Can not access pixel_array attribute pydicom
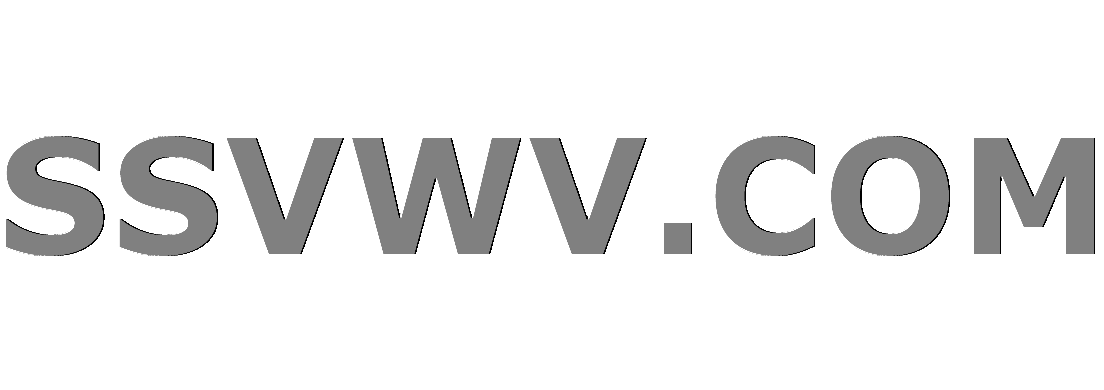
Multi tool use
up vote
1
down vote
favorite
I am trying to do a project. Unfortuantely I can read the dicom files, but when I try to access pixel_array attribute and plot it, it is throwing error.
def readDcm(self):
#path of the decomFolder
print("Path to the DICOM directory is: ",self.dcmPath)
PathDicom = self.dcmPath
lstFilesDCM = # creating an empty list
for dirName, subdirList, fileList in os.walk(PathDicom):
for filename in fileList:
if ".dcm" in filename.lower(): # checking whether the file's DICOM
lstFilesDCM.append(os.path.join(dirName,filename))
print(len(lstFilesDCM))
for filename in lstFilesDCM:
currentDcm = pydicom.read_file(lstFilesDCM[0])
dcm_data = currentDcm.PixelData
pixeldata= currentDcm.pixel_array
the error is:
File "C:Anaconda3libsite-packagespydicompixel_data_handlerspillow_handler.py", line 199, in get_pixeldata
raise NotImplementedError(e.strerror)
NotImplementedError: None
Any suggestion would be nice. Thank you in advance.
Solved Solution:
def load_scan(path):
slices = [dicom.read_file(path + '/' + s) for s in os.listdir(path)]
slices.sort(key = lambda x: float(x.ImagePositionPatient[2]))
pos1 = slices[int(len(slices)/2)].ImagePositionPatient[2]
pos2 = slices[(int(len(slices)/2)) + 1].ImagePositionPatient[2]
diff = pos2 - pos1
if diff > 0:
slices = np.flipud(slices)
try:
slice_thickness = np.abs(slices[0].ImagePositionPatient[2] - slices[1].ImagePositionPatient[2])
except:
slice_thickness = np.abs(slices[0].SliceLocation - slices[1].SliceLocation)
for s in slices:
s.SliceThickness = slice_thickness
return slices
python pydicom
add a comment |
up vote
1
down vote
favorite
I am trying to do a project. Unfortuantely I can read the dicom files, but when I try to access pixel_array attribute and plot it, it is throwing error.
def readDcm(self):
#path of the decomFolder
print("Path to the DICOM directory is: ",self.dcmPath)
PathDicom = self.dcmPath
lstFilesDCM = # creating an empty list
for dirName, subdirList, fileList in os.walk(PathDicom):
for filename in fileList:
if ".dcm" in filename.lower(): # checking whether the file's DICOM
lstFilesDCM.append(os.path.join(dirName,filename))
print(len(lstFilesDCM))
for filename in lstFilesDCM:
currentDcm = pydicom.read_file(lstFilesDCM[0])
dcm_data = currentDcm.PixelData
pixeldata= currentDcm.pixel_array
the error is:
File "C:Anaconda3libsite-packagespydicompixel_data_handlerspillow_handler.py", line 199, in get_pixeldata
raise NotImplementedError(e.strerror)
NotImplementedError: None
Any suggestion would be nice. Thank you in advance.
Solved Solution:
def load_scan(path):
slices = [dicom.read_file(path + '/' + s) for s in os.listdir(path)]
slices.sort(key = lambda x: float(x.ImagePositionPatient[2]))
pos1 = slices[int(len(slices)/2)].ImagePositionPatient[2]
pos2 = slices[(int(len(slices)/2)) + 1].ImagePositionPatient[2]
diff = pos2 - pos1
if diff > 0:
slices = np.flipud(slices)
try:
slice_thickness = np.abs(slices[0].ImagePositionPatient[2] - slices[1].ImagePositionPatient[2])
except:
slice_thickness = np.abs(slices[0].SliceLocation - slices[1].SliceLocation)
for s in slices:
s.SliceThickness = slice_thickness
return slices
python pydicom
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am trying to do a project. Unfortuantely I can read the dicom files, but when I try to access pixel_array attribute and plot it, it is throwing error.
def readDcm(self):
#path of the decomFolder
print("Path to the DICOM directory is: ",self.dcmPath)
PathDicom = self.dcmPath
lstFilesDCM = # creating an empty list
for dirName, subdirList, fileList in os.walk(PathDicom):
for filename in fileList:
if ".dcm" in filename.lower(): # checking whether the file's DICOM
lstFilesDCM.append(os.path.join(dirName,filename))
print(len(lstFilesDCM))
for filename in lstFilesDCM:
currentDcm = pydicom.read_file(lstFilesDCM[0])
dcm_data = currentDcm.PixelData
pixeldata= currentDcm.pixel_array
the error is:
File "C:Anaconda3libsite-packagespydicompixel_data_handlerspillow_handler.py", line 199, in get_pixeldata
raise NotImplementedError(e.strerror)
NotImplementedError: None
Any suggestion would be nice. Thank you in advance.
Solved Solution:
def load_scan(path):
slices = [dicom.read_file(path + '/' + s) for s in os.listdir(path)]
slices.sort(key = lambda x: float(x.ImagePositionPatient[2]))
pos1 = slices[int(len(slices)/2)].ImagePositionPatient[2]
pos2 = slices[(int(len(slices)/2)) + 1].ImagePositionPatient[2]
diff = pos2 - pos1
if diff > 0:
slices = np.flipud(slices)
try:
slice_thickness = np.abs(slices[0].ImagePositionPatient[2] - slices[1].ImagePositionPatient[2])
except:
slice_thickness = np.abs(slices[0].SliceLocation - slices[1].SliceLocation)
for s in slices:
s.SliceThickness = slice_thickness
return slices
python pydicom
I am trying to do a project. Unfortuantely I can read the dicom files, but when I try to access pixel_array attribute and plot it, it is throwing error.
def readDcm(self):
#path of the decomFolder
print("Path to the DICOM directory is: ",self.dcmPath)
PathDicom = self.dcmPath
lstFilesDCM = # creating an empty list
for dirName, subdirList, fileList in os.walk(PathDicom):
for filename in fileList:
if ".dcm" in filename.lower(): # checking whether the file's DICOM
lstFilesDCM.append(os.path.join(dirName,filename))
print(len(lstFilesDCM))
for filename in lstFilesDCM:
currentDcm = pydicom.read_file(lstFilesDCM[0])
dcm_data = currentDcm.PixelData
pixeldata= currentDcm.pixel_array
the error is:
File "C:Anaconda3libsite-packagespydicompixel_data_handlerspillow_handler.py", line 199, in get_pixeldata
raise NotImplementedError(e.strerror)
NotImplementedError: None
Any suggestion would be nice. Thank you in advance.
Solved Solution:
def load_scan(path):
slices = [dicom.read_file(path + '/' + s) for s in os.listdir(path)]
slices.sort(key = lambda x: float(x.ImagePositionPatient[2]))
pos1 = slices[int(len(slices)/2)].ImagePositionPatient[2]
pos2 = slices[(int(len(slices)/2)) + 1].ImagePositionPatient[2]
diff = pos2 - pos1
if diff > 0:
slices = np.flipud(slices)
try:
slice_thickness = np.abs(slices[0].ImagePositionPatient[2] - slices[1].ImagePositionPatient[2])
except:
slice_thickness = np.abs(slices[0].SliceLocation - slices[1].SliceLocation)
for s in slices:
s.SliceThickness = slice_thickness
return slices
python pydicom
python pydicom
edited Nov 30 at 12:25
asked Nov 20 at 8:51


Makau
215
215
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
Without any further context, it's difficult to assert anything. However, when I look at the way you collect the potential DICOM files, you are probably constructing not existing path (it looks like you skip one hierarchy level when joining path parts back; you may want to check that the constructed path actually points to an existing files using os.path.exists()).
You may want to change the iterating part:
for dirName, subdirList, fileList in os.walk(PathDicom):
for filename in fileList:
if ".dcm" in filename.lower(): # checking whether the file's DICOM
lstFilesDCM.append(os.path.join(dirName,filename)) # <-you cut one potential hierarchy level
with something like:
dicom_files =
for d, dirs, files in os.walk('./data/'):
for x in files:
if x.endswith(".png"):
dicom_path = os.path.join(d, x)
if os.path.exists(dicom_path):
dicom_files.append(dicom_path)
I tried, but problem still exists.
– Makau
Nov 20 at 9:31
Just to let you know, I can access other attributes but not pixel_array
– Makau
Nov 20 at 9:38
Can you reproduce the error with the Matplotlib way of displaying the array? pydicom.github.io/pydicom/stable/viewing_images.html
– leoburgy
Nov 20 at 9:42
Yes, I am using exactly the same syntex. Using the test data files, it is ok. But with my data. I get the error.
– Makau
Nov 20 at 9:47
Looking at the source code of pillow_handler.py, it looks like your error is piping when checking the jpeg plugin. Did you check that the jpeg plugin is installed in your environment? Maybe useful: stackoverflow.com/a/10109941/5202571
– leoburgy
Nov 20 at 11:42
|
show 1 more comment
up vote
0
down vote
accepted
I sorted the dicom files according to the axis which was in increasing order by using ImagePositionPatient attribute. Which solved my problem. I was able to access pixel_array attribute and plot. The code is added to original question. I add it below too.
def load_scan(path):
slices = [dicom.read_file(path + '/' + s) for s in os.listdir(path)]
slices.sort(key = lambda x: float(x.ImagePositionPatient[2]))
pos1 = slices[int(len(slices)/2)].ImagePositionPatient[2]
pos2 = slices[(int(len(slices)/2)) + 1].ImagePositionPatient[2]
diff = pos2 - pos1
if diff > 0:
slices = np.flipud(slices)
try:
slice_thickness = np.abs(slices[0].ImagePositionPatient[2] - slices[1].ImagePositionPatient[2])
except:
slice_thickness = np.abs(slices[0].SliceLocation - slices[1].SliceLocation)
for s in slices:
s.SliceThickness = slice_thickness
return slices
code source:https://www.programcreek.com/python/example/97517/dicom.read_file
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53389288%2fcan-not-access-pixel-array-attribute-pydicom%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Without any further context, it's difficult to assert anything. However, when I look at the way you collect the potential DICOM files, you are probably constructing not existing path (it looks like you skip one hierarchy level when joining path parts back; you may want to check that the constructed path actually points to an existing files using os.path.exists()).
You may want to change the iterating part:
for dirName, subdirList, fileList in os.walk(PathDicom):
for filename in fileList:
if ".dcm" in filename.lower(): # checking whether the file's DICOM
lstFilesDCM.append(os.path.join(dirName,filename)) # <-you cut one potential hierarchy level
with something like:
dicom_files =
for d, dirs, files in os.walk('./data/'):
for x in files:
if x.endswith(".png"):
dicom_path = os.path.join(d, x)
if os.path.exists(dicom_path):
dicom_files.append(dicom_path)
I tried, but problem still exists.
– Makau
Nov 20 at 9:31
Just to let you know, I can access other attributes but not pixel_array
– Makau
Nov 20 at 9:38
Can you reproduce the error with the Matplotlib way of displaying the array? pydicom.github.io/pydicom/stable/viewing_images.html
– leoburgy
Nov 20 at 9:42
Yes, I am using exactly the same syntex. Using the test data files, it is ok. But with my data. I get the error.
– Makau
Nov 20 at 9:47
Looking at the source code of pillow_handler.py, it looks like your error is piping when checking the jpeg plugin. Did you check that the jpeg plugin is installed in your environment? Maybe useful: stackoverflow.com/a/10109941/5202571
– leoburgy
Nov 20 at 11:42
|
show 1 more comment
up vote
0
down vote
Without any further context, it's difficult to assert anything. However, when I look at the way you collect the potential DICOM files, you are probably constructing not existing path (it looks like you skip one hierarchy level when joining path parts back; you may want to check that the constructed path actually points to an existing files using os.path.exists()).
You may want to change the iterating part:
for dirName, subdirList, fileList in os.walk(PathDicom):
for filename in fileList:
if ".dcm" in filename.lower(): # checking whether the file's DICOM
lstFilesDCM.append(os.path.join(dirName,filename)) # <-you cut one potential hierarchy level
with something like:
dicom_files =
for d, dirs, files in os.walk('./data/'):
for x in files:
if x.endswith(".png"):
dicom_path = os.path.join(d, x)
if os.path.exists(dicom_path):
dicom_files.append(dicom_path)
I tried, but problem still exists.
– Makau
Nov 20 at 9:31
Just to let you know, I can access other attributes but not pixel_array
– Makau
Nov 20 at 9:38
Can you reproduce the error with the Matplotlib way of displaying the array? pydicom.github.io/pydicom/stable/viewing_images.html
– leoburgy
Nov 20 at 9:42
Yes, I am using exactly the same syntex. Using the test data files, it is ok. But with my data. I get the error.
– Makau
Nov 20 at 9:47
Looking at the source code of pillow_handler.py, it looks like your error is piping when checking the jpeg plugin. Did you check that the jpeg plugin is installed in your environment? Maybe useful: stackoverflow.com/a/10109941/5202571
– leoburgy
Nov 20 at 11:42
|
show 1 more comment
up vote
0
down vote
up vote
0
down vote
Without any further context, it's difficult to assert anything. However, when I look at the way you collect the potential DICOM files, you are probably constructing not existing path (it looks like you skip one hierarchy level when joining path parts back; you may want to check that the constructed path actually points to an existing files using os.path.exists()).
You may want to change the iterating part:
for dirName, subdirList, fileList in os.walk(PathDicom):
for filename in fileList:
if ".dcm" in filename.lower(): # checking whether the file's DICOM
lstFilesDCM.append(os.path.join(dirName,filename)) # <-you cut one potential hierarchy level
with something like:
dicom_files =
for d, dirs, files in os.walk('./data/'):
for x in files:
if x.endswith(".png"):
dicom_path = os.path.join(d, x)
if os.path.exists(dicom_path):
dicom_files.append(dicom_path)
Without any further context, it's difficult to assert anything. However, when I look at the way you collect the potential DICOM files, you are probably constructing not existing path (it looks like you skip one hierarchy level when joining path parts back; you may want to check that the constructed path actually points to an existing files using os.path.exists()).
You may want to change the iterating part:
for dirName, subdirList, fileList in os.walk(PathDicom):
for filename in fileList:
if ".dcm" in filename.lower(): # checking whether the file's DICOM
lstFilesDCM.append(os.path.join(dirName,filename)) # <-you cut one potential hierarchy level
with something like:
dicom_files =
for d, dirs, files in os.walk('./data/'):
for x in files:
if x.endswith(".png"):
dicom_path = os.path.join(d, x)
if os.path.exists(dicom_path):
dicom_files.append(dicom_path)
edited Nov 20 at 9:27
answered Nov 20 at 8:57


leoburgy
1086
1086
I tried, but problem still exists.
– Makau
Nov 20 at 9:31
Just to let you know, I can access other attributes but not pixel_array
– Makau
Nov 20 at 9:38
Can you reproduce the error with the Matplotlib way of displaying the array? pydicom.github.io/pydicom/stable/viewing_images.html
– leoburgy
Nov 20 at 9:42
Yes, I am using exactly the same syntex. Using the test data files, it is ok. But with my data. I get the error.
– Makau
Nov 20 at 9:47
Looking at the source code of pillow_handler.py, it looks like your error is piping when checking the jpeg plugin. Did you check that the jpeg plugin is installed in your environment? Maybe useful: stackoverflow.com/a/10109941/5202571
– leoburgy
Nov 20 at 11:42
|
show 1 more comment
I tried, but problem still exists.
– Makau
Nov 20 at 9:31
Just to let you know, I can access other attributes but not pixel_array
– Makau
Nov 20 at 9:38
Can you reproduce the error with the Matplotlib way of displaying the array? pydicom.github.io/pydicom/stable/viewing_images.html
– leoburgy
Nov 20 at 9:42
Yes, I am using exactly the same syntex. Using the test data files, it is ok. But with my data. I get the error.
– Makau
Nov 20 at 9:47
Looking at the source code of pillow_handler.py, it looks like your error is piping when checking the jpeg plugin. Did you check that the jpeg plugin is installed in your environment? Maybe useful: stackoverflow.com/a/10109941/5202571
– leoburgy
Nov 20 at 11:42
I tried, but problem still exists.
– Makau
Nov 20 at 9:31
I tried, but problem still exists.
– Makau
Nov 20 at 9:31
Just to let you know, I can access other attributes but not pixel_array
– Makau
Nov 20 at 9:38
Just to let you know, I can access other attributes but not pixel_array
– Makau
Nov 20 at 9:38
Can you reproduce the error with the Matplotlib way of displaying the array? pydicom.github.io/pydicom/stable/viewing_images.html
– leoburgy
Nov 20 at 9:42
Can you reproduce the error with the Matplotlib way of displaying the array? pydicom.github.io/pydicom/stable/viewing_images.html
– leoburgy
Nov 20 at 9:42
Yes, I am using exactly the same syntex. Using the test data files, it is ok. But with my data. I get the error.
– Makau
Nov 20 at 9:47
Yes, I am using exactly the same syntex. Using the test data files, it is ok. But with my data. I get the error.
– Makau
Nov 20 at 9:47
Looking at the source code of pillow_handler.py, it looks like your error is piping when checking the jpeg plugin. Did you check that the jpeg plugin is installed in your environment? Maybe useful: stackoverflow.com/a/10109941/5202571
– leoburgy
Nov 20 at 11:42
Looking at the source code of pillow_handler.py, it looks like your error is piping when checking the jpeg plugin. Did you check that the jpeg plugin is installed in your environment? Maybe useful: stackoverflow.com/a/10109941/5202571
– leoburgy
Nov 20 at 11:42
|
show 1 more comment
up vote
0
down vote
accepted
I sorted the dicom files according to the axis which was in increasing order by using ImagePositionPatient attribute. Which solved my problem. I was able to access pixel_array attribute and plot. The code is added to original question. I add it below too.
def load_scan(path):
slices = [dicom.read_file(path + '/' + s) for s in os.listdir(path)]
slices.sort(key = lambda x: float(x.ImagePositionPatient[2]))
pos1 = slices[int(len(slices)/2)].ImagePositionPatient[2]
pos2 = slices[(int(len(slices)/2)) + 1].ImagePositionPatient[2]
diff = pos2 - pos1
if diff > 0:
slices = np.flipud(slices)
try:
slice_thickness = np.abs(slices[0].ImagePositionPatient[2] - slices[1].ImagePositionPatient[2])
except:
slice_thickness = np.abs(slices[0].SliceLocation - slices[1].SliceLocation)
for s in slices:
s.SliceThickness = slice_thickness
return slices
code source:https://www.programcreek.com/python/example/97517/dicom.read_file
add a comment |
up vote
0
down vote
accepted
I sorted the dicom files according to the axis which was in increasing order by using ImagePositionPatient attribute. Which solved my problem. I was able to access pixel_array attribute and plot. The code is added to original question. I add it below too.
def load_scan(path):
slices = [dicom.read_file(path + '/' + s) for s in os.listdir(path)]
slices.sort(key = lambda x: float(x.ImagePositionPatient[2]))
pos1 = slices[int(len(slices)/2)].ImagePositionPatient[2]
pos2 = slices[(int(len(slices)/2)) + 1].ImagePositionPatient[2]
diff = pos2 - pos1
if diff > 0:
slices = np.flipud(slices)
try:
slice_thickness = np.abs(slices[0].ImagePositionPatient[2] - slices[1].ImagePositionPatient[2])
except:
slice_thickness = np.abs(slices[0].SliceLocation - slices[1].SliceLocation)
for s in slices:
s.SliceThickness = slice_thickness
return slices
code source:https://www.programcreek.com/python/example/97517/dicom.read_file
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
I sorted the dicom files according to the axis which was in increasing order by using ImagePositionPatient attribute. Which solved my problem. I was able to access pixel_array attribute and plot. The code is added to original question. I add it below too.
def load_scan(path):
slices = [dicom.read_file(path + '/' + s) for s in os.listdir(path)]
slices.sort(key = lambda x: float(x.ImagePositionPatient[2]))
pos1 = slices[int(len(slices)/2)].ImagePositionPatient[2]
pos2 = slices[(int(len(slices)/2)) + 1].ImagePositionPatient[2]
diff = pos2 - pos1
if diff > 0:
slices = np.flipud(slices)
try:
slice_thickness = np.abs(slices[0].ImagePositionPatient[2] - slices[1].ImagePositionPatient[2])
except:
slice_thickness = np.abs(slices[0].SliceLocation - slices[1].SliceLocation)
for s in slices:
s.SliceThickness = slice_thickness
return slices
code source:https://www.programcreek.com/python/example/97517/dicom.read_file
I sorted the dicom files according to the axis which was in increasing order by using ImagePositionPatient attribute. Which solved my problem. I was able to access pixel_array attribute and plot. The code is added to original question. I add it below too.
def load_scan(path):
slices = [dicom.read_file(path + '/' + s) for s in os.listdir(path)]
slices.sort(key = lambda x: float(x.ImagePositionPatient[2]))
pos1 = slices[int(len(slices)/2)].ImagePositionPatient[2]
pos2 = slices[(int(len(slices)/2)) + 1].ImagePositionPatient[2]
diff = pos2 - pos1
if diff > 0:
slices = np.flipud(slices)
try:
slice_thickness = np.abs(slices[0].ImagePositionPatient[2] - slices[1].ImagePositionPatient[2])
except:
slice_thickness = np.abs(slices[0].SliceLocation - slices[1].SliceLocation)
for s in slices:
s.SliceThickness = slice_thickness
return slices
code source:https://www.programcreek.com/python/example/97517/dicom.read_file
answered Nov 30 at 12:27


Makau
215
215
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53389288%2fcan-not-access-pixel-array-attribute-pydicom%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MesXShgIrYAwWYx gl NYTyFz2