custom listview has stopped android studio 2.3.3 version
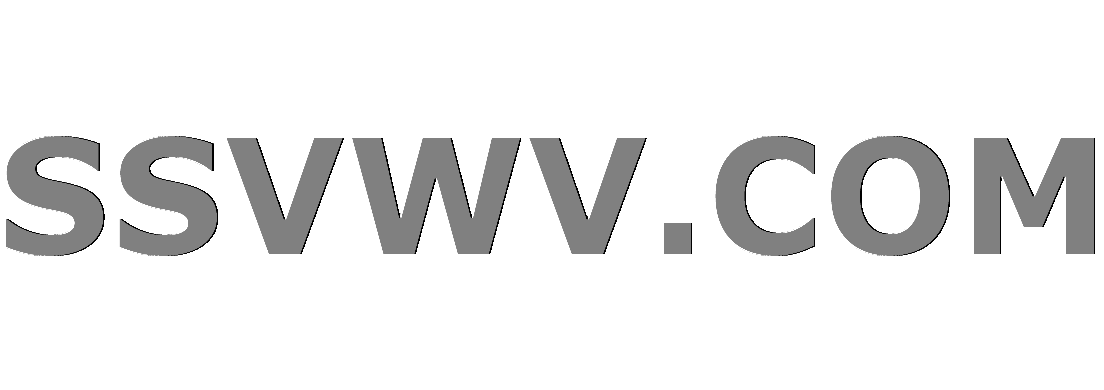
Multi tool use
I want to know why it tells me: "custom listview has stopped"? How can I solve it out?
This is my code in the Main Activity
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layoutDirection="rtl"
android:padding="16dp"
android:orientation="vertical"
tools:context="customlistviews.oxbir.com.customlistview.ActivityMain">
<EditText
android:id="@+id/editTitle"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btnAdd"
android:text="Add Row"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<ListView
android:id="@+id/listView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal" />
</LinearLayout>
ActivityMain.java
package customlistviews.oxbir.com.customlistview;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import java.util.ArrayList;
public class ActivityMain extends AppCompatActivity {
Button btnAdd;
EditText editTitle;
ArrayList<News> newsArrayList;
ListView listView = (ListView)findViewById(R.id.listView);
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editTitle = (EditText)findViewById(R.id.editTitle);
btnAdd = (Button)findViewById(R.id.btnAdd);
newsArrayList = new ArrayList<>();
final NewsAdapter newsAdapter = new NewsAdapter(ActivityMain.this, R.layout.activity_customnews, newsArrayList);
listView.setAdapter(newsAdapter);
btnAdd.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
newsArrayList.add(new News(R.drawable.newsletter, editTitle.getText().toString()));
newsAdapter.notifyDataSetChanged();
}
});
}
}
activity_customnews.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
android:layoutDirection="rtl">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:id="@+id/imageNews"
android:layout_width="100dp"
android:layout_height="100dp"
android:src="@drawable/newsletter" />
<TextView
android:id="@+id/txtTitle"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textAlignment="center"
android:layout_gravity="center"
android:textSize="20dp"
android:text="Title" />
</LinearLayout>
</LinearLayout>
News.java
package customlistviews.oxbir.com.customlistview;
public class News {
public int imageNews;
public String titleNews;
public News (int imageNews, String titleNews) {
this.imageNews = imageNews;
this.titleNews = titleNews;
}
}
NewsAdapter.java
package customlistviews.oxbir.com.customlistview;
import android.app.Activity;
import android.media.Image;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import java.util.ArrayList;
public class NewsAdapter extends ArrayAdapter {
public int resourceID;
public Activity activity;
public ArrayList<News> data;
public NewsAdapter(Activity activity, int resourceID, ArrayList<News> object) {
super(activity, resourceID, object);
this.resourceID = resourceID;
this.activity = activity;
this.data = object;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View view = convertView;
view = this.activity.getLayoutInflater().inflate(this.resourceID, null);
ImageView imageView = (ImageView) view.findViewById(R.id.imageNews);
TextView txtTitle = (TextView) view.findViewById(R.id.txtTitle);
News news = data.get(position);
imageView.setImageResource(R.drawable.newsletter);
txtTitle.setText(news.titleNews);
return view;
}
}
Right now, I'm just trying to write something in a text field, and after every time I click on the button, I will display a photo and text that appears in my listview.
java

add a comment |
I want to know why it tells me: "custom listview has stopped"? How can I solve it out?
This is my code in the Main Activity
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layoutDirection="rtl"
android:padding="16dp"
android:orientation="vertical"
tools:context="customlistviews.oxbir.com.customlistview.ActivityMain">
<EditText
android:id="@+id/editTitle"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btnAdd"
android:text="Add Row"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<ListView
android:id="@+id/listView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal" />
</LinearLayout>
ActivityMain.java
package customlistviews.oxbir.com.customlistview;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import java.util.ArrayList;
public class ActivityMain extends AppCompatActivity {
Button btnAdd;
EditText editTitle;
ArrayList<News> newsArrayList;
ListView listView = (ListView)findViewById(R.id.listView);
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editTitle = (EditText)findViewById(R.id.editTitle);
btnAdd = (Button)findViewById(R.id.btnAdd);
newsArrayList = new ArrayList<>();
final NewsAdapter newsAdapter = new NewsAdapter(ActivityMain.this, R.layout.activity_customnews, newsArrayList);
listView.setAdapter(newsAdapter);
btnAdd.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
newsArrayList.add(new News(R.drawable.newsletter, editTitle.getText().toString()));
newsAdapter.notifyDataSetChanged();
}
});
}
}
activity_customnews.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
android:layoutDirection="rtl">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:id="@+id/imageNews"
android:layout_width="100dp"
android:layout_height="100dp"
android:src="@drawable/newsletter" />
<TextView
android:id="@+id/txtTitle"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textAlignment="center"
android:layout_gravity="center"
android:textSize="20dp"
android:text="Title" />
</LinearLayout>
</LinearLayout>
News.java
package customlistviews.oxbir.com.customlistview;
public class News {
public int imageNews;
public String titleNews;
public News (int imageNews, String titleNews) {
this.imageNews = imageNews;
this.titleNews = titleNews;
}
}
NewsAdapter.java
package customlistviews.oxbir.com.customlistview;
import android.app.Activity;
import android.media.Image;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import java.util.ArrayList;
public class NewsAdapter extends ArrayAdapter {
public int resourceID;
public Activity activity;
public ArrayList<News> data;
public NewsAdapter(Activity activity, int resourceID, ArrayList<News> object) {
super(activity, resourceID, object);
this.resourceID = resourceID;
this.activity = activity;
this.data = object;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View view = convertView;
view = this.activity.getLayoutInflater().inflate(this.resourceID, null);
ImageView imageView = (ImageView) view.findViewById(R.id.imageNews);
TextView txtTitle = (TextView) view.findViewById(R.id.txtTitle);
News news = data.get(position);
imageView.setImageResource(R.drawable.newsletter);
txtTitle.setText(news.titleNews);
return view;
}
}
Right now, I'm just trying to write something in a text field, and after every time I click on the button, I will display a photo and text that appears in my listview.
java

Look at the stack trace to determine the cause of the crash.
– Mike M.
Nov 20 at 20:02
ok how chabge my codes canyou help me
– Mahmoud Khosravi
Nov 20 at 20:17
You need to learn to use logcat, among other debugging skills. Otherwise, you're going to posting a question every time something goes wrong. Please follow the link I provided above.
– Mike M.
Nov 20 at 20:20
add a comment |
I want to know why it tells me: "custom listview has stopped"? How can I solve it out?
This is my code in the Main Activity
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layoutDirection="rtl"
android:padding="16dp"
android:orientation="vertical"
tools:context="customlistviews.oxbir.com.customlistview.ActivityMain">
<EditText
android:id="@+id/editTitle"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btnAdd"
android:text="Add Row"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<ListView
android:id="@+id/listView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal" />
</LinearLayout>
ActivityMain.java
package customlistviews.oxbir.com.customlistview;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import java.util.ArrayList;
public class ActivityMain extends AppCompatActivity {
Button btnAdd;
EditText editTitle;
ArrayList<News> newsArrayList;
ListView listView = (ListView)findViewById(R.id.listView);
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editTitle = (EditText)findViewById(R.id.editTitle);
btnAdd = (Button)findViewById(R.id.btnAdd);
newsArrayList = new ArrayList<>();
final NewsAdapter newsAdapter = new NewsAdapter(ActivityMain.this, R.layout.activity_customnews, newsArrayList);
listView.setAdapter(newsAdapter);
btnAdd.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
newsArrayList.add(new News(R.drawable.newsletter, editTitle.getText().toString()));
newsAdapter.notifyDataSetChanged();
}
});
}
}
activity_customnews.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
android:layoutDirection="rtl">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:id="@+id/imageNews"
android:layout_width="100dp"
android:layout_height="100dp"
android:src="@drawable/newsletter" />
<TextView
android:id="@+id/txtTitle"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textAlignment="center"
android:layout_gravity="center"
android:textSize="20dp"
android:text="Title" />
</LinearLayout>
</LinearLayout>
News.java
package customlistviews.oxbir.com.customlistview;
public class News {
public int imageNews;
public String titleNews;
public News (int imageNews, String titleNews) {
this.imageNews = imageNews;
this.titleNews = titleNews;
}
}
NewsAdapter.java
package customlistviews.oxbir.com.customlistview;
import android.app.Activity;
import android.media.Image;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import java.util.ArrayList;
public class NewsAdapter extends ArrayAdapter {
public int resourceID;
public Activity activity;
public ArrayList<News> data;
public NewsAdapter(Activity activity, int resourceID, ArrayList<News> object) {
super(activity, resourceID, object);
this.resourceID = resourceID;
this.activity = activity;
this.data = object;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View view = convertView;
view = this.activity.getLayoutInflater().inflate(this.resourceID, null);
ImageView imageView = (ImageView) view.findViewById(R.id.imageNews);
TextView txtTitle = (TextView) view.findViewById(R.id.txtTitle);
News news = data.get(position);
imageView.setImageResource(R.drawable.newsletter);
txtTitle.setText(news.titleNews);
return view;
}
}
Right now, I'm just trying to write something in a text field, and after every time I click on the button, I will display a photo and text that appears in my listview.
java

I want to know why it tells me: "custom listview has stopped"? How can I solve it out?
This is my code in the Main Activity
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layoutDirection="rtl"
android:padding="16dp"
android:orientation="vertical"
tools:context="customlistviews.oxbir.com.customlistview.ActivityMain">
<EditText
android:id="@+id/editTitle"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btnAdd"
android:text="Add Row"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<ListView
android:id="@+id/listView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal" />
</LinearLayout>
ActivityMain.java
package customlistviews.oxbir.com.customlistview;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import java.util.ArrayList;
public class ActivityMain extends AppCompatActivity {
Button btnAdd;
EditText editTitle;
ArrayList<News> newsArrayList;
ListView listView = (ListView)findViewById(R.id.listView);
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editTitle = (EditText)findViewById(R.id.editTitle);
btnAdd = (Button)findViewById(R.id.btnAdd);
newsArrayList = new ArrayList<>();
final NewsAdapter newsAdapter = new NewsAdapter(ActivityMain.this, R.layout.activity_customnews, newsArrayList);
listView.setAdapter(newsAdapter);
btnAdd.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
newsArrayList.add(new News(R.drawable.newsletter, editTitle.getText().toString()));
newsAdapter.notifyDataSetChanged();
}
});
}
}
activity_customnews.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
android:layoutDirection="rtl">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:id="@+id/imageNews"
android:layout_width="100dp"
android:layout_height="100dp"
android:src="@drawable/newsletter" />
<TextView
android:id="@+id/txtTitle"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textAlignment="center"
android:layout_gravity="center"
android:textSize="20dp"
android:text="Title" />
</LinearLayout>
</LinearLayout>
News.java
package customlistviews.oxbir.com.customlistview;
public class News {
public int imageNews;
public String titleNews;
public News (int imageNews, String titleNews) {
this.imageNews = imageNews;
this.titleNews = titleNews;
}
}
NewsAdapter.java
package customlistviews.oxbir.com.customlistview;
import android.app.Activity;
import android.media.Image;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import java.util.ArrayList;
public class NewsAdapter extends ArrayAdapter {
public int resourceID;
public Activity activity;
public ArrayList<News> data;
public NewsAdapter(Activity activity, int resourceID, ArrayList<News> object) {
super(activity, resourceID, object);
this.resourceID = resourceID;
this.activity = activity;
this.data = object;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View view = convertView;
view = this.activity.getLayoutInflater().inflate(this.resourceID, null);
ImageView imageView = (ImageView) view.findViewById(R.id.imageNews);
TextView txtTitle = (TextView) view.findViewById(R.id.txtTitle);
News news = data.get(position);
imageView.setImageResource(R.drawable.newsletter);
txtTitle.setText(news.titleNews);
return view;
}
}
Right now, I'm just trying to write something in a text field, and after every time I click on the button, I will display a photo and text that appears in my listview.
java

java

asked Nov 20 at 20:00


Mahmoud Khosravi
177
177
Look at the stack trace to determine the cause of the crash.
– Mike M.
Nov 20 at 20:02
ok how chabge my codes canyou help me
– Mahmoud Khosravi
Nov 20 at 20:17
You need to learn to use logcat, among other debugging skills. Otherwise, you're going to posting a question every time something goes wrong. Please follow the link I provided above.
– Mike M.
Nov 20 at 20:20
add a comment |
Look at the stack trace to determine the cause of the crash.
– Mike M.
Nov 20 at 20:02
ok how chabge my codes canyou help me
– Mahmoud Khosravi
Nov 20 at 20:17
You need to learn to use logcat, among other debugging skills. Otherwise, you're going to posting a question every time something goes wrong. Please follow the link I provided above.
– Mike M.
Nov 20 at 20:20
Look at the stack trace to determine the cause of the crash.
– Mike M.
Nov 20 at 20:02
Look at the stack trace to determine the cause of the crash.
– Mike M.
Nov 20 at 20:02
ok how chabge my codes canyou help me
– Mahmoud Khosravi
Nov 20 at 20:17
ok how chabge my codes canyou help me
– Mahmoud Khosravi
Nov 20 at 20:17
You need to learn to use logcat, among other debugging skills. Otherwise, you're going to posting a question every time something goes wrong. Please follow the link I provided above.
– Mike M.
Nov 20 at 20:20
You need to learn to use logcat, among other debugging skills. Otherwise, you're going to posting a question every time something goes wrong. Please follow the link I provided above.
– Mike M.
Nov 20 at 20:20
add a comment |
1 Answer
1
active
oldest
votes
Your first error is in the line
ListView listView = (ListView)findViewById(R.id.listView);
in your main activity class.
You can not call findViewById
before calling setContentView
.
Member initialization happens when an object (of your activity class) is instantiated (the system calls new ActivityMain()
) which is before onCreate
is called.
So, findViewById
will return null
.
Then, when you try this:
listView.setAdapter(newsAdapter);
You will get a NullPointerException
because you are trying to call setAdapter
on a null reference.
There may be other errors, I did not read all your code, but this is the firs thing that will cause your app to crash.
"So,findViewById
will returnnull
." – Actually, it'll throw aNullPointerException
right at thefindViewById()
call.
– Mike M.
Nov 20 at 20:34
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53400668%2fcustom-listview-has-stopped-android-studio-2-3-3-version%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your first error is in the line
ListView listView = (ListView)findViewById(R.id.listView);
in your main activity class.
You can not call findViewById
before calling setContentView
.
Member initialization happens when an object (of your activity class) is instantiated (the system calls new ActivityMain()
) which is before onCreate
is called.
So, findViewById
will return null
.
Then, when you try this:
listView.setAdapter(newsAdapter);
You will get a NullPointerException
because you are trying to call setAdapter
on a null reference.
There may be other errors, I did not read all your code, but this is the firs thing that will cause your app to crash.
"So,findViewById
will returnnull
." – Actually, it'll throw aNullPointerException
right at thefindViewById()
call.
– Mike M.
Nov 20 at 20:34
add a comment |
Your first error is in the line
ListView listView = (ListView)findViewById(R.id.listView);
in your main activity class.
You can not call findViewById
before calling setContentView
.
Member initialization happens when an object (of your activity class) is instantiated (the system calls new ActivityMain()
) which is before onCreate
is called.
So, findViewById
will return null
.
Then, when you try this:
listView.setAdapter(newsAdapter);
You will get a NullPointerException
because you are trying to call setAdapter
on a null reference.
There may be other errors, I did not read all your code, but this is the firs thing that will cause your app to crash.
"So,findViewById
will returnnull
." – Actually, it'll throw aNullPointerException
right at thefindViewById()
call.
– Mike M.
Nov 20 at 20:34
add a comment |
Your first error is in the line
ListView listView = (ListView)findViewById(R.id.listView);
in your main activity class.
You can not call findViewById
before calling setContentView
.
Member initialization happens when an object (of your activity class) is instantiated (the system calls new ActivityMain()
) which is before onCreate
is called.
So, findViewById
will return null
.
Then, when you try this:
listView.setAdapter(newsAdapter);
You will get a NullPointerException
because you are trying to call setAdapter
on a null reference.
There may be other errors, I did not read all your code, but this is the firs thing that will cause your app to crash.
Your first error is in the line
ListView listView = (ListView)findViewById(R.id.listView);
in your main activity class.
You can not call findViewById
before calling setContentView
.
Member initialization happens when an object (of your activity class) is instantiated (the system calls new ActivityMain()
) which is before onCreate
is called.
So, findViewById
will return null
.
Then, when you try this:
listView.setAdapter(newsAdapter);
You will get a NullPointerException
because you are trying to call setAdapter
on a null reference.
There may be other errors, I did not read all your code, but this is the firs thing that will cause your app to crash.
answered Nov 20 at 20:17


Lev M.
618112
618112
"So,findViewById
will returnnull
." – Actually, it'll throw aNullPointerException
right at thefindViewById()
call.
– Mike M.
Nov 20 at 20:34
add a comment |
"So,findViewById
will returnnull
." – Actually, it'll throw aNullPointerException
right at thefindViewById()
call.
– Mike M.
Nov 20 at 20:34
"So,
findViewById
will return null
." – Actually, it'll throw a NullPointerException
right at the findViewById()
call.– Mike M.
Nov 20 at 20:34
"So,
findViewById
will return null
." – Actually, it'll throw a NullPointerException
right at the findViewById()
call.– Mike M.
Nov 20 at 20:34
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53400668%2fcustom-listview-has-stopped-android-studio-2-3-3-version%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VJ WrSUBN,Wc 5PHERF7Baz2a,8f94ksKhU
Look at the stack trace to determine the cause of the crash.
– Mike M.
Nov 20 at 20:02
ok how chabge my codes canyou help me
– Mahmoud Khosravi
Nov 20 at 20:17
You need to learn to use logcat, among other debugging skills. Otherwise, you're going to posting a question every time something goes wrong. Please follow the link I provided above.
– Mike M.
Nov 20 at 20:20