JS - Enable ghost element effect with preventDefault call
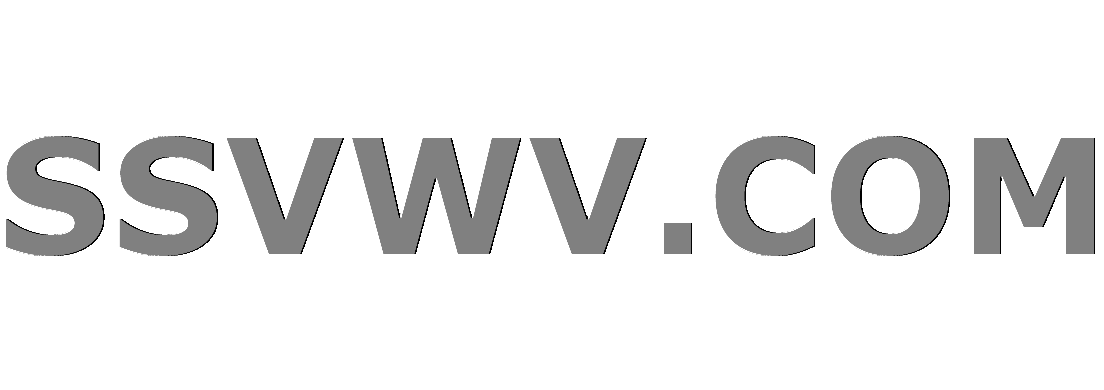
Multi tool use
up vote
0
down vote
favorite
I'm trying to implement operation, I want to drag element from a list of img
tags to different svg:rect
containers.
Using mousedown
and mouseup
is sufficient for understand which img
is picked from the list and in which svg:rect
is dropped.
The code is the following:
<body>
<div style="border: 1px solid black">
<svg width="300" height="100">
<rect id="container" width="60" height="60"></rect>
<rect id="container2" x="70" width="60" height="60" fill="salmon"></rect>
</svg>
</div>
<div id="list">
<img id="item" src="img/cat.png" width="64" />
</div>
<script>
const container = document.getElementById('container');
const container2 = document.getElementById('container2');
const item = document.getElementById('item');
let drag = null
item.addEventListener('mousedown', function (e) {
e.preventDefault();
console.log('mouse down from IMG');
drag = e.target;
});
container.addEventListener('mouseup', function (e) {
e.preventDefault();
console.log('Container 1', drag);
drag = null;
});
container2.addEventListener('mouseup', function (e) {
e.preventDefault();
console.log('Container 2', drag);
drag = null;
});
</script>
My problem is that with e.preventDefault()
in the img
event listener I lost the ghost element effect while user drag the img
.
How to enable that and use preventDefault()
call?
javascript dom svg mouseevent
add a comment |
up vote
0
down vote
favorite
I'm trying to implement operation, I want to drag element from a list of img
tags to different svg:rect
containers.
Using mousedown
and mouseup
is sufficient for understand which img
is picked from the list and in which svg:rect
is dropped.
The code is the following:
<body>
<div style="border: 1px solid black">
<svg width="300" height="100">
<rect id="container" width="60" height="60"></rect>
<rect id="container2" x="70" width="60" height="60" fill="salmon"></rect>
</svg>
</div>
<div id="list">
<img id="item" src="img/cat.png" width="64" />
</div>
<script>
const container = document.getElementById('container');
const container2 = document.getElementById('container2');
const item = document.getElementById('item');
let drag = null
item.addEventListener('mousedown', function (e) {
e.preventDefault();
console.log('mouse down from IMG');
drag = e.target;
});
container.addEventListener('mouseup', function (e) {
e.preventDefault();
console.log('Container 1', drag);
drag = null;
});
container2.addEventListener('mouseup', function (e) {
e.preventDefault();
console.log('Container 2', drag);
drag = null;
});
</script>
My problem is that with e.preventDefault()
in the img
event listener I lost the ghost element effect while user drag the img
.
How to enable that and use preventDefault()
call?
javascript dom svg mouseevent
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm trying to implement operation, I want to drag element from a list of img
tags to different svg:rect
containers.
Using mousedown
and mouseup
is sufficient for understand which img
is picked from the list and in which svg:rect
is dropped.
The code is the following:
<body>
<div style="border: 1px solid black">
<svg width="300" height="100">
<rect id="container" width="60" height="60"></rect>
<rect id="container2" x="70" width="60" height="60" fill="salmon"></rect>
</svg>
</div>
<div id="list">
<img id="item" src="img/cat.png" width="64" />
</div>
<script>
const container = document.getElementById('container');
const container2 = document.getElementById('container2');
const item = document.getElementById('item');
let drag = null
item.addEventListener('mousedown', function (e) {
e.preventDefault();
console.log('mouse down from IMG');
drag = e.target;
});
container.addEventListener('mouseup', function (e) {
e.preventDefault();
console.log('Container 1', drag);
drag = null;
});
container2.addEventListener('mouseup', function (e) {
e.preventDefault();
console.log('Container 2', drag);
drag = null;
});
</script>
My problem is that with e.preventDefault()
in the img
event listener I lost the ghost element effect while user drag the img
.
How to enable that and use preventDefault()
call?
javascript dom svg mouseevent
I'm trying to implement operation, I want to drag element from a list of img
tags to different svg:rect
containers.
Using mousedown
and mouseup
is sufficient for understand which img
is picked from the list and in which svg:rect
is dropped.
The code is the following:
<body>
<div style="border: 1px solid black">
<svg width="300" height="100">
<rect id="container" width="60" height="60"></rect>
<rect id="container2" x="70" width="60" height="60" fill="salmon"></rect>
</svg>
</div>
<div id="list">
<img id="item" src="img/cat.png" width="64" />
</div>
<script>
const container = document.getElementById('container');
const container2 = document.getElementById('container2');
const item = document.getElementById('item');
let drag = null
item.addEventListener('mousedown', function (e) {
e.preventDefault();
console.log('mouse down from IMG');
drag = e.target;
});
container.addEventListener('mouseup', function (e) {
e.preventDefault();
console.log('Container 1', drag);
drag = null;
});
container2.addEventListener('mouseup', function (e) {
e.preventDefault();
console.log('Container 2', drag);
drag = null;
});
</script>
My problem is that with e.preventDefault()
in the img
event listener I lost the ghost element effect while user drag the img
.
How to enable that and use preventDefault()
call?
javascript dom svg mouseevent
javascript dom svg mouseevent
asked Nov 19 at 16:02
EduBic
426
426
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
The ghost element effect comes from the default draggable
property of <img>
Using ondragstart
on the image and ondrop
on an other element would be perfect. See that exemple.
Sadly it's not supported on rect
elements. You can do something with the onmouseover
event on the rect
elements but the user will need to move the mouse after the drop for it to work.
const container = document.getElementById('container');
const container2 = document.getElementById('container2');
const item = document.getElementById('item');
let drag = null
function dragImg (e) {
//e.preventDefault();
console.log('ondrag IMG');
drag = e.target;
};
// Not working
function ondrop1 (e) {
//e.preventDefault();
console.log('Container 1', drag);
drag = null;
};
// Not working
function ondrop2 (e) {
//e.preventDefault();
console.log('Container 2', drag);
drag = null;
};
<div style="border: 1px solid black">
<svg width="300" height="100">
<rect id="container" onmouseover="ondrop1(event)" width="60" height="60"></rect>
<rect id="container2" onmouseover="ondrop2(event)" x="70" width="60" height="60" fill="salmon"></rect>
</svg>
</div>
<div id="list">
<img ondragstart="dragImg(event)" id="item" src="https://ddragon.leagueoflegends.com/cdn/8.22.1/img/champion/Velkoz.png" width="64" />
</div>
[EDIT] If you want to keep using rect
elements with a perfect solution you will need to use ondrop
on the svg and you will find the rect
element under event.target
see documentation here.
Good luck !
Thank you for your tips. I've found two solutions (with mouseover they are 3) that I described in my answer.
– EduBic
Nov 20 at 14:47
add a comment |
up vote
0
down vote
accepted
After some search and tests, I came up with two possible solutions:
First solution: don't use html5 drag and drop at all.
Instead use mousedown
applied to the drag element and mouseup
applied to the drop element. In mousedown
event the preventDefault()
call is needed. This remove the ghost element effect while user is dragging. In order to reintroduce that effect you need to build your own ghost element with the css properties pointer-events: none
and position: absolute
.
Second solution: use partially drag and drop html5 feature.
Set dragstart
event on your drag element (img) and drop
and dragover
on your drop element (svg). Check e.target
in the drop
event and you have the reference of your svg:rect
element.
An example here:
https://gist.github.com/EduBic/49e36485c70c5a6d15df7db1861333de
N.B. remember to add the other event and to manage other cases.
Well the Second solution with e.target looks perfect ! Good job ! I'm going to edit my answer if I can.
– GuySake
Nov 21 at 15:16
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
The ghost element effect comes from the default draggable
property of <img>
Using ondragstart
on the image and ondrop
on an other element would be perfect. See that exemple.
Sadly it's not supported on rect
elements. You can do something with the onmouseover
event on the rect
elements but the user will need to move the mouse after the drop for it to work.
const container = document.getElementById('container');
const container2 = document.getElementById('container2');
const item = document.getElementById('item');
let drag = null
function dragImg (e) {
//e.preventDefault();
console.log('ondrag IMG');
drag = e.target;
};
// Not working
function ondrop1 (e) {
//e.preventDefault();
console.log('Container 1', drag);
drag = null;
};
// Not working
function ondrop2 (e) {
//e.preventDefault();
console.log('Container 2', drag);
drag = null;
};
<div style="border: 1px solid black">
<svg width="300" height="100">
<rect id="container" onmouseover="ondrop1(event)" width="60" height="60"></rect>
<rect id="container2" onmouseover="ondrop2(event)" x="70" width="60" height="60" fill="salmon"></rect>
</svg>
</div>
<div id="list">
<img ondragstart="dragImg(event)" id="item" src="https://ddragon.leagueoflegends.com/cdn/8.22.1/img/champion/Velkoz.png" width="64" />
</div>
[EDIT] If you want to keep using rect
elements with a perfect solution you will need to use ondrop
on the svg and you will find the rect
element under event.target
see documentation here.
Good luck !
Thank you for your tips. I've found two solutions (with mouseover they are 3) that I described in my answer.
– EduBic
Nov 20 at 14:47
add a comment |
up vote
1
down vote
The ghost element effect comes from the default draggable
property of <img>
Using ondragstart
on the image and ondrop
on an other element would be perfect. See that exemple.
Sadly it's not supported on rect
elements. You can do something with the onmouseover
event on the rect
elements but the user will need to move the mouse after the drop for it to work.
const container = document.getElementById('container');
const container2 = document.getElementById('container2');
const item = document.getElementById('item');
let drag = null
function dragImg (e) {
//e.preventDefault();
console.log('ondrag IMG');
drag = e.target;
};
// Not working
function ondrop1 (e) {
//e.preventDefault();
console.log('Container 1', drag);
drag = null;
};
// Not working
function ondrop2 (e) {
//e.preventDefault();
console.log('Container 2', drag);
drag = null;
};
<div style="border: 1px solid black">
<svg width="300" height="100">
<rect id="container" onmouseover="ondrop1(event)" width="60" height="60"></rect>
<rect id="container2" onmouseover="ondrop2(event)" x="70" width="60" height="60" fill="salmon"></rect>
</svg>
</div>
<div id="list">
<img ondragstart="dragImg(event)" id="item" src="https://ddragon.leagueoflegends.com/cdn/8.22.1/img/champion/Velkoz.png" width="64" />
</div>
[EDIT] If you want to keep using rect
elements with a perfect solution you will need to use ondrop
on the svg and you will find the rect
element under event.target
see documentation here.
Good luck !
Thank you for your tips. I've found two solutions (with mouseover they are 3) that I described in my answer.
– EduBic
Nov 20 at 14:47
add a comment |
up vote
1
down vote
up vote
1
down vote
The ghost element effect comes from the default draggable
property of <img>
Using ondragstart
on the image and ondrop
on an other element would be perfect. See that exemple.
Sadly it's not supported on rect
elements. You can do something with the onmouseover
event on the rect
elements but the user will need to move the mouse after the drop for it to work.
const container = document.getElementById('container');
const container2 = document.getElementById('container2');
const item = document.getElementById('item');
let drag = null
function dragImg (e) {
//e.preventDefault();
console.log('ondrag IMG');
drag = e.target;
};
// Not working
function ondrop1 (e) {
//e.preventDefault();
console.log('Container 1', drag);
drag = null;
};
// Not working
function ondrop2 (e) {
//e.preventDefault();
console.log('Container 2', drag);
drag = null;
};
<div style="border: 1px solid black">
<svg width="300" height="100">
<rect id="container" onmouseover="ondrop1(event)" width="60" height="60"></rect>
<rect id="container2" onmouseover="ondrop2(event)" x="70" width="60" height="60" fill="salmon"></rect>
</svg>
</div>
<div id="list">
<img ondragstart="dragImg(event)" id="item" src="https://ddragon.leagueoflegends.com/cdn/8.22.1/img/champion/Velkoz.png" width="64" />
</div>
[EDIT] If you want to keep using rect
elements with a perfect solution you will need to use ondrop
on the svg and you will find the rect
element under event.target
see documentation here.
Good luck !
The ghost element effect comes from the default draggable
property of <img>
Using ondragstart
on the image and ondrop
on an other element would be perfect. See that exemple.
Sadly it's not supported on rect
elements. You can do something with the onmouseover
event on the rect
elements but the user will need to move the mouse after the drop for it to work.
const container = document.getElementById('container');
const container2 = document.getElementById('container2');
const item = document.getElementById('item');
let drag = null
function dragImg (e) {
//e.preventDefault();
console.log('ondrag IMG');
drag = e.target;
};
// Not working
function ondrop1 (e) {
//e.preventDefault();
console.log('Container 1', drag);
drag = null;
};
// Not working
function ondrop2 (e) {
//e.preventDefault();
console.log('Container 2', drag);
drag = null;
};
<div style="border: 1px solid black">
<svg width="300" height="100">
<rect id="container" onmouseover="ondrop1(event)" width="60" height="60"></rect>
<rect id="container2" onmouseover="ondrop2(event)" x="70" width="60" height="60" fill="salmon"></rect>
</svg>
</div>
<div id="list">
<img ondragstart="dragImg(event)" id="item" src="https://ddragon.leagueoflegends.com/cdn/8.22.1/img/champion/Velkoz.png" width="64" />
</div>
[EDIT] If you want to keep using rect
elements with a perfect solution you will need to use ondrop
on the svg and you will find the rect
element under event.target
see documentation here.
Good luck !
const container = document.getElementById('container');
const container2 = document.getElementById('container2');
const item = document.getElementById('item');
let drag = null
function dragImg (e) {
//e.preventDefault();
console.log('ondrag IMG');
drag = e.target;
};
// Not working
function ondrop1 (e) {
//e.preventDefault();
console.log('Container 1', drag);
drag = null;
};
// Not working
function ondrop2 (e) {
//e.preventDefault();
console.log('Container 2', drag);
drag = null;
};
<div style="border: 1px solid black">
<svg width="300" height="100">
<rect id="container" onmouseover="ondrop1(event)" width="60" height="60"></rect>
<rect id="container2" onmouseover="ondrop2(event)" x="70" width="60" height="60" fill="salmon"></rect>
</svg>
</div>
<div id="list">
<img ondragstart="dragImg(event)" id="item" src="https://ddragon.leagueoflegends.com/cdn/8.22.1/img/champion/Velkoz.png" width="64" />
</div>
const container = document.getElementById('container');
const container2 = document.getElementById('container2');
const item = document.getElementById('item');
let drag = null
function dragImg (e) {
//e.preventDefault();
console.log('ondrag IMG');
drag = e.target;
};
// Not working
function ondrop1 (e) {
//e.preventDefault();
console.log('Container 1', drag);
drag = null;
};
// Not working
function ondrop2 (e) {
//e.preventDefault();
console.log('Container 2', drag);
drag = null;
};
<div style="border: 1px solid black">
<svg width="300" height="100">
<rect id="container" onmouseover="ondrop1(event)" width="60" height="60"></rect>
<rect id="container2" onmouseover="ondrop2(event)" x="70" width="60" height="60" fill="salmon"></rect>
</svg>
</div>
<div id="list">
<img ondragstart="dragImg(event)" id="item" src="https://ddragon.leagueoflegends.com/cdn/8.22.1/img/champion/Velkoz.png" width="64" />
</div>
edited Nov 21 at 15:22
answered Nov 19 at 17:04
GuySake
874
874
Thank you for your tips. I've found two solutions (with mouseover they are 3) that I described in my answer.
– EduBic
Nov 20 at 14:47
add a comment |
Thank you for your tips. I've found two solutions (with mouseover they are 3) that I described in my answer.
– EduBic
Nov 20 at 14:47
Thank you for your tips. I've found two solutions (with mouseover they are 3) that I described in my answer.
– EduBic
Nov 20 at 14:47
Thank you for your tips. I've found two solutions (with mouseover they are 3) that I described in my answer.
– EduBic
Nov 20 at 14:47
add a comment |
up vote
0
down vote
accepted
After some search and tests, I came up with two possible solutions:
First solution: don't use html5 drag and drop at all.
Instead use mousedown
applied to the drag element and mouseup
applied to the drop element. In mousedown
event the preventDefault()
call is needed. This remove the ghost element effect while user is dragging. In order to reintroduce that effect you need to build your own ghost element with the css properties pointer-events: none
and position: absolute
.
Second solution: use partially drag and drop html5 feature.
Set dragstart
event on your drag element (img) and drop
and dragover
on your drop element (svg). Check e.target
in the drop
event and you have the reference of your svg:rect
element.
An example here:
https://gist.github.com/EduBic/49e36485c70c5a6d15df7db1861333de
N.B. remember to add the other event and to manage other cases.
Well the Second solution with e.target looks perfect ! Good job ! I'm going to edit my answer if I can.
– GuySake
Nov 21 at 15:16
add a comment |
up vote
0
down vote
accepted
After some search and tests, I came up with two possible solutions:
First solution: don't use html5 drag and drop at all.
Instead use mousedown
applied to the drag element and mouseup
applied to the drop element. In mousedown
event the preventDefault()
call is needed. This remove the ghost element effect while user is dragging. In order to reintroduce that effect you need to build your own ghost element with the css properties pointer-events: none
and position: absolute
.
Second solution: use partially drag and drop html5 feature.
Set dragstart
event on your drag element (img) and drop
and dragover
on your drop element (svg). Check e.target
in the drop
event and you have the reference of your svg:rect
element.
An example here:
https://gist.github.com/EduBic/49e36485c70c5a6d15df7db1861333de
N.B. remember to add the other event and to manage other cases.
Well the Second solution with e.target looks perfect ! Good job ! I'm going to edit my answer if I can.
– GuySake
Nov 21 at 15:16
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
After some search and tests, I came up with two possible solutions:
First solution: don't use html5 drag and drop at all.
Instead use mousedown
applied to the drag element and mouseup
applied to the drop element. In mousedown
event the preventDefault()
call is needed. This remove the ghost element effect while user is dragging. In order to reintroduce that effect you need to build your own ghost element with the css properties pointer-events: none
and position: absolute
.
Second solution: use partially drag and drop html5 feature.
Set dragstart
event on your drag element (img) and drop
and dragover
on your drop element (svg). Check e.target
in the drop
event and you have the reference of your svg:rect
element.
An example here:
https://gist.github.com/EduBic/49e36485c70c5a6d15df7db1861333de
N.B. remember to add the other event and to manage other cases.
After some search and tests, I came up with two possible solutions:
First solution: don't use html5 drag and drop at all.
Instead use mousedown
applied to the drag element and mouseup
applied to the drop element. In mousedown
event the preventDefault()
call is needed. This remove the ghost element effect while user is dragging. In order to reintroduce that effect you need to build your own ghost element with the css properties pointer-events: none
and position: absolute
.
Second solution: use partially drag and drop html5 feature.
Set dragstart
event on your drag element (img) and drop
and dragover
on your drop element (svg). Check e.target
in the drop
event and you have the reference of your svg:rect
element.
An example here:
https://gist.github.com/EduBic/49e36485c70c5a6d15df7db1861333de
N.B. remember to add the other event and to manage other cases.
answered Nov 20 at 14:44
EduBic
426
426
Well the Second solution with e.target looks perfect ! Good job ! I'm going to edit my answer if I can.
– GuySake
Nov 21 at 15:16
add a comment |
Well the Second solution with e.target looks perfect ! Good job ! I'm going to edit my answer if I can.
– GuySake
Nov 21 at 15:16
Well the Second solution with e.target looks perfect ! Good job ! I'm going to edit my answer if I can.
– GuySake
Nov 21 at 15:16
Well the Second solution with e.target looks perfect ! Good job ! I'm going to edit my answer if I can.
– GuySake
Nov 21 at 15:16
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53378472%2fjs-enable-ghost-element-effect-with-preventdefault-call%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
s2,6u PHLF,A