Is a function in a for loop declaration will be called for many times in Python?
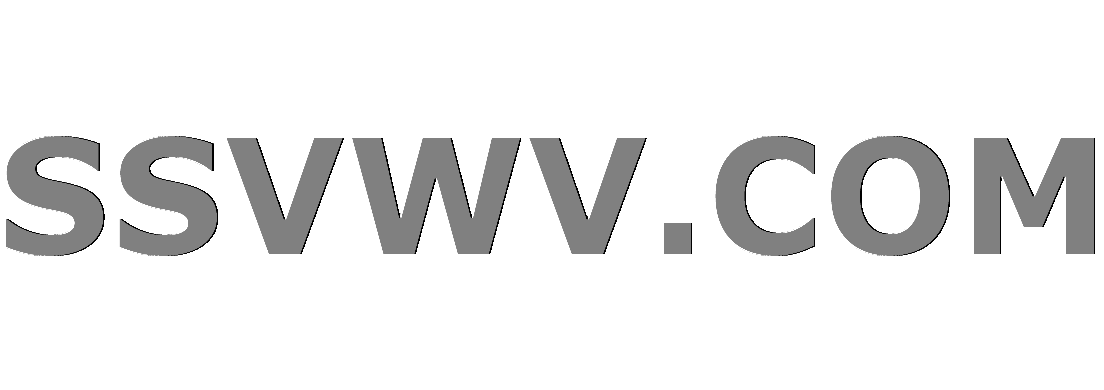
Multi tool use
There is a function and a for loop:
def helper():
return [1,2,3]
for i in helper():
print(i)
I am wondering if the helper function would only be called once at the initialization of the for loop. As I am thinking that if I call the function and assign the return array to a variable in advance, which would be used in the for loop like this:
def helper():
return [1,2,3]
temp = helper()
for i in temp:
print(i)
Is that with less time complexity?
Thanks!
python for-loop time-complexity
add a comment |
There is a function and a for loop:
def helper():
return [1,2,3]
for i in helper():
print(i)
I am wondering if the helper function would only be called once at the initialization of the for loop. As I am thinking that if I call the function and assign the return array to a variable in advance, which would be used in the for loop like this:
def helper():
return [1,2,3]
temp = helper()
for i in temp:
print(i)
Is that with less time complexity?
Thanks!
python for-loop time-complexity
3
No, that is pretty much the same thing, except your first example doesn't need the temp variable so looks cleaner, easier to read etc. If you are ever unsure about things like this, just put print statements in your code to see what's happening.
– SuperShoot
Nov 25 '18 at 21:34
2
It is only evaluated once
– juanpa.arrivillaga
Nov 25 '18 at 21:35
3
You could test this yourself by printing a message inhelper()
, and seeing if that message gets printed once, or more than once.
– John Gordon
Nov 25 '18 at 21:37
Got it, thanks!
– Aurora
Nov 25 '18 at 21:42
Yeah, adding some so-simple-it’s-a-no-brainerprint
statements is all you need to go fromwondering
Toknowing
– barny
Nov 25 '18 at 21:57
add a comment |
There is a function and a for loop:
def helper():
return [1,2,3]
for i in helper():
print(i)
I am wondering if the helper function would only be called once at the initialization of the for loop. As I am thinking that if I call the function and assign the return array to a variable in advance, which would be used in the for loop like this:
def helper():
return [1,2,3]
temp = helper()
for i in temp:
print(i)
Is that with less time complexity?
Thanks!
python for-loop time-complexity
There is a function and a for loop:
def helper():
return [1,2,3]
for i in helper():
print(i)
I am wondering if the helper function would only be called once at the initialization of the for loop. As I am thinking that if I call the function and assign the return array to a variable in advance, which would be used in the for loop like this:
def helper():
return [1,2,3]
temp = helper()
for i in temp:
print(i)
Is that with less time complexity?
Thanks!
python for-loop time-complexity
python for-loop time-complexity
asked Nov 25 '18 at 21:32


AuroraAurora
1098
1098
3
No, that is pretty much the same thing, except your first example doesn't need the temp variable so looks cleaner, easier to read etc. If you are ever unsure about things like this, just put print statements in your code to see what's happening.
– SuperShoot
Nov 25 '18 at 21:34
2
It is only evaluated once
– juanpa.arrivillaga
Nov 25 '18 at 21:35
3
You could test this yourself by printing a message inhelper()
, and seeing if that message gets printed once, or more than once.
– John Gordon
Nov 25 '18 at 21:37
Got it, thanks!
– Aurora
Nov 25 '18 at 21:42
Yeah, adding some so-simple-it’s-a-no-brainerprint
statements is all you need to go fromwondering
Toknowing
– barny
Nov 25 '18 at 21:57
add a comment |
3
No, that is pretty much the same thing, except your first example doesn't need the temp variable so looks cleaner, easier to read etc. If you are ever unsure about things like this, just put print statements in your code to see what's happening.
– SuperShoot
Nov 25 '18 at 21:34
2
It is only evaluated once
– juanpa.arrivillaga
Nov 25 '18 at 21:35
3
You could test this yourself by printing a message inhelper()
, and seeing if that message gets printed once, or more than once.
– John Gordon
Nov 25 '18 at 21:37
Got it, thanks!
– Aurora
Nov 25 '18 at 21:42
Yeah, adding some so-simple-it’s-a-no-brainerprint
statements is all you need to go fromwondering
Toknowing
– barny
Nov 25 '18 at 21:57
3
3
No, that is pretty much the same thing, except your first example doesn't need the temp variable so looks cleaner, easier to read etc. If you are ever unsure about things like this, just put print statements in your code to see what's happening.
– SuperShoot
Nov 25 '18 at 21:34
No, that is pretty much the same thing, except your first example doesn't need the temp variable so looks cleaner, easier to read etc. If you are ever unsure about things like this, just put print statements in your code to see what's happening.
– SuperShoot
Nov 25 '18 at 21:34
2
2
It is only evaluated once
– juanpa.arrivillaga
Nov 25 '18 at 21:35
It is only evaluated once
– juanpa.arrivillaga
Nov 25 '18 at 21:35
3
3
You could test this yourself by printing a message in
helper()
, and seeing if that message gets printed once, or more than once.– John Gordon
Nov 25 '18 at 21:37
You could test this yourself by printing a message in
helper()
, and seeing if that message gets printed once, or more than once.– John Gordon
Nov 25 '18 at 21:37
Got it, thanks!
– Aurora
Nov 25 '18 at 21:42
Got it, thanks!
– Aurora
Nov 25 '18 at 21:42
Yeah, adding some so-simple-it’s-a-no-brainer
print
statements is all you need to go from wondering
To knowing
– barny
Nov 25 '18 at 21:57
Yeah, adding some so-simple-it’s-a-no-brainer
print
statements is all you need to go from wondering
To knowing
– barny
Nov 25 '18 at 21:57
add a comment |
1 Answer
1
active
oldest
votes
use the yield operation:
def helper():
for i in [1,2,3]:
yield i
for i in helper():
print(i)
in this case the helper()
method would return the i
value during each iteration to the calling for
loop.
Cool, thanks so much for your answer.
– Aurora
Nov 27 '18 at 18:25
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472218%2fis-a-function-in-a-for-loop-declaration-will-be-called-for-many-times-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
use the yield operation:
def helper():
for i in [1,2,3]:
yield i
for i in helper():
print(i)
in this case the helper()
method would return the i
value during each iteration to the calling for
loop.
Cool, thanks so much for your answer.
– Aurora
Nov 27 '18 at 18:25
add a comment |
use the yield operation:
def helper():
for i in [1,2,3]:
yield i
for i in helper():
print(i)
in this case the helper()
method would return the i
value during each iteration to the calling for
loop.
Cool, thanks so much for your answer.
– Aurora
Nov 27 '18 at 18:25
add a comment |
use the yield operation:
def helper():
for i in [1,2,3]:
yield i
for i in helper():
print(i)
in this case the helper()
method would return the i
value during each iteration to the calling for
loop.
use the yield operation:
def helper():
for i in [1,2,3]:
yield i
for i in helper():
print(i)
in this case the helper()
method would return the i
value during each iteration to the calling for
loop.
answered Nov 26 '18 at 6:27


Gautham MGautham M
234110
234110
Cool, thanks so much for your answer.
– Aurora
Nov 27 '18 at 18:25
add a comment |
Cool, thanks so much for your answer.
– Aurora
Nov 27 '18 at 18:25
Cool, thanks so much for your answer.
– Aurora
Nov 27 '18 at 18:25
Cool, thanks so much for your answer.
– Aurora
Nov 27 '18 at 18:25
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53472218%2fis-a-function-in-a-for-loop-declaration-will-be-called-for-many-times-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UrcR9 DjrBvwo6,emLoFPBLAuY5SLR4 LRZHwJC 2nYGB0W5KfM2NH4Aub5Ue0Afea,wTfpbP NvJCdXWAvVa0t
3
No, that is pretty much the same thing, except your first example doesn't need the temp variable so looks cleaner, easier to read etc. If you are ever unsure about things like this, just put print statements in your code to see what's happening.
– SuperShoot
Nov 25 '18 at 21:34
2
It is only evaluated once
– juanpa.arrivillaga
Nov 25 '18 at 21:35
3
You could test this yourself by printing a message in
helper()
, and seeing if that message gets printed once, or more than once.– John Gordon
Nov 25 '18 at 21:37
Got it, thanks!
– Aurora
Nov 25 '18 at 21:42
Yeah, adding some so-simple-it’s-a-no-brainer
print
statements is all you need to go fromwondering
Toknowing
– barny
Nov 25 '18 at 21:57