mypy and django models: how to detect errors on nonexistent attributes
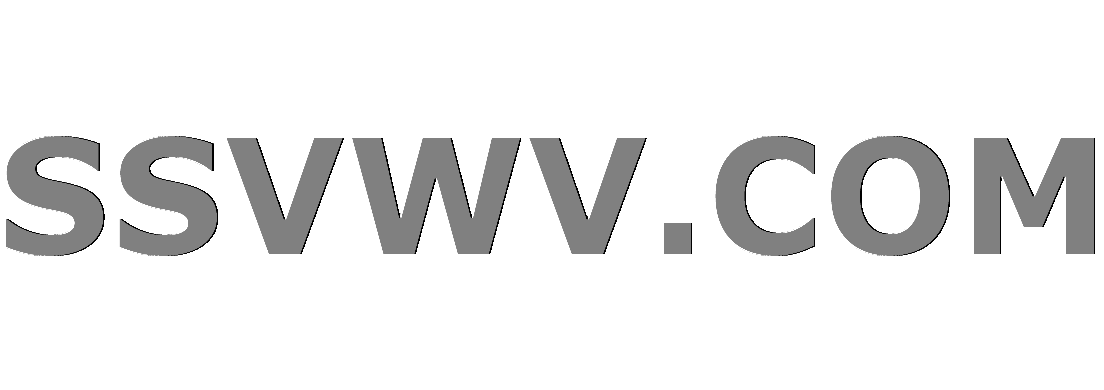
Multi tool use
up vote
2
down vote
favorite
Consider this model definition and usage:
from django.db import models
class User(models.Model):
name: str = models.CharField(max_length=100)
def do_stuff(user: User) -> None:
# accessing existing field
print(user.name.strip())
# accessing existing field with a wrong operation: will fail at runtime
print(user.name + 1)
# acessing nonexistent field: will fail at runtime
print(user.name_abc.strip())
While running mypy
on this, we will get an error for user.name + 1
:
error: Unsupported operand types for + ("str" and "int")
This is fine. But there's another error in the code - user.name_abc
does not exist and will result in AttributeError in runtime.
However, mypy will not see this because it lets the code access any django attributes, also treating them as Any
:
u = User(name='abc')
reveal_type(user.abcdef)
....
> error: Revealed type is 'Any
So, how do I make mypy see such errors?
django mypy
add a comment |
up vote
2
down vote
favorite
Consider this model definition and usage:
from django.db import models
class User(models.Model):
name: str = models.CharField(max_length=100)
def do_stuff(user: User) -> None:
# accessing existing field
print(user.name.strip())
# accessing existing field with a wrong operation: will fail at runtime
print(user.name + 1)
# acessing nonexistent field: will fail at runtime
print(user.name_abc.strip())
While running mypy
on this, we will get an error for user.name + 1
:
error: Unsupported operand types for + ("str" and "int")
This is fine. But there's another error in the code - user.name_abc
does not exist and will result in AttributeError in runtime.
However, mypy will not see this because it lets the code access any django attributes, also treating them as Any
:
u = User(name='abc')
reveal_type(user.abcdef)
....
> error: Revealed type is 'Any
So, how do I make mypy see such errors?
django mypy
It seems that this is related to the Django model class, not yours. If you are not subclassing Django model you get an error: stackoverflow.com/questions/50889677/…
– danielfranca
19 hours ago
@danielfranca exactly. This is related to django models. The question is - how do I work around it.
– kurtgn
18 hours ago
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
Consider this model definition and usage:
from django.db import models
class User(models.Model):
name: str = models.CharField(max_length=100)
def do_stuff(user: User) -> None:
# accessing existing field
print(user.name.strip())
# accessing existing field with a wrong operation: will fail at runtime
print(user.name + 1)
# acessing nonexistent field: will fail at runtime
print(user.name_abc.strip())
While running mypy
on this, we will get an error for user.name + 1
:
error: Unsupported operand types for + ("str" and "int")
This is fine. But there's another error in the code - user.name_abc
does not exist and will result in AttributeError in runtime.
However, mypy will not see this because it lets the code access any django attributes, also treating them as Any
:
u = User(name='abc')
reveal_type(user.abcdef)
....
> error: Revealed type is 'Any
So, how do I make mypy see such errors?
django mypy
Consider this model definition and usage:
from django.db import models
class User(models.Model):
name: str = models.CharField(max_length=100)
def do_stuff(user: User) -> None:
# accessing existing field
print(user.name.strip())
# accessing existing field with a wrong operation: will fail at runtime
print(user.name + 1)
# acessing nonexistent field: will fail at runtime
print(user.name_abc.strip())
While running mypy
on this, we will get an error for user.name + 1
:
error: Unsupported operand types for + ("str" and "int")
This is fine. But there's another error in the code - user.name_abc
does not exist and will result in AttributeError in runtime.
However, mypy will not see this because it lets the code access any django attributes, also treating them as Any
:
u = User(name='abc')
reveal_type(user.abcdef)
....
> error: Revealed type is 'Any
So, how do I make mypy see such errors?
django mypy
django mypy
asked 21 hours ago
kurtgn
1,67721938
1,67721938
It seems that this is related to the Django model class, not yours. If you are not subclassing Django model you get an error: stackoverflow.com/questions/50889677/…
– danielfranca
19 hours ago
@danielfranca exactly. This is related to django models. The question is - how do I work around it.
– kurtgn
18 hours ago
add a comment |
It seems that this is related to the Django model class, not yours. If you are not subclassing Django model you get an error: stackoverflow.com/questions/50889677/…
– danielfranca
19 hours ago
@danielfranca exactly. This is related to django models. The question is - how do I work around it.
– kurtgn
18 hours ago
It seems that this is related to the Django model class, not yours. If you are not subclassing Django model you get an error: stackoverflow.com/questions/50889677/…
– danielfranca
19 hours ago
It seems that this is related to the Django model class, not yours. If you are not subclassing Django model you get an error: stackoverflow.com/questions/50889677/…
– danielfranca
19 hours ago
@danielfranca exactly. This is related to django models. The question is - how do I work around it.
– kurtgn
18 hours ago
@danielfranca exactly. This is related to django models. The question is - how do I work around it.
– kurtgn
18 hours ago
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53370377%2fmypy-and-django-models-how-to-detect-errors-on-nonexistent-attributes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0GFhSZY2Eum57vVYc,XK0HB9h2C,anx2WQ,s3f wz,CzB41gL8n2Z3ByL,aqtuMU6wjM8s0Wi,rd,x,eY Vm JoknVT rxfsQjU4Pd1
It seems that this is related to the Django model class, not yours. If you are not subclassing Django model you get an error: stackoverflow.com/questions/50889677/…
– danielfranca
19 hours ago
@danielfranca exactly. This is related to django models. The question is - how do I work around it.
– kurtgn
18 hours ago