Trying to convert a numeric value to css
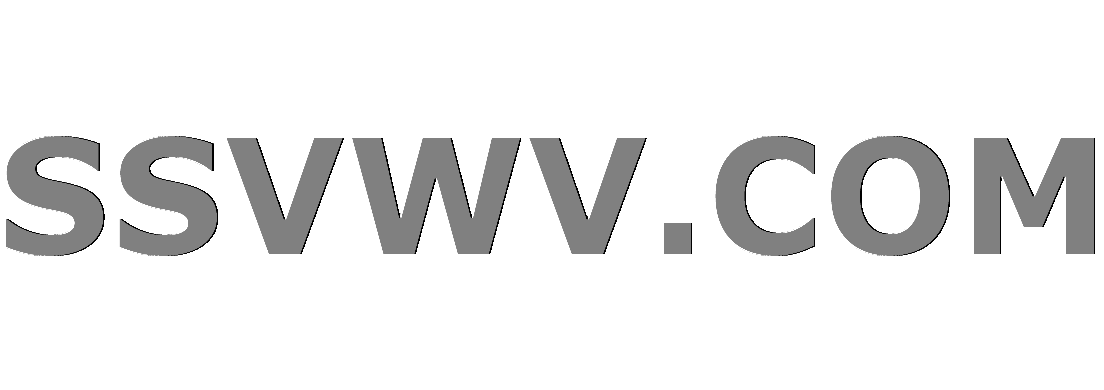
Multi tool use
up vote
1
down vote
favorite
I am trying to iterate over a number of DIVS, pull a numeric value from said DIVs, if that value is == to a specific value in the JS, apply a specific CSS Class back to the DIV it came from.
This is the code I have so far
const getRating = document.getElementsByClassName('my-ratings');
let getRatingValues = ;
for(var i = 0; i < getRating.length; i++) {
getRatingValues += getRating[i].textContent;
if (getRatingValues == 5) {
getRating.classList.add('rating-5');
}
if (getRatingValues == 4) {
getRating.classList.add('rating-4');
}
if (getRatingValues == 3) {
getRating.classList.add('rating-3');
}
if (getRatingValues == 2) {
getRating.classList.add('rating-2');
}
if (getRatingValues == 1) {
getRating.classList.add('rating-1');
}
}
I feel like I am close, but just can't break the back of it...
At the moment, the script seems to fill the getRatingValues as a string, so if I have values of 5, 3, 1, 2, 2, 4 the getRatingValues will be 531224. Could someone point me in the right direction please?
javascript css
|
show 1 more comment
up vote
1
down vote
favorite
I am trying to iterate over a number of DIVS, pull a numeric value from said DIVs, if that value is == to a specific value in the JS, apply a specific CSS Class back to the DIV it came from.
This is the code I have so far
const getRating = document.getElementsByClassName('my-ratings');
let getRatingValues = ;
for(var i = 0; i < getRating.length; i++) {
getRatingValues += getRating[i].textContent;
if (getRatingValues == 5) {
getRating.classList.add('rating-5');
}
if (getRatingValues == 4) {
getRating.classList.add('rating-4');
}
if (getRatingValues == 3) {
getRating.classList.add('rating-3');
}
if (getRatingValues == 2) {
getRating.classList.add('rating-2');
}
if (getRatingValues == 1) {
getRating.classList.add('rating-1');
}
}
I feel like I am close, but just can't break the back of it...
At the moment, the script seems to fill the getRatingValues as a string, so if I have values of 5, 3, 1, 2, 2, 4 the getRatingValues will be 531224. Could someone point me in the right direction please?
javascript css
5
+=
does not add elements to an array. You wantgetRatingValues.push(...)
. Once you fix that, things still don't make sense; why is it an array in the first place? What are you trying to do?
– Pointy
Nov 19 at 16:02
why you define getRatingValues as an array but use it as an integer? thats not clear :) And you can save many space if you write your code dynamical like: getRating.classList.add('rating-' + getRatingValues);
– episch
Nov 19 at 16:04
+=
also does something different when the operators are strings (andtextContent
is a string).
– Sergiu Paraschiv
Nov 19 at 16:04
Why are you adding a string to an array?
– epascarello
Nov 19 at 16:05
I am collecting the values from the DIV with classmy-ratings
, I am then transforming that value into a CSS Class, so if the DIV has text in it that is 3, I generate a css class called ratings-3 and apply it to the DIV
– Takuhii
Nov 19 at 16:23
|
show 1 more comment
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am trying to iterate over a number of DIVS, pull a numeric value from said DIVs, if that value is == to a specific value in the JS, apply a specific CSS Class back to the DIV it came from.
This is the code I have so far
const getRating = document.getElementsByClassName('my-ratings');
let getRatingValues = ;
for(var i = 0; i < getRating.length; i++) {
getRatingValues += getRating[i].textContent;
if (getRatingValues == 5) {
getRating.classList.add('rating-5');
}
if (getRatingValues == 4) {
getRating.classList.add('rating-4');
}
if (getRatingValues == 3) {
getRating.classList.add('rating-3');
}
if (getRatingValues == 2) {
getRating.classList.add('rating-2');
}
if (getRatingValues == 1) {
getRating.classList.add('rating-1');
}
}
I feel like I am close, but just can't break the back of it...
At the moment, the script seems to fill the getRatingValues as a string, so if I have values of 5, 3, 1, 2, 2, 4 the getRatingValues will be 531224. Could someone point me in the right direction please?
javascript css
I am trying to iterate over a number of DIVS, pull a numeric value from said DIVs, if that value is == to a specific value in the JS, apply a specific CSS Class back to the DIV it came from.
This is the code I have so far
const getRating = document.getElementsByClassName('my-ratings');
let getRatingValues = ;
for(var i = 0; i < getRating.length; i++) {
getRatingValues += getRating[i].textContent;
if (getRatingValues == 5) {
getRating.classList.add('rating-5');
}
if (getRatingValues == 4) {
getRating.classList.add('rating-4');
}
if (getRatingValues == 3) {
getRating.classList.add('rating-3');
}
if (getRatingValues == 2) {
getRating.classList.add('rating-2');
}
if (getRatingValues == 1) {
getRating.classList.add('rating-1');
}
}
I feel like I am close, but just can't break the back of it...
At the moment, the script seems to fill the getRatingValues as a string, so if I have values of 5, 3, 1, 2, 2, 4 the getRatingValues will be 531224. Could someone point me in the right direction please?
javascript css
javascript css
asked Nov 19 at 16:01


Takuhii
1631217
1631217
5
+=
does not add elements to an array. You wantgetRatingValues.push(...)
. Once you fix that, things still don't make sense; why is it an array in the first place? What are you trying to do?
– Pointy
Nov 19 at 16:02
why you define getRatingValues as an array but use it as an integer? thats not clear :) And you can save many space if you write your code dynamical like: getRating.classList.add('rating-' + getRatingValues);
– episch
Nov 19 at 16:04
+=
also does something different when the operators are strings (andtextContent
is a string).
– Sergiu Paraschiv
Nov 19 at 16:04
Why are you adding a string to an array?
– epascarello
Nov 19 at 16:05
I am collecting the values from the DIV with classmy-ratings
, I am then transforming that value into a CSS Class, so if the DIV has text in it that is 3, I generate a css class called ratings-3 and apply it to the DIV
– Takuhii
Nov 19 at 16:23
|
show 1 more comment
5
+=
does not add elements to an array. You wantgetRatingValues.push(...)
. Once you fix that, things still don't make sense; why is it an array in the first place? What are you trying to do?
– Pointy
Nov 19 at 16:02
why you define getRatingValues as an array but use it as an integer? thats not clear :) And you can save many space if you write your code dynamical like: getRating.classList.add('rating-' + getRatingValues);
– episch
Nov 19 at 16:04
+=
also does something different when the operators are strings (andtextContent
is a string).
– Sergiu Paraschiv
Nov 19 at 16:04
Why are you adding a string to an array?
– epascarello
Nov 19 at 16:05
I am collecting the values from the DIV with classmy-ratings
, I am then transforming that value into a CSS Class, so if the DIV has text in it that is 3, I generate a css class called ratings-3 and apply it to the DIV
– Takuhii
Nov 19 at 16:23
5
5
+=
does not add elements to an array. You want getRatingValues.push(...)
. Once you fix that, things still don't make sense; why is it an array in the first place? What are you trying to do?– Pointy
Nov 19 at 16:02
+=
does not add elements to an array. You want getRatingValues.push(...)
. Once you fix that, things still don't make sense; why is it an array in the first place? What are you trying to do?– Pointy
Nov 19 at 16:02
why you define getRatingValues as an array but use it as an integer? thats not clear :) And you can save many space if you write your code dynamical like: getRating.classList.add('rating-' + getRatingValues);
– episch
Nov 19 at 16:04
why you define getRatingValues as an array but use it as an integer? thats not clear :) And you can save many space if you write your code dynamical like: getRating.classList.add('rating-' + getRatingValues);
– episch
Nov 19 at 16:04
+=
also does something different when the operators are strings (and textContent
is a string).– Sergiu Paraschiv
Nov 19 at 16:04
+=
also does something different when the operators are strings (and textContent
is a string).– Sergiu Paraschiv
Nov 19 at 16:04
Why are you adding a string to an array?
– epascarello
Nov 19 at 16:05
Why are you adding a string to an array?
– epascarello
Nov 19 at 16:05
I am collecting the values from the DIV with class
my-ratings
, I am then transforming that value into a CSS Class, so if the DIV has text in it that is 3, I generate a css class called ratings-3 and apply it to the DIV– Takuhii
Nov 19 at 16:23
I am collecting the values from the DIV with class
my-ratings
, I am then transforming that value into a CSS Class, so if the DIV has text in it that is 3, I generate a css class called ratings-3 and apply it to the DIV– Takuhii
Nov 19 at 16:23
|
show 1 more comment
4 Answers
4
active
oldest
votes
up vote
3
down vote
accepted
DRY - and cast to number:
let getRatingValues = 0;
document.querySelectorAll('.my-ratings').forEach(function(rate) { // for each my rating
let val = rate.textContent; // get the rate
getRatingValues += isNaN(val)?0:+val; // convert to number if is IS a number and add
rate.classList.add('rating-'+val); // add the class - here or outside the loop?
})
I would have thought getRating.classList.add('rating-'+val);
should be outside the loop since now you will get 5 classes on one div if all 5 are set
For IE you need
var ratings = document.querySelectorAll('my-ratings');
for (var i=0;i<ratings.length;i++) {
let val = ratings[i].innerText, rate = ratings[i];
Ok, updated.....
– mplungjan
Nov 19 at 16:09
This is really tidy, but I am gettingdocument.getElementsByClassName('my-ratings').forEach is not a function
– Takuhii
Nov 19 at 16:21
Please see update. I changed to use querySelectorAll and IE does not like forEach on nodes - I could have created a working example if I'd had the HTML
– mplungjan
Nov 19 at 16:23
One more thing, getRating is now not defined, do I just create a const and place the document.querySelectorAll in it?
– Takuhii
Nov 19 at 16:32
Ah no. I assume rate.classList.add
– mplungjan
Nov 19 at 16:34
add a comment |
up vote
1
down vote
You need to convert the string to Number
.
Change
getRatingValues += getRating[i].textContent;
to
getRatingValues += Number(getRating[i].textContent);
Also, your getRatingValues
should not be initialized to , instead it should be
let getRatingValues = 0;
For completeness, it's probably worth mentioning you can cast the value to a number in a variety of different ways:+= Number(var)
or+= parseInt(var)
or, my favorite,+= +var
....
– cale_b
Nov 19 at 16:07
add a comment |
up vote
0
down vote
Like my comment you can optimize your code very easy:
that optimized Code:
const getRating = document.getElementsByClassName('my-ratings');
for(var i = 0; i < getRating.length; i++) {
getRatingValues += Number(getRating[i].textContent);
if (getRatingValues !== undefined) {
getRating.classList.add('rating-' + i);
}
}
add a comment |
up vote
0
down vote
Try parseInt
getRatingValues += parseInt(getRating[i].textContent);
Reference : https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/parseInt
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
DRY - and cast to number:
let getRatingValues = 0;
document.querySelectorAll('.my-ratings').forEach(function(rate) { // for each my rating
let val = rate.textContent; // get the rate
getRatingValues += isNaN(val)?0:+val; // convert to number if is IS a number and add
rate.classList.add('rating-'+val); // add the class - here or outside the loop?
})
I would have thought getRating.classList.add('rating-'+val);
should be outside the loop since now you will get 5 classes on one div if all 5 are set
For IE you need
var ratings = document.querySelectorAll('my-ratings');
for (var i=0;i<ratings.length;i++) {
let val = ratings[i].innerText, rate = ratings[i];
Ok, updated.....
– mplungjan
Nov 19 at 16:09
This is really tidy, but I am gettingdocument.getElementsByClassName('my-ratings').forEach is not a function
– Takuhii
Nov 19 at 16:21
Please see update. I changed to use querySelectorAll and IE does not like forEach on nodes - I could have created a working example if I'd had the HTML
– mplungjan
Nov 19 at 16:23
One more thing, getRating is now not defined, do I just create a const and place the document.querySelectorAll in it?
– Takuhii
Nov 19 at 16:32
Ah no. I assume rate.classList.add
– mplungjan
Nov 19 at 16:34
add a comment |
up vote
3
down vote
accepted
DRY - and cast to number:
let getRatingValues = 0;
document.querySelectorAll('.my-ratings').forEach(function(rate) { // for each my rating
let val = rate.textContent; // get the rate
getRatingValues += isNaN(val)?0:+val; // convert to number if is IS a number and add
rate.classList.add('rating-'+val); // add the class - here or outside the loop?
})
I would have thought getRating.classList.add('rating-'+val);
should be outside the loop since now you will get 5 classes on one div if all 5 are set
For IE you need
var ratings = document.querySelectorAll('my-ratings');
for (var i=0;i<ratings.length;i++) {
let val = ratings[i].innerText, rate = ratings[i];
Ok, updated.....
– mplungjan
Nov 19 at 16:09
This is really tidy, but I am gettingdocument.getElementsByClassName('my-ratings').forEach is not a function
– Takuhii
Nov 19 at 16:21
Please see update. I changed to use querySelectorAll and IE does not like forEach on nodes - I could have created a working example if I'd had the HTML
– mplungjan
Nov 19 at 16:23
One more thing, getRating is now not defined, do I just create a const and place the document.querySelectorAll in it?
– Takuhii
Nov 19 at 16:32
Ah no. I assume rate.classList.add
– mplungjan
Nov 19 at 16:34
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
DRY - and cast to number:
let getRatingValues = 0;
document.querySelectorAll('.my-ratings').forEach(function(rate) { // for each my rating
let val = rate.textContent; // get the rate
getRatingValues += isNaN(val)?0:+val; // convert to number if is IS a number and add
rate.classList.add('rating-'+val); // add the class - here or outside the loop?
})
I would have thought getRating.classList.add('rating-'+val);
should be outside the loop since now you will get 5 classes on one div if all 5 are set
For IE you need
var ratings = document.querySelectorAll('my-ratings');
for (var i=0;i<ratings.length;i++) {
let val = ratings[i].innerText, rate = ratings[i];
DRY - and cast to number:
let getRatingValues = 0;
document.querySelectorAll('.my-ratings').forEach(function(rate) { // for each my rating
let val = rate.textContent; // get the rate
getRatingValues += isNaN(val)?0:+val; // convert to number if is IS a number and add
rate.classList.add('rating-'+val); // add the class - here or outside the loop?
})
I would have thought getRating.classList.add('rating-'+val);
should be outside the loop since now you will get 5 classes on one div if all 5 are set
For IE you need
var ratings = document.querySelectorAll('my-ratings');
for (var i=0;i<ratings.length;i++) {
let val = ratings[i].innerText, rate = ratings[i];
edited Nov 20 at 5:56
answered Nov 19 at 16:07
mplungjan
86k20121180
86k20121180
Ok, updated.....
– mplungjan
Nov 19 at 16:09
This is really tidy, but I am gettingdocument.getElementsByClassName('my-ratings').forEach is not a function
– Takuhii
Nov 19 at 16:21
Please see update. I changed to use querySelectorAll and IE does not like forEach on nodes - I could have created a working example if I'd had the HTML
– mplungjan
Nov 19 at 16:23
One more thing, getRating is now not defined, do I just create a const and place the document.querySelectorAll in it?
– Takuhii
Nov 19 at 16:32
Ah no. I assume rate.classList.add
– mplungjan
Nov 19 at 16:34
add a comment |
Ok, updated.....
– mplungjan
Nov 19 at 16:09
This is really tidy, but I am gettingdocument.getElementsByClassName('my-ratings').forEach is not a function
– Takuhii
Nov 19 at 16:21
Please see update. I changed to use querySelectorAll and IE does not like forEach on nodes - I could have created a working example if I'd had the HTML
– mplungjan
Nov 19 at 16:23
One more thing, getRating is now not defined, do I just create a const and place the document.querySelectorAll in it?
– Takuhii
Nov 19 at 16:32
Ah no. I assume rate.classList.add
– mplungjan
Nov 19 at 16:34
Ok, updated.....
– mplungjan
Nov 19 at 16:09
Ok, updated.....
– mplungjan
Nov 19 at 16:09
This is really tidy, but I am getting
document.getElementsByClassName('my-ratings').forEach is not a function
– Takuhii
Nov 19 at 16:21
This is really tidy, but I am getting
document.getElementsByClassName('my-ratings').forEach is not a function
– Takuhii
Nov 19 at 16:21
Please see update. I changed to use querySelectorAll and IE does not like forEach on nodes - I could have created a working example if I'd had the HTML
– mplungjan
Nov 19 at 16:23
Please see update. I changed to use querySelectorAll and IE does not like forEach on nodes - I could have created a working example if I'd had the HTML
– mplungjan
Nov 19 at 16:23
One more thing, getRating is now not defined, do I just create a const and place the document.querySelectorAll in it?
– Takuhii
Nov 19 at 16:32
One more thing, getRating is now not defined, do I just create a const and place the document.querySelectorAll in it?
– Takuhii
Nov 19 at 16:32
Ah no. I assume rate.classList.add
– mplungjan
Nov 19 at 16:34
Ah no. I assume rate.classList.add
– mplungjan
Nov 19 at 16:34
add a comment |
up vote
1
down vote
You need to convert the string to Number
.
Change
getRatingValues += getRating[i].textContent;
to
getRatingValues += Number(getRating[i].textContent);
Also, your getRatingValues
should not be initialized to , instead it should be
let getRatingValues = 0;
For completeness, it's probably worth mentioning you can cast the value to a number in a variety of different ways:+= Number(var)
or+= parseInt(var)
or, my favorite,+= +var
....
– cale_b
Nov 19 at 16:07
add a comment |
up vote
1
down vote
You need to convert the string to Number
.
Change
getRatingValues += getRating[i].textContent;
to
getRatingValues += Number(getRating[i].textContent);
Also, your getRatingValues
should not be initialized to , instead it should be
let getRatingValues = 0;
For completeness, it's probably worth mentioning you can cast the value to a number in a variety of different ways:+= Number(var)
or+= parseInt(var)
or, my favorite,+= +var
....
– cale_b
Nov 19 at 16:07
add a comment |
up vote
1
down vote
up vote
1
down vote
You need to convert the string to Number
.
Change
getRatingValues += getRating[i].textContent;
to
getRatingValues += Number(getRating[i].textContent);
Also, your getRatingValues
should not be initialized to , instead it should be
let getRatingValues = 0;
You need to convert the string to Number
.
Change
getRatingValues += getRating[i].textContent;
to
getRatingValues += Number(getRating[i].textContent);
Also, your getRatingValues
should not be initialized to , instead it should be
let getRatingValues = 0;
answered Nov 19 at 16:04


Dinesh Pandiyan
1,454722
1,454722
For completeness, it's probably worth mentioning you can cast the value to a number in a variety of different ways:+= Number(var)
or+= parseInt(var)
or, my favorite,+= +var
....
– cale_b
Nov 19 at 16:07
add a comment |
For completeness, it's probably worth mentioning you can cast the value to a number in a variety of different ways:+= Number(var)
or+= parseInt(var)
or, my favorite,+= +var
....
– cale_b
Nov 19 at 16:07
For completeness, it's probably worth mentioning you can cast the value to a number in a variety of different ways:
+= Number(var)
or += parseInt(var)
or, my favorite, += +var
....– cale_b
Nov 19 at 16:07
For completeness, it's probably worth mentioning you can cast the value to a number in a variety of different ways:
+= Number(var)
or += parseInt(var)
or, my favorite, += +var
....– cale_b
Nov 19 at 16:07
add a comment |
up vote
0
down vote
Like my comment you can optimize your code very easy:
that optimized Code:
const getRating = document.getElementsByClassName('my-ratings');
for(var i = 0; i < getRating.length; i++) {
getRatingValues += Number(getRating[i].textContent);
if (getRatingValues !== undefined) {
getRating.classList.add('rating-' + i);
}
}
add a comment |
up vote
0
down vote
Like my comment you can optimize your code very easy:
that optimized Code:
const getRating = document.getElementsByClassName('my-ratings');
for(var i = 0; i < getRating.length; i++) {
getRatingValues += Number(getRating[i].textContent);
if (getRatingValues !== undefined) {
getRating.classList.add('rating-' + i);
}
}
add a comment |
up vote
0
down vote
up vote
0
down vote
Like my comment you can optimize your code very easy:
that optimized Code:
const getRating = document.getElementsByClassName('my-ratings');
for(var i = 0; i < getRating.length; i++) {
getRatingValues += Number(getRating[i].textContent);
if (getRatingValues !== undefined) {
getRating.classList.add('rating-' + i);
}
}
Like my comment you can optimize your code very easy:
that optimized Code:
const getRating = document.getElementsByClassName('my-ratings');
for(var i = 0; i < getRating.length; i++) {
getRatingValues += Number(getRating[i].textContent);
if (getRatingValues !== undefined) {
getRating.classList.add('rating-' + i);
}
}
answered Nov 19 at 16:07


episch
302114
302114
add a comment |
add a comment |
up vote
0
down vote
Try parseInt
getRatingValues += parseInt(getRating[i].textContent);
Reference : https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/parseInt
add a comment |
up vote
0
down vote
Try parseInt
getRatingValues += parseInt(getRating[i].textContent);
Reference : https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/parseInt
add a comment |
up vote
0
down vote
up vote
0
down vote
Try parseInt
getRatingValues += parseInt(getRating[i].textContent);
Reference : https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/parseInt
Try parseInt
getRatingValues += parseInt(getRating[i].textContent);
Reference : https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/parseInt
edited Nov 19 at 16:15
mplungjan
86k20121180
86k20121180
answered Nov 19 at 16:05


Anurag Sinha
418512
418512
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53378452%2ftrying-to-convert-a-numeric-value-to-css%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
lkxeRbvQodD1uq6,0UsJP,CGRnC66rhIXElCpa 910gwg2D8EJKco2xWnTqfFiID84N0g1JF
5
+=
does not add elements to an array. You wantgetRatingValues.push(...)
. Once you fix that, things still don't make sense; why is it an array in the first place? What are you trying to do?– Pointy
Nov 19 at 16:02
why you define getRatingValues as an array but use it as an integer? thats not clear :) And you can save many space if you write your code dynamical like: getRating.classList.add('rating-' + getRatingValues);
– episch
Nov 19 at 16:04
+=
also does something different when the operators are strings (andtextContent
is a string).– Sergiu Paraschiv
Nov 19 at 16:04
Why are you adding a string to an array?
– epascarello
Nov 19 at 16:05
I am collecting the values from the DIV with class
my-ratings
, I am then transforming that value into a CSS Class, so if the DIV has text in it that is 3, I generate a css class called ratings-3 and apply it to the DIV– Takuhii
Nov 19 at 16:23