Retrieve records from Active Directory using data structure algorithm in c#
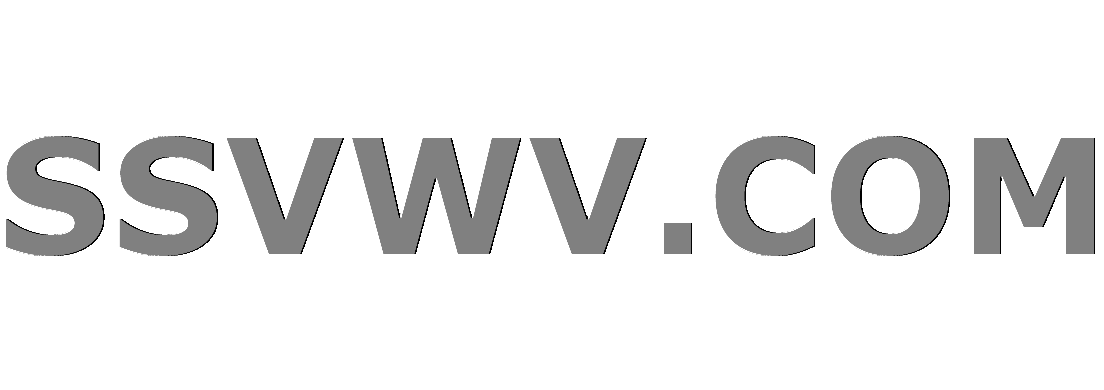
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I need to fetch active directory records and insert in SQL database. There are approximately 10,000 records. I have used this code:
List<ADUser> users = new List<ADUser>();
DirectoryEntry entry = new DirectoryEntry("LDAP://xyz.com");
ADUser userToAdd = null;
IList<string> dict = new List<string>();
DirectorySearcher search = new DirectorySearcher(entry);
search.Filter = "(&(objectClass=user))";
search.PropertiesToLoad.Add("samaccountname");
search.PageSize = 1000;
foreach (SearchResult result in search.FindAll())
{
DirectoryEntry user = result.GetDirectoryEntry();
if (user != null && user.Properties["displayName"].Value!=null)
{
userToAdd = new ADUser
{
FullName = Convert.ToString(user.Properties["displayName"].Value),
LanId = Convert.ToString(user.Properties["sAMAccountName"].Value)
};
users.Add(userToAdd);
}
}
How can I optimize the above code in terms of speed and space complexity? Can I use traversal in binary tree as Active Directory structure looks similar to binary tree.
c# c#-4.0 active-directory binary-tree active-directory-group
add a comment |
I need to fetch active directory records and insert in SQL database. There are approximately 10,000 records. I have used this code:
List<ADUser> users = new List<ADUser>();
DirectoryEntry entry = new DirectoryEntry("LDAP://xyz.com");
ADUser userToAdd = null;
IList<string> dict = new List<string>();
DirectorySearcher search = new DirectorySearcher(entry);
search.Filter = "(&(objectClass=user))";
search.PropertiesToLoad.Add("samaccountname");
search.PageSize = 1000;
foreach (SearchResult result in search.FindAll())
{
DirectoryEntry user = result.GetDirectoryEntry();
if (user != null && user.Properties["displayName"].Value!=null)
{
userToAdd = new ADUser
{
FullName = Convert.ToString(user.Properties["displayName"].Value),
LanId = Convert.ToString(user.Properties["sAMAccountName"].Value)
};
users.Add(userToAdd);
}
}
How can I optimize the above code in terms of speed and space complexity? Can I use traversal in binary tree as Active Directory structure looks similar to binary tree.
c# c#-4.0 active-directory binary-tree active-directory-group
add a comment |
I need to fetch active directory records and insert in SQL database. There are approximately 10,000 records. I have used this code:
List<ADUser> users = new List<ADUser>();
DirectoryEntry entry = new DirectoryEntry("LDAP://xyz.com");
ADUser userToAdd = null;
IList<string> dict = new List<string>();
DirectorySearcher search = new DirectorySearcher(entry);
search.Filter = "(&(objectClass=user))";
search.PropertiesToLoad.Add("samaccountname");
search.PageSize = 1000;
foreach (SearchResult result in search.FindAll())
{
DirectoryEntry user = result.GetDirectoryEntry();
if (user != null && user.Properties["displayName"].Value!=null)
{
userToAdd = new ADUser
{
FullName = Convert.ToString(user.Properties["displayName"].Value),
LanId = Convert.ToString(user.Properties["sAMAccountName"].Value)
};
users.Add(userToAdd);
}
}
How can I optimize the above code in terms of speed and space complexity? Can I use traversal in binary tree as Active Directory structure looks similar to binary tree.
c# c#-4.0 active-directory binary-tree active-directory-group
I need to fetch active directory records and insert in SQL database. There are approximately 10,000 records. I have used this code:
List<ADUser> users = new List<ADUser>();
DirectoryEntry entry = new DirectoryEntry("LDAP://xyz.com");
ADUser userToAdd = null;
IList<string> dict = new List<string>();
DirectorySearcher search = new DirectorySearcher(entry);
search.Filter = "(&(objectClass=user))";
search.PropertiesToLoad.Add("samaccountname");
search.PageSize = 1000;
foreach (SearchResult result in search.FindAll())
{
DirectoryEntry user = result.GetDirectoryEntry();
if (user != null && user.Properties["displayName"].Value!=null)
{
userToAdd = new ADUser
{
FullName = Convert.ToString(user.Properties["displayName"].Value),
LanId = Convert.ToString(user.Properties["sAMAccountName"].Value)
};
users.Add(userToAdd);
}
}
How can I optimize the above code in terms of speed and space complexity? Can I use traversal in binary tree as Active Directory structure looks similar to binary tree.
c# c#-4.0 active-directory binary-tree active-directory-group
c# c#-4.0 active-directory binary-tree active-directory-group
edited Nov 26 '18 at 18:22
marc_s
584k13011241271
584k13011241271
asked Nov 26 '18 at 17:24
piyushpiyush
237
237
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
The list returned by DirectorySearcher.FindAll()
is just a list. So you can't traverse it any better than you already are.
To optimize this, don't use GetDirectoryEntry()
. That is doing two things:
- Making another network request to AD that is unnecessary since the search can return any attributes you want, and
- Taking up memory since all those
DirectoryEntry
objects will stay in memory until you callDispose()
or the GC has time to run (which it won't till your loop finally ends).
First, add displayName
to your PropertiesToLoad
to make sure that gets returned too. Then you can access each property by using result.Properties[propertyName][0]
. Used this way, every property is returned as an array, even if it is a single-value attribute in AD, hence you need the [0]
.
Also, if your app is staying open after this search completes, make sure you call Dispose()
on the SearchResultCollection
that comes out of FindAll()
. In the documentation for FindAll()
, the "Remarks" section indicates you could have a memory leak if you don't. Or you can just put it in a using
block:
DirectorySearcher search = new DirectorySearcher(entry);
search.Filter = "(&(objectClass=user))";
search.PropertiesToLoad.Add("sAMAccountName");
search.PropertiesToLoad.Add("displayName");
search.PageSize = 1000;
using (SearchResultCollection results = search.FindAll()) {
foreach (SearchResult result in results) {
if (result.Properties["displayName"][0] != null) {
userToAdd = new ADUser {
FullName = (string) result.Properties["displayName"][0],
LanId = (string) result.Properties["sAMAccountName"][0]
};
users.Add(userToAdd);
}
}
}
Is there any other way to traverse the active directory ? using recursion or binary any other algorithm?.
– piyush
Nov 27 '18 at 7:28
Of course, but it would be slower than this method you're already using. To optimize speed, you need to keep the number of network requests down. This search you're doing will get 1000 accounts at a time. If you try to traverse AD through the tree, you can only get the contents of 1 OU at a time, so you'll end up doing more network requests than you would with this search.
– Gabriel Luci
Nov 27 '18 at 13:01
Thank you it worked ...now its fetching the 5000 records in 1.3 seconds.
– piyush
Nov 28 '18 at 13:42
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53486150%2fretrieve-records-from-active-directory-using-data-structure-algorithm-in-c-sharp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The list returned by DirectorySearcher.FindAll()
is just a list. So you can't traverse it any better than you already are.
To optimize this, don't use GetDirectoryEntry()
. That is doing two things:
- Making another network request to AD that is unnecessary since the search can return any attributes you want, and
- Taking up memory since all those
DirectoryEntry
objects will stay in memory until you callDispose()
or the GC has time to run (which it won't till your loop finally ends).
First, add displayName
to your PropertiesToLoad
to make sure that gets returned too. Then you can access each property by using result.Properties[propertyName][0]
. Used this way, every property is returned as an array, even if it is a single-value attribute in AD, hence you need the [0]
.
Also, if your app is staying open after this search completes, make sure you call Dispose()
on the SearchResultCollection
that comes out of FindAll()
. In the documentation for FindAll()
, the "Remarks" section indicates you could have a memory leak if you don't. Or you can just put it in a using
block:
DirectorySearcher search = new DirectorySearcher(entry);
search.Filter = "(&(objectClass=user))";
search.PropertiesToLoad.Add("sAMAccountName");
search.PropertiesToLoad.Add("displayName");
search.PageSize = 1000;
using (SearchResultCollection results = search.FindAll()) {
foreach (SearchResult result in results) {
if (result.Properties["displayName"][0] != null) {
userToAdd = new ADUser {
FullName = (string) result.Properties["displayName"][0],
LanId = (string) result.Properties["sAMAccountName"][0]
};
users.Add(userToAdd);
}
}
}
Is there any other way to traverse the active directory ? using recursion or binary any other algorithm?.
– piyush
Nov 27 '18 at 7:28
Of course, but it would be slower than this method you're already using. To optimize speed, you need to keep the number of network requests down. This search you're doing will get 1000 accounts at a time. If you try to traverse AD through the tree, you can only get the contents of 1 OU at a time, so you'll end up doing more network requests than you would with this search.
– Gabriel Luci
Nov 27 '18 at 13:01
Thank you it worked ...now its fetching the 5000 records in 1.3 seconds.
– piyush
Nov 28 '18 at 13:42
add a comment |
The list returned by DirectorySearcher.FindAll()
is just a list. So you can't traverse it any better than you already are.
To optimize this, don't use GetDirectoryEntry()
. That is doing two things:
- Making another network request to AD that is unnecessary since the search can return any attributes you want, and
- Taking up memory since all those
DirectoryEntry
objects will stay in memory until you callDispose()
or the GC has time to run (which it won't till your loop finally ends).
First, add displayName
to your PropertiesToLoad
to make sure that gets returned too. Then you can access each property by using result.Properties[propertyName][0]
. Used this way, every property is returned as an array, even if it is a single-value attribute in AD, hence you need the [0]
.
Also, if your app is staying open after this search completes, make sure you call Dispose()
on the SearchResultCollection
that comes out of FindAll()
. In the documentation for FindAll()
, the "Remarks" section indicates you could have a memory leak if you don't. Or you can just put it in a using
block:
DirectorySearcher search = new DirectorySearcher(entry);
search.Filter = "(&(objectClass=user))";
search.PropertiesToLoad.Add("sAMAccountName");
search.PropertiesToLoad.Add("displayName");
search.PageSize = 1000;
using (SearchResultCollection results = search.FindAll()) {
foreach (SearchResult result in results) {
if (result.Properties["displayName"][0] != null) {
userToAdd = new ADUser {
FullName = (string) result.Properties["displayName"][0],
LanId = (string) result.Properties["sAMAccountName"][0]
};
users.Add(userToAdd);
}
}
}
Is there any other way to traverse the active directory ? using recursion or binary any other algorithm?.
– piyush
Nov 27 '18 at 7:28
Of course, but it would be slower than this method you're already using. To optimize speed, you need to keep the number of network requests down. This search you're doing will get 1000 accounts at a time. If you try to traverse AD through the tree, you can only get the contents of 1 OU at a time, so you'll end up doing more network requests than you would with this search.
– Gabriel Luci
Nov 27 '18 at 13:01
Thank you it worked ...now its fetching the 5000 records in 1.3 seconds.
– piyush
Nov 28 '18 at 13:42
add a comment |
The list returned by DirectorySearcher.FindAll()
is just a list. So you can't traverse it any better than you already are.
To optimize this, don't use GetDirectoryEntry()
. That is doing two things:
- Making another network request to AD that is unnecessary since the search can return any attributes you want, and
- Taking up memory since all those
DirectoryEntry
objects will stay in memory until you callDispose()
or the GC has time to run (which it won't till your loop finally ends).
First, add displayName
to your PropertiesToLoad
to make sure that gets returned too. Then you can access each property by using result.Properties[propertyName][0]
. Used this way, every property is returned as an array, even if it is a single-value attribute in AD, hence you need the [0]
.
Also, if your app is staying open after this search completes, make sure you call Dispose()
on the SearchResultCollection
that comes out of FindAll()
. In the documentation for FindAll()
, the "Remarks" section indicates you could have a memory leak if you don't. Or you can just put it in a using
block:
DirectorySearcher search = new DirectorySearcher(entry);
search.Filter = "(&(objectClass=user))";
search.PropertiesToLoad.Add("sAMAccountName");
search.PropertiesToLoad.Add("displayName");
search.PageSize = 1000;
using (SearchResultCollection results = search.FindAll()) {
foreach (SearchResult result in results) {
if (result.Properties["displayName"][0] != null) {
userToAdd = new ADUser {
FullName = (string) result.Properties["displayName"][0],
LanId = (string) result.Properties["sAMAccountName"][0]
};
users.Add(userToAdd);
}
}
}
The list returned by DirectorySearcher.FindAll()
is just a list. So you can't traverse it any better than you already are.
To optimize this, don't use GetDirectoryEntry()
. That is doing two things:
- Making another network request to AD that is unnecessary since the search can return any attributes you want, and
- Taking up memory since all those
DirectoryEntry
objects will stay in memory until you callDispose()
or the GC has time to run (which it won't till your loop finally ends).
First, add displayName
to your PropertiesToLoad
to make sure that gets returned too. Then you can access each property by using result.Properties[propertyName][0]
. Used this way, every property is returned as an array, even if it is a single-value attribute in AD, hence you need the [0]
.
Also, if your app is staying open after this search completes, make sure you call Dispose()
on the SearchResultCollection
that comes out of FindAll()
. In the documentation for FindAll()
, the "Remarks" section indicates you could have a memory leak if you don't. Or you can just put it in a using
block:
DirectorySearcher search = new DirectorySearcher(entry);
search.Filter = "(&(objectClass=user))";
search.PropertiesToLoad.Add("sAMAccountName");
search.PropertiesToLoad.Add("displayName");
search.PageSize = 1000;
using (SearchResultCollection results = search.FindAll()) {
foreach (SearchResult result in results) {
if (result.Properties["displayName"][0] != null) {
userToAdd = new ADUser {
FullName = (string) result.Properties["displayName"][0],
LanId = (string) result.Properties["sAMAccountName"][0]
};
users.Add(userToAdd);
}
}
}
edited Nov 26 '18 at 18:23
answered Nov 26 '18 at 18:09
Gabriel LuciGabriel Luci
11.5k11525
11.5k11525
Is there any other way to traverse the active directory ? using recursion or binary any other algorithm?.
– piyush
Nov 27 '18 at 7:28
Of course, but it would be slower than this method you're already using. To optimize speed, you need to keep the number of network requests down. This search you're doing will get 1000 accounts at a time. If you try to traverse AD through the tree, you can only get the contents of 1 OU at a time, so you'll end up doing more network requests than you would with this search.
– Gabriel Luci
Nov 27 '18 at 13:01
Thank you it worked ...now its fetching the 5000 records in 1.3 seconds.
– piyush
Nov 28 '18 at 13:42
add a comment |
Is there any other way to traverse the active directory ? using recursion or binary any other algorithm?.
– piyush
Nov 27 '18 at 7:28
Of course, but it would be slower than this method you're already using. To optimize speed, you need to keep the number of network requests down. This search you're doing will get 1000 accounts at a time. If you try to traverse AD through the tree, you can only get the contents of 1 OU at a time, so you'll end up doing more network requests than you would with this search.
– Gabriel Luci
Nov 27 '18 at 13:01
Thank you it worked ...now its fetching the 5000 records in 1.3 seconds.
– piyush
Nov 28 '18 at 13:42
Is there any other way to traverse the active directory ? using recursion or binary any other algorithm?.
– piyush
Nov 27 '18 at 7:28
Is there any other way to traverse the active directory ? using recursion or binary any other algorithm?.
– piyush
Nov 27 '18 at 7:28
Of course, but it would be slower than this method you're already using. To optimize speed, you need to keep the number of network requests down. This search you're doing will get 1000 accounts at a time. If you try to traverse AD through the tree, you can only get the contents of 1 OU at a time, so you'll end up doing more network requests than you would with this search.
– Gabriel Luci
Nov 27 '18 at 13:01
Of course, but it would be slower than this method you're already using. To optimize speed, you need to keep the number of network requests down. This search you're doing will get 1000 accounts at a time. If you try to traverse AD through the tree, you can only get the contents of 1 OU at a time, so you'll end up doing more network requests than you would with this search.
– Gabriel Luci
Nov 27 '18 at 13:01
Thank you it worked ...now its fetching the 5000 records in 1.3 seconds.
– piyush
Nov 28 '18 at 13:42
Thank you it worked ...now its fetching the 5000 records in 1.3 seconds.
– piyush
Nov 28 '18 at 13:42
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53486150%2fretrieve-records-from-active-directory-using-data-structure-algorithm-in-c-sharp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QX8GHmtvDA4XlNv,Qfr,d pwY7ati7vdRx92fGF8A42 JGj,JAGDKA45YOD