Complex relationship formation in Laravel 5
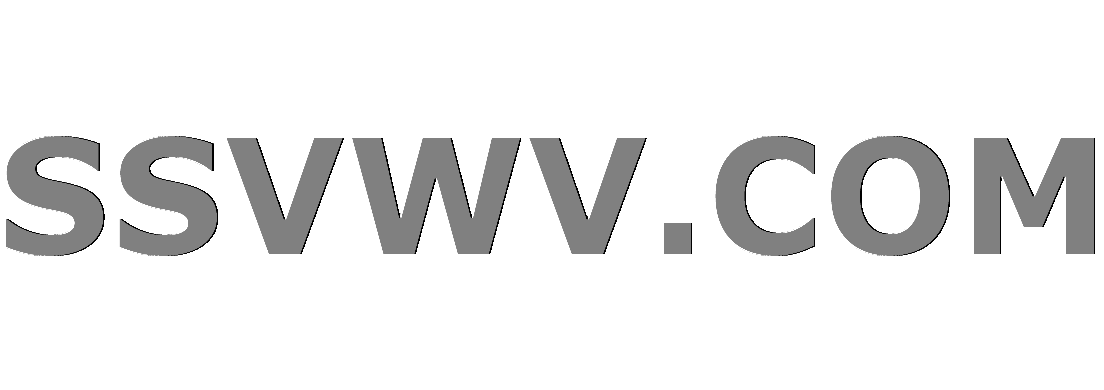
Multi tool use
I'm working on a complex shopping cart project. I have relationships like this
CategoryGroup model
// AppCategoryGroup
class CategoryGroup extend Model
{
public function categories()
{
return $this->hasMany(Category::class);
}
}
Category model
// AppCategory
class Inventory extend Model
{
public function categoryGroup()
{
return $this->belongsTo(CategoryGroup::class);
}
public function products()
{
return $this->belongsToMany(Product::class);
}
public function listings()
{
return $this->belongsToMany(
Inventory::class,
'category_product',
null,
'product_id',
null,
'product_id'
);
}
}
Product model
// AppProduct
class Product extend Model
{
public function categories()
{
return $this->belongsToMany(Category::class);
}
public function listings()
{
return $this->hasMany(Inventory::class);
}
}
Inventory model
// AppInventory
class Inventory extend Model
{
public function products()
{
return $this->belongsTo(Product::class);
}
}
Now I'm stuck on the situation where I need to create a relationship between The CategoryGroups and the Inventory model like this:
// AppCategoryGroup
class CategoryGroup extend Model
{
public function categories()
{
return $this->hasMany(Category::class);
}
public function listings()
{
// Can't figured out the way
// A belongsToMany like the AppCategory would be great
}
}
Is there a good way to achieve this kind of relationship?
laravel-5 eloquent laravel-5.5 laravel-query-builder eloquent--relationship
add a comment |
I'm working on a complex shopping cart project. I have relationships like this
CategoryGroup model
// AppCategoryGroup
class CategoryGroup extend Model
{
public function categories()
{
return $this->hasMany(Category::class);
}
}
Category model
// AppCategory
class Inventory extend Model
{
public function categoryGroup()
{
return $this->belongsTo(CategoryGroup::class);
}
public function products()
{
return $this->belongsToMany(Product::class);
}
public function listings()
{
return $this->belongsToMany(
Inventory::class,
'category_product',
null,
'product_id',
null,
'product_id'
);
}
}
Product model
// AppProduct
class Product extend Model
{
public function categories()
{
return $this->belongsToMany(Category::class);
}
public function listings()
{
return $this->hasMany(Inventory::class);
}
}
Inventory model
// AppInventory
class Inventory extend Model
{
public function products()
{
return $this->belongsTo(Product::class);
}
}
Now I'm stuck on the situation where I need to create a relationship between The CategoryGroups and the Inventory model like this:
// AppCategoryGroup
class CategoryGroup extend Model
{
public function categories()
{
return $this->hasMany(Category::class);
}
public function listings()
{
// Can't figured out the way
// A belongsToMany like the AppCategory would be great
}
}
Is there a good way to achieve this kind of relationship?
laravel-5 eloquent laravel-5.5 laravel-query-builder eloquent--relationship
add a comment |
I'm working on a complex shopping cart project. I have relationships like this
CategoryGroup model
// AppCategoryGroup
class CategoryGroup extend Model
{
public function categories()
{
return $this->hasMany(Category::class);
}
}
Category model
// AppCategory
class Inventory extend Model
{
public function categoryGroup()
{
return $this->belongsTo(CategoryGroup::class);
}
public function products()
{
return $this->belongsToMany(Product::class);
}
public function listings()
{
return $this->belongsToMany(
Inventory::class,
'category_product',
null,
'product_id',
null,
'product_id'
);
}
}
Product model
// AppProduct
class Product extend Model
{
public function categories()
{
return $this->belongsToMany(Category::class);
}
public function listings()
{
return $this->hasMany(Inventory::class);
}
}
Inventory model
// AppInventory
class Inventory extend Model
{
public function products()
{
return $this->belongsTo(Product::class);
}
}
Now I'm stuck on the situation where I need to create a relationship between The CategoryGroups and the Inventory model like this:
// AppCategoryGroup
class CategoryGroup extend Model
{
public function categories()
{
return $this->hasMany(Category::class);
}
public function listings()
{
// Can't figured out the way
// A belongsToMany like the AppCategory would be great
}
}
Is there a good way to achieve this kind of relationship?
laravel-5 eloquent laravel-5.5 laravel-query-builder eloquent--relationship
I'm working on a complex shopping cart project. I have relationships like this
CategoryGroup model
// AppCategoryGroup
class CategoryGroup extend Model
{
public function categories()
{
return $this->hasMany(Category::class);
}
}
Category model
// AppCategory
class Inventory extend Model
{
public function categoryGroup()
{
return $this->belongsTo(CategoryGroup::class);
}
public function products()
{
return $this->belongsToMany(Product::class);
}
public function listings()
{
return $this->belongsToMany(
Inventory::class,
'category_product',
null,
'product_id',
null,
'product_id'
);
}
}
Product model
// AppProduct
class Product extend Model
{
public function categories()
{
return $this->belongsToMany(Category::class);
}
public function listings()
{
return $this->hasMany(Inventory::class);
}
}
Inventory model
// AppInventory
class Inventory extend Model
{
public function products()
{
return $this->belongsTo(Product::class);
}
}
Now I'm stuck on the situation where I need to create a relationship between The CategoryGroups and the Inventory model like this:
// AppCategoryGroup
class CategoryGroup extend Model
{
public function categories()
{
return $this->hasMany(Category::class);
}
public function listings()
{
// Can't figured out the way
// A belongsToMany like the AppCategory would be great
}
}
Is there a good way to achieve this kind of relationship?
laravel-5 eloquent laravel-5.5 laravel-query-builder eloquent--relationship
laravel-5 eloquent laravel-5.5 laravel-query-builder eloquent--relationship
edited Mar 6 at 19:34
Munna Khan
asked Nov 25 '18 at 21:21
Munna KhanMunna Khan
410519
410519
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Laravel has no native support for a direct relationship.
I created a package for cases like this: https://github.com/staudenmeir/eloquent-has-many-deep
You can use it like this:
class CategoryGroup extends Model {
use StaudenmeirEloquentHasManyDeepHasRelationships;
public function inventories() {
return $this->hasManyDeep(
Inventory::class,
[Category::class, 'category_product', Product::class]
);
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472108%2fcomplex-relationship-formation-in-laravel-5%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Laravel has no native support for a direct relationship.
I created a package for cases like this: https://github.com/staudenmeir/eloquent-has-many-deep
You can use it like this:
class CategoryGroup extends Model {
use StaudenmeirEloquentHasManyDeepHasRelationships;
public function inventories() {
return $this->hasManyDeep(
Inventory::class,
[Category::class, 'category_product', Product::class]
);
}
}
add a comment |
Laravel has no native support for a direct relationship.
I created a package for cases like this: https://github.com/staudenmeir/eloquent-has-many-deep
You can use it like this:
class CategoryGroup extends Model {
use StaudenmeirEloquentHasManyDeepHasRelationships;
public function inventories() {
return $this->hasManyDeep(
Inventory::class,
[Category::class, 'category_product', Product::class]
);
}
}
add a comment |
Laravel has no native support for a direct relationship.
I created a package for cases like this: https://github.com/staudenmeir/eloquent-has-many-deep
You can use it like this:
class CategoryGroup extends Model {
use StaudenmeirEloquentHasManyDeepHasRelationships;
public function inventories() {
return $this->hasManyDeep(
Inventory::class,
[Category::class, 'category_product', Product::class]
);
}
}
Laravel has no native support for a direct relationship.
I created a package for cases like this: https://github.com/staudenmeir/eloquent-has-many-deep
You can use it like this:
class CategoryGroup extends Model {
use StaudenmeirEloquentHasManyDeepHasRelationships;
public function inventories() {
return $this->hasManyDeep(
Inventory::class,
[Category::class, 'category_product', Product::class]
);
}
}
answered Nov 26 '18 at 1:47


Jonas StaudenmeirJonas Staudenmeir
13.3k2937
13.3k2937
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472108%2fcomplex-relationship-formation-in-laravel-5%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uc,skgHLZ74URt7CwkI20sMYquF VF,Y,N51P7yxZToEPyv1hwGJpoQ66ug