Write a function to return diagonal elements of an array(NxN) as an array in python
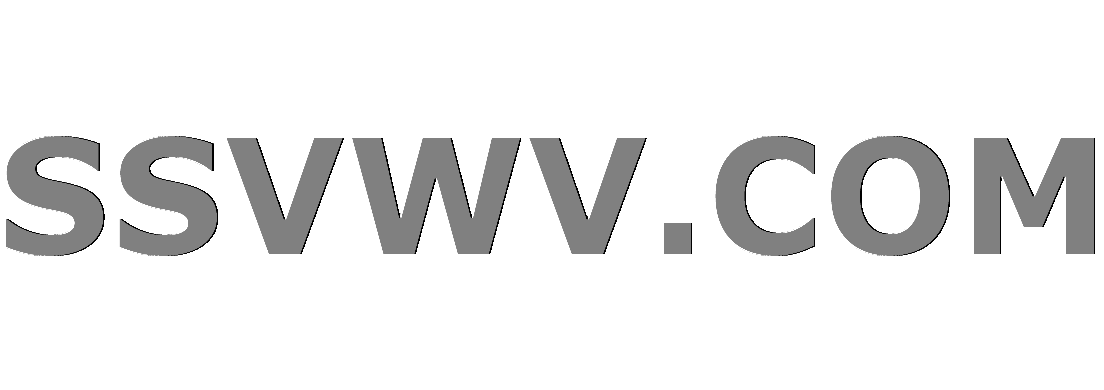
Multi tool use
import numpy as np
#getting number of rows and columns for the arrat
nr=input("enter rows")
nc=input("enter columns")
print("Please enter same rows and columns")
n=nr*nc
ar1=
#checking for square matrix
if(nr==nc):
#loop to append elements into the empty-list ar1
for i in range(n):
ele=input("enter elements")
ar1.append(ele)
#getting the number of rows of array1
array1=np.array(ar1).shape
#function to get the diagonal elements of array
def diagonal(a):
global n
n=str(n)
for i in n:
for j in n:
if i==j:
newarr=np.array(a[i][j])
#print(newarr)
diagonal(array1)
newarr=np.array(a[i][j]) is resulting in an error showing:
#TypeError: tuple indices must be integers, not str for below code
python numpy
add a comment |
import numpy as np
#getting number of rows and columns for the arrat
nr=input("enter rows")
nc=input("enter columns")
print("Please enter same rows and columns")
n=nr*nc
ar1=
#checking for square matrix
if(nr==nc):
#loop to append elements into the empty-list ar1
for i in range(n):
ele=input("enter elements")
ar1.append(ele)
#getting the number of rows of array1
array1=np.array(ar1).shape
#function to get the diagonal elements of array
def diagonal(a):
global n
n=str(n)
for i in n:
for j in n:
if i==j:
newarr=np.array(a[i][j])
#print(newarr)
diagonal(array1)
newarr=np.array(a[i][j]) is resulting in an error showing:
#TypeError: tuple indices must be integers, not str for below code
python numpy
Isn't the error clear? Why don't you fix this (pretty obvious) issue first? Also consider a Minimal, Complete, and Verifiable example, if you're not clear where they come from and how to fix them.
– Ulrich Eckhardt
Nov 26 '18 at 9:23
add a comment |
import numpy as np
#getting number of rows and columns for the arrat
nr=input("enter rows")
nc=input("enter columns")
print("Please enter same rows and columns")
n=nr*nc
ar1=
#checking for square matrix
if(nr==nc):
#loop to append elements into the empty-list ar1
for i in range(n):
ele=input("enter elements")
ar1.append(ele)
#getting the number of rows of array1
array1=np.array(ar1).shape
#function to get the diagonal elements of array
def diagonal(a):
global n
n=str(n)
for i in n:
for j in n:
if i==j:
newarr=np.array(a[i][j])
#print(newarr)
diagonal(array1)
newarr=np.array(a[i][j]) is resulting in an error showing:
#TypeError: tuple indices must be integers, not str for below code
python numpy
import numpy as np
#getting number of rows and columns for the arrat
nr=input("enter rows")
nc=input("enter columns")
print("Please enter same rows and columns")
n=nr*nc
ar1=
#checking for square matrix
if(nr==nc):
#loop to append elements into the empty-list ar1
for i in range(n):
ele=input("enter elements")
ar1.append(ele)
#getting the number of rows of array1
array1=np.array(ar1).shape
#function to get the diagonal elements of array
def diagonal(a):
global n
n=str(n)
for i in n:
for j in n:
if i==j:
newarr=np.array(a[i][j])
#print(newarr)
diagonal(array1)
newarr=np.array(a[i][j]) is resulting in an error showing:
#TypeError: tuple indices must be integers, not str for below code
python numpy
python numpy
asked Nov 26 '18 at 9:10
SethuSethu
269
269
Isn't the error clear? Why don't you fix this (pretty obvious) issue first? Also consider a Minimal, Complete, and Verifiable example, if you're not clear where they come from and how to fix them.
– Ulrich Eckhardt
Nov 26 '18 at 9:23
add a comment |
Isn't the error clear? Why don't you fix this (pretty obvious) issue first? Also consider a Minimal, Complete, and Verifiable example, if you're not clear where they come from and how to fix them.
– Ulrich Eckhardt
Nov 26 '18 at 9:23
Isn't the error clear? Why don't you fix this (pretty obvious) issue first? Also consider a Minimal, Complete, and Verifiable example, if you're not clear where they come from and how to fix them.
– Ulrich Eckhardt
Nov 26 '18 at 9:23
Isn't the error clear? Why don't you fix this (pretty obvious) issue first? Also consider a Minimal, Complete, and Verifiable example, if you're not clear where they come from and how to fix them.
– Ulrich Eckhardt
Nov 26 '18 at 9:23
add a comment |
2 Answers
2
active
oldest
votes
If you know that your matrix is going to be a square one, you need not take the row and column input separately. You can just take the value n. Your diagonal function will then look like :
newa=
def diag(arr):
for i,a in enumerate(arr):
newa[i]=arr[i,i]
add a comment |
I do agree with @Gautam, by the way here's a workaround solution which uses inbuilt diagonal
function:
lst =
size = int(input('Enter size of sqaure matrix'))
for i in range(1, (size**2)+1):
lst.append(int(input('Enter ' + str(i) +'th element')))
lst
Enter size of sqaure matrix2
Enter 1th element5
Enter 2th element6
Enter 3th element8
Enter 4th element7
[5, 6, 8, 7]
arr = np.array(lst).reshape(size,size)
arr.diagonal()
[5, 7]
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53477795%2fwrite-a-function-to-return-diagonal-elements-of-an-arraynxn-as-an-array-in-pyt%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you know that your matrix is going to be a square one, you need not take the row and column input separately. You can just take the value n. Your diagonal function will then look like :
newa=
def diag(arr):
for i,a in enumerate(arr):
newa[i]=arr[i,i]
add a comment |
If you know that your matrix is going to be a square one, you need not take the row and column input separately. You can just take the value n. Your diagonal function will then look like :
newa=
def diag(arr):
for i,a in enumerate(arr):
newa[i]=arr[i,i]
add a comment |
If you know that your matrix is going to be a square one, you need not take the row and column input separately. You can just take the value n. Your diagonal function will then look like :
newa=
def diag(arr):
for i,a in enumerate(arr):
newa[i]=arr[i,i]
If you know that your matrix is going to be a square one, you need not take the row and column input separately. You can just take the value n. Your diagonal function will then look like :
newa=
def diag(arr):
for i,a in enumerate(arr):
newa[i]=arr[i,i]
answered Nov 26 '18 at 9:17
GautamGautam
1,12329
1,12329
add a comment |
add a comment |
I do agree with @Gautam, by the way here's a workaround solution which uses inbuilt diagonal
function:
lst =
size = int(input('Enter size of sqaure matrix'))
for i in range(1, (size**2)+1):
lst.append(int(input('Enter ' + str(i) +'th element')))
lst
Enter size of sqaure matrix2
Enter 1th element5
Enter 2th element6
Enter 3th element8
Enter 4th element7
[5, 6, 8, 7]
arr = np.array(lst).reshape(size,size)
arr.diagonal()
[5, 7]
add a comment |
I do agree with @Gautam, by the way here's a workaround solution which uses inbuilt diagonal
function:
lst =
size = int(input('Enter size of sqaure matrix'))
for i in range(1, (size**2)+1):
lst.append(int(input('Enter ' + str(i) +'th element')))
lst
Enter size of sqaure matrix2
Enter 1th element5
Enter 2th element6
Enter 3th element8
Enter 4th element7
[5, 6, 8, 7]
arr = np.array(lst).reshape(size,size)
arr.diagonal()
[5, 7]
add a comment |
I do agree with @Gautam, by the way here's a workaround solution which uses inbuilt diagonal
function:
lst =
size = int(input('Enter size of sqaure matrix'))
for i in range(1, (size**2)+1):
lst.append(int(input('Enter ' + str(i) +'th element')))
lst
Enter size of sqaure matrix2
Enter 1th element5
Enter 2th element6
Enter 3th element8
Enter 4th element7
[5, 6, 8, 7]
arr = np.array(lst).reshape(size,size)
arr.diagonal()
[5, 7]
I do agree with @Gautam, by the way here's a workaround solution which uses inbuilt diagonal
function:
lst =
size = int(input('Enter size of sqaure matrix'))
for i in range(1, (size**2)+1):
lst.append(int(input('Enter ' + str(i) +'th element')))
lst
Enter size of sqaure matrix2
Enter 1th element5
Enter 2th element6
Enter 3th element8
Enter 4th element7
[5, 6, 8, 7]
arr = np.array(lst).reshape(size,size)
arr.diagonal()
[5, 7]
answered Nov 26 '18 at 9:21


dataLeodataLeo
6431519
6431519
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53477795%2fwrite-a-function-to-return-diagonal-elements-of-an-arraynxn-as-an-array-in-pyt%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
EHxO,YHNxgklph sKKukj I2y170c6HDG,YizI0WG1YPkRfj
Isn't the error clear? Why don't you fix this (pretty obvious) issue first? Also consider a Minimal, Complete, and Verifiable example, if you're not clear where they come from and how to fix them.
– Ulrich Eckhardt
Nov 26 '18 at 9:23