Return results mongoose in find query to a variable
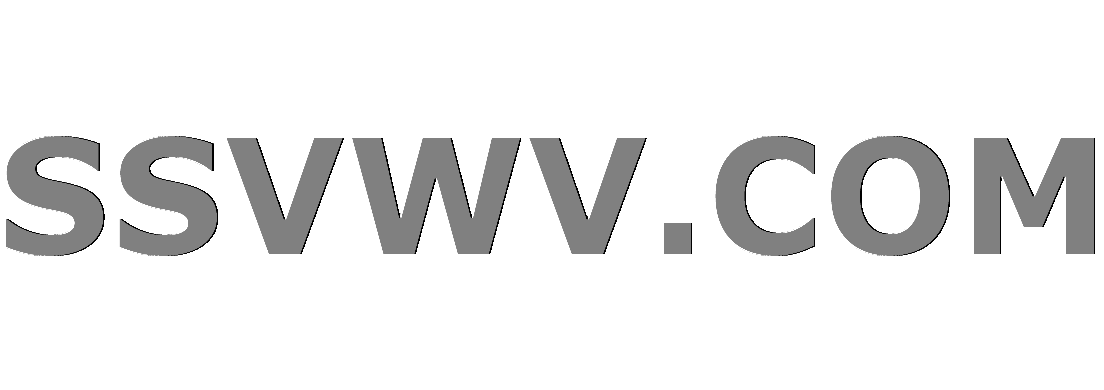
Multi tool use
up vote
20
down vote
favorite
I need to return the results of a query with mongoose in node.js.
How do you return the value to set the value to a variable?
What I need to do is:
var results = users.findOne({_id : users_list[i]['user_id']},{email : 1, credits : 1},{}, function(err, docs) {
if( err || !docs) {
console.log("No user found");
} else {
return docs;
};
});
In order to have:
results = docs
Thanks a lot for your reply .
I also have another problem.
How to pass variable in a query operator with find or findOne? Like :
var foo = "Blaa";
users.findOne({_id : users_list[i]['user_id']},{email : 1, credits : 1},{}, function(err, docs) {
if( err || !docs) {
console.log("No user found");
} else {
// I want to use the foo variable here
console.log(foo);
};
});
node.js mongodb mongoose mongodb-query
add a comment |
up vote
20
down vote
favorite
I need to return the results of a query with mongoose in node.js.
How do you return the value to set the value to a variable?
What I need to do is:
var results = users.findOne({_id : users_list[i]['user_id']},{email : 1, credits : 1},{}, function(err, docs) {
if( err || !docs) {
console.log("No user found");
} else {
return docs;
};
});
In order to have:
results = docs
Thanks a lot for your reply .
I also have another problem.
How to pass variable in a query operator with find or findOne? Like :
var foo = "Blaa";
users.findOne({_id : users_list[i]['user_id']},{email : 1, credits : 1},{}, function(err, docs) {
if( err || !docs) {
console.log("No user found");
} else {
// I want to use the foo variable here
console.log(foo);
};
});
node.js mongodb mongoose mongodb-query
add a comment |
up vote
20
down vote
favorite
up vote
20
down vote
favorite
I need to return the results of a query with mongoose in node.js.
How do you return the value to set the value to a variable?
What I need to do is:
var results = users.findOne({_id : users_list[i]['user_id']},{email : 1, credits : 1},{}, function(err, docs) {
if( err || !docs) {
console.log("No user found");
} else {
return docs;
};
});
In order to have:
results = docs
Thanks a lot for your reply .
I also have another problem.
How to pass variable in a query operator with find or findOne? Like :
var foo = "Blaa";
users.findOne({_id : users_list[i]['user_id']},{email : 1, credits : 1},{}, function(err, docs) {
if( err || !docs) {
console.log("No user found");
} else {
// I want to use the foo variable here
console.log(foo);
};
});
node.js mongodb mongoose mongodb-query
I need to return the results of a query with mongoose in node.js.
How do you return the value to set the value to a variable?
What I need to do is:
var results = users.findOne({_id : users_list[i]['user_id']},{email : 1, credits : 1},{}, function(err, docs) {
if( err || !docs) {
console.log("No user found");
} else {
return docs;
};
});
In order to have:
results = docs
Thanks a lot for your reply .
I also have another problem.
How to pass variable in a query operator with find or findOne? Like :
var foo = "Blaa";
users.findOne({_id : users_list[i]['user_id']},{email : 1, credits : 1},{}, function(err, docs) {
if( err || !docs) {
console.log("No user found");
} else {
// I want to use the foo variable here
console.log(foo);
};
});
node.js mongodb mongoose mongodb-query
node.js mongodb mongoose mongodb-query
edited Jun 4 '14 at 13:24


Julien Le Coupanec
2,71313045
2,71313045
asked Jun 4 '14 at 11:10


Xanarus
4961315
4961315
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
up vote
58
down vote
accepted
There are several ways to achieve what you want.
1. Using Mongoose Queries
In this strategy, your function returns a Mongoose query which you can later use to invoke the method exec
and use it to get the results.
function getJedisQuery(name){
var query = Jedi.find({name:name});
return query;
}
Then you can use it simply doing:
var query = getJedisQuery('Obi-wan');
query.exec(function(err,jedis){
if(err)
return console.log(err);
jedis.forEach(function(jedi){
console.log(jedi.name);
});
});
2. Using Mongoose Promises
Moogose provides support for promises. All you have to do is something somewhat similar to what I did above, but this time, you invoke the exec
method without a callback.
function getJedisPromise(name){
var promise = Jedi.find({name:name}).exec();
return promise;
}
Then you can use it by simply doing:
var promise = getJedisPromise('Luke');
promise.then(function(jedis){
jedis.forEach(function(jedi){
console.log(jedi.name);
});
}).error(function(error){
console.log(error);
});
3. Using Mongoose Streams
Finally, Mongoose has also support for streams and streams are event emitters. So, you could get a stream and then subscribe for 'data' and 'error' events. Like this:
function getjedisStream(name){
var stream = Jedi.find({name:name}).stream();
return stream;
}
Then you can simply do:
var stream = getJedisStream('Anakin');
stream.on('data', function(jedis){
jedis.forEach(function(jedi){
console.log(jedi.name);
});
});
stream.on('error', function(error){
console.log(error);
});
Source, for future reference.
2
One small edit to your answer. There is no .error function in mongoose promises. It gives the following error when I use it - TypeError: promise.then(...).error is not a function nodejs If we want error handling then we should do something like mongoose.Promise = require('q').Promise; and then use the function .catch(function(err) {
– vivekp
Mar 14 '16 at 8:32
5
I know OP was happy with the answer, but you're not actually explaining how to put the result in aresults
variable that can be used in the rest of the code.
– David Ammouial
May 19 '17 at 0:53
@vivekp: I'm also getting same TypeError. Kindly update the working code here
– sankar muniyappa
Jun 9 '17 at 11:04
add a comment |
up vote
5
down vote
it is being executed before the assignment.
async function(req, res) {
var user;
await users.findOne({}, function(err,pro){
user=pro;
});
console.log(user); \ it's define
});
add a comment |
up vote
0
down vote
You achieve the desired result by the following code. Hope this will helps you a lot..
var async = require('async');
// custom imports
var User = require('../models/user');
var Article = require('../models/article');
var List1Objects = User.find({});
var List2Objects = Article.find({});
var resourcesStack = {
usersList: List1Objects.exec.bind(List1Objects),
articlesList: List2Objects.exec.bind(List2Objects),
};
async.parallel(resourcesStack, function (error, resultSet){
if (error) {
res.status(500).send(error);
return;
}
res.render('home', resultSet);
});
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
58
down vote
accepted
There are several ways to achieve what you want.
1. Using Mongoose Queries
In this strategy, your function returns a Mongoose query which you can later use to invoke the method exec
and use it to get the results.
function getJedisQuery(name){
var query = Jedi.find({name:name});
return query;
}
Then you can use it simply doing:
var query = getJedisQuery('Obi-wan');
query.exec(function(err,jedis){
if(err)
return console.log(err);
jedis.forEach(function(jedi){
console.log(jedi.name);
});
});
2. Using Mongoose Promises
Moogose provides support for promises. All you have to do is something somewhat similar to what I did above, but this time, you invoke the exec
method without a callback.
function getJedisPromise(name){
var promise = Jedi.find({name:name}).exec();
return promise;
}
Then you can use it by simply doing:
var promise = getJedisPromise('Luke');
promise.then(function(jedis){
jedis.forEach(function(jedi){
console.log(jedi.name);
});
}).error(function(error){
console.log(error);
});
3. Using Mongoose Streams
Finally, Mongoose has also support for streams and streams are event emitters. So, you could get a stream and then subscribe for 'data' and 'error' events. Like this:
function getjedisStream(name){
var stream = Jedi.find({name:name}).stream();
return stream;
}
Then you can simply do:
var stream = getJedisStream('Anakin');
stream.on('data', function(jedis){
jedis.forEach(function(jedi){
console.log(jedi.name);
});
});
stream.on('error', function(error){
console.log(error);
});
Source, for future reference.
2
One small edit to your answer. There is no .error function in mongoose promises. It gives the following error when I use it - TypeError: promise.then(...).error is not a function nodejs If we want error handling then we should do something like mongoose.Promise = require('q').Promise; and then use the function .catch(function(err) {
– vivekp
Mar 14 '16 at 8:32
5
I know OP was happy with the answer, but you're not actually explaining how to put the result in aresults
variable that can be used in the rest of the code.
– David Ammouial
May 19 '17 at 0:53
@vivekp: I'm also getting same TypeError. Kindly update the working code here
– sankar muniyappa
Jun 9 '17 at 11:04
add a comment |
up vote
58
down vote
accepted
There are several ways to achieve what you want.
1. Using Mongoose Queries
In this strategy, your function returns a Mongoose query which you can later use to invoke the method exec
and use it to get the results.
function getJedisQuery(name){
var query = Jedi.find({name:name});
return query;
}
Then you can use it simply doing:
var query = getJedisQuery('Obi-wan');
query.exec(function(err,jedis){
if(err)
return console.log(err);
jedis.forEach(function(jedi){
console.log(jedi.name);
});
});
2. Using Mongoose Promises
Moogose provides support for promises. All you have to do is something somewhat similar to what I did above, but this time, you invoke the exec
method without a callback.
function getJedisPromise(name){
var promise = Jedi.find({name:name}).exec();
return promise;
}
Then you can use it by simply doing:
var promise = getJedisPromise('Luke');
promise.then(function(jedis){
jedis.forEach(function(jedi){
console.log(jedi.name);
});
}).error(function(error){
console.log(error);
});
3. Using Mongoose Streams
Finally, Mongoose has also support for streams and streams are event emitters. So, you could get a stream and then subscribe for 'data' and 'error' events. Like this:
function getjedisStream(name){
var stream = Jedi.find({name:name}).stream();
return stream;
}
Then you can simply do:
var stream = getJedisStream('Anakin');
stream.on('data', function(jedis){
jedis.forEach(function(jedi){
console.log(jedi.name);
});
});
stream.on('error', function(error){
console.log(error);
});
Source, for future reference.
2
One small edit to your answer. There is no .error function in mongoose promises. It gives the following error when I use it - TypeError: promise.then(...).error is not a function nodejs If we want error handling then we should do something like mongoose.Promise = require('q').Promise; and then use the function .catch(function(err) {
– vivekp
Mar 14 '16 at 8:32
5
I know OP was happy with the answer, but you're not actually explaining how to put the result in aresults
variable that can be used in the rest of the code.
– David Ammouial
May 19 '17 at 0:53
@vivekp: I'm also getting same TypeError. Kindly update the working code here
– sankar muniyappa
Jun 9 '17 at 11:04
add a comment |
up vote
58
down vote
accepted
up vote
58
down vote
accepted
There are several ways to achieve what you want.
1. Using Mongoose Queries
In this strategy, your function returns a Mongoose query which you can later use to invoke the method exec
and use it to get the results.
function getJedisQuery(name){
var query = Jedi.find({name:name});
return query;
}
Then you can use it simply doing:
var query = getJedisQuery('Obi-wan');
query.exec(function(err,jedis){
if(err)
return console.log(err);
jedis.forEach(function(jedi){
console.log(jedi.name);
});
});
2. Using Mongoose Promises
Moogose provides support for promises. All you have to do is something somewhat similar to what I did above, but this time, you invoke the exec
method without a callback.
function getJedisPromise(name){
var promise = Jedi.find({name:name}).exec();
return promise;
}
Then you can use it by simply doing:
var promise = getJedisPromise('Luke');
promise.then(function(jedis){
jedis.forEach(function(jedi){
console.log(jedi.name);
});
}).error(function(error){
console.log(error);
});
3. Using Mongoose Streams
Finally, Mongoose has also support for streams and streams are event emitters. So, you could get a stream and then subscribe for 'data' and 'error' events. Like this:
function getjedisStream(name){
var stream = Jedi.find({name:name}).stream();
return stream;
}
Then you can simply do:
var stream = getJedisStream('Anakin');
stream.on('data', function(jedis){
jedis.forEach(function(jedi){
console.log(jedi.name);
});
});
stream.on('error', function(error){
console.log(error);
});
Source, for future reference.
There are several ways to achieve what you want.
1. Using Mongoose Queries
In this strategy, your function returns a Mongoose query which you can later use to invoke the method exec
and use it to get the results.
function getJedisQuery(name){
var query = Jedi.find({name:name});
return query;
}
Then you can use it simply doing:
var query = getJedisQuery('Obi-wan');
query.exec(function(err,jedis){
if(err)
return console.log(err);
jedis.forEach(function(jedi){
console.log(jedi.name);
});
});
2. Using Mongoose Promises
Moogose provides support for promises. All you have to do is something somewhat similar to what I did above, but this time, you invoke the exec
method without a callback.
function getJedisPromise(name){
var promise = Jedi.find({name:name}).exec();
return promise;
}
Then you can use it by simply doing:
var promise = getJedisPromise('Luke');
promise.then(function(jedis){
jedis.forEach(function(jedi){
console.log(jedi.name);
});
}).error(function(error){
console.log(error);
});
3. Using Mongoose Streams
Finally, Mongoose has also support for streams and streams are event emitters. So, you could get a stream and then subscribe for 'data' and 'error' events. Like this:
function getjedisStream(name){
var stream = Jedi.find({name:name}).stream();
return stream;
}
Then you can simply do:
var stream = getJedisStream('Anakin');
stream.on('data', function(jedis){
jedis.forEach(function(jedi){
console.log(jedi.name);
});
});
stream.on('error', function(error){
console.log(error);
});
Source, for future reference.
edited Jul 17 '16 at 20:48
wuhkuh
4210
4210
answered Jun 4 '14 at 12:51
Edwin Dalorzo
53.9k20110163
53.9k20110163
2
One small edit to your answer. There is no .error function in mongoose promises. It gives the following error when I use it - TypeError: promise.then(...).error is not a function nodejs If we want error handling then we should do something like mongoose.Promise = require('q').Promise; and then use the function .catch(function(err) {
– vivekp
Mar 14 '16 at 8:32
5
I know OP was happy with the answer, but you're not actually explaining how to put the result in aresults
variable that can be used in the rest of the code.
– David Ammouial
May 19 '17 at 0:53
@vivekp: I'm also getting same TypeError. Kindly update the working code here
– sankar muniyappa
Jun 9 '17 at 11:04
add a comment |
2
One small edit to your answer. There is no .error function in mongoose promises. It gives the following error when I use it - TypeError: promise.then(...).error is not a function nodejs If we want error handling then we should do something like mongoose.Promise = require('q').Promise; and then use the function .catch(function(err) {
– vivekp
Mar 14 '16 at 8:32
5
I know OP was happy with the answer, but you're not actually explaining how to put the result in aresults
variable that can be used in the rest of the code.
– David Ammouial
May 19 '17 at 0:53
@vivekp: I'm also getting same TypeError. Kindly update the working code here
– sankar muniyappa
Jun 9 '17 at 11:04
2
2
One small edit to your answer. There is no .error function in mongoose promises. It gives the following error when I use it - TypeError: promise.then(...).error is not a function nodejs If we want error handling then we should do something like mongoose.Promise = require('q').Promise; and then use the function .catch(function(err) {
– vivekp
Mar 14 '16 at 8:32
One small edit to your answer. There is no .error function in mongoose promises. It gives the following error when I use it - TypeError: promise.then(...).error is not a function nodejs If we want error handling then we should do something like mongoose.Promise = require('q').Promise; and then use the function .catch(function(err) {
– vivekp
Mar 14 '16 at 8:32
5
5
I know OP was happy with the answer, but you're not actually explaining how to put the result in a
results
variable that can be used in the rest of the code.– David Ammouial
May 19 '17 at 0:53
I know OP was happy with the answer, but you're not actually explaining how to put the result in a
results
variable that can be used in the rest of the code.– David Ammouial
May 19 '17 at 0:53
@vivekp: I'm also getting same TypeError. Kindly update the working code here
– sankar muniyappa
Jun 9 '17 at 11:04
@vivekp: I'm also getting same TypeError. Kindly update the working code here
– sankar muniyappa
Jun 9 '17 at 11:04
add a comment |
up vote
5
down vote
it is being executed before the assignment.
async function(req, res) {
var user;
await users.findOne({}, function(err,pro){
user=pro;
});
console.log(user); \ it's define
});
add a comment |
up vote
5
down vote
it is being executed before the assignment.
async function(req, res) {
var user;
await users.findOne({}, function(err,pro){
user=pro;
});
console.log(user); \ it's define
});
add a comment |
up vote
5
down vote
up vote
5
down vote
it is being executed before the assignment.
async function(req, res) {
var user;
await users.findOne({}, function(err,pro){
user=pro;
});
console.log(user); \ it's define
});
it is being executed before the assignment.
async function(req, res) {
var user;
await users.findOne({}, function(err,pro){
user=pro;
});
console.log(user); \ it's define
});
answered Feb 5 at 12:50


Javad.mrz
5112
5112
add a comment |
add a comment |
up vote
0
down vote
You achieve the desired result by the following code. Hope this will helps you a lot..
var async = require('async');
// custom imports
var User = require('../models/user');
var Article = require('../models/article');
var List1Objects = User.find({});
var List2Objects = Article.find({});
var resourcesStack = {
usersList: List1Objects.exec.bind(List1Objects),
articlesList: List2Objects.exec.bind(List2Objects),
};
async.parallel(resourcesStack, function (error, resultSet){
if (error) {
res.status(500).send(error);
return;
}
res.render('home', resultSet);
});
add a comment |
up vote
0
down vote
You achieve the desired result by the following code. Hope this will helps you a lot..
var async = require('async');
// custom imports
var User = require('../models/user');
var Article = require('../models/article');
var List1Objects = User.find({});
var List2Objects = Article.find({});
var resourcesStack = {
usersList: List1Objects.exec.bind(List1Objects),
articlesList: List2Objects.exec.bind(List2Objects),
};
async.parallel(resourcesStack, function (error, resultSet){
if (error) {
res.status(500).send(error);
return;
}
res.render('home', resultSet);
});
add a comment |
up vote
0
down vote
up vote
0
down vote
You achieve the desired result by the following code. Hope this will helps you a lot..
var async = require('async');
// custom imports
var User = require('../models/user');
var Article = require('../models/article');
var List1Objects = User.find({});
var List2Objects = Article.find({});
var resourcesStack = {
usersList: List1Objects.exec.bind(List1Objects),
articlesList: List2Objects.exec.bind(List2Objects),
};
async.parallel(resourcesStack, function (error, resultSet){
if (error) {
res.status(500).send(error);
return;
}
res.render('home', resultSet);
});
You achieve the desired result by the following code. Hope this will helps you a lot..
var async = require('async');
// custom imports
var User = require('../models/user');
var Article = require('../models/article');
var List1Objects = User.find({});
var List2Objects = Article.find({});
var resourcesStack = {
usersList: List1Objects.exec.bind(List1Objects),
articlesList: List2Objects.exec.bind(List2Objects),
};
async.parallel(resourcesStack, function (error, resultSet){
if (error) {
res.status(500).send(error);
return;
}
res.render('home', resultSet);
});
answered Jun 24 at 18:55


Surender Singh
212
212
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f24035872%2freturn-results-mongoose-in-find-query-to-a-variable%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
PRvToNAJQeBP,pp,b8u1oM5xNWBjuULTLPv NjG4lhoC,e,f