Programmatically Pushing a ViewController in Swift
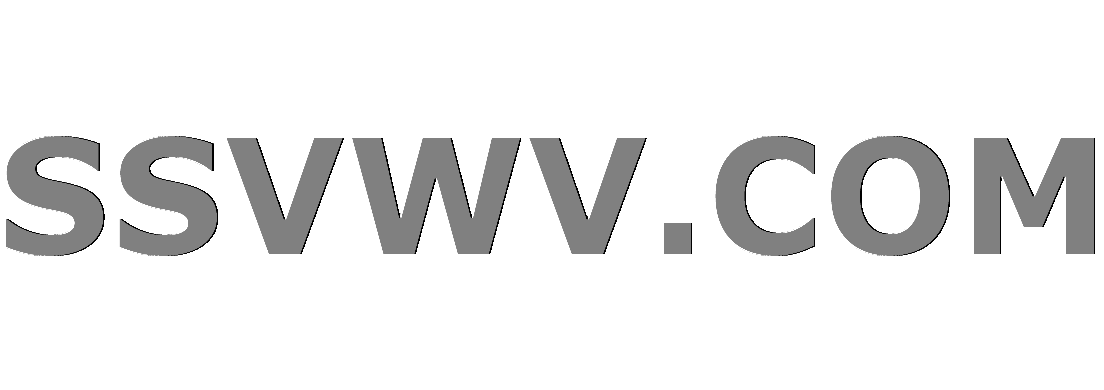
Multi tool use
up vote
1
down vote
favorite
I'm trying to push a ViewController programmatically.
Code:
var plus = UIButton()
plus.addTarget(self, action: #selector(plusPressed), for: .touchUpInside)
@objc func plusPressed() {
print("plus")
let createJournalVC = CreateJournalViewController()
self.navigationController?.pushViewController(createJournalVC, animated: true)
}
What works:
- Once the button is pressed, "plus" is printed to the console.
What doesn't work:
- The ViewController is not pushed.
Details
- I am using a Navigation Controller & Tab Bar Controller.
- I am making this only programmatic, no storyboards.
- There is no error printed to the console, nothing actually happens.
ios swift uiviewcontroller viewcontroller pushviewcontroller
|
show 3 more comments
up vote
1
down vote
favorite
I'm trying to push a ViewController programmatically.
Code:
var plus = UIButton()
plus.addTarget(self, action: #selector(plusPressed), for: .touchUpInside)
@objc func plusPressed() {
print("plus")
let createJournalVC = CreateJournalViewController()
self.navigationController?.pushViewController(createJournalVC, animated: true)
}
What works:
- Once the button is pressed, "plus" is printed to the console.
What doesn't work:
- The ViewController is not pushed.
Details
- I am using a Navigation Controller & Tab Bar Controller.
- I am making this only programmatic, no storyboards.
- There is no error printed to the console, nothing actually happens.
ios swift uiviewcontroller viewcontroller pushviewcontroller
1
see this for help : stackoverflow.com/questions/39929592/…
– Anbu.karthik
22 hours ago
Check if the navigationController exists in theplusPressed
method.
– Rakesha Shastri
22 hours ago
@Anbu.karthik But how come I don't have this issue in any of my other projects?
– Version Swift
22 hours ago
@RakeshaShastri expand please, sorry, I'm new.
– Version Swift
22 hours ago
set a breakpoint on the line where you push the viewController and when the execution stops there, check the value ofnavigationController
– Rakesha Shastri
22 hours ago
|
show 3 more comments
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm trying to push a ViewController programmatically.
Code:
var plus = UIButton()
plus.addTarget(self, action: #selector(plusPressed), for: .touchUpInside)
@objc func plusPressed() {
print("plus")
let createJournalVC = CreateJournalViewController()
self.navigationController?.pushViewController(createJournalVC, animated: true)
}
What works:
- Once the button is pressed, "plus" is printed to the console.
What doesn't work:
- The ViewController is not pushed.
Details
- I am using a Navigation Controller & Tab Bar Controller.
- I am making this only programmatic, no storyboards.
- There is no error printed to the console, nothing actually happens.
ios swift uiviewcontroller viewcontroller pushviewcontroller
I'm trying to push a ViewController programmatically.
Code:
var plus = UIButton()
plus.addTarget(self, action: #selector(plusPressed), for: .touchUpInside)
@objc func plusPressed() {
print("plus")
let createJournalVC = CreateJournalViewController()
self.navigationController?.pushViewController(createJournalVC, animated: true)
}
What works:
- Once the button is pressed, "plus" is printed to the console.
What doesn't work:
- The ViewController is not pushed.
Details
- I am using a Navigation Controller & Tab Bar Controller.
- I am making this only programmatic, no storyboards.
- There is no error printed to the console, nothing actually happens.
ios swift uiviewcontroller viewcontroller pushviewcontroller
ios swift uiviewcontroller viewcontroller pushviewcontroller
asked 22 hours ago


Version Swift
258
258
1
see this for help : stackoverflow.com/questions/39929592/…
– Anbu.karthik
22 hours ago
Check if the navigationController exists in theplusPressed
method.
– Rakesha Shastri
22 hours ago
@Anbu.karthik But how come I don't have this issue in any of my other projects?
– Version Swift
22 hours ago
@RakeshaShastri expand please, sorry, I'm new.
– Version Swift
22 hours ago
set a breakpoint on the line where you push the viewController and when the execution stops there, check the value ofnavigationController
– Rakesha Shastri
22 hours ago
|
show 3 more comments
1
see this for help : stackoverflow.com/questions/39929592/…
– Anbu.karthik
22 hours ago
Check if the navigationController exists in theplusPressed
method.
– Rakesha Shastri
22 hours ago
@Anbu.karthik But how come I don't have this issue in any of my other projects?
– Version Swift
22 hours ago
@RakeshaShastri expand please, sorry, I'm new.
– Version Swift
22 hours ago
set a breakpoint on the line where you push the viewController and when the execution stops there, check the value ofnavigationController
– Rakesha Shastri
22 hours ago
1
1
see this for help : stackoverflow.com/questions/39929592/…
– Anbu.karthik
22 hours ago
see this for help : stackoverflow.com/questions/39929592/…
– Anbu.karthik
22 hours ago
Check if the navigationController exists in the
plusPressed
method.– Rakesha Shastri
22 hours ago
Check if the navigationController exists in the
plusPressed
method.– Rakesha Shastri
22 hours ago
@Anbu.karthik But how come I don't have this issue in any of my other projects?
– Version Swift
22 hours ago
@Anbu.karthik But how come I don't have this issue in any of my other projects?
– Version Swift
22 hours ago
@RakeshaShastri expand please, sorry, I'm new.
– Version Swift
22 hours ago
@RakeshaShastri expand please, sorry, I'm new.
– Version Swift
22 hours ago
set a breakpoint on the line where you push the viewController and when the execution stops there, check the value of
navigationController
– Rakesha Shastri
22 hours ago
set a breakpoint on the line where you push the viewController and when the execution stops there, check the value of
navigationController
– Rakesha Shastri
22 hours ago
|
show 3 more comments
3 Answers
3
active
oldest
votes
up vote
0
down vote
in the navigation stack after the navigation your UItabbar will come then UItabbar will active as Root controller so in here navigationcontroller can become nil. if you faced this scenario then you need to initilize the navigation controller progrmatically or embed with uitabbarcontroller
let createJournalVC = CreateJournalViewController()
if let navigationController = self.navigationController { navigationController.pushViewController(createJournalVC, animated: true)
}else{
let navigationController = UINavigationController.init(rootViewController: createJournalVC)
self.navigationController?.pushViewController(createJournalVC, animated: true)
}
add a comment |
up vote
0
down vote
If TabBarController comes after NavigationController then NavigationController can become nil. You should rather put TabBarController first and then put each ViewController (that are related to each tab) into there own NavigationController.
Storyboard:
Programmatically:
You need to create your TabBarController like this...
let tabCon = UITabBarController()
let navCon1 = UINavigationController(rootViewController: CreateJournalViewController())
let navCon2 = UINavigationController(rootViewController: ViewController())
let navCon3 = UINavigationController(rootViewController: AnotherViewController())
tabCon.viewControllers = [navCon1, navCon2, navCon3]
add a comment |
up vote
0
down vote
It seems navigationController
is nil
, that's why the view controller is not pushed.
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
in the navigation stack after the navigation your UItabbar will come then UItabbar will active as Root controller so in here navigationcontroller can become nil. if you faced this scenario then you need to initilize the navigation controller progrmatically or embed with uitabbarcontroller
let createJournalVC = CreateJournalViewController()
if let navigationController = self.navigationController { navigationController.pushViewController(createJournalVC, animated: true)
}else{
let navigationController = UINavigationController.init(rootViewController: createJournalVC)
self.navigationController?.pushViewController(createJournalVC, animated: true)
}
add a comment |
up vote
0
down vote
in the navigation stack after the navigation your UItabbar will come then UItabbar will active as Root controller so in here navigationcontroller can become nil. if you faced this scenario then you need to initilize the navigation controller progrmatically or embed with uitabbarcontroller
let createJournalVC = CreateJournalViewController()
if let navigationController = self.navigationController { navigationController.pushViewController(createJournalVC, animated: true)
}else{
let navigationController = UINavigationController.init(rootViewController: createJournalVC)
self.navigationController?.pushViewController(createJournalVC, animated: true)
}
add a comment |
up vote
0
down vote
up vote
0
down vote
in the navigation stack after the navigation your UItabbar will come then UItabbar will active as Root controller so in here navigationcontroller can become nil. if you faced this scenario then you need to initilize the navigation controller progrmatically or embed with uitabbarcontroller
let createJournalVC = CreateJournalViewController()
if let navigationController = self.navigationController { navigationController.pushViewController(createJournalVC, animated: true)
}else{
let navigationController = UINavigationController.init(rootViewController: createJournalVC)
self.navigationController?.pushViewController(createJournalVC, animated: true)
}
in the navigation stack after the navigation your UItabbar will come then UItabbar will active as Root controller so in here navigationcontroller can become nil. if you faced this scenario then you need to initilize the navigation controller progrmatically or embed with uitabbarcontroller
let createJournalVC = CreateJournalViewController()
if let navigationController = self.navigationController { navigationController.pushViewController(createJournalVC, animated: true)
}else{
let navigationController = UINavigationController.init(rootViewController: createJournalVC)
self.navigationController?.pushViewController(createJournalVC, animated: true)
}
edited 22 hours ago
answered 22 hours ago


Anbu.karthik
59.1k15121111
59.1k15121111
add a comment |
add a comment |
up vote
0
down vote
If TabBarController comes after NavigationController then NavigationController can become nil. You should rather put TabBarController first and then put each ViewController (that are related to each tab) into there own NavigationController.
Storyboard:
Programmatically:
You need to create your TabBarController like this...
let tabCon = UITabBarController()
let navCon1 = UINavigationController(rootViewController: CreateJournalViewController())
let navCon2 = UINavigationController(rootViewController: ViewController())
let navCon3 = UINavigationController(rootViewController: AnotherViewController())
tabCon.viewControllers = [navCon1, navCon2, navCon3]
add a comment |
up vote
0
down vote
If TabBarController comes after NavigationController then NavigationController can become nil. You should rather put TabBarController first and then put each ViewController (that are related to each tab) into there own NavigationController.
Storyboard:
Programmatically:
You need to create your TabBarController like this...
let tabCon = UITabBarController()
let navCon1 = UINavigationController(rootViewController: CreateJournalViewController())
let navCon2 = UINavigationController(rootViewController: ViewController())
let navCon3 = UINavigationController(rootViewController: AnotherViewController())
tabCon.viewControllers = [navCon1, navCon2, navCon3]
add a comment |
up vote
0
down vote
up vote
0
down vote
If TabBarController comes after NavigationController then NavigationController can become nil. You should rather put TabBarController first and then put each ViewController (that are related to each tab) into there own NavigationController.
Storyboard:
Programmatically:
You need to create your TabBarController like this...
let tabCon = UITabBarController()
let navCon1 = UINavigationController(rootViewController: CreateJournalViewController())
let navCon2 = UINavigationController(rootViewController: ViewController())
let navCon3 = UINavigationController(rootViewController: AnotherViewController())
tabCon.viewControllers = [navCon1, navCon2, navCon3]
If TabBarController comes after NavigationController then NavigationController can become nil. You should rather put TabBarController first and then put each ViewController (that are related to each tab) into there own NavigationController.
Storyboard:
Programmatically:
You need to create your TabBarController like this...
let tabCon = UITabBarController()
let navCon1 = UINavigationController(rootViewController: CreateJournalViewController())
let navCon2 = UINavigationController(rootViewController: ViewController())
let navCon3 = UINavigationController(rootViewController: AnotherViewController())
tabCon.viewControllers = [navCon1, navCon2, navCon3]
edited 21 hours ago
answered 22 hours ago
N4SK
126113
126113
add a comment |
add a comment |
up vote
0
down vote
It seems navigationController
is nil
, that's why the view controller is not pushed.
add a comment |
up vote
0
down vote
It seems navigationController
is nil
, that's why the view controller is not pushed.
add a comment |
up vote
0
down vote
up vote
0
down vote
It seems navigationController
is nil
, that's why the view controller is not pushed.
It seems navigationController
is nil
, that's why the view controller is not pushed.
answered 19 hours ago


Vladimir Kaltyrin
335112
335112
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53371608%2fprogrammatically-pushing-a-viewcontroller-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ia4cSn1nsErA51HYPZ XCsWW4
1
see this for help : stackoverflow.com/questions/39929592/…
– Anbu.karthik
22 hours ago
Check if the navigationController exists in the
plusPressed
method.– Rakesha Shastri
22 hours ago
@Anbu.karthik But how come I don't have this issue in any of my other projects?
– Version Swift
22 hours ago
@RakeshaShastri expand please, sorry, I'm new.
– Version Swift
22 hours ago
set a breakpoint on the line where you push the viewController and when the execution stops there, check the value of
navigationController
– Rakesha Shastri
22 hours ago