How to get values from a defaultdict using a new combination of keys?
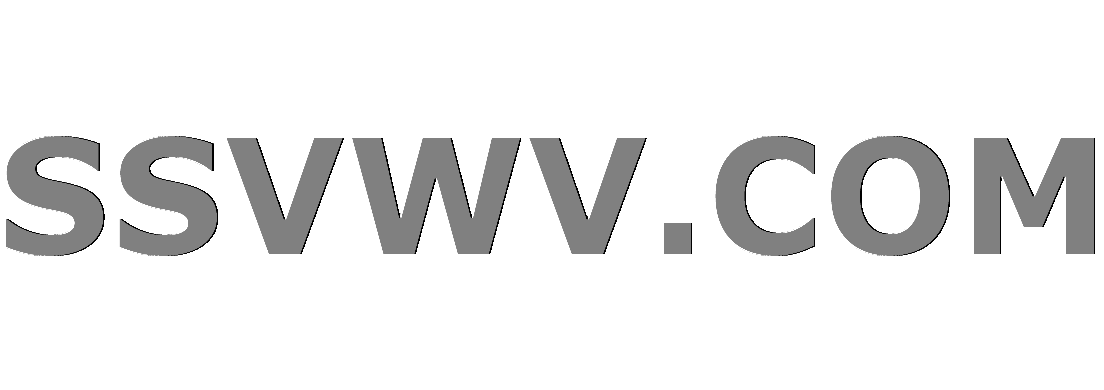
Multi tool use
up vote
-1
down vote
favorite
I have the following defaultdict:
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
From this dict, I need to append the values to a list using the following combination of keys:
order = [("b","b"), ("b","a"), ("b","c"), ("a","b", ("a","a"), ("a","c"), ("c","b"), ("c","a"), ("c","c")]
If the keys combination are the same (for example ("a", "a")), the value should be zero. So the final result should look something like the following:
result = [0, 0.1, 0.3, 0.1, 0, 0.2, 0.3, 0.2, 0]
I have tried the following
dist =
for index1, member1 in enumerate(order):
curr = dictA.get(member1, {})
for index2, member2 in enumerate(order):
val = curr.get(member2)
if member1 == member2:
val = 0
if member2 not in curr:
val = None
dist.append(val)
But obviously this is not working as intended. Can someone help me with this? Thank you
python defaultdict
add a comment |
up vote
-1
down vote
favorite
I have the following defaultdict:
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
From this dict, I need to append the values to a list using the following combination of keys:
order = [("b","b"), ("b","a"), ("b","c"), ("a","b", ("a","a"), ("a","c"), ("c","b"), ("c","a"), ("c","c")]
If the keys combination are the same (for example ("a", "a")), the value should be zero. So the final result should look something like the following:
result = [0, 0.1, 0.3, 0.1, 0, 0.2, 0.3, 0.2, 0]
I have tried the following
dist =
for index1, member1 in enumerate(order):
curr = dictA.get(member1, {})
for index2, member2 in enumerate(order):
val = curr.get(member2)
if member1 == member2:
val = 0
if member2 not in curr:
val = None
dist.append(val)
But obviously this is not working as intended. Can someone help me with this? Thank you
python defaultdict
1
IsdictA
arbitrarily deep or does it just have 1 level?
– slider
Nov 19 at 23:01
It only has one level
– Giratina86
Nov 19 at 23:51
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I have the following defaultdict:
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
From this dict, I need to append the values to a list using the following combination of keys:
order = [("b","b"), ("b","a"), ("b","c"), ("a","b", ("a","a"), ("a","c"), ("c","b"), ("c","a"), ("c","c")]
If the keys combination are the same (for example ("a", "a")), the value should be zero. So the final result should look something like the following:
result = [0, 0.1, 0.3, 0.1, 0, 0.2, 0.3, 0.2, 0]
I have tried the following
dist =
for index1, member1 in enumerate(order):
curr = dictA.get(member1, {})
for index2, member2 in enumerate(order):
val = curr.get(member2)
if member1 == member2:
val = 0
if member2 not in curr:
val = None
dist.append(val)
But obviously this is not working as intended. Can someone help me with this? Thank you
python defaultdict
I have the following defaultdict:
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
From this dict, I need to append the values to a list using the following combination of keys:
order = [("b","b"), ("b","a"), ("b","c"), ("a","b", ("a","a"), ("a","c"), ("c","b"), ("c","a"), ("c","c")]
If the keys combination are the same (for example ("a", "a")), the value should be zero. So the final result should look something like the following:
result = [0, 0.1, 0.3, 0.1, 0, 0.2, 0.3, 0.2, 0]
I have tried the following
dist =
for index1, member1 in enumerate(order):
curr = dictA.get(member1, {})
for index2, member2 in enumerate(order):
val = curr.get(member2)
if member1 == member2:
val = 0
if member2 not in curr:
val = None
dist.append(val)
But obviously this is not working as intended. Can someone help me with this? Thank you
python defaultdict
python defaultdict
asked Nov 19 at 22:58
Giratina86
31
31
1
IsdictA
arbitrarily deep or does it just have 1 level?
– slider
Nov 19 at 23:01
It only has one level
– Giratina86
Nov 19 at 23:51
add a comment |
1
IsdictA
arbitrarily deep or does it just have 1 level?
– slider
Nov 19 at 23:01
It only has one level
– Giratina86
Nov 19 at 23:51
1
1
Is
dictA
arbitrarily deep or does it just have 1 level?– slider
Nov 19 at 23:01
Is
dictA
arbitrarily deep or does it just have 1 level?– slider
Nov 19 at 23:01
It only has one level
– Giratina86
Nov 19 at 23:51
It only has one level
– Giratina86
Nov 19 at 23:51
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
You could do something like this:
def get(d, first, second):
return d.get(second, {}).get(first, 0.0)
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
order = [("b", "b"), ("b", "a"), ("b", "c"), ("a", "b"), ("a", "a"), ("a", "c"), ("c", "b"), ("c", "a"), ("c", "c")]
result = [get(dictA, first, second) or get(dictA, second, first) for first, second in order]
print(result)
Output
[0.0, 0.1, 0.3, 0.1, 0.0, 0.2, 0.3, 0.2, 0.0]
Or the less pythonic alternative:
def get(d, first, second):
return d.get(second, {}).get(first, 0.0)
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
order = [("b", "b"), ("b", "a"), ("b", "c"), ("a", "b"), ("a", "a"), ("a", "c"), ("c", "b"), ("c", "a"), ("c", "c")]
result =
for first, second in order:
value = get(dictA, first, second)
if value:
result.append(value)
else:
value = get(dictA, second, first)
result.append(value)
print(result)
Output
[0.0, 0.1, 0.3, 0.1, 0.0, 0.2, 0.3, 0.2, 0.0]
This worked like a charm! I probably tried the less pythonic approach. But I see my mistake now.
– Giratina86
Nov 19 at 23:51
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
You could do something like this:
def get(d, first, second):
return d.get(second, {}).get(first, 0.0)
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
order = [("b", "b"), ("b", "a"), ("b", "c"), ("a", "b"), ("a", "a"), ("a", "c"), ("c", "b"), ("c", "a"), ("c", "c")]
result = [get(dictA, first, second) or get(dictA, second, first) for first, second in order]
print(result)
Output
[0.0, 0.1, 0.3, 0.1, 0.0, 0.2, 0.3, 0.2, 0.0]
Or the less pythonic alternative:
def get(d, first, second):
return d.get(second, {}).get(first, 0.0)
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
order = [("b", "b"), ("b", "a"), ("b", "c"), ("a", "b"), ("a", "a"), ("a", "c"), ("c", "b"), ("c", "a"), ("c", "c")]
result =
for first, second in order:
value = get(dictA, first, second)
if value:
result.append(value)
else:
value = get(dictA, second, first)
result.append(value)
print(result)
Output
[0.0, 0.1, 0.3, 0.1, 0.0, 0.2, 0.3, 0.2, 0.0]
This worked like a charm! I probably tried the less pythonic approach. But I see my mistake now.
– Giratina86
Nov 19 at 23:51
add a comment |
up vote
0
down vote
accepted
You could do something like this:
def get(d, first, second):
return d.get(second, {}).get(first, 0.0)
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
order = [("b", "b"), ("b", "a"), ("b", "c"), ("a", "b"), ("a", "a"), ("a", "c"), ("c", "b"), ("c", "a"), ("c", "c")]
result = [get(dictA, first, second) or get(dictA, second, first) for first, second in order]
print(result)
Output
[0.0, 0.1, 0.3, 0.1, 0.0, 0.2, 0.3, 0.2, 0.0]
Or the less pythonic alternative:
def get(d, first, second):
return d.get(second, {}).get(first, 0.0)
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
order = [("b", "b"), ("b", "a"), ("b", "c"), ("a", "b"), ("a", "a"), ("a", "c"), ("c", "b"), ("c", "a"), ("c", "c")]
result =
for first, second in order:
value = get(dictA, first, second)
if value:
result.append(value)
else:
value = get(dictA, second, first)
result.append(value)
print(result)
Output
[0.0, 0.1, 0.3, 0.1, 0.0, 0.2, 0.3, 0.2, 0.0]
This worked like a charm! I probably tried the less pythonic approach. But I see my mistake now.
– Giratina86
Nov 19 at 23:51
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
You could do something like this:
def get(d, first, second):
return d.get(second, {}).get(first, 0.0)
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
order = [("b", "b"), ("b", "a"), ("b", "c"), ("a", "b"), ("a", "a"), ("a", "c"), ("c", "b"), ("c", "a"), ("c", "c")]
result = [get(dictA, first, second) or get(dictA, second, first) for first, second in order]
print(result)
Output
[0.0, 0.1, 0.3, 0.1, 0.0, 0.2, 0.3, 0.2, 0.0]
Or the less pythonic alternative:
def get(d, first, second):
return d.get(second, {}).get(first, 0.0)
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
order = [("b", "b"), ("b", "a"), ("b", "c"), ("a", "b"), ("a", "a"), ("a", "c"), ("c", "b"), ("c", "a"), ("c", "c")]
result =
for first, second in order:
value = get(dictA, first, second)
if value:
result.append(value)
else:
value = get(dictA, second, first)
result.append(value)
print(result)
Output
[0.0, 0.1, 0.3, 0.1, 0.0, 0.2, 0.3, 0.2, 0.0]
You could do something like this:
def get(d, first, second):
return d.get(second, {}).get(first, 0.0)
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
order = [("b", "b"), ("b", "a"), ("b", "c"), ("a", "b"), ("a", "a"), ("a", "c"), ("c", "b"), ("c", "a"), ("c", "c")]
result = [get(dictA, first, second) or get(dictA, second, first) for first, second in order]
print(result)
Output
[0.0, 0.1, 0.3, 0.1, 0.0, 0.2, 0.3, 0.2, 0.0]
Or the less pythonic alternative:
def get(d, first, second):
return d.get(second, {}).get(first, 0.0)
dictA = {"a": {"b": 0.1, "c": 0.2}, "b": {"c": 0.3}}
order = [("b", "b"), ("b", "a"), ("b", "c"), ("a", "b"), ("a", "a"), ("a", "c"), ("c", "b"), ("c", "a"), ("c", "c")]
result =
for first, second in order:
value = get(dictA, first, second)
if value:
result.append(value)
else:
value = get(dictA, second, first)
result.append(value)
print(result)
Output
[0.0, 0.1, 0.3, 0.1, 0.0, 0.2, 0.3, 0.2, 0.0]
answered Nov 19 at 23:20


Daniel Mesejo
9,5331923
9,5331923
This worked like a charm! I probably tried the less pythonic approach. But I see my mistake now.
– Giratina86
Nov 19 at 23:51
add a comment |
This worked like a charm! I probably tried the less pythonic approach. But I see my mistake now.
– Giratina86
Nov 19 at 23:51
This worked like a charm! I probably tried the less pythonic approach. But I see my mistake now.
– Giratina86
Nov 19 at 23:51
This worked like a charm! I probably tried the less pythonic approach. But I see my mistake now.
– Giratina86
Nov 19 at 23:51
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53383845%2fhow-to-get-values-from-a-defaultdict-using-a-new-combination-of-keys%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
az FDx3jhCz iYyD9D KKF4VkToKlaeEb9MFjAfelH8uGLKoVheSMWsWAA6CZRmH9W
1
Is
dictA
arbitrarily deep or does it just have 1 level?– slider
Nov 19 at 23:01
It only has one level
– Giratina86
Nov 19 at 23:51