How to correctly add a date and a time (string) in PHP?
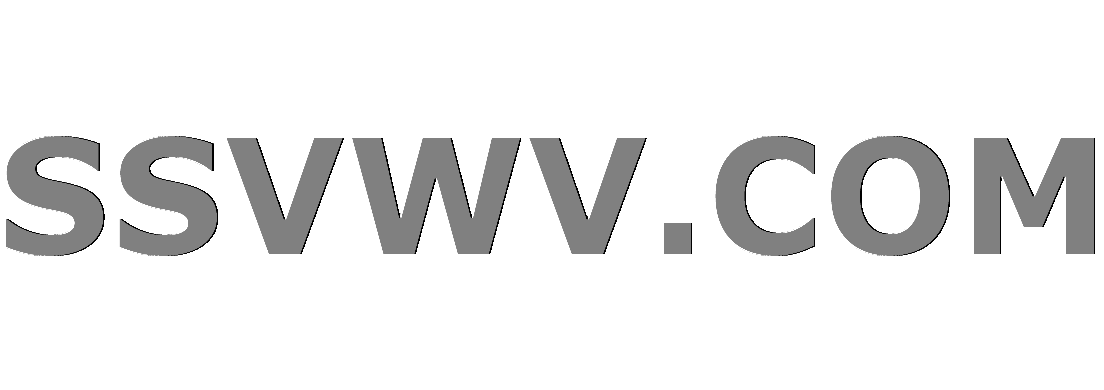
Multi tool use
What is the "cleanest" way to add a date and a time string in PHP?
Albeit having read that DateTime::add
expects a DateInterval
, I tried
$date = new DateTime('17.03.2016');
$time = new DateTime('20:20');
$result = $date->add($time);
Which was no good and returned nothing to $result
.
To make a DateInterval
from '20:20'
, I only found very complex solutions...
Maybe I should use timestamps?
$date = strtotime($datestring);
$timeObj = new DateTime($timestring);
// quirk to only get time in seconds from string date
$time = $timeObj->format('H') * 3600 + $timeObj->format('i') * 60 + $timeObj->format('s');
$datetime = $date+$time;
$result = new DateTime;
$result->setTimestamp($datetime);
In my case, this returns the desired result, with the correct timezone offset. But what do you think, is this robust? Is there a better way?
php datetime dateinterval
add a comment |
What is the "cleanest" way to add a date and a time string in PHP?
Albeit having read that DateTime::add
expects a DateInterval
, I tried
$date = new DateTime('17.03.2016');
$time = new DateTime('20:20');
$result = $date->add($time);
Which was no good and returned nothing to $result
.
To make a DateInterval
from '20:20'
, I only found very complex solutions...
Maybe I should use timestamps?
$date = strtotime($datestring);
$timeObj = new DateTime($timestring);
// quirk to only get time in seconds from string date
$time = $timeObj->format('H') * 3600 + $timeObj->format('i') * 60 + $timeObj->format('s');
$datetime = $date+$time;
$result = new DateTime;
$result->setTimestamp($datetime);
In my case, this returns the desired result, with the correct timezone offset. But what do you think, is this robust? Is there a better way?
php datetime dateinterval
1
I like to use Carbon (carbon.nesbot.com)
– Felippe Duarte
Nov 23 '18 at 18:12
Nice one! For a bigger project
– Urs
Nov 23 '18 at 19:24
add a comment |
What is the "cleanest" way to add a date and a time string in PHP?
Albeit having read that DateTime::add
expects a DateInterval
, I tried
$date = new DateTime('17.03.2016');
$time = new DateTime('20:20');
$result = $date->add($time);
Which was no good and returned nothing to $result
.
To make a DateInterval
from '20:20'
, I only found very complex solutions...
Maybe I should use timestamps?
$date = strtotime($datestring);
$timeObj = new DateTime($timestring);
// quirk to only get time in seconds from string date
$time = $timeObj->format('H') * 3600 + $timeObj->format('i') * 60 + $timeObj->format('s');
$datetime = $date+$time;
$result = new DateTime;
$result->setTimestamp($datetime);
In my case, this returns the desired result, with the correct timezone offset. But what do you think, is this robust? Is there a better way?
php datetime dateinterval
What is the "cleanest" way to add a date and a time string in PHP?
Albeit having read that DateTime::add
expects a DateInterval
, I tried
$date = new DateTime('17.03.2016');
$time = new DateTime('20:20');
$result = $date->add($time);
Which was no good and returned nothing to $result
.
To make a DateInterval
from '20:20'
, I only found very complex solutions...
Maybe I should use timestamps?
$date = strtotime($datestring);
$timeObj = new DateTime($timestring);
// quirk to only get time in seconds from string date
$time = $timeObj->format('H') * 3600 + $timeObj->format('i') * 60 + $timeObj->format('s');
$datetime = $date+$time;
$result = new DateTime;
$result->setTimestamp($datetime);
In my case, this returns the desired result, with the correct timezone offset. But what do you think, is this robust? Is there a better way?
php datetime dateinterval
php datetime dateinterval
asked Nov 23 '18 at 17:42
UrsUrs
3,28343384
3,28343384
1
I like to use Carbon (carbon.nesbot.com)
– Felippe Duarte
Nov 23 '18 at 18:12
Nice one! For a bigger project
– Urs
Nov 23 '18 at 19:24
add a comment |
1
I like to use Carbon (carbon.nesbot.com)
– Felippe Duarte
Nov 23 '18 at 18:12
Nice one! For a bigger project
– Urs
Nov 23 '18 at 19:24
1
1
I like to use Carbon (carbon.nesbot.com)
– Felippe Duarte
Nov 23 '18 at 18:12
I like to use Carbon (carbon.nesbot.com)
– Felippe Duarte
Nov 23 '18 at 18:12
Nice one! For a bigger project
– Urs
Nov 23 '18 at 19:24
Nice one! For a bigger project
– Urs
Nov 23 '18 at 19:24
add a comment |
2 Answers
2
active
oldest
votes
If you want to add 20 hours and 20 minutes to a DateTime
:
$date = new DateTime('17.03.2016');
$date->add($new DateInterval('PT20H20M'));
You do not need to get the result of add()
, calling add()
on a DateTime
object will change it. The return value of add()
is the DateTime
object itself so you can chain methods.
See DateInterval::__construct to see how to set the intervals.
add a comment |
DateTime
(and DateTimeImmutable
) has a modify
method which you could leverage to modify the time by adding 20 hours
and 20 minutes
.
Updated
I've included examples for both DateTime
and DateTimeImmutable
as per the comment made, you don't need to assign the outcome of modify
to a variable because it mutates the original object. Whereas DateTimeImmutable
creates a new instance and doesn't mutate the original object.
DateTime
<?php
$start = new DateTimeImmutable('2018-10-23 00:00:00');
echo $start->modify('+20 hours +20 minutes')->format('Y-m-d H:i:s');
// 2018-10-23 20:20:00
Using DateTime
: https://3v4l.org/6eon8
DateTimeImmutable
<?php
$start = new DateTimeImmutable('2018-10-23 00:00:00');
$datetime = $start->modify('+20 hours +20 minutes');
var_dump($start->format('Y-m-d H:i:s'));
var_dump($datetime->format('Y-m-d H:i:s'));
Output
string(19) "2018-10-23 00:00:00"
string(19) "2018-10-23 20:20:00"
Using DateTimeImmutable
: https://3v4l.org/oRehh
There is no need to create another reference to theDateTime
object.modify()
returns theDateTime
object itself. So$start
and$datetime
simply point to the sameDateTime
object.
– Ron Dobley
Nov 23 '18 at 18:26
Yes that's correct, my original example should have usedDateTimeImmutable
instead. I've updated my answer.
– steadweb
Nov 23 '18 at 18:44
So in the first block, it should be$start = new DateTime('2018-10-23 00:00:00');
right?
– Urs
Nov 23 '18 at 19:23
Any advantages ofmodify
overadd
as shown by @ron-dobley ?
– Urs
Nov 23 '18 at 19:25
As far as I'm aware, they're pretty similar. It'll be preference of how the code looks compared to performance.
– steadweb
Nov 23 '18 at 19:30
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53451000%2fhow-to-correctly-add-a-date-and-a-time-string-in-php%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you want to add 20 hours and 20 minutes to a DateTime
:
$date = new DateTime('17.03.2016');
$date->add($new DateInterval('PT20H20M'));
You do not need to get the result of add()
, calling add()
on a DateTime
object will change it. The return value of add()
is the DateTime
object itself so you can chain methods.
See DateInterval::__construct to see how to set the intervals.
add a comment |
If you want to add 20 hours and 20 minutes to a DateTime
:
$date = new DateTime('17.03.2016');
$date->add($new DateInterval('PT20H20M'));
You do not need to get the result of add()
, calling add()
on a DateTime
object will change it. The return value of add()
is the DateTime
object itself so you can chain methods.
See DateInterval::__construct to see how to set the intervals.
add a comment |
If you want to add 20 hours and 20 minutes to a DateTime
:
$date = new DateTime('17.03.2016');
$date->add($new DateInterval('PT20H20M'));
You do not need to get the result of add()
, calling add()
on a DateTime
object will change it. The return value of add()
is the DateTime
object itself so you can chain methods.
See DateInterval::__construct to see how to set the intervals.
If you want to add 20 hours and 20 minutes to a DateTime
:
$date = new DateTime('17.03.2016');
$date->add($new DateInterval('PT20H20M'));
You do not need to get the result of add()
, calling add()
on a DateTime
object will change it. The return value of add()
is the DateTime
object itself so you can chain methods.
See DateInterval::__construct to see how to set the intervals.
edited Nov 23 '18 at 18:23
answered Nov 23 '18 at 18:16


Ron DobleyRon Dobley
887
887
add a comment |
add a comment |
DateTime
(and DateTimeImmutable
) has a modify
method which you could leverage to modify the time by adding 20 hours
and 20 minutes
.
Updated
I've included examples for both DateTime
and DateTimeImmutable
as per the comment made, you don't need to assign the outcome of modify
to a variable because it mutates the original object. Whereas DateTimeImmutable
creates a new instance and doesn't mutate the original object.
DateTime
<?php
$start = new DateTimeImmutable('2018-10-23 00:00:00');
echo $start->modify('+20 hours +20 minutes')->format('Y-m-d H:i:s');
// 2018-10-23 20:20:00
Using DateTime
: https://3v4l.org/6eon8
DateTimeImmutable
<?php
$start = new DateTimeImmutable('2018-10-23 00:00:00');
$datetime = $start->modify('+20 hours +20 minutes');
var_dump($start->format('Y-m-d H:i:s'));
var_dump($datetime->format('Y-m-d H:i:s'));
Output
string(19) "2018-10-23 00:00:00"
string(19) "2018-10-23 20:20:00"
Using DateTimeImmutable
: https://3v4l.org/oRehh
There is no need to create another reference to theDateTime
object.modify()
returns theDateTime
object itself. So$start
and$datetime
simply point to the sameDateTime
object.
– Ron Dobley
Nov 23 '18 at 18:26
Yes that's correct, my original example should have usedDateTimeImmutable
instead. I've updated my answer.
– steadweb
Nov 23 '18 at 18:44
So in the first block, it should be$start = new DateTime('2018-10-23 00:00:00');
right?
– Urs
Nov 23 '18 at 19:23
Any advantages ofmodify
overadd
as shown by @ron-dobley ?
– Urs
Nov 23 '18 at 19:25
As far as I'm aware, they're pretty similar. It'll be preference of how the code looks compared to performance.
– steadweb
Nov 23 '18 at 19:30
|
show 1 more comment
DateTime
(and DateTimeImmutable
) has a modify
method which you could leverage to modify the time by adding 20 hours
and 20 minutes
.
Updated
I've included examples for both DateTime
and DateTimeImmutable
as per the comment made, you don't need to assign the outcome of modify
to a variable because it mutates the original object. Whereas DateTimeImmutable
creates a new instance and doesn't mutate the original object.
DateTime
<?php
$start = new DateTimeImmutable('2018-10-23 00:00:00');
echo $start->modify('+20 hours +20 minutes')->format('Y-m-d H:i:s');
// 2018-10-23 20:20:00
Using DateTime
: https://3v4l.org/6eon8
DateTimeImmutable
<?php
$start = new DateTimeImmutable('2018-10-23 00:00:00');
$datetime = $start->modify('+20 hours +20 minutes');
var_dump($start->format('Y-m-d H:i:s'));
var_dump($datetime->format('Y-m-d H:i:s'));
Output
string(19) "2018-10-23 00:00:00"
string(19) "2018-10-23 20:20:00"
Using DateTimeImmutable
: https://3v4l.org/oRehh
There is no need to create another reference to theDateTime
object.modify()
returns theDateTime
object itself. So$start
and$datetime
simply point to the sameDateTime
object.
– Ron Dobley
Nov 23 '18 at 18:26
Yes that's correct, my original example should have usedDateTimeImmutable
instead. I've updated my answer.
– steadweb
Nov 23 '18 at 18:44
So in the first block, it should be$start = new DateTime('2018-10-23 00:00:00');
right?
– Urs
Nov 23 '18 at 19:23
Any advantages ofmodify
overadd
as shown by @ron-dobley ?
– Urs
Nov 23 '18 at 19:25
As far as I'm aware, they're pretty similar. It'll be preference of how the code looks compared to performance.
– steadweb
Nov 23 '18 at 19:30
|
show 1 more comment
DateTime
(and DateTimeImmutable
) has a modify
method which you could leverage to modify the time by adding 20 hours
and 20 minutes
.
Updated
I've included examples for both DateTime
and DateTimeImmutable
as per the comment made, you don't need to assign the outcome of modify
to a variable because it mutates the original object. Whereas DateTimeImmutable
creates a new instance and doesn't mutate the original object.
DateTime
<?php
$start = new DateTimeImmutable('2018-10-23 00:00:00');
echo $start->modify('+20 hours +20 minutes')->format('Y-m-d H:i:s');
// 2018-10-23 20:20:00
Using DateTime
: https://3v4l.org/6eon8
DateTimeImmutable
<?php
$start = new DateTimeImmutable('2018-10-23 00:00:00');
$datetime = $start->modify('+20 hours +20 minutes');
var_dump($start->format('Y-m-d H:i:s'));
var_dump($datetime->format('Y-m-d H:i:s'));
Output
string(19) "2018-10-23 00:00:00"
string(19) "2018-10-23 20:20:00"
Using DateTimeImmutable
: https://3v4l.org/oRehh
DateTime
(and DateTimeImmutable
) has a modify
method which you could leverage to modify the time by adding 20 hours
and 20 minutes
.
Updated
I've included examples for both DateTime
and DateTimeImmutable
as per the comment made, you don't need to assign the outcome of modify
to a variable because it mutates the original object. Whereas DateTimeImmutable
creates a new instance and doesn't mutate the original object.
DateTime
<?php
$start = new DateTimeImmutable('2018-10-23 00:00:00');
echo $start->modify('+20 hours +20 minutes')->format('Y-m-d H:i:s');
// 2018-10-23 20:20:00
Using DateTime
: https://3v4l.org/6eon8
DateTimeImmutable
<?php
$start = new DateTimeImmutable('2018-10-23 00:00:00');
$datetime = $start->modify('+20 hours +20 minutes');
var_dump($start->format('Y-m-d H:i:s'));
var_dump($datetime->format('Y-m-d H:i:s'));
Output
string(19) "2018-10-23 00:00:00"
string(19) "2018-10-23 20:20:00"
Using DateTimeImmutable
: https://3v4l.org/oRehh
edited Nov 23 '18 at 18:43
answered Nov 23 '18 at 18:12
steadwebsteadweb
5,41011223
5,41011223
There is no need to create another reference to theDateTime
object.modify()
returns theDateTime
object itself. So$start
and$datetime
simply point to the sameDateTime
object.
– Ron Dobley
Nov 23 '18 at 18:26
Yes that's correct, my original example should have usedDateTimeImmutable
instead. I've updated my answer.
– steadweb
Nov 23 '18 at 18:44
So in the first block, it should be$start = new DateTime('2018-10-23 00:00:00');
right?
– Urs
Nov 23 '18 at 19:23
Any advantages ofmodify
overadd
as shown by @ron-dobley ?
– Urs
Nov 23 '18 at 19:25
As far as I'm aware, they're pretty similar. It'll be preference of how the code looks compared to performance.
– steadweb
Nov 23 '18 at 19:30
|
show 1 more comment
There is no need to create another reference to theDateTime
object.modify()
returns theDateTime
object itself. So$start
and$datetime
simply point to the sameDateTime
object.
– Ron Dobley
Nov 23 '18 at 18:26
Yes that's correct, my original example should have usedDateTimeImmutable
instead. I've updated my answer.
– steadweb
Nov 23 '18 at 18:44
So in the first block, it should be$start = new DateTime('2018-10-23 00:00:00');
right?
– Urs
Nov 23 '18 at 19:23
Any advantages ofmodify
overadd
as shown by @ron-dobley ?
– Urs
Nov 23 '18 at 19:25
As far as I'm aware, they're pretty similar. It'll be preference of how the code looks compared to performance.
– steadweb
Nov 23 '18 at 19:30
There is no need to create another reference to the
DateTime
object. modify()
returns the DateTime
object itself. So $start
and $datetime
simply point to the same DateTime
object.– Ron Dobley
Nov 23 '18 at 18:26
There is no need to create another reference to the
DateTime
object. modify()
returns the DateTime
object itself. So $start
and $datetime
simply point to the same DateTime
object.– Ron Dobley
Nov 23 '18 at 18:26
Yes that's correct, my original example should have used
DateTimeImmutable
instead. I've updated my answer.– steadweb
Nov 23 '18 at 18:44
Yes that's correct, my original example should have used
DateTimeImmutable
instead. I've updated my answer.– steadweb
Nov 23 '18 at 18:44
So in the first block, it should be
$start = new DateTime('2018-10-23 00:00:00');
right?– Urs
Nov 23 '18 at 19:23
So in the first block, it should be
$start = new DateTime('2018-10-23 00:00:00');
right?– Urs
Nov 23 '18 at 19:23
Any advantages of
modify
over add
as shown by @ron-dobley ?– Urs
Nov 23 '18 at 19:25
Any advantages of
modify
over add
as shown by @ron-dobley ?– Urs
Nov 23 '18 at 19:25
As far as I'm aware, they're pretty similar. It'll be preference of how the code looks compared to performance.
– steadweb
Nov 23 '18 at 19:30
As far as I'm aware, they're pretty similar. It'll be preference of how the code looks compared to performance.
– steadweb
Nov 23 '18 at 19:30
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53451000%2fhow-to-correctly-add-a-date-and-a-time-string-in-php%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
SE9M1M3gz7ni y c LJg
1
I like to use Carbon (carbon.nesbot.com)
– Felippe Duarte
Nov 23 '18 at 18:12
Nice one! For a bigger project
– Urs
Nov 23 '18 at 19:24