Passing multiple functions to a template
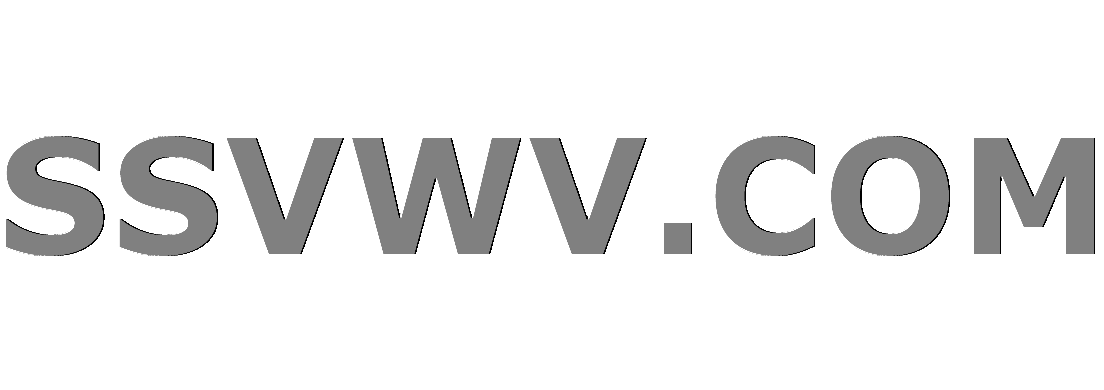
Multi tool use
The following code doesn't compile:
template< typename Fn1, typename Fn2 >
bool templateFunctionOne( Fn1&& fn1, Fn2&& fn2 )
{
int v = 5;
fn1( v );
return fn2( v );
}
template < typename Fn1, typename Fn2 >
bool templateFunctionTwo( Fn1&& fn1, Fn2&& fn2 )
{
std::future< bool > tk( std::async( std::launch::async,
&templateFunctionOne< typename std::remove_reference<Fn1>::type,
typename std::remove_reference<Fn2>::type >,
std::forward<Fn1>(fn1),
std::forward<Fn2>(fn2) ) );
return tk.get();
}
bool printThis( int value )
{
cout << "this value = "
<< value
<< endl;
return true;
}
bool printThat( int value )
{
cout << "that value = "
<< value
<< endl;
return true;
}
int main()
{
auto func1 = std::bind( &printThis, std::placeholders::_1 );
auto func2 = std::bind( &printThat, std::placeholders::_2 );
return templateFunctionTwo( func1, func2 );
}
I'm getting errors like these:
error: no match for call to '(std::_Bind<boo (*(std::_Placeholder<2>))(int)>) (int&)'
return fn2( thatValue );
~~~^~~~~~~~~~~~~
template argument deduction/substitution failed:
candidate: template<class ... _Args, class _Result> _Result std::_Bind<_Functor(_Bound_args ...)>::operator()(_Args&& ...) const [with _Args = {_Args ...}; _Result = _Result; _Functor = bool (*)(int); _Bound_args = {std::_Placeholder<2>}]
operator()(_Args&&... --args) cont
^~~~~~~~
I know it has something to do with passing multiple function pointers to the template, but just don't know where and what. Could anyone help point out where my mistakes are please?
c++ templates
add a comment |
The following code doesn't compile:
template< typename Fn1, typename Fn2 >
bool templateFunctionOne( Fn1&& fn1, Fn2&& fn2 )
{
int v = 5;
fn1( v );
return fn2( v );
}
template < typename Fn1, typename Fn2 >
bool templateFunctionTwo( Fn1&& fn1, Fn2&& fn2 )
{
std::future< bool > tk( std::async( std::launch::async,
&templateFunctionOne< typename std::remove_reference<Fn1>::type,
typename std::remove_reference<Fn2>::type >,
std::forward<Fn1>(fn1),
std::forward<Fn2>(fn2) ) );
return tk.get();
}
bool printThis( int value )
{
cout << "this value = "
<< value
<< endl;
return true;
}
bool printThat( int value )
{
cout << "that value = "
<< value
<< endl;
return true;
}
int main()
{
auto func1 = std::bind( &printThis, std::placeholders::_1 );
auto func2 = std::bind( &printThat, std::placeholders::_2 );
return templateFunctionTwo( func1, func2 );
}
I'm getting errors like these:
error: no match for call to '(std::_Bind<boo (*(std::_Placeholder<2>))(int)>) (int&)'
return fn2( thatValue );
~~~^~~~~~~~~~~~~
template argument deduction/substitution failed:
candidate: template<class ... _Args, class _Result> _Result std::_Bind<_Functor(_Bound_args ...)>::operator()(_Args&& ...) const [with _Args = {_Args ...}; _Result = _Result; _Functor = bool (*)(int); _Bound_args = {std::_Placeholder<2>}]
operator()(_Args&&... --args) cont
^~~~~~~~
I know it has something to do with passing multiple function pointers to the template, but just don't know where and what. Could anyone help point out where my mistakes are please?
c++ templates
3
Your secondbind
call creates a function object expecting two arguments; it ignores the first and passes the second toprintThat
. ButtemplateFunctionOne
calls it with one argument. You likely meantstd::placeholders::_1
in both places. In fact, you can dropbind
calls and just passprintThis
andprintThat
directly.
– Igor Tandetnik
Nov 25 '18 at 17:49
auto func2 = std::bind( &printThat, std::placeholders::_2 );
->auto func2 = std::bind( &printThat, std::placeholders::_1 );
– Matthieu Brucher
Nov 25 '18 at 17:50
Thank you Mathieu, that was it!! Greatly appreciate it!
– mark
Nov 25 '18 at 17:52
All the merit rests with @IgorTandetnik
– Matthieu Brucher
Nov 25 '18 at 17:55
Works best with return 0;
– QuentinUK
Nov 25 '18 at 18:05
add a comment |
The following code doesn't compile:
template< typename Fn1, typename Fn2 >
bool templateFunctionOne( Fn1&& fn1, Fn2&& fn2 )
{
int v = 5;
fn1( v );
return fn2( v );
}
template < typename Fn1, typename Fn2 >
bool templateFunctionTwo( Fn1&& fn1, Fn2&& fn2 )
{
std::future< bool > tk( std::async( std::launch::async,
&templateFunctionOne< typename std::remove_reference<Fn1>::type,
typename std::remove_reference<Fn2>::type >,
std::forward<Fn1>(fn1),
std::forward<Fn2>(fn2) ) );
return tk.get();
}
bool printThis( int value )
{
cout << "this value = "
<< value
<< endl;
return true;
}
bool printThat( int value )
{
cout << "that value = "
<< value
<< endl;
return true;
}
int main()
{
auto func1 = std::bind( &printThis, std::placeholders::_1 );
auto func2 = std::bind( &printThat, std::placeholders::_2 );
return templateFunctionTwo( func1, func2 );
}
I'm getting errors like these:
error: no match for call to '(std::_Bind<boo (*(std::_Placeholder<2>))(int)>) (int&)'
return fn2( thatValue );
~~~^~~~~~~~~~~~~
template argument deduction/substitution failed:
candidate: template<class ... _Args, class _Result> _Result std::_Bind<_Functor(_Bound_args ...)>::operator()(_Args&& ...) const [with _Args = {_Args ...}; _Result = _Result; _Functor = bool (*)(int); _Bound_args = {std::_Placeholder<2>}]
operator()(_Args&&... --args) cont
^~~~~~~~
I know it has something to do with passing multiple function pointers to the template, but just don't know where and what. Could anyone help point out where my mistakes are please?
c++ templates
The following code doesn't compile:
template< typename Fn1, typename Fn2 >
bool templateFunctionOne( Fn1&& fn1, Fn2&& fn2 )
{
int v = 5;
fn1( v );
return fn2( v );
}
template < typename Fn1, typename Fn2 >
bool templateFunctionTwo( Fn1&& fn1, Fn2&& fn2 )
{
std::future< bool > tk( std::async( std::launch::async,
&templateFunctionOne< typename std::remove_reference<Fn1>::type,
typename std::remove_reference<Fn2>::type >,
std::forward<Fn1>(fn1),
std::forward<Fn2>(fn2) ) );
return tk.get();
}
bool printThis( int value )
{
cout << "this value = "
<< value
<< endl;
return true;
}
bool printThat( int value )
{
cout << "that value = "
<< value
<< endl;
return true;
}
int main()
{
auto func1 = std::bind( &printThis, std::placeholders::_1 );
auto func2 = std::bind( &printThat, std::placeholders::_2 );
return templateFunctionTwo( func1, func2 );
}
I'm getting errors like these:
error: no match for call to '(std::_Bind<boo (*(std::_Placeholder<2>))(int)>) (int&)'
return fn2( thatValue );
~~~^~~~~~~~~~~~~
template argument deduction/substitution failed:
candidate: template<class ... _Args, class _Result> _Result std::_Bind<_Functor(_Bound_args ...)>::operator()(_Args&& ...) const [with _Args = {_Args ...}; _Result = _Result; _Functor = bool (*)(int); _Bound_args = {std::_Placeholder<2>}]
operator()(_Args&&... --args) cont
^~~~~~~~
I know it has something to do with passing multiple function pointers to the template, but just don't know where and what. Could anyone help point out where my mistakes are please?
c++ templates
c++ templates
edited Nov 25 '18 at 17:48
Matthieu Brucher
16.6k32243
16.6k32243
asked Nov 25 '18 at 17:45


markmark
325
325
3
Your secondbind
call creates a function object expecting two arguments; it ignores the first and passes the second toprintThat
. ButtemplateFunctionOne
calls it with one argument. You likely meantstd::placeholders::_1
in both places. In fact, you can dropbind
calls and just passprintThis
andprintThat
directly.
– Igor Tandetnik
Nov 25 '18 at 17:49
auto func2 = std::bind( &printThat, std::placeholders::_2 );
->auto func2 = std::bind( &printThat, std::placeholders::_1 );
– Matthieu Brucher
Nov 25 '18 at 17:50
Thank you Mathieu, that was it!! Greatly appreciate it!
– mark
Nov 25 '18 at 17:52
All the merit rests with @IgorTandetnik
– Matthieu Brucher
Nov 25 '18 at 17:55
Works best with return 0;
– QuentinUK
Nov 25 '18 at 18:05
add a comment |
3
Your secondbind
call creates a function object expecting two arguments; it ignores the first and passes the second toprintThat
. ButtemplateFunctionOne
calls it with one argument. You likely meantstd::placeholders::_1
in both places. In fact, you can dropbind
calls and just passprintThis
andprintThat
directly.
– Igor Tandetnik
Nov 25 '18 at 17:49
auto func2 = std::bind( &printThat, std::placeholders::_2 );
->auto func2 = std::bind( &printThat, std::placeholders::_1 );
– Matthieu Brucher
Nov 25 '18 at 17:50
Thank you Mathieu, that was it!! Greatly appreciate it!
– mark
Nov 25 '18 at 17:52
All the merit rests with @IgorTandetnik
– Matthieu Brucher
Nov 25 '18 at 17:55
Works best with return 0;
– QuentinUK
Nov 25 '18 at 18:05
3
3
Your second
bind
call creates a function object expecting two arguments; it ignores the first and passes the second to printThat
. But templateFunctionOne
calls it with one argument. You likely meant std::placeholders::_1
in both places. In fact, you can drop bind
calls and just pass printThis
and printThat
directly.– Igor Tandetnik
Nov 25 '18 at 17:49
Your second
bind
call creates a function object expecting two arguments; it ignores the first and passes the second to printThat
. But templateFunctionOne
calls it with one argument. You likely meant std::placeholders::_1
in both places. In fact, you can drop bind
calls and just pass printThis
and printThat
directly.– Igor Tandetnik
Nov 25 '18 at 17:49
auto func2 = std::bind( &printThat, std::placeholders::_2 );
-> auto func2 = std::bind( &printThat, std::placeholders::_1 );
– Matthieu Brucher
Nov 25 '18 at 17:50
auto func2 = std::bind( &printThat, std::placeholders::_2 );
-> auto func2 = std::bind( &printThat, std::placeholders::_1 );
– Matthieu Brucher
Nov 25 '18 at 17:50
Thank you Mathieu, that was it!! Greatly appreciate it!
– mark
Nov 25 '18 at 17:52
Thank you Mathieu, that was it!! Greatly appreciate it!
– mark
Nov 25 '18 at 17:52
All the merit rests with @IgorTandetnik
– Matthieu Brucher
Nov 25 '18 at 17:55
All the merit rests with @IgorTandetnik
– Matthieu Brucher
Nov 25 '18 at 17:55
Works best with return 0;
– QuentinUK
Nov 25 '18 at 18:05
Works best with return 0;
– QuentinUK
Nov 25 '18 at 18:05
add a comment |
1 Answer
1
active
oldest
votes
Stop using std bind.
return templateFunctionTwo( &printThis, &printThat );
every use of it in the above code makes your code less clear and more error prone.
This is also a mess:
&templateFunctionOne< typename std::remove_reference<Fn1>::type,
typename std::remove_reference<Fn2>::type >,
std::forward<Fn1>(fn1),
std::forward<Fn2>(fn2)
why not
[fn1=std::forward<Fn1>(fn1),fn2=std::forward<Fn2>(fn2)]()mutable{ templateFunctionOne(std::move(fn1), std::move(fn2)); }
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53470187%2fpassing-multiple-functions-to-a-template%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Stop using std bind.
return templateFunctionTwo( &printThis, &printThat );
every use of it in the above code makes your code less clear and more error prone.
This is also a mess:
&templateFunctionOne< typename std::remove_reference<Fn1>::type,
typename std::remove_reference<Fn2>::type >,
std::forward<Fn1>(fn1),
std::forward<Fn2>(fn2)
why not
[fn1=std::forward<Fn1>(fn1),fn2=std::forward<Fn2>(fn2)]()mutable{ templateFunctionOne(std::move(fn1), std::move(fn2)); }
add a comment |
Stop using std bind.
return templateFunctionTwo( &printThis, &printThat );
every use of it in the above code makes your code less clear and more error prone.
This is also a mess:
&templateFunctionOne< typename std::remove_reference<Fn1>::type,
typename std::remove_reference<Fn2>::type >,
std::forward<Fn1>(fn1),
std::forward<Fn2>(fn2)
why not
[fn1=std::forward<Fn1>(fn1),fn2=std::forward<Fn2>(fn2)]()mutable{ templateFunctionOne(std::move(fn1), std::move(fn2)); }
add a comment |
Stop using std bind.
return templateFunctionTwo( &printThis, &printThat );
every use of it in the above code makes your code less clear and more error prone.
This is also a mess:
&templateFunctionOne< typename std::remove_reference<Fn1>::type,
typename std::remove_reference<Fn2>::type >,
std::forward<Fn1>(fn1),
std::forward<Fn2>(fn2)
why not
[fn1=std::forward<Fn1>(fn1),fn2=std::forward<Fn2>(fn2)]()mutable{ templateFunctionOne(std::move(fn1), std::move(fn2)); }
Stop using std bind.
return templateFunctionTwo( &printThis, &printThat );
every use of it in the above code makes your code less clear and more error prone.
This is also a mess:
&templateFunctionOne< typename std::remove_reference<Fn1>::type,
typename std::remove_reference<Fn2>::type >,
std::forward<Fn1>(fn1),
std::forward<Fn2>(fn2)
why not
[fn1=std::forward<Fn1>(fn1),fn2=std::forward<Fn2>(fn2)]()mutable{ templateFunctionOne(std::move(fn1), std::move(fn2)); }
answered Nov 25 '18 at 18:29
Yakk - Adam NevraumontYakk - Adam Nevraumont
187k21199384
187k21199384
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53470187%2fpassing-multiple-functions-to-a-template%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Q,OJpNEJEgm3UoMBmLXW Ca6GISpB7jFYnpXcmBi6,QpZbhiI9LttL
3
Your second
bind
call creates a function object expecting two arguments; it ignores the first and passes the second toprintThat
. ButtemplateFunctionOne
calls it with one argument. You likely meantstd::placeholders::_1
in both places. In fact, you can dropbind
calls and just passprintThis
andprintThat
directly.– Igor Tandetnik
Nov 25 '18 at 17:49
auto func2 = std::bind( &printThat, std::placeholders::_2 );
->auto func2 = std::bind( &printThat, std::placeholders::_1 );
– Matthieu Brucher
Nov 25 '18 at 17:50
Thank you Mathieu, that was it!! Greatly appreciate it!
– mark
Nov 25 '18 at 17:52
All the merit rests with @IgorTandetnik
– Matthieu Brucher
Nov 25 '18 at 17:55
Works best with return 0;
– QuentinUK
Nov 25 '18 at 18:05