How to perform deconvolution in Keras/ Theano?
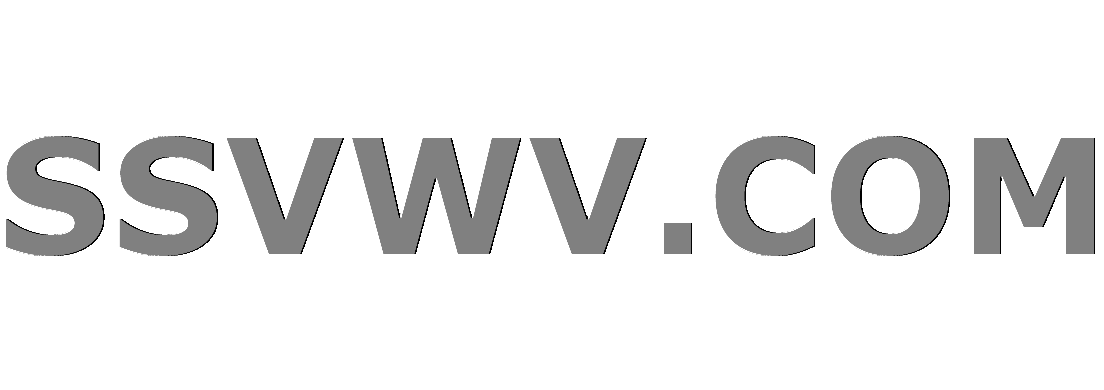
Multi tool use
I am trying to implement deconvolution in Keras. My model definition is as follows:
model=Sequential()
model.add(Convolution2D(32, 3, 3, border_mode='same',
input_shape=X_train.shape[1:]))
model.add(Activation('relu'))
model.add(Convolution2D(32, 3, 3, border_mode='same'))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Convolution2D(64, 3, 3, border_mode='same'))
model.add(Activation('relu'))
model.add(Convolution2D(64, 3, 3,border_mode='same'))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(512))
model.add(Activation('relu'))
model.add(Dropout(0.5))
model.add(Dense(nb_classes))
model.add(Activation('softmax'))
I want to perform deconvolution or transposed convolution on the output given by the first convolution layer i.e. convolution2d_1
.
Lets say the feature map we have after first convolution layer is X
which is of (9, 32, 32, 32)
where 9 is the no of images of dimension 32x32
I have passed through the layer. The weight matrix of the first layer obtained by get_weights()
function of Keras. The dimension of weight matrix is (32, 3, 3, 2)
.
The code I am using for performing transposed convolution is
conv_out = K.deconv2d(self.x, W, (9,3,32,32), dim_ordering = "th")
deconv_func = K.function([self.x, K.learning_phase()], conv_out)
X_deconv = deconv_func([X, 0 ])
But getting error:
CorrMM shape inconsistency:
bottom shape: 9 32 34 34
weight shape: 3 32 3 3
top shape: 9 32 32 32 (expected 9 3 32 32)
Can anyone please tell me where I am going wrong?
python deep-learning theano keras keras-layer
add a comment |
I am trying to implement deconvolution in Keras. My model definition is as follows:
model=Sequential()
model.add(Convolution2D(32, 3, 3, border_mode='same',
input_shape=X_train.shape[1:]))
model.add(Activation('relu'))
model.add(Convolution2D(32, 3, 3, border_mode='same'))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Convolution2D(64, 3, 3, border_mode='same'))
model.add(Activation('relu'))
model.add(Convolution2D(64, 3, 3,border_mode='same'))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(512))
model.add(Activation('relu'))
model.add(Dropout(0.5))
model.add(Dense(nb_classes))
model.add(Activation('softmax'))
I want to perform deconvolution or transposed convolution on the output given by the first convolution layer i.e. convolution2d_1
.
Lets say the feature map we have after first convolution layer is X
which is of (9, 32, 32, 32)
where 9 is the no of images of dimension 32x32
I have passed through the layer. The weight matrix of the first layer obtained by get_weights()
function of Keras. The dimension of weight matrix is (32, 3, 3, 2)
.
The code I am using for performing transposed convolution is
conv_out = K.deconv2d(self.x, W, (9,3,32,32), dim_ordering = "th")
deconv_func = K.function([self.x, K.learning_phase()], conv_out)
X_deconv = deconv_func([X, 0 ])
But getting error:
CorrMM shape inconsistency:
bottom shape: 9 32 34 34
weight shape: 3 32 3 3
top shape: 9 32 32 32 (expected 9 3 32 32)
Can anyone please tell me where I am going wrong?
python deep-learning theano keras keras-layer
add a comment |
I am trying to implement deconvolution in Keras. My model definition is as follows:
model=Sequential()
model.add(Convolution2D(32, 3, 3, border_mode='same',
input_shape=X_train.shape[1:]))
model.add(Activation('relu'))
model.add(Convolution2D(32, 3, 3, border_mode='same'))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Convolution2D(64, 3, 3, border_mode='same'))
model.add(Activation('relu'))
model.add(Convolution2D(64, 3, 3,border_mode='same'))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(512))
model.add(Activation('relu'))
model.add(Dropout(0.5))
model.add(Dense(nb_classes))
model.add(Activation('softmax'))
I want to perform deconvolution or transposed convolution on the output given by the first convolution layer i.e. convolution2d_1
.
Lets say the feature map we have after first convolution layer is X
which is of (9, 32, 32, 32)
where 9 is the no of images of dimension 32x32
I have passed through the layer. The weight matrix of the first layer obtained by get_weights()
function of Keras. The dimension of weight matrix is (32, 3, 3, 2)
.
The code I am using for performing transposed convolution is
conv_out = K.deconv2d(self.x, W, (9,3,32,32), dim_ordering = "th")
deconv_func = K.function([self.x, K.learning_phase()], conv_out)
X_deconv = deconv_func([X, 0 ])
But getting error:
CorrMM shape inconsistency:
bottom shape: 9 32 34 34
weight shape: 3 32 3 3
top shape: 9 32 32 32 (expected 9 3 32 32)
Can anyone please tell me where I am going wrong?
python deep-learning theano keras keras-layer
I am trying to implement deconvolution in Keras. My model definition is as follows:
model=Sequential()
model.add(Convolution2D(32, 3, 3, border_mode='same',
input_shape=X_train.shape[1:]))
model.add(Activation('relu'))
model.add(Convolution2D(32, 3, 3, border_mode='same'))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Convolution2D(64, 3, 3, border_mode='same'))
model.add(Activation('relu'))
model.add(Convolution2D(64, 3, 3,border_mode='same'))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(512))
model.add(Activation('relu'))
model.add(Dropout(0.5))
model.add(Dense(nb_classes))
model.add(Activation('softmax'))
I want to perform deconvolution or transposed convolution on the output given by the first convolution layer i.e. convolution2d_1
.
Lets say the feature map we have after first convolution layer is X
which is of (9, 32, 32, 32)
where 9 is the no of images of dimension 32x32
I have passed through the layer. The weight matrix of the first layer obtained by get_weights()
function of Keras. The dimension of weight matrix is (32, 3, 3, 2)
.
The code I am using for performing transposed convolution is
conv_out = K.deconv2d(self.x, W, (9,3,32,32), dim_ordering = "th")
deconv_func = K.function([self.x, K.learning_phase()], conv_out)
X_deconv = deconv_func([X, 0 ])
But getting error:
CorrMM shape inconsistency:
bottom shape: 9 32 34 34
weight shape: 3 32 3 3
top shape: 9 32 32 32 (expected 9 3 32 32)
Can anyone please tell me where I am going wrong?
python deep-learning theano keras keras-layer
python deep-learning theano keras keras-layer
asked Nov 23 '16 at 16:16
Avijit DasguptaAvijit Dasgupta
1,0221128
1,0221128
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You can easily use Deconvolution2D layer.
Here is what you are trying to achieve:
batch_sz = 1
output_shape = (batch_sz, ) + X_train.shape[1:]
conv_out = Deconvolution2D(3, 3, 3, output_shape, border_mode='same')(model.layers[0].output)
deconv_func = K.function([model.input, K.learning_phase()], [conv_out])
test_x = np.random.random(output_shape)
X_deconv = deconv_func([test_x, 0 ])
But its better to create a functional model which will help both for training and prediction..
batch_sz = 10
output_shape = (batch_sz, ) + X_train.shape[1:]
conv_out = Deconvolution2D(3, 3, 3, output_shape, border_mode='same')(model.layers[0].output)
model2 = Model(model.input, [model.output, conv_out])
model2.summary()
model2.compile(loss=['categorical_crossentropy', 'mse'], optimizer='adam')
model2.fit(X_train, [Y_train, X_train], batch_size=batch_sz)
add a comment |
In Keras, Conv2DTranspose layer perform transposed convolution in other terms deconvolution. It supports both backend lib i.e. Theano & Keras.
Keras Documentation says:
Conv2DTranspose
Transposed convolution layer (sometimes called Deconvolution).
The need for transposed convolutions generally arises from the desire
to use a transformation going in the opposite direction of a normal
convolution, i.e., from something that has the shape of the output of
some convolution to something that has the shape of its input while
maintaining a connectivity pattern that is compatible with said
convolution.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f40769363%2fhow-to-perform-deconvolution-in-keras-theano%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can easily use Deconvolution2D layer.
Here is what you are trying to achieve:
batch_sz = 1
output_shape = (batch_sz, ) + X_train.shape[1:]
conv_out = Deconvolution2D(3, 3, 3, output_shape, border_mode='same')(model.layers[0].output)
deconv_func = K.function([model.input, K.learning_phase()], [conv_out])
test_x = np.random.random(output_shape)
X_deconv = deconv_func([test_x, 0 ])
But its better to create a functional model which will help both for training and prediction..
batch_sz = 10
output_shape = (batch_sz, ) + X_train.shape[1:]
conv_out = Deconvolution2D(3, 3, 3, output_shape, border_mode='same')(model.layers[0].output)
model2 = Model(model.input, [model.output, conv_out])
model2.summary()
model2.compile(loss=['categorical_crossentropy', 'mse'], optimizer='adam')
model2.fit(X_train, [Y_train, X_train], batch_size=batch_sz)
add a comment |
You can easily use Deconvolution2D layer.
Here is what you are trying to achieve:
batch_sz = 1
output_shape = (batch_sz, ) + X_train.shape[1:]
conv_out = Deconvolution2D(3, 3, 3, output_shape, border_mode='same')(model.layers[0].output)
deconv_func = K.function([model.input, K.learning_phase()], [conv_out])
test_x = np.random.random(output_shape)
X_deconv = deconv_func([test_x, 0 ])
But its better to create a functional model which will help both for training and prediction..
batch_sz = 10
output_shape = (batch_sz, ) + X_train.shape[1:]
conv_out = Deconvolution2D(3, 3, 3, output_shape, border_mode='same')(model.layers[0].output)
model2 = Model(model.input, [model.output, conv_out])
model2.summary()
model2.compile(loss=['categorical_crossentropy', 'mse'], optimizer='adam')
model2.fit(X_train, [Y_train, X_train], batch_size=batch_sz)
add a comment |
You can easily use Deconvolution2D layer.
Here is what you are trying to achieve:
batch_sz = 1
output_shape = (batch_sz, ) + X_train.shape[1:]
conv_out = Deconvolution2D(3, 3, 3, output_shape, border_mode='same')(model.layers[0].output)
deconv_func = K.function([model.input, K.learning_phase()], [conv_out])
test_x = np.random.random(output_shape)
X_deconv = deconv_func([test_x, 0 ])
But its better to create a functional model which will help both for training and prediction..
batch_sz = 10
output_shape = (batch_sz, ) + X_train.shape[1:]
conv_out = Deconvolution2D(3, 3, 3, output_shape, border_mode='same')(model.layers[0].output)
model2 = Model(model.input, [model.output, conv_out])
model2.summary()
model2.compile(loss=['categorical_crossentropy', 'mse'], optimizer='adam')
model2.fit(X_train, [Y_train, X_train], batch_size=batch_sz)
You can easily use Deconvolution2D layer.
Here is what you are trying to achieve:
batch_sz = 1
output_shape = (batch_sz, ) + X_train.shape[1:]
conv_out = Deconvolution2D(3, 3, 3, output_shape, border_mode='same')(model.layers[0].output)
deconv_func = K.function([model.input, K.learning_phase()], [conv_out])
test_x = np.random.random(output_shape)
X_deconv = deconv_func([test_x, 0 ])
But its better to create a functional model which will help both for training and prediction..
batch_sz = 10
output_shape = (batch_sz, ) + X_train.shape[1:]
conv_out = Deconvolution2D(3, 3, 3, output_shape, border_mode='same')(model.layers[0].output)
model2 = Model(model.input, [model.output, conv_out])
model2.summary()
model2.compile(loss=['categorical_crossentropy', 'mse'], optimizer='adam')
model2.fit(X_train, [Y_train, X_train], batch_size=batch_sz)
edited Nov 23 '18 at 11:43
19greg96
2,10643348
2,10643348
answered Jan 28 '17 at 10:10


indraforyouindraforyou
5,58313633
5,58313633
add a comment |
add a comment |
In Keras, Conv2DTranspose layer perform transposed convolution in other terms deconvolution. It supports both backend lib i.e. Theano & Keras.
Keras Documentation says:
Conv2DTranspose
Transposed convolution layer (sometimes called Deconvolution).
The need for transposed convolutions generally arises from the desire
to use a transformation going in the opposite direction of a normal
convolution, i.e., from something that has the shape of the output of
some convolution to something that has the shape of its input while
maintaining a connectivity pattern that is compatible with said
convolution.
add a comment |
In Keras, Conv2DTranspose layer perform transposed convolution in other terms deconvolution. It supports both backend lib i.e. Theano & Keras.
Keras Documentation says:
Conv2DTranspose
Transposed convolution layer (sometimes called Deconvolution).
The need for transposed convolutions generally arises from the desire
to use a transformation going in the opposite direction of a normal
convolution, i.e., from something that has the shape of the output of
some convolution to something that has the shape of its input while
maintaining a connectivity pattern that is compatible with said
convolution.
add a comment |
In Keras, Conv2DTranspose layer perform transposed convolution in other terms deconvolution. It supports both backend lib i.e. Theano & Keras.
Keras Documentation says:
Conv2DTranspose
Transposed convolution layer (sometimes called Deconvolution).
The need for transposed convolutions generally arises from the desire
to use a transformation going in the opposite direction of a normal
convolution, i.e., from something that has the shape of the output of
some convolution to something that has the shape of its input while
maintaining a connectivity pattern that is compatible with said
convolution.
In Keras, Conv2DTranspose layer perform transposed convolution in other terms deconvolution. It supports both backend lib i.e. Theano & Keras.
Keras Documentation says:
Conv2DTranspose
Transposed convolution layer (sometimes called Deconvolution).
The need for transposed convolutions generally arises from the desire
to use a transformation going in the opposite direction of a normal
convolution, i.e., from something that has the shape of the output of
some convolution to something that has the shape of its input while
maintaining a connectivity pattern that is compatible with said
convolution.
answered Aug 1 '17 at 13:43


mkocabasmkocabas
319113
319113
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f40769363%2fhow-to-perform-deconvolution-in-keras-theano%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Qlic nQxSTGP4HP7kOrrh,KvaFiHv,2V7t,UQxQBthXmU8 TR823Q