Keep html dom manipulation on refresh
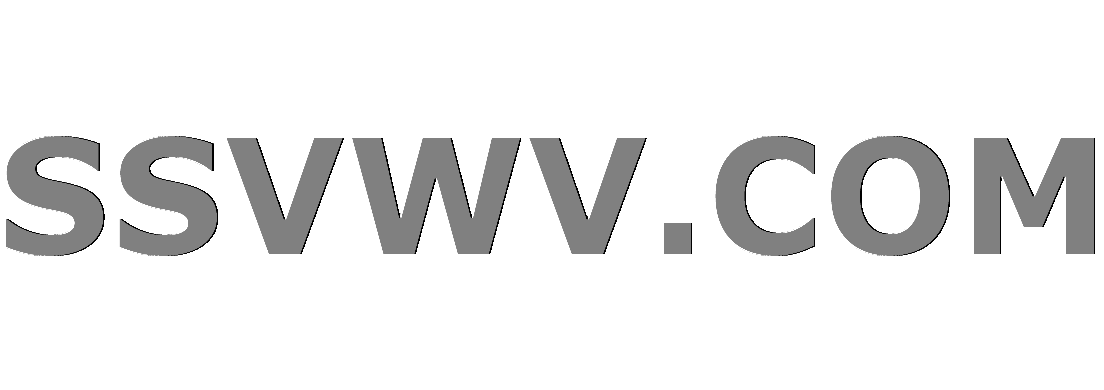
Multi tool use
I'm trying to make a comment section using just html, and javascript. I create a new dom element when the user presses post, but when you refresh, obviously it goes away. How can i make it add permanently. I'd like to do this without a database because I've never used one before.
Here is the html:
<form class="commentForm">
<textarea name="comment" id="comment" rows="6" cols="50" required="required"></textarea><br>
<button type="button" class="btn btn-dark btn-submit" style="margin-right: 25%;" onclick="postComment()">Post</button>
</form>
JS:
function postComment() {
var message = document.getElementById("comment").value;
var div = document.createElement("div");
div.style.width = "500px";
div.style.height = "50px";
div.style.color = "black";
div.innerHTML = message;
document.getElementById("comments").appendChild(div);
document.getElementById("comment").value = "";
}
javascript html5 dom-manipulation
add a comment |
I'm trying to make a comment section using just html, and javascript. I create a new dom element when the user presses post, but when you refresh, obviously it goes away. How can i make it add permanently. I'd like to do this without a database because I've never used one before.
Here is the html:
<form class="commentForm">
<textarea name="comment" id="comment" rows="6" cols="50" required="required"></textarea><br>
<button type="button" class="btn btn-dark btn-submit" style="margin-right: 25%;" onclick="postComment()">Post</button>
</form>
JS:
function postComment() {
var message = document.getElementById("comment").value;
var div = document.createElement("div");
div.style.width = "500px";
div.style.height = "50px";
div.style.color = "black";
div.innerHTML = message;
document.getElementById("comments").appendChild(div);
document.getElementById("comment").value = "";
}
javascript html5 dom-manipulation
1
You might consider using local storage for this, but really a DB/session server-side is the right way to go.
– ggorlen
Nov 24 '18 at 17:34
Any local solution, like cookies or localStorage, would only be visible to the individual -- not all users.
– j08691
Nov 24 '18 at 17:38
You can use local storage as everyone says, but why do you want to do this without a server side? I see it's a comment example and is supposed to be viewed by someone else.
– CME64
Nov 24 '18 at 17:42
add a comment |
I'm trying to make a comment section using just html, and javascript. I create a new dom element when the user presses post, but when you refresh, obviously it goes away. How can i make it add permanently. I'd like to do this without a database because I've never used one before.
Here is the html:
<form class="commentForm">
<textarea name="comment" id="comment" rows="6" cols="50" required="required"></textarea><br>
<button type="button" class="btn btn-dark btn-submit" style="margin-right: 25%;" onclick="postComment()">Post</button>
</form>
JS:
function postComment() {
var message = document.getElementById("comment").value;
var div = document.createElement("div");
div.style.width = "500px";
div.style.height = "50px";
div.style.color = "black";
div.innerHTML = message;
document.getElementById("comments").appendChild(div);
document.getElementById("comment").value = "";
}
javascript html5 dom-manipulation
I'm trying to make a comment section using just html, and javascript. I create a new dom element when the user presses post, but when you refresh, obviously it goes away. How can i make it add permanently. I'd like to do this without a database because I've never used one before.
Here is the html:
<form class="commentForm">
<textarea name="comment" id="comment" rows="6" cols="50" required="required"></textarea><br>
<button type="button" class="btn btn-dark btn-submit" style="margin-right: 25%;" onclick="postComment()">Post</button>
</form>
JS:
function postComment() {
var message = document.getElementById("comment").value;
var div = document.createElement("div");
div.style.width = "500px";
div.style.height = "50px";
div.style.color = "black";
div.innerHTML = message;
document.getElementById("comments").appendChild(div);
document.getElementById("comment").value = "";
}
javascript html5 dom-manipulation
javascript html5 dom-manipulation
asked Nov 24 '18 at 17:21


Ibrahim FadelIbrahim Fadel
948
948
1
You might consider using local storage for this, but really a DB/session server-side is the right way to go.
– ggorlen
Nov 24 '18 at 17:34
Any local solution, like cookies or localStorage, would only be visible to the individual -- not all users.
– j08691
Nov 24 '18 at 17:38
You can use local storage as everyone says, but why do you want to do this without a server side? I see it's a comment example and is supposed to be viewed by someone else.
– CME64
Nov 24 '18 at 17:42
add a comment |
1
You might consider using local storage for this, but really a DB/session server-side is the right way to go.
– ggorlen
Nov 24 '18 at 17:34
Any local solution, like cookies or localStorage, would only be visible to the individual -- not all users.
– j08691
Nov 24 '18 at 17:38
You can use local storage as everyone says, but why do you want to do this without a server side? I see it's a comment example and is supposed to be viewed by someone else.
– CME64
Nov 24 '18 at 17:42
1
1
You might consider using local storage for this, but really a DB/session server-side is the right way to go.
– ggorlen
Nov 24 '18 at 17:34
You might consider using local storage for this, but really a DB/session server-side is the right way to go.
– ggorlen
Nov 24 '18 at 17:34
Any local solution, like cookies or localStorage, would only be visible to the individual -- not all users.
– j08691
Nov 24 '18 at 17:38
Any local solution, like cookies or localStorage, would only be visible to the individual -- not all users.
– j08691
Nov 24 '18 at 17:38
You can use local storage as everyone says, but why do you want to do this without a server side? I see it's a comment example and is supposed to be viewed by someone else.
– CME64
Nov 24 '18 at 17:42
You can use local storage as everyone says, but why do you want to do this without a server side? I see it's a comment example and is supposed to be viewed by someone else.
– CME64
Nov 24 '18 at 17:42
add a comment |
2 Answers
2
active
oldest
votes
Short answer: use localStorage
.
Create a function with only purpose to add one div based on a value in your comments:
function addNewDiv (message) {
var div = document.createElement('div');
div.style.width = '500px';
div.style.height = '50px';
div.style.color = 'black';
div.innerHTML = message;
document.getElementById('comments').appendChild(div);
};
To manipulate localStorage, you should use getItem
and setItem
methods like this:
function saveItem(message) {
var comments = JSON.parse(localStorage.getItem('comments') || '');
comments.push(message);
localStorage.setItem('comments', JSON.stringify(comments));
}
Now, update your current function to use those functions above:
function postComment() {
var message = document.getElementById('comment').value;
addNewDiv(message);
saveItem(message);
document.getElementById('comment').value = '';
}
All your comments are saved. To load them on F5-refresh, use this script after your HTML has been rendered:
JSON.parse(localStorage.getItem('comments') || '').forEach(addNewDiv);
You are all done, to clear the localStorage, use:
localStorage.clear();
Or:
localStorage.removeItem('comments');
Hope it helps.
This might sound stupid, but where do i call the postComment function. How does this all tie togethor?
– Ibrahim Fadel
Nov 24 '18 at 17:44
It's ok i figured it out. Thanks
– Ibrahim Fadel
Nov 24 '18 at 17:47
add a comment |
You could use cookies to store the text area data. Hope this helps. The timer below reads the text box every 5 seconds so it will auto store the data.
setInterval(timer, 5000);
var com = document.getElementById("comment");
function timer() {
var text = com.innerHTML;
document.cookie = "text_area="+text;
}
window.onload = function(){
if(readCookie("text_area")){
com.innerHTML = readCookie("text_area");
}
}
//Read cookies reads all cookies and returns the value from name variable
function readCookie(name) {
var nameEQ = name + "=";
var ca = document.cookie.split(';');
for(var i=0;i < ca.length;i++) {
var c = ca[i];
while (c.charAt(0)==' ') c = c.substring(1,c.length);
if (c.indexOf(nameEQ) == 0) return c.substring(nameEQ.length,c.length);
}
return null;
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53460615%2fkeep-html-dom-manipulation-on-refresh%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Short answer: use localStorage
.
Create a function with only purpose to add one div based on a value in your comments:
function addNewDiv (message) {
var div = document.createElement('div');
div.style.width = '500px';
div.style.height = '50px';
div.style.color = 'black';
div.innerHTML = message;
document.getElementById('comments').appendChild(div);
};
To manipulate localStorage, you should use getItem
and setItem
methods like this:
function saveItem(message) {
var comments = JSON.parse(localStorage.getItem('comments') || '');
comments.push(message);
localStorage.setItem('comments', JSON.stringify(comments));
}
Now, update your current function to use those functions above:
function postComment() {
var message = document.getElementById('comment').value;
addNewDiv(message);
saveItem(message);
document.getElementById('comment').value = '';
}
All your comments are saved. To load them on F5-refresh, use this script after your HTML has been rendered:
JSON.parse(localStorage.getItem('comments') || '').forEach(addNewDiv);
You are all done, to clear the localStorage, use:
localStorage.clear();
Or:
localStorage.removeItem('comments');
Hope it helps.
This might sound stupid, but where do i call the postComment function. How does this all tie togethor?
– Ibrahim Fadel
Nov 24 '18 at 17:44
It's ok i figured it out. Thanks
– Ibrahim Fadel
Nov 24 '18 at 17:47
add a comment |
Short answer: use localStorage
.
Create a function with only purpose to add one div based on a value in your comments:
function addNewDiv (message) {
var div = document.createElement('div');
div.style.width = '500px';
div.style.height = '50px';
div.style.color = 'black';
div.innerHTML = message;
document.getElementById('comments').appendChild(div);
};
To manipulate localStorage, you should use getItem
and setItem
methods like this:
function saveItem(message) {
var comments = JSON.parse(localStorage.getItem('comments') || '');
comments.push(message);
localStorage.setItem('comments', JSON.stringify(comments));
}
Now, update your current function to use those functions above:
function postComment() {
var message = document.getElementById('comment').value;
addNewDiv(message);
saveItem(message);
document.getElementById('comment').value = '';
}
All your comments are saved. To load them on F5-refresh, use this script after your HTML has been rendered:
JSON.parse(localStorage.getItem('comments') || '').forEach(addNewDiv);
You are all done, to clear the localStorage, use:
localStorage.clear();
Or:
localStorage.removeItem('comments');
Hope it helps.
This might sound stupid, but where do i call the postComment function. How does this all tie togethor?
– Ibrahim Fadel
Nov 24 '18 at 17:44
It's ok i figured it out. Thanks
– Ibrahim Fadel
Nov 24 '18 at 17:47
add a comment |
Short answer: use localStorage
.
Create a function with only purpose to add one div based on a value in your comments:
function addNewDiv (message) {
var div = document.createElement('div');
div.style.width = '500px';
div.style.height = '50px';
div.style.color = 'black';
div.innerHTML = message;
document.getElementById('comments').appendChild(div);
};
To manipulate localStorage, you should use getItem
and setItem
methods like this:
function saveItem(message) {
var comments = JSON.parse(localStorage.getItem('comments') || '');
comments.push(message);
localStorage.setItem('comments', JSON.stringify(comments));
}
Now, update your current function to use those functions above:
function postComment() {
var message = document.getElementById('comment').value;
addNewDiv(message);
saveItem(message);
document.getElementById('comment').value = '';
}
All your comments are saved. To load them on F5-refresh, use this script after your HTML has been rendered:
JSON.parse(localStorage.getItem('comments') || '').forEach(addNewDiv);
You are all done, to clear the localStorage, use:
localStorage.clear();
Or:
localStorage.removeItem('comments');
Hope it helps.
Short answer: use localStorage
.
Create a function with only purpose to add one div based on a value in your comments:
function addNewDiv (message) {
var div = document.createElement('div');
div.style.width = '500px';
div.style.height = '50px';
div.style.color = 'black';
div.innerHTML = message;
document.getElementById('comments').appendChild(div);
};
To manipulate localStorage, you should use getItem
and setItem
methods like this:
function saveItem(message) {
var comments = JSON.parse(localStorage.getItem('comments') || '');
comments.push(message);
localStorage.setItem('comments', JSON.stringify(comments));
}
Now, update your current function to use those functions above:
function postComment() {
var message = document.getElementById('comment').value;
addNewDiv(message);
saveItem(message);
document.getElementById('comment').value = '';
}
All your comments are saved. To load them on F5-refresh, use this script after your HTML has been rendered:
JSON.parse(localStorage.getItem('comments') || '').forEach(addNewDiv);
You are all done, to clear the localStorage, use:
localStorage.clear();
Or:
localStorage.removeItem('comments');
Hope it helps.
answered Nov 24 '18 at 17:36


Washington GuedesWashington Guedes
2,47711331
2,47711331
This might sound stupid, but where do i call the postComment function. How does this all tie togethor?
– Ibrahim Fadel
Nov 24 '18 at 17:44
It's ok i figured it out. Thanks
– Ibrahim Fadel
Nov 24 '18 at 17:47
add a comment |
This might sound stupid, but where do i call the postComment function. How does this all tie togethor?
– Ibrahim Fadel
Nov 24 '18 at 17:44
It's ok i figured it out. Thanks
– Ibrahim Fadel
Nov 24 '18 at 17:47
This might sound stupid, but where do i call the postComment function. How does this all tie togethor?
– Ibrahim Fadel
Nov 24 '18 at 17:44
This might sound stupid, but where do i call the postComment function. How does this all tie togethor?
– Ibrahim Fadel
Nov 24 '18 at 17:44
It's ok i figured it out. Thanks
– Ibrahim Fadel
Nov 24 '18 at 17:47
It's ok i figured it out. Thanks
– Ibrahim Fadel
Nov 24 '18 at 17:47
add a comment |
You could use cookies to store the text area data. Hope this helps. The timer below reads the text box every 5 seconds so it will auto store the data.
setInterval(timer, 5000);
var com = document.getElementById("comment");
function timer() {
var text = com.innerHTML;
document.cookie = "text_area="+text;
}
window.onload = function(){
if(readCookie("text_area")){
com.innerHTML = readCookie("text_area");
}
}
//Read cookies reads all cookies and returns the value from name variable
function readCookie(name) {
var nameEQ = name + "=";
var ca = document.cookie.split(';');
for(var i=0;i < ca.length;i++) {
var c = ca[i];
while (c.charAt(0)==' ') c = c.substring(1,c.length);
if (c.indexOf(nameEQ) == 0) return c.substring(nameEQ.length,c.length);
}
return null;
}
add a comment |
You could use cookies to store the text area data. Hope this helps. The timer below reads the text box every 5 seconds so it will auto store the data.
setInterval(timer, 5000);
var com = document.getElementById("comment");
function timer() {
var text = com.innerHTML;
document.cookie = "text_area="+text;
}
window.onload = function(){
if(readCookie("text_area")){
com.innerHTML = readCookie("text_area");
}
}
//Read cookies reads all cookies and returns the value from name variable
function readCookie(name) {
var nameEQ = name + "=";
var ca = document.cookie.split(';');
for(var i=0;i < ca.length;i++) {
var c = ca[i];
while (c.charAt(0)==' ') c = c.substring(1,c.length);
if (c.indexOf(nameEQ) == 0) return c.substring(nameEQ.length,c.length);
}
return null;
}
add a comment |
You could use cookies to store the text area data. Hope this helps. The timer below reads the text box every 5 seconds so it will auto store the data.
setInterval(timer, 5000);
var com = document.getElementById("comment");
function timer() {
var text = com.innerHTML;
document.cookie = "text_area="+text;
}
window.onload = function(){
if(readCookie("text_area")){
com.innerHTML = readCookie("text_area");
}
}
//Read cookies reads all cookies and returns the value from name variable
function readCookie(name) {
var nameEQ = name + "=";
var ca = document.cookie.split(';');
for(var i=0;i < ca.length;i++) {
var c = ca[i];
while (c.charAt(0)==' ') c = c.substring(1,c.length);
if (c.indexOf(nameEQ) == 0) return c.substring(nameEQ.length,c.length);
}
return null;
}
You could use cookies to store the text area data. Hope this helps. The timer below reads the text box every 5 seconds so it will auto store the data.
setInterval(timer, 5000);
var com = document.getElementById("comment");
function timer() {
var text = com.innerHTML;
document.cookie = "text_area="+text;
}
window.onload = function(){
if(readCookie("text_area")){
com.innerHTML = readCookie("text_area");
}
}
//Read cookies reads all cookies and returns the value from name variable
function readCookie(name) {
var nameEQ = name + "=";
var ca = document.cookie.split(';');
for(var i=0;i < ca.length;i++) {
var c = ca[i];
while (c.charAt(0)==' ') c = c.substring(1,c.length);
if (c.indexOf(nameEQ) == 0) return c.substring(nameEQ.length,c.length);
}
return null;
}
answered Nov 24 '18 at 17:37


Martin BradleyMartin Bradley
212
212
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53460615%2fkeep-html-dom-manipulation-on-refresh%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
d,PhwV6JRbJCbX34hKhtJJt05IWIVZ5GQ1s,Dm48MlFZ3A,CFh2W,JdpcSIU01EDO3o m3 MjcSihLnA
1
You might consider using local storage for this, but really a DB/session server-side is the right way to go.
– ggorlen
Nov 24 '18 at 17:34
Any local solution, like cookies or localStorage, would only be visible to the individual -- not all users.
– j08691
Nov 24 '18 at 17:38
You can use local storage as everyone says, but why do you want to do this without a server side? I see it's a comment example and is supposed to be viewed by someone else.
– CME64
Nov 24 '18 at 17:42