spring auth error No AuthenticationProvider
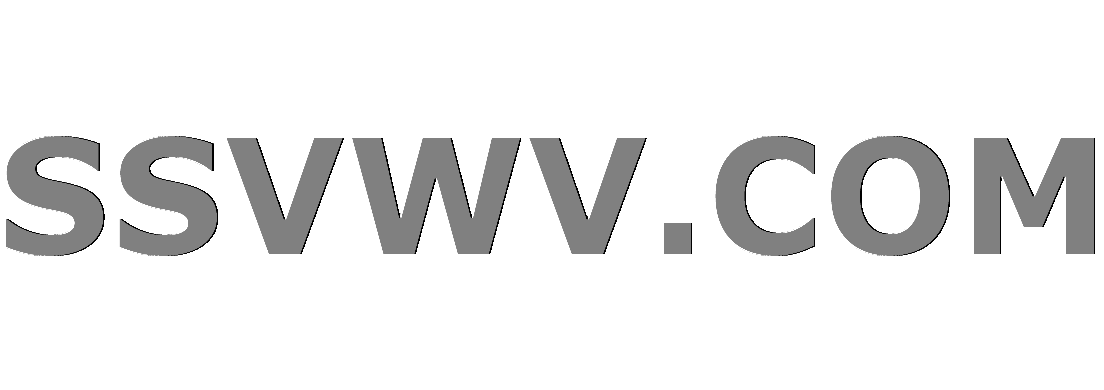
Multi tool use
I'm trying to build auth system with Spring Boot Security.
So I have custom auth provider (without @Component annotation)
public class CustomAuthProvider extends DaoAuthenticationProvider {
@Override
public Authentication authenticate(Authentication authentication)
throws AuthenticationException {
String name = authentication.getName();
String password = authentication.getCredentials().toString();
if (authentication.isAuthenticated()) {
return authentication;
}
if ("user".equals(name) && "password".equals(password)) {
return new UsernamePasswordAuthenticationToken(
name, password, new ArrayList<GrantedAuthority>(Arrays.asList(new SimpleGrantedAuthority("ROLE_USER"))));
} else {
return null;
}
}
@Override
public boolean supports(Class<?> authentication) {
return (UsernamePasswordAuthenticationToken.class.isAssignableFrom(authentication));
}
}
which declared as a bean
@Bean
public DaoAuthenticationProvider authProvider() {
final CustomAuthProvider authProvider = new CustomAuthProvider();
authProvider.setUserDetailsService(userDetailsService);
return authProvider;
}
Here is configuration:
@Override
protected void configure(
AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(authProvider());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/anonymous*").anonymous()
.antMatchers("/login*").permitAll()
.antMatchers("/user/registration*").permitAll()
.anyRequest().authenticated()
.and().formLogin()
.loginProcessingUrl("/login");
}
When I'm trying post query to localhost:8080/login I'm getting sign-in form with message
No AuthenticationProvider found for
org.springframework.security.authentication.UsernamePasswordAuthenticationToken
java spring spring-boot spring-security
add a comment |
I'm trying to build auth system with Spring Boot Security.
So I have custom auth provider (without @Component annotation)
public class CustomAuthProvider extends DaoAuthenticationProvider {
@Override
public Authentication authenticate(Authentication authentication)
throws AuthenticationException {
String name = authentication.getName();
String password = authentication.getCredentials().toString();
if (authentication.isAuthenticated()) {
return authentication;
}
if ("user".equals(name) && "password".equals(password)) {
return new UsernamePasswordAuthenticationToken(
name, password, new ArrayList<GrantedAuthority>(Arrays.asList(new SimpleGrantedAuthority("ROLE_USER"))));
} else {
return null;
}
}
@Override
public boolean supports(Class<?> authentication) {
return (UsernamePasswordAuthenticationToken.class.isAssignableFrom(authentication));
}
}
which declared as a bean
@Bean
public DaoAuthenticationProvider authProvider() {
final CustomAuthProvider authProvider = new CustomAuthProvider();
authProvider.setUserDetailsService(userDetailsService);
return authProvider;
}
Here is configuration:
@Override
protected void configure(
AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(authProvider());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/anonymous*").anonymous()
.antMatchers("/login*").permitAll()
.antMatchers("/user/registration*").permitAll()
.anyRequest().authenticated()
.and().formLogin()
.loginProcessingUrl("/login");
}
When I'm trying post query to localhost:8080/login I'm getting sign-in form with message
No AuthenticationProvider found for
org.springframework.security.authentication.UsernamePasswordAuthenticationToken
java spring spring-boot spring-security
can you add the request you post to /login?
– stacker
Nov 23 '18 at 18:48
@slimane does it matter with such error? I send simple post with few fields (email and password) without headers via Postman
– Twice
Nov 23 '18 at 19:52
your exception doesn't only reflect messing authprovider, it can be also that your authprovider had an exception while processing your request
– stacker
Nov 23 '18 at 20:18
@slimane pastebin.com/SzT0t1Yu
– Twice
Nov 23 '18 at 21:29
add a comment |
I'm trying to build auth system with Spring Boot Security.
So I have custom auth provider (without @Component annotation)
public class CustomAuthProvider extends DaoAuthenticationProvider {
@Override
public Authentication authenticate(Authentication authentication)
throws AuthenticationException {
String name = authentication.getName();
String password = authentication.getCredentials().toString();
if (authentication.isAuthenticated()) {
return authentication;
}
if ("user".equals(name) && "password".equals(password)) {
return new UsernamePasswordAuthenticationToken(
name, password, new ArrayList<GrantedAuthority>(Arrays.asList(new SimpleGrantedAuthority("ROLE_USER"))));
} else {
return null;
}
}
@Override
public boolean supports(Class<?> authentication) {
return (UsernamePasswordAuthenticationToken.class.isAssignableFrom(authentication));
}
}
which declared as a bean
@Bean
public DaoAuthenticationProvider authProvider() {
final CustomAuthProvider authProvider = new CustomAuthProvider();
authProvider.setUserDetailsService(userDetailsService);
return authProvider;
}
Here is configuration:
@Override
protected void configure(
AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(authProvider());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/anonymous*").anonymous()
.antMatchers("/login*").permitAll()
.antMatchers("/user/registration*").permitAll()
.anyRequest().authenticated()
.and().formLogin()
.loginProcessingUrl("/login");
}
When I'm trying post query to localhost:8080/login I'm getting sign-in form with message
No AuthenticationProvider found for
org.springframework.security.authentication.UsernamePasswordAuthenticationToken
java spring spring-boot spring-security
I'm trying to build auth system with Spring Boot Security.
So I have custom auth provider (without @Component annotation)
public class CustomAuthProvider extends DaoAuthenticationProvider {
@Override
public Authentication authenticate(Authentication authentication)
throws AuthenticationException {
String name = authentication.getName();
String password = authentication.getCredentials().toString();
if (authentication.isAuthenticated()) {
return authentication;
}
if ("user".equals(name) && "password".equals(password)) {
return new UsernamePasswordAuthenticationToken(
name, password, new ArrayList<GrantedAuthority>(Arrays.asList(new SimpleGrantedAuthority("ROLE_USER"))));
} else {
return null;
}
}
@Override
public boolean supports(Class<?> authentication) {
return (UsernamePasswordAuthenticationToken.class.isAssignableFrom(authentication));
}
}
which declared as a bean
@Bean
public DaoAuthenticationProvider authProvider() {
final CustomAuthProvider authProvider = new CustomAuthProvider();
authProvider.setUserDetailsService(userDetailsService);
return authProvider;
}
Here is configuration:
@Override
protected void configure(
AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(authProvider());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/anonymous*").anonymous()
.antMatchers("/login*").permitAll()
.antMatchers("/user/registration*").permitAll()
.anyRequest().authenticated()
.and().formLogin()
.loginProcessingUrl("/login");
}
When I'm trying post query to localhost:8080/login I'm getting sign-in form with message
No AuthenticationProvider found for
org.springframework.security.authentication.UsernamePasswordAuthenticationToken
java spring spring-boot spring-security
java spring spring-boot spring-security
asked Nov 23 '18 at 17:37
TwiceTwice
1517
1517
can you add the request you post to /login?
– stacker
Nov 23 '18 at 18:48
@slimane does it matter with such error? I send simple post with few fields (email and password) without headers via Postman
– Twice
Nov 23 '18 at 19:52
your exception doesn't only reflect messing authprovider, it can be also that your authprovider had an exception while processing your request
– stacker
Nov 23 '18 at 20:18
@slimane pastebin.com/SzT0t1Yu
– Twice
Nov 23 '18 at 21:29
add a comment |
can you add the request you post to /login?
– stacker
Nov 23 '18 at 18:48
@slimane does it matter with such error? I send simple post with few fields (email and password) without headers via Postman
– Twice
Nov 23 '18 at 19:52
your exception doesn't only reflect messing authprovider, it can be also that your authprovider had an exception while processing your request
– stacker
Nov 23 '18 at 20:18
@slimane pastebin.com/SzT0t1Yu
– Twice
Nov 23 '18 at 21:29
can you add the request you post to /login?
– stacker
Nov 23 '18 at 18:48
can you add the request you post to /login?
– stacker
Nov 23 '18 at 18:48
@slimane does it matter with such error? I send simple post with few fields (email and password) without headers via Postman
– Twice
Nov 23 '18 at 19:52
@slimane does it matter with such error? I send simple post with few fields (email and password) without headers via Postman
– Twice
Nov 23 '18 at 19:52
your exception doesn't only reflect messing authprovider, it can be also that your authprovider had an exception while processing your request
– stacker
Nov 23 '18 at 20:18
your exception doesn't only reflect messing authprovider, it can be also that your authprovider had an exception while processing your request
– stacker
Nov 23 '18 at 20:18
@slimane pastebin.com/SzT0t1Yu
– Twice
Nov 23 '18 at 21:29
@slimane pastebin.com/SzT0t1Yu
– Twice
Nov 23 '18 at 21:29
add a comment |
1 Answer
1
active
oldest
votes
spring's default request parameters for authentication are: username
and password
you are sending email
instead of username
so it throws an exception somewhere in your authentication manager.
if you want to override the default values you can simply specify that in your HTTP security configs:
.formLogin()
.loginProcessingUrl("/login")
.usernameParameter("email")
.passwordParameter("password")
Hmm, it's anyway throws this error
– Twice
Nov 28 '18 at 9:50
This error appears even I login through form
– Twice
Nov 28 '18 at 10:46
it would be nice if we can access your code, is it possible to have it publicly?
– stacker
Nov 28 '18 at 10:56
it seems that I need to send data with form-data
– Twice
Nov 28 '18 at 14:18
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450956%2fspring-auth-error-no-authenticationprovider%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
spring's default request parameters for authentication are: username
and password
you are sending email
instead of username
so it throws an exception somewhere in your authentication manager.
if you want to override the default values you can simply specify that in your HTTP security configs:
.formLogin()
.loginProcessingUrl("/login")
.usernameParameter("email")
.passwordParameter("password")
Hmm, it's anyway throws this error
– Twice
Nov 28 '18 at 9:50
This error appears even I login through form
– Twice
Nov 28 '18 at 10:46
it would be nice if we can access your code, is it possible to have it publicly?
– stacker
Nov 28 '18 at 10:56
it seems that I need to send data with form-data
– Twice
Nov 28 '18 at 14:18
add a comment |
spring's default request parameters for authentication are: username
and password
you are sending email
instead of username
so it throws an exception somewhere in your authentication manager.
if you want to override the default values you can simply specify that in your HTTP security configs:
.formLogin()
.loginProcessingUrl("/login")
.usernameParameter("email")
.passwordParameter("password")
Hmm, it's anyway throws this error
– Twice
Nov 28 '18 at 9:50
This error appears even I login through form
– Twice
Nov 28 '18 at 10:46
it would be nice if we can access your code, is it possible to have it publicly?
– stacker
Nov 28 '18 at 10:56
it seems that I need to send data with form-data
– Twice
Nov 28 '18 at 14:18
add a comment |
spring's default request parameters for authentication are: username
and password
you are sending email
instead of username
so it throws an exception somewhere in your authentication manager.
if you want to override the default values you can simply specify that in your HTTP security configs:
.formLogin()
.loginProcessingUrl("/login")
.usernameParameter("email")
.passwordParameter("password")
spring's default request parameters for authentication are: username
and password
you are sending email
instead of username
so it throws an exception somewhere in your authentication manager.
if you want to override the default values you can simply specify that in your HTTP security configs:
.formLogin()
.loginProcessingUrl("/login")
.usernameParameter("email")
.passwordParameter("password")
answered Nov 23 '18 at 21:49
stackerstacker
1,833128
1,833128
Hmm, it's anyway throws this error
– Twice
Nov 28 '18 at 9:50
This error appears even I login through form
– Twice
Nov 28 '18 at 10:46
it would be nice if we can access your code, is it possible to have it publicly?
– stacker
Nov 28 '18 at 10:56
it seems that I need to send data with form-data
– Twice
Nov 28 '18 at 14:18
add a comment |
Hmm, it's anyway throws this error
– Twice
Nov 28 '18 at 9:50
This error appears even I login through form
– Twice
Nov 28 '18 at 10:46
it would be nice if we can access your code, is it possible to have it publicly?
– stacker
Nov 28 '18 at 10:56
it seems that I need to send data with form-data
– Twice
Nov 28 '18 at 14:18
Hmm, it's anyway throws this error
– Twice
Nov 28 '18 at 9:50
Hmm, it's anyway throws this error
– Twice
Nov 28 '18 at 9:50
This error appears even I login through form
– Twice
Nov 28 '18 at 10:46
This error appears even I login through form
– Twice
Nov 28 '18 at 10:46
it would be nice if we can access your code, is it possible to have it publicly?
– stacker
Nov 28 '18 at 10:56
it would be nice if we can access your code, is it possible to have it publicly?
– stacker
Nov 28 '18 at 10:56
it seems that I need to send data with form-data
– Twice
Nov 28 '18 at 14:18
it seems that I need to send data with form-data
– Twice
Nov 28 '18 at 14:18
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450956%2fspring-auth-error-no-authenticationprovider%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gWRWGqDYDSA
can you add the request you post to /login?
– stacker
Nov 23 '18 at 18:48
@slimane does it matter with such error? I send simple post with few fields (email and password) without headers via Postman
– Twice
Nov 23 '18 at 19:52
your exception doesn't only reflect messing authprovider, it can be also that your authprovider had an exception while processing your request
– stacker
Nov 23 '18 at 20:18
@slimane pastebin.com/SzT0t1Yu
– Twice
Nov 23 '18 at 21:29