access files from s3 bucket on client side meteor.js
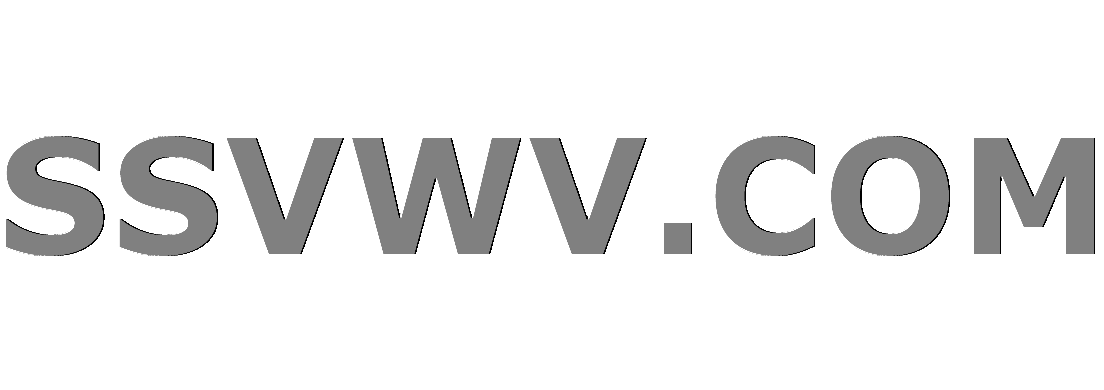
Multi tool use
up vote
0
down vote
favorite
So I am struggling getting images from an s3 bucket to show up client side in a meteor app. I am using aws-sdk node package. I am getting the objects in my console on the server side and if I change my bucket policy to public it works as well. The fact that the objects show up in the console on startup but are not showing on the client side make me think it is a meteor problem I am just having trouble finding good documentation on the subject.
server.js
Meteor.startup(() => {
AWS.config.update({
accessKeyId: Meteor.settings.AWS.S3.accessId,
secretAccessKey: Meteor.settings.AWS.S3.secret
});
s3 = new AWS.S3({
region: "<my-region>"
});
var params = {
Bucket: "<my-bucket-name>"
};
s3.listObjects(
params,
Meteor.bindEnvironment(function(err, data) {
if (err) throw err;
console.log(data);
})
);
})
Here is my bucket policy( when I set the resource to * it works but I want the resource set to a specific IAM account):
{
"Version": "2008-10-17",
"Id": "Policy-some-number",
"Statement": [
{
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::<current-iam-account-in>"
},
"Action": "s3:GetObject",
"Resource": "arn:aws:s3:::<bucket-name>/*"
},
{
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::<current-iam-account-in>"
},
"Action": "s3:PutObject",
"Resource": "arn:aws:s3:::<bucket-name>/*"
},
{
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::<current-iam-account-in>"
},
"Action": "s3:ListBucket",
"Resource": "arn:aws:s3:::<bucket-name>"
}
]
}
node.js meteor amazon-s3
add a comment |
up vote
0
down vote
favorite
So I am struggling getting images from an s3 bucket to show up client side in a meteor app. I am using aws-sdk node package. I am getting the objects in my console on the server side and if I change my bucket policy to public it works as well. The fact that the objects show up in the console on startup but are not showing on the client side make me think it is a meteor problem I am just having trouble finding good documentation on the subject.
server.js
Meteor.startup(() => {
AWS.config.update({
accessKeyId: Meteor.settings.AWS.S3.accessId,
secretAccessKey: Meteor.settings.AWS.S3.secret
});
s3 = new AWS.S3({
region: "<my-region>"
});
var params = {
Bucket: "<my-bucket-name>"
};
s3.listObjects(
params,
Meteor.bindEnvironment(function(err, data) {
if (err) throw err;
console.log(data);
})
);
})
Here is my bucket policy( when I set the resource to * it works but I want the resource set to a specific IAM account):
{
"Version": "2008-10-17",
"Id": "Policy-some-number",
"Statement": [
{
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::<current-iam-account-in>"
},
"Action": "s3:GetObject",
"Resource": "arn:aws:s3:::<bucket-name>/*"
},
{
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::<current-iam-account-in>"
},
"Action": "s3:PutObject",
"Resource": "arn:aws:s3:::<bucket-name>/*"
},
{
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::<current-iam-account-in>"
},
"Action": "s3:ListBucket",
"Resource": "arn:aws:s3:::<bucket-name>"
}
]
}
node.js meteor amazon-s3
Can you add the client code that is used to display the fetched images?
– Jankapunkt
Nov 20 at 8:03
@jankapunkt right now the code that displays the images is the s3 url that is saved in our db
– Kris
Nov 20 at 17:10
I am referring to the code that receives this URL and renders the image using the front end rendering engine of your choice
– Jankapunkt
Nov 20 at 17:12
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
So I am struggling getting images from an s3 bucket to show up client side in a meteor app. I am using aws-sdk node package. I am getting the objects in my console on the server side and if I change my bucket policy to public it works as well. The fact that the objects show up in the console on startup but are not showing on the client side make me think it is a meteor problem I am just having trouble finding good documentation on the subject.
server.js
Meteor.startup(() => {
AWS.config.update({
accessKeyId: Meteor.settings.AWS.S3.accessId,
secretAccessKey: Meteor.settings.AWS.S3.secret
});
s3 = new AWS.S3({
region: "<my-region>"
});
var params = {
Bucket: "<my-bucket-name>"
};
s3.listObjects(
params,
Meteor.bindEnvironment(function(err, data) {
if (err) throw err;
console.log(data);
})
);
})
Here is my bucket policy( when I set the resource to * it works but I want the resource set to a specific IAM account):
{
"Version": "2008-10-17",
"Id": "Policy-some-number",
"Statement": [
{
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::<current-iam-account-in>"
},
"Action": "s3:GetObject",
"Resource": "arn:aws:s3:::<bucket-name>/*"
},
{
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::<current-iam-account-in>"
},
"Action": "s3:PutObject",
"Resource": "arn:aws:s3:::<bucket-name>/*"
},
{
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::<current-iam-account-in>"
},
"Action": "s3:ListBucket",
"Resource": "arn:aws:s3:::<bucket-name>"
}
]
}
node.js meteor amazon-s3
So I am struggling getting images from an s3 bucket to show up client side in a meteor app. I am using aws-sdk node package. I am getting the objects in my console on the server side and if I change my bucket policy to public it works as well. The fact that the objects show up in the console on startup but are not showing on the client side make me think it is a meteor problem I am just having trouble finding good documentation on the subject.
server.js
Meteor.startup(() => {
AWS.config.update({
accessKeyId: Meteor.settings.AWS.S3.accessId,
secretAccessKey: Meteor.settings.AWS.S3.secret
});
s3 = new AWS.S3({
region: "<my-region>"
});
var params = {
Bucket: "<my-bucket-name>"
};
s3.listObjects(
params,
Meteor.bindEnvironment(function(err, data) {
if (err) throw err;
console.log(data);
})
);
})
Here is my bucket policy( when I set the resource to * it works but I want the resource set to a specific IAM account):
{
"Version": "2008-10-17",
"Id": "Policy-some-number",
"Statement": [
{
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::<current-iam-account-in>"
},
"Action": "s3:GetObject",
"Resource": "arn:aws:s3:::<bucket-name>/*"
},
{
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::<current-iam-account-in>"
},
"Action": "s3:PutObject",
"Resource": "arn:aws:s3:::<bucket-name>/*"
},
{
"Effect": "Allow",
"Principal": {
"AWS": "arn:aws:iam::<current-iam-account-in>"
},
"Action": "s3:ListBucket",
"Resource": "arn:aws:s3:::<bucket-name>"
}
]
}
node.js meteor amazon-s3
node.js meteor amazon-s3
asked Nov 20 at 0:15
Kris
315
315
Can you add the client code that is used to display the fetched images?
– Jankapunkt
Nov 20 at 8:03
@jankapunkt right now the code that displays the images is the s3 url that is saved in our db
– Kris
Nov 20 at 17:10
I am referring to the code that receives this URL and renders the image using the front end rendering engine of your choice
– Jankapunkt
Nov 20 at 17:12
add a comment |
Can you add the client code that is used to display the fetched images?
– Jankapunkt
Nov 20 at 8:03
@jankapunkt right now the code that displays the images is the s3 url that is saved in our db
– Kris
Nov 20 at 17:10
I am referring to the code that receives this URL and renders the image using the front end rendering engine of your choice
– Jankapunkt
Nov 20 at 17:12
Can you add the client code that is used to display the fetched images?
– Jankapunkt
Nov 20 at 8:03
Can you add the client code that is used to display the fetched images?
– Jankapunkt
Nov 20 at 8:03
@jankapunkt right now the code that displays the images is the s3 url that is saved in our db
– Kris
Nov 20 at 17:10
@jankapunkt right now the code that displays the images is the s3 url that is saved in our db
– Kris
Nov 20 at 17:10
I am referring to the code that receives this URL and renders the image using the front end rendering engine of your choice
– Jankapunkt
Nov 20 at 17:12
I am referring to the code that receives this URL and renders the image using the front end rendering engine of your choice
– Jankapunkt
Nov 20 at 17:12
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384458%2faccess-files-from-s3-bucket-on-client-side-meteor-js%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2I5ti97WSz,alxKKUUlWxIwKux7 JC1Esf3
Can you add the client code that is used to display the fetched images?
– Jankapunkt
Nov 20 at 8:03
@jankapunkt right now the code that displays the images is the s3 url that is saved in our db
– Kris
Nov 20 at 17:10
I am referring to the code that receives this URL and renders the image using the front end rendering engine of your choice
– Jankapunkt
Nov 20 at 17:12