How to Generate A Slug on AppSync Mutation
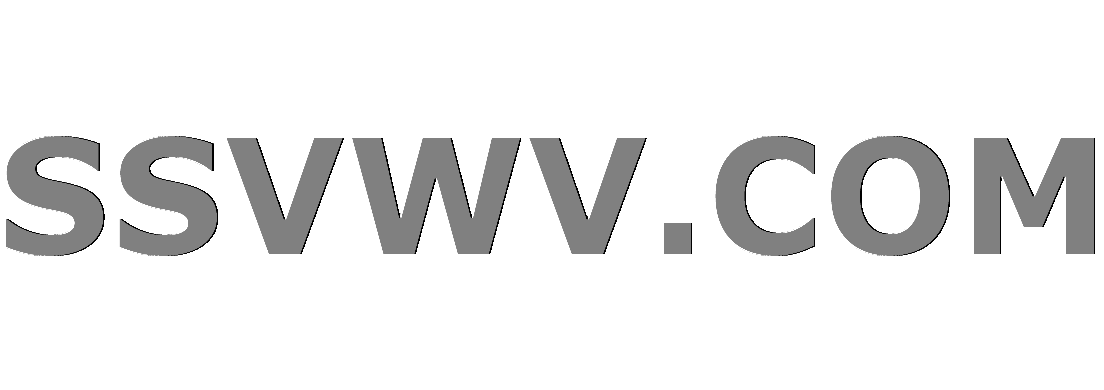
Multi tool use
I have a mutation to create a new Event
. However I want to be able to reference/get that Event
using a friendly id or slug instead of the DynamoDB primary key of id
. Ideally this would use the city
field from the input to generate the slug. However each slug must be unique per author
How can I generate a friendly slug in my mutation that is based on the city name and unique (per author) ?
Schema
type Event {
id: ID!
subtitle: String!
city: String!
author: String!
created: AWSDateTime
}
mutation.CreateEvent
#set( $attribs = $util.dynamodb.toMapValues($ctx.args.input))
#set( $attribs.author = $util.dynamodb.toDynamoDB($ctx.identity.username))
#set( $attribs.created = $util.dynamodb.toDynamoDB($util.time.nowFormatted("yyyy-MM-dd HH:mm:ssZ")))
{
"version": "2017-02-28",
"operation": "PutItem",
"key": {
"id": $util.dynamodb.toDynamoDBJson($util.autoId()),
},
"attributeValues": $util.toJson($attribs),
"condition": {
"expression": "attribute_not_exists(#id)",
"expressionNames": {
"#id": "id",
},
},
}
```
aws-appsync
add a comment |
I have a mutation to create a new Event
. However I want to be able to reference/get that Event
using a friendly id or slug instead of the DynamoDB primary key of id
. Ideally this would use the city
field from the input to generate the slug. However each slug must be unique per author
How can I generate a friendly slug in my mutation that is based on the city name and unique (per author) ?
Schema
type Event {
id: ID!
subtitle: String!
city: String!
author: String!
created: AWSDateTime
}
mutation.CreateEvent
#set( $attribs = $util.dynamodb.toMapValues($ctx.args.input))
#set( $attribs.author = $util.dynamodb.toDynamoDB($ctx.identity.username))
#set( $attribs.created = $util.dynamodb.toDynamoDB($util.time.nowFormatted("yyyy-MM-dd HH:mm:ssZ")))
{
"version": "2017-02-28",
"operation": "PutItem",
"key": {
"id": $util.dynamodb.toDynamoDBJson($util.autoId()),
},
"attributeValues": $util.toJson($attribs),
"condition": {
"expression": "attribute_not_exists(#id)",
"expressionNames": {
"#id": "id",
},
},
}
```
aws-appsync
You would probably have to store the slug in a different table, where the author is the hash key and the slug probably the sort key. Also, you will have to write logic that checks if a slug candidate is available in the table before creating it, and trying something different if it fails. In that case I would recommend using the Lambda data source.
– Tinou
Nov 23 '18 at 22:12
@Tinou could i use the new Pipeline Resolvers instead in this case somehow?
– CraigH
Nov 27 '18 at 20:42
add a comment |
I have a mutation to create a new Event
. However I want to be able to reference/get that Event
using a friendly id or slug instead of the DynamoDB primary key of id
. Ideally this would use the city
field from the input to generate the slug. However each slug must be unique per author
How can I generate a friendly slug in my mutation that is based on the city name and unique (per author) ?
Schema
type Event {
id: ID!
subtitle: String!
city: String!
author: String!
created: AWSDateTime
}
mutation.CreateEvent
#set( $attribs = $util.dynamodb.toMapValues($ctx.args.input))
#set( $attribs.author = $util.dynamodb.toDynamoDB($ctx.identity.username))
#set( $attribs.created = $util.dynamodb.toDynamoDB($util.time.nowFormatted("yyyy-MM-dd HH:mm:ssZ")))
{
"version": "2017-02-28",
"operation": "PutItem",
"key": {
"id": $util.dynamodb.toDynamoDBJson($util.autoId()),
},
"attributeValues": $util.toJson($attribs),
"condition": {
"expression": "attribute_not_exists(#id)",
"expressionNames": {
"#id": "id",
},
},
}
```
aws-appsync
I have a mutation to create a new Event
. However I want to be able to reference/get that Event
using a friendly id or slug instead of the DynamoDB primary key of id
. Ideally this would use the city
field from the input to generate the slug. However each slug must be unique per author
How can I generate a friendly slug in my mutation that is based on the city name and unique (per author) ?
Schema
type Event {
id: ID!
subtitle: String!
city: String!
author: String!
created: AWSDateTime
}
mutation.CreateEvent
#set( $attribs = $util.dynamodb.toMapValues($ctx.args.input))
#set( $attribs.author = $util.dynamodb.toDynamoDB($ctx.identity.username))
#set( $attribs.created = $util.dynamodb.toDynamoDB($util.time.nowFormatted("yyyy-MM-dd HH:mm:ssZ")))
{
"version": "2017-02-28",
"operation": "PutItem",
"key": {
"id": $util.dynamodb.toDynamoDBJson($util.autoId()),
},
"attributeValues": $util.toJson($attribs),
"condition": {
"expression": "attribute_not_exists(#id)",
"expressionNames": {
"#id": "id",
},
},
}
```
aws-appsync
aws-appsync
asked Nov 23 '18 at 17:42
CraigHCraigH
86711638
86711638
You would probably have to store the slug in a different table, where the author is the hash key and the slug probably the sort key. Also, you will have to write logic that checks if a slug candidate is available in the table before creating it, and trying something different if it fails. In that case I would recommend using the Lambda data source.
– Tinou
Nov 23 '18 at 22:12
@Tinou could i use the new Pipeline Resolvers instead in this case somehow?
– CraigH
Nov 27 '18 at 20:42
add a comment |
You would probably have to store the slug in a different table, where the author is the hash key and the slug probably the sort key. Also, you will have to write logic that checks if a slug candidate is available in the table before creating it, and trying something different if it fails. In that case I would recommend using the Lambda data source.
– Tinou
Nov 23 '18 at 22:12
@Tinou could i use the new Pipeline Resolvers instead in this case somehow?
– CraigH
Nov 27 '18 at 20:42
You would probably have to store the slug in a different table, where the author is the hash key and the slug probably the sort key. Also, you will have to write logic that checks if a slug candidate is available in the table before creating it, and trying something different if it fails. In that case I would recommend using the Lambda data source.
– Tinou
Nov 23 '18 at 22:12
You would probably have to store the slug in a different table, where the author is the hash key and the slug probably the sort key. Also, you will have to write logic that checks if a slug candidate is available in the table before creating it, and trying something different if it fails. In that case I would recommend using the Lambda data source.
– Tinou
Nov 23 '18 at 22:12
@Tinou could i use the new Pipeline Resolvers instead in this case somehow?
– CraigH
Nov 27 '18 at 20:42
@Tinou could i use the new Pipeline Resolvers instead in this case somehow?
– CraigH
Nov 27 '18 at 20:42
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450995%2fhow-to-generate-a-slug-on-appsync-mutation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450995%2fhow-to-generate-a-slug-on-appsync-mutation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3ZJo28d5k2,u,xe 8pEymMW949Xwd,836h4f
You would probably have to store the slug in a different table, where the author is the hash key and the slug probably the sort key. Also, you will have to write logic that checks if a slug candidate is available in the table before creating it, and trying something different if it fails. In that case I would recommend using the Lambda data source.
– Tinou
Nov 23 '18 at 22:12
@Tinou could i use the new Pipeline Resolvers instead in this case somehow?
– CraigH
Nov 27 '18 at 20:42