How to fit a model to an image in Python
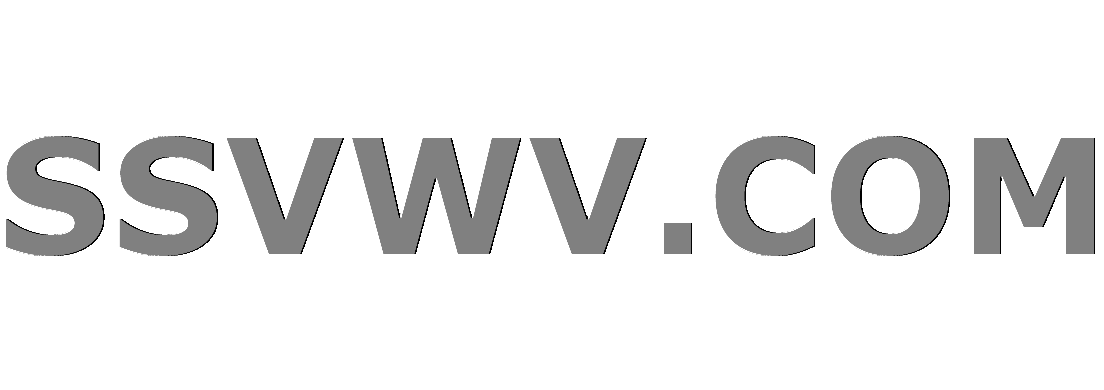
Multi tool use
I'm having issues with Scipy's Curve_fit function and was wondering if anyone knew what I could do about this. I'm working on a simulation and want to find a way of optimizing its parameters to a 'real' test image.
Band = Simulation(fname, other_params)
Here, Band is a class type variable which helps me to run simulations. The following function is a wrapper for the actual simulation, essentially the important thing is it returns a 1d array which is just a flattened image.
def wrapper(xy, X_off, Y_off):
Image = Band.weight(201,757,5000,5000, fill_length=7, offset_X = X_off, offset_Y = Y_off, rand_tup=(300,0,2000))
print X_off, Y_off
return Image.flatten()
Below I set some initial parameters for X_off, Y_off (the X/Y offsets, where X and Y are a quasi-Cartesian grid based on the satellite position). I also import a 'real' image which I will use as my dependent variable - essentially I'm asking this program to spit out optimal parameters such that the simulation will produce the closest image possible to this 'real' one.
p0 = [-14,4]
Real_image = Band.Im[some_range]
Notice I have 'xy' defined in my wrapper function. This is actually pretty useless, it's only there so I can say I have an independent variable - in reality I don't! The simulation doesn't really have an independent variable but I still want to find a way to optimize it and thought this would work.
xy = np.zeros(10)
optimal_params, covariance = curve_fit(wrapper, xy, F1_portion.flatten(), p0)
The results of the final line are always that optimal_params is incredibly similar to the initial guess p0, maybe off by a small amount. Upon closer study it seems that the algorithm doesn't vary the parameters by very much at all. I want it to vary the parameters in at least integer steps, and I'm not sure how I can help do this.
Essentially, I almost always get my initial parameters returned to me. Is this an issue with the algorithm or my code? Is there another algorithm I can use to find the optimal parameters?
Thanks
arrays python-2.7 scipy least-squares
add a comment |
I'm having issues with Scipy's Curve_fit function and was wondering if anyone knew what I could do about this. I'm working on a simulation and want to find a way of optimizing its parameters to a 'real' test image.
Band = Simulation(fname, other_params)
Here, Band is a class type variable which helps me to run simulations. The following function is a wrapper for the actual simulation, essentially the important thing is it returns a 1d array which is just a flattened image.
def wrapper(xy, X_off, Y_off):
Image = Band.weight(201,757,5000,5000, fill_length=7, offset_X = X_off, offset_Y = Y_off, rand_tup=(300,0,2000))
print X_off, Y_off
return Image.flatten()
Below I set some initial parameters for X_off, Y_off (the X/Y offsets, where X and Y are a quasi-Cartesian grid based on the satellite position). I also import a 'real' image which I will use as my dependent variable - essentially I'm asking this program to spit out optimal parameters such that the simulation will produce the closest image possible to this 'real' one.
p0 = [-14,4]
Real_image = Band.Im[some_range]
Notice I have 'xy' defined in my wrapper function. This is actually pretty useless, it's only there so I can say I have an independent variable - in reality I don't! The simulation doesn't really have an independent variable but I still want to find a way to optimize it and thought this would work.
xy = np.zeros(10)
optimal_params, covariance = curve_fit(wrapper, xy, F1_portion.flatten(), p0)
The results of the final line are always that optimal_params is incredibly similar to the initial guess p0, maybe off by a small amount. Upon closer study it seems that the algorithm doesn't vary the parameters by very much at all. I want it to vary the parameters in at least integer steps, and I'm not sure how I can help do this.
Essentially, I almost always get my initial parameters returned to me. Is this an issue with the algorithm or my code? Is there another algorithm I can use to find the optimal parameters?
Thanks
arrays python-2.7 scipy least-squares
1
FYI: The function that you passed toleastsq
must return all the residuals, not the sum of the residuals.
– Warren Weckesser
Nov 23 '18 at 18:05
add a comment |
I'm having issues with Scipy's Curve_fit function and was wondering if anyone knew what I could do about this. I'm working on a simulation and want to find a way of optimizing its parameters to a 'real' test image.
Band = Simulation(fname, other_params)
Here, Band is a class type variable which helps me to run simulations. The following function is a wrapper for the actual simulation, essentially the important thing is it returns a 1d array which is just a flattened image.
def wrapper(xy, X_off, Y_off):
Image = Band.weight(201,757,5000,5000, fill_length=7, offset_X = X_off, offset_Y = Y_off, rand_tup=(300,0,2000))
print X_off, Y_off
return Image.flatten()
Below I set some initial parameters for X_off, Y_off (the X/Y offsets, where X and Y are a quasi-Cartesian grid based on the satellite position). I also import a 'real' image which I will use as my dependent variable - essentially I'm asking this program to spit out optimal parameters such that the simulation will produce the closest image possible to this 'real' one.
p0 = [-14,4]
Real_image = Band.Im[some_range]
Notice I have 'xy' defined in my wrapper function. This is actually pretty useless, it's only there so I can say I have an independent variable - in reality I don't! The simulation doesn't really have an independent variable but I still want to find a way to optimize it and thought this would work.
xy = np.zeros(10)
optimal_params, covariance = curve_fit(wrapper, xy, F1_portion.flatten(), p0)
The results of the final line are always that optimal_params is incredibly similar to the initial guess p0, maybe off by a small amount. Upon closer study it seems that the algorithm doesn't vary the parameters by very much at all. I want it to vary the parameters in at least integer steps, and I'm not sure how I can help do this.
Essentially, I almost always get my initial parameters returned to me. Is this an issue with the algorithm or my code? Is there another algorithm I can use to find the optimal parameters?
Thanks
arrays python-2.7 scipy least-squares
I'm having issues with Scipy's Curve_fit function and was wondering if anyone knew what I could do about this. I'm working on a simulation and want to find a way of optimizing its parameters to a 'real' test image.
Band = Simulation(fname, other_params)
Here, Band is a class type variable which helps me to run simulations. The following function is a wrapper for the actual simulation, essentially the important thing is it returns a 1d array which is just a flattened image.
def wrapper(xy, X_off, Y_off):
Image = Band.weight(201,757,5000,5000, fill_length=7, offset_X = X_off, offset_Y = Y_off, rand_tup=(300,0,2000))
print X_off, Y_off
return Image.flatten()
Below I set some initial parameters for X_off, Y_off (the X/Y offsets, where X and Y are a quasi-Cartesian grid based on the satellite position). I also import a 'real' image which I will use as my dependent variable - essentially I'm asking this program to spit out optimal parameters such that the simulation will produce the closest image possible to this 'real' one.
p0 = [-14,4]
Real_image = Band.Im[some_range]
Notice I have 'xy' defined in my wrapper function. This is actually pretty useless, it's only there so I can say I have an independent variable - in reality I don't! The simulation doesn't really have an independent variable but I still want to find a way to optimize it and thought this would work.
xy = np.zeros(10)
optimal_params, covariance = curve_fit(wrapper, xy, F1_portion.flatten(), p0)
The results of the final line are always that optimal_params is incredibly similar to the initial guess p0, maybe off by a small amount. Upon closer study it seems that the algorithm doesn't vary the parameters by very much at all. I want it to vary the parameters in at least integer steps, and I'm not sure how I can help do this.
Essentially, I almost always get my initial parameters returned to me. Is this an issue with the algorithm or my code? Is there another algorithm I can use to find the optimal parameters?
Thanks
arrays python-2.7 scipy least-squares
arrays python-2.7 scipy least-squares
edited Nov 26 '18 at 18:23
Yewdent Niedtaknow
asked Nov 23 '18 at 17:37


Yewdent NiedtaknowYewdent Niedtaknow
185
185
1
FYI: The function that you passed toleastsq
must return all the residuals, not the sum of the residuals.
– Warren Weckesser
Nov 23 '18 at 18:05
add a comment |
1
FYI: The function that you passed toleastsq
must return all the residuals, not the sum of the residuals.
– Warren Weckesser
Nov 23 '18 at 18:05
1
1
FYI: The function that you passed to
leastsq
must return all the residuals, not the sum of the residuals.– Warren Weckesser
Nov 23 '18 at 18:05
FYI: The function that you passed to
leastsq
must return all the residuals, not the sum of the residuals.– Warren Weckesser
Nov 23 '18 at 18:05
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450958%2fhow-to-fit-a-model-to-an-image-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450958%2fhow-to-fit-a-model-to-an-image-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
k6X8,lhDNQD64A0Jt73rocoHy43T,vNeeuGwyT2Z8k1JoJ94WSx4
1
FYI: The function that you passed to
leastsq
must return all the residuals, not the sum of the residuals.– Warren Weckesser
Nov 23 '18 at 18:05