Passing labels for tiny-yolo model using keras, expected activation_7 to have 4 dimensions, but got array...
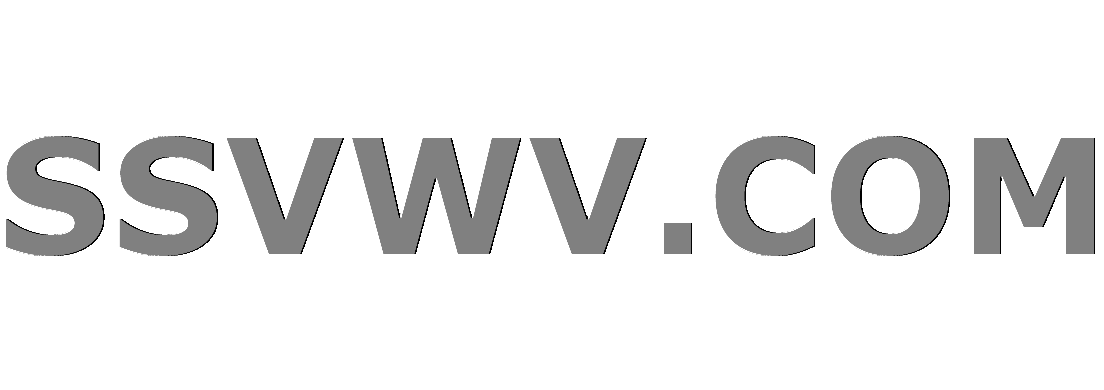
Multi tool use
up vote
0
down vote
favorite
I am trying to pass my own data from scratch through the tiny-YOLO architecture built using Keras.
In my understanding,
the labels for each image should be of the form:
[p_c, b_x, b_y, b_h, b_w, c_1, c_2] or variants for other cases.
In this case, there are 7 elements in each label for an image.
Final layer is a convolutional layer with output (3, 3, 7), with each channel representing the label.
However, during training and at the final layer, the linear activation of the convolutional layer expects 4 dimensions whilst the labels I've provided is of the form (m, 7) as explained above. (note: m = number of training data).
What should I do to fix this? Any help will be appreciated!
Below is a model summary and the error at the bottom.
Layer (type) Output Shape Param #
=================================================================
conv2d_1 (Conv2D) (None, 100, 100, 16) 448
_________________________________________________________________
activation_1 (Activation) (None, 100, 100, 16) 0
_________________________________________________________________
max_pooling2d_1 (MaxPooling2 (None, 50, 50, 16) 0
_________________________________________________________________
conv2d_2 (Conv2D) (None, 50, 50, 32) 4640
_________________________________________________________________
leaky_re_lu_1 (LeakyReLU) (None, 50, 50, 32) 0
_________________________________________________________________
max_pooling2d_2 (MaxPooling2 (None, 25, 25, 32) 0
_________________________________________________________________
conv2d_3 (Conv2D) (None, 25, 25, 64) 18496
_________________________________________________________________
activation_2 (Activation) (None, 25, 25, 64) 0
_________________________________________________________________
max_pooling2d_3 (MaxPooling2 (None, 12, 12, 64) 0
_________________________________________________________________
conv2d_4 (Conv2D) (None, 12, 12, 128) 73856
_________________________________________________________________
activation_3 (Activation) (None, 12, 12, 128) 0
_________________________________________________________________
max_pooling2d_4 (MaxPooling2 (None, 6, 6, 128) 0
_________________________________________________________________
conv2d_5 (Conv2D) (None, 6, 6, 512) 590336
_________________________________________________________________
activation_4 (Activation) (None, 6, 6, 512) 0
_________________________________________________________________
max_pooling2d_5 (MaxPooling2 (None, 3, 3, 512) 0
_________________________________________________________________
conv2d_6 (Conv2D) (None, 3, 3, 1024) 4719616
_________________________________________________________________
activation_5 (Activation) (None, 3, 3, 1024) 0
_________________________________________________________________
conv2d_7 (Conv2D) (None, 3, 3, 1024) 9438208
_________________________________________________________________
activation_6 (Activation) (None, 3, 3, 1024) 0
_________________________________________________________________
conv2d_8 (Conv2D) (None, 3, 3, 7) 7175
_________________________________________________________________
activation_7 (Activation) (None, 3, 3, 7) 0
=================================================================
Total params: 14,852,775
Trainable params: 14,852,775
Non-trainable params: 0
_________________________________________________________________
Error:
ValueError: Error when checking target: expected activation_7 to have 4 dimensions, but got array with shape (246, 7)
Model Code:
def build_model(img_rows, img_cols, num_channels):
model = Sequential()
input_shape = (img_rows, img_cols, num_channels)
if K.image_data_format() == "channels_first":
input_shape = (num_channels, img_rows, img_cols)
model.add(Conv2D(filters=16, kernel_size=3, padding="same", input_shape=input_shape))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=32, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=64, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=128, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=512, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=1024, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(Conv2D(filters=1024, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
#Softmax layer (filters: p_c, b_x, b_y, b_w, b_h, 2 classes)
model.add(Conv2D(filters=7, kernel_size=1, padding="same"))
model.add(Activation("linear"))
return model
Training Code:
def main():
# Put training data and labels into variables.
(train_images, train_labels), (test_images, test_labels) = get_data()
# Normalize training data variables.
train_images = train_images.astype("float32")/225.0
test_images = test_images.astype("float32")/225.0
# Create tiny-Yolo model
model = TinyYolo.build_model(100, 100, 3)
model.summary()
optimizer = SGD(lr = 0.01)
model.compile(loss = "categorical_crossentropy", optimizer=optimizer, metrics=["accuracy"])
model.fit(train_images, train_labels, batch_size=12, epochs=20, verbose=1)
(loss, accuracy) = model.evaluate(test_images, test_labels, batch_size=12,verbose=1)
python tensorflow keras yolo
add a comment |
up vote
0
down vote
favorite
I am trying to pass my own data from scratch through the tiny-YOLO architecture built using Keras.
In my understanding,
the labels for each image should be of the form:
[p_c, b_x, b_y, b_h, b_w, c_1, c_2] or variants for other cases.
In this case, there are 7 elements in each label for an image.
Final layer is a convolutional layer with output (3, 3, 7), with each channel representing the label.
However, during training and at the final layer, the linear activation of the convolutional layer expects 4 dimensions whilst the labels I've provided is of the form (m, 7) as explained above. (note: m = number of training data).
What should I do to fix this? Any help will be appreciated!
Below is a model summary and the error at the bottom.
Layer (type) Output Shape Param #
=================================================================
conv2d_1 (Conv2D) (None, 100, 100, 16) 448
_________________________________________________________________
activation_1 (Activation) (None, 100, 100, 16) 0
_________________________________________________________________
max_pooling2d_1 (MaxPooling2 (None, 50, 50, 16) 0
_________________________________________________________________
conv2d_2 (Conv2D) (None, 50, 50, 32) 4640
_________________________________________________________________
leaky_re_lu_1 (LeakyReLU) (None, 50, 50, 32) 0
_________________________________________________________________
max_pooling2d_2 (MaxPooling2 (None, 25, 25, 32) 0
_________________________________________________________________
conv2d_3 (Conv2D) (None, 25, 25, 64) 18496
_________________________________________________________________
activation_2 (Activation) (None, 25, 25, 64) 0
_________________________________________________________________
max_pooling2d_3 (MaxPooling2 (None, 12, 12, 64) 0
_________________________________________________________________
conv2d_4 (Conv2D) (None, 12, 12, 128) 73856
_________________________________________________________________
activation_3 (Activation) (None, 12, 12, 128) 0
_________________________________________________________________
max_pooling2d_4 (MaxPooling2 (None, 6, 6, 128) 0
_________________________________________________________________
conv2d_5 (Conv2D) (None, 6, 6, 512) 590336
_________________________________________________________________
activation_4 (Activation) (None, 6, 6, 512) 0
_________________________________________________________________
max_pooling2d_5 (MaxPooling2 (None, 3, 3, 512) 0
_________________________________________________________________
conv2d_6 (Conv2D) (None, 3, 3, 1024) 4719616
_________________________________________________________________
activation_5 (Activation) (None, 3, 3, 1024) 0
_________________________________________________________________
conv2d_7 (Conv2D) (None, 3, 3, 1024) 9438208
_________________________________________________________________
activation_6 (Activation) (None, 3, 3, 1024) 0
_________________________________________________________________
conv2d_8 (Conv2D) (None, 3, 3, 7) 7175
_________________________________________________________________
activation_7 (Activation) (None, 3, 3, 7) 0
=================================================================
Total params: 14,852,775
Trainable params: 14,852,775
Non-trainable params: 0
_________________________________________________________________
Error:
ValueError: Error when checking target: expected activation_7 to have 4 dimensions, but got array with shape (246, 7)
Model Code:
def build_model(img_rows, img_cols, num_channels):
model = Sequential()
input_shape = (img_rows, img_cols, num_channels)
if K.image_data_format() == "channels_first":
input_shape = (num_channels, img_rows, img_cols)
model.add(Conv2D(filters=16, kernel_size=3, padding="same", input_shape=input_shape))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=32, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=64, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=128, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=512, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=1024, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(Conv2D(filters=1024, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
#Softmax layer (filters: p_c, b_x, b_y, b_w, b_h, 2 classes)
model.add(Conv2D(filters=7, kernel_size=1, padding="same"))
model.add(Activation("linear"))
return model
Training Code:
def main():
# Put training data and labels into variables.
(train_images, train_labels), (test_images, test_labels) = get_data()
# Normalize training data variables.
train_images = train_images.astype("float32")/225.0
test_images = test_images.astype("float32")/225.0
# Create tiny-Yolo model
model = TinyYolo.build_model(100, 100, 3)
model.summary()
optimizer = SGD(lr = 0.01)
model.compile(loss = "categorical_crossentropy", optimizer=optimizer, metrics=["accuracy"])
model.fit(train_images, train_labels, batch_size=12, epochs=20, verbose=1)
(loss, accuracy) = model.evaluate(test_images, test_labels, batch_size=12,verbose=1)
python tensorflow keras yolo
1
Add the code for creating the model. and training.
– Or Dinari
2 days ago
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am trying to pass my own data from scratch through the tiny-YOLO architecture built using Keras.
In my understanding,
the labels for each image should be of the form:
[p_c, b_x, b_y, b_h, b_w, c_1, c_2] or variants for other cases.
In this case, there are 7 elements in each label for an image.
Final layer is a convolutional layer with output (3, 3, 7), with each channel representing the label.
However, during training and at the final layer, the linear activation of the convolutional layer expects 4 dimensions whilst the labels I've provided is of the form (m, 7) as explained above. (note: m = number of training data).
What should I do to fix this? Any help will be appreciated!
Below is a model summary and the error at the bottom.
Layer (type) Output Shape Param #
=================================================================
conv2d_1 (Conv2D) (None, 100, 100, 16) 448
_________________________________________________________________
activation_1 (Activation) (None, 100, 100, 16) 0
_________________________________________________________________
max_pooling2d_1 (MaxPooling2 (None, 50, 50, 16) 0
_________________________________________________________________
conv2d_2 (Conv2D) (None, 50, 50, 32) 4640
_________________________________________________________________
leaky_re_lu_1 (LeakyReLU) (None, 50, 50, 32) 0
_________________________________________________________________
max_pooling2d_2 (MaxPooling2 (None, 25, 25, 32) 0
_________________________________________________________________
conv2d_3 (Conv2D) (None, 25, 25, 64) 18496
_________________________________________________________________
activation_2 (Activation) (None, 25, 25, 64) 0
_________________________________________________________________
max_pooling2d_3 (MaxPooling2 (None, 12, 12, 64) 0
_________________________________________________________________
conv2d_4 (Conv2D) (None, 12, 12, 128) 73856
_________________________________________________________________
activation_3 (Activation) (None, 12, 12, 128) 0
_________________________________________________________________
max_pooling2d_4 (MaxPooling2 (None, 6, 6, 128) 0
_________________________________________________________________
conv2d_5 (Conv2D) (None, 6, 6, 512) 590336
_________________________________________________________________
activation_4 (Activation) (None, 6, 6, 512) 0
_________________________________________________________________
max_pooling2d_5 (MaxPooling2 (None, 3, 3, 512) 0
_________________________________________________________________
conv2d_6 (Conv2D) (None, 3, 3, 1024) 4719616
_________________________________________________________________
activation_5 (Activation) (None, 3, 3, 1024) 0
_________________________________________________________________
conv2d_7 (Conv2D) (None, 3, 3, 1024) 9438208
_________________________________________________________________
activation_6 (Activation) (None, 3, 3, 1024) 0
_________________________________________________________________
conv2d_8 (Conv2D) (None, 3, 3, 7) 7175
_________________________________________________________________
activation_7 (Activation) (None, 3, 3, 7) 0
=================================================================
Total params: 14,852,775
Trainable params: 14,852,775
Non-trainable params: 0
_________________________________________________________________
Error:
ValueError: Error when checking target: expected activation_7 to have 4 dimensions, but got array with shape (246, 7)
Model Code:
def build_model(img_rows, img_cols, num_channels):
model = Sequential()
input_shape = (img_rows, img_cols, num_channels)
if K.image_data_format() == "channels_first":
input_shape = (num_channels, img_rows, img_cols)
model.add(Conv2D(filters=16, kernel_size=3, padding="same", input_shape=input_shape))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=32, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=64, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=128, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=512, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=1024, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(Conv2D(filters=1024, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
#Softmax layer (filters: p_c, b_x, b_y, b_w, b_h, 2 classes)
model.add(Conv2D(filters=7, kernel_size=1, padding="same"))
model.add(Activation("linear"))
return model
Training Code:
def main():
# Put training data and labels into variables.
(train_images, train_labels), (test_images, test_labels) = get_data()
# Normalize training data variables.
train_images = train_images.astype("float32")/225.0
test_images = test_images.astype("float32")/225.0
# Create tiny-Yolo model
model = TinyYolo.build_model(100, 100, 3)
model.summary()
optimizer = SGD(lr = 0.01)
model.compile(loss = "categorical_crossentropy", optimizer=optimizer, metrics=["accuracy"])
model.fit(train_images, train_labels, batch_size=12, epochs=20, verbose=1)
(loss, accuracy) = model.evaluate(test_images, test_labels, batch_size=12,verbose=1)
python tensorflow keras yolo
I am trying to pass my own data from scratch through the tiny-YOLO architecture built using Keras.
In my understanding,
the labels for each image should be of the form:
[p_c, b_x, b_y, b_h, b_w, c_1, c_2] or variants for other cases.
In this case, there are 7 elements in each label for an image.
Final layer is a convolutional layer with output (3, 3, 7), with each channel representing the label.
However, during training and at the final layer, the linear activation of the convolutional layer expects 4 dimensions whilst the labels I've provided is of the form (m, 7) as explained above. (note: m = number of training data).
What should I do to fix this? Any help will be appreciated!
Below is a model summary and the error at the bottom.
Layer (type) Output Shape Param #
=================================================================
conv2d_1 (Conv2D) (None, 100, 100, 16) 448
_________________________________________________________________
activation_1 (Activation) (None, 100, 100, 16) 0
_________________________________________________________________
max_pooling2d_1 (MaxPooling2 (None, 50, 50, 16) 0
_________________________________________________________________
conv2d_2 (Conv2D) (None, 50, 50, 32) 4640
_________________________________________________________________
leaky_re_lu_1 (LeakyReLU) (None, 50, 50, 32) 0
_________________________________________________________________
max_pooling2d_2 (MaxPooling2 (None, 25, 25, 32) 0
_________________________________________________________________
conv2d_3 (Conv2D) (None, 25, 25, 64) 18496
_________________________________________________________________
activation_2 (Activation) (None, 25, 25, 64) 0
_________________________________________________________________
max_pooling2d_3 (MaxPooling2 (None, 12, 12, 64) 0
_________________________________________________________________
conv2d_4 (Conv2D) (None, 12, 12, 128) 73856
_________________________________________________________________
activation_3 (Activation) (None, 12, 12, 128) 0
_________________________________________________________________
max_pooling2d_4 (MaxPooling2 (None, 6, 6, 128) 0
_________________________________________________________________
conv2d_5 (Conv2D) (None, 6, 6, 512) 590336
_________________________________________________________________
activation_4 (Activation) (None, 6, 6, 512) 0
_________________________________________________________________
max_pooling2d_5 (MaxPooling2 (None, 3, 3, 512) 0
_________________________________________________________________
conv2d_6 (Conv2D) (None, 3, 3, 1024) 4719616
_________________________________________________________________
activation_5 (Activation) (None, 3, 3, 1024) 0
_________________________________________________________________
conv2d_7 (Conv2D) (None, 3, 3, 1024) 9438208
_________________________________________________________________
activation_6 (Activation) (None, 3, 3, 1024) 0
_________________________________________________________________
conv2d_8 (Conv2D) (None, 3, 3, 7) 7175
_________________________________________________________________
activation_7 (Activation) (None, 3, 3, 7) 0
=================================================================
Total params: 14,852,775
Trainable params: 14,852,775
Non-trainable params: 0
_________________________________________________________________
Error:
ValueError: Error when checking target: expected activation_7 to have 4 dimensions, but got array with shape (246, 7)
Model Code:
def build_model(img_rows, img_cols, num_channels):
model = Sequential()
input_shape = (img_rows, img_cols, num_channels)
if K.image_data_format() == "channels_first":
input_shape = (num_channels, img_rows, img_cols)
model.add(Conv2D(filters=16, kernel_size=3, padding="same", input_shape=input_shape))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=32, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=64, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=128, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=512, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(MaxPooling2D(pool_size=(2,2), strides=2))
model.add(Conv2D(filters=1024, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
model.add(Conv2D(filters=1024, kernel_size=3, padding="same"))
# model.add(LeakyReLU())
model.add(Activation("relu"))
#Softmax layer (filters: p_c, b_x, b_y, b_w, b_h, 2 classes)
model.add(Conv2D(filters=7, kernel_size=1, padding="same"))
model.add(Activation("linear"))
return model
Training Code:
def main():
# Put training data and labels into variables.
(train_images, train_labels), (test_images, test_labels) = get_data()
# Normalize training data variables.
train_images = train_images.astype("float32")/225.0
test_images = test_images.astype("float32")/225.0
# Create tiny-Yolo model
model = TinyYolo.build_model(100, 100, 3)
model.summary()
optimizer = SGD(lr = 0.01)
model.compile(loss = "categorical_crossentropy", optimizer=optimizer, metrics=["accuracy"])
model.fit(train_images, train_labels, batch_size=12, epochs=20, verbose=1)
(loss, accuracy) = model.evaluate(test_images, test_labels, batch_size=12,verbose=1)
python tensorflow keras yolo
python tensorflow keras yolo
edited 2 days ago
asked 2 days ago
Jack-P
42
42
1
Add the code for creating the model. and training.
– Or Dinari
2 days ago
add a comment |
1
Add the code for creating the model. and training.
– Or Dinari
2 days ago
1
1
Add the code for creating the model. and training.
– Or Dinari
2 days ago
Add the code for creating the model. and training.
– Or Dinari
2 days ago
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53372672%2fpassing-labels-for-tiny-yolo-model-using-keras-expected-activation-7-to-have-4%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
SGbq2PY
1
Add the code for creating the model. and training.
– Or Dinari
2 days ago