How to ping a URL in an Android Service?
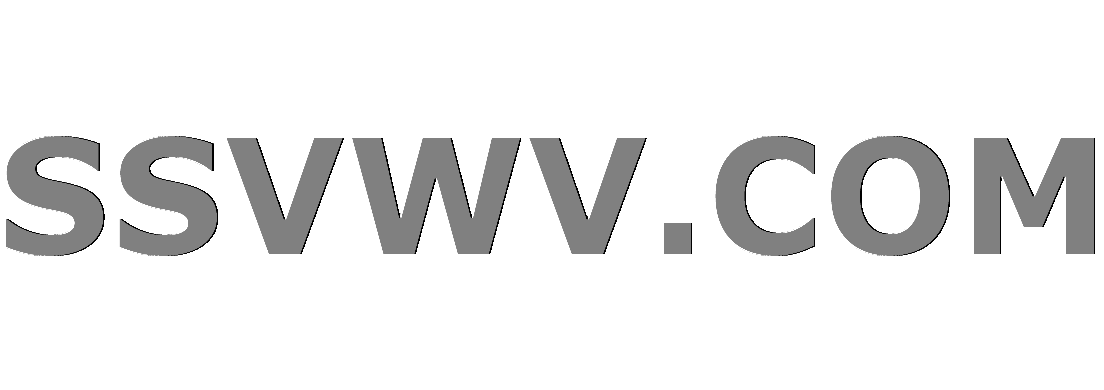
Multi tool use
up vote
9
down vote
favorite
How can I use Android Service to do a ping callback? I need to open a webpage on a button click, but in the background, go ping another URL for stats collection.

add a comment |
up vote
9
down vote
favorite
How can I use Android Service to do a ping callback? I need to open a webpage on a button click, but in the background, go ping another URL for stats collection.

Kotlin implementation supporting API 25+ can be found here: stackoverflow.com/questions/53402128/…
– quietbits
Nov 20 at 23:33
add a comment |
up vote
9
down vote
favorite
up vote
9
down vote
favorite
How can I use Android Service to do a ping callback? I need to open a webpage on a button click, but in the background, go ping another URL for stats collection.

How can I use Android Service to do a ping callback? I need to open a webpage on a button click, but in the background, go ping another URL for stats collection.


edited Nov 20 at 22:04


halfer
14.2k757106
14.2k757106
asked May 7 '10 at 6:47
Chris
2,334113547
2,334113547
Kotlin implementation supporting API 25+ can be found here: stackoverflow.com/questions/53402128/…
– quietbits
Nov 20 at 23:33
add a comment |
Kotlin implementation supporting API 25+ can be found here: stackoverflow.com/questions/53402128/…
– quietbits
Nov 20 at 23:33
Kotlin implementation supporting API 25+ can be found here: stackoverflow.com/questions/53402128/…
– quietbits
Nov 20 at 23:33
Kotlin implementation supporting API 25+ can be found here: stackoverflow.com/questions/53402128/…
– quietbits
Nov 20 at 23:33
add a comment |
1 Answer
1
active
oldest
votes
up vote
18
down vote
accepted
I think if you just want to ping an url, you can use this code :
try {
URL url = new URL("http://"+params[0]);
HttpURLConnection urlc = (HttpURLConnection) url.openConnection();
urlc.setRequestProperty("User-Agent", "Android Application:"+Z.APP_VERSION);
urlc.setRequestProperty("Connection", "close");
urlc.setConnectTimeout(1000 * 30); // mTimeout is in seconds
urlc.connect();
if (urlc.getResponseCode() == 200) {
Main.Log("getResponseCode == 200");
return new Boolean(true);
}
} catch (MalformedURLException e1) {
e1.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
And, if you want to have the equivalent of a ping, you can put a System.currentTimeMillis() before and after the call, and calculate the difference.
hopes that helps
code snippet found in here
This has been working fine for me except for Android 4 devices where it always fails. Any ideas?
– dee
Feb 10 '12 at 16:29
10
You need to move the call off your main thread. Android 4 enforces no network calls on the main thread to prevent ANR issues.
– Nick Campion
Feb 13 '12 at 22:47
2
This will establish a TCP connection with a full 3-way handshake. So the time is nothing like a ping (ICMP-echo -> ICMP-reply
)
– Fredrik Erlandsson
Mar 4 '15 at 19:51
@NickCampion: for me this code is not working on 4.2.2 device which includes samsung s3 and other , any solution
– Punit Sharma
Feb 10 '16 at 10:32
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
18
down vote
accepted
I think if you just want to ping an url, you can use this code :
try {
URL url = new URL("http://"+params[0]);
HttpURLConnection urlc = (HttpURLConnection) url.openConnection();
urlc.setRequestProperty("User-Agent", "Android Application:"+Z.APP_VERSION);
urlc.setRequestProperty("Connection", "close");
urlc.setConnectTimeout(1000 * 30); // mTimeout is in seconds
urlc.connect();
if (urlc.getResponseCode() == 200) {
Main.Log("getResponseCode == 200");
return new Boolean(true);
}
} catch (MalformedURLException e1) {
e1.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
And, if you want to have the equivalent of a ping, you can put a System.currentTimeMillis() before and after the call, and calculate the difference.
hopes that helps
code snippet found in here
This has been working fine for me except for Android 4 devices where it always fails. Any ideas?
– dee
Feb 10 '12 at 16:29
10
You need to move the call off your main thread. Android 4 enforces no network calls on the main thread to prevent ANR issues.
– Nick Campion
Feb 13 '12 at 22:47
2
This will establish a TCP connection with a full 3-way handshake. So the time is nothing like a ping (ICMP-echo -> ICMP-reply
)
– Fredrik Erlandsson
Mar 4 '15 at 19:51
@NickCampion: for me this code is not working on 4.2.2 device which includes samsung s3 and other , any solution
– Punit Sharma
Feb 10 '16 at 10:32
add a comment |
up vote
18
down vote
accepted
I think if you just want to ping an url, you can use this code :
try {
URL url = new URL("http://"+params[0]);
HttpURLConnection urlc = (HttpURLConnection) url.openConnection();
urlc.setRequestProperty("User-Agent", "Android Application:"+Z.APP_VERSION);
urlc.setRequestProperty("Connection", "close");
urlc.setConnectTimeout(1000 * 30); // mTimeout is in seconds
urlc.connect();
if (urlc.getResponseCode() == 200) {
Main.Log("getResponseCode == 200");
return new Boolean(true);
}
} catch (MalformedURLException e1) {
e1.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
And, if you want to have the equivalent of a ping, you can put a System.currentTimeMillis() before and after the call, and calculate the difference.
hopes that helps
code snippet found in here
This has been working fine for me except for Android 4 devices where it always fails. Any ideas?
– dee
Feb 10 '12 at 16:29
10
You need to move the call off your main thread. Android 4 enforces no network calls on the main thread to prevent ANR issues.
– Nick Campion
Feb 13 '12 at 22:47
2
This will establish a TCP connection with a full 3-way handshake. So the time is nothing like a ping (ICMP-echo -> ICMP-reply
)
– Fredrik Erlandsson
Mar 4 '15 at 19:51
@NickCampion: for me this code is not working on 4.2.2 device which includes samsung s3 and other , any solution
– Punit Sharma
Feb 10 '16 at 10:32
add a comment |
up vote
18
down vote
accepted
up vote
18
down vote
accepted
I think if you just want to ping an url, you can use this code :
try {
URL url = new URL("http://"+params[0]);
HttpURLConnection urlc = (HttpURLConnection) url.openConnection();
urlc.setRequestProperty("User-Agent", "Android Application:"+Z.APP_VERSION);
urlc.setRequestProperty("Connection", "close");
urlc.setConnectTimeout(1000 * 30); // mTimeout is in seconds
urlc.connect();
if (urlc.getResponseCode() == 200) {
Main.Log("getResponseCode == 200");
return new Boolean(true);
}
} catch (MalformedURLException e1) {
e1.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
And, if you want to have the equivalent of a ping, you can put a System.currentTimeMillis() before and after the call, and calculate the difference.
hopes that helps
code snippet found in here
I think if you just want to ping an url, you can use this code :
try {
URL url = new URL("http://"+params[0]);
HttpURLConnection urlc = (HttpURLConnection) url.openConnection();
urlc.setRequestProperty("User-Agent", "Android Application:"+Z.APP_VERSION);
urlc.setRequestProperty("Connection", "close");
urlc.setConnectTimeout(1000 * 30); // mTimeout is in seconds
urlc.connect();
if (urlc.getResponseCode() == 200) {
Main.Log("getResponseCode == 200");
return new Boolean(true);
}
} catch (MalformedURLException e1) {
e1.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
And, if you want to have the equivalent of a ping, you can put a System.currentTimeMillis() before and after the call, and calculate the difference.
hopes that helps
code snippet found in here
edited May 23 '17 at 12:09
Community♦
11
11
answered May 7 '10 at 8:34
Sephy
37.1k27106122
37.1k27106122
This has been working fine for me except for Android 4 devices where it always fails. Any ideas?
– dee
Feb 10 '12 at 16:29
10
You need to move the call off your main thread. Android 4 enforces no network calls on the main thread to prevent ANR issues.
– Nick Campion
Feb 13 '12 at 22:47
2
This will establish a TCP connection with a full 3-way handshake. So the time is nothing like a ping (ICMP-echo -> ICMP-reply
)
– Fredrik Erlandsson
Mar 4 '15 at 19:51
@NickCampion: for me this code is not working on 4.2.2 device which includes samsung s3 and other , any solution
– Punit Sharma
Feb 10 '16 at 10:32
add a comment |
This has been working fine for me except for Android 4 devices where it always fails. Any ideas?
– dee
Feb 10 '12 at 16:29
10
You need to move the call off your main thread. Android 4 enforces no network calls on the main thread to prevent ANR issues.
– Nick Campion
Feb 13 '12 at 22:47
2
This will establish a TCP connection with a full 3-way handshake. So the time is nothing like a ping (ICMP-echo -> ICMP-reply
)
– Fredrik Erlandsson
Mar 4 '15 at 19:51
@NickCampion: for me this code is not working on 4.2.2 device which includes samsung s3 and other , any solution
– Punit Sharma
Feb 10 '16 at 10:32
This has been working fine for me except for Android 4 devices where it always fails. Any ideas?
– dee
Feb 10 '12 at 16:29
This has been working fine for me except for Android 4 devices where it always fails. Any ideas?
– dee
Feb 10 '12 at 16:29
10
10
You need to move the call off your main thread. Android 4 enforces no network calls on the main thread to prevent ANR issues.
– Nick Campion
Feb 13 '12 at 22:47
You need to move the call off your main thread. Android 4 enforces no network calls on the main thread to prevent ANR issues.
– Nick Campion
Feb 13 '12 at 22:47
2
2
This will establish a TCP connection with a full 3-way handshake. So the time is nothing like a ping (
ICMP-echo -> ICMP-reply
)– Fredrik Erlandsson
Mar 4 '15 at 19:51
This will establish a TCP connection with a full 3-way handshake. So the time is nothing like a ping (
ICMP-echo -> ICMP-reply
)– Fredrik Erlandsson
Mar 4 '15 at 19:51
@NickCampion: for me this code is not working on 4.2.2 device which includes samsung s3 and other , any solution
– Punit Sharma
Feb 10 '16 at 10:32
@NickCampion: for me this code is not working on 4.2.2 device which includes samsung s3 and other , any solution
– Punit Sharma
Feb 10 '16 at 10:32
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f2786720%2fhow-to-ping-a-url-in-an-android-service%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZcXIQvA,5m8mSabsz5G7G5cPHAUgw9oM FXZj8C606XJ9JXg8t
Kotlin implementation supporting API 25+ can be found here: stackoverflow.com/questions/53402128/…
– quietbits
Nov 20 at 23:33