How to get onclick to display contents of php file
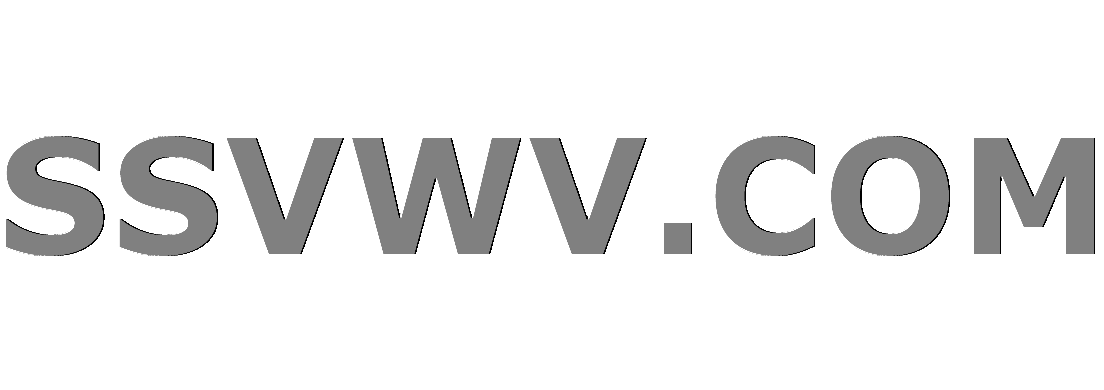
Multi tool use
I am having a problem getting an onclick function to work.
Clicking on "units" in the HTML file, "unit_test" disappears and the contents of unit_display.php shows up instead. This is what I want to happen.
If I click on "Phoenix", however, "prop_test" remains and the results of prop_display.php do not appear.
I can browse to prop_display.php?city=Phoenix and it will pull up the list of properties from that table, so this rules out prop_display.php code as the culprit.
Here is my html file:
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Frameset//EN" "http://www.w3.org/TR/html4/frameset.dtd">
<html>
<head>
<script>
function showProp(str) {
xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("propDisplay").innerHTML = this.responseText;
}
};
xmlhttp.open("GET","prop_display.php?city="+str,true);
xmlhttp.send();
}
function showUnit(str) {
xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("unitDisplay").innerHTML = this.responseText;
}
};
xmlhttp.open("GET","unit_display.php?prop_id="+str,true);
xmlhttp.send();
}
</script>
</head>
<body>
<div onclick="showProp(Phoenix)"><a href="#">Properties</a></div>
<div onclick="showUnit(8)"><a href="#">units</a></div>
<br>
<div id="propDisplay">prop_test</div>
<div id="unitDisplay">unit_test</div>
</body>
</html>
Here is prop_display.php:
<?php
include('vars.php');
$city = strval($_GET['city']);
print $city;
if (!$link) {
die('Could not connect: ' . mysqli_error($con));
}
if ($prop_query = mysqli_query($link, "SELECT * FROM property WHERE city='$city' ORDER BY street asc")){}
while ($prop_array = mysqli_fetch_array($prop_query, MYSQLI_BOTH)){
$prop_id=$prop_array['prop_id'];
print " <li><div onclick="showUnit($prop_id)"><a href="#">" . $prop_array['name'] . "<br>
" . $prop_array['street'] . "</a></div>
";
}
mysqli_close($link);
?>
And here is unit_display.php:
<?php
include('vars.php');
$prop_id = intval($_GET['prop_id']);
print $prop_id;
if (!$link) {
die('Could not connect: ' . mysqli_error($con));
}
if ($unit_query = mysqli_query($link, "SELECT * FROM units WHERE prop_id='$prop_id' ORDER BY unit asc")){}
while ($unit_array = mysqli_fetch_array($unit_query, MYSQLI_BOTH)){
print " <li><a href="#" target="_blank">" . $unit_array['unit'] . "</a></li>
";
}
mysqli_close($link);
?>
javascript php onclick
add a comment |
I am having a problem getting an onclick function to work.
Clicking on "units" in the HTML file, "unit_test" disappears and the contents of unit_display.php shows up instead. This is what I want to happen.
If I click on "Phoenix", however, "prop_test" remains and the results of prop_display.php do not appear.
I can browse to prop_display.php?city=Phoenix and it will pull up the list of properties from that table, so this rules out prop_display.php code as the culprit.
Here is my html file:
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Frameset//EN" "http://www.w3.org/TR/html4/frameset.dtd">
<html>
<head>
<script>
function showProp(str) {
xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("propDisplay").innerHTML = this.responseText;
}
};
xmlhttp.open("GET","prop_display.php?city="+str,true);
xmlhttp.send();
}
function showUnit(str) {
xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("unitDisplay").innerHTML = this.responseText;
}
};
xmlhttp.open("GET","unit_display.php?prop_id="+str,true);
xmlhttp.send();
}
</script>
</head>
<body>
<div onclick="showProp(Phoenix)"><a href="#">Properties</a></div>
<div onclick="showUnit(8)"><a href="#">units</a></div>
<br>
<div id="propDisplay">prop_test</div>
<div id="unitDisplay">unit_test</div>
</body>
</html>
Here is prop_display.php:
<?php
include('vars.php');
$city = strval($_GET['city']);
print $city;
if (!$link) {
die('Could not connect: ' . mysqli_error($con));
}
if ($prop_query = mysqli_query($link, "SELECT * FROM property WHERE city='$city' ORDER BY street asc")){}
while ($prop_array = mysqli_fetch_array($prop_query, MYSQLI_BOTH)){
$prop_id=$prop_array['prop_id'];
print " <li><div onclick="showUnit($prop_id)"><a href="#">" . $prop_array['name'] . "<br>
" . $prop_array['street'] . "</a></div>
";
}
mysqli_close($link);
?>
And here is unit_display.php:
<?php
include('vars.php');
$prop_id = intval($_GET['prop_id']);
print $prop_id;
if (!$link) {
die('Could not connect: ' . mysqli_error($con));
}
if ($unit_query = mysqli_query($link, "SELECT * FROM units WHERE prop_id='$prop_id' ORDER BY unit asc")){}
while ($unit_array = mysqli_fetch_array($unit_query, MYSQLI_BOTH)){
print " <li><a href="#" target="_blank">" . $unit_array['unit'] . "</a></li>
";
}
mysqli_close($link);
?>
javascript php onclick
1
This is open to an serious SQL injection. Best for you to use a prepared statement if you don't want your database to go *Poof!*
– Funk Forty Niner
Nov 24 '18 at 3:03
add a comment |
I am having a problem getting an onclick function to work.
Clicking on "units" in the HTML file, "unit_test" disappears and the contents of unit_display.php shows up instead. This is what I want to happen.
If I click on "Phoenix", however, "prop_test" remains and the results of prop_display.php do not appear.
I can browse to prop_display.php?city=Phoenix and it will pull up the list of properties from that table, so this rules out prop_display.php code as the culprit.
Here is my html file:
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Frameset//EN" "http://www.w3.org/TR/html4/frameset.dtd">
<html>
<head>
<script>
function showProp(str) {
xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("propDisplay").innerHTML = this.responseText;
}
};
xmlhttp.open("GET","prop_display.php?city="+str,true);
xmlhttp.send();
}
function showUnit(str) {
xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("unitDisplay").innerHTML = this.responseText;
}
};
xmlhttp.open("GET","unit_display.php?prop_id="+str,true);
xmlhttp.send();
}
</script>
</head>
<body>
<div onclick="showProp(Phoenix)"><a href="#">Properties</a></div>
<div onclick="showUnit(8)"><a href="#">units</a></div>
<br>
<div id="propDisplay">prop_test</div>
<div id="unitDisplay">unit_test</div>
</body>
</html>
Here is prop_display.php:
<?php
include('vars.php');
$city = strval($_GET['city']);
print $city;
if (!$link) {
die('Could not connect: ' . mysqli_error($con));
}
if ($prop_query = mysqli_query($link, "SELECT * FROM property WHERE city='$city' ORDER BY street asc")){}
while ($prop_array = mysqli_fetch_array($prop_query, MYSQLI_BOTH)){
$prop_id=$prop_array['prop_id'];
print " <li><div onclick="showUnit($prop_id)"><a href="#">" . $prop_array['name'] . "<br>
" . $prop_array['street'] . "</a></div>
";
}
mysqli_close($link);
?>
And here is unit_display.php:
<?php
include('vars.php');
$prop_id = intval($_GET['prop_id']);
print $prop_id;
if (!$link) {
die('Could not connect: ' . mysqli_error($con));
}
if ($unit_query = mysqli_query($link, "SELECT * FROM units WHERE prop_id='$prop_id' ORDER BY unit asc")){}
while ($unit_array = mysqli_fetch_array($unit_query, MYSQLI_BOTH)){
print " <li><a href="#" target="_blank">" . $unit_array['unit'] . "</a></li>
";
}
mysqli_close($link);
?>
javascript php onclick
I am having a problem getting an onclick function to work.
Clicking on "units" in the HTML file, "unit_test" disappears and the contents of unit_display.php shows up instead. This is what I want to happen.
If I click on "Phoenix", however, "prop_test" remains and the results of prop_display.php do not appear.
I can browse to prop_display.php?city=Phoenix and it will pull up the list of properties from that table, so this rules out prop_display.php code as the culprit.
Here is my html file:
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Frameset//EN" "http://www.w3.org/TR/html4/frameset.dtd">
<html>
<head>
<script>
function showProp(str) {
xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("propDisplay").innerHTML = this.responseText;
}
};
xmlhttp.open("GET","prop_display.php?city="+str,true);
xmlhttp.send();
}
function showUnit(str) {
xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("unitDisplay").innerHTML = this.responseText;
}
};
xmlhttp.open("GET","unit_display.php?prop_id="+str,true);
xmlhttp.send();
}
</script>
</head>
<body>
<div onclick="showProp(Phoenix)"><a href="#">Properties</a></div>
<div onclick="showUnit(8)"><a href="#">units</a></div>
<br>
<div id="propDisplay">prop_test</div>
<div id="unitDisplay">unit_test</div>
</body>
</html>
Here is prop_display.php:
<?php
include('vars.php');
$city = strval($_GET['city']);
print $city;
if (!$link) {
die('Could not connect: ' . mysqli_error($con));
}
if ($prop_query = mysqli_query($link, "SELECT * FROM property WHERE city='$city' ORDER BY street asc")){}
while ($prop_array = mysqli_fetch_array($prop_query, MYSQLI_BOTH)){
$prop_id=$prop_array['prop_id'];
print " <li><div onclick="showUnit($prop_id)"><a href="#">" . $prop_array['name'] . "<br>
" . $prop_array['street'] . "</a></div>
";
}
mysqli_close($link);
?>
And here is unit_display.php:
<?php
include('vars.php');
$prop_id = intval($_GET['prop_id']);
print $prop_id;
if (!$link) {
die('Could not connect: ' . mysqli_error($con));
}
if ($unit_query = mysqli_query($link, "SELECT * FROM units WHERE prop_id='$prop_id' ORDER BY unit asc")){}
while ($unit_array = mysqli_fetch_array($unit_query, MYSQLI_BOTH)){
print " <li><a href="#" target="_blank">" . $unit_array['unit'] . "</a></li>
";
}
mysqli_close($link);
?>
javascript php onclick
javascript php onclick
edited Nov 24 '18 at 3:02


Funk Forty Niner
1
1
asked Nov 24 '18 at 1:52
DaveDave
113
113
1
This is open to an serious SQL injection. Best for you to use a prepared statement if you don't want your database to go *Poof!*
– Funk Forty Niner
Nov 24 '18 at 3:03
add a comment |
1
This is open to an serious SQL injection. Best for you to use a prepared statement if you don't want your database to go *Poof!*
– Funk Forty Niner
Nov 24 '18 at 3:03
1
1
This is open to an serious SQL injection. Best for you to use a prepared statement if you don't want your database to go *Poof!*
– Funk Forty Niner
Nov 24 '18 at 3:03
This is open to an serious SQL injection. Best for you to use a prepared statement if you don't want your database to go *Poof!*
– Funk Forty Niner
Nov 24 '18 at 3:03
add a comment |
1 Answer
1
active
oldest
votes
It seems like you are trying to have them click with Phoenix
being an object when it should be a string.
Replace this:
<div onclick="showProp(Phoenix)"><a href="#">Properties</a></div>
with this:
<div onclick="showProp('Phoenix')"><a href="#">Properties</a></div>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454535%2fhow-to-get-onclick-to-display-contents-of-php-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
It seems like you are trying to have them click with Phoenix
being an object when it should be a string.
Replace this:
<div onclick="showProp(Phoenix)"><a href="#">Properties</a></div>
with this:
<div onclick="showProp('Phoenix')"><a href="#">Properties</a></div>
add a comment |
It seems like you are trying to have them click with Phoenix
being an object when it should be a string.
Replace this:
<div onclick="showProp(Phoenix)"><a href="#">Properties</a></div>
with this:
<div onclick="showProp('Phoenix')"><a href="#">Properties</a></div>
add a comment |
It seems like you are trying to have them click with Phoenix
being an object when it should be a string.
Replace this:
<div onclick="showProp(Phoenix)"><a href="#">Properties</a></div>
with this:
<div onclick="showProp('Phoenix')"><a href="#">Properties</a></div>
It seems like you are trying to have them click with Phoenix
being an object when it should be a string.
Replace this:
<div onclick="showProp(Phoenix)"><a href="#">Properties</a></div>
with this:
<div onclick="showProp('Phoenix')"><a href="#">Properties</a></div>
answered Nov 24 '18 at 1:57


DanDan
1815
1815
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454535%2fhow-to-get-onclick-to-display-contents-of-php-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
dtngT3rvIj18CkvYLscI
1
This is open to an serious SQL injection. Best for you to use a prepared statement if you don't want your database to go *Poof!*
– Funk Forty Niner
Nov 24 '18 at 3:03