Add custom button on Mapbox's navigation view
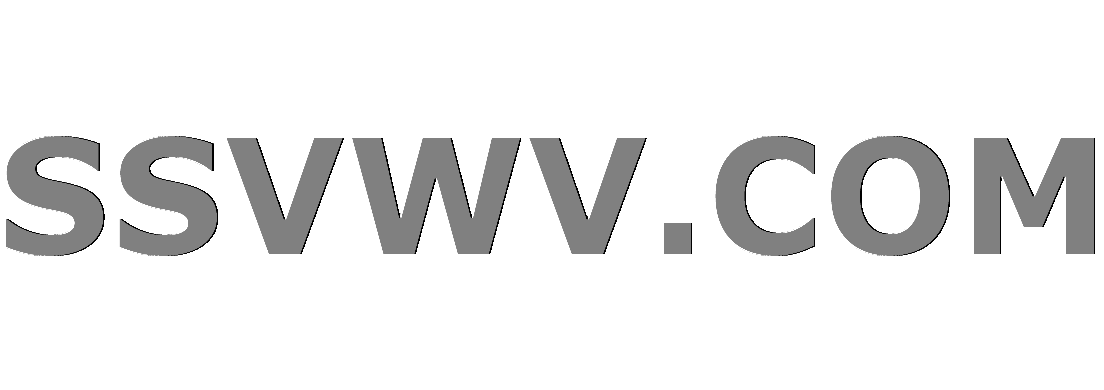
Multi tool use
I am using Mapbox to allow users to navigate between locations. I am using basic navigation feature as stated in the documentation (https://www.mapbox.com/ios-sdk/navigation/examples/basic/). This gives me the navigation view as below.
I would like to add a custom button at the bottom of the view. For this, I tried the following code.
Directions.shared.calculate(options) { (waypoints, routes, error) in
guard let route = routes?.first else {
self.showAlertMessage("No possible routes detected")
return
}
self.mapNavigationViewController = NavigationViewController(for: route)
self.mapNavigationViewController.delegate = self
self.present(self.mapNavigationViewController, animated: true, completion: {
let customButton = UIButton()
customButton.setTitle("Press", for: .normal)
customButton.translatesAutoresizingMaskIntoConstraints = false
customButton.backgroundColor = .blue
customButton.contentEdgeInsets = UIEdgeInsets(top: 10, left: 20, bottom: 10, right: 20)
self.mapNavigationViewController.view.addSubview(customButton)
customButton.bottomAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
customButton.leftAnchor.constraint(equalTo: self.mapNavigationViewController.view.leftAnchor, constant: 0).isActive = true
customButton.rightAnchor.constraint(equalTo: self.mapNavigationViewController.view.rightAnchor, constant: 0).isActive = true
self.mapNavigationViewController.view.setNeedsLayout()
})
}
With this, I got the button as below.
Now, the next thing to be done is to shift the mapbox's view so that its bottom aligns with the custom button's top. How can I achieve this?
ios swift autolayout mapbox nslayoutconstraint
add a comment |
I am using Mapbox to allow users to navigate between locations. I am using basic navigation feature as stated in the documentation (https://www.mapbox.com/ios-sdk/navigation/examples/basic/). This gives me the navigation view as below.
I would like to add a custom button at the bottom of the view. For this, I tried the following code.
Directions.shared.calculate(options) { (waypoints, routes, error) in
guard let route = routes?.first else {
self.showAlertMessage("No possible routes detected")
return
}
self.mapNavigationViewController = NavigationViewController(for: route)
self.mapNavigationViewController.delegate = self
self.present(self.mapNavigationViewController, animated: true, completion: {
let customButton = UIButton()
customButton.setTitle("Press", for: .normal)
customButton.translatesAutoresizingMaskIntoConstraints = false
customButton.backgroundColor = .blue
customButton.contentEdgeInsets = UIEdgeInsets(top: 10, left: 20, bottom: 10, right: 20)
self.mapNavigationViewController.view.addSubview(customButton)
customButton.bottomAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
customButton.leftAnchor.constraint(equalTo: self.mapNavigationViewController.view.leftAnchor, constant: 0).isActive = true
customButton.rightAnchor.constraint(equalTo: self.mapNavigationViewController.view.rightAnchor, constant: 0).isActive = true
self.mapNavigationViewController.view.setNeedsLayout()
})
}
With this, I got the button as below.
Now, the next thing to be done is to shift the mapbox's view so that its bottom aligns with the custom button's top. How can I achieve this?
ios swift autolayout mapbox nslayoutconstraint
1
autolayout constraints
– Daniel Springer
Nov 23 '18 at 6:36
I am facing difficulty in using auto layout constraints programmatically @DaniSpringer
– Sujal
Nov 23 '18 at 6:42
Instead ofcustomButton.bottomAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
. Why notcustomButton.topAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
– Daniel Springer
Nov 23 '18 at 6:44
The button does not display with the above substitution.
– Sujal
Nov 23 '18 at 7:24
Are you able to write code to affect the constraints of the map? Or is that out of your control? If yes: set map top to safe are top, map bottom to button top, and button bottom to safe area bottom.
– Daniel Springer
Nov 23 '18 at 7:49
add a comment |
I am using Mapbox to allow users to navigate between locations. I am using basic navigation feature as stated in the documentation (https://www.mapbox.com/ios-sdk/navigation/examples/basic/). This gives me the navigation view as below.
I would like to add a custom button at the bottom of the view. For this, I tried the following code.
Directions.shared.calculate(options) { (waypoints, routes, error) in
guard let route = routes?.first else {
self.showAlertMessage("No possible routes detected")
return
}
self.mapNavigationViewController = NavigationViewController(for: route)
self.mapNavigationViewController.delegate = self
self.present(self.mapNavigationViewController, animated: true, completion: {
let customButton = UIButton()
customButton.setTitle("Press", for: .normal)
customButton.translatesAutoresizingMaskIntoConstraints = false
customButton.backgroundColor = .blue
customButton.contentEdgeInsets = UIEdgeInsets(top: 10, left: 20, bottom: 10, right: 20)
self.mapNavigationViewController.view.addSubview(customButton)
customButton.bottomAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
customButton.leftAnchor.constraint(equalTo: self.mapNavigationViewController.view.leftAnchor, constant: 0).isActive = true
customButton.rightAnchor.constraint(equalTo: self.mapNavigationViewController.view.rightAnchor, constant: 0).isActive = true
self.mapNavigationViewController.view.setNeedsLayout()
})
}
With this, I got the button as below.
Now, the next thing to be done is to shift the mapbox's view so that its bottom aligns with the custom button's top. How can I achieve this?
ios swift autolayout mapbox nslayoutconstraint
I am using Mapbox to allow users to navigate between locations. I am using basic navigation feature as stated in the documentation (https://www.mapbox.com/ios-sdk/navigation/examples/basic/). This gives me the navigation view as below.
I would like to add a custom button at the bottom of the view. For this, I tried the following code.
Directions.shared.calculate(options) { (waypoints, routes, error) in
guard let route = routes?.first else {
self.showAlertMessage("No possible routes detected")
return
}
self.mapNavigationViewController = NavigationViewController(for: route)
self.mapNavigationViewController.delegate = self
self.present(self.mapNavigationViewController, animated: true, completion: {
let customButton = UIButton()
customButton.setTitle("Press", for: .normal)
customButton.translatesAutoresizingMaskIntoConstraints = false
customButton.backgroundColor = .blue
customButton.contentEdgeInsets = UIEdgeInsets(top: 10, left: 20, bottom: 10, right: 20)
self.mapNavigationViewController.view.addSubview(customButton)
customButton.bottomAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
customButton.leftAnchor.constraint(equalTo: self.mapNavigationViewController.view.leftAnchor, constant: 0).isActive = true
customButton.rightAnchor.constraint(equalTo: self.mapNavigationViewController.view.rightAnchor, constant: 0).isActive = true
self.mapNavigationViewController.view.setNeedsLayout()
})
}
With this, I got the button as below.
Now, the next thing to be done is to shift the mapbox's view so that its bottom aligns with the custom button's top. How can I achieve this?
ios swift autolayout mapbox nslayoutconstraint
ios swift autolayout mapbox nslayoutconstraint
asked Nov 23 '18 at 6:35
SujalSujal
467413
467413
1
autolayout constraints
– Daniel Springer
Nov 23 '18 at 6:36
I am facing difficulty in using auto layout constraints programmatically @DaniSpringer
– Sujal
Nov 23 '18 at 6:42
Instead ofcustomButton.bottomAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
. Why notcustomButton.topAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
– Daniel Springer
Nov 23 '18 at 6:44
The button does not display with the above substitution.
– Sujal
Nov 23 '18 at 7:24
Are you able to write code to affect the constraints of the map? Or is that out of your control? If yes: set map top to safe are top, map bottom to button top, and button bottom to safe area bottom.
– Daniel Springer
Nov 23 '18 at 7:49
add a comment |
1
autolayout constraints
– Daniel Springer
Nov 23 '18 at 6:36
I am facing difficulty in using auto layout constraints programmatically @DaniSpringer
– Sujal
Nov 23 '18 at 6:42
Instead ofcustomButton.bottomAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
. Why notcustomButton.topAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
– Daniel Springer
Nov 23 '18 at 6:44
The button does not display with the above substitution.
– Sujal
Nov 23 '18 at 7:24
Are you able to write code to affect the constraints of the map? Or is that out of your control? If yes: set map top to safe are top, map bottom to button top, and button bottom to safe area bottom.
– Daniel Springer
Nov 23 '18 at 7:49
1
1
autolayout constraints
– Daniel Springer
Nov 23 '18 at 6:36
autolayout constraints
– Daniel Springer
Nov 23 '18 at 6:36
I am facing difficulty in using auto layout constraints programmatically @DaniSpringer
– Sujal
Nov 23 '18 at 6:42
I am facing difficulty in using auto layout constraints programmatically @DaniSpringer
– Sujal
Nov 23 '18 at 6:42
Instead of
customButton.bottomAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
. Why not customButton.topAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
– Daniel Springer
Nov 23 '18 at 6:44
Instead of
customButton.bottomAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
. Why not customButton.topAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
– Daniel Springer
Nov 23 '18 at 6:44
The button does not display with the above substitution.
– Sujal
Nov 23 '18 at 7:24
The button does not display with the above substitution.
– Sujal
Nov 23 '18 at 7:24
Are you able to write code to affect the constraints of the map? Or is that out of your control? If yes: set map top to safe are top, map bottom to button top, and button bottom to safe area bottom.
– Daniel Springer
Nov 23 '18 at 7:49
Are you able to write code to affect the constraints of the map? Or is that out of your control? If yes: set map top to safe are top, map bottom to button top, and button bottom to safe area bottom.
– Daniel Springer
Nov 23 '18 at 7:49
add a comment |
2 Answers
2
active
oldest
votes
To achieve your objective, you will have to change the bottom constraint of the map view implemented in the library. So that you can set its bottom constraint equal to the top constraint of your customButton
See if you have access to the library's map view or its bottom constraint.
add a comment |
I am able to accomplish the task following this Mapbox documentation.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53441668%2fadd-custom-button-on-mapboxs-navigation-view%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
To achieve your objective, you will have to change the bottom constraint of the map view implemented in the library. So that you can set its bottom constraint equal to the top constraint of your customButton
See if you have access to the library's map view or its bottom constraint.
add a comment |
To achieve your objective, you will have to change the bottom constraint of the map view implemented in the library. So that you can set its bottom constraint equal to the top constraint of your customButton
See if you have access to the library's map view or its bottom constraint.
add a comment |
To achieve your objective, you will have to change the bottom constraint of the map view implemented in the library. So that you can set its bottom constraint equal to the top constraint of your customButton
See if you have access to the library's map view or its bottom constraint.
To achieve your objective, you will have to change the bottom constraint of the map view implemented in the library. So that you can set its bottom constraint equal to the top constraint of your customButton
See if you have access to the library's map view or its bottom constraint.
answered Nov 23 '18 at 7:18
DamonDamon
520318
520318
add a comment |
add a comment |
I am able to accomplish the task following this Mapbox documentation.
add a comment |
I am able to accomplish the task following this Mapbox documentation.
add a comment |
I am able to accomplish the task following this Mapbox documentation.
I am able to accomplish the task following this Mapbox documentation.
answered Nov 26 '18 at 10:20
SujalSujal
467413
467413
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53441668%2fadd-custom-button-on-mapboxs-navigation-view%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UNc8Z16EicDgEoMiA4oksdPrlWey22 JdBcIDWa94Lj,W1
1
autolayout constraints
– Daniel Springer
Nov 23 '18 at 6:36
I am facing difficulty in using auto layout constraints programmatically @DaniSpringer
– Sujal
Nov 23 '18 at 6:42
Instead of
customButton.bottomAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
. Why notcustomButton.topAnchor.constraint(equalTo: self.mapNavigationViewController.view.bottomAnchor, constant: 0).isActive = true
– Daniel Springer
Nov 23 '18 at 6:44
The button does not display with the above substitution.
– Sujal
Nov 23 '18 at 7:24
Are you able to write code to affect the constraints of the map? Or is that out of your control? If yes: set map top to safe are top, map bottom to button top, and button bottom to safe area bottom.
– Daniel Springer
Nov 23 '18 at 7:49