep your data tidy (tidier), with one observation per row. That said, to extract the values of a certain row, e.g. 3, as a vector:DT[ , count[[3]]]
orunlist(DT[3]$count)
.
– Henrik
Nov 20 at 19:14
Thank you for your help, your answer was able to help me determine the solution I was looking for:ggplot(data = DT, aes(x = unlist(lapply(count, '[', 1)), y = unlist(lapply(count, '[', 2)))) + geom_point()
– Avlayort
Nov 20 at 20:01
Thank you, this is exactly the solution I was looking for! As a follow up question, is it possible to subset an item of the list from the data table to use in ggplot? Thank you.
– Avlayort
Nov 20 at 18:17
Thank you, this is exactly the solution I was looking for! As a follow up question, is it possible to subset an item of the list from the data table to use in ggplot? Thank you.
– Avlayort
Nov 20 at 18:17
I would like to be able to plot the first item (or second or third) of the list in
count
against another variable in my data set. In the data set I am working with, it is useful to have count
as a list for simplicity of statistical analysis but also because it makes the data set less wieldy and easier to peruse for data exploration (I have over 100 variables in my data set). Having it as a list, however, has posed a new challenge in subsetting specific items in the list to use as a variable to plot against.– Avlayort
Nov 20 at 19:03
I would like to be able to plot the first item (or second or third) of the list in
count
against another variable in my data set. In the data set I am working with, it is useful to have count
as a list for simplicity of statistical analysis but also because it makes the data set less wieldy and easier to peruse for data exploration (I have over 100 variables in my data set). Having it as a list, however, has posed a new challenge in subsetting specific items in the list to use as a variable to plot against.– Avlayort
Nov 20 at 19:03
2
2
For plotting (especially with
ggplot
) I think you should avoid list columns and rather keep your data tidy (tidier), with one observation per row. That said, to extract the values of a certain row, e.g. 3, as a vector: DT[ , count[[3]]]
or unlist(DT[3]$count)
.– Henrik
Nov 20 at 19:14
For plotting (especially with
ggplot
) I think you should avoid list columns and rather keep your data tidy (tidier), with one observation per row. That said, to extract the values of a certain row, e.g. 3, as a vector: DT[ , count[[3]]]
or unlist(DT[3]$count)
.– Henrik
Nov 20 at 19:14
Thank you for your help, your answer was able to help me determine the solution I was looking for:
ggplot(data = DT, aes(x = unlist(lapply(count, '[', 1)), y = unlist(lapply(count, '[', 2)))) + geom_point()
– Avlayort
Nov 20 at 20:01
Thank you for your help, your answer was able to help me determine the solution I was looking for:
ggplot(data = DT, aes(x = unlist(lapply(count, '[', 1)), y = unlist(lapply(count, '[', 2)))) + geom_point()
– Avlayort
Nov 20 at 20:01
add a comment |
up vote
3
down vote
Assigning a list of columns to a single name can result in the warning message. Instead it can be
DT[, c('n', 'n2', 'n3') := .(str_count(strings, 'A'),
str_count(strings, 'AA'), str_count(strings, 'AAA'))]
1
Surely there is an existing Q&A for this? (I searched but couldn't find one)
– smci
Nov 19 at 22:46
add a comment |
up vote
3
down vote
Assigning a list of columns to a single name can result in the warning message. Instead it can be
DT[, c('n', 'n2', 'n3') := .(str_count(strings, 'A'),
str_count(strings, 'AA'), str_count(strings, 'AAA'))]
1
Surely there is an existing Q&A for this? (I searched but couldn't find one)
– smci
Nov 19 at 22:46
add a comment |
up vote
3
down vote
up vote
3
down vote
Assigning a list of columns to a single name can result in the warning message. Instead it can be
DT[, c('n', 'n2', 'n3') := .(str_count(strings, 'A'),
str_count(strings, 'AA'), str_count(strings, 'AAA'))]
Assigning a list of columns to a single name can result in the warning message. Instead it can be
DT[, c('n', 'n2', 'n3') := .(str_count(strings, 'A'),
str_count(strings, 'AA'), str_count(strings, 'AAA'))]
answered Nov 19 at 22:12
akrun
392k13181253
392k13181253
1
Surely there is an existing Q&A for this? (I searched but couldn't find one)
– smci
Nov 19 at 22:46
add a comment |
1
Surely there is an existing Q&A for this? (I searched but couldn't find one)
– smci
Nov 19 at 22:46
1
1
Surely there is an existing Q&A for this? (I searched but couldn't find one)
– smci
Nov 19 at 22:46
Surely there is an existing Q&A for this? (I searched but couldn't find one)
– smci
Nov 19 at 22:46
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53383344%2fhow-to-assign-list-as-observation-in-data-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
42 7qaD0,zAI 3YV,Ws g 5hk JRfarLWNjp4z2gA68VTczXd6O fR,qvqExzzOhNrrL,6d6mNFonS4Adr4d8G6OoLey JPW4YuyXA9jOT
I understand what you're trying to do. However, it is unclear to me how you want you output? Can you provide or describe your wanted output?
– Anders Ellern Bilgrau
Nov 19 at 22:12