Using Javascript to Send 1 GET Request And 2 POST Requests in a Infinite Loop
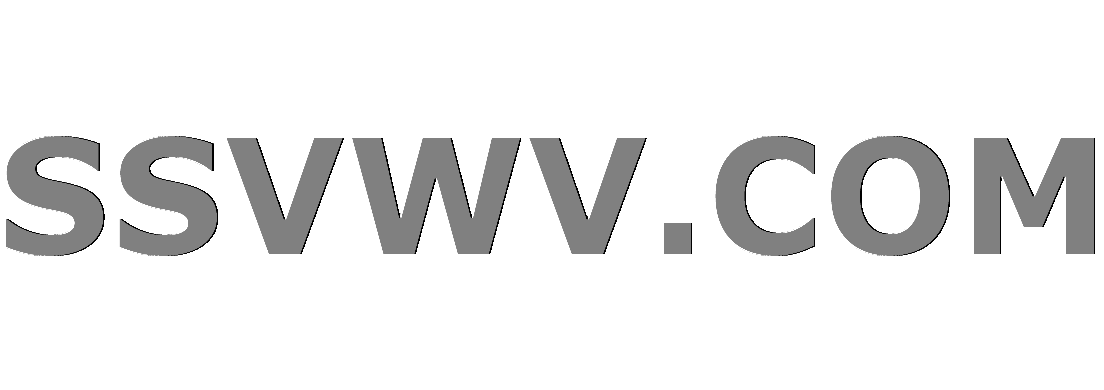
Multi tool use
I am not very familiar with JavaScript, but hoping this is possible.
I need the script to do 3 things:
Send a GET request to
https://example.com/test.php
which replies back with this JSON:[{"user_id":"12345","email":"test@test.com","status":"active"}]
Send a POST request to
https://example.com/checker.php
with these parametersaction=testing&email=test@test.com&status=active
- the second two parameters are retrieved from the step above. Responses to this post request will vary.Send a POST request to
https://example.com/verified.php
with these parameterresponse=RESPONSE-FROM-STEP-2-HERE
.
And I need it to repeat all 3 steps in an infinite loop for as long as the visitor is on the page (should automatically start as soon as the page is loaded).
It looks like in JavaScript I can use xhttp.open
for both GET and POST requests. What I'm unsure about is how to then use those responses in steps 2 and 3.
javascript
add a comment |
I am not very familiar with JavaScript, but hoping this is possible.
I need the script to do 3 things:
Send a GET request to
https://example.com/test.php
which replies back with this JSON:[{"user_id":"12345","email":"test@test.com","status":"active"}]
Send a POST request to
https://example.com/checker.php
with these parametersaction=testing&email=test@test.com&status=active
- the second two parameters are retrieved from the step above. Responses to this post request will vary.Send a POST request to
https://example.com/verified.php
with these parameterresponse=RESPONSE-FROM-STEP-2-HERE
.
And I need it to repeat all 3 steps in an infinite loop for as long as the visitor is on the page (should automatically start as soon as the page is loaded).
It looks like in JavaScript I can use xhttp.open
for both GET and POST requests. What I'm unsure about is how to then use those responses in steps 2 and 3.
javascript
Do you want to send them one after the other, where you wait for the GET to finish and then fire the first POST? Also, your subsequent GET responses might pull from cache instead of hitting the server.
– J.D. Pace
Nov 24 '18 at 2:18
Yes, I do want to send one after another. It's okay if I run into issues with cache.
– Just A Person
Nov 24 '18 at 2:19
Check out the documentation forfetch()
as a starting point.
– Jim B.
Nov 24 '18 at 2:41
add a comment |
I am not very familiar with JavaScript, but hoping this is possible.
I need the script to do 3 things:
Send a GET request to
https://example.com/test.php
which replies back with this JSON:[{"user_id":"12345","email":"test@test.com","status":"active"}]
Send a POST request to
https://example.com/checker.php
with these parametersaction=testing&email=test@test.com&status=active
- the second two parameters are retrieved from the step above. Responses to this post request will vary.Send a POST request to
https://example.com/verified.php
with these parameterresponse=RESPONSE-FROM-STEP-2-HERE
.
And I need it to repeat all 3 steps in an infinite loop for as long as the visitor is on the page (should automatically start as soon as the page is loaded).
It looks like in JavaScript I can use xhttp.open
for both GET and POST requests. What I'm unsure about is how to then use those responses in steps 2 and 3.
javascript
I am not very familiar with JavaScript, but hoping this is possible.
I need the script to do 3 things:
Send a GET request to
https://example.com/test.php
which replies back with this JSON:[{"user_id":"12345","email":"test@test.com","status":"active"}]
Send a POST request to
https://example.com/checker.php
with these parametersaction=testing&email=test@test.com&status=active
- the second two parameters are retrieved from the step above. Responses to this post request will vary.Send a POST request to
https://example.com/verified.php
with these parameterresponse=RESPONSE-FROM-STEP-2-HERE
.
And I need it to repeat all 3 steps in an infinite loop for as long as the visitor is on the page (should automatically start as soon as the page is loaded).
It looks like in JavaScript I can use xhttp.open
for both GET and POST requests. What I'm unsure about is how to then use those responses in steps 2 and 3.
javascript
javascript
edited Nov 27 '18 at 20:36


halfer
14.6k758112
14.6k758112
asked Nov 24 '18 at 2:12


Just A PersonJust A Person
11
11
Do you want to send them one after the other, where you wait for the GET to finish and then fire the first POST? Also, your subsequent GET responses might pull from cache instead of hitting the server.
– J.D. Pace
Nov 24 '18 at 2:18
Yes, I do want to send one after another. It's okay if I run into issues with cache.
– Just A Person
Nov 24 '18 at 2:19
Check out the documentation forfetch()
as a starting point.
– Jim B.
Nov 24 '18 at 2:41
add a comment |
Do you want to send them one after the other, where you wait for the GET to finish and then fire the first POST? Also, your subsequent GET responses might pull from cache instead of hitting the server.
– J.D. Pace
Nov 24 '18 at 2:18
Yes, I do want to send one after another. It's okay if I run into issues with cache.
– Just A Person
Nov 24 '18 at 2:19
Check out the documentation forfetch()
as a starting point.
– Jim B.
Nov 24 '18 at 2:41
Do you want to send them one after the other, where you wait for the GET to finish and then fire the first POST? Also, your subsequent GET responses might pull from cache instead of hitting the server.
– J.D. Pace
Nov 24 '18 at 2:18
Do you want to send them one after the other, where you wait for the GET to finish and then fire the first POST? Also, your subsequent GET responses might pull from cache instead of hitting the server.
– J.D. Pace
Nov 24 '18 at 2:18
Yes, I do want to send one after another. It's okay if I run into issues with cache.
– Just A Person
Nov 24 '18 at 2:19
Yes, I do want to send one after another. It's okay if I run into issues with cache.
– Just A Person
Nov 24 '18 at 2:19
Check out the documentation for
fetch()
as a starting point.– Jim B.
Nov 24 '18 at 2:41
Check out the documentation for
fetch()
as a starting point.– Jim B.
Nov 24 '18 at 2:41
add a comment |
2 Answers
2
active
oldest
votes
Do you think something like this will be useful? you can also use await. But this should give you an idea.
var getTest = () => new Promise((res, rej) => {
console.log('request getUsersId');
return res([{"user_id":"12345","email":"test@test.com","status":"active"}]);
});
var postChecker = (requestParams) => new Promise((res, rej) => {
console.log('request postChecker' + JSON.stringify(requestParams));
return res({"step2response": "RandomResponse"})
});
var postVerified = (requestParams) => new Promise((res, rej) => {
console.log('request postVerified' + JSON.stringify(requestParams));
return res({"step3Response": "step3Response"})
});
getTest().then((response)=>{
postChecker(response).then((response)=>{
postVerified(response);
})
})
add a comment |
function step1() {
return new Promise(resolve => {
setTimeout(() => {
console.log(1)
resolve(1)
}, 1000)
})
}
function step2() {
return new Promise(resolve => {
setTimeout(() => {
console.log(2)
resolve(2)
}, 500)
})
}
function step3() {
return new Promise(resolve => {
setTimeout(() => {
console.log(3)
resolve(3)
}, 200)
})
}
step1().then(data1 => {
step2().then(data2 => {
step3().then(data3 => {
console.log(data1, data2, data3)
})
})
})
Please read... "What I'm unsure about is how to then use those responses in steps 2 and 3."
– Just A Person
Nov 24 '18 at 3:30
you can learn es6 Promise
– zhangwei
Nov 24 '18 at 4:35
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454628%2fusing-javascript-to-send-1-get-request-and-2-post-requests-in-a-infinite-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Do you think something like this will be useful? you can also use await. But this should give you an idea.
var getTest = () => new Promise((res, rej) => {
console.log('request getUsersId');
return res([{"user_id":"12345","email":"test@test.com","status":"active"}]);
});
var postChecker = (requestParams) => new Promise((res, rej) => {
console.log('request postChecker' + JSON.stringify(requestParams));
return res({"step2response": "RandomResponse"})
});
var postVerified = (requestParams) => new Promise((res, rej) => {
console.log('request postVerified' + JSON.stringify(requestParams));
return res({"step3Response": "step3Response"})
});
getTest().then((response)=>{
postChecker(response).then((response)=>{
postVerified(response);
})
})
add a comment |
Do you think something like this will be useful? you can also use await. But this should give you an idea.
var getTest = () => new Promise((res, rej) => {
console.log('request getUsersId');
return res([{"user_id":"12345","email":"test@test.com","status":"active"}]);
});
var postChecker = (requestParams) => new Promise((res, rej) => {
console.log('request postChecker' + JSON.stringify(requestParams));
return res({"step2response": "RandomResponse"})
});
var postVerified = (requestParams) => new Promise((res, rej) => {
console.log('request postVerified' + JSON.stringify(requestParams));
return res({"step3Response": "step3Response"})
});
getTest().then((response)=>{
postChecker(response).then((response)=>{
postVerified(response);
})
})
add a comment |
Do you think something like this will be useful? you can also use await. But this should give you an idea.
var getTest = () => new Promise((res, rej) => {
console.log('request getUsersId');
return res([{"user_id":"12345","email":"test@test.com","status":"active"}]);
});
var postChecker = (requestParams) => new Promise((res, rej) => {
console.log('request postChecker' + JSON.stringify(requestParams));
return res({"step2response": "RandomResponse"})
});
var postVerified = (requestParams) => new Promise((res, rej) => {
console.log('request postVerified' + JSON.stringify(requestParams));
return res({"step3Response": "step3Response"})
});
getTest().then((response)=>{
postChecker(response).then((response)=>{
postVerified(response);
})
})
Do you think something like this will be useful? you can also use await. But this should give you an idea.
var getTest = () => new Promise((res, rej) => {
console.log('request getUsersId');
return res([{"user_id":"12345","email":"test@test.com","status":"active"}]);
});
var postChecker = (requestParams) => new Promise((res, rej) => {
console.log('request postChecker' + JSON.stringify(requestParams));
return res({"step2response": "RandomResponse"})
});
var postVerified = (requestParams) => new Promise((res, rej) => {
console.log('request postVerified' + JSON.stringify(requestParams));
return res({"step3Response": "step3Response"})
});
getTest().then((response)=>{
postChecker(response).then((response)=>{
postVerified(response);
})
})
answered Nov 24 '18 at 4:39
BalaBala
954719
954719
add a comment |
add a comment |
function step1() {
return new Promise(resolve => {
setTimeout(() => {
console.log(1)
resolve(1)
}, 1000)
})
}
function step2() {
return new Promise(resolve => {
setTimeout(() => {
console.log(2)
resolve(2)
}, 500)
})
}
function step3() {
return new Promise(resolve => {
setTimeout(() => {
console.log(3)
resolve(3)
}, 200)
})
}
step1().then(data1 => {
step2().then(data2 => {
step3().then(data3 => {
console.log(data1, data2, data3)
})
})
})
Please read... "What I'm unsure about is how to then use those responses in steps 2 and 3."
– Just A Person
Nov 24 '18 at 3:30
you can learn es6 Promise
– zhangwei
Nov 24 '18 at 4:35
add a comment |
function step1() {
return new Promise(resolve => {
setTimeout(() => {
console.log(1)
resolve(1)
}, 1000)
})
}
function step2() {
return new Promise(resolve => {
setTimeout(() => {
console.log(2)
resolve(2)
}, 500)
})
}
function step3() {
return new Promise(resolve => {
setTimeout(() => {
console.log(3)
resolve(3)
}, 200)
})
}
step1().then(data1 => {
step2().then(data2 => {
step3().then(data3 => {
console.log(data1, data2, data3)
})
})
})
Please read... "What I'm unsure about is how to then use those responses in steps 2 and 3."
– Just A Person
Nov 24 '18 at 3:30
you can learn es6 Promise
– zhangwei
Nov 24 '18 at 4:35
add a comment |
function step1() {
return new Promise(resolve => {
setTimeout(() => {
console.log(1)
resolve(1)
}, 1000)
})
}
function step2() {
return new Promise(resolve => {
setTimeout(() => {
console.log(2)
resolve(2)
}, 500)
})
}
function step3() {
return new Promise(resolve => {
setTimeout(() => {
console.log(3)
resolve(3)
}, 200)
})
}
step1().then(data1 => {
step2().then(data2 => {
step3().then(data3 => {
console.log(data1, data2, data3)
})
})
})
function step1() {
return new Promise(resolve => {
setTimeout(() => {
console.log(1)
resolve(1)
}, 1000)
})
}
function step2() {
return new Promise(resolve => {
setTimeout(() => {
console.log(2)
resolve(2)
}, 500)
})
}
function step3() {
return new Promise(resolve => {
setTimeout(() => {
console.log(3)
resolve(3)
}, 200)
})
}
step1().then(data1 => {
step2().then(data2 => {
step3().then(data3 => {
console.log(data1, data2, data3)
})
})
})
answered Nov 24 '18 at 2:40
zhangweizhangwei
214
214
Please read... "What I'm unsure about is how to then use those responses in steps 2 and 3."
– Just A Person
Nov 24 '18 at 3:30
you can learn es6 Promise
– zhangwei
Nov 24 '18 at 4:35
add a comment |
Please read... "What I'm unsure about is how to then use those responses in steps 2 and 3."
– Just A Person
Nov 24 '18 at 3:30
you can learn es6 Promise
– zhangwei
Nov 24 '18 at 4:35
Please read... "What I'm unsure about is how to then use those responses in steps 2 and 3."
– Just A Person
Nov 24 '18 at 3:30
Please read... "What I'm unsure about is how to then use those responses in steps 2 and 3."
– Just A Person
Nov 24 '18 at 3:30
you can learn es6 Promise
– zhangwei
Nov 24 '18 at 4:35
you can learn es6 Promise
– zhangwei
Nov 24 '18 at 4:35
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454628%2fusing-javascript-to-send-1-get-request-and-2-post-requests-in-a-infinite-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1WQt4,VqpeQcUkh6i 0amK6ewCFZ6Y4b jUByXJIDxH SxM z7iFG6BV iFVp2Tzx7C4Yi5 dgbnXDMufCkyjyaIZ,yGCoxDKsagbO
Do you want to send them one after the other, where you wait for the GET to finish and then fire the first POST? Also, your subsequent GET responses might pull from cache instead of hitting the server.
– J.D. Pace
Nov 24 '18 at 2:18
Yes, I do want to send one after another. It's okay if I run into issues with cache.
– Just A Person
Nov 24 '18 at 2:19
Check out the documentation for
fetch()
as a starting point.– Jim B.
Nov 24 '18 at 2:41