Implement transfer learning on niftynet
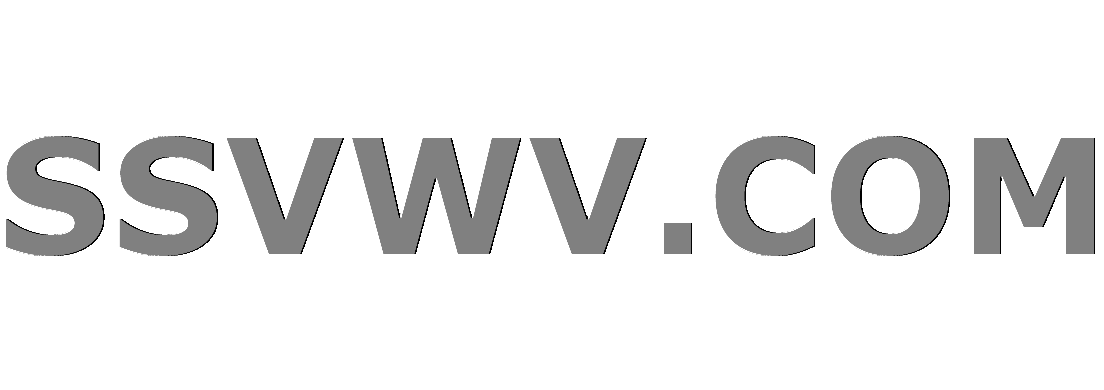
Multi tool use
I want to implement transfer learning using the Dense V-Net architecture. As I was searching on how to do this, I found that this feature is currently being worked on (How do I implement transfer learning in NiftyNet?).
Although from that answer it is quite clear that there is not a straight way to implement it, I was trying to:
1) Create the Dense V-Net
2) Restore weigths from the .ckpt file
3) Implement transfer learning on my own
To perform step 1, I thought I could use the niftynet.network.dense_vnet module. Therefore, I tried the following:
checkpoint = '/path_to_ckpt/model.ckpt-3000.index'
x = tf.placeholder(dtype=tf.float32, shape=[None,1,144,144,144])
architecture_parameters = dict(
use_bdo=False,
use_prior=False,
use_dense_connections=True,
use_coords=False)
hyperparameters = dict(
prior_size=12,
n_dense_channels=(4, 8, 16),
n_seg_channels=(12, 24, 24),
n_input_channels=(24, 24, 24),
dilation_rates=([1] * 5, [1] * 10, [1] * 10),
final_kernel=3,
augmentation_scale=0)
model_instance = DenseVNet(num_classes=9,hyperparameters=hyperparameters,
architecture_parameters=architecture_parameters)
model_net = DenseVNet.layer_op(model_instance, x)
However, I get the following error:
TypeError: Failed to convert object of type <type 'list'> to Tensor. Contents: [None, 1, 72, 72, 24]. Consider casting elements to a supported type.
So, the question is:
Is there any way to implement this?
python tensorflow deep-learning niftynet
add a comment |
I want to implement transfer learning using the Dense V-Net architecture. As I was searching on how to do this, I found that this feature is currently being worked on (How do I implement transfer learning in NiftyNet?).
Although from that answer it is quite clear that there is not a straight way to implement it, I was trying to:
1) Create the Dense V-Net
2) Restore weigths from the .ckpt file
3) Implement transfer learning on my own
To perform step 1, I thought I could use the niftynet.network.dense_vnet module. Therefore, I tried the following:
checkpoint = '/path_to_ckpt/model.ckpt-3000.index'
x = tf.placeholder(dtype=tf.float32, shape=[None,1,144,144,144])
architecture_parameters = dict(
use_bdo=False,
use_prior=False,
use_dense_connections=True,
use_coords=False)
hyperparameters = dict(
prior_size=12,
n_dense_channels=(4, 8, 16),
n_seg_channels=(12, 24, 24),
n_input_channels=(24, 24, 24),
dilation_rates=([1] * 5, [1] * 10, [1] * 10),
final_kernel=3,
augmentation_scale=0)
model_instance = DenseVNet(num_classes=9,hyperparameters=hyperparameters,
architecture_parameters=architecture_parameters)
model_net = DenseVNet.layer_op(model_instance, x)
However, I get the following error:
TypeError: Failed to convert object of type <type 'list'> to Tensor. Contents: [None, 1, 72, 72, 24]. Consider casting elements to a supported type.
So, the question is:
Is there any way to implement this?
python tensorflow deep-learning niftynet
add a comment |
I want to implement transfer learning using the Dense V-Net architecture. As I was searching on how to do this, I found that this feature is currently being worked on (How do I implement transfer learning in NiftyNet?).
Although from that answer it is quite clear that there is not a straight way to implement it, I was trying to:
1) Create the Dense V-Net
2) Restore weigths from the .ckpt file
3) Implement transfer learning on my own
To perform step 1, I thought I could use the niftynet.network.dense_vnet module. Therefore, I tried the following:
checkpoint = '/path_to_ckpt/model.ckpt-3000.index'
x = tf.placeholder(dtype=tf.float32, shape=[None,1,144,144,144])
architecture_parameters = dict(
use_bdo=False,
use_prior=False,
use_dense_connections=True,
use_coords=False)
hyperparameters = dict(
prior_size=12,
n_dense_channels=(4, 8, 16),
n_seg_channels=(12, 24, 24),
n_input_channels=(24, 24, 24),
dilation_rates=([1] * 5, [1] * 10, [1] * 10),
final_kernel=3,
augmentation_scale=0)
model_instance = DenseVNet(num_classes=9,hyperparameters=hyperparameters,
architecture_parameters=architecture_parameters)
model_net = DenseVNet.layer_op(model_instance, x)
However, I get the following error:
TypeError: Failed to convert object of type <type 'list'> to Tensor. Contents: [None, 1, 72, 72, 24]. Consider casting elements to a supported type.
So, the question is:
Is there any way to implement this?
python tensorflow deep-learning niftynet
I want to implement transfer learning using the Dense V-Net architecture. As I was searching on how to do this, I found that this feature is currently being worked on (How do I implement transfer learning in NiftyNet?).
Although from that answer it is quite clear that there is not a straight way to implement it, I was trying to:
1) Create the Dense V-Net
2) Restore weigths from the .ckpt file
3) Implement transfer learning on my own
To perform step 1, I thought I could use the niftynet.network.dense_vnet module. Therefore, I tried the following:
checkpoint = '/path_to_ckpt/model.ckpt-3000.index'
x = tf.placeholder(dtype=tf.float32, shape=[None,1,144,144,144])
architecture_parameters = dict(
use_bdo=False,
use_prior=False,
use_dense_connections=True,
use_coords=False)
hyperparameters = dict(
prior_size=12,
n_dense_channels=(4, 8, 16),
n_seg_channels=(12, 24, 24),
n_input_channels=(24, 24, 24),
dilation_rates=([1] * 5, [1] * 10, [1] * 10),
final_kernel=3,
augmentation_scale=0)
model_instance = DenseVNet(num_classes=9,hyperparameters=hyperparameters,
architecture_parameters=architecture_parameters)
model_net = DenseVNet.layer_op(model_instance, x)
However, I get the following error:
TypeError: Failed to convert object of type <type 'list'> to Tensor. Contents: [None, 1, 72, 72, 24]. Consider casting elements to a supported type.
So, the question is:
Is there any way to implement this?
python tensorflow deep-learning niftynet
python tensorflow deep-learning niftynet
asked Oct 29 '18 at 17:36
Manuel Concepción Brito
64
64
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Transfer learning has been added been added to NiftyNet.
You can select which variables you want to restore through the vars_to_restore
config parameter and which variables to freeze through the vars_to_freeze
config parameter.
See here for more information.
add a comment |
A simple transfer learning can be achieved with restoring weights from existing model in the way that you set the parameter starting_iter
in [TRAINING]
section of your config file to the number of pretrained model. In your example starting_iter=3000
.
This will restore the weights from your model and new iterations will start with this initialisation.
Here the architecture of your model has to be exactly the same, otherwise you will get an error.
For more sophisticated transfer learning or maybe also fine tunning where you can restore only a part of weights, there is a great implementation here. It will be probably merged with official niftynet repository very soon, but you can already use it.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53050961%2fimplement-transfer-learning-on-niftynet%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Transfer learning has been added been added to NiftyNet.
You can select which variables you want to restore through the vars_to_restore
config parameter and which variables to freeze through the vars_to_freeze
config parameter.
See here for more information.
add a comment |
Transfer learning has been added been added to NiftyNet.
You can select which variables you want to restore through the vars_to_restore
config parameter and which variables to freeze through the vars_to_freeze
config parameter.
See here for more information.
add a comment |
Transfer learning has been added been added to NiftyNet.
You can select which variables you want to restore through the vars_to_restore
config parameter and which variables to freeze through the vars_to_freeze
config parameter.
See here for more information.
Transfer learning has been added been added to NiftyNet.
You can select which variables you want to restore through the vars_to_restore
config parameter and which variables to freeze through the vars_to_freeze
config parameter.
See here for more information.
answered Nov 23 '18 at 6:54
Aleksandar Djuric
476
476
add a comment |
add a comment |
A simple transfer learning can be achieved with restoring weights from existing model in the way that you set the parameter starting_iter
in [TRAINING]
section of your config file to the number of pretrained model. In your example starting_iter=3000
.
This will restore the weights from your model and new iterations will start with this initialisation.
Here the architecture of your model has to be exactly the same, otherwise you will get an error.
For more sophisticated transfer learning or maybe also fine tunning where you can restore only a part of weights, there is a great implementation here. It will be probably merged with official niftynet repository very soon, but you can already use it.
add a comment |
A simple transfer learning can be achieved with restoring weights from existing model in the way that you set the parameter starting_iter
in [TRAINING]
section of your config file to the number of pretrained model. In your example starting_iter=3000
.
This will restore the weights from your model and new iterations will start with this initialisation.
Here the architecture of your model has to be exactly the same, otherwise you will get an error.
For more sophisticated transfer learning or maybe also fine tunning where you can restore only a part of weights, there is a great implementation here. It will be probably merged with official niftynet repository very soon, but you can already use it.
add a comment |
A simple transfer learning can be achieved with restoring weights from existing model in the way that you set the parameter starting_iter
in [TRAINING]
section of your config file to the number of pretrained model. In your example starting_iter=3000
.
This will restore the weights from your model and new iterations will start with this initialisation.
Here the architecture of your model has to be exactly the same, otherwise you will get an error.
For more sophisticated transfer learning or maybe also fine tunning where you can restore only a part of weights, there is a great implementation here. It will be probably merged with official niftynet repository very soon, but you can already use it.
A simple transfer learning can be achieved with restoring weights from existing model in the way that you set the parameter starting_iter
in [TRAINING]
section of your config file to the number of pretrained model. In your example starting_iter=3000
.
This will restore the weights from your model and new iterations will start with this initialisation.
Here the architecture of your model has to be exactly the same, otherwise you will get an error.
For more sophisticated transfer learning or maybe also fine tunning where you can restore only a part of weights, there is a great implementation here. It will be probably merged with official niftynet repository very soon, but you can already use it.
answered Nov 21 '18 at 10:32
manza
708
708
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53050961%2fimplement-transfer-learning-on-niftynet%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VE AKrMV6QAXUvss,aedXR5P19znH5LDx F3WkA fXSoNTTObrWM,jd6OG4NqrjFB,7n,VXDVwO2PuY4jwqsvus TbHjEM Jz2F