React with Redux, component unable to recognize a property passed from a parent
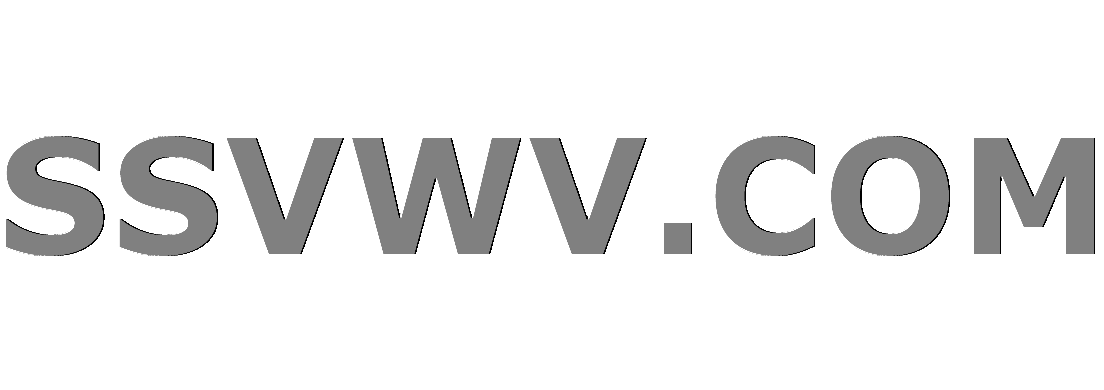
Multi tool use
up vote
0
down vote
favorite
Kindly go over the code below, if its not clear enough, Here is a codesandbox for an extended version of my issue,
so I am passing some properties from a parent to a child comp., the parent is my <Main />
comp., which is wrapped in a <Provider>
comp. to allow it to access the store. Now, in the child, I have a function that renders info based on the passed props from the parent, here is my child comp. code
//Home Component
...
const RenderCard = ({item, isLoading, errMess}) => {
console.log(item.name)
if(isLoading) {
return(
<Loading />
)
}
else if (errMess) {
return (
<h4>{errMess}</h4>
)
}
else {
return(
<h1>{item.name}</h1>
)
}
}
const Home = props => {
return (
<RenderCard item={props.dish} isLoading={props.dishesLoading} errMess={props.dishesErrMess} />
)
}
...
Here is how I pass the prop from the parent,
const mapStateToProps = state => {
return {
dishes: state.dishes,
comments: state.comments,
leaders: state.leaders,
promotions: state.promotions
}
}
const mapDispatchToProps = dispatch => ({
addComment: (dishId, rating, author, comment) => dispatch(addComment(dishId, rating, author, comment)),
fetchDishes: () => {
dispatch(fetchDishes())
}
})
class Main extends Component {
constructor(props) {
super(props)
}
componentDidMount() {
this.props.fetchDishes()
console.log(this.props.dishes.dishes.filter(dish => dish.featured)[0])
}
render() {
const HomePage = () => {
<Home dish = {this.props.dishes.dishes.filter(dish => dish.featured)[0]} />
}
}
}
export default withRouter(connect(mapStateToProps, mapDispatchToProps)(Main));
the strange part is, in the first code snippet, line 4, the console.log(..) msg, successfully logs the desired info to the console, which means the props are passed and reached to the <RenderCard />
comp. However, instead of rendering it on the window, I get a TypeError Cannot read property name of undefined.
please leave a comment below if any further parts of the code are needed.
thanks in advance.
javascript reactjs redux react-redux
add a comment |
up vote
0
down vote
favorite
Kindly go over the code below, if its not clear enough, Here is a codesandbox for an extended version of my issue,
so I am passing some properties from a parent to a child comp., the parent is my <Main />
comp., which is wrapped in a <Provider>
comp. to allow it to access the store. Now, in the child, I have a function that renders info based on the passed props from the parent, here is my child comp. code
//Home Component
...
const RenderCard = ({item, isLoading, errMess}) => {
console.log(item.name)
if(isLoading) {
return(
<Loading />
)
}
else if (errMess) {
return (
<h4>{errMess}</h4>
)
}
else {
return(
<h1>{item.name}</h1>
)
}
}
const Home = props => {
return (
<RenderCard item={props.dish} isLoading={props.dishesLoading} errMess={props.dishesErrMess} />
)
}
...
Here is how I pass the prop from the parent,
const mapStateToProps = state => {
return {
dishes: state.dishes,
comments: state.comments,
leaders: state.leaders,
promotions: state.promotions
}
}
const mapDispatchToProps = dispatch => ({
addComment: (dishId, rating, author, comment) => dispatch(addComment(dishId, rating, author, comment)),
fetchDishes: () => {
dispatch(fetchDishes())
}
})
class Main extends Component {
constructor(props) {
super(props)
}
componentDidMount() {
this.props.fetchDishes()
console.log(this.props.dishes.dishes.filter(dish => dish.featured)[0])
}
render() {
const HomePage = () => {
<Home dish = {this.props.dishes.dishes.filter(dish => dish.featured)[0]} />
}
}
}
export default withRouter(connect(mapStateToProps, mapDispatchToProps)(Main));
the strange part is, in the first code snippet, line 4, the console.log(..) msg, successfully logs the desired info to the console, which means the props are passed and reached to the <RenderCard />
comp. However, instead of rendering it on the window, I get a TypeError Cannot read property name of undefined.
please leave a comment below if any further parts of the code are needed.
thanks in advance.
javascript reactjs redux react-redux
About style: You don't needreturn
statement after arrow if you only return something, it don't help reading. Useprop-types
library and catch place where first you get wrong type ofitem
. Use unit tests like jest - it's really easy to use it and you don't waste time on such questions.
– Zydnar
Nov 19 at 11:30
If you don't want to useprop-types
library use at least js docs for better IDE support.
– Zydnar
Nov 19 at 11:32
thanks for your comment, But I am only using the return when conditionally rendering something based on the props passed from the parent. regarding the jest testing, well, I need to get this problem solved for now and then I will consider looking into unit testing
– MoSwilam
Nov 19 at 11:50
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Kindly go over the code below, if its not clear enough, Here is a codesandbox for an extended version of my issue,
so I am passing some properties from a parent to a child comp., the parent is my <Main />
comp., which is wrapped in a <Provider>
comp. to allow it to access the store. Now, in the child, I have a function that renders info based on the passed props from the parent, here is my child comp. code
//Home Component
...
const RenderCard = ({item, isLoading, errMess}) => {
console.log(item.name)
if(isLoading) {
return(
<Loading />
)
}
else if (errMess) {
return (
<h4>{errMess}</h4>
)
}
else {
return(
<h1>{item.name}</h1>
)
}
}
const Home = props => {
return (
<RenderCard item={props.dish} isLoading={props.dishesLoading} errMess={props.dishesErrMess} />
)
}
...
Here is how I pass the prop from the parent,
const mapStateToProps = state => {
return {
dishes: state.dishes,
comments: state.comments,
leaders: state.leaders,
promotions: state.promotions
}
}
const mapDispatchToProps = dispatch => ({
addComment: (dishId, rating, author, comment) => dispatch(addComment(dishId, rating, author, comment)),
fetchDishes: () => {
dispatch(fetchDishes())
}
})
class Main extends Component {
constructor(props) {
super(props)
}
componentDidMount() {
this.props.fetchDishes()
console.log(this.props.dishes.dishes.filter(dish => dish.featured)[0])
}
render() {
const HomePage = () => {
<Home dish = {this.props.dishes.dishes.filter(dish => dish.featured)[0]} />
}
}
}
export default withRouter(connect(mapStateToProps, mapDispatchToProps)(Main));
the strange part is, in the first code snippet, line 4, the console.log(..) msg, successfully logs the desired info to the console, which means the props are passed and reached to the <RenderCard />
comp. However, instead of rendering it on the window, I get a TypeError Cannot read property name of undefined.
please leave a comment below if any further parts of the code are needed.
thanks in advance.
javascript reactjs redux react-redux
Kindly go over the code below, if its not clear enough, Here is a codesandbox for an extended version of my issue,
so I am passing some properties from a parent to a child comp., the parent is my <Main />
comp., which is wrapped in a <Provider>
comp. to allow it to access the store. Now, in the child, I have a function that renders info based on the passed props from the parent, here is my child comp. code
//Home Component
...
const RenderCard = ({item, isLoading, errMess}) => {
console.log(item.name)
if(isLoading) {
return(
<Loading />
)
}
else if (errMess) {
return (
<h4>{errMess}</h4>
)
}
else {
return(
<h1>{item.name}</h1>
)
}
}
const Home = props => {
return (
<RenderCard item={props.dish} isLoading={props.dishesLoading} errMess={props.dishesErrMess} />
)
}
...
Here is how I pass the prop from the parent,
const mapStateToProps = state => {
return {
dishes: state.dishes,
comments: state.comments,
leaders: state.leaders,
promotions: state.promotions
}
}
const mapDispatchToProps = dispatch => ({
addComment: (dishId, rating, author, comment) => dispatch(addComment(dishId, rating, author, comment)),
fetchDishes: () => {
dispatch(fetchDishes())
}
})
class Main extends Component {
constructor(props) {
super(props)
}
componentDidMount() {
this.props.fetchDishes()
console.log(this.props.dishes.dishes.filter(dish => dish.featured)[0])
}
render() {
const HomePage = () => {
<Home dish = {this.props.dishes.dishes.filter(dish => dish.featured)[0]} />
}
}
}
export default withRouter(connect(mapStateToProps, mapDispatchToProps)(Main));
the strange part is, in the first code snippet, line 4, the console.log(..) msg, successfully logs the desired info to the console, which means the props are passed and reached to the <RenderCard />
comp. However, instead of rendering it on the window, I get a TypeError Cannot read property name of undefined.
please leave a comment below if any further parts of the code are needed.
thanks in advance.
//Home Component
...
const RenderCard = ({item, isLoading, errMess}) => {
console.log(item.name)
if(isLoading) {
return(
<Loading />
)
}
else if (errMess) {
return (
<h4>{errMess}</h4>
)
}
else {
return(
<h1>{item.name}</h1>
)
}
}
const Home = props => {
return (
<RenderCard item={props.dish} isLoading={props.dishesLoading} errMess={props.dishesErrMess} />
)
}
...
//Home Component
...
const RenderCard = ({item, isLoading, errMess}) => {
console.log(item.name)
if(isLoading) {
return(
<Loading />
)
}
else if (errMess) {
return (
<h4>{errMess}</h4>
)
}
else {
return(
<h1>{item.name}</h1>
)
}
}
const Home = props => {
return (
<RenderCard item={props.dish} isLoading={props.dishesLoading} errMess={props.dishesErrMess} />
)
}
...
const mapStateToProps = state => {
return {
dishes: state.dishes,
comments: state.comments,
leaders: state.leaders,
promotions: state.promotions
}
}
const mapDispatchToProps = dispatch => ({
addComment: (dishId, rating, author, comment) => dispatch(addComment(dishId, rating, author, comment)),
fetchDishes: () => {
dispatch(fetchDishes())
}
})
class Main extends Component {
constructor(props) {
super(props)
}
componentDidMount() {
this.props.fetchDishes()
console.log(this.props.dishes.dishes.filter(dish => dish.featured)[0])
}
render() {
const HomePage = () => {
<Home dish = {this.props.dishes.dishes.filter(dish => dish.featured)[0]} />
}
}
}
export default withRouter(connect(mapStateToProps, mapDispatchToProps)(Main));
const mapStateToProps = state => {
return {
dishes: state.dishes,
comments: state.comments,
leaders: state.leaders,
promotions: state.promotions
}
}
const mapDispatchToProps = dispatch => ({
addComment: (dishId, rating, author, comment) => dispatch(addComment(dishId, rating, author, comment)),
fetchDishes: () => {
dispatch(fetchDishes())
}
})
class Main extends Component {
constructor(props) {
super(props)
}
componentDidMount() {
this.props.fetchDishes()
console.log(this.props.dishes.dishes.filter(dish => dish.featured)[0])
}
render() {
const HomePage = () => {
<Home dish = {this.props.dishes.dishes.filter(dish => dish.featured)[0]} />
}
}
}
export default withRouter(connect(mapStateToProps, mapDispatchToProps)(Main));
javascript reactjs redux react-redux
javascript reactjs redux react-redux
asked Nov 19 at 11:15
MoSwilam
418
418
About style: You don't needreturn
statement after arrow if you only return something, it don't help reading. Useprop-types
library and catch place where first you get wrong type ofitem
. Use unit tests like jest - it's really easy to use it and you don't waste time on such questions.
– Zydnar
Nov 19 at 11:30
If you don't want to useprop-types
library use at least js docs for better IDE support.
– Zydnar
Nov 19 at 11:32
thanks for your comment, But I am only using the return when conditionally rendering something based on the props passed from the parent. regarding the jest testing, well, I need to get this problem solved for now and then I will consider looking into unit testing
– MoSwilam
Nov 19 at 11:50
add a comment |
About style: You don't needreturn
statement after arrow if you only return something, it don't help reading. Useprop-types
library and catch place where first you get wrong type ofitem
. Use unit tests like jest - it's really easy to use it and you don't waste time on such questions.
– Zydnar
Nov 19 at 11:30
If you don't want to useprop-types
library use at least js docs for better IDE support.
– Zydnar
Nov 19 at 11:32
thanks for your comment, But I am only using the return when conditionally rendering something based on the props passed from the parent. regarding the jest testing, well, I need to get this problem solved for now and then I will consider looking into unit testing
– MoSwilam
Nov 19 at 11:50
About style: You don't need
return
statement after arrow if you only return something, it don't help reading. Use prop-types
library and catch place where first you get wrong type of item
. Use unit tests like jest - it's really easy to use it and you don't waste time on such questions.– Zydnar
Nov 19 at 11:30
About style: You don't need
return
statement after arrow if you only return something, it don't help reading. Use prop-types
library and catch place where first you get wrong type of item
. Use unit tests like jest - it's really easy to use it and you don't waste time on such questions.– Zydnar
Nov 19 at 11:30
If you don't want to use
prop-types
library use at least js docs for better IDE support.– Zydnar
Nov 19 at 11:32
If you don't want to use
prop-types
library use at least js docs for better IDE support.– Zydnar
Nov 19 at 11:32
thanks for your comment, But I am only using the return when conditionally rendering something based on the props passed from the parent. regarding the jest testing, well, I need to get this problem solved for now and then I will consider looking into unit testing
– MoSwilam
Nov 19 at 11:50
thanks for your comment, But I am only using the return when conditionally rendering something based on the props passed from the parent. regarding the jest testing, well, I need to get this problem solved for now and then I will consider looking into unit testing
– MoSwilam
Nov 19 at 11:50
add a comment |
2 Answers
2
active
oldest
votes
up vote
2
down vote
PRoblem is that in your main component you are passing the dishesLoading prop to the Home component incorrectly. You need to access it from props with the name isLoading
and not dishesLoading
const HomePage = () => {
return (
<Home
dish={this.props.dishes.dishes.filter(dish => dish.featured)[0]}
dishesLoading={this.props.dishes.isLoading}
dishesErrMess={this.props.dishes.errMess}
/>
);
};
Working sandbox
Also in the RenderCard
component you shouldn't access item.name
before the data is loaded since initially its undefined
in console.log(item.name)
thanks very much, its working now, thanks again for your time :)
– MoSwilam
Nov 19 at 12:06
Glad to have helped
– Shubham Khatri
Nov 19 at 12:17
add a comment |
up vote
2
down vote
Problem is, that you're not returning any component in your Main
component's render method. You've declared a method HomePage
instead.
Refer the code below. This should work.
UPDATE:
Here's the sandbox that works: https://codesandbox.io/s/8nopnq3jo0
You were trying to render before the dishes loaded. Hence, added a condition check if the dishes were loaded or not.
function Home(props) {
return (
!props.dishesLoading && (
<div className="container">
<div className="row align-items-start">
<div className="col-12 col-md m-1">
<RenderCard
item={props.dish}
isLoading={props.dishesLoading}
errMess={props.dishesErrMess}
/>
</div>
</div>
</div>
)
);
}
could you please recheck the codesandbox, I initially forgot to add the <Redirect> to the <switch>, now its added since I am using the router to render the home if no routes are selected. thanks
– MoSwilam
Nov 19 at 11:48
Updated my answer
– Vishal Sharma
Nov 19 at 12:04
thanks very much Vishal, Problem solved brother :)
– MoSwilam
Nov 19 at 12:07
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
PRoblem is that in your main component you are passing the dishesLoading prop to the Home component incorrectly. You need to access it from props with the name isLoading
and not dishesLoading
const HomePage = () => {
return (
<Home
dish={this.props.dishes.dishes.filter(dish => dish.featured)[0]}
dishesLoading={this.props.dishes.isLoading}
dishesErrMess={this.props.dishes.errMess}
/>
);
};
Working sandbox
Also in the RenderCard
component you shouldn't access item.name
before the data is loaded since initially its undefined
in console.log(item.name)
thanks very much, its working now, thanks again for your time :)
– MoSwilam
Nov 19 at 12:06
Glad to have helped
– Shubham Khatri
Nov 19 at 12:17
add a comment |
up vote
2
down vote
PRoblem is that in your main component you are passing the dishesLoading prop to the Home component incorrectly. You need to access it from props with the name isLoading
and not dishesLoading
const HomePage = () => {
return (
<Home
dish={this.props.dishes.dishes.filter(dish => dish.featured)[0]}
dishesLoading={this.props.dishes.isLoading}
dishesErrMess={this.props.dishes.errMess}
/>
);
};
Working sandbox
Also in the RenderCard
component you shouldn't access item.name
before the data is loaded since initially its undefined
in console.log(item.name)
thanks very much, its working now, thanks again for your time :)
– MoSwilam
Nov 19 at 12:06
Glad to have helped
– Shubham Khatri
Nov 19 at 12:17
add a comment |
up vote
2
down vote
up vote
2
down vote
PRoblem is that in your main component you are passing the dishesLoading prop to the Home component incorrectly. You need to access it from props with the name isLoading
and not dishesLoading
const HomePage = () => {
return (
<Home
dish={this.props.dishes.dishes.filter(dish => dish.featured)[0]}
dishesLoading={this.props.dishes.isLoading}
dishesErrMess={this.props.dishes.errMess}
/>
);
};
Working sandbox
Also in the RenderCard
component you shouldn't access item.name
before the data is loaded since initially its undefined
in console.log(item.name)
PRoblem is that in your main component you are passing the dishesLoading prop to the Home component incorrectly. You need to access it from props with the name isLoading
and not dishesLoading
const HomePage = () => {
return (
<Home
dish={this.props.dishes.dishes.filter(dish => dish.featured)[0]}
dishesLoading={this.props.dishes.isLoading}
dishesErrMess={this.props.dishes.errMess}
/>
);
};
Working sandbox
Also in the RenderCard
component you shouldn't access item.name
before the data is loaded since initially its undefined
in console.log(item.name)
answered Nov 19 at 11:50


Shubham Khatri
74.6k1384123
74.6k1384123
thanks very much, its working now, thanks again for your time :)
– MoSwilam
Nov 19 at 12:06
Glad to have helped
– Shubham Khatri
Nov 19 at 12:17
add a comment |
thanks very much, its working now, thanks again for your time :)
– MoSwilam
Nov 19 at 12:06
Glad to have helped
– Shubham Khatri
Nov 19 at 12:17
thanks very much, its working now, thanks again for your time :)
– MoSwilam
Nov 19 at 12:06
thanks very much, its working now, thanks again for your time :)
– MoSwilam
Nov 19 at 12:06
Glad to have helped
– Shubham Khatri
Nov 19 at 12:17
Glad to have helped
– Shubham Khatri
Nov 19 at 12:17
add a comment |
up vote
2
down vote
Problem is, that you're not returning any component in your Main
component's render method. You've declared a method HomePage
instead.
Refer the code below. This should work.
UPDATE:
Here's the sandbox that works: https://codesandbox.io/s/8nopnq3jo0
You were trying to render before the dishes loaded. Hence, added a condition check if the dishes were loaded or not.
function Home(props) {
return (
!props.dishesLoading && (
<div className="container">
<div className="row align-items-start">
<div className="col-12 col-md m-1">
<RenderCard
item={props.dish}
isLoading={props.dishesLoading}
errMess={props.dishesErrMess}
/>
</div>
</div>
</div>
)
);
}
could you please recheck the codesandbox, I initially forgot to add the <Redirect> to the <switch>, now its added since I am using the router to render the home if no routes are selected. thanks
– MoSwilam
Nov 19 at 11:48
Updated my answer
– Vishal Sharma
Nov 19 at 12:04
thanks very much Vishal, Problem solved brother :)
– MoSwilam
Nov 19 at 12:07
add a comment |
up vote
2
down vote
Problem is, that you're not returning any component in your Main
component's render method. You've declared a method HomePage
instead.
Refer the code below. This should work.
UPDATE:
Here's the sandbox that works: https://codesandbox.io/s/8nopnq3jo0
You were trying to render before the dishes loaded. Hence, added a condition check if the dishes were loaded or not.
function Home(props) {
return (
!props.dishesLoading && (
<div className="container">
<div className="row align-items-start">
<div className="col-12 col-md m-1">
<RenderCard
item={props.dish}
isLoading={props.dishesLoading}
errMess={props.dishesErrMess}
/>
</div>
</div>
</div>
)
);
}
could you please recheck the codesandbox, I initially forgot to add the <Redirect> to the <switch>, now its added since I am using the router to render the home if no routes are selected. thanks
– MoSwilam
Nov 19 at 11:48
Updated my answer
– Vishal Sharma
Nov 19 at 12:04
thanks very much Vishal, Problem solved brother :)
– MoSwilam
Nov 19 at 12:07
add a comment |
up vote
2
down vote
up vote
2
down vote
Problem is, that you're not returning any component in your Main
component's render method. You've declared a method HomePage
instead.
Refer the code below. This should work.
UPDATE:
Here's the sandbox that works: https://codesandbox.io/s/8nopnq3jo0
You were trying to render before the dishes loaded. Hence, added a condition check if the dishes were loaded or not.
function Home(props) {
return (
!props.dishesLoading && (
<div className="container">
<div className="row align-items-start">
<div className="col-12 col-md m-1">
<RenderCard
item={props.dish}
isLoading={props.dishesLoading}
errMess={props.dishesErrMess}
/>
</div>
</div>
</div>
)
);
}
Problem is, that you're not returning any component in your Main
component's render method. You've declared a method HomePage
instead.
Refer the code below. This should work.
UPDATE:
Here's the sandbox that works: https://codesandbox.io/s/8nopnq3jo0
You were trying to render before the dishes loaded. Hence, added a condition check if the dishes were loaded or not.
function Home(props) {
return (
!props.dishesLoading && (
<div className="container">
<div className="row align-items-start">
<div className="col-12 col-md m-1">
<RenderCard
item={props.dish}
isLoading={props.dishesLoading}
errMess={props.dishesErrMess}
/>
</div>
</div>
</div>
)
);
}
edited Nov 19 at 12:03
answered Nov 19 at 11:39


Vishal Sharma
1,255927
1,255927
could you please recheck the codesandbox, I initially forgot to add the <Redirect> to the <switch>, now its added since I am using the router to render the home if no routes are selected. thanks
– MoSwilam
Nov 19 at 11:48
Updated my answer
– Vishal Sharma
Nov 19 at 12:04
thanks very much Vishal, Problem solved brother :)
– MoSwilam
Nov 19 at 12:07
add a comment |
could you please recheck the codesandbox, I initially forgot to add the <Redirect> to the <switch>, now its added since I am using the router to render the home if no routes are selected. thanks
– MoSwilam
Nov 19 at 11:48
Updated my answer
– Vishal Sharma
Nov 19 at 12:04
thanks very much Vishal, Problem solved brother :)
– MoSwilam
Nov 19 at 12:07
could you please recheck the codesandbox, I initially forgot to add the <Redirect> to the <switch>, now its added since I am using the router to render the home if no routes are selected. thanks
– MoSwilam
Nov 19 at 11:48
could you please recheck the codesandbox, I initially forgot to add the <Redirect> to the <switch>, now its added since I am using the router to render the home if no routes are selected. thanks
– MoSwilam
Nov 19 at 11:48
Updated my answer
– Vishal Sharma
Nov 19 at 12:04
Updated my answer
– Vishal Sharma
Nov 19 at 12:04
thanks very much Vishal, Problem solved brother :)
– MoSwilam
Nov 19 at 12:07
thanks very much Vishal, Problem solved brother :)
– MoSwilam
Nov 19 at 12:07
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53373449%2freact-with-redux-component-unable-to-recognize-a-property-passed-from-a-parent%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bHg,CEZUgKOzf3gVqda3hMMvsq2k,MIbQsa,eAps,UNoXxc9XDJ
About style: You don't need
return
statement after arrow if you only return something, it don't help reading. Useprop-types
library and catch place where first you get wrong type ofitem
. Use unit tests like jest - it's really easy to use it and you don't waste time on such questions.– Zydnar
Nov 19 at 11:30
If you don't want to use
prop-types
library use at least js docs for better IDE support.– Zydnar
Nov 19 at 11:32
thanks for your comment, But I am only using the return when conditionally rendering something based on the props passed from the parent. regarding the jest testing, well, I need to get this problem solved for now and then I will consider looking into unit testing
– MoSwilam
Nov 19 at 11:50