Object type specification not defined in body Oracle SQL
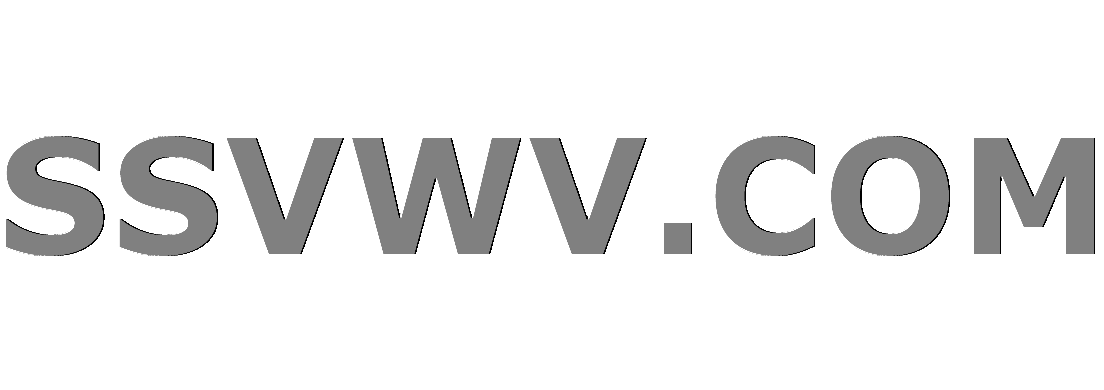
Multi tool use
I've got this problem with a PL/SQL
object I'm trying to develop. The error message is this:
PLS-00538: subprogram or cursor 'BASE_T' is declared in an
object type specification and must be defined in the object type
body
And my object is this:
-- Create the first object
CREATE OR REPLACE TYPE base_t IS OBJECT (
oname VARCHAR2 (30),
name VARCHAR2 (30),
CONSTRUCTOR FUNCTION base_t RETURN SELF AS RESULT,
CONSTRUCTOR FUNCTION base_t (oname VARCHAR2, name VARCHAR2) RETURN SELF AS RESULT,
MEMBER FUNCTION get_oname RETURN VARCHAR2,
MEMBER FUNCTION get_name RETURN VARCHAR2,
MEMBER PROCEDURE set_oname (oname VARCHAR2),
MEMBER PROCEDURE to_string
) INSTANTIABLE NOT FINAL;
/
-- Body of the object
CREATE OR REPLACE TYPE BODY base_t AS
CONSTRUCTOR FUNCTION base_t (oname VARCHAR2 , name VARCHAR2 ) RETURN SELF AS RESULT IS BEGIN
SELF.oname := oname;
SELF.name := name;
END;
MEMBER PROCEDURE set_oname (oname VARCHAR2 ) IS BEGIN
SELF.oname := oname;
END set_oname;
MEMBER FUNCTION get_oname RETURN VARCHAR2 IS BEGIN
RETURN SELF.oname;
END get_oname;
MEMBER FUNCTION get_name RETURN VARCHAR2 IS BEGIN
RETURN SELF.name;
END get_name;
MEMBER PROCEDURE to_string IS BEGIN
dbms_output.put_line('Hello ['||self.oname||'].');
END to_string;
END;
/
The problem is, usually this error message is called by inconsistent function/procedure definitions/names (as shown in this SO question here: Oracle Error PLS-00323: subprogram or cursor is declared in a package specification and must be defined in the package body), but I've checked through and I don't believe any of my names are messed up and all of my definitions are consistent. How else can you get that error message?
sql oracle plsql user-defined-types
add a comment |
I've got this problem with a PL/SQL
object I'm trying to develop. The error message is this:
PLS-00538: subprogram or cursor 'BASE_T' is declared in an
object type specification and must be defined in the object type
body
And my object is this:
-- Create the first object
CREATE OR REPLACE TYPE base_t IS OBJECT (
oname VARCHAR2 (30),
name VARCHAR2 (30),
CONSTRUCTOR FUNCTION base_t RETURN SELF AS RESULT,
CONSTRUCTOR FUNCTION base_t (oname VARCHAR2, name VARCHAR2) RETURN SELF AS RESULT,
MEMBER FUNCTION get_oname RETURN VARCHAR2,
MEMBER FUNCTION get_name RETURN VARCHAR2,
MEMBER PROCEDURE set_oname (oname VARCHAR2),
MEMBER PROCEDURE to_string
) INSTANTIABLE NOT FINAL;
/
-- Body of the object
CREATE OR REPLACE TYPE BODY base_t AS
CONSTRUCTOR FUNCTION base_t (oname VARCHAR2 , name VARCHAR2 ) RETURN SELF AS RESULT IS BEGIN
SELF.oname := oname;
SELF.name := name;
END;
MEMBER PROCEDURE set_oname (oname VARCHAR2 ) IS BEGIN
SELF.oname := oname;
END set_oname;
MEMBER FUNCTION get_oname RETURN VARCHAR2 IS BEGIN
RETURN SELF.oname;
END get_oname;
MEMBER FUNCTION get_name RETURN VARCHAR2 IS BEGIN
RETURN SELF.name;
END get_name;
MEMBER PROCEDURE to_string IS BEGIN
dbms_output.put_line('Hello ['||self.oname||'].');
END to_string;
END;
/
The problem is, usually this error message is called by inconsistent function/procedure definitions/names (as shown in this SO question here: Oracle Error PLS-00323: subprogram or cursor is declared in a package specification and must be defined in the package body), but I've checked through and I don't believe any of my names are messed up and all of my definitions are consistent. How else can you get that error message?
sql oracle plsql user-defined-types
3
You haven't provided an implementation forCONSTRUCTOR FUNCTION base_t RETURN SELF AS RESULT
.
– Bob Jarvis
Nov 24 '18 at 4:44
add a comment |
I've got this problem with a PL/SQL
object I'm trying to develop. The error message is this:
PLS-00538: subprogram or cursor 'BASE_T' is declared in an
object type specification and must be defined in the object type
body
And my object is this:
-- Create the first object
CREATE OR REPLACE TYPE base_t IS OBJECT (
oname VARCHAR2 (30),
name VARCHAR2 (30),
CONSTRUCTOR FUNCTION base_t RETURN SELF AS RESULT,
CONSTRUCTOR FUNCTION base_t (oname VARCHAR2, name VARCHAR2) RETURN SELF AS RESULT,
MEMBER FUNCTION get_oname RETURN VARCHAR2,
MEMBER FUNCTION get_name RETURN VARCHAR2,
MEMBER PROCEDURE set_oname (oname VARCHAR2),
MEMBER PROCEDURE to_string
) INSTANTIABLE NOT FINAL;
/
-- Body of the object
CREATE OR REPLACE TYPE BODY base_t AS
CONSTRUCTOR FUNCTION base_t (oname VARCHAR2 , name VARCHAR2 ) RETURN SELF AS RESULT IS BEGIN
SELF.oname := oname;
SELF.name := name;
END;
MEMBER PROCEDURE set_oname (oname VARCHAR2 ) IS BEGIN
SELF.oname := oname;
END set_oname;
MEMBER FUNCTION get_oname RETURN VARCHAR2 IS BEGIN
RETURN SELF.oname;
END get_oname;
MEMBER FUNCTION get_name RETURN VARCHAR2 IS BEGIN
RETURN SELF.name;
END get_name;
MEMBER PROCEDURE to_string IS BEGIN
dbms_output.put_line('Hello ['||self.oname||'].');
END to_string;
END;
/
The problem is, usually this error message is called by inconsistent function/procedure definitions/names (as shown in this SO question here: Oracle Error PLS-00323: subprogram or cursor is declared in a package specification and must be defined in the package body), but I've checked through and I don't believe any of my names are messed up and all of my definitions are consistent. How else can you get that error message?
sql oracle plsql user-defined-types
I've got this problem with a PL/SQL
object I'm trying to develop. The error message is this:
PLS-00538: subprogram or cursor 'BASE_T' is declared in an
object type specification and must be defined in the object type
body
And my object is this:
-- Create the first object
CREATE OR REPLACE TYPE base_t IS OBJECT (
oname VARCHAR2 (30),
name VARCHAR2 (30),
CONSTRUCTOR FUNCTION base_t RETURN SELF AS RESULT,
CONSTRUCTOR FUNCTION base_t (oname VARCHAR2, name VARCHAR2) RETURN SELF AS RESULT,
MEMBER FUNCTION get_oname RETURN VARCHAR2,
MEMBER FUNCTION get_name RETURN VARCHAR2,
MEMBER PROCEDURE set_oname (oname VARCHAR2),
MEMBER PROCEDURE to_string
) INSTANTIABLE NOT FINAL;
/
-- Body of the object
CREATE OR REPLACE TYPE BODY base_t AS
CONSTRUCTOR FUNCTION base_t (oname VARCHAR2 , name VARCHAR2 ) RETURN SELF AS RESULT IS BEGIN
SELF.oname := oname;
SELF.name := name;
END;
MEMBER PROCEDURE set_oname (oname VARCHAR2 ) IS BEGIN
SELF.oname := oname;
END set_oname;
MEMBER FUNCTION get_oname RETURN VARCHAR2 IS BEGIN
RETURN SELF.oname;
END get_oname;
MEMBER FUNCTION get_name RETURN VARCHAR2 IS BEGIN
RETURN SELF.name;
END get_name;
MEMBER PROCEDURE to_string IS BEGIN
dbms_output.put_line('Hello ['||self.oname||'].');
END to_string;
END;
/
The problem is, usually this error message is called by inconsistent function/procedure definitions/names (as shown in this SO question here: Oracle Error PLS-00323: subprogram or cursor is declared in a package specification and must be defined in the package body), but I've checked through and I don't believe any of my names are messed up and all of my definitions are consistent. How else can you get that error message?
sql oracle plsql user-defined-types
sql oracle plsql user-defined-types
edited Nov 24 '18 at 7:15
APC
119k15118229
119k15118229
asked Nov 23 '18 at 23:53
Adam McGurkAdam McGurk
388319
388319
3
You haven't provided an implementation forCONSTRUCTOR FUNCTION base_t RETURN SELF AS RESULT
.
– Bob Jarvis
Nov 24 '18 at 4:44
add a comment |
3
You haven't provided an implementation forCONSTRUCTOR FUNCTION base_t RETURN SELF AS RESULT
.
– Bob Jarvis
Nov 24 '18 at 4:44
3
3
You haven't provided an implementation for
CONSTRUCTOR FUNCTION base_t RETURN SELF AS RESULT
.– Bob Jarvis
Nov 24 '18 at 4:44
You haven't provided an implementation for
CONSTRUCTOR FUNCTION base_t RETURN SELF AS RESULT
.– Bob Jarvis
Nov 24 '18 at 4:44
add a comment |
1 Answer
1
active
oldest
votes
Add some code for the default ctor into the TYPE BODY eg
SQL> CREATE OR REPLACE TYPE BODY base_t AS
2
3 constructor function base_t return self as result
4 is
5 begin
6 self.oname := null ;
7 self.name := null ;
8 end ;
9
10 CONSTRUCTOR FUNCTION base_t (oname VARCHAR2 , name VARCHAR2 ) RETURN SELF AS RESULT IS BEGIN
11 SELF.oname := oname;
12 SELF.name := name;
13 END;
14 MEMBER PROCEDURE set_oname (oname VARCHAR2 ) IS BEGIN
15 SELF.oname := oname;
16 END set_oname;
17 MEMBER FUNCTION get_oname RETURN VARCHAR2 IS BEGIN
18 RETURN SELF.oname;
19 END get_oname;
20 MEMBER FUNCTION get_name RETURN VARCHAR2 IS BEGIN
21 RETURN SELF.name;
22 END get_name;
23 MEMBER PROCEDURE to_string IS BEGIN
24 dbms_output.put_line('Hello ['||self.oname||'].');
25 END to_string;
26 END;
27 /
Type Body BASE_T compiled
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454000%2fobject-type-specification-not-defined-in-body-oracle-sql%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Add some code for the default ctor into the TYPE BODY eg
SQL> CREATE OR REPLACE TYPE BODY base_t AS
2
3 constructor function base_t return self as result
4 is
5 begin
6 self.oname := null ;
7 self.name := null ;
8 end ;
9
10 CONSTRUCTOR FUNCTION base_t (oname VARCHAR2 , name VARCHAR2 ) RETURN SELF AS RESULT IS BEGIN
11 SELF.oname := oname;
12 SELF.name := name;
13 END;
14 MEMBER PROCEDURE set_oname (oname VARCHAR2 ) IS BEGIN
15 SELF.oname := oname;
16 END set_oname;
17 MEMBER FUNCTION get_oname RETURN VARCHAR2 IS BEGIN
18 RETURN SELF.oname;
19 END get_oname;
20 MEMBER FUNCTION get_name RETURN VARCHAR2 IS BEGIN
21 RETURN SELF.name;
22 END get_name;
23 MEMBER PROCEDURE to_string IS BEGIN
24 dbms_output.put_line('Hello ['||self.oname||'].');
25 END to_string;
26 END;
27 /
Type Body BASE_T compiled
add a comment |
Add some code for the default ctor into the TYPE BODY eg
SQL> CREATE OR REPLACE TYPE BODY base_t AS
2
3 constructor function base_t return self as result
4 is
5 begin
6 self.oname := null ;
7 self.name := null ;
8 end ;
9
10 CONSTRUCTOR FUNCTION base_t (oname VARCHAR2 , name VARCHAR2 ) RETURN SELF AS RESULT IS BEGIN
11 SELF.oname := oname;
12 SELF.name := name;
13 END;
14 MEMBER PROCEDURE set_oname (oname VARCHAR2 ) IS BEGIN
15 SELF.oname := oname;
16 END set_oname;
17 MEMBER FUNCTION get_oname RETURN VARCHAR2 IS BEGIN
18 RETURN SELF.oname;
19 END get_oname;
20 MEMBER FUNCTION get_name RETURN VARCHAR2 IS BEGIN
21 RETURN SELF.name;
22 END get_name;
23 MEMBER PROCEDURE to_string IS BEGIN
24 dbms_output.put_line('Hello ['||self.oname||'].');
25 END to_string;
26 END;
27 /
Type Body BASE_T compiled
add a comment |
Add some code for the default ctor into the TYPE BODY eg
SQL> CREATE OR REPLACE TYPE BODY base_t AS
2
3 constructor function base_t return self as result
4 is
5 begin
6 self.oname := null ;
7 self.name := null ;
8 end ;
9
10 CONSTRUCTOR FUNCTION base_t (oname VARCHAR2 , name VARCHAR2 ) RETURN SELF AS RESULT IS BEGIN
11 SELF.oname := oname;
12 SELF.name := name;
13 END;
14 MEMBER PROCEDURE set_oname (oname VARCHAR2 ) IS BEGIN
15 SELF.oname := oname;
16 END set_oname;
17 MEMBER FUNCTION get_oname RETURN VARCHAR2 IS BEGIN
18 RETURN SELF.oname;
19 END get_oname;
20 MEMBER FUNCTION get_name RETURN VARCHAR2 IS BEGIN
21 RETURN SELF.name;
22 END get_name;
23 MEMBER PROCEDURE to_string IS BEGIN
24 dbms_output.put_line('Hello ['||self.oname||'].');
25 END to_string;
26 END;
27 /
Type Body BASE_T compiled
Add some code for the default ctor into the TYPE BODY eg
SQL> CREATE OR REPLACE TYPE BODY base_t AS
2
3 constructor function base_t return self as result
4 is
5 begin
6 self.oname := null ;
7 self.name := null ;
8 end ;
9
10 CONSTRUCTOR FUNCTION base_t (oname VARCHAR2 , name VARCHAR2 ) RETURN SELF AS RESULT IS BEGIN
11 SELF.oname := oname;
12 SELF.name := name;
13 END;
14 MEMBER PROCEDURE set_oname (oname VARCHAR2 ) IS BEGIN
15 SELF.oname := oname;
16 END set_oname;
17 MEMBER FUNCTION get_oname RETURN VARCHAR2 IS BEGIN
18 RETURN SELF.oname;
19 END get_oname;
20 MEMBER FUNCTION get_name RETURN VARCHAR2 IS BEGIN
21 RETURN SELF.name;
22 END get_name;
23 MEMBER PROCEDURE to_string IS BEGIN
24 dbms_output.put_line('Hello ['||self.oname||'].');
25 END to_string;
26 END;
27 /
Type Body BASE_T compiled
answered Nov 24 '18 at 10:04


stefanstefan
886146
886146
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454000%2fobject-type-specification-not-defined-in-body-oracle-sql%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
CEMuMSV8Kx3k4FEicS0iICYBgl,hoRET Kv ynlmopl44K 1h5CRxm
3
You haven't provided an implementation for
CONSTRUCTOR FUNCTION base_t RETURN SELF AS RESULT
.– Bob Jarvis
Nov 24 '18 at 4:44