Compare two lists of different types using HashMap
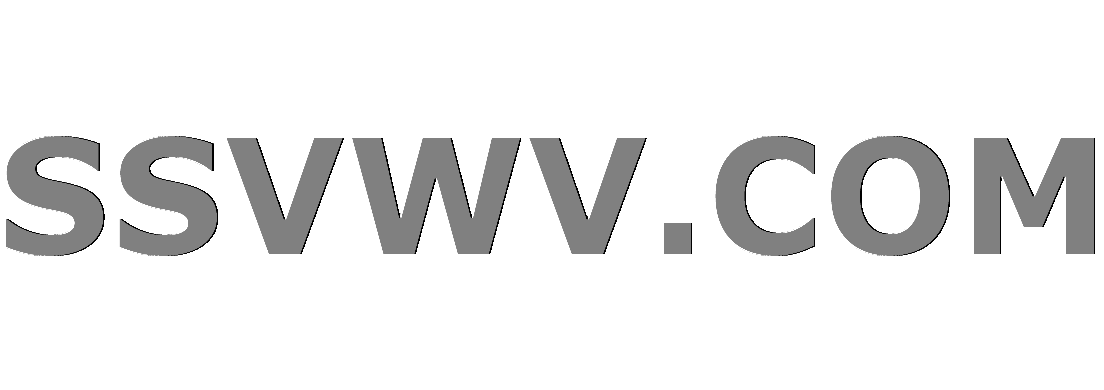
Multi tool use
up vote
2
down vote
favorite
I have two classes:
public class AClass{
String name;
int id;
int total;
}
public class BClass{
String batchName;
int id;
}
Now I have two lists:
List<AClass> aLst;
List<BClass> bLst;
Between these two list i need to check if AClass.id==BClass.id
.
One way to achieve this is to have two for loops and compare. But this is not the efficient way.
Other way is by using HashMap
. Traverse the BClass
list and use the BClass.id
as the key and the respective object as the value:
Map<int,BClass> map = new HashMap<int,BClass>();
List requiredLst <BClass> = new ArrayList<BClass>();
foreach(BClass b : bLst){
map.put(BClass.id, b);
}
foreach(AClass a : aLst){
BClass b = map.get(a.id);
requiredLst.add(b);
}
Is this efficient way to compare?
java arraylist collections hashmap processing-efficiency
add a comment |
up vote
2
down vote
favorite
I have two classes:
public class AClass{
String name;
int id;
int total;
}
public class BClass{
String batchName;
int id;
}
Now I have two lists:
List<AClass> aLst;
List<BClass> bLst;
Between these two list i need to check if AClass.id==BClass.id
.
One way to achieve this is to have two for loops and compare. But this is not the efficient way.
Other way is by using HashMap
. Traverse the BClass
list and use the BClass.id
as the key and the respective object as the value:
Map<int,BClass> map = new HashMap<int,BClass>();
List requiredLst <BClass> = new ArrayList<BClass>();
foreach(BClass b : bLst){
map.put(BClass.id, b);
}
foreach(AClass a : aLst){
BClass b = map.get(a.id);
requiredLst.add(b);
}
Is this efficient way to compare?
java arraylist collections hashmap processing-efficiency
You haven't sufficiently described the actual comparison procedure. What does the procedure return, a boolean? What if the 5th element in the first list has the same id as the 13th element in the second list?
– amn
Nov 19 at 19:34
Are you looking just for missing ids or for entire objects?
– Karol Dowbecki
Nov 19 at 19:52
I am looking for all the objects of BClass in bLst having the same id's as that of the ids in the AClass objects ..
– James Patty
Nov 19 at 20:36
We can safely assume that IDs are unquie...
– James Patty
Nov 19 at 20:37
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I have two classes:
public class AClass{
String name;
int id;
int total;
}
public class BClass{
String batchName;
int id;
}
Now I have two lists:
List<AClass> aLst;
List<BClass> bLst;
Between these two list i need to check if AClass.id==BClass.id
.
One way to achieve this is to have two for loops and compare. But this is not the efficient way.
Other way is by using HashMap
. Traverse the BClass
list and use the BClass.id
as the key and the respective object as the value:
Map<int,BClass> map = new HashMap<int,BClass>();
List requiredLst <BClass> = new ArrayList<BClass>();
foreach(BClass b : bLst){
map.put(BClass.id, b);
}
foreach(AClass a : aLst){
BClass b = map.get(a.id);
requiredLst.add(b);
}
Is this efficient way to compare?
java arraylist collections hashmap processing-efficiency
I have two classes:
public class AClass{
String name;
int id;
int total;
}
public class BClass{
String batchName;
int id;
}
Now I have two lists:
List<AClass> aLst;
List<BClass> bLst;
Between these two list i need to check if AClass.id==BClass.id
.
One way to achieve this is to have two for loops and compare. But this is not the efficient way.
Other way is by using HashMap
. Traverse the BClass
list and use the BClass.id
as the key and the respective object as the value:
Map<int,BClass> map = new HashMap<int,BClass>();
List requiredLst <BClass> = new ArrayList<BClass>();
foreach(BClass b : bLst){
map.put(BClass.id, b);
}
foreach(AClass a : aLst){
BClass b = map.get(a.id);
requiredLst.add(b);
}
Is this efficient way to compare?
java arraylist collections hashmap processing-efficiency
java arraylist collections hashmap processing-efficiency
edited Nov 19 at 21:13


Karol Dowbecki
14k72745
14k72745
asked Nov 19 at 19:30
James Patty
111
111
You haven't sufficiently described the actual comparison procedure. What does the procedure return, a boolean? What if the 5th element in the first list has the same id as the 13th element in the second list?
– amn
Nov 19 at 19:34
Are you looking just for missing ids or for entire objects?
– Karol Dowbecki
Nov 19 at 19:52
I am looking for all the objects of BClass in bLst having the same id's as that of the ids in the AClass objects ..
– James Patty
Nov 19 at 20:36
We can safely assume that IDs are unquie...
– James Patty
Nov 19 at 20:37
add a comment |
You haven't sufficiently described the actual comparison procedure. What does the procedure return, a boolean? What if the 5th element in the first list has the same id as the 13th element in the second list?
– amn
Nov 19 at 19:34
Are you looking just for missing ids or for entire objects?
– Karol Dowbecki
Nov 19 at 19:52
I am looking for all the objects of BClass in bLst having the same id's as that of the ids in the AClass objects ..
– James Patty
Nov 19 at 20:36
We can safely assume that IDs are unquie...
– James Patty
Nov 19 at 20:37
You haven't sufficiently described the actual comparison procedure. What does the procedure return, a boolean? What if the 5th element in the first list has the same id as the 13th element in the second list?
– amn
Nov 19 at 19:34
You haven't sufficiently described the actual comparison procedure. What does the procedure return, a boolean? What if the 5th element in the first list has the same id as the 13th element in the second list?
– amn
Nov 19 at 19:34
Are you looking just for missing ids or for entire objects?
– Karol Dowbecki
Nov 19 at 19:52
Are you looking just for missing ids or for entire objects?
– Karol Dowbecki
Nov 19 at 19:52
I am looking for all the objects of BClass in bLst having the same id's as that of the ids in the AClass objects ..
– James Patty
Nov 19 at 20:36
I am looking for all the objects of BClass in bLst having the same id's as that of the ids in the AClass objects ..
– James Patty
Nov 19 at 20:36
We can safely assume that IDs are unquie...
– James Patty
Nov 19 at 20:37
We can safely assume that IDs are unquie...
– James Patty
Nov 19 at 20:37
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
It would be easier with a Set<Integer>
to store the identifiers from AClass
. Since Set.contains()
is O(1) the total complexity will be O(n).
List<AClass> aLst = ...;
List<BClass> bLst = ...;
Set<Integer> ids = aLst.stream()
.map(AClass::getId)
.collect(Collectors.toSet());
List<BClass> required = bLst.stream()
.filter(b -> ids.contains(b.getId()))
.collect(Collectors.toList());
However for small lists the cost of creating additional Set
and boxing identifiers into Integer
might outweigh the benefit of reduced number of iterations.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
It would be easier with a Set<Integer>
to store the identifiers from AClass
. Since Set.contains()
is O(1) the total complexity will be O(n).
List<AClass> aLst = ...;
List<BClass> bLst = ...;
Set<Integer> ids = aLst.stream()
.map(AClass::getId)
.collect(Collectors.toSet());
List<BClass> required = bLst.stream()
.filter(b -> ids.contains(b.getId()))
.collect(Collectors.toList());
However for small lists the cost of creating additional Set
and boxing identifiers into Integer
might outweigh the benefit of reduced number of iterations.
add a comment |
up vote
2
down vote
It would be easier with a Set<Integer>
to store the identifiers from AClass
. Since Set.contains()
is O(1) the total complexity will be O(n).
List<AClass> aLst = ...;
List<BClass> bLst = ...;
Set<Integer> ids = aLst.stream()
.map(AClass::getId)
.collect(Collectors.toSet());
List<BClass> required = bLst.stream()
.filter(b -> ids.contains(b.getId()))
.collect(Collectors.toList());
However for small lists the cost of creating additional Set
and boxing identifiers into Integer
might outweigh the benefit of reduced number of iterations.
add a comment |
up vote
2
down vote
up vote
2
down vote
It would be easier with a Set<Integer>
to store the identifiers from AClass
. Since Set.contains()
is O(1) the total complexity will be O(n).
List<AClass> aLst = ...;
List<BClass> bLst = ...;
Set<Integer> ids = aLst.stream()
.map(AClass::getId)
.collect(Collectors.toSet());
List<BClass> required = bLst.stream()
.filter(b -> ids.contains(b.getId()))
.collect(Collectors.toList());
However for small lists the cost of creating additional Set
and boxing identifiers into Integer
might outweigh the benefit of reduced number of iterations.
It would be easier with a Set<Integer>
to store the identifiers from AClass
. Since Set.contains()
is O(1) the total complexity will be O(n).
List<AClass> aLst = ...;
List<BClass> bLst = ...;
Set<Integer> ids = aLst.stream()
.map(AClass::getId)
.collect(Collectors.toSet());
List<BClass> required = bLst.stream()
.filter(b -> ids.contains(b.getId()))
.collect(Collectors.toList());
However for small lists the cost of creating additional Set
and boxing identifiers into Integer
might outweigh the benefit of reduced number of iterations.
answered Nov 19 at 20:06


Karol Dowbecki
14k72745
14k72745
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53381403%2fcompare-two-lists-of-different-types-using-hashmap%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
RWJ,bPQPJ9,JbgxndS DWh
You haven't sufficiently described the actual comparison procedure. What does the procedure return, a boolean? What if the 5th element in the first list has the same id as the 13th element in the second list?
– amn
Nov 19 at 19:34
Are you looking just for missing ids or for entire objects?
– Karol Dowbecki
Nov 19 at 19:52
I am looking for all the objects of BClass in bLst having the same id's as that of the ids in the AClass objects ..
– James Patty
Nov 19 at 20:36
We can safely assume that IDs are unquie...
– James Patty
Nov 19 at 20:37