Left-aligning vertical UILabel in Swift
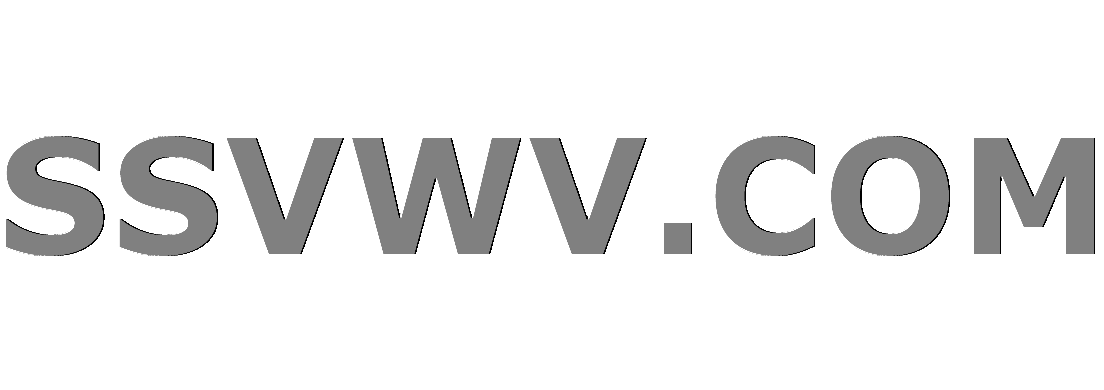
Multi tool use
I'm working inside a UICollectionViewCell, trying to get a rotated UILabel to stick to the left of the label at a fixed width. This is what I have been able achieve:
As you can see, the label's dimensions seem relative to the length of the text and disabling auto-resizing has no effect. I would like to constrain the label to ~80 and occupy the full height of the cell, enough for the font with some spacing. The entire code for the UICollectionViewCell:
import Foundation
import UIKit
class DayOfWeekCell: UICollectionViewCell {
let categoryLabel = UILabel()
override init(frame: CGRect) {
super.init(frame: frame)
backgroundColor = .red
categoryLabel.transform = CGAffineTransform.init(rotationAngle: -CGFloat.pi/2)
categoryLabel.textColor = UIColor.white
categoryLabel.font = UIFont.systemFont(ofSize: 25)
categoryLabel.translatesAutoresizingMaskIntoConstraints = false
addSubview(categoryLabel)
categoryLabel.backgroundColor = .blue
categoryLabel.leadingAnchor.constraint(equalTo: self.leadingAnchor, constant: 0).isActive = true
categoryLabel.topAnchor.constraint(equalTo: self.topAnchor, constant: 0).isActive = true
categoryLabel.bottomAnchor.constraint(equalTo: self.bottomAnchor, constant: 0).isActive = true
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
The ViewController sizeForItem returns the view width with 180 height. It does not play with anything of the cell, only setting the label's text.
I am still relatively new to iOS but have spent the past hour tinkering with this and just cannot get it to play nice! SnapKit is imported but I have had no success with it either. Is there some autosizing going on I'm not aware of? Any help would be greatly appreciated!
ios swift alignment uilabel snapkit
add a comment |
I'm working inside a UICollectionViewCell, trying to get a rotated UILabel to stick to the left of the label at a fixed width. This is what I have been able achieve:
As you can see, the label's dimensions seem relative to the length of the text and disabling auto-resizing has no effect. I would like to constrain the label to ~80 and occupy the full height of the cell, enough for the font with some spacing. The entire code for the UICollectionViewCell:
import Foundation
import UIKit
class DayOfWeekCell: UICollectionViewCell {
let categoryLabel = UILabel()
override init(frame: CGRect) {
super.init(frame: frame)
backgroundColor = .red
categoryLabel.transform = CGAffineTransform.init(rotationAngle: -CGFloat.pi/2)
categoryLabel.textColor = UIColor.white
categoryLabel.font = UIFont.systemFont(ofSize: 25)
categoryLabel.translatesAutoresizingMaskIntoConstraints = false
addSubview(categoryLabel)
categoryLabel.backgroundColor = .blue
categoryLabel.leadingAnchor.constraint(equalTo: self.leadingAnchor, constant: 0).isActive = true
categoryLabel.topAnchor.constraint(equalTo: self.topAnchor, constant: 0).isActive = true
categoryLabel.bottomAnchor.constraint(equalTo: self.bottomAnchor, constant: 0).isActive = true
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
The ViewController sizeForItem returns the view width with 180 height. It does not play with anything of the cell, only setting the label's text.
I am still relatively new to iOS but have spent the past hour tinkering with this and just cannot get it to play nice! SnapKit is imported but I have had no success with it either. Is there some autosizing going on I'm not aware of? Any help would be greatly appreciated!
ios swift alignment uilabel snapkit
Can you try to: set the content hugging priority to required, then increase the constant in the leading constraint? developer.apple.com/documentation/uikit/uiview/…
– josh-fuggle
Nov 25 '18 at 22:23
@josh-fuggle Gave that a go. The hugging priority doesn't seem to have any effect (tried on both axis'), increasing the constant pushes the label away from the left
– Ox1
Nov 25 '18 at 22:34
add a comment |
I'm working inside a UICollectionViewCell, trying to get a rotated UILabel to stick to the left of the label at a fixed width. This is what I have been able achieve:
As you can see, the label's dimensions seem relative to the length of the text and disabling auto-resizing has no effect. I would like to constrain the label to ~80 and occupy the full height of the cell, enough for the font with some spacing. The entire code for the UICollectionViewCell:
import Foundation
import UIKit
class DayOfWeekCell: UICollectionViewCell {
let categoryLabel = UILabel()
override init(frame: CGRect) {
super.init(frame: frame)
backgroundColor = .red
categoryLabel.transform = CGAffineTransform.init(rotationAngle: -CGFloat.pi/2)
categoryLabel.textColor = UIColor.white
categoryLabel.font = UIFont.systemFont(ofSize: 25)
categoryLabel.translatesAutoresizingMaskIntoConstraints = false
addSubview(categoryLabel)
categoryLabel.backgroundColor = .blue
categoryLabel.leadingAnchor.constraint(equalTo: self.leadingAnchor, constant: 0).isActive = true
categoryLabel.topAnchor.constraint(equalTo: self.topAnchor, constant: 0).isActive = true
categoryLabel.bottomAnchor.constraint(equalTo: self.bottomAnchor, constant: 0).isActive = true
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
The ViewController sizeForItem returns the view width with 180 height. It does not play with anything of the cell, only setting the label's text.
I am still relatively new to iOS but have spent the past hour tinkering with this and just cannot get it to play nice! SnapKit is imported but I have had no success with it either. Is there some autosizing going on I'm not aware of? Any help would be greatly appreciated!
ios swift alignment uilabel snapkit
I'm working inside a UICollectionViewCell, trying to get a rotated UILabel to stick to the left of the label at a fixed width. This is what I have been able achieve:
As you can see, the label's dimensions seem relative to the length of the text and disabling auto-resizing has no effect. I would like to constrain the label to ~80 and occupy the full height of the cell, enough for the font with some spacing. The entire code for the UICollectionViewCell:
import Foundation
import UIKit
class DayOfWeekCell: UICollectionViewCell {
let categoryLabel = UILabel()
override init(frame: CGRect) {
super.init(frame: frame)
backgroundColor = .red
categoryLabel.transform = CGAffineTransform.init(rotationAngle: -CGFloat.pi/2)
categoryLabel.textColor = UIColor.white
categoryLabel.font = UIFont.systemFont(ofSize: 25)
categoryLabel.translatesAutoresizingMaskIntoConstraints = false
addSubview(categoryLabel)
categoryLabel.backgroundColor = .blue
categoryLabel.leadingAnchor.constraint(equalTo: self.leadingAnchor, constant: 0).isActive = true
categoryLabel.topAnchor.constraint(equalTo: self.topAnchor, constant: 0).isActive = true
categoryLabel.bottomAnchor.constraint(equalTo: self.bottomAnchor, constant: 0).isActive = true
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
The ViewController sizeForItem returns the view width with 180 height. It does not play with anything of the cell, only setting the label's text.
I am still relatively new to iOS but have spent the past hour tinkering with this and just cannot get it to play nice! SnapKit is imported but I have had no success with it either. Is there some autosizing going on I'm not aware of? Any help would be greatly appreciated!
ios swift alignment uilabel snapkit
ios swift alignment uilabel snapkit
asked Nov 25 '18 at 21:31


Ox1Ox1
5018
5018
Can you try to: set the content hugging priority to required, then increase the constant in the leading constraint? developer.apple.com/documentation/uikit/uiview/…
– josh-fuggle
Nov 25 '18 at 22:23
@josh-fuggle Gave that a go. The hugging priority doesn't seem to have any effect (tried on both axis'), increasing the constant pushes the label away from the left
– Ox1
Nov 25 '18 at 22:34
add a comment |
Can you try to: set the content hugging priority to required, then increase the constant in the leading constraint? developer.apple.com/documentation/uikit/uiview/…
– josh-fuggle
Nov 25 '18 at 22:23
@josh-fuggle Gave that a go. The hugging priority doesn't seem to have any effect (tried on both axis'), increasing the constant pushes the label away from the left
– Ox1
Nov 25 '18 at 22:34
Can you try to: set the content hugging priority to required, then increase the constant in the leading constraint? developer.apple.com/documentation/uikit/uiview/…
– josh-fuggle
Nov 25 '18 at 22:23
Can you try to: set the content hugging priority to required, then increase the constant in the leading constraint? developer.apple.com/documentation/uikit/uiview/…
– josh-fuggle
Nov 25 '18 at 22:23
@josh-fuggle Gave that a go. The hugging priority doesn't seem to have any effect (tried on both axis'), increasing the constant pushes the label away from the left
– Ox1
Nov 25 '18 at 22:34
@josh-fuggle Gave that a go. The hugging priority doesn't seem to have any effect (tried on both axis'), increasing the constant pushes the label away from the left
– Ox1
Nov 25 '18 at 22:34
add a comment |
1 Answer
1
active
oldest
votes
I guess there is an issue with the constraints applied using anchors. As all the bottom anchor is also applied, the anchor point is different for different texts. I managed get a decent alignment by applying translating the position again by doing
let transform = CGAffineTransform(rotationAngle: -.pi / 2).translatedBy(x: -25, y: -50)
categoryLabel.transform = transform
Constraints applied to the category label was aligned to leftAnchor, topAnchor and with a width of 100.
Transforming on the axis turned out to be the issue. The length of text determined the width which anchoring just wouldn't mix with. In the end I used a frame to position the label since the alignment must be dead center. Good solution though, I tried it!
– Ox1
Nov 26 '18 at 13:12
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472212%2fleft-aligning-vertical-uilabel-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I guess there is an issue with the constraints applied using anchors. As all the bottom anchor is also applied, the anchor point is different for different texts. I managed get a decent alignment by applying translating the position again by doing
let transform = CGAffineTransform(rotationAngle: -.pi / 2).translatedBy(x: -25, y: -50)
categoryLabel.transform = transform
Constraints applied to the category label was aligned to leftAnchor, topAnchor and with a width of 100.
Transforming on the axis turned out to be the issue. The length of text determined the width which anchoring just wouldn't mix with. In the end I used a frame to position the label since the alignment must be dead center. Good solution though, I tried it!
– Ox1
Nov 26 '18 at 13:12
add a comment |
I guess there is an issue with the constraints applied using anchors. As all the bottom anchor is also applied, the anchor point is different for different texts. I managed get a decent alignment by applying translating the position again by doing
let transform = CGAffineTransform(rotationAngle: -.pi / 2).translatedBy(x: -25, y: -50)
categoryLabel.transform = transform
Constraints applied to the category label was aligned to leftAnchor, topAnchor and with a width of 100.
Transforming on the axis turned out to be the issue. The length of text determined the width which anchoring just wouldn't mix with. In the end I used a frame to position the label since the alignment must be dead center. Good solution though, I tried it!
– Ox1
Nov 26 '18 at 13:12
add a comment |
I guess there is an issue with the constraints applied using anchors. As all the bottom anchor is also applied, the anchor point is different for different texts. I managed get a decent alignment by applying translating the position again by doing
let transform = CGAffineTransform(rotationAngle: -.pi / 2).translatedBy(x: -25, y: -50)
categoryLabel.transform = transform
Constraints applied to the category label was aligned to leftAnchor, topAnchor and with a width of 100.
I guess there is an issue with the constraints applied using anchors. As all the bottom anchor is also applied, the anchor point is different for different texts. I managed get a decent alignment by applying translating the position again by doing
let transform = CGAffineTransform(rotationAngle: -.pi / 2).translatedBy(x: -25, y: -50)
categoryLabel.transform = transform
Constraints applied to the category label was aligned to leftAnchor, topAnchor and with a width of 100.
answered Nov 26 '18 at 3:08
Arun BArun B
1,171821
1,171821
Transforming on the axis turned out to be the issue. The length of text determined the width which anchoring just wouldn't mix with. In the end I used a frame to position the label since the alignment must be dead center. Good solution though, I tried it!
– Ox1
Nov 26 '18 at 13:12
add a comment |
Transforming on the axis turned out to be the issue. The length of text determined the width which anchoring just wouldn't mix with. In the end I used a frame to position the label since the alignment must be dead center. Good solution though, I tried it!
– Ox1
Nov 26 '18 at 13:12
Transforming on the axis turned out to be the issue. The length of text determined the width which anchoring just wouldn't mix with. In the end I used a frame to position the label since the alignment must be dead center. Good solution though, I tried it!
– Ox1
Nov 26 '18 at 13:12
Transforming on the axis turned out to be the issue. The length of text determined the width which anchoring just wouldn't mix with. In the end I used a frame to position the label since the alignment must be dead center. Good solution though, I tried it!
– Ox1
Nov 26 '18 at 13:12
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472212%2fleft-aligning-vertical-uilabel-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Q,Qy8FI7P,iKHVSrAE6V GE5pqp37s,zIR6 CeB Nzk 3Vof0Wqvtl
Can you try to: set the content hugging priority to required, then increase the constant in the leading constraint? developer.apple.com/documentation/uikit/uiview/…
– josh-fuggle
Nov 25 '18 at 22:23
@josh-fuggle Gave that a go. The hugging priority doesn't seem to have any effect (tried on both axis'), increasing the constant pushes the label away from the left
– Ox1
Nov 25 '18 at 22:34