How can I prevent users from selecting multiple answers in my quiz?
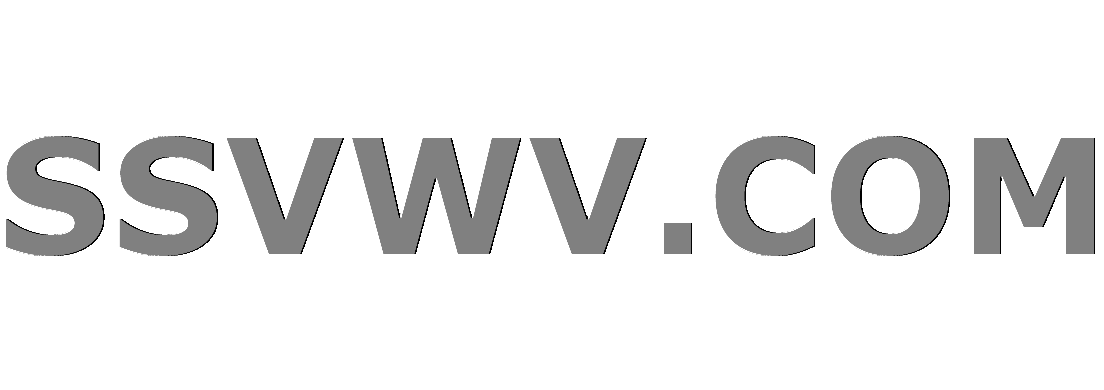
Multi tool use
The issue that I am having is that once a user selects an answer and then clicks 'Submit Answer' and receives their feedback they are able to continue to click around and select other answers before progressing onto the next question. How can I prevent a user from being able to do that once they submit one answer?
let score = 0;
let currentQuestion = 0;
let questions = [{
title: "At what age was Harry Potter when he received his Hogwarts letter?",
answers: ['7', '10', '11', '13'],
correct: 1
},
{
title: "Which is not a Hogwarts house?",
answers: ['Dunder Mifflin', 'Ravenclaw', 'Slytherin', 'Gryffindor'],
correct: 0
},
{
title: "Who teaches Transfiguration at Hogwarts?",
answers: ['Rubeus Hagrid', 'Albus Dumbledore', 'Severus Snape', 'Minerva McGonnagle'],
correct: 3
},
{
title: "Where is Hogwarts School for Witchcraft and Wizardry located?",
answers: ['France', 'USA', 'UK', 'New Zealand'],
correct: 2
},
{
title: "What form does Harry Potter's patronus charm take?",
answers: ['Stag', 'Eagle', 'Bear', 'Dragon'],
correct: 0
},
{
title: "What type of animal is Harry's pet?",
answers: ['Dog', 'Owl', 'Cat', 'Snake'],
correct: 1
},
{
title: "Who is not a member of the Order of the Phoenix?",
answers: ['Remus Lupin', 'Siruis Black', 'Lucious Malfoy', 'Severus Snape'],
correct: 2
},
{
title: "What is not a piece of the Deathly Hallows?",
answers: ['Elder Wand', 'Cloak of Invisibility', 'Resurrection Stone', 'Sword of Gryffindor'],
correct: 3
},
{
title: "In which house is Harry sorted into after arriving at Hogwarts?",
answers: ['Slytherin', 'Ravenclaw', 'Gryffindor', 'Hufflepuff'],
correct: 2
},
{
title: "What prevented Voldemort from being able to kill Harry Potter when he was a baby?",
answers: ['Love', 'Anger', 'Friendship', 'Joy'],
correct: 0
},
];
$(document).ready(function() {
$('.start a').click(function(e) {
e.preventDefault();
$('.start').hide();
$('.quiz').show();
showQuestion();
});
$('.quiz').on('click', 'button', function() {
$('.selected').removeClass('selected');
$(this).addClass('selected');
});
$('.quiz a.submit').click(function(e) {
e.preventDefault();
if ($('button.selected').length) {
let guess = parseInt($('button.selected').attr('id'));
checkAnswer(guess);
} else {
alert('Please select an answer');
}
});
$('.summary a').click(function(e) {
e.preventDefault();
restartQuiz();
});
});
function showQuestion() {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
$('.quiz div:nth-child(2)').append(`<button id="${i}">${question.answers[i]}</button>`);
}
showProgress();
}
function showIncorrectQuestion(guess) {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
let cls = i === question.correct ? "selected" : guess === i ? "wrong" : ""
$('.quiz div:nth-child(2)').append(`<button id="${i}" class="${cls}">${question.answers[i]}</button>`);
}
showProgress();
}
function checkAnswer(guess) {
let question = questions[currentQuestion];
if (question.correct === guess) {
if (!question.alreadyAnswered) {
score++;
}
showIsCorrect(true);
} else {
showIsCorrect(false);
showIncorrectQuestion(guess);
}
question.alreadyAnswered = true;
}
function showSummary() {
$('.quiz').hide();
$('.summary').show();
$('.summary p').text("Thank you for taking the quiz! Here's how you scored. You answered " + score + " out of " + questions.length + " correctly! Care to try again?")
}
function restartQuiz() {
questions.forEach(q => q.alreadyAnswered = false);
$('.summary').hide();
$('.quiz').show();
score = 0;
currentQuestion = 0;
showQuestion();
}
function showProgress() {
$('#currentQuestion').html(currentQuestion + " out of " + questions.length);
}
function showIsCorrect(isCorrect) {
var result = "Wrong";
if (isCorrect) {
result = "Correct";
}
$('#isCorrect').html(result);
$('.navigate').show();
$('.submit').hide();
}
$('.navigate').click(function() {
currentQuestion++;
if (currentQuestion >= questions.length) {
showSummary();
} else {
showQuestion();
}
$('.navigate').hide();
$('.submit').show();
$('#isCorrect').html('');
})
* {
padding: 0;
margin: 0;
box-sizing: border-box;
text-decoration: none;
text-align: center;
background-color: #837F73;
}
h1 {
font-family: 'Poor Story', cursive;
background-color: #950002;
padding: 60px;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
h2 {
font-family: 'Poor Story', cursive;
font-size: 30px;
padding: 60px;
background-color: #950002;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
p {
font-family: 'Poor Story', cursive;
background-color: #FFAB0D;
font-size: 20px;
font-weight: bold;
}
a {
border: 1px solid #222F5B;
padding: 10px;
background-color: #222F5B;
color: silver;
border-radius: 5px;
margin-top: 50px;
display: inline-block;
}
a:hover {
border: 1px solid #000000;
background-color: #000000;
color: #FCBF2B;
}
.quiz button {
cursor: pointer;
border: 1px solid;
display: inline-block;
width: 200px;
margin: 10px;
font-family: 'Poor Story', cursive;
font-size: 26px;
}
#currentQuestion {
color: #000000;
font-size: 18px;
font-family: 'Poor Story', cursive;
margin-top: 10px;
}
#isCorrect {
color: white;
font-family: 'Poor Story', cursive;
font-weight: bold;
font-size: 18px;
}
.quiz,
.summary {
display: none;
}
.quiz ul {
margin-top: 20px;
list-style: none;
padding: 0;
}
.selected {
background-color: #398C3F;
}
.wrong {
background-color: red;
}
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link href="https://fonts.googleapis.com/css?family=Poor+Story" rel="stylesheet">
<link href="style.css" rel="stylesheet" type="text/css" />
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Harry Potter Quiz</title>
</head>
<body>
<header role="banner">
<div class="start">
<h1>How Well Do You Know Harry Potter?</h1>
<a href="#">Start Quiz</a>
</div>
</header>
<main role="main">
<div class="quiz">
<h2>Question Title</h2>
<div>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
</div>
<a class="submit" href="#">Submit Answer</a>
<a class="navigate" style="display:none;" href="#">Next Question</a>
<div id="currentQuestion"></div>
<footer role="contentinfo">
<div id="isCorrect"></div>
</footer>
</div>
</main>
<div class="summary">
<h2>Results</h2>
<p>Thank you for taking the quiz! Here's how you scored. You answered x out of y correctly! Care to try again?</p>
<a href="#">Retake Quiz</a>
</div>
<script src="index.js"></script>
<!--jQuery script when using JSBin-->
<!--<script src="https://code.jquery.com/jquery-3.1.0.js"></script>-->
</body>
</html>
javascript jquery html css
add a comment |
The issue that I am having is that once a user selects an answer and then clicks 'Submit Answer' and receives their feedback they are able to continue to click around and select other answers before progressing onto the next question. How can I prevent a user from being able to do that once they submit one answer?
let score = 0;
let currentQuestion = 0;
let questions = [{
title: "At what age was Harry Potter when he received his Hogwarts letter?",
answers: ['7', '10', '11', '13'],
correct: 1
},
{
title: "Which is not a Hogwarts house?",
answers: ['Dunder Mifflin', 'Ravenclaw', 'Slytherin', 'Gryffindor'],
correct: 0
},
{
title: "Who teaches Transfiguration at Hogwarts?",
answers: ['Rubeus Hagrid', 'Albus Dumbledore', 'Severus Snape', 'Minerva McGonnagle'],
correct: 3
},
{
title: "Where is Hogwarts School for Witchcraft and Wizardry located?",
answers: ['France', 'USA', 'UK', 'New Zealand'],
correct: 2
},
{
title: "What form does Harry Potter's patronus charm take?",
answers: ['Stag', 'Eagle', 'Bear', 'Dragon'],
correct: 0
},
{
title: "What type of animal is Harry's pet?",
answers: ['Dog', 'Owl', 'Cat', 'Snake'],
correct: 1
},
{
title: "Who is not a member of the Order of the Phoenix?",
answers: ['Remus Lupin', 'Siruis Black', 'Lucious Malfoy', 'Severus Snape'],
correct: 2
},
{
title: "What is not a piece of the Deathly Hallows?",
answers: ['Elder Wand', 'Cloak of Invisibility', 'Resurrection Stone', 'Sword of Gryffindor'],
correct: 3
},
{
title: "In which house is Harry sorted into after arriving at Hogwarts?",
answers: ['Slytherin', 'Ravenclaw', 'Gryffindor', 'Hufflepuff'],
correct: 2
},
{
title: "What prevented Voldemort from being able to kill Harry Potter when he was a baby?",
answers: ['Love', 'Anger', 'Friendship', 'Joy'],
correct: 0
},
];
$(document).ready(function() {
$('.start a').click(function(e) {
e.preventDefault();
$('.start').hide();
$('.quiz').show();
showQuestion();
});
$('.quiz').on('click', 'button', function() {
$('.selected').removeClass('selected');
$(this).addClass('selected');
});
$('.quiz a.submit').click(function(e) {
e.preventDefault();
if ($('button.selected').length) {
let guess = parseInt($('button.selected').attr('id'));
checkAnswer(guess);
} else {
alert('Please select an answer');
}
});
$('.summary a').click(function(e) {
e.preventDefault();
restartQuiz();
});
});
function showQuestion() {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
$('.quiz div:nth-child(2)').append(`<button id="${i}">${question.answers[i]}</button>`);
}
showProgress();
}
function showIncorrectQuestion(guess) {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
let cls = i === question.correct ? "selected" : guess === i ? "wrong" : ""
$('.quiz div:nth-child(2)').append(`<button id="${i}" class="${cls}">${question.answers[i]}</button>`);
}
showProgress();
}
function checkAnswer(guess) {
let question = questions[currentQuestion];
if (question.correct === guess) {
if (!question.alreadyAnswered) {
score++;
}
showIsCorrect(true);
} else {
showIsCorrect(false);
showIncorrectQuestion(guess);
}
question.alreadyAnswered = true;
}
function showSummary() {
$('.quiz').hide();
$('.summary').show();
$('.summary p').text("Thank you for taking the quiz! Here's how you scored. You answered " + score + " out of " + questions.length + " correctly! Care to try again?")
}
function restartQuiz() {
questions.forEach(q => q.alreadyAnswered = false);
$('.summary').hide();
$('.quiz').show();
score = 0;
currentQuestion = 0;
showQuestion();
}
function showProgress() {
$('#currentQuestion').html(currentQuestion + " out of " + questions.length);
}
function showIsCorrect(isCorrect) {
var result = "Wrong";
if (isCorrect) {
result = "Correct";
}
$('#isCorrect').html(result);
$('.navigate').show();
$('.submit').hide();
}
$('.navigate').click(function() {
currentQuestion++;
if (currentQuestion >= questions.length) {
showSummary();
} else {
showQuestion();
}
$('.navigate').hide();
$('.submit').show();
$('#isCorrect').html('');
})
* {
padding: 0;
margin: 0;
box-sizing: border-box;
text-decoration: none;
text-align: center;
background-color: #837F73;
}
h1 {
font-family: 'Poor Story', cursive;
background-color: #950002;
padding: 60px;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
h2 {
font-family: 'Poor Story', cursive;
font-size: 30px;
padding: 60px;
background-color: #950002;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
p {
font-family: 'Poor Story', cursive;
background-color: #FFAB0D;
font-size: 20px;
font-weight: bold;
}
a {
border: 1px solid #222F5B;
padding: 10px;
background-color: #222F5B;
color: silver;
border-radius: 5px;
margin-top: 50px;
display: inline-block;
}
a:hover {
border: 1px solid #000000;
background-color: #000000;
color: #FCBF2B;
}
.quiz button {
cursor: pointer;
border: 1px solid;
display: inline-block;
width: 200px;
margin: 10px;
font-family: 'Poor Story', cursive;
font-size: 26px;
}
#currentQuestion {
color: #000000;
font-size: 18px;
font-family: 'Poor Story', cursive;
margin-top: 10px;
}
#isCorrect {
color: white;
font-family: 'Poor Story', cursive;
font-weight: bold;
font-size: 18px;
}
.quiz,
.summary {
display: none;
}
.quiz ul {
margin-top: 20px;
list-style: none;
padding: 0;
}
.selected {
background-color: #398C3F;
}
.wrong {
background-color: red;
}
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link href="https://fonts.googleapis.com/css?family=Poor+Story" rel="stylesheet">
<link href="style.css" rel="stylesheet" type="text/css" />
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Harry Potter Quiz</title>
</head>
<body>
<header role="banner">
<div class="start">
<h1>How Well Do You Know Harry Potter?</h1>
<a href="#">Start Quiz</a>
</div>
</header>
<main role="main">
<div class="quiz">
<h2>Question Title</h2>
<div>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
</div>
<a class="submit" href="#">Submit Answer</a>
<a class="navigate" style="display:none;" href="#">Next Question</a>
<div id="currentQuestion"></div>
<footer role="contentinfo">
<div id="isCorrect"></div>
</footer>
</div>
</main>
<div class="summary">
<h2>Results</h2>
<p>Thank you for taking the quiz! Here's how you scored. You answered x out of y correctly! Care to try again?</p>
<a href="#">Retake Quiz</a>
</div>
<script src="index.js"></script>
<!--jQuery script when using JSBin-->
<!--<script src="https://code.jquery.com/jquery-3.1.0.js"></script>-->
</body>
</html>
javascript jquery html css
3
Use radio elements instead of<button>
– Satpal
Nov 23 '18 at 6:39
2
Addingdisabled
prop after submit and removing on next question click
– siddhant sankhe
Nov 23 '18 at 6:44
<input type="radio" name= "choice" value="someValue1"> <input type="radio" name= "choice" value="someValue2">
something like this.
– Kunal Mukherjee
Nov 23 '18 at 6:45
document.getElementsByTagName("button").disabled = true; will disable the buttons. By the way, I had all the answers right. What do I win?
– Gerard
Nov 23 '18 at 6:48
add a comment |
The issue that I am having is that once a user selects an answer and then clicks 'Submit Answer' and receives their feedback they are able to continue to click around and select other answers before progressing onto the next question. How can I prevent a user from being able to do that once they submit one answer?
let score = 0;
let currentQuestion = 0;
let questions = [{
title: "At what age was Harry Potter when he received his Hogwarts letter?",
answers: ['7', '10', '11', '13'],
correct: 1
},
{
title: "Which is not a Hogwarts house?",
answers: ['Dunder Mifflin', 'Ravenclaw', 'Slytherin', 'Gryffindor'],
correct: 0
},
{
title: "Who teaches Transfiguration at Hogwarts?",
answers: ['Rubeus Hagrid', 'Albus Dumbledore', 'Severus Snape', 'Minerva McGonnagle'],
correct: 3
},
{
title: "Where is Hogwarts School for Witchcraft and Wizardry located?",
answers: ['France', 'USA', 'UK', 'New Zealand'],
correct: 2
},
{
title: "What form does Harry Potter's patronus charm take?",
answers: ['Stag', 'Eagle', 'Bear', 'Dragon'],
correct: 0
},
{
title: "What type of animal is Harry's pet?",
answers: ['Dog', 'Owl', 'Cat', 'Snake'],
correct: 1
},
{
title: "Who is not a member of the Order of the Phoenix?",
answers: ['Remus Lupin', 'Siruis Black', 'Lucious Malfoy', 'Severus Snape'],
correct: 2
},
{
title: "What is not a piece of the Deathly Hallows?",
answers: ['Elder Wand', 'Cloak of Invisibility', 'Resurrection Stone', 'Sword of Gryffindor'],
correct: 3
},
{
title: "In which house is Harry sorted into after arriving at Hogwarts?",
answers: ['Slytherin', 'Ravenclaw', 'Gryffindor', 'Hufflepuff'],
correct: 2
},
{
title: "What prevented Voldemort from being able to kill Harry Potter when he was a baby?",
answers: ['Love', 'Anger', 'Friendship', 'Joy'],
correct: 0
},
];
$(document).ready(function() {
$('.start a').click(function(e) {
e.preventDefault();
$('.start').hide();
$('.quiz').show();
showQuestion();
});
$('.quiz').on('click', 'button', function() {
$('.selected').removeClass('selected');
$(this).addClass('selected');
});
$('.quiz a.submit').click(function(e) {
e.preventDefault();
if ($('button.selected').length) {
let guess = parseInt($('button.selected').attr('id'));
checkAnswer(guess);
} else {
alert('Please select an answer');
}
});
$('.summary a').click(function(e) {
e.preventDefault();
restartQuiz();
});
});
function showQuestion() {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
$('.quiz div:nth-child(2)').append(`<button id="${i}">${question.answers[i]}</button>`);
}
showProgress();
}
function showIncorrectQuestion(guess) {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
let cls = i === question.correct ? "selected" : guess === i ? "wrong" : ""
$('.quiz div:nth-child(2)').append(`<button id="${i}" class="${cls}">${question.answers[i]}</button>`);
}
showProgress();
}
function checkAnswer(guess) {
let question = questions[currentQuestion];
if (question.correct === guess) {
if (!question.alreadyAnswered) {
score++;
}
showIsCorrect(true);
} else {
showIsCorrect(false);
showIncorrectQuestion(guess);
}
question.alreadyAnswered = true;
}
function showSummary() {
$('.quiz').hide();
$('.summary').show();
$('.summary p').text("Thank you for taking the quiz! Here's how you scored. You answered " + score + " out of " + questions.length + " correctly! Care to try again?")
}
function restartQuiz() {
questions.forEach(q => q.alreadyAnswered = false);
$('.summary').hide();
$('.quiz').show();
score = 0;
currentQuestion = 0;
showQuestion();
}
function showProgress() {
$('#currentQuestion').html(currentQuestion + " out of " + questions.length);
}
function showIsCorrect(isCorrect) {
var result = "Wrong";
if (isCorrect) {
result = "Correct";
}
$('#isCorrect').html(result);
$('.navigate').show();
$('.submit').hide();
}
$('.navigate').click(function() {
currentQuestion++;
if (currentQuestion >= questions.length) {
showSummary();
} else {
showQuestion();
}
$('.navigate').hide();
$('.submit').show();
$('#isCorrect').html('');
})
* {
padding: 0;
margin: 0;
box-sizing: border-box;
text-decoration: none;
text-align: center;
background-color: #837F73;
}
h1 {
font-family: 'Poor Story', cursive;
background-color: #950002;
padding: 60px;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
h2 {
font-family: 'Poor Story', cursive;
font-size: 30px;
padding: 60px;
background-color: #950002;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
p {
font-family: 'Poor Story', cursive;
background-color: #FFAB0D;
font-size: 20px;
font-weight: bold;
}
a {
border: 1px solid #222F5B;
padding: 10px;
background-color: #222F5B;
color: silver;
border-radius: 5px;
margin-top: 50px;
display: inline-block;
}
a:hover {
border: 1px solid #000000;
background-color: #000000;
color: #FCBF2B;
}
.quiz button {
cursor: pointer;
border: 1px solid;
display: inline-block;
width: 200px;
margin: 10px;
font-family: 'Poor Story', cursive;
font-size: 26px;
}
#currentQuestion {
color: #000000;
font-size: 18px;
font-family: 'Poor Story', cursive;
margin-top: 10px;
}
#isCorrect {
color: white;
font-family: 'Poor Story', cursive;
font-weight: bold;
font-size: 18px;
}
.quiz,
.summary {
display: none;
}
.quiz ul {
margin-top: 20px;
list-style: none;
padding: 0;
}
.selected {
background-color: #398C3F;
}
.wrong {
background-color: red;
}
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link href="https://fonts.googleapis.com/css?family=Poor+Story" rel="stylesheet">
<link href="style.css" rel="stylesheet" type="text/css" />
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Harry Potter Quiz</title>
</head>
<body>
<header role="banner">
<div class="start">
<h1>How Well Do You Know Harry Potter?</h1>
<a href="#">Start Quiz</a>
</div>
</header>
<main role="main">
<div class="quiz">
<h2>Question Title</h2>
<div>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
</div>
<a class="submit" href="#">Submit Answer</a>
<a class="navigate" style="display:none;" href="#">Next Question</a>
<div id="currentQuestion"></div>
<footer role="contentinfo">
<div id="isCorrect"></div>
</footer>
</div>
</main>
<div class="summary">
<h2>Results</h2>
<p>Thank you for taking the quiz! Here's how you scored. You answered x out of y correctly! Care to try again?</p>
<a href="#">Retake Quiz</a>
</div>
<script src="index.js"></script>
<!--jQuery script when using JSBin-->
<!--<script src="https://code.jquery.com/jquery-3.1.0.js"></script>-->
</body>
</html>
javascript jquery html css
The issue that I am having is that once a user selects an answer and then clicks 'Submit Answer' and receives their feedback they are able to continue to click around and select other answers before progressing onto the next question. How can I prevent a user from being able to do that once they submit one answer?
let score = 0;
let currentQuestion = 0;
let questions = [{
title: "At what age was Harry Potter when he received his Hogwarts letter?",
answers: ['7', '10', '11', '13'],
correct: 1
},
{
title: "Which is not a Hogwarts house?",
answers: ['Dunder Mifflin', 'Ravenclaw', 'Slytherin', 'Gryffindor'],
correct: 0
},
{
title: "Who teaches Transfiguration at Hogwarts?",
answers: ['Rubeus Hagrid', 'Albus Dumbledore', 'Severus Snape', 'Minerva McGonnagle'],
correct: 3
},
{
title: "Where is Hogwarts School for Witchcraft and Wizardry located?",
answers: ['France', 'USA', 'UK', 'New Zealand'],
correct: 2
},
{
title: "What form does Harry Potter's patronus charm take?",
answers: ['Stag', 'Eagle', 'Bear', 'Dragon'],
correct: 0
},
{
title: "What type of animal is Harry's pet?",
answers: ['Dog', 'Owl', 'Cat', 'Snake'],
correct: 1
},
{
title: "Who is not a member of the Order of the Phoenix?",
answers: ['Remus Lupin', 'Siruis Black', 'Lucious Malfoy', 'Severus Snape'],
correct: 2
},
{
title: "What is not a piece of the Deathly Hallows?",
answers: ['Elder Wand', 'Cloak of Invisibility', 'Resurrection Stone', 'Sword of Gryffindor'],
correct: 3
},
{
title: "In which house is Harry sorted into after arriving at Hogwarts?",
answers: ['Slytherin', 'Ravenclaw', 'Gryffindor', 'Hufflepuff'],
correct: 2
},
{
title: "What prevented Voldemort from being able to kill Harry Potter when he was a baby?",
answers: ['Love', 'Anger', 'Friendship', 'Joy'],
correct: 0
},
];
$(document).ready(function() {
$('.start a').click(function(e) {
e.preventDefault();
$('.start').hide();
$('.quiz').show();
showQuestion();
});
$('.quiz').on('click', 'button', function() {
$('.selected').removeClass('selected');
$(this).addClass('selected');
});
$('.quiz a.submit').click(function(e) {
e.preventDefault();
if ($('button.selected').length) {
let guess = parseInt($('button.selected').attr('id'));
checkAnswer(guess);
} else {
alert('Please select an answer');
}
});
$('.summary a').click(function(e) {
e.preventDefault();
restartQuiz();
});
});
function showQuestion() {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
$('.quiz div:nth-child(2)').append(`<button id="${i}">${question.answers[i]}</button>`);
}
showProgress();
}
function showIncorrectQuestion(guess) {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
let cls = i === question.correct ? "selected" : guess === i ? "wrong" : ""
$('.quiz div:nth-child(2)').append(`<button id="${i}" class="${cls}">${question.answers[i]}</button>`);
}
showProgress();
}
function checkAnswer(guess) {
let question = questions[currentQuestion];
if (question.correct === guess) {
if (!question.alreadyAnswered) {
score++;
}
showIsCorrect(true);
} else {
showIsCorrect(false);
showIncorrectQuestion(guess);
}
question.alreadyAnswered = true;
}
function showSummary() {
$('.quiz').hide();
$('.summary').show();
$('.summary p').text("Thank you for taking the quiz! Here's how you scored. You answered " + score + " out of " + questions.length + " correctly! Care to try again?")
}
function restartQuiz() {
questions.forEach(q => q.alreadyAnswered = false);
$('.summary').hide();
$('.quiz').show();
score = 0;
currentQuestion = 0;
showQuestion();
}
function showProgress() {
$('#currentQuestion').html(currentQuestion + " out of " + questions.length);
}
function showIsCorrect(isCorrect) {
var result = "Wrong";
if (isCorrect) {
result = "Correct";
}
$('#isCorrect').html(result);
$('.navigate').show();
$('.submit').hide();
}
$('.navigate').click(function() {
currentQuestion++;
if (currentQuestion >= questions.length) {
showSummary();
} else {
showQuestion();
}
$('.navigate').hide();
$('.submit').show();
$('#isCorrect').html('');
})
* {
padding: 0;
margin: 0;
box-sizing: border-box;
text-decoration: none;
text-align: center;
background-color: #837F73;
}
h1 {
font-family: 'Poor Story', cursive;
background-color: #950002;
padding: 60px;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
h2 {
font-family: 'Poor Story', cursive;
font-size: 30px;
padding: 60px;
background-color: #950002;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
p {
font-family: 'Poor Story', cursive;
background-color: #FFAB0D;
font-size: 20px;
font-weight: bold;
}
a {
border: 1px solid #222F5B;
padding: 10px;
background-color: #222F5B;
color: silver;
border-radius: 5px;
margin-top: 50px;
display: inline-block;
}
a:hover {
border: 1px solid #000000;
background-color: #000000;
color: #FCBF2B;
}
.quiz button {
cursor: pointer;
border: 1px solid;
display: inline-block;
width: 200px;
margin: 10px;
font-family: 'Poor Story', cursive;
font-size: 26px;
}
#currentQuestion {
color: #000000;
font-size: 18px;
font-family: 'Poor Story', cursive;
margin-top: 10px;
}
#isCorrect {
color: white;
font-family: 'Poor Story', cursive;
font-weight: bold;
font-size: 18px;
}
.quiz,
.summary {
display: none;
}
.quiz ul {
margin-top: 20px;
list-style: none;
padding: 0;
}
.selected {
background-color: #398C3F;
}
.wrong {
background-color: red;
}
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link href="https://fonts.googleapis.com/css?family=Poor+Story" rel="stylesheet">
<link href="style.css" rel="stylesheet" type="text/css" />
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Harry Potter Quiz</title>
</head>
<body>
<header role="banner">
<div class="start">
<h1>How Well Do You Know Harry Potter?</h1>
<a href="#">Start Quiz</a>
</div>
</header>
<main role="main">
<div class="quiz">
<h2>Question Title</h2>
<div>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
</div>
<a class="submit" href="#">Submit Answer</a>
<a class="navigate" style="display:none;" href="#">Next Question</a>
<div id="currentQuestion"></div>
<footer role="contentinfo">
<div id="isCorrect"></div>
</footer>
</div>
</main>
<div class="summary">
<h2>Results</h2>
<p>Thank you for taking the quiz! Here's how you scored. You answered x out of y correctly! Care to try again?</p>
<a href="#">Retake Quiz</a>
</div>
<script src="index.js"></script>
<!--jQuery script when using JSBin-->
<!--<script src="https://code.jquery.com/jquery-3.1.0.js"></script>-->
</body>
</html>
let score = 0;
let currentQuestion = 0;
let questions = [{
title: "At what age was Harry Potter when he received his Hogwarts letter?",
answers: ['7', '10', '11', '13'],
correct: 1
},
{
title: "Which is not a Hogwarts house?",
answers: ['Dunder Mifflin', 'Ravenclaw', 'Slytherin', 'Gryffindor'],
correct: 0
},
{
title: "Who teaches Transfiguration at Hogwarts?",
answers: ['Rubeus Hagrid', 'Albus Dumbledore', 'Severus Snape', 'Minerva McGonnagle'],
correct: 3
},
{
title: "Where is Hogwarts School for Witchcraft and Wizardry located?",
answers: ['France', 'USA', 'UK', 'New Zealand'],
correct: 2
},
{
title: "What form does Harry Potter's patronus charm take?",
answers: ['Stag', 'Eagle', 'Bear', 'Dragon'],
correct: 0
},
{
title: "What type of animal is Harry's pet?",
answers: ['Dog', 'Owl', 'Cat', 'Snake'],
correct: 1
},
{
title: "Who is not a member of the Order of the Phoenix?",
answers: ['Remus Lupin', 'Siruis Black', 'Lucious Malfoy', 'Severus Snape'],
correct: 2
},
{
title: "What is not a piece of the Deathly Hallows?",
answers: ['Elder Wand', 'Cloak of Invisibility', 'Resurrection Stone', 'Sword of Gryffindor'],
correct: 3
},
{
title: "In which house is Harry sorted into after arriving at Hogwarts?",
answers: ['Slytherin', 'Ravenclaw', 'Gryffindor', 'Hufflepuff'],
correct: 2
},
{
title: "What prevented Voldemort from being able to kill Harry Potter when he was a baby?",
answers: ['Love', 'Anger', 'Friendship', 'Joy'],
correct: 0
},
];
$(document).ready(function() {
$('.start a').click(function(e) {
e.preventDefault();
$('.start').hide();
$('.quiz').show();
showQuestion();
});
$('.quiz').on('click', 'button', function() {
$('.selected').removeClass('selected');
$(this).addClass('selected');
});
$('.quiz a.submit').click(function(e) {
e.preventDefault();
if ($('button.selected').length) {
let guess = parseInt($('button.selected').attr('id'));
checkAnswer(guess);
} else {
alert('Please select an answer');
}
});
$('.summary a').click(function(e) {
e.preventDefault();
restartQuiz();
});
});
function showQuestion() {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
$('.quiz div:nth-child(2)').append(`<button id="${i}">${question.answers[i]}</button>`);
}
showProgress();
}
function showIncorrectQuestion(guess) {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
let cls = i === question.correct ? "selected" : guess === i ? "wrong" : ""
$('.quiz div:nth-child(2)').append(`<button id="${i}" class="${cls}">${question.answers[i]}</button>`);
}
showProgress();
}
function checkAnswer(guess) {
let question = questions[currentQuestion];
if (question.correct === guess) {
if (!question.alreadyAnswered) {
score++;
}
showIsCorrect(true);
} else {
showIsCorrect(false);
showIncorrectQuestion(guess);
}
question.alreadyAnswered = true;
}
function showSummary() {
$('.quiz').hide();
$('.summary').show();
$('.summary p').text("Thank you for taking the quiz! Here's how you scored. You answered " + score + " out of " + questions.length + " correctly! Care to try again?")
}
function restartQuiz() {
questions.forEach(q => q.alreadyAnswered = false);
$('.summary').hide();
$('.quiz').show();
score = 0;
currentQuestion = 0;
showQuestion();
}
function showProgress() {
$('#currentQuestion').html(currentQuestion + " out of " + questions.length);
}
function showIsCorrect(isCorrect) {
var result = "Wrong";
if (isCorrect) {
result = "Correct";
}
$('#isCorrect').html(result);
$('.navigate').show();
$('.submit').hide();
}
$('.navigate').click(function() {
currentQuestion++;
if (currentQuestion >= questions.length) {
showSummary();
} else {
showQuestion();
}
$('.navigate').hide();
$('.submit').show();
$('#isCorrect').html('');
})
* {
padding: 0;
margin: 0;
box-sizing: border-box;
text-decoration: none;
text-align: center;
background-color: #837F73;
}
h1 {
font-family: 'Poor Story', cursive;
background-color: #950002;
padding: 60px;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
h2 {
font-family: 'Poor Story', cursive;
font-size: 30px;
padding: 60px;
background-color: #950002;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
p {
font-family: 'Poor Story', cursive;
background-color: #FFAB0D;
font-size: 20px;
font-weight: bold;
}
a {
border: 1px solid #222F5B;
padding: 10px;
background-color: #222F5B;
color: silver;
border-radius: 5px;
margin-top: 50px;
display: inline-block;
}
a:hover {
border: 1px solid #000000;
background-color: #000000;
color: #FCBF2B;
}
.quiz button {
cursor: pointer;
border: 1px solid;
display: inline-block;
width: 200px;
margin: 10px;
font-family: 'Poor Story', cursive;
font-size: 26px;
}
#currentQuestion {
color: #000000;
font-size: 18px;
font-family: 'Poor Story', cursive;
margin-top: 10px;
}
#isCorrect {
color: white;
font-family: 'Poor Story', cursive;
font-weight: bold;
font-size: 18px;
}
.quiz,
.summary {
display: none;
}
.quiz ul {
margin-top: 20px;
list-style: none;
padding: 0;
}
.selected {
background-color: #398C3F;
}
.wrong {
background-color: red;
}
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link href="https://fonts.googleapis.com/css?family=Poor+Story" rel="stylesheet">
<link href="style.css" rel="stylesheet" type="text/css" />
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Harry Potter Quiz</title>
</head>
<body>
<header role="banner">
<div class="start">
<h1>How Well Do You Know Harry Potter?</h1>
<a href="#">Start Quiz</a>
</div>
</header>
<main role="main">
<div class="quiz">
<h2>Question Title</h2>
<div>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
</div>
<a class="submit" href="#">Submit Answer</a>
<a class="navigate" style="display:none;" href="#">Next Question</a>
<div id="currentQuestion"></div>
<footer role="contentinfo">
<div id="isCorrect"></div>
</footer>
</div>
</main>
<div class="summary">
<h2>Results</h2>
<p>Thank you for taking the quiz! Here's how you scored. You answered x out of y correctly! Care to try again?</p>
<a href="#">Retake Quiz</a>
</div>
<script src="index.js"></script>
<!--jQuery script when using JSBin-->
<!--<script src="https://code.jquery.com/jquery-3.1.0.js"></script>-->
</body>
</html>
let score = 0;
let currentQuestion = 0;
let questions = [{
title: "At what age was Harry Potter when he received his Hogwarts letter?",
answers: ['7', '10', '11', '13'],
correct: 1
},
{
title: "Which is not a Hogwarts house?",
answers: ['Dunder Mifflin', 'Ravenclaw', 'Slytherin', 'Gryffindor'],
correct: 0
},
{
title: "Who teaches Transfiguration at Hogwarts?",
answers: ['Rubeus Hagrid', 'Albus Dumbledore', 'Severus Snape', 'Minerva McGonnagle'],
correct: 3
},
{
title: "Where is Hogwarts School for Witchcraft and Wizardry located?",
answers: ['France', 'USA', 'UK', 'New Zealand'],
correct: 2
},
{
title: "What form does Harry Potter's patronus charm take?",
answers: ['Stag', 'Eagle', 'Bear', 'Dragon'],
correct: 0
},
{
title: "What type of animal is Harry's pet?",
answers: ['Dog', 'Owl', 'Cat', 'Snake'],
correct: 1
},
{
title: "Who is not a member of the Order of the Phoenix?",
answers: ['Remus Lupin', 'Siruis Black', 'Lucious Malfoy', 'Severus Snape'],
correct: 2
},
{
title: "What is not a piece of the Deathly Hallows?",
answers: ['Elder Wand', 'Cloak of Invisibility', 'Resurrection Stone', 'Sword of Gryffindor'],
correct: 3
},
{
title: "In which house is Harry sorted into after arriving at Hogwarts?",
answers: ['Slytherin', 'Ravenclaw', 'Gryffindor', 'Hufflepuff'],
correct: 2
},
{
title: "What prevented Voldemort from being able to kill Harry Potter when he was a baby?",
answers: ['Love', 'Anger', 'Friendship', 'Joy'],
correct: 0
},
];
$(document).ready(function() {
$('.start a').click(function(e) {
e.preventDefault();
$('.start').hide();
$('.quiz').show();
showQuestion();
});
$('.quiz').on('click', 'button', function() {
$('.selected').removeClass('selected');
$(this).addClass('selected');
});
$('.quiz a.submit').click(function(e) {
e.preventDefault();
if ($('button.selected').length) {
let guess = parseInt($('button.selected').attr('id'));
checkAnswer(guess);
} else {
alert('Please select an answer');
}
});
$('.summary a').click(function(e) {
e.preventDefault();
restartQuiz();
});
});
function showQuestion() {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
$('.quiz div:nth-child(2)').append(`<button id="${i}">${question.answers[i]}</button>`);
}
showProgress();
}
function showIncorrectQuestion(guess) {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
let cls = i === question.correct ? "selected" : guess === i ? "wrong" : ""
$('.quiz div:nth-child(2)').append(`<button id="${i}" class="${cls}">${question.answers[i]}</button>`);
}
showProgress();
}
function checkAnswer(guess) {
let question = questions[currentQuestion];
if (question.correct === guess) {
if (!question.alreadyAnswered) {
score++;
}
showIsCorrect(true);
} else {
showIsCorrect(false);
showIncorrectQuestion(guess);
}
question.alreadyAnswered = true;
}
function showSummary() {
$('.quiz').hide();
$('.summary').show();
$('.summary p').text("Thank you for taking the quiz! Here's how you scored. You answered " + score + " out of " + questions.length + " correctly! Care to try again?")
}
function restartQuiz() {
questions.forEach(q => q.alreadyAnswered = false);
$('.summary').hide();
$('.quiz').show();
score = 0;
currentQuestion = 0;
showQuestion();
}
function showProgress() {
$('#currentQuestion').html(currentQuestion + " out of " + questions.length);
}
function showIsCorrect(isCorrect) {
var result = "Wrong";
if (isCorrect) {
result = "Correct";
}
$('#isCorrect').html(result);
$('.navigate').show();
$('.submit').hide();
}
$('.navigate').click(function() {
currentQuestion++;
if (currentQuestion >= questions.length) {
showSummary();
} else {
showQuestion();
}
$('.navigate').hide();
$('.submit').show();
$('#isCorrect').html('');
})
* {
padding: 0;
margin: 0;
box-sizing: border-box;
text-decoration: none;
text-align: center;
background-color: #837F73;
}
h1 {
font-family: 'Poor Story', cursive;
background-color: #950002;
padding: 60px;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
h2 {
font-family: 'Poor Story', cursive;
font-size: 30px;
padding: 60px;
background-color: #950002;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
p {
font-family: 'Poor Story', cursive;
background-color: #FFAB0D;
font-size: 20px;
font-weight: bold;
}
a {
border: 1px solid #222F5B;
padding: 10px;
background-color: #222F5B;
color: silver;
border-radius: 5px;
margin-top: 50px;
display: inline-block;
}
a:hover {
border: 1px solid #000000;
background-color: #000000;
color: #FCBF2B;
}
.quiz button {
cursor: pointer;
border: 1px solid;
display: inline-block;
width: 200px;
margin: 10px;
font-family: 'Poor Story', cursive;
font-size: 26px;
}
#currentQuestion {
color: #000000;
font-size: 18px;
font-family: 'Poor Story', cursive;
margin-top: 10px;
}
#isCorrect {
color: white;
font-family: 'Poor Story', cursive;
font-weight: bold;
font-size: 18px;
}
.quiz,
.summary {
display: none;
}
.quiz ul {
margin-top: 20px;
list-style: none;
padding: 0;
}
.selected {
background-color: #398C3F;
}
.wrong {
background-color: red;
}
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link href="https://fonts.googleapis.com/css?family=Poor+Story" rel="stylesheet">
<link href="style.css" rel="stylesheet" type="text/css" />
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Harry Potter Quiz</title>
</head>
<body>
<header role="banner">
<div class="start">
<h1>How Well Do You Know Harry Potter?</h1>
<a href="#">Start Quiz</a>
</div>
</header>
<main role="main">
<div class="quiz">
<h2>Question Title</h2>
<div>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
</div>
<a class="submit" href="#">Submit Answer</a>
<a class="navigate" style="display:none;" href="#">Next Question</a>
<div id="currentQuestion"></div>
<footer role="contentinfo">
<div id="isCorrect"></div>
</footer>
</div>
</main>
<div class="summary">
<h2>Results</h2>
<p>Thank you for taking the quiz! Here's how you scored. You answered x out of y correctly! Care to try again?</p>
<a href="#">Retake Quiz</a>
</div>
<script src="index.js"></script>
<!--jQuery script when using JSBin-->
<!--<script src="https://code.jquery.com/jquery-3.1.0.js"></script>-->
</body>
</html>
javascript jquery html css
javascript jquery html css
asked Nov 23 '18 at 6:38


Hungry MooseHungry Moose
494
494
3
Use radio elements instead of<button>
– Satpal
Nov 23 '18 at 6:39
2
Addingdisabled
prop after submit and removing on next question click
– siddhant sankhe
Nov 23 '18 at 6:44
<input type="radio" name= "choice" value="someValue1"> <input type="radio" name= "choice" value="someValue2">
something like this.
– Kunal Mukherjee
Nov 23 '18 at 6:45
document.getElementsByTagName("button").disabled = true; will disable the buttons. By the way, I had all the answers right. What do I win?
– Gerard
Nov 23 '18 at 6:48
add a comment |
3
Use radio elements instead of<button>
– Satpal
Nov 23 '18 at 6:39
2
Addingdisabled
prop after submit and removing on next question click
– siddhant sankhe
Nov 23 '18 at 6:44
<input type="radio" name= "choice" value="someValue1"> <input type="radio" name= "choice" value="someValue2">
something like this.
– Kunal Mukherjee
Nov 23 '18 at 6:45
document.getElementsByTagName("button").disabled = true; will disable the buttons. By the way, I had all the answers right. What do I win?
– Gerard
Nov 23 '18 at 6:48
3
3
Use radio elements instead of
<button>
– Satpal
Nov 23 '18 at 6:39
Use radio elements instead of
<button>
– Satpal
Nov 23 '18 at 6:39
2
2
Adding
disabled
prop after submit and removing on next question click– siddhant sankhe
Nov 23 '18 at 6:44
Adding
disabled
prop after submit and removing on next question click– siddhant sankhe
Nov 23 '18 at 6:44
<input type="radio" name= "choice" value="someValue1"> <input type="radio" name= "choice" value="someValue2">
something like this.– Kunal Mukherjee
Nov 23 '18 at 6:45
<input type="radio" name= "choice" value="someValue1"> <input type="radio" name= "choice" value="someValue2">
something like this.– Kunal Mukherjee
Nov 23 '18 at 6:45
document.getElementsByTagName("button").disabled = true; will disable the buttons. By the way, I had all the answers right. What do I win?
– Gerard
Nov 23 '18 at 6:48
document.getElementsByTagName("button").disabled = true; will disable the buttons. By the way, I had all the answers right. What do I win?
– Gerard
Nov 23 '18 at 6:48
add a comment |
3 Answers
3
active
oldest
votes
I have declared a variable called buttonClickable
and used it as a flag on every button click. when the user submits the answer, buttonclickable turns false and when ever you are rendering a new question buttonclickable turns true.
let score = 0;
let currentQuestion = 0;
let buttonClickable = true;
let questions = [{
title: "At what age was Harry Potter when he received his Hogwarts letter?",
answers: ['7', '10', '11', '13'],
correct: 1
},
{
title: "Which is not a Hogwarts house?",
answers: ['Dunder Mifflin', 'Ravenclaw', 'Slytherin', 'Gryffindor'],
correct: 0
},
{
title: "Who teaches Transfiguration at Hogwarts?",
answers: ['Rubeus Hagrid', 'Albus Dumbledore', 'Severus Snape', 'Minerva McGonnagle'],
correct: 3
},
{
title: "Where is Hogwarts School for Witchcraft and Wizardry located?",
answers: ['France', 'USA', 'UK', 'New Zealand'],
correct: 2
},
{
title: "What form does Harry Potter's patronus charm take?",
answers: ['Stag', 'Eagle', 'Bear', 'Dragon'],
correct: 0
},
{
title: "What type of animal is Harry's pet?",
answers: ['Dog', 'Owl', 'Cat', 'Snake'],
correct: 1
},
{
title: "Who is not a member of the Order of the Phoenix?",
answers: ['Remus Lupin', 'Siruis Black', 'Lucious Malfoy', 'Severus Snape'],
correct: 2
},
{
title: "What is not a piece of the Deathly Hallows?",
answers: ['Elder Wand', 'Cloak of Invisibility', 'Resurrection Stone', 'Sword of Gryffindor'],
correct: 3
},
{
title: "In which house is Harry sorted into after arriving at Hogwarts?",
answers: ['Slytherin', 'Ravenclaw', 'Gryffindor', 'Hufflepuff'],
correct: 2
},
{
title: "What prevented Voldemort from being able to kill Harry Potter when he was a baby?",
answers: ['Love', 'Anger', 'Friendship', 'Joy'],
correct: 0
},
];
$(document).ready(function() {
$('.start a').click(function(e) {
e.preventDefault();
$('.start').hide();
$('.quiz').show();
showQuestion();
});
$('.quiz').on('click', 'button', function() {
if(!buttonClickable) return;
$('.selected').removeClass('selected');
$(this).addClass('selected');
});
$('.quiz a.submit').click(function(e) {
e.preventDefault();
if ($('button.selected').length) {
let guess = parseInt($('button.selected').attr('id'));
checkAnswer(guess);
} else {
alert('Please select an answer');
}
});
$('.summary a').click(function(e) {
e.preventDefault();
restartQuiz();
});
});
function showQuestion() {
buttonClickable = true;
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
$('.quiz div:nth-child(2)').append(`<button id="${i}">${question.answers[i]}</button>`);
}
showProgress();
}
function showIncorrectQuestion(guess) {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
let cls = i === question.correct ? "selected" : guess === i ? "wrong" : ""
$('.quiz div:nth-child(2)').append(`<button id="${i}" class="${cls}">${question.answers[i]}</button>`);
}
showProgress();
}
function checkAnswer(guess) {
buttonClickable = false;
let question = questions[currentQuestion];
if (question.correct === guess) {
if (!question.alreadyAnswered) {
score++;
}
showIsCorrect(true);
} else {
showIsCorrect(false);
showIncorrectQuestion(guess);
}
question.alreadyAnswered = true;
}
function showSummary() {
$('.quiz').hide();
$('.summary').show();
$('.summary p').text("Thank you for taking the quiz! Here's how you scored. You answered " + score + " out of " + questions.length + " correctly! Care to try again?")
}
function restartQuiz() {
questions.forEach(q => q.alreadyAnswered = false);
$('.summary').hide();
$('.quiz').show();
score = 0;
currentQuestion = 0;
showQuestion();
}
function showProgress() {
$('#currentQuestion').html(currentQuestion + " out of " + questions.length);
}
function showIsCorrect(isCorrect) {
var result = "Wrong";
if (isCorrect) {
result = "Correct";
}
$('#isCorrect').html(result);
$('.navigate').show();
$('.submit').hide();
}
$('.navigate').click(function() {
currentQuestion++;
if (currentQuestion >= questions.length) {
showSummary();
} else {
showQuestion();
}
$('.navigate').hide();
$('.submit').show();
$('#isCorrect').html('');
})
* {
padding: 0;
margin: 0;
box-sizing: border-box;
text-decoration: none;
text-align: center;
background-color: #837F73;
}
h1 {
font-family: 'Poor Story', cursive;
background-color: #950002;
padding: 60px;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
h2 {
font-family: 'Poor Story', cursive;
font-size: 30px;
padding: 60px;
background-color: #950002;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
p {
font-family: 'Poor Story', cursive;
background-color: #FFAB0D;
font-size: 20px;
font-weight: bold;
}
a {
border: 1px solid #222F5B;
padding: 10px;
background-color: #222F5B;
color: silver;
border-radius: 5px;
margin-top: 50px;
display: inline-block;
}
a:hover {
border: 1px solid #000000;
background-color: #000000;
color: #FCBF2B;
}
.quiz button {
cursor: pointer;
border: 1px solid;
display: inline-block;
width: 200px;
margin: 10px;
font-family: 'Poor Story', cursive;
font-size: 26px;
}
#currentQuestion {
color: #000000;
font-size: 18px;
font-family: 'Poor Story', cursive;
margin-top: 10px;
}
#isCorrect {
color: white;
font-family: 'Poor Story', cursive;
font-weight: bold;
font-size: 18px;
}
.quiz,
.summary {
display: none;
}
.quiz ul {
margin-top: 20px;
list-style: none;
padding: 0;
}
.selected {
background-color: #398C3F;
}
.wrong {
background-color: red;
}
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link href="https://fonts.googleapis.com/css?family=Poor+Story" rel="stylesheet">
<link href="style.css" rel="stylesheet" type="text/css" />
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Harry Potter Quiz</title>
</head>
<body>
<header role="banner">
<div class="start">
<h1>How Well Do You Know Harry Potter?</h1>
<a href="#">Start Quiz</a>
</div>
</header>
<main role="main">
<div class="quiz">
<h2>Question Title</h2>
<div>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
</div>
<a class="submit" href="#">Submit Answer</a>
<a class="navigate" style="display:none;" href="#">Next Question</a>
<div id="currentQuestion"></div>
<footer role="contentinfo">
<div id="isCorrect"></div>
</footer>
</div>
</main>
<div class="summary">
<h2>Results</h2>
<p>Thank you for taking the quiz! Here's how you scored. You answered x out of y correctly! Care to try again?</p>
<a href="#">Retake Quiz</a>
</div>
<script src="index.js"></script>
<!--jQuery script when using JSBin-->
<!--<script src="https://code.jquery.com/jquery-3.1.0.js"></script>-->
</body>
</html>
Thank you so much, I really appreciate your help!
– Hungry Moose
Nov 23 '18 at 7:08
I'm glad it helped! cheers :)
– new_user
Nov 23 '18 at 7:15
add a comment |
Having skimmed through your JS, I'm assuming that the feedback shows on the same page as the question, rather than a new page resulting from submitting it to a server.
When you display the feedback, add a disabled="disabled"
property to the item in question, so that your user can't change it. Alternately, hide the selection and display an <output>
element: <output>Your Answer: USA<br />Correct Answer: UK</output>
add a comment |
disable All button after checking answers.
$('button').prop('disabled', true);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53441712%2fhow-can-i-prevent-users-from-selecting-multiple-answers-in-my-quiz%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
I have declared a variable called buttonClickable
and used it as a flag on every button click. when the user submits the answer, buttonclickable turns false and when ever you are rendering a new question buttonclickable turns true.
let score = 0;
let currentQuestion = 0;
let buttonClickable = true;
let questions = [{
title: "At what age was Harry Potter when he received his Hogwarts letter?",
answers: ['7', '10', '11', '13'],
correct: 1
},
{
title: "Which is not a Hogwarts house?",
answers: ['Dunder Mifflin', 'Ravenclaw', 'Slytherin', 'Gryffindor'],
correct: 0
},
{
title: "Who teaches Transfiguration at Hogwarts?",
answers: ['Rubeus Hagrid', 'Albus Dumbledore', 'Severus Snape', 'Minerva McGonnagle'],
correct: 3
},
{
title: "Where is Hogwarts School for Witchcraft and Wizardry located?",
answers: ['France', 'USA', 'UK', 'New Zealand'],
correct: 2
},
{
title: "What form does Harry Potter's patronus charm take?",
answers: ['Stag', 'Eagle', 'Bear', 'Dragon'],
correct: 0
},
{
title: "What type of animal is Harry's pet?",
answers: ['Dog', 'Owl', 'Cat', 'Snake'],
correct: 1
},
{
title: "Who is not a member of the Order of the Phoenix?",
answers: ['Remus Lupin', 'Siruis Black', 'Lucious Malfoy', 'Severus Snape'],
correct: 2
},
{
title: "What is not a piece of the Deathly Hallows?",
answers: ['Elder Wand', 'Cloak of Invisibility', 'Resurrection Stone', 'Sword of Gryffindor'],
correct: 3
},
{
title: "In which house is Harry sorted into after arriving at Hogwarts?",
answers: ['Slytherin', 'Ravenclaw', 'Gryffindor', 'Hufflepuff'],
correct: 2
},
{
title: "What prevented Voldemort from being able to kill Harry Potter when he was a baby?",
answers: ['Love', 'Anger', 'Friendship', 'Joy'],
correct: 0
},
];
$(document).ready(function() {
$('.start a').click(function(e) {
e.preventDefault();
$('.start').hide();
$('.quiz').show();
showQuestion();
});
$('.quiz').on('click', 'button', function() {
if(!buttonClickable) return;
$('.selected').removeClass('selected');
$(this).addClass('selected');
});
$('.quiz a.submit').click(function(e) {
e.preventDefault();
if ($('button.selected').length) {
let guess = parseInt($('button.selected').attr('id'));
checkAnswer(guess);
} else {
alert('Please select an answer');
}
});
$('.summary a').click(function(e) {
e.preventDefault();
restartQuiz();
});
});
function showQuestion() {
buttonClickable = true;
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
$('.quiz div:nth-child(2)').append(`<button id="${i}">${question.answers[i]}</button>`);
}
showProgress();
}
function showIncorrectQuestion(guess) {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
let cls = i === question.correct ? "selected" : guess === i ? "wrong" : ""
$('.quiz div:nth-child(2)').append(`<button id="${i}" class="${cls}">${question.answers[i]}</button>`);
}
showProgress();
}
function checkAnswer(guess) {
buttonClickable = false;
let question = questions[currentQuestion];
if (question.correct === guess) {
if (!question.alreadyAnswered) {
score++;
}
showIsCorrect(true);
} else {
showIsCorrect(false);
showIncorrectQuestion(guess);
}
question.alreadyAnswered = true;
}
function showSummary() {
$('.quiz').hide();
$('.summary').show();
$('.summary p').text("Thank you for taking the quiz! Here's how you scored. You answered " + score + " out of " + questions.length + " correctly! Care to try again?")
}
function restartQuiz() {
questions.forEach(q => q.alreadyAnswered = false);
$('.summary').hide();
$('.quiz').show();
score = 0;
currentQuestion = 0;
showQuestion();
}
function showProgress() {
$('#currentQuestion').html(currentQuestion + " out of " + questions.length);
}
function showIsCorrect(isCorrect) {
var result = "Wrong";
if (isCorrect) {
result = "Correct";
}
$('#isCorrect').html(result);
$('.navigate').show();
$('.submit').hide();
}
$('.navigate').click(function() {
currentQuestion++;
if (currentQuestion >= questions.length) {
showSummary();
} else {
showQuestion();
}
$('.navigate').hide();
$('.submit').show();
$('#isCorrect').html('');
})
* {
padding: 0;
margin: 0;
box-sizing: border-box;
text-decoration: none;
text-align: center;
background-color: #837F73;
}
h1 {
font-family: 'Poor Story', cursive;
background-color: #950002;
padding: 60px;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
h2 {
font-family: 'Poor Story', cursive;
font-size: 30px;
padding: 60px;
background-color: #950002;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
p {
font-family: 'Poor Story', cursive;
background-color: #FFAB0D;
font-size: 20px;
font-weight: bold;
}
a {
border: 1px solid #222F5B;
padding: 10px;
background-color: #222F5B;
color: silver;
border-radius: 5px;
margin-top: 50px;
display: inline-block;
}
a:hover {
border: 1px solid #000000;
background-color: #000000;
color: #FCBF2B;
}
.quiz button {
cursor: pointer;
border: 1px solid;
display: inline-block;
width: 200px;
margin: 10px;
font-family: 'Poor Story', cursive;
font-size: 26px;
}
#currentQuestion {
color: #000000;
font-size: 18px;
font-family: 'Poor Story', cursive;
margin-top: 10px;
}
#isCorrect {
color: white;
font-family: 'Poor Story', cursive;
font-weight: bold;
font-size: 18px;
}
.quiz,
.summary {
display: none;
}
.quiz ul {
margin-top: 20px;
list-style: none;
padding: 0;
}
.selected {
background-color: #398C3F;
}
.wrong {
background-color: red;
}
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link href="https://fonts.googleapis.com/css?family=Poor+Story" rel="stylesheet">
<link href="style.css" rel="stylesheet" type="text/css" />
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Harry Potter Quiz</title>
</head>
<body>
<header role="banner">
<div class="start">
<h1>How Well Do You Know Harry Potter?</h1>
<a href="#">Start Quiz</a>
</div>
</header>
<main role="main">
<div class="quiz">
<h2>Question Title</h2>
<div>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
</div>
<a class="submit" href="#">Submit Answer</a>
<a class="navigate" style="display:none;" href="#">Next Question</a>
<div id="currentQuestion"></div>
<footer role="contentinfo">
<div id="isCorrect"></div>
</footer>
</div>
</main>
<div class="summary">
<h2>Results</h2>
<p>Thank you for taking the quiz! Here's how you scored. You answered x out of y correctly! Care to try again?</p>
<a href="#">Retake Quiz</a>
</div>
<script src="index.js"></script>
<!--jQuery script when using JSBin-->
<!--<script src="https://code.jquery.com/jquery-3.1.0.js"></script>-->
</body>
</html>
Thank you so much, I really appreciate your help!
– Hungry Moose
Nov 23 '18 at 7:08
I'm glad it helped! cheers :)
– new_user
Nov 23 '18 at 7:15
add a comment |
I have declared a variable called buttonClickable
and used it as a flag on every button click. when the user submits the answer, buttonclickable turns false and when ever you are rendering a new question buttonclickable turns true.
let score = 0;
let currentQuestion = 0;
let buttonClickable = true;
let questions = [{
title: "At what age was Harry Potter when he received his Hogwarts letter?",
answers: ['7', '10', '11', '13'],
correct: 1
},
{
title: "Which is not a Hogwarts house?",
answers: ['Dunder Mifflin', 'Ravenclaw', 'Slytherin', 'Gryffindor'],
correct: 0
},
{
title: "Who teaches Transfiguration at Hogwarts?",
answers: ['Rubeus Hagrid', 'Albus Dumbledore', 'Severus Snape', 'Minerva McGonnagle'],
correct: 3
},
{
title: "Where is Hogwarts School for Witchcraft and Wizardry located?",
answers: ['France', 'USA', 'UK', 'New Zealand'],
correct: 2
},
{
title: "What form does Harry Potter's patronus charm take?",
answers: ['Stag', 'Eagle', 'Bear', 'Dragon'],
correct: 0
},
{
title: "What type of animal is Harry's pet?",
answers: ['Dog', 'Owl', 'Cat', 'Snake'],
correct: 1
},
{
title: "Who is not a member of the Order of the Phoenix?",
answers: ['Remus Lupin', 'Siruis Black', 'Lucious Malfoy', 'Severus Snape'],
correct: 2
},
{
title: "What is not a piece of the Deathly Hallows?",
answers: ['Elder Wand', 'Cloak of Invisibility', 'Resurrection Stone', 'Sword of Gryffindor'],
correct: 3
},
{
title: "In which house is Harry sorted into after arriving at Hogwarts?",
answers: ['Slytherin', 'Ravenclaw', 'Gryffindor', 'Hufflepuff'],
correct: 2
},
{
title: "What prevented Voldemort from being able to kill Harry Potter when he was a baby?",
answers: ['Love', 'Anger', 'Friendship', 'Joy'],
correct: 0
},
];
$(document).ready(function() {
$('.start a').click(function(e) {
e.preventDefault();
$('.start').hide();
$('.quiz').show();
showQuestion();
});
$('.quiz').on('click', 'button', function() {
if(!buttonClickable) return;
$('.selected').removeClass('selected');
$(this).addClass('selected');
});
$('.quiz a.submit').click(function(e) {
e.preventDefault();
if ($('button.selected').length) {
let guess = parseInt($('button.selected').attr('id'));
checkAnswer(guess);
} else {
alert('Please select an answer');
}
});
$('.summary a').click(function(e) {
e.preventDefault();
restartQuiz();
});
});
function showQuestion() {
buttonClickable = true;
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
$('.quiz div:nth-child(2)').append(`<button id="${i}">${question.answers[i]}</button>`);
}
showProgress();
}
function showIncorrectQuestion(guess) {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
let cls = i === question.correct ? "selected" : guess === i ? "wrong" : ""
$('.quiz div:nth-child(2)').append(`<button id="${i}" class="${cls}">${question.answers[i]}</button>`);
}
showProgress();
}
function checkAnswer(guess) {
buttonClickable = false;
let question = questions[currentQuestion];
if (question.correct === guess) {
if (!question.alreadyAnswered) {
score++;
}
showIsCorrect(true);
} else {
showIsCorrect(false);
showIncorrectQuestion(guess);
}
question.alreadyAnswered = true;
}
function showSummary() {
$('.quiz').hide();
$('.summary').show();
$('.summary p').text("Thank you for taking the quiz! Here's how you scored. You answered " + score + " out of " + questions.length + " correctly! Care to try again?")
}
function restartQuiz() {
questions.forEach(q => q.alreadyAnswered = false);
$('.summary').hide();
$('.quiz').show();
score = 0;
currentQuestion = 0;
showQuestion();
}
function showProgress() {
$('#currentQuestion').html(currentQuestion + " out of " + questions.length);
}
function showIsCorrect(isCorrect) {
var result = "Wrong";
if (isCorrect) {
result = "Correct";
}
$('#isCorrect').html(result);
$('.navigate').show();
$('.submit').hide();
}
$('.navigate').click(function() {
currentQuestion++;
if (currentQuestion >= questions.length) {
showSummary();
} else {
showQuestion();
}
$('.navigate').hide();
$('.submit').show();
$('#isCorrect').html('');
})
* {
padding: 0;
margin: 0;
box-sizing: border-box;
text-decoration: none;
text-align: center;
background-color: #837F73;
}
h1 {
font-family: 'Poor Story', cursive;
background-color: #950002;
padding: 60px;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
h2 {
font-family: 'Poor Story', cursive;
font-size: 30px;
padding: 60px;
background-color: #950002;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
p {
font-family: 'Poor Story', cursive;
background-color: #FFAB0D;
font-size: 20px;
font-weight: bold;
}
a {
border: 1px solid #222F5B;
padding: 10px;
background-color: #222F5B;
color: silver;
border-radius: 5px;
margin-top: 50px;
display: inline-block;
}
a:hover {
border: 1px solid #000000;
background-color: #000000;
color: #FCBF2B;
}
.quiz button {
cursor: pointer;
border: 1px solid;
display: inline-block;
width: 200px;
margin: 10px;
font-family: 'Poor Story', cursive;
font-size: 26px;
}
#currentQuestion {
color: #000000;
font-size: 18px;
font-family: 'Poor Story', cursive;
margin-top: 10px;
}
#isCorrect {
color: white;
font-family: 'Poor Story', cursive;
font-weight: bold;
font-size: 18px;
}
.quiz,
.summary {
display: none;
}
.quiz ul {
margin-top: 20px;
list-style: none;
padding: 0;
}
.selected {
background-color: #398C3F;
}
.wrong {
background-color: red;
}
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link href="https://fonts.googleapis.com/css?family=Poor+Story" rel="stylesheet">
<link href="style.css" rel="stylesheet" type="text/css" />
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Harry Potter Quiz</title>
</head>
<body>
<header role="banner">
<div class="start">
<h1>How Well Do You Know Harry Potter?</h1>
<a href="#">Start Quiz</a>
</div>
</header>
<main role="main">
<div class="quiz">
<h2>Question Title</h2>
<div>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
</div>
<a class="submit" href="#">Submit Answer</a>
<a class="navigate" style="display:none;" href="#">Next Question</a>
<div id="currentQuestion"></div>
<footer role="contentinfo">
<div id="isCorrect"></div>
</footer>
</div>
</main>
<div class="summary">
<h2>Results</h2>
<p>Thank you for taking the quiz! Here's how you scored. You answered x out of y correctly! Care to try again?</p>
<a href="#">Retake Quiz</a>
</div>
<script src="index.js"></script>
<!--jQuery script when using JSBin-->
<!--<script src="https://code.jquery.com/jquery-3.1.0.js"></script>-->
</body>
</html>
Thank you so much, I really appreciate your help!
– Hungry Moose
Nov 23 '18 at 7:08
I'm glad it helped! cheers :)
– new_user
Nov 23 '18 at 7:15
add a comment |
I have declared a variable called buttonClickable
and used it as a flag on every button click. when the user submits the answer, buttonclickable turns false and when ever you are rendering a new question buttonclickable turns true.
let score = 0;
let currentQuestion = 0;
let buttonClickable = true;
let questions = [{
title: "At what age was Harry Potter when he received his Hogwarts letter?",
answers: ['7', '10', '11', '13'],
correct: 1
},
{
title: "Which is not a Hogwarts house?",
answers: ['Dunder Mifflin', 'Ravenclaw', 'Slytherin', 'Gryffindor'],
correct: 0
},
{
title: "Who teaches Transfiguration at Hogwarts?",
answers: ['Rubeus Hagrid', 'Albus Dumbledore', 'Severus Snape', 'Minerva McGonnagle'],
correct: 3
},
{
title: "Where is Hogwarts School for Witchcraft and Wizardry located?",
answers: ['France', 'USA', 'UK', 'New Zealand'],
correct: 2
},
{
title: "What form does Harry Potter's patronus charm take?",
answers: ['Stag', 'Eagle', 'Bear', 'Dragon'],
correct: 0
},
{
title: "What type of animal is Harry's pet?",
answers: ['Dog', 'Owl', 'Cat', 'Snake'],
correct: 1
},
{
title: "Who is not a member of the Order of the Phoenix?",
answers: ['Remus Lupin', 'Siruis Black', 'Lucious Malfoy', 'Severus Snape'],
correct: 2
},
{
title: "What is not a piece of the Deathly Hallows?",
answers: ['Elder Wand', 'Cloak of Invisibility', 'Resurrection Stone', 'Sword of Gryffindor'],
correct: 3
},
{
title: "In which house is Harry sorted into after arriving at Hogwarts?",
answers: ['Slytherin', 'Ravenclaw', 'Gryffindor', 'Hufflepuff'],
correct: 2
},
{
title: "What prevented Voldemort from being able to kill Harry Potter when he was a baby?",
answers: ['Love', 'Anger', 'Friendship', 'Joy'],
correct: 0
},
];
$(document).ready(function() {
$('.start a').click(function(e) {
e.preventDefault();
$('.start').hide();
$('.quiz').show();
showQuestion();
});
$('.quiz').on('click', 'button', function() {
if(!buttonClickable) return;
$('.selected').removeClass('selected');
$(this).addClass('selected');
});
$('.quiz a.submit').click(function(e) {
e.preventDefault();
if ($('button.selected').length) {
let guess = parseInt($('button.selected').attr('id'));
checkAnswer(guess);
} else {
alert('Please select an answer');
}
});
$('.summary a').click(function(e) {
e.preventDefault();
restartQuiz();
});
});
function showQuestion() {
buttonClickable = true;
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
$('.quiz div:nth-child(2)').append(`<button id="${i}">${question.answers[i]}</button>`);
}
showProgress();
}
function showIncorrectQuestion(guess) {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
let cls = i === question.correct ? "selected" : guess === i ? "wrong" : ""
$('.quiz div:nth-child(2)').append(`<button id="${i}" class="${cls}">${question.answers[i]}</button>`);
}
showProgress();
}
function checkAnswer(guess) {
buttonClickable = false;
let question = questions[currentQuestion];
if (question.correct === guess) {
if (!question.alreadyAnswered) {
score++;
}
showIsCorrect(true);
} else {
showIsCorrect(false);
showIncorrectQuestion(guess);
}
question.alreadyAnswered = true;
}
function showSummary() {
$('.quiz').hide();
$('.summary').show();
$('.summary p').text("Thank you for taking the quiz! Here's how you scored. You answered " + score + " out of " + questions.length + " correctly! Care to try again?")
}
function restartQuiz() {
questions.forEach(q => q.alreadyAnswered = false);
$('.summary').hide();
$('.quiz').show();
score = 0;
currentQuestion = 0;
showQuestion();
}
function showProgress() {
$('#currentQuestion').html(currentQuestion + " out of " + questions.length);
}
function showIsCorrect(isCorrect) {
var result = "Wrong";
if (isCorrect) {
result = "Correct";
}
$('#isCorrect').html(result);
$('.navigate').show();
$('.submit').hide();
}
$('.navigate').click(function() {
currentQuestion++;
if (currentQuestion >= questions.length) {
showSummary();
} else {
showQuestion();
}
$('.navigate').hide();
$('.submit').show();
$('#isCorrect').html('');
})
* {
padding: 0;
margin: 0;
box-sizing: border-box;
text-decoration: none;
text-align: center;
background-color: #837F73;
}
h1 {
font-family: 'Poor Story', cursive;
background-color: #950002;
padding: 60px;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
h2 {
font-family: 'Poor Story', cursive;
font-size: 30px;
padding: 60px;
background-color: #950002;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
p {
font-family: 'Poor Story', cursive;
background-color: #FFAB0D;
font-size: 20px;
font-weight: bold;
}
a {
border: 1px solid #222F5B;
padding: 10px;
background-color: #222F5B;
color: silver;
border-radius: 5px;
margin-top: 50px;
display: inline-block;
}
a:hover {
border: 1px solid #000000;
background-color: #000000;
color: #FCBF2B;
}
.quiz button {
cursor: pointer;
border: 1px solid;
display: inline-block;
width: 200px;
margin: 10px;
font-family: 'Poor Story', cursive;
font-size: 26px;
}
#currentQuestion {
color: #000000;
font-size: 18px;
font-family: 'Poor Story', cursive;
margin-top: 10px;
}
#isCorrect {
color: white;
font-family: 'Poor Story', cursive;
font-weight: bold;
font-size: 18px;
}
.quiz,
.summary {
display: none;
}
.quiz ul {
margin-top: 20px;
list-style: none;
padding: 0;
}
.selected {
background-color: #398C3F;
}
.wrong {
background-color: red;
}
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link href="https://fonts.googleapis.com/css?family=Poor+Story" rel="stylesheet">
<link href="style.css" rel="stylesheet" type="text/css" />
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Harry Potter Quiz</title>
</head>
<body>
<header role="banner">
<div class="start">
<h1>How Well Do You Know Harry Potter?</h1>
<a href="#">Start Quiz</a>
</div>
</header>
<main role="main">
<div class="quiz">
<h2>Question Title</h2>
<div>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
</div>
<a class="submit" href="#">Submit Answer</a>
<a class="navigate" style="display:none;" href="#">Next Question</a>
<div id="currentQuestion"></div>
<footer role="contentinfo">
<div id="isCorrect"></div>
</footer>
</div>
</main>
<div class="summary">
<h2>Results</h2>
<p>Thank you for taking the quiz! Here's how you scored. You answered x out of y correctly! Care to try again?</p>
<a href="#">Retake Quiz</a>
</div>
<script src="index.js"></script>
<!--jQuery script when using JSBin-->
<!--<script src="https://code.jquery.com/jquery-3.1.0.js"></script>-->
</body>
</html>
I have declared a variable called buttonClickable
and used it as a flag on every button click. when the user submits the answer, buttonclickable turns false and when ever you are rendering a new question buttonclickable turns true.
let score = 0;
let currentQuestion = 0;
let buttonClickable = true;
let questions = [{
title: "At what age was Harry Potter when he received his Hogwarts letter?",
answers: ['7', '10', '11', '13'],
correct: 1
},
{
title: "Which is not a Hogwarts house?",
answers: ['Dunder Mifflin', 'Ravenclaw', 'Slytherin', 'Gryffindor'],
correct: 0
},
{
title: "Who teaches Transfiguration at Hogwarts?",
answers: ['Rubeus Hagrid', 'Albus Dumbledore', 'Severus Snape', 'Minerva McGonnagle'],
correct: 3
},
{
title: "Where is Hogwarts School for Witchcraft and Wizardry located?",
answers: ['France', 'USA', 'UK', 'New Zealand'],
correct: 2
},
{
title: "What form does Harry Potter's patronus charm take?",
answers: ['Stag', 'Eagle', 'Bear', 'Dragon'],
correct: 0
},
{
title: "What type of animal is Harry's pet?",
answers: ['Dog', 'Owl', 'Cat', 'Snake'],
correct: 1
},
{
title: "Who is not a member of the Order of the Phoenix?",
answers: ['Remus Lupin', 'Siruis Black', 'Lucious Malfoy', 'Severus Snape'],
correct: 2
},
{
title: "What is not a piece of the Deathly Hallows?",
answers: ['Elder Wand', 'Cloak of Invisibility', 'Resurrection Stone', 'Sword of Gryffindor'],
correct: 3
},
{
title: "In which house is Harry sorted into after arriving at Hogwarts?",
answers: ['Slytherin', 'Ravenclaw', 'Gryffindor', 'Hufflepuff'],
correct: 2
},
{
title: "What prevented Voldemort from being able to kill Harry Potter when he was a baby?",
answers: ['Love', 'Anger', 'Friendship', 'Joy'],
correct: 0
},
];
$(document).ready(function() {
$('.start a').click(function(e) {
e.preventDefault();
$('.start').hide();
$('.quiz').show();
showQuestion();
});
$('.quiz').on('click', 'button', function() {
if(!buttonClickable) return;
$('.selected').removeClass('selected');
$(this).addClass('selected');
});
$('.quiz a.submit').click(function(e) {
e.preventDefault();
if ($('button.selected').length) {
let guess = parseInt($('button.selected').attr('id'));
checkAnswer(guess);
} else {
alert('Please select an answer');
}
});
$('.summary a').click(function(e) {
e.preventDefault();
restartQuiz();
});
});
function showQuestion() {
buttonClickable = true;
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
$('.quiz div:nth-child(2)').append(`<button id="${i}">${question.answers[i]}</button>`);
}
showProgress();
}
function showIncorrectQuestion(guess) {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
let cls = i === question.correct ? "selected" : guess === i ? "wrong" : ""
$('.quiz div:nth-child(2)').append(`<button id="${i}" class="${cls}">${question.answers[i]}</button>`);
}
showProgress();
}
function checkAnswer(guess) {
buttonClickable = false;
let question = questions[currentQuestion];
if (question.correct === guess) {
if (!question.alreadyAnswered) {
score++;
}
showIsCorrect(true);
} else {
showIsCorrect(false);
showIncorrectQuestion(guess);
}
question.alreadyAnswered = true;
}
function showSummary() {
$('.quiz').hide();
$('.summary').show();
$('.summary p').text("Thank you for taking the quiz! Here's how you scored. You answered " + score + " out of " + questions.length + " correctly! Care to try again?")
}
function restartQuiz() {
questions.forEach(q => q.alreadyAnswered = false);
$('.summary').hide();
$('.quiz').show();
score = 0;
currentQuestion = 0;
showQuestion();
}
function showProgress() {
$('#currentQuestion').html(currentQuestion + " out of " + questions.length);
}
function showIsCorrect(isCorrect) {
var result = "Wrong";
if (isCorrect) {
result = "Correct";
}
$('#isCorrect').html(result);
$('.navigate').show();
$('.submit').hide();
}
$('.navigate').click(function() {
currentQuestion++;
if (currentQuestion >= questions.length) {
showSummary();
} else {
showQuestion();
}
$('.navigate').hide();
$('.submit').show();
$('#isCorrect').html('');
})
* {
padding: 0;
margin: 0;
box-sizing: border-box;
text-decoration: none;
text-align: center;
background-color: #837F73;
}
h1 {
font-family: 'Poor Story', cursive;
background-color: #950002;
padding: 60px;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
h2 {
font-family: 'Poor Story', cursive;
font-size: 30px;
padding: 60px;
background-color: #950002;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
p {
font-family: 'Poor Story', cursive;
background-color: #FFAB0D;
font-size: 20px;
font-weight: bold;
}
a {
border: 1px solid #222F5B;
padding: 10px;
background-color: #222F5B;
color: silver;
border-radius: 5px;
margin-top: 50px;
display: inline-block;
}
a:hover {
border: 1px solid #000000;
background-color: #000000;
color: #FCBF2B;
}
.quiz button {
cursor: pointer;
border: 1px solid;
display: inline-block;
width: 200px;
margin: 10px;
font-family: 'Poor Story', cursive;
font-size: 26px;
}
#currentQuestion {
color: #000000;
font-size: 18px;
font-family: 'Poor Story', cursive;
margin-top: 10px;
}
#isCorrect {
color: white;
font-family: 'Poor Story', cursive;
font-weight: bold;
font-size: 18px;
}
.quiz,
.summary {
display: none;
}
.quiz ul {
margin-top: 20px;
list-style: none;
padding: 0;
}
.selected {
background-color: #398C3F;
}
.wrong {
background-color: red;
}
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link href="https://fonts.googleapis.com/css?family=Poor+Story" rel="stylesheet">
<link href="style.css" rel="stylesheet" type="text/css" />
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Harry Potter Quiz</title>
</head>
<body>
<header role="banner">
<div class="start">
<h1>How Well Do You Know Harry Potter?</h1>
<a href="#">Start Quiz</a>
</div>
</header>
<main role="main">
<div class="quiz">
<h2>Question Title</h2>
<div>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
</div>
<a class="submit" href="#">Submit Answer</a>
<a class="navigate" style="display:none;" href="#">Next Question</a>
<div id="currentQuestion"></div>
<footer role="contentinfo">
<div id="isCorrect"></div>
</footer>
</div>
</main>
<div class="summary">
<h2>Results</h2>
<p>Thank you for taking the quiz! Here's how you scored. You answered x out of y correctly! Care to try again?</p>
<a href="#">Retake Quiz</a>
</div>
<script src="index.js"></script>
<!--jQuery script when using JSBin-->
<!--<script src="https://code.jquery.com/jquery-3.1.0.js"></script>-->
</body>
</html>
let score = 0;
let currentQuestion = 0;
let buttonClickable = true;
let questions = [{
title: "At what age was Harry Potter when he received his Hogwarts letter?",
answers: ['7', '10', '11', '13'],
correct: 1
},
{
title: "Which is not a Hogwarts house?",
answers: ['Dunder Mifflin', 'Ravenclaw', 'Slytherin', 'Gryffindor'],
correct: 0
},
{
title: "Who teaches Transfiguration at Hogwarts?",
answers: ['Rubeus Hagrid', 'Albus Dumbledore', 'Severus Snape', 'Minerva McGonnagle'],
correct: 3
},
{
title: "Where is Hogwarts School for Witchcraft and Wizardry located?",
answers: ['France', 'USA', 'UK', 'New Zealand'],
correct: 2
},
{
title: "What form does Harry Potter's patronus charm take?",
answers: ['Stag', 'Eagle', 'Bear', 'Dragon'],
correct: 0
},
{
title: "What type of animal is Harry's pet?",
answers: ['Dog', 'Owl', 'Cat', 'Snake'],
correct: 1
},
{
title: "Who is not a member of the Order of the Phoenix?",
answers: ['Remus Lupin', 'Siruis Black', 'Lucious Malfoy', 'Severus Snape'],
correct: 2
},
{
title: "What is not a piece of the Deathly Hallows?",
answers: ['Elder Wand', 'Cloak of Invisibility', 'Resurrection Stone', 'Sword of Gryffindor'],
correct: 3
},
{
title: "In which house is Harry sorted into after arriving at Hogwarts?",
answers: ['Slytherin', 'Ravenclaw', 'Gryffindor', 'Hufflepuff'],
correct: 2
},
{
title: "What prevented Voldemort from being able to kill Harry Potter when he was a baby?",
answers: ['Love', 'Anger', 'Friendship', 'Joy'],
correct: 0
},
];
$(document).ready(function() {
$('.start a').click(function(e) {
e.preventDefault();
$('.start').hide();
$('.quiz').show();
showQuestion();
});
$('.quiz').on('click', 'button', function() {
if(!buttonClickable) return;
$('.selected').removeClass('selected');
$(this).addClass('selected');
});
$('.quiz a.submit').click(function(e) {
e.preventDefault();
if ($('button.selected').length) {
let guess = parseInt($('button.selected').attr('id'));
checkAnswer(guess);
} else {
alert('Please select an answer');
}
});
$('.summary a').click(function(e) {
e.preventDefault();
restartQuiz();
});
});
function showQuestion() {
buttonClickable = true;
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
$('.quiz div:nth-child(2)').append(`<button id="${i}">${question.answers[i]}</button>`);
}
showProgress();
}
function showIncorrectQuestion(guess) {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
let cls = i === question.correct ? "selected" : guess === i ? "wrong" : ""
$('.quiz div:nth-child(2)').append(`<button id="${i}" class="${cls}">${question.answers[i]}</button>`);
}
showProgress();
}
function checkAnswer(guess) {
buttonClickable = false;
let question = questions[currentQuestion];
if (question.correct === guess) {
if (!question.alreadyAnswered) {
score++;
}
showIsCorrect(true);
} else {
showIsCorrect(false);
showIncorrectQuestion(guess);
}
question.alreadyAnswered = true;
}
function showSummary() {
$('.quiz').hide();
$('.summary').show();
$('.summary p').text("Thank you for taking the quiz! Here's how you scored. You answered " + score + " out of " + questions.length + " correctly! Care to try again?")
}
function restartQuiz() {
questions.forEach(q => q.alreadyAnswered = false);
$('.summary').hide();
$('.quiz').show();
score = 0;
currentQuestion = 0;
showQuestion();
}
function showProgress() {
$('#currentQuestion').html(currentQuestion + " out of " + questions.length);
}
function showIsCorrect(isCorrect) {
var result = "Wrong";
if (isCorrect) {
result = "Correct";
}
$('#isCorrect').html(result);
$('.navigate').show();
$('.submit').hide();
}
$('.navigate').click(function() {
currentQuestion++;
if (currentQuestion >= questions.length) {
showSummary();
} else {
showQuestion();
}
$('.navigate').hide();
$('.submit').show();
$('#isCorrect').html('');
})
* {
padding: 0;
margin: 0;
box-sizing: border-box;
text-decoration: none;
text-align: center;
background-color: #837F73;
}
h1 {
font-family: 'Poor Story', cursive;
background-color: #950002;
padding: 60px;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
h2 {
font-family: 'Poor Story', cursive;
font-size: 30px;
padding: 60px;
background-color: #950002;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
p {
font-family: 'Poor Story', cursive;
background-color: #FFAB0D;
font-size: 20px;
font-weight: bold;
}
a {
border: 1px solid #222F5B;
padding: 10px;
background-color: #222F5B;
color: silver;
border-radius: 5px;
margin-top: 50px;
display: inline-block;
}
a:hover {
border: 1px solid #000000;
background-color: #000000;
color: #FCBF2B;
}
.quiz button {
cursor: pointer;
border: 1px solid;
display: inline-block;
width: 200px;
margin: 10px;
font-family: 'Poor Story', cursive;
font-size: 26px;
}
#currentQuestion {
color: #000000;
font-size: 18px;
font-family: 'Poor Story', cursive;
margin-top: 10px;
}
#isCorrect {
color: white;
font-family: 'Poor Story', cursive;
font-weight: bold;
font-size: 18px;
}
.quiz,
.summary {
display: none;
}
.quiz ul {
margin-top: 20px;
list-style: none;
padding: 0;
}
.selected {
background-color: #398C3F;
}
.wrong {
background-color: red;
}
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link href="https://fonts.googleapis.com/css?family=Poor+Story" rel="stylesheet">
<link href="style.css" rel="stylesheet" type="text/css" />
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Harry Potter Quiz</title>
</head>
<body>
<header role="banner">
<div class="start">
<h1>How Well Do You Know Harry Potter?</h1>
<a href="#">Start Quiz</a>
</div>
</header>
<main role="main">
<div class="quiz">
<h2>Question Title</h2>
<div>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
</div>
<a class="submit" href="#">Submit Answer</a>
<a class="navigate" style="display:none;" href="#">Next Question</a>
<div id="currentQuestion"></div>
<footer role="contentinfo">
<div id="isCorrect"></div>
</footer>
</div>
</main>
<div class="summary">
<h2>Results</h2>
<p>Thank you for taking the quiz! Here's how you scored. You answered x out of y correctly! Care to try again?</p>
<a href="#">Retake Quiz</a>
</div>
<script src="index.js"></script>
<!--jQuery script when using JSBin-->
<!--<script src="https://code.jquery.com/jquery-3.1.0.js"></script>-->
</body>
</html>
let score = 0;
let currentQuestion = 0;
let buttonClickable = true;
let questions = [{
title: "At what age was Harry Potter when he received his Hogwarts letter?",
answers: ['7', '10', '11', '13'],
correct: 1
},
{
title: "Which is not a Hogwarts house?",
answers: ['Dunder Mifflin', 'Ravenclaw', 'Slytherin', 'Gryffindor'],
correct: 0
},
{
title: "Who teaches Transfiguration at Hogwarts?",
answers: ['Rubeus Hagrid', 'Albus Dumbledore', 'Severus Snape', 'Minerva McGonnagle'],
correct: 3
},
{
title: "Where is Hogwarts School for Witchcraft and Wizardry located?",
answers: ['France', 'USA', 'UK', 'New Zealand'],
correct: 2
},
{
title: "What form does Harry Potter's patronus charm take?",
answers: ['Stag', 'Eagle', 'Bear', 'Dragon'],
correct: 0
},
{
title: "What type of animal is Harry's pet?",
answers: ['Dog', 'Owl', 'Cat', 'Snake'],
correct: 1
},
{
title: "Who is not a member of the Order of the Phoenix?",
answers: ['Remus Lupin', 'Siruis Black', 'Lucious Malfoy', 'Severus Snape'],
correct: 2
},
{
title: "What is not a piece of the Deathly Hallows?",
answers: ['Elder Wand', 'Cloak of Invisibility', 'Resurrection Stone', 'Sword of Gryffindor'],
correct: 3
},
{
title: "In which house is Harry sorted into after arriving at Hogwarts?",
answers: ['Slytherin', 'Ravenclaw', 'Gryffindor', 'Hufflepuff'],
correct: 2
},
{
title: "What prevented Voldemort from being able to kill Harry Potter when he was a baby?",
answers: ['Love', 'Anger', 'Friendship', 'Joy'],
correct: 0
},
];
$(document).ready(function() {
$('.start a').click(function(e) {
e.preventDefault();
$('.start').hide();
$('.quiz').show();
showQuestion();
});
$('.quiz').on('click', 'button', function() {
if(!buttonClickable) return;
$('.selected').removeClass('selected');
$(this).addClass('selected');
});
$('.quiz a.submit').click(function(e) {
e.preventDefault();
if ($('button.selected').length) {
let guess = parseInt($('button.selected').attr('id'));
checkAnswer(guess);
} else {
alert('Please select an answer');
}
});
$('.summary a').click(function(e) {
e.preventDefault();
restartQuiz();
});
});
function showQuestion() {
buttonClickable = true;
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
$('.quiz div:nth-child(2)').append(`<button id="${i}">${question.answers[i]}</button>`);
}
showProgress();
}
function showIncorrectQuestion(guess) {
let question = questions[currentQuestion];
$('.quiz h2').text(question.title);
$('.quiz div:nth-child(2)').html('');
for (var i = 0; i < question.answers.length; i++) {
let cls = i === question.correct ? "selected" : guess === i ? "wrong" : ""
$('.quiz div:nth-child(2)').append(`<button id="${i}" class="${cls}">${question.answers[i]}</button>`);
}
showProgress();
}
function checkAnswer(guess) {
buttonClickable = false;
let question = questions[currentQuestion];
if (question.correct === guess) {
if (!question.alreadyAnswered) {
score++;
}
showIsCorrect(true);
} else {
showIsCorrect(false);
showIncorrectQuestion(guess);
}
question.alreadyAnswered = true;
}
function showSummary() {
$('.quiz').hide();
$('.summary').show();
$('.summary p').text("Thank you for taking the quiz! Here's how you scored. You answered " + score + " out of " + questions.length + " correctly! Care to try again?")
}
function restartQuiz() {
questions.forEach(q => q.alreadyAnswered = false);
$('.summary').hide();
$('.quiz').show();
score = 0;
currentQuestion = 0;
showQuestion();
}
function showProgress() {
$('#currentQuestion').html(currentQuestion + " out of " + questions.length);
}
function showIsCorrect(isCorrect) {
var result = "Wrong";
if (isCorrect) {
result = "Correct";
}
$('#isCorrect').html(result);
$('.navigate').show();
$('.submit').hide();
}
$('.navigate').click(function() {
currentQuestion++;
if (currentQuestion >= questions.length) {
showSummary();
} else {
showQuestion();
}
$('.navigate').hide();
$('.submit').show();
$('#isCorrect').html('');
})
* {
padding: 0;
margin: 0;
box-sizing: border-box;
text-decoration: none;
text-align: center;
background-color: #837F73;
}
h1 {
font-family: 'Poor Story', cursive;
background-color: #950002;
padding: 60px;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
h2 {
font-family: 'Poor Story', cursive;
font-size: 30px;
padding: 60px;
background-color: #950002;
color: #FFAB0D;
text-shadow: -1px 0 black, 0 1px black, 1px 0 black, 0 -1px black;
}
p {
font-family: 'Poor Story', cursive;
background-color: #FFAB0D;
font-size: 20px;
font-weight: bold;
}
a {
border: 1px solid #222F5B;
padding: 10px;
background-color: #222F5B;
color: silver;
border-radius: 5px;
margin-top: 50px;
display: inline-block;
}
a:hover {
border: 1px solid #000000;
background-color: #000000;
color: #FCBF2B;
}
.quiz button {
cursor: pointer;
border: 1px solid;
display: inline-block;
width: 200px;
margin: 10px;
font-family: 'Poor Story', cursive;
font-size: 26px;
}
#currentQuestion {
color: #000000;
font-size: 18px;
font-family: 'Poor Story', cursive;
margin-top: 10px;
}
#isCorrect {
color: white;
font-family: 'Poor Story', cursive;
font-weight: bold;
font-size: 18px;
}
.quiz,
.summary {
display: none;
}
.quiz ul {
margin-top: 20px;
list-style: none;
padding: 0;
}
.selected {
background-color: #398C3F;
}
.wrong {
background-color: red;
}
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link href="https://fonts.googleapis.com/css?family=Poor+Story" rel="stylesheet">
<link href="style.css" rel="stylesheet" type="text/css" />
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Harry Potter Quiz</title>
</head>
<body>
<header role="banner">
<div class="start">
<h1>How Well Do You Know Harry Potter?</h1>
<a href="#">Start Quiz</a>
</div>
</header>
<main role="main">
<div class="quiz">
<h2>Question Title</h2>
<div>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
<button>Choice</button>
</div>
<a class="submit" href="#">Submit Answer</a>
<a class="navigate" style="display:none;" href="#">Next Question</a>
<div id="currentQuestion"></div>
<footer role="contentinfo">
<div id="isCorrect"></div>
</footer>
</div>
</main>
<div class="summary">
<h2>Results</h2>
<p>Thank you for taking the quiz! Here's how you scored. You answered x out of y correctly! Care to try again?</p>
<a href="#">Retake Quiz</a>
</div>
<script src="index.js"></script>
<!--jQuery script when using JSBin-->
<!--<script src="https://code.jquery.com/jquery-3.1.0.js"></script>-->
</body>
</html>
answered Nov 23 '18 at 6:49
new_usernew_user
1,3051017
1,3051017
Thank you so much, I really appreciate your help!
– Hungry Moose
Nov 23 '18 at 7:08
I'm glad it helped! cheers :)
– new_user
Nov 23 '18 at 7:15
add a comment |
Thank you so much, I really appreciate your help!
– Hungry Moose
Nov 23 '18 at 7:08
I'm glad it helped! cheers :)
– new_user
Nov 23 '18 at 7:15
Thank you so much, I really appreciate your help!
– Hungry Moose
Nov 23 '18 at 7:08
Thank you so much, I really appreciate your help!
– Hungry Moose
Nov 23 '18 at 7:08
I'm glad it helped! cheers :)
– new_user
Nov 23 '18 at 7:15
I'm glad it helped! cheers :)
– new_user
Nov 23 '18 at 7:15
add a comment |
Having skimmed through your JS, I'm assuming that the feedback shows on the same page as the question, rather than a new page resulting from submitting it to a server.
When you display the feedback, add a disabled="disabled"
property to the item in question, so that your user can't change it. Alternately, hide the selection and display an <output>
element: <output>Your Answer: USA<br />Correct Answer: UK</output>
add a comment |
Having skimmed through your JS, I'm assuming that the feedback shows on the same page as the question, rather than a new page resulting from submitting it to a server.
When you display the feedback, add a disabled="disabled"
property to the item in question, so that your user can't change it. Alternately, hide the selection and display an <output>
element: <output>Your Answer: USA<br />Correct Answer: UK</output>
add a comment |
Having skimmed through your JS, I'm assuming that the feedback shows on the same page as the question, rather than a new page resulting from submitting it to a server.
When you display the feedback, add a disabled="disabled"
property to the item in question, so that your user can't change it. Alternately, hide the selection and display an <output>
element: <output>Your Answer: USA<br />Correct Answer: UK</output>
Having skimmed through your JS, I'm assuming that the feedback shows on the same page as the question, rather than a new page resulting from submitting it to a server.
When you display the feedback, add a disabled="disabled"
property to the item in question, so that your user can't change it. Alternately, hide the selection and display an <output>
element: <output>Your Answer: USA<br />Correct Answer: UK</output>
answered Nov 23 '18 at 6:48


Agi HammerthiefAgi Hammerthief
1,2301426
1,2301426
add a comment |
add a comment |
disable All button after checking answers.
$('button').prop('disabled', true);
add a comment |
disable All button after checking answers.
$('button').prop('disabled', true);
add a comment |
disable All button after checking answers.
$('button').prop('disabled', true);
disable All button after checking answers.
$('button').prop('disabled', true);
answered Nov 23 '18 at 6:49
SameerSameer
316210
316210
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53441712%2fhow-can-i-prevent-users-from-selecting-multiple-answers-in-my-quiz%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
PYgf4 CFGFGN2
3
Use radio elements instead of
<button>
– Satpal
Nov 23 '18 at 6:39
2
Adding
disabled
prop after submit and removing on next question click– siddhant sankhe
Nov 23 '18 at 6:44
<input type="radio" name= "choice" value="someValue1"> <input type="radio" name= "choice" value="someValue2">
something like this.– Kunal Mukherjee
Nov 23 '18 at 6:45
document.getElementsByTagName("button").disabled = true; will disable the buttons. By the way, I had all the answers right. What do I win?
– Gerard
Nov 23 '18 at 6:48