Return array index from a lambda but throws IndexOutOfRangeException
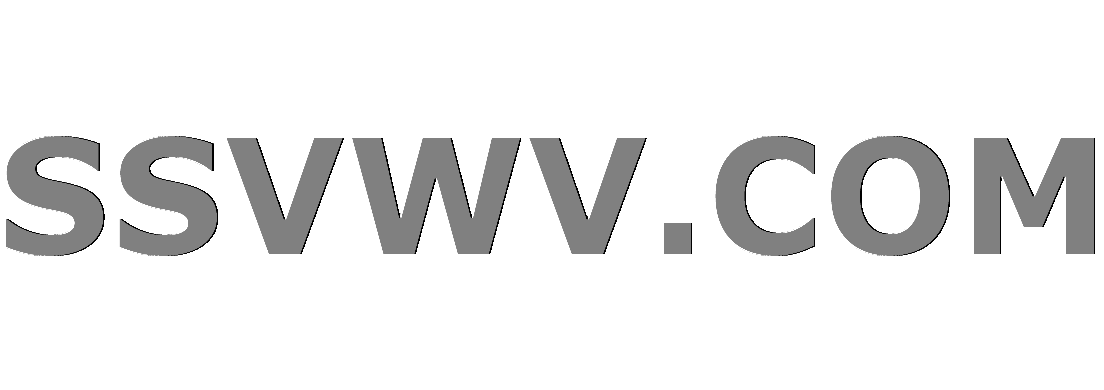
Multi tool use
I'm trying to learn how to use lambdas and in this code I'm trying to get index of some value that is available in the array, but it just return for values 5 and 8 fine and for the other values it keeps throwing IndexOutOfRangeException
!
int nums = { 2, 3, 5, 8, 9 };
int rez = nums.Where(i => nums[i] == 2).FirstOrDefault();
Console.WriteLine(rez);
Please tell me what would happen to "index" return value while trying to retrieving it?
Thanks in advance.
c# linq lambda return indexoutofboundsexception
|
show 1 more comment
I'm trying to learn how to use lambdas and in this code I'm trying to get index of some value that is available in the array, but it just return for values 5 and 8 fine and for the other values it keeps throwing IndexOutOfRangeException
!
int nums = { 2, 3, 5, 8, 9 };
int rez = nums.Where(i => nums[i] == 2).FirstOrDefault();
Console.WriteLine(rez);
Please tell me what would happen to "index" return value while trying to retrieving it?
Thanks in advance.
c# linq lambda return indexoutofboundsexception
Do you understand what the "IndexOutOfRange" execption indicates? It means that thei
used innums[i]
is not a valid index for thenum
array. Ask yourself this: What is the range of valid indexes for yournum
array?
– elgonzo
Nov 25 '18 at 17:48
Yes of course. in this case for 2 should be 0. Is it out?
– imanonami
Nov 25 '18 at 17:49
Look up the documentation forWhere
. It does not do what you think it does... (docs.microsoft.com/en-gb/dotnet/api/…)
– elgonzo
Nov 25 '18 at 17:50
But shouldn't the "i" evaluates to 0?
– imanonami
Nov 25 '18 at 17:51
Again, look up the documentation to know what it does. Do not insist on wild blind guessing...
– elgonzo
Nov 25 '18 at 17:52
|
show 1 more comment
I'm trying to learn how to use lambdas and in this code I'm trying to get index of some value that is available in the array, but it just return for values 5 and 8 fine and for the other values it keeps throwing IndexOutOfRangeException
!
int nums = { 2, 3, 5, 8, 9 };
int rez = nums.Where(i => nums[i] == 2).FirstOrDefault();
Console.WriteLine(rez);
Please tell me what would happen to "index" return value while trying to retrieving it?
Thanks in advance.
c# linq lambda return indexoutofboundsexception
I'm trying to learn how to use lambdas and in this code I'm trying to get index of some value that is available in the array, but it just return for values 5 and 8 fine and for the other values it keeps throwing IndexOutOfRangeException
!
int nums = { 2, 3, 5, 8, 9 };
int rez = nums.Where(i => nums[i] == 2).FirstOrDefault();
Console.WriteLine(rez);
Please tell me what would happen to "index" return value while trying to retrieving it?
Thanks in advance.
c# linq lambda return indexoutofboundsexception
c# linq lambda return indexoutofboundsexception
edited Nov 25 '18 at 18:14


egor.zhdan
3,53053348
3,53053348
asked Nov 25 '18 at 17:39


imanonamiimanonami
132
132
Do you understand what the "IndexOutOfRange" execption indicates? It means that thei
used innums[i]
is not a valid index for thenum
array. Ask yourself this: What is the range of valid indexes for yournum
array?
– elgonzo
Nov 25 '18 at 17:48
Yes of course. in this case for 2 should be 0. Is it out?
– imanonami
Nov 25 '18 at 17:49
Look up the documentation forWhere
. It does not do what you think it does... (docs.microsoft.com/en-gb/dotnet/api/…)
– elgonzo
Nov 25 '18 at 17:50
But shouldn't the "i" evaluates to 0?
– imanonami
Nov 25 '18 at 17:51
Again, look up the documentation to know what it does. Do not insist on wild blind guessing...
– elgonzo
Nov 25 '18 at 17:52
|
show 1 more comment
Do you understand what the "IndexOutOfRange" execption indicates? It means that thei
used innums[i]
is not a valid index for thenum
array. Ask yourself this: What is the range of valid indexes for yournum
array?
– elgonzo
Nov 25 '18 at 17:48
Yes of course. in this case for 2 should be 0. Is it out?
– imanonami
Nov 25 '18 at 17:49
Look up the documentation forWhere
. It does not do what you think it does... (docs.microsoft.com/en-gb/dotnet/api/…)
– elgonzo
Nov 25 '18 at 17:50
But shouldn't the "i" evaluates to 0?
– imanonami
Nov 25 '18 at 17:51
Again, look up the documentation to know what it does. Do not insist on wild blind guessing...
– elgonzo
Nov 25 '18 at 17:52
Do you understand what the "IndexOutOfRange" execption indicates? It means that the
i
used in nums[i]
is not a valid index for the num
array. Ask yourself this: What is the range of valid indexes for your num
array?– elgonzo
Nov 25 '18 at 17:48
Do you understand what the "IndexOutOfRange" execption indicates? It means that the
i
used in nums[i]
is not a valid index for the num
array. Ask yourself this: What is the range of valid indexes for your num
array?– elgonzo
Nov 25 '18 at 17:48
Yes of course. in this case for 2 should be 0. Is it out?
– imanonami
Nov 25 '18 at 17:49
Yes of course. in this case for 2 should be 0. Is it out?
– imanonami
Nov 25 '18 at 17:49
Look up the documentation for
Where
. It does not do what you think it does... (docs.microsoft.com/en-gb/dotnet/api/…)– elgonzo
Nov 25 '18 at 17:50
Look up the documentation for
Where
. It does not do what you think it does... (docs.microsoft.com/en-gb/dotnet/api/…)– elgonzo
Nov 25 '18 at 17:50
But shouldn't the "i" evaluates to 0?
– imanonami
Nov 25 '18 at 17:51
But shouldn't the "i" evaluates to 0?
– imanonami
Nov 25 '18 at 17:51
Again, look up the documentation to know what it does. Do not insist on wild blind guessing...
– elgonzo
Nov 25 '18 at 17:52
Again, look up the documentation to know what it does. Do not insist on wild blind guessing...
– elgonzo
Nov 25 '18 at 17:52
|
show 1 more comment
2 Answers
2
active
oldest
votes
in your lambda expression (i => nums[i] == 2)
, i
would represent the number itself not its index so nums[i]
won't work.
You can simply do this using Array.IndexOf()
:
int rez = Array.IndexOf(nums, 2);
Or if you insist on doing it by Linq (not recommended):
int rez = nums.Select((x, i) => new {x, i}).FirstOrDefault(a => a.x == 2).i;
Great Akhkan, could you explain how your Linq works? I mean how for other indexes it work?
– imanonami
Nov 25 '18 at 18:13
@Ashkan you need to add a condition to .FirstOrDefault(n => n.x == 2). Otherwise, you just get the first element.
– Dmitry Stepanov
Nov 25 '18 at 18:16
in(x,i)
,x
is the item itself andi
is the index, so theSelect would return:
[{x =2, i =0}, {x=3, i= 1}, {x=5, i=2}, ...], so
FirstOrDefault(a => a.x == 2)` would mean the item from our new collection where it'sx
value is2
, and after finding itsi
property would be the index. but it is much slower than indexof, so i reccomand using indexof.
– Ashkan Mobayen Khiabani
Nov 25 '18 at 18:18
@DmitryS Thank you, your suggestion works!
– imanonami
Nov 25 '18 at 18:20
@AshkanMobayenKhiabani Great, I just need to learn for future use of Linq and Lambda
– imanonami
Nov 25 '18 at 18:24
|
show 2 more comments
i
in your lambda is an element (and not the index) of the nums
array.
So, first i
is equal to 2 (the first element of nums
). nums[2] != 2
, so it goes further.
i
is equal to 3 (the second element of nums
). nums[3] != 2
, so it goes further.
Then, i
is equal to 5 (the third element of nums
).nums[5] != 2
but your array has 5 elements and the last element has index 4 (because index is zero based). So, when you tried to access nums[5]
you get an IndexOutOfRangeException
expectedly.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53470138%2freturn-array-index-from-a-lambda-but-throws-indexoutofrangeexception%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
in your lambda expression (i => nums[i] == 2)
, i
would represent the number itself not its index so nums[i]
won't work.
You can simply do this using Array.IndexOf()
:
int rez = Array.IndexOf(nums, 2);
Or if you insist on doing it by Linq (not recommended):
int rez = nums.Select((x, i) => new {x, i}).FirstOrDefault(a => a.x == 2).i;
Great Akhkan, could you explain how your Linq works? I mean how for other indexes it work?
– imanonami
Nov 25 '18 at 18:13
@Ashkan you need to add a condition to .FirstOrDefault(n => n.x == 2). Otherwise, you just get the first element.
– Dmitry Stepanov
Nov 25 '18 at 18:16
in(x,i)
,x
is the item itself andi
is the index, so theSelect would return:
[{x =2, i =0}, {x=3, i= 1}, {x=5, i=2}, ...], so
FirstOrDefault(a => a.x == 2)` would mean the item from our new collection where it'sx
value is2
, and after finding itsi
property would be the index. but it is much slower than indexof, so i reccomand using indexof.
– Ashkan Mobayen Khiabani
Nov 25 '18 at 18:18
@DmitryS Thank you, your suggestion works!
– imanonami
Nov 25 '18 at 18:20
@AshkanMobayenKhiabani Great, I just need to learn for future use of Linq and Lambda
– imanonami
Nov 25 '18 at 18:24
|
show 2 more comments
in your lambda expression (i => nums[i] == 2)
, i
would represent the number itself not its index so nums[i]
won't work.
You can simply do this using Array.IndexOf()
:
int rez = Array.IndexOf(nums, 2);
Or if you insist on doing it by Linq (not recommended):
int rez = nums.Select((x, i) => new {x, i}).FirstOrDefault(a => a.x == 2).i;
Great Akhkan, could you explain how your Linq works? I mean how for other indexes it work?
– imanonami
Nov 25 '18 at 18:13
@Ashkan you need to add a condition to .FirstOrDefault(n => n.x == 2). Otherwise, you just get the first element.
– Dmitry Stepanov
Nov 25 '18 at 18:16
in(x,i)
,x
is the item itself andi
is the index, so theSelect would return:
[{x =2, i =0}, {x=3, i= 1}, {x=5, i=2}, ...], so
FirstOrDefault(a => a.x == 2)` would mean the item from our new collection where it'sx
value is2
, and after finding itsi
property would be the index. but it is much slower than indexof, so i reccomand using indexof.
– Ashkan Mobayen Khiabani
Nov 25 '18 at 18:18
@DmitryS Thank you, your suggestion works!
– imanonami
Nov 25 '18 at 18:20
@AshkanMobayenKhiabani Great, I just need to learn for future use of Linq and Lambda
– imanonami
Nov 25 '18 at 18:24
|
show 2 more comments
in your lambda expression (i => nums[i] == 2)
, i
would represent the number itself not its index so nums[i]
won't work.
You can simply do this using Array.IndexOf()
:
int rez = Array.IndexOf(nums, 2);
Or if you insist on doing it by Linq (not recommended):
int rez = nums.Select((x, i) => new {x, i}).FirstOrDefault(a => a.x == 2).i;
in your lambda expression (i => nums[i] == 2)
, i
would represent the number itself not its index so nums[i]
won't work.
You can simply do this using Array.IndexOf()
:
int rez = Array.IndexOf(nums, 2);
Or if you insist on doing it by Linq (not recommended):
int rez = nums.Select((x, i) => new {x, i}).FirstOrDefault(a => a.x == 2).i;
edited Nov 25 '18 at 18:16
answered Nov 25 '18 at 18:02


Ashkan Mobayen KhiabaniAshkan Mobayen Khiabani
20.9k1667119
20.9k1667119
Great Akhkan, could you explain how your Linq works? I mean how for other indexes it work?
– imanonami
Nov 25 '18 at 18:13
@Ashkan you need to add a condition to .FirstOrDefault(n => n.x == 2). Otherwise, you just get the first element.
– Dmitry Stepanov
Nov 25 '18 at 18:16
in(x,i)
,x
is the item itself andi
is the index, so theSelect would return:
[{x =2, i =0}, {x=3, i= 1}, {x=5, i=2}, ...], so
FirstOrDefault(a => a.x == 2)` would mean the item from our new collection where it'sx
value is2
, and after finding itsi
property would be the index. but it is much slower than indexof, so i reccomand using indexof.
– Ashkan Mobayen Khiabani
Nov 25 '18 at 18:18
@DmitryS Thank you, your suggestion works!
– imanonami
Nov 25 '18 at 18:20
@AshkanMobayenKhiabani Great, I just need to learn for future use of Linq and Lambda
– imanonami
Nov 25 '18 at 18:24
|
show 2 more comments
Great Akhkan, could you explain how your Linq works? I mean how for other indexes it work?
– imanonami
Nov 25 '18 at 18:13
@Ashkan you need to add a condition to .FirstOrDefault(n => n.x == 2). Otherwise, you just get the first element.
– Dmitry Stepanov
Nov 25 '18 at 18:16
in(x,i)
,x
is the item itself andi
is the index, so theSelect would return:
[{x =2, i =0}, {x=3, i= 1}, {x=5, i=2}, ...], so
FirstOrDefault(a => a.x == 2)` would mean the item from our new collection where it'sx
value is2
, and after finding itsi
property would be the index. but it is much slower than indexof, so i reccomand using indexof.
– Ashkan Mobayen Khiabani
Nov 25 '18 at 18:18
@DmitryS Thank you, your suggestion works!
– imanonami
Nov 25 '18 at 18:20
@AshkanMobayenKhiabani Great, I just need to learn for future use of Linq and Lambda
– imanonami
Nov 25 '18 at 18:24
Great Akhkan, could you explain how your Linq works? I mean how for other indexes it work?
– imanonami
Nov 25 '18 at 18:13
Great Akhkan, could you explain how your Linq works? I mean how for other indexes it work?
– imanonami
Nov 25 '18 at 18:13
@Ashkan you need to add a condition to .FirstOrDefault(n => n.x == 2). Otherwise, you just get the first element.
– Dmitry Stepanov
Nov 25 '18 at 18:16
@Ashkan you need to add a condition to .FirstOrDefault(n => n.x == 2). Otherwise, you just get the first element.
– Dmitry Stepanov
Nov 25 '18 at 18:16
in
(x,i)
, x
is the item itself and i
is the index, so the Select would return:
[{x =2, i =0}, {x=3, i= 1}, {x=5, i=2}, ...], so
FirstOrDefault(a => a.x == 2)` would mean the item from our new collection where it's x
value is 2
, and after finding its i
property would be the index. but it is much slower than indexof, so i reccomand using indexof.– Ashkan Mobayen Khiabani
Nov 25 '18 at 18:18
in
(x,i)
, x
is the item itself and i
is the index, so the Select would return:
[{x =2, i =0}, {x=3, i= 1}, {x=5, i=2}, ...], so
FirstOrDefault(a => a.x == 2)` would mean the item from our new collection where it's x
value is 2
, and after finding its i
property would be the index. but it is much slower than indexof, so i reccomand using indexof.– Ashkan Mobayen Khiabani
Nov 25 '18 at 18:18
@DmitryS Thank you, your suggestion works!
– imanonami
Nov 25 '18 at 18:20
@DmitryS Thank you, your suggestion works!
– imanonami
Nov 25 '18 at 18:20
@AshkanMobayenKhiabani Great, I just need to learn for future use of Linq and Lambda
– imanonami
Nov 25 '18 at 18:24
@AshkanMobayenKhiabani Great, I just need to learn for future use of Linq and Lambda
– imanonami
Nov 25 '18 at 18:24
|
show 2 more comments
i
in your lambda is an element (and not the index) of the nums
array.
So, first i
is equal to 2 (the first element of nums
). nums[2] != 2
, so it goes further.
i
is equal to 3 (the second element of nums
). nums[3] != 2
, so it goes further.
Then, i
is equal to 5 (the third element of nums
).nums[5] != 2
but your array has 5 elements and the last element has index 4 (because index is zero based). So, when you tried to access nums[5]
you get an IndexOutOfRangeException
expectedly.
add a comment |
i
in your lambda is an element (and not the index) of the nums
array.
So, first i
is equal to 2 (the first element of nums
). nums[2] != 2
, so it goes further.
i
is equal to 3 (the second element of nums
). nums[3] != 2
, so it goes further.
Then, i
is equal to 5 (the third element of nums
).nums[5] != 2
but your array has 5 elements and the last element has index 4 (because index is zero based). So, when you tried to access nums[5]
you get an IndexOutOfRangeException
expectedly.
add a comment |
i
in your lambda is an element (and not the index) of the nums
array.
So, first i
is equal to 2 (the first element of nums
). nums[2] != 2
, so it goes further.
i
is equal to 3 (the second element of nums
). nums[3] != 2
, so it goes further.
Then, i
is equal to 5 (the third element of nums
).nums[5] != 2
but your array has 5 elements and the last element has index 4 (because index is zero based). So, when you tried to access nums[5]
you get an IndexOutOfRangeException
expectedly.
i
in your lambda is an element (and not the index) of the nums
array.
So, first i
is equal to 2 (the first element of nums
). nums[2] != 2
, so it goes further.
i
is equal to 3 (the second element of nums
). nums[3] != 2
, so it goes further.
Then, i
is equal to 5 (the third element of nums
).nums[5] != 2
but your array has 5 elements and the last element has index 4 (because index is zero based). So, when you tried to access nums[5]
you get an IndexOutOfRangeException
expectedly.
edited Nov 25 '18 at 18:01
answered Nov 25 '18 at 17:51


Dmitry StepanovDmitry Stepanov
1,1491123
1,1491123
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53470138%2freturn-array-index-from-a-lambda-but-throws-indexoutofrangeexception%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Dup0nZ kqrJSMNRF3Q3cA5bd2Om GFxjAZA,4K,7cIoXH 7E4tARjJhY
Do you understand what the "IndexOutOfRange" execption indicates? It means that the
i
used innums[i]
is not a valid index for thenum
array. Ask yourself this: What is the range of valid indexes for yournum
array?– elgonzo
Nov 25 '18 at 17:48
Yes of course. in this case for 2 should be 0. Is it out?
– imanonami
Nov 25 '18 at 17:49
Look up the documentation for
Where
. It does not do what you think it does... (docs.microsoft.com/en-gb/dotnet/api/…)– elgonzo
Nov 25 '18 at 17:50
But shouldn't the "i" evaluates to 0?
– imanonami
Nov 25 '18 at 17:51
Again, look up the documentation to know what it does. Do not insist on wild blind guessing...
– elgonzo
Nov 25 '18 at 17:52