how to send message from websocket client to Akka Source using Akka Streams in Play
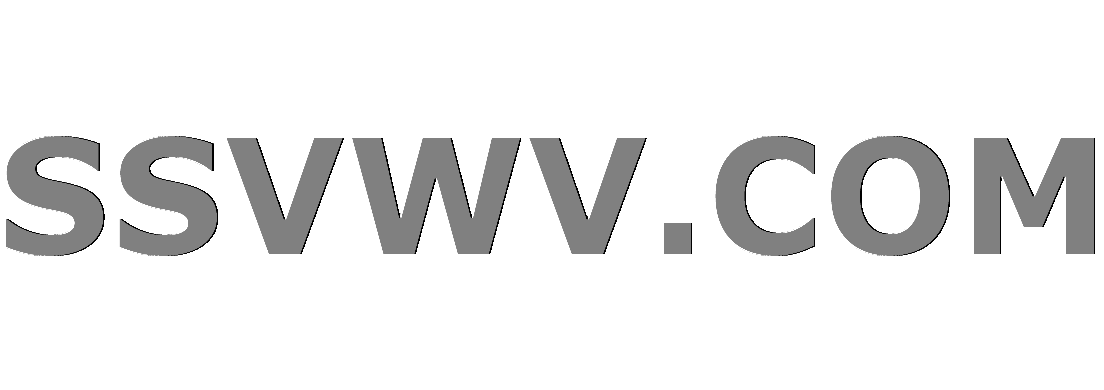
Multi tool use
up vote
0
down vote
favorite
I have an Akka Source that streams numbers using Play Akka WebSocket. The websocket client can send filter message such as 0 or 1 to get even or odd messages.
I do not understand how to send the filter to the Source.
Appreciate any feedback
def numbersSource(filter: Int) =
Source(1 to 1000)
.throttle(1, 1.second, 1, ThrottleMode.Shaping)
.filter{ e =>
filter match {
case 0 =>
e % 2 == 0
case 1 =>
e % 2 != 0
case _ =>
true
}
}.map(_.toString)
def testFlow(): Flow[String, String, NotUsed] = {
Flow[String]
.filter { msg =>
//message from ws client
println(">>"+ msg)
//validate
msg != null && !msg.isEmpty
}
.via(Flow.fromSinkAndSourceCoupled(Sink.ignore, numbersSource(1)))
}
def testWSFlow ():WebSocket = {
WebSocket.acceptOrResult[String, String] {
case rh if sameOriginCheck(rh) =>
Future.successful(
Right(testFlow()))
case rejected =>
LOGGER.error(s"Request $rejected failed same origin check")
Future.successful {
Left(Forbidden("forbidden"))
}
}
}
scala playframework akka akka-stream akka-http
|
show 4 more comments
up vote
0
down vote
favorite
I have an Akka Source that streams numbers using Play Akka WebSocket. The websocket client can send filter message such as 0 or 1 to get even or odd messages.
I do not understand how to send the filter to the Source.
Appreciate any feedback
def numbersSource(filter: Int) =
Source(1 to 1000)
.throttle(1, 1.second, 1, ThrottleMode.Shaping)
.filter{ e =>
filter match {
case 0 =>
e % 2 == 0
case 1 =>
e % 2 != 0
case _ =>
true
}
}.map(_.toString)
def testFlow(): Flow[String, String, NotUsed] = {
Flow[String]
.filter { msg =>
//message from ws client
println(">>"+ msg)
//validate
msg != null && !msg.isEmpty
}
.via(Flow.fromSinkAndSourceCoupled(Sink.ignore, numbersSource(1)))
}
def testWSFlow ():WebSocket = {
WebSocket.acceptOrResult[String, String] {
case rh if sameOriginCheck(rh) =>
Future.successful(
Right(testFlow()))
case rejected =>
LOGGER.error(s"Request $rejected failed same origin check")
Future.successful {
Left(Forbidden("forbidden"))
}
}
}
scala playframework akka akka-stream akka-http
What do you mean "send the filter to the source"?
– erip
Nov 19 at 23:50
What are you seeing?
– erip
Nov 19 at 23:51
In the sample code, I am send value 1 to the numbersSource. How can i send "msg" from client to the numbersSource.
– Vms
Nov 20 at 1:00
You're not sending anything to the source. You're creating a source each time you callnumbersSource
with some argument.
– erip
Nov 20 at 1:05
Can the argument be the message from the websocket client?
– Vms
Nov 20 at 1:13
|
show 4 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have an Akka Source that streams numbers using Play Akka WebSocket. The websocket client can send filter message such as 0 or 1 to get even or odd messages.
I do not understand how to send the filter to the Source.
Appreciate any feedback
def numbersSource(filter: Int) =
Source(1 to 1000)
.throttle(1, 1.second, 1, ThrottleMode.Shaping)
.filter{ e =>
filter match {
case 0 =>
e % 2 == 0
case 1 =>
e % 2 != 0
case _ =>
true
}
}.map(_.toString)
def testFlow(): Flow[String, String, NotUsed] = {
Flow[String]
.filter { msg =>
//message from ws client
println(">>"+ msg)
//validate
msg != null && !msg.isEmpty
}
.via(Flow.fromSinkAndSourceCoupled(Sink.ignore, numbersSource(1)))
}
def testWSFlow ():WebSocket = {
WebSocket.acceptOrResult[String, String] {
case rh if sameOriginCheck(rh) =>
Future.successful(
Right(testFlow()))
case rejected =>
LOGGER.error(s"Request $rejected failed same origin check")
Future.successful {
Left(Forbidden("forbidden"))
}
}
}
scala playframework akka akka-stream akka-http
I have an Akka Source that streams numbers using Play Akka WebSocket. The websocket client can send filter message such as 0 or 1 to get even or odd messages.
I do not understand how to send the filter to the Source.
Appreciate any feedback
def numbersSource(filter: Int) =
Source(1 to 1000)
.throttle(1, 1.second, 1, ThrottleMode.Shaping)
.filter{ e =>
filter match {
case 0 =>
e % 2 == 0
case 1 =>
e % 2 != 0
case _ =>
true
}
}.map(_.toString)
def testFlow(): Flow[String, String, NotUsed] = {
Flow[String]
.filter { msg =>
//message from ws client
println(">>"+ msg)
//validate
msg != null && !msg.isEmpty
}
.via(Flow.fromSinkAndSourceCoupled(Sink.ignore, numbersSource(1)))
}
def testWSFlow ():WebSocket = {
WebSocket.acceptOrResult[String, String] {
case rh if sameOriginCheck(rh) =>
Future.successful(
Right(testFlow()))
case rejected =>
LOGGER.error(s"Request $rejected failed same origin check")
Future.successful {
Left(Forbidden("forbidden"))
}
}
}
scala playframework akka akka-stream akka-http
scala playframework akka akka-stream akka-http
asked Nov 19 at 20:02
Vms
799
799
What do you mean "send the filter to the source"?
– erip
Nov 19 at 23:50
What are you seeing?
– erip
Nov 19 at 23:51
In the sample code, I am send value 1 to the numbersSource. How can i send "msg" from client to the numbersSource.
– Vms
Nov 20 at 1:00
You're not sending anything to the source. You're creating a source each time you callnumbersSource
with some argument.
– erip
Nov 20 at 1:05
Can the argument be the message from the websocket client?
– Vms
Nov 20 at 1:13
|
show 4 more comments
What do you mean "send the filter to the source"?
– erip
Nov 19 at 23:50
What are you seeing?
– erip
Nov 19 at 23:51
In the sample code, I am send value 1 to the numbersSource. How can i send "msg" from client to the numbersSource.
– Vms
Nov 20 at 1:00
You're not sending anything to the source. You're creating a source each time you callnumbersSource
with some argument.
– erip
Nov 20 at 1:05
Can the argument be the message from the websocket client?
– Vms
Nov 20 at 1:13
What do you mean "send the filter to the source"?
– erip
Nov 19 at 23:50
What do you mean "send the filter to the source"?
– erip
Nov 19 at 23:50
What are you seeing?
– erip
Nov 19 at 23:51
What are you seeing?
– erip
Nov 19 at 23:51
In the sample code, I am send value 1 to the numbersSource. How can i send "msg" from client to the numbersSource.
– Vms
Nov 20 at 1:00
In the sample code, I am send value 1 to the numbersSource. How can i send "msg" from client to the numbersSource.
– Vms
Nov 20 at 1:00
You're not sending anything to the source. You're creating a source each time you call
numbersSource
with some argument.– erip
Nov 20 at 1:05
You're not sending anything to the source. You're creating a source each time you call
numbersSource
with some argument.– erip
Nov 20 at 1:05
Can the argument be the message from the websocket client?
– Vms
Nov 20 at 1:13
Can the argument be the message from the websocket client?
– Vms
Nov 20 at 1:13
|
show 4 more comments
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53381824%2fhow-to-send-message-from-websocket-client-to-akka-source-using-akka-streams-in-p%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
peYhDFW,cycoBAYNzWIkC HuFfZPwG9Jr,HHH,tQ,np5CY8dHDykT peCzJGBzdRR,RY0sBnGX,M5X hyWJi3rfhNjQquw4,UYrj5,JQ
What do you mean "send the filter to the source"?
– erip
Nov 19 at 23:50
What are you seeing?
– erip
Nov 19 at 23:51
In the sample code, I am send value 1 to the numbersSource. How can i send "msg" from client to the numbersSource.
– Vms
Nov 20 at 1:00
You're not sending anything to the source. You're creating a source each time you call
numbersSource
with some argument.– erip
Nov 20 at 1:05
Can the argument be the message from the websocket client?
– Vms
Nov 20 at 1:13