How do I implement collision detection?
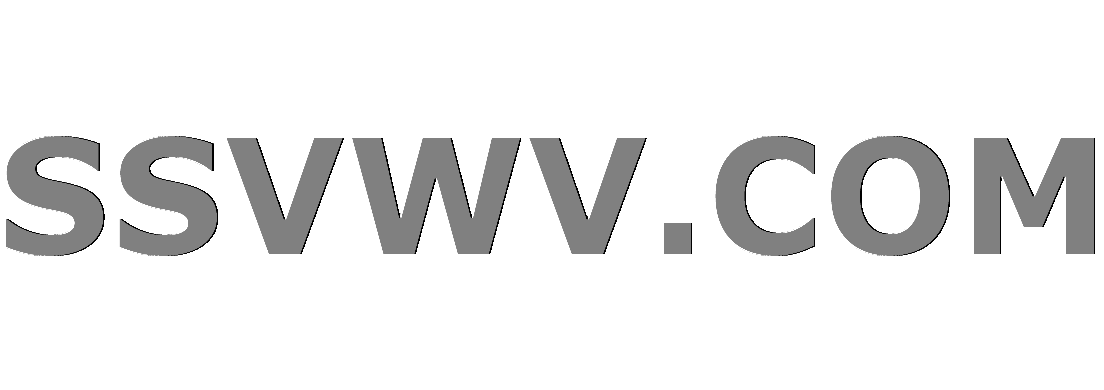
Multi tool use
from graphics import*
import time
import random
def main():
numx=random.randint(10,700)
wn=GraphWin("AK",700,700)
wn.setBackground("white")
msg=Text(Point(25,30),"Score")
msg.setSize(12)
msg.setTextColor('blue')
msg.draw(wn)
inch=Entry(Point(60,30),2)
inch.setFill('white')
inch.draw(wn)
sqrg=Rectangle(Point(330,650),Point(430,665))
sqrg.setFill("red")
sqrg.draw(wn)
blx=Circle(Point(numx,80),20)
blx.setFill("blue")
blx.draw(wn)
xval=10
yval=0
wn.getMouse()
for i in range(150):
sqrg.move(xval,yval)
symbl=wn.checkKey()
if symbl=="Right":
xval=10
yval=0
if symbl=="Left":
xval=-10
yval=0
time.sleep(0.08)
blx.move(0,20)
main()
I'm very confused my professor is very confusing, and I need to do this for a project where when collision is detected the score goes up.
python python-3.x collision-detection zelle-graphics
add a comment |
from graphics import*
import time
import random
def main():
numx=random.randint(10,700)
wn=GraphWin("AK",700,700)
wn.setBackground("white")
msg=Text(Point(25,30),"Score")
msg.setSize(12)
msg.setTextColor('blue')
msg.draw(wn)
inch=Entry(Point(60,30),2)
inch.setFill('white')
inch.draw(wn)
sqrg=Rectangle(Point(330,650),Point(430,665))
sqrg.setFill("red")
sqrg.draw(wn)
blx=Circle(Point(numx,80),20)
blx.setFill("blue")
blx.draw(wn)
xval=10
yval=0
wn.getMouse()
for i in range(150):
sqrg.move(xval,yval)
symbl=wn.checkKey()
if symbl=="Right":
xval=10
yval=0
if symbl=="Left":
xval=-10
yval=0
time.sleep(0.08)
blx.move(0,20)
main()
I'm very confused my professor is very confusing, and I need to do this for a project where when collision is detected the score goes up.
python python-3.x collision-detection zelle-graphics
Looks like if you know the location of sqrg and blx with the dimensions, you should be able to determine if those points intersect each iteration.
– bison
Nov 21 '18 at 23:00
add a comment |
from graphics import*
import time
import random
def main():
numx=random.randint(10,700)
wn=GraphWin("AK",700,700)
wn.setBackground("white")
msg=Text(Point(25,30),"Score")
msg.setSize(12)
msg.setTextColor('blue')
msg.draw(wn)
inch=Entry(Point(60,30),2)
inch.setFill('white')
inch.draw(wn)
sqrg=Rectangle(Point(330,650),Point(430,665))
sqrg.setFill("red")
sqrg.draw(wn)
blx=Circle(Point(numx,80),20)
blx.setFill("blue")
blx.draw(wn)
xval=10
yval=0
wn.getMouse()
for i in range(150):
sqrg.move(xval,yval)
symbl=wn.checkKey()
if symbl=="Right":
xval=10
yval=0
if symbl=="Left":
xval=-10
yval=0
time.sleep(0.08)
blx.move(0,20)
main()
I'm very confused my professor is very confusing, and I need to do this for a project where when collision is detected the score goes up.
python python-3.x collision-detection zelle-graphics
from graphics import*
import time
import random
def main():
numx=random.randint(10,700)
wn=GraphWin("AK",700,700)
wn.setBackground("white")
msg=Text(Point(25,30),"Score")
msg.setSize(12)
msg.setTextColor('blue')
msg.draw(wn)
inch=Entry(Point(60,30),2)
inch.setFill('white')
inch.draw(wn)
sqrg=Rectangle(Point(330,650),Point(430,665))
sqrg.setFill("red")
sqrg.draw(wn)
blx=Circle(Point(numx,80),20)
blx.setFill("blue")
blx.draw(wn)
xval=10
yval=0
wn.getMouse()
for i in range(150):
sqrg.move(xval,yval)
symbl=wn.checkKey()
if symbl=="Right":
xval=10
yval=0
if symbl=="Left":
xval=-10
yval=0
time.sleep(0.08)
blx.move(0,20)
main()
I'm very confused my professor is very confusing, and I need to do this for a project where when collision is detected the score goes up.
python python-3.x collision-detection zelle-graphics
python python-3.x collision-detection zelle-graphics
edited Nov 23 '18 at 21:11
cdlane
17.7k21144
17.7k21144
asked Nov 21 '18 at 22:54


joseph vivianojoseph viviano
122
122
Looks like if you know the location of sqrg and blx with the dimensions, you should be able to determine if those points intersect each iteration.
– bison
Nov 21 '18 at 23:00
add a comment |
Looks like if you know the location of sqrg and blx with the dimensions, you should be able to determine if those points intersect each iteration.
– bison
Nov 21 '18 at 23:00
Looks like if you know the location of sqrg and blx with the dimensions, you should be able to determine if those points intersect each iteration.
– bison
Nov 21 '18 at 23:00
Looks like if you know the location of sqrg and blx with the dimensions, you should be able to determine if those points intersect each iteration.
– bison
Nov 21 '18 at 23:00
add a comment |
2 Answers
2
active
oldest
votes
Your radius is twenty. Inside the loop, just test whether Euclidean distance between sqrg and blx is within 20.
add a comment |
Below is a stripped down example based on your code. It measures the distance between the centers of the two moving objects to determine if a collision has occurred. If you manage to get the ball to hit the square, the ball should bounce straight up:
from random import randint
from time import sleep
from graphics import *
def distance(p1, p2):
return ((p2.x - p1.x) ** 2 + (p2.y - p1.y) ** 2) ** 0.5
wn = GraphWin("AK", 700, 700)
sqrg = Rectangle(Point(325, 625), Point(375, 675))
sqrg.setFill("red")
sqrg.draw(wn)
numx = randint(10, 700)
blx = Circle(Point(numx, 80), 20)
blx.setFill("blue")
blx.draw(wn)
xval, yval = 10, 0
bheading = 1
wn.getMouse()
for i in range(150):
sqrg.move(xval, yval)
if distance(blx.getCenter(), sqrg.getCenter()) < 25:
bheading *= -1
symbl = wn.checkKey()
if symbl == "Right":
xval = 10
elif symbl == "Left":
xval = -10
sleep(0.1)
blx.move(0, bheading * 20)
Cleary not a viable game as-is, but a demonstration of collision detection.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53421574%2fhow-do-i-implement-collision-detection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your radius is twenty. Inside the loop, just test whether Euclidean distance between sqrg and blx is within 20.
add a comment |
Your radius is twenty. Inside the loop, just test whether Euclidean distance between sqrg and blx is within 20.
add a comment |
Your radius is twenty. Inside the loop, just test whether Euclidean distance between sqrg and blx is within 20.
Your radius is twenty. Inside the loop, just test whether Euclidean distance between sqrg and blx is within 20.
answered Nov 22 '18 at 1:45


J_HJ_H
3,3051617
3,3051617
add a comment |
add a comment |
Below is a stripped down example based on your code. It measures the distance between the centers of the two moving objects to determine if a collision has occurred. If you manage to get the ball to hit the square, the ball should bounce straight up:
from random import randint
from time import sleep
from graphics import *
def distance(p1, p2):
return ((p2.x - p1.x) ** 2 + (p2.y - p1.y) ** 2) ** 0.5
wn = GraphWin("AK", 700, 700)
sqrg = Rectangle(Point(325, 625), Point(375, 675))
sqrg.setFill("red")
sqrg.draw(wn)
numx = randint(10, 700)
blx = Circle(Point(numx, 80), 20)
blx.setFill("blue")
blx.draw(wn)
xval, yval = 10, 0
bheading = 1
wn.getMouse()
for i in range(150):
sqrg.move(xval, yval)
if distance(blx.getCenter(), sqrg.getCenter()) < 25:
bheading *= -1
symbl = wn.checkKey()
if symbl == "Right":
xval = 10
elif symbl == "Left":
xval = -10
sleep(0.1)
blx.move(0, bheading * 20)
Cleary not a viable game as-is, but a demonstration of collision detection.
add a comment |
Below is a stripped down example based on your code. It measures the distance between the centers of the two moving objects to determine if a collision has occurred. If you manage to get the ball to hit the square, the ball should bounce straight up:
from random import randint
from time import sleep
from graphics import *
def distance(p1, p2):
return ((p2.x - p1.x) ** 2 + (p2.y - p1.y) ** 2) ** 0.5
wn = GraphWin("AK", 700, 700)
sqrg = Rectangle(Point(325, 625), Point(375, 675))
sqrg.setFill("red")
sqrg.draw(wn)
numx = randint(10, 700)
blx = Circle(Point(numx, 80), 20)
blx.setFill("blue")
blx.draw(wn)
xval, yval = 10, 0
bheading = 1
wn.getMouse()
for i in range(150):
sqrg.move(xval, yval)
if distance(blx.getCenter(), sqrg.getCenter()) < 25:
bheading *= -1
symbl = wn.checkKey()
if symbl == "Right":
xval = 10
elif symbl == "Left":
xval = -10
sleep(0.1)
blx.move(0, bheading * 20)
Cleary not a viable game as-is, but a demonstration of collision detection.
add a comment |
Below is a stripped down example based on your code. It measures the distance between the centers of the two moving objects to determine if a collision has occurred. If you manage to get the ball to hit the square, the ball should bounce straight up:
from random import randint
from time import sleep
from graphics import *
def distance(p1, p2):
return ((p2.x - p1.x) ** 2 + (p2.y - p1.y) ** 2) ** 0.5
wn = GraphWin("AK", 700, 700)
sqrg = Rectangle(Point(325, 625), Point(375, 675))
sqrg.setFill("red")
sqrg.draw(wn)
numx = randint(10, 700)
blx = Circle(Point(numx, 80), 20)
blx.setFill("blue")
blx.draw(wn)
xval, yval = 10, 0
bheading = 1
wn.getMouse()
for i in range(150):
sqrg.move(xval, yval)
if distance(blx.getCenter(), sqrg.getCenter()) < 25:
bheading *= -1
symbl = wn.checkKey()
if symbl == "Right":
xval = 10
elif symbl == "Left":
xval = -10
sleep(0.1)
blx.move(0, bheading * 20)
Cleary not a viable game as-is, but a demonstration of collision detection.
Below is a stripped down example based on your code. It measures the distance between the centers of the two moving objects to determine if a collision has occurred. If you manage to get the ball to hit the square, the ball should bounce straight up:
from random import randint
from time import sleep
from graphics import *
def distance(p1, p2):
return ((p2.x - p1.x) ** 2 + (p2.y - p1.y) ** 2) ** 0.5
wn = GraphWin("AK", 700, 700)
sqrg = Rectangle(Point(325, 625), Point(375, 675))
sqrg.setFill("red")
sqrg.draw(wn)
numx = randint(10, 700)
blx = Circle(Point(numx, 80), 20)
blx.setFill("blue")
blx.draw(wn)
xval, yval = 10, 0
bheading = 1
wn.getMouse()
for i in range(150):
sqrg.move(xval, yval)
if distance(blx.getCenter(), sqrg.getCenter()) < 25:
bheading *= -1
symbl = wn.checkKey()
if symbl == "Right":
xval = 10
elif symbl == "Left":
xval = -10
sleep(0.1)
blx.move(0, bheading * 20)
Cleary not a viable game as-is, but a demonstration of collision detection.
answered Nov 23 '18 at 21:10
cdlanecdlane
17.7k21144
17.7k21144
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53421574%2fhow-do-i-implement-collision-detection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
58 iaBh9XKh,K n wimVI5 8ONhtjXj nLM9K6uKv8toDUU8Ws9
Looks like if you know the location of sqrg and blx with the dimensions, you should be able to determine if those points intersect each iteration.
– bison
Nov 21 '18 at 23:00