Laravel 5.5 Validation errors are not displaying in case form validation fails
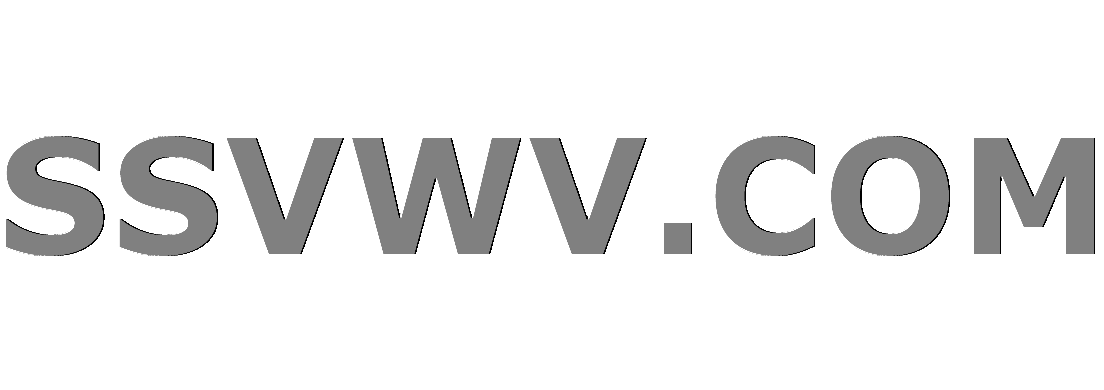
Multi tool use
up vote
0
down vote
favorite
I am trying to display form errors in case form validation fails. Everything works fine and form is validated correctly but it does not display form errors in view. Every time an empty array is returned as errors.
namespace AppHttpControllers;
use IlluminateHttpRequest;
use IlluminateSupportFacadesAuth;
class HomeController extends Controller
{
public function storeProjectDetails(Request $request)
{
$messages = [
'title.required' => 'Please enter trip title',
'title.max' => 'Only 254 characters are allowed as trip title',
'startDate.required' => 'Please enter trip start date',
'startDate.date' => 'Only date formats are allowed as start date',
'endDate.required' => 'Please enter trip end date',
'endDate.date' => 'Only date formats are allowed as end date',
];
$this->validate($request,[
'title' => 'required|string|max:254',
'startDate' => 'required|date',
'endDate' => 'required|date',
]);
}
}
View:
print_r($errors->all());
laravel laravel-5.5
add a comment |
up vote
0
down vote
favorite
I am trying to display form errors in case form validation fails. Everything works fine and form is validated correctly but it does not display form errors in view. Every time an empty array is returned as errors.
namespace AppHttpControllers;
use IlluminateHttpRequest;
use IlluminateSupportFacadesAuth;
class HomeController extends Controller
{
public function storeProjectDetails(Request $request)
{
$messages = [
'title.required' => 'Please enter trip title',
'title.max' => 'Only 254 characters are allowed as trip title',
'startDate.required' => 'Please enter trip start date',
'startDate.date' => 'Only date formats are allowed as start date',
'endDate.required' => 'Please enter trip end date',
'endDate.date' => 'Only date formats are allowed as end date',
];
$this->validate($request,[
'title' => 'required|string|max:254',
'startDate' => 'required|date',
'endDate' => 'required|date',
]);
}
}
View:
print_r($errors->all());
laravel laravel-5.5
What if you trydd($errors)
instead ofprint_r($errors->all());
?
– cbaconnier
Nov 20 at 8:20
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am trying to display form errors in case form validation fails. Everything works fine and form is validated correctly but it does not display form errors in view. Every time an empty array is returned as errors.
namespace AppHttpControllers;
use IlluminateHttpRequest;
use IlluminateSupportFacadesAuth;
class HomeController extends Controller
{
public function storeProjectDetails(Request $request)
{
$messages = [
'title.required' => 'Please enter trip title',
'title.max' => 'Only 254 characters are allowed as trip title',
'startDate.required' => 'Please enter trip start date',
'startDate.date' => 'Only date formats are allowed as start date',
'endDate.required' => 'Please enter trip end date',
'endDate.date' => 'Only date formats are allowed as end date',
];
$this->validate($request,[
'title' => 'required|string|max:254',
'startDate' => 'required|date',
'endDate' => 'required|date',
]);
}
}
View:
print_r($errors->all());
laravel laravel-5.5
I am trying to display form errors in case form validation fails. Everything works fine and form is validated correctly but it does not display form errors in view. Every time an empty array is returned as errors.
namespace AppHttpControllers;
use IlluminateHttpRequest;
use IlluminateSupportFacadesAuth;
class HomeController extends Controller
{
public function storeProjectDetails(Request $request)
{
$messages = [
'title.required' => 'Please enter trip title',
'title.max' => 'Only 254 characters are allowed as trip title',
'startDate.required' => 'Please enter trip start date',
'startDate.date' => 'Only date formats are allowed as start date',
'endDate.required' => 'Please enter trip end date',
'endDate.date' => 'Only date formats are allowed as end date',
];
$this->validate($request,[
'title' => 'required|string|max:254',
'startDate' => 'required|date',
'endDate' => 'required|date',
]);
}
}
View:
print_r($errors->all());
laravel laravel-5.5
laravel laravel-5.5
asked Nov 20 at 7:56
aishazafar
3122520
3122520
What if you trydd($errors)
instead ofprint_r($errors->all());
?
– cbaconnier
Nov 20 at 8:20
add a comment |
What if you trydd($errors)
instead ofprint_r($errors->all());
?
– cbaconnier
Nov 20 at 8:20
What if you try
dd($errors)
instead of print_r($errors->all());
?– cbaconnier
Nov 20 at 8:20
What if you try
dd($errors)
instead of print_r($errors->all());
?– cbaconnier
Nov 20 at 8:20
add a comment |
3 Answers
3
active
oldest
votes
up vote
0
down vote
used this
@if($errors->has())
@foreach ($errors->all() as $error)
<div>{{ $error }}</div>
@endforeach
@endif
add a comment |
up vote
0
down vote
First you need to check for errors using an if
condition then you need to print the errors using a loop as given below
@if ($errors->any())
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
@endif
In your case, you are missing to return the errors array in your controller. Try the validation check given below.
$this->validate($request,[
'title' => 'required|string|max:254',
'startDate' => 'required|date',
'endDate' => 'required|date',
], $messages);
If we don't return messages with validate function it displays default messages for required and other rules. So this is not the issue. There is something else due to that errors are not being displayed.
– aishazafar
Nov 20 at 8:11
did you try displaying errors as shown in my answer?
– Bonish Koirala
Nov 20 at 8:13
yes. That's only a way to display errors. Which can be done with print_r too.
– aishazafar
Nov 20 at 8:16
How did you know that its validating correctly yet you didn't able to see the error?
– Jesus Erwin Suarez
Nov 20 at 8:23
@JesusErwinSuarez after your question I modified the code and checked again thinking there may be my mistake. Even only with required rule it does not display any error.
– aishazafar
Nov 20 at 8:48
|
show 1 more comment
up vote
0
down vote
You are building the messages array in a wrong format, it should look like this:
$messages = [
'required' => 'Please enter :attribute',
'date' => 'Only date formats are allowed as :attribute',
...
];
There is no issue in messages. messages can be set like this too.
– aishazafar
Nov 22 at 6:27
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
used this
@if($errors->has())
@foreach ($errors->all() as $error)
<div>{{ $error }}</div>
@endforeach
@endif
add a comment |
up vote
0
down vote
used this
@if($errors->has())
@foreach ($errors->all() as $error)
<div>{{ $error }}</div>
@endforeach
@endif
add a comment |
up vote
0
down vote
up vote
0
down vote
used this
@if($errors->has())
@foreach ($errors->all() as $error)
<div>{{ $error }}</div>
@endforeach
@endif
used this
@if($errors->has())
@foreach ($errors->all() as $error)
<div>{{ $error }}</div>
@endforeach
@endif
answered Nov 20 at 8:00
Usman Jdn
437113
437113
add a comment |
add a comment |
up vote
0
down vote
First you need to check for errors using an if
condition then you need to print the errors using a loop as given below
@if ($errors->any())
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
@endif
In your case, you are missing to return the errors array in your controller. Try the validation check given below.
$this->validate($request,[
'title' => 'required|string|max:254',
'startDate' => 'required|date',
'endDate' => 'required|date',
], $messages);
If we don't return messages with validate function it displays default messages for required and other rules. So this is not the issue. There is something else due to that errors are not being displayed.
– aishazafar
Nov 20 at 8:11
did you try displaying errors as shown in my answer?
– Bonish Koirala
Nov 20 at 8:13
yes. That's only a way to display errors. Which can be done with print_r too.
– aishazafar
Nov 20 at 8:16
How did you know that its validating correctly yet you didn't able to see the error?
– Jesus Erwin Suarez
Nov 20 at 8:23
@JesusErwinSuarez after your question I modified the code and checked again thinking there may be my mistake. Even only with required rule it does not display any error.
– aishazafar
Nov 20 at 8:48
|
show 1 more comment
up vote
0
down vote
First you need to check for errors using an if
condition then you need to print the errors using a loop as given below
@if ($errors->any())
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
@endif
In your case, you are missing to return the errors array in your controller. Try the validation check given below.
$this->validate($request,[
'title' => 'required|string|max:254',
'startDate' => 'required|date',
'endDate' => 'required|date',
], $messages);
If we don't return messages with validate function it displays default messages for required and other rules. So this is not the issue. There is something else due to that errors are not being displayed.
– aishazafar
Nov 20 at 8:11
did you try displaying errors as shown in my answer?
– Bonish Koirala
Nov 20 at 8:13
yes. That's only a way to display errors. Which can be done with print_r too.
– aishazafar
Nov 20 at 8:16
How did you know that its validating correctly yet you didn't able to see the error?
– Jesus Erwin Suarez
Nov 20 at 8:23
@JesusErwinSuarez after your question I modified the code and checked again thinking there may be my mistake. Even only with required rule it does not display any error.
– aishazafar
Nov 20 at 8:48
|
show 1 more comment
up vote
0
down vote
up vote
0
down vote
First you need to check for errors using an if
condition then you need to print the errors using a loop as given below
@if ($errors->any())
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
@endif
In your case, you are missing to return the errors array in your controller. Try the validation check given below.
$this->validate($request,[
'title' => 'required|string|max:254',
'startDate' => 'required|date',
'endDate' => 'required|date',
], $messages);
First you need to check for errors using an if
condition then you need to print the errors using a loop as given below
@if ($errors->any())
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
@endif
In your case, you are missing to return the errors array in your controller. Try the validation check given below.
$this->validate($request,[
'title' => 'required|string|max:254',
'startDate' => 'required|date',
'endDate' => 'required|date',
], $messages);
answered Nov 20 at 8:02


Bonish Koirala
4038
4038
If we don't return messages with validate function it displays default messages for required and other rules. So this is not the issue. There is something else due to that errors are not being displayed.
– aishazafar
Nov 20 at 8:11
did you try displaying errors as shown in my answer?
– Bonish Koirala
Nov 20 at 8:13
yes. That's only a way to display errors. Which can be done with print_r too.
– aishazafar
Nov 20 at 8:16
How did you know that its validating correctly yet you didn't able to see the error?
– Jesus Erwin Suarez
Nov 20 at 8:23
@JesusErwinSuarez after your question I modified the code and checked again thinking there may be my mistake. Even only with required rule it does not display any error.
– aishazafar
Nov 20 at 8:48
|
show 1 more comment
If we don't return messages with validate function it displays default messages for required and other rules. So this is not the issue. There is something else due to that errors are not being displayed.
– aishazafar
Nov 20 at 8:11
did you try displaying errors as shown in my answer?
– Bonish Koirala
Nov 20 at 8:13
yes. That's only a way to display errors. Which can be done with print_r too.
– aishazafar
Nov 20 at 8:16
How did you know that its validating correctly yet you didn't able to see the error?
– Jesus Erwin Suarez
Nov 20 at 8:23
@JesusErwinSuarez after your question I modified the code and checked again thinking there may be my mistake. Even only with required rule it does not display any error.
– aishazafar
Nov 20 at 8:48
If we don't return messages with validate function it displays default messages for required and other rules. So this is not the issue. There is something else due to that errors are not being displayed.
– aishazafar
Nov 20 at 8:11
If we don't return messages with validate function it displays default messages for required and other rules. So this is not the issue. There is something else due to that errors are not being displayed.
– aishazafar
Nov 20 at 8:11
did you try displaying errors as shown in my answer?
– Bonish Koirala
Nov 20 at 8:13
did you try displaying errors as shown in my answer?
– Bonish Koirala
Nov 20 at 8:13
yes. That's only a way to display errors. Which can be done with print_r too.
– aishazafar
Nov 20 at 8:16
yes. That's only a way to display errors. Which can be done with print_r too.
– aishazafar
Nov 20 at 8:16
How did you know that its validating correctly yet you didn't able to see the error?
– Jesus Erwin Suarez
Nov 20 at 8:23
How did you know that its validating correctly yet you didn't able to see the error?
– Jesus Erwin Suarez
Nov 20 at 8:23
@JesusErwinSuarez after your question I modified the code and checked again thinking there may be my mistake. Even only with required rule it does not display any error.
– aishazafar
Nov 20 at 8:48
@JesusErwinSuarez after your question I modified the code and checked again thinking there may be my mistake. Even only with required rule it does not display any error.
– aishazafar
Nov 20 at 8:48
|
show 1 more comment
up vote
0
down vote
You are building the messages array in a wrong format, it should look like this:
$messages = [
'required' => 'Please enter :attribute',
'date' => 'Only date formats are allowed as :attribute',
...
];
There is no issue in messages. messages can be set like this too.
– aishazafar
Nov 22 at 6:27
add a comment |
up vote
0
down vote
You are building the messages array in a wrong format, it should look like this:
$messages = [
'required' => 'Please enter :attribute',
'date' => 'Only date formats are allowed as :attribute',
...
];
There is no issue in messages. messages can be set like this too.
– aishazafar
Nov 22 at 6:27
add a comment |
up vote
0
down vote
up vote
0
down vote
You are building the messages array in a wrong format, it should look like this:
$messages = [
'required' => 'Please enter :attribute',
'date' => 'Only date formats are allowed as :attribute',
...
];
You are building the messages array in a wrong format, it should look like this:
$messages = [
'required' => 'Please enter :attribute',
'date' => 'Only date formats are allowed as :attribute',
...
];
answered Nov 21 at 21:22


Arthur Samarcos
2,1451420
2,1451420
There is no issue in messages. messages can be set like this too.
– aishazafar
Nov 22 at 6:27
add a comment |
There is no issue in messages. messages can be set like this too.
– aishazafar
Nov 22 at 6:27
There is no issue in messages. messages can be set like this too.
– aishazafar
Nov 22 at 6:27
There is no issue in messages. messages can be set like this too.
– aishazafar
Nov 22 at 6:27
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53388506%2flaravel-5-5-validation-errors-are-not-displaying-in-case-form-validation-fails%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
y2XVLhk51Udy uOL5JY6A,fXHoMtchQlP9kFWXsXzro l mGISij6J6uF 2TNCZ lRlPSsIbZ,c
What if you try
dd($errors)
instead ofprint_r($errors->all());
?– cbaconnier
Nov 20 at 8:20