Parse LocalDateTime depending on input string
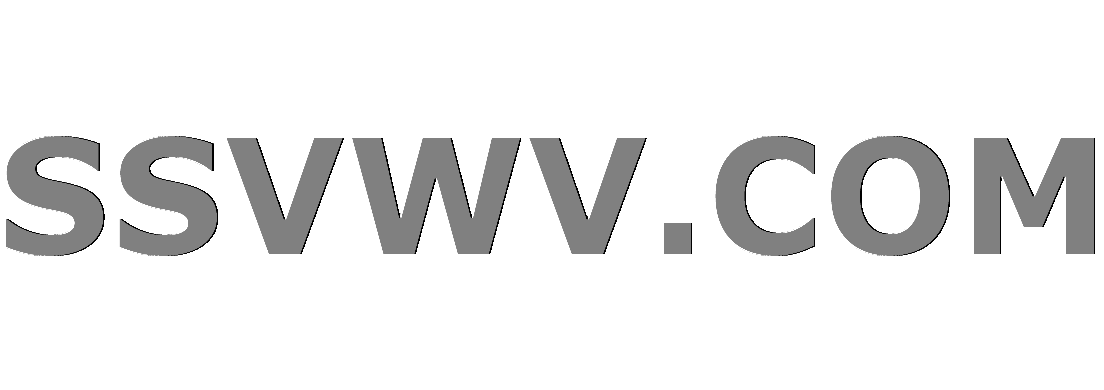
Multi tool use
The client could be able to send either String
in format
"yyyy-MM-dd HH:mm:ss"
or "yyyy-MM-dd"
and depending on it I need to either just parse full LocalDateTime
if he sent me full format or to create LocalDateTime
object with default Time
part "23:59:59"
For now I have written this solution but it seems to be bad as I am using exceptions for controlling business logic.
public class LocalDateTimeConverter implements IStringConverter<LocalDateTime> {
private static final DateTimeFormatter DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
@Override
public LocalDateTime convert(String value) {
LocalDateTime localDateTime;
try {
localDateTime = LocalDateTime.parse(value, DATE_TIME_FORMATTER);
} catch (DateTimeParseException ex) {
localDateTime = LocalDateTime.of(LocalDate.parse(value), LocalTime.of(23, 59, 59));
}
return localDateTime;
}
}
Any suggestions about how to implement it more clearly?
java java-8 java-time
add a comment |
The client could be able to send either String
in format
"yyyy-MM-dd HH:mm:ss"
or "yyyy-MM-dd"
and depending on it I need to either just parse full LocalDateTime
if he sent me full format or to create LocalDateTime
object with default Time
part "23:59:59"
For now I have written this solution but it seems to be bad as I am using exceptions for controlling business logic.
public class LocalDateTimeConverter implements IStringConverter<LocalDateTime> {
private static final DateTimeFormatter DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
@Override
public LocalDateTime convert(String value) {
LocalDateTime localDateTime;
try {
localDateTime = LocalDateTime.parse(value, DATE_TIME_FORMATTER);
} catch (DateTimeParseException ex) {
localDateTime = LocalDateTime.of(LocalDate.parse(value), LocalTime.of(23, 59, 59));
}
return localDateTime;
}
}
Any suggestions about how to implement it more clearly?
java java-8 java-time
Close duplicate of stackoverflow.com/questions/42310409/… but doens't answer the 'default time' part.
– Mark Jeronimus
Nov 21 '18 at 10:38
add a comment |
The client could be able to send either String
in format
"yyyy-MM-dd HH:mm:ss"
or "yyyy-MM-dd"
and depending on it I need to either just parse full LocalDateTime
if he sent me full format or to create LocalDateTime
object with default Time
part "23:59:59"
For now I have written this solution but it seems to be bad as I am using exceptions for controlling business logic.
public class LocalDateTimeConverter implements IStringConverter<LocalDateTime> {
private static final DateTimeFormatter DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
@Override
public LocalDateTime convert(String value) {
LocalDateTime localDateTime;
try {
localDateTime = LocalDateTime.parse(value, DATE_TIME_FORMATTER);
} catch (DateTimeParseException ex) {
localDateTime = LocalDateTime.of(LocalDate.parse(value), LocalTime.of(23, 59, 59));
}
return localDateTime;
}
}
Any suggestions about how to implement it more clearly?
java java-8 java-time
The client could be able to send either String
in format
"yyyy-MM-dd HH:mm:ss"
or "yyyy-MM-dd"
and depending on it I need to either just parse full LocalDateTime
if he sent me full format or to create LocalDateTime
object with default Time
part "23:59:59"
For now I have written this solution but it seems to be bad as I am using exceptions for controlling business logic.
public class LocalDateTimeConverter implements IStringConverter<LocalDateTime> {
private static final DateTimeFormatter DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
@Override
public LocalDateTime convert(String value) {
LocalDateTime localDateTime;
try {
localDateTime = LocalDateTime.parse(value, DATE_TIME_FORMATTER);
} catch (DateTimeParseException ex) {
localDateTime = LocalDateTime.of(LocalDate.parse(value), LocalTime.of(23, 59, 59));
}
return localDateTime;
}
}
Any suggestions about how to implement it more clearly?
java java-8 java-time
java java-8 java-time
asked Nov 21 '18 at 10:30
Oleksandr Riznyk
303111
303111
Close duplicate of stackoverflow.com/questions/42310409/… but doens't answer the 'default time' part.
– Mark Jeronimus
Nov 21 '18 at 10:38
add a comment |
Close duplicate of stackoverflow.com/questions/42310409/… but doens't answer the 'default time' part.
– Mark Jeronimus
Nov 21 '18 at 10:38
Close duplicate of stackoverflow.com/questions/42310409/… but doens't answer the 'default time' part.
– Mark Jeronimus
Nov 21 '18 at 10:38
Close duplicate of stackoverflow.com/questions/42310409/… but doens't answer the 'default time' part.
– Mark Jeronimus
Nov 21 '18 at 10:38
add a comment |
2 Answers
2
active
oldest
votes
I've never worked with parseDefaulting
but a quick shot at it seems to work.
private static final DateTimeFormatter DATE_TIME_FORMATTER = new DateTimeFormatterBuilder()
.appendValue(ChronoField.YEAR_OF_ERA, 4, 4, SignStyle.NEVER)
.appendLiteral('-')
.appendValue(ChronoField.MONTH_OF_YEAR, 2, 2, SignStyle.NEVER)
.appendLiteral('-')
.appendValue(ChronoField.DAY_OF_MONTH, 2, 2, SignStyle.NEVER)
.optionalStart()
.appendLiteral(' ')
.appendValue(ChronoField.HOUR_OF_DAY, 2)
.appendLiteral(':')
.appendValue(ChronoField.MINUTE_OF_HOUR, 2)
.appendLiteral(':')
.appendValue(ChronoField.SECOND_OF_MINUTE, 2)
.optionalEnd()
.parseDefaulting(ChronoField.HOUR_OF_DAY, 23)
.parseDefaulting(ChronoField.MINUTE_OF_HOUR, 59)
.parseDefaulting(ChronoField.SECOND_OF_MINUTE, 59)
.toFormatter();
LocalDateTime.parse("2000-01-01 01:02:03", DATE_TIME_FORMATTER) // 2000-01-01T01:02:03
LocalDateTime.parse("2000-01-01", DATE_TIME_FORMATTER) // 2000-01-01T23:59:59
add a comment |
Check the length of the input string to decide which format must be applied, like this:
public LocalDateTime convert(String value) {
value = value.trim();
boolean isShort = value.length() <= 10;
DateTimeFormatter DATE_TIME_FORMATTER;
if (isShort) {
DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd");
} else {
DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
}
LocalDateTime localDateTime;
try {
localDateTime = LocalDateTime.parse(value, DATE_TIME_FORMATTER);
if (isShort) {
localDateTime = localDateTime.with(LocalTime.of(23, 59, 59));
}
} catch (DateTimeParseException ex) {
localDateTime = null;
}
return localDateTime;
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53410100%2fparse-localdatetime-depending-on-input-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I've never worked with parseDefaulting
but a quick shot at it seems to work.
private static final DateTimeFormatter DATE_TIME_FORMATTER = new DateTimeFormatterBuilder()
.appendValue(ChronoField.YEAR_OF_ERA, 4, 4, SignStyle.NEVER)
.appendLiteral('-')
.appendValue(ChronoField.MONTH_OF_YEAR, 2, 2, SignStyle.NEVER)
.appendLiteral('-')
.appendValue(ChronoField.DAY_OF_MONTH, 2, 2, SignStyle.NEVER)
.optionalStart()
.appendLiteral(' ')
.appendValue(ChronoField.HOUR_OF_DAY, 2)
.appendLiteral(':')
.appendValue(ChronoField.MINUTE_OF_HOUR, 2)
.appendLiteral(':')
.appendValue(ChronoField.SECOND_OF_MINUTE, 2)
.optionalEnd()
.parseDefaulting(ChronoField.HOUR_OF_DAY, 23)
.parseDefaulting(ChronoField.MINUTE_OF_HOUR, 59)
.parseDefaulting(ChronoField.SECOND_OF_MINUTE, 59)
.toFormatter();
LocalDateTime.parse("2000-01-01 01:02:03", DATE_TIME_FORMATTER) // 2000-01-01T01:02:03
LocalDateTime.parse("2000-01-01", DATE_TIME_FORMATTER) // 2000-01-01T23:59:59
add a comment |
I've never worked with parseDefaulting
but a quick shot at it seems to work.
private static final DateTimeFormatter DATE_TIME_FORMATTER = new DateTimeFormatterBuilder()
.appendValue(ChronoField.YEAR_OF_ERA, 4, 4, SignStyle.NEVER)
.appendLiteral('-')
.appendValue(ChronoField.MONTH_OF_YEAR, 2, 2, SignStyle.NEVER)
.appendLiteral('-')
.appendValue(ChronoField.DAY_OF_MONTH, 2, 2, SignStyle.NEVER)
.optionalStart()
.appendLiteral(' ')
.appendValue(ChronoField.HOUR_OF_DAY, 2)
.appendLiteral(':')
.appendValue(ChronoField.MINUTE_OF_HOUR, 2)
.appendLiteral(':')
.appendValue(ChronoField.SECOND_OF_MINUTE, 2)
.optionalEnd()
.parseDefaulting(ChronoField.HOUR_OF_DAY, 23)
.parseDefaulting(ChronoField.MINUTE_OF_HOUR, 59)
.parseDefaulting(ChronoField.SECOND_OF_MINUTE, 59)
.toFormatter();
LocalDateTime.parse("2000-01-01 01:02:03", DATE_TIME_FORMATTER) // 2000-01-01T01:02:03
LocalDateTime.parse("2000-01-01", DATE_TIME_FORMATTER) // 2000-01-01T23:59:59
add a comment |
I've never worked with parseDefaulting
but a quick shot at it seems to work.
private static final DateTimeFormatter DATE_TIME_FORMATTER = new DateTimeFormatterBuilder()
.appendValue(ChronoField.YEAR_OF_ERA, 4, 4, SignStyle.NEVER)
.appendLiteral('-')
.appendValue(ChronoField.MONTH_OF_YEAR, 2, 2, SignStyle.NEVER)
.appendLiteral('-')
.appendValue(ChronoField.DAY_OF_MONTH, 2, 2, SignStyle.NEVER)
.optionalStart()
.appendLiteral(' ')
.appendValue(ChronoField.HOUR_OF_DAY, 2)
.appendLiteral(':')
.appendValue(ChronoField.MINUTE_OF_HOUR, 2)
.appendLiteral(':')
.appendValue(ChronoField.SECOND_OF_MINUTE, 2)
.optionalEnd()
.parseDefaulting(ChronoField.HOUR_OF_DAY, 23)
.parseDefaulting(ChronoField.MINUTE_OF_HOUR, 59)
.parseDefaulting(ChronoField.SECOND_OF_MINUTE, 59)
.toFormatter();
LocalDateTime.parse("2000-01-01 01:02:03", DATE_TIME_FORMATTER) // 2000-01-01T01:02:03
LocalDateTime.parse("2000-01-01", DATE_TIME_FORMATTER) // 2000-01-01T23:59:59
I've never worked with parseDefaulting
but a quick shot at it seems to work.
private static final DateTimeFormatter DATE_TIME_FORMATTER = new DateTimeFormatterBuilder()
.appendValue(ChronoField.YEAR_OF_ERA, 4, 4, SignStyle.NEVER)
.appendLiteral('-')
.appendValue(ChronoField.MONTH_OF_YEAR, 2, 2, SignStyle.NEVER)
.appendLiteral('-')
.appendValue(ChronoField.DAY_OF_MONTH, 2, 2, SignStyle.NEVER)
.optionalStart()
.appendLiteral(' ')
.appendValue(ChronoField.HOUR_OF_DAY, 2)
.appendLiteral(':')
.appendValue(ChronoField.MINUTE_OF_HOUR, 2)
.appendLiteral(':')
.appendValue(ChronoField.SECOND_OF_MINUTE, 2)
.optionalEnd()
.parseDefaulting(ChronoField.HOUR_OF_DAY, 23)
.parseDefaulting(ChronoField.MINUTE_OF_HOUR, 59)
.parseDefaulting(ChronoField.SECOND_OF_MINUTE, 59)
.toFormatter();
LocalDateTime.parse("2000-01-01 01:02:03", DATE_TIME_FORMATTER) // 2000-01-01T01:02:03
LocalDateTime.parse("2000-01-01", DATE_TIME_FORMATTER) // 2000-01-01T23:59:59
edited Nov 21 '18 at 11:14
answered Nov 21 '18 at 10:43
Mark Jeronimus
5,04622036
5,04622036
add a comment |
add a comment |
Check the length of the input string to decide which format must be applied, like this:
public LocalDateTime convert(String value) {
value = value.trim();
boolean isShort = value.length() <= 10;
DateTimeFormatter DATE_TIME_FORMATTER;
if (isShort) {
DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd");
} else {
DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
}
LocalDateTime localDateTime;
try {
localDateTime = LocalDateTime.parse(value, DATE_TIME_FORMATTER);
if (isShort) {
localDateTime = localDateTime.with(LocalTime.of(23, 59, 59));
}
} catch (DateTimeParseException ex) {
localDateTime = null;
}
return localDateTime;
}
add a comment |
Check the length of the input string to decide which format must be applied, like this:
public LocalDateTime convert(String value) {
value = value.trim();
boolean isShort = value.length() <= 10;
DateTimeFormatter DATE_TIME_FORMATTER;
if (isShort) {
DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd");
} else {
DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
}
LocalDateTime localDateTime;
try {
localDateTime = LocalDateTime.parse(value, DATE_TIME_FORMATTER);
if (isShort) {
localDateTime = localDateTime.with(LocalTime.of(23, 59, 59));
}
} catch (DateTimeParseException ex) {
localDateTime = null;
}
return localDateTime;
}
add a comment |
Check the length of the input string to decide which format must be applied, like this:
public LocalDateTime convert(String value) {
value = value.trim();
boolean isShort = value.length() <= 10;
DateTimeFormatter DATE_TIME_FORMATTER;
if (isShort) {
DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd");
} else {
DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
}
LocalDateTime localDateTime;
try {
localDateTime = LocalDateTime.parse(value, DATE_TIME_FORMATTER);
if (isShort) {
localDateTime = localDateTime.with(LocalTime.of(23, 59, 59));
}
} catch (DateTimeParseException ex) {
localDateTime = null;
}
return localDateTime;
}
Check the length of the input string to decide which format must be applied, like this:
public LocalDateTime convert(String value) {
value = value.trim();
boolean isShort = value.length() <= 10;
DateTimeFormatter DATE_TIME_FORMATTER;
if (isShort) {
DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd");
} else {
DATE_TIME_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
}
LocalDateTime localDateTime;
try {
localDateTime = LocalDateTime.parse(value, DATE_TIME_FORMATTER);
if (isShort) {
localDateTime = localDateTime.with(LocalTime.of(23, 59, 59));
}
} catch (DateTimeParseException ex) {
localDateTime = null;
}
return localDateTime;
}
answered Nov 21 '18 at 10:42
forpas
8,8441421
8,8441421
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53410100%2fparse-localdatetime-depending-on-input-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sV B7QAEzjfZRI,iexESvaMpnkWfqW CufcNL9 CelR,UCsML7u6
Close duplicate of stackoverflow.com/questions/42310409/… but doens't answer the 'default time' part.
– Mark Jeronimus
Nov 21 '18 at 10:38